Git SCM (Source Control Management) is a distributed version control system that enables developers to track changes in their codebase effectively.
Here’s a simple example of cloning a repository using Git:
git clone https://github.com/username/repository.git
Introduction to Git SCM
What is Git?
Git is a powerful open-source version control system that enables developers to track changes in their codebase over time. Unlike traditional version control tools, Git allows for distributed development, making it easier for multiple contributors to work concurrently. With features such as branching, merging, and history tracking, Git enhances collaboration while minimizing conflicts.
Understanding SCM (Source Control Management)
Source Control Management (SCM) is a system that manages changes to source code over time. It acts as a repository for storing code, tracking revisions, and supporting collaborations among developers. Git is a leading SCM tool that offers an efficient way to manage code, providing features that streamline the development process, support teamwork, and ensure the integrity of the project.
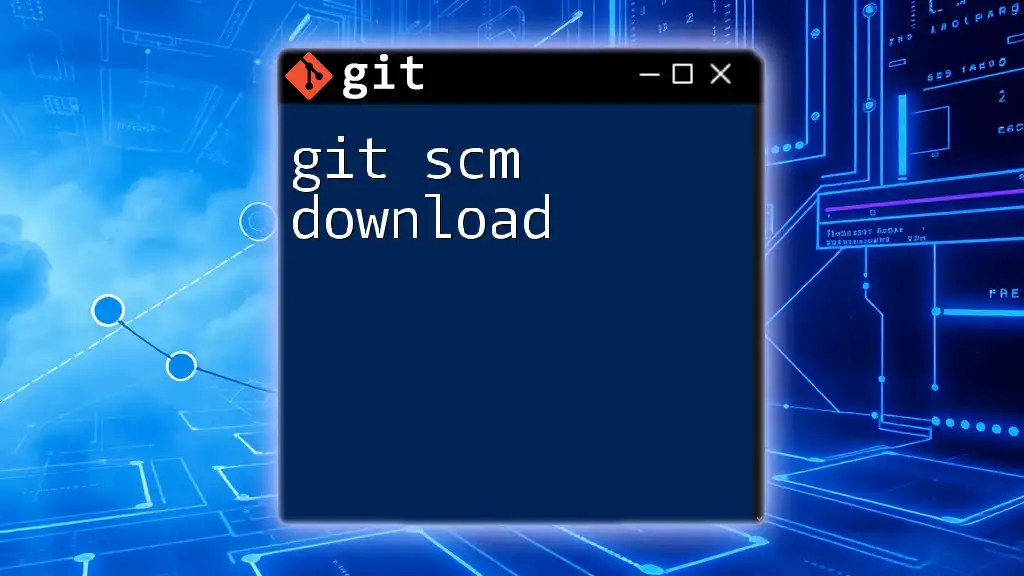
Setting Up Git
Installation of Git
To get started with Git, you first need to install it on your machine.
For Windows: Download the installer from the official Git website. The installation process is straightforward—just follow the prompts until installation is complete.
