To untrack a file in Git without deleting it from your working directory, use the following command to update the index while keeping the file on disk:
git rm --cached <file_to_untrack>
Replace `<file_to_untrack>` with the name of the file you want to untrack.
Understanding Git Tracking
What is a Tracked File?
Tracked files in Git are files that have been added to the version control system. This means that any changes made to these files will be monitored by Git, allowing you to commit modifications, revert to previous versions, and collaborate with others effectively. When a file is tracked, it is included in the staging area and will be committed when you run the `git commit` command.
What is an Untracked File?
Untracked files, on the other hand, are files that Git is not monitoring. They have not been staged or committed, meaning that any changes made to them are not part of the version history. Untracked files are typically new files created in your working directory that have not yet been added to Git’s oversight.
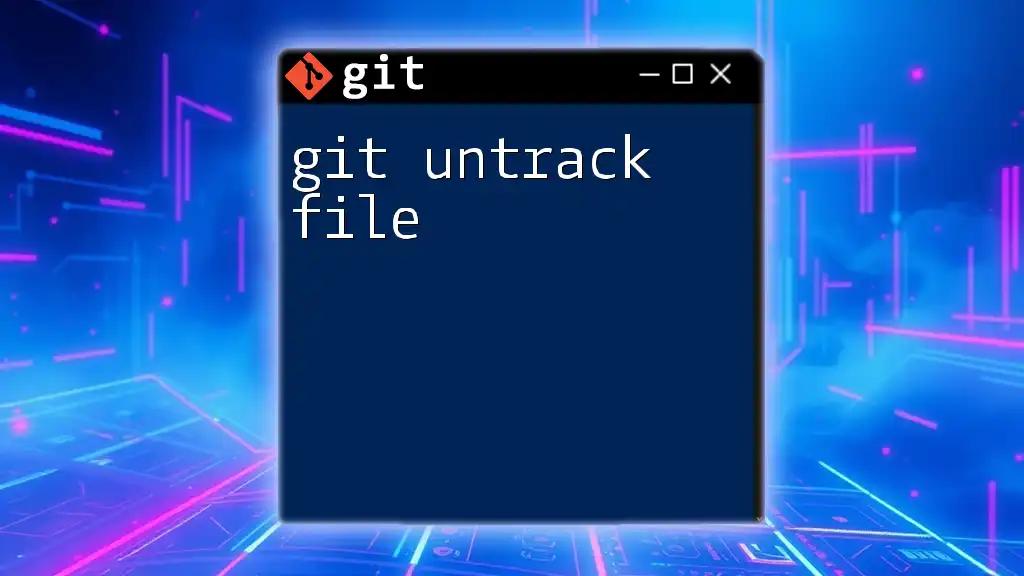
When to Untrack a File
Common Scenarios
There are various scenarios where you may want to untrack a file without deleting it. For instance:
- Configuration Files: Local configuration files (like `.env` files) that are necessary for your application but should not be part of the repository for security or localization reasons.
- Build Artifacts: Files generated during the build process (such as logs or compiled code) often do not need to be tracked, as they can be recreated from the source code.
- Temporary Files: Files like those created by your IDE or text editor (e.g., swap files or temporary backups) should be excluded from version control to keep your repository clean.
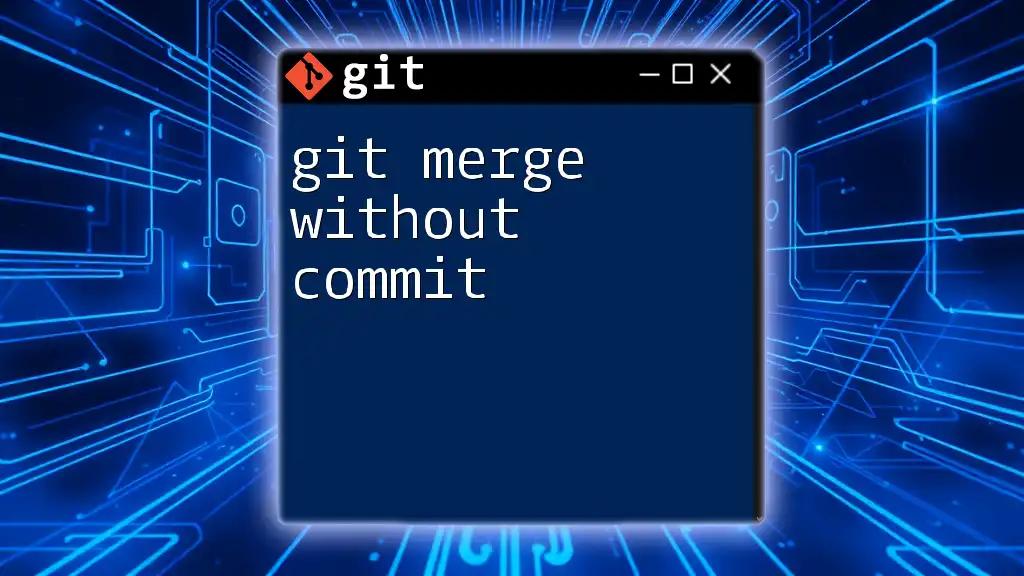
Steps to Untrack a File without Deleting it
Using `.gitignore`
What is a `.gitignore` File?