For macOS: Use Homebrew by running the following command in your terminal:
brew install git
For Linux: Depending on your distribution, use one of the following commands. For Debian/Ubuntu, you can execute:
sudo apt-get install git
For Fedora:
sudo dnf install git
Initial Configuration
After installation, it is crucial to perform some initial configuration to personalize your Git experience. Start by setting your user information with these commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
These details will be associated with your commits, so make sure they are accurate. You can check your configuration at any time with:
git config --list
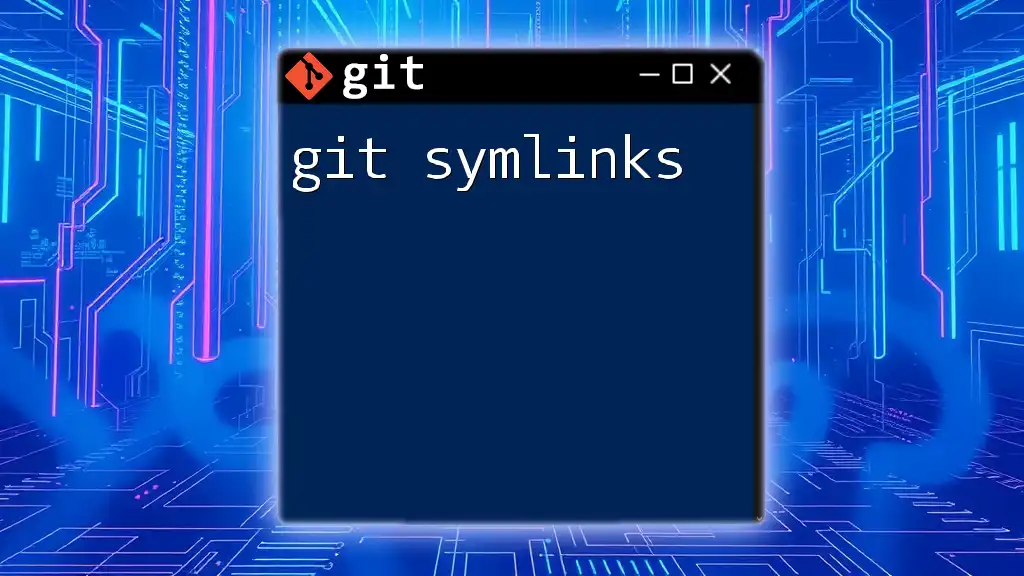
Basic Git Commands
Creating a Repository
Creating a Git repository is your first step in using Git for version control. You can either create a new local repository or clone an existing one.
To create a new repository, navigate to your project folder and run:
git init
This command initializes a new Git repository in that directory.
If you want to start working on an existing project, use the clone command not just to download, but also to set up a connection to the repository:
git clone https://github.com/user/repo.git
Staging Changes
Staging allows you to select which of your changes will be included in the next commit. It’s a careful way of preparing changes incrementally. Use the following command to add specific files:
git add filename.txt
If you want to stage all modified files in the directory, run:
git add .
Committing Changes
After staging your changes, the next step is committing them. A commit captures a snapshot of your currently staged changes. Use the command below, including a descriptive commit message:
git commit -m "Your commit message"
Keeping your commit messages clear and descriptive is essential for understanding the history of your project.
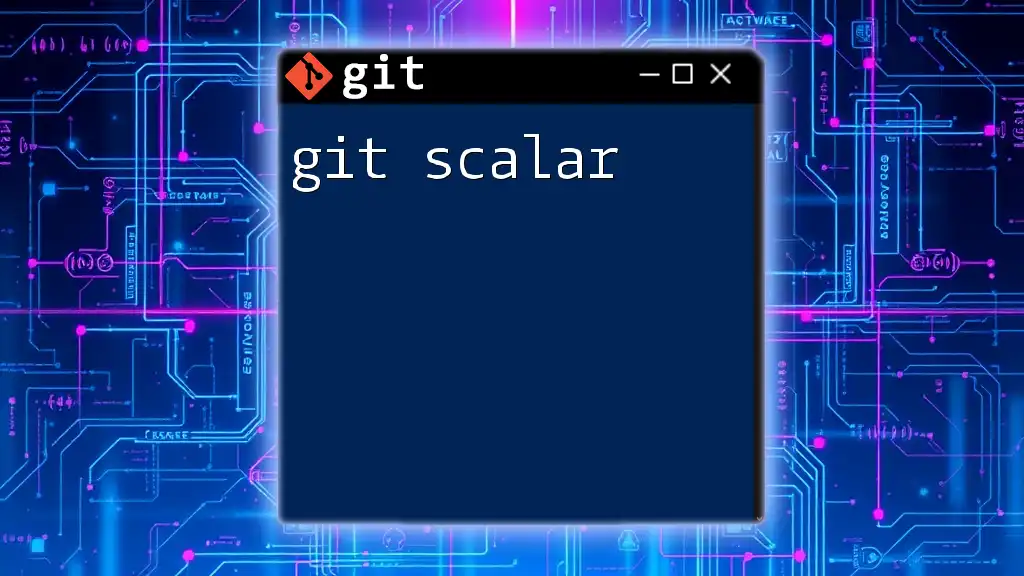
Branching in Git
Understanding Branches
Branches are an essential feature in Git, allowing you to diverge from the main line of development and continue to work independently. This enables you to experiment and develop features without affecting the main codebase.
Creating and Switching Branches
To create a new branch, use:
git branch new-branch
To switch from the current branch to the newly created one, run:
git checkout new-branch
This allows you to work on separate features without interfering with the main project.
Merging Branches
After completing work on a branch, you may want to merge it back into the main branch. Ensure you switch to the main branch before merging:
git checkout main
git merge new-branch
Sometimes, you might encounter merge conflicts if changes were made in both branches. Git will highlight these conflicts, allowing you to manually resolve them during the merge process.
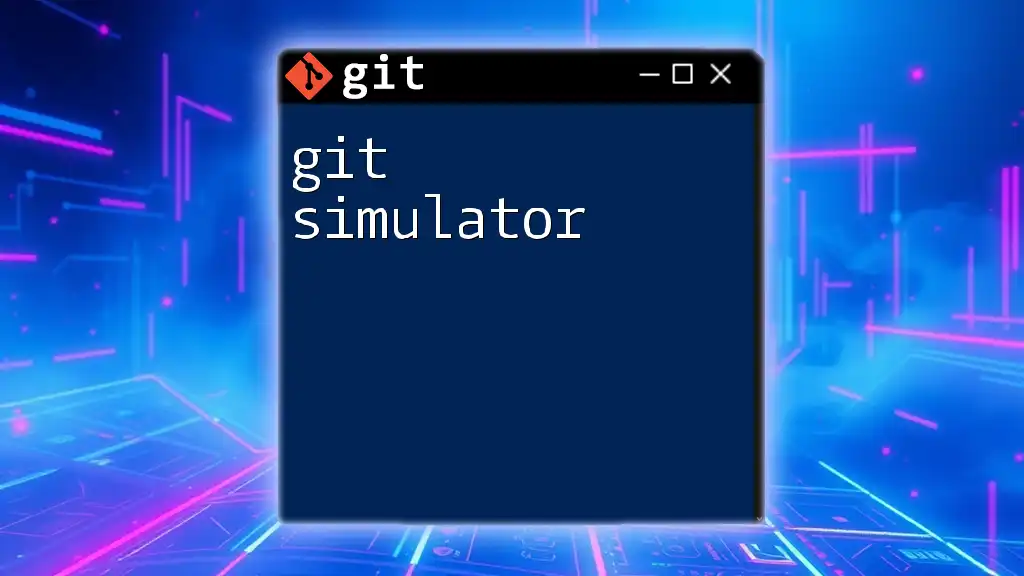
Remote Repositories
Working with Remote Repositories
Remote repositories are essential for collaborative work and backup. To connect a local repository to a remote one, use:
git remote add origin https://github.com/user/repo.git
You can verify the connection by executing:
git remote -v
Pushing and Pulling Changes
When you want to upload your local changes to a remote repository, you need to push them:
git push origin main
Conversely, to fetch and integrate changes from the remote repository into your local master branch, use:
git pull origin main
Understanding the differences between these two commands is crucial for smooth collaboration.