A `.gitignore` file is a plain text file where you can specify which files or directories Git should ignore. By defining patterns in this file, you can optimize your repository by preventing unneeded files from being tracked.
Steps to Untrack a File
-
Edit the `.gitignore` File To untrack a file, you first need to add it to your `.gitignore` file. Open your `.gitignore` file in your text editor and add a new line for each file you want to untrack. For example, to ignore a file called `config.txt`, you would add:
# .gitignore path/to/config.txt
-
Use the `git rm --cached` Command After updating the `.gitignore` file, you need to instruct Git to untrack the already tracked file without deleting it from your filesystem. This can be done using the `git rm --cached` command. The syntax is simple:
git rm --cached <file>
Example: To untrack the `config.txt` file located in the `path/to/` directory, you would run:
git rm --cached path/to/config.txt
By executing this command, Git will remove the file from its tracking system while leaving it intact in your project directory.
Verifying Untracking
To verify that the file has been successfully untracked, you can use the `git status` command. Run the command in your terminal:
git status
In the output, you should no longer see your file listed as “modified” or “new file” under the tracked files section. Instead, it should now appear in the untracked files section (if it was newly created) or simply not appear at all if it was previously committed without any untracked changes.
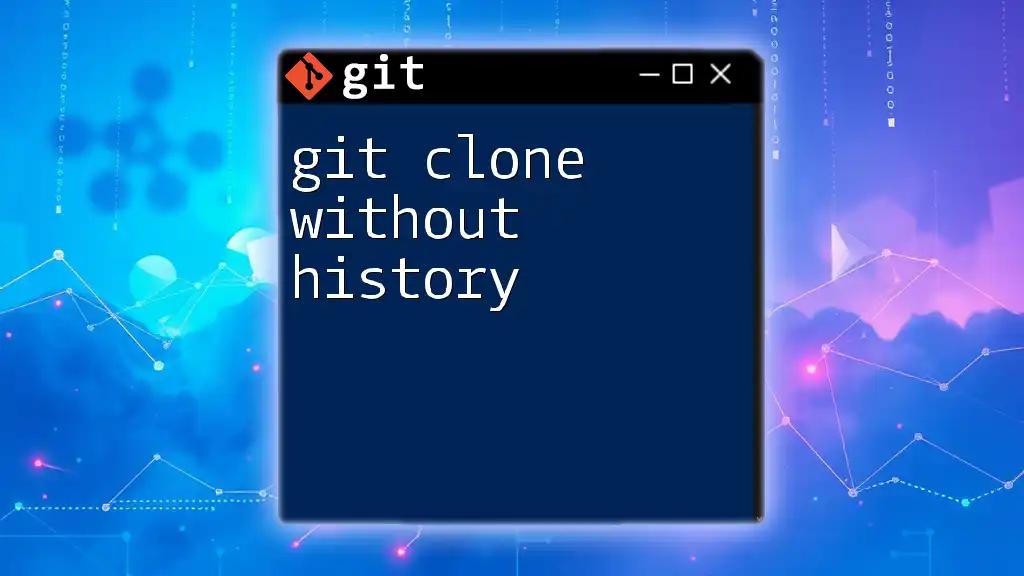
Additional Considerations
Reverting the Change
If you change your mind about untracking a file and decide that you want to track it again, you can easily do so. All you need to do is stage it back into Git’s tracking using the `git add` command:
git add path/to/config.txt
Be aware that this command will also include the file in your next commit, which could reintroduce sensitive or unnecessary information.
Best Practices for Using `.gitignore`
To maintain a clean project structure, consider the following best practices for using the `.gitignore` file:
- Organize Entries: Keep entries organized by category, such as temporary files, build artifacts, and