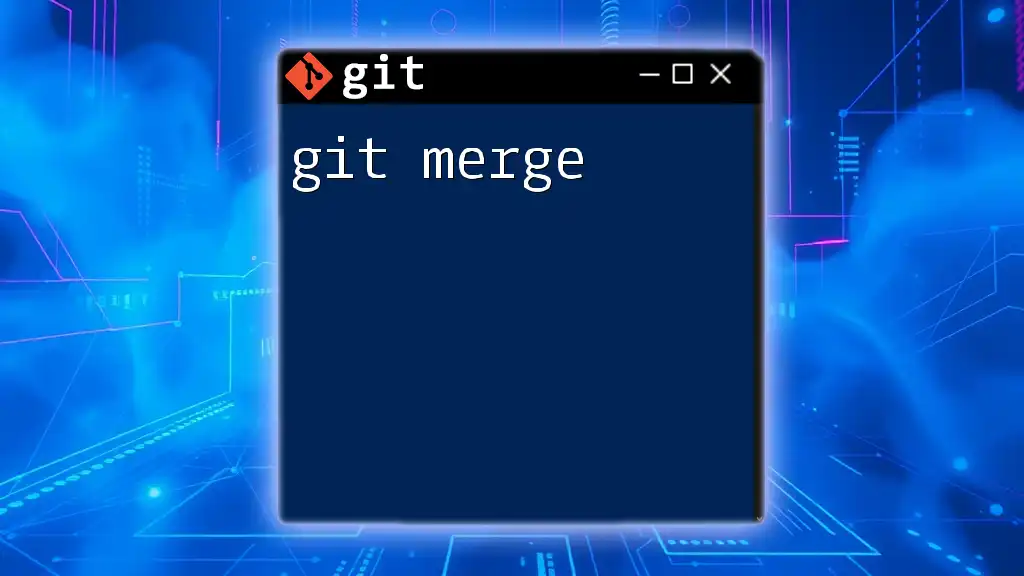
Advanced Git Commands
Undoing Changes
Mistakes happen frequently in development; Git provides mechanisms to rectify them. If you want to remove uncommitted changes from a file, you can run:
git checkout -- filename.txt
To undo your last commit but keep your changes in the staging area, use:
git reset HEAD~1
This way, you can adjust your changes and recommit them if necessary.
Viewing History
Git makes it easy to look back at your project's history. Use this command to view commits:
git log
To make the output more manageable, you can use options such as:
git log --oneline --graph
This shows a condensed view of your commit history in a graphical format.
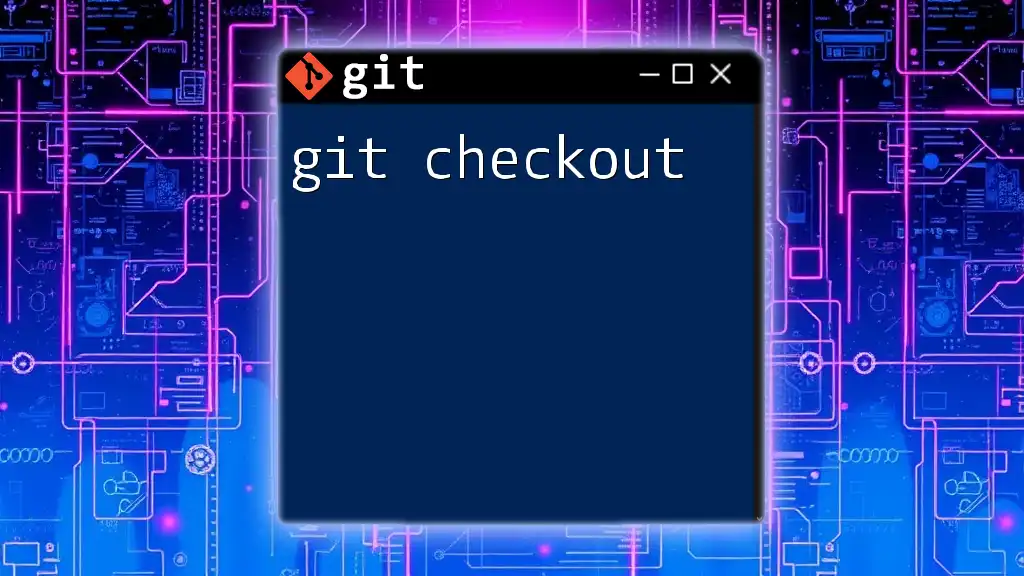
Best Practices in Using Git
Git Workflow Models
Establishing a clear workflow model is essential for maintaining consistency across your project. Common models include the Feature Branch Workflow, where each new feature is developed in its own branch, and Git Flow, which provides a structured approach to version control.
Commit Regularly and Meaningfully
Frequent commits with meaningful messages ensure that your project’s history is clear and manageable. Each commit message should convey the purpose of the changes made.
Collaborate and Communicate
Using pull requests when contributing to shared repositories allows for code reviews and discussions around the changes. This practice fosters better collaboration among developers.
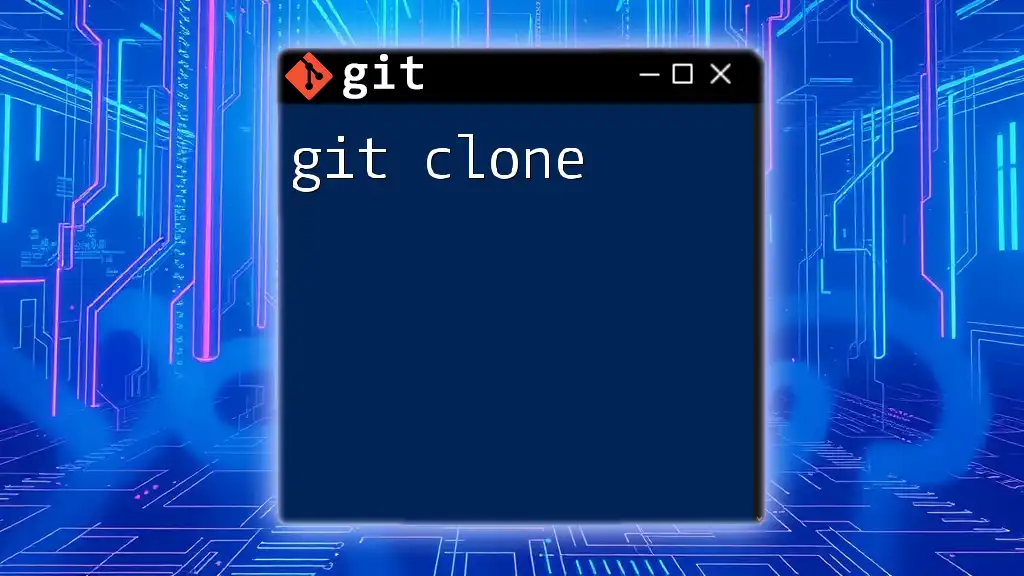
Conclusion
In summary, understanding Git SCM is invaluable for any developer looking to manage code effectively. With its powerful features, Git enhances collaboration, aids in tracking changes, and ensures project integrity.
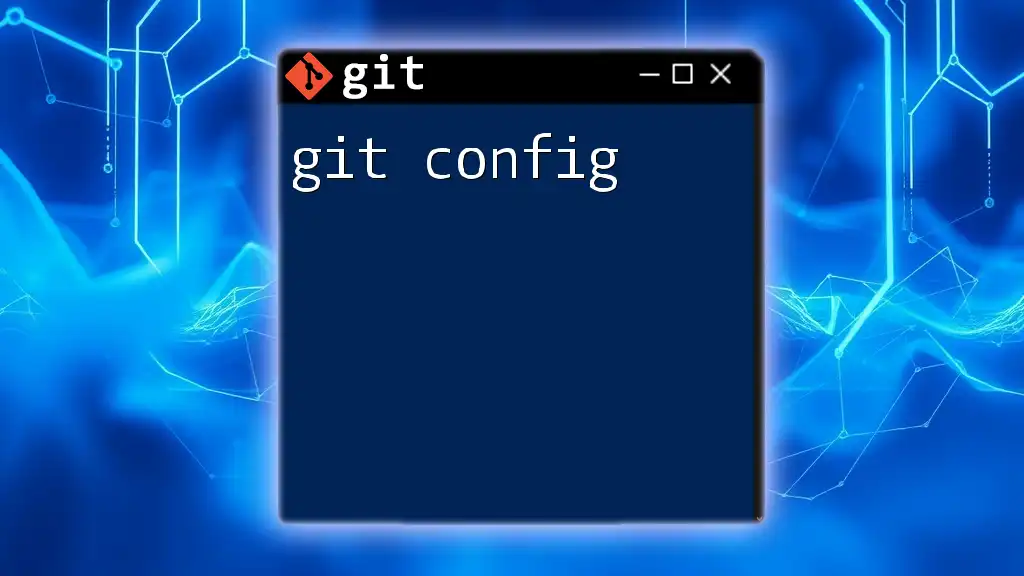
Additional Resources
For further learning and exploration, consider delving into the official Git documentation, following video tutorials, or engaging with GitHub’s guides and community forums. Continuous learning will enhance your expertise and adapt your skills to the evolving landscape of software development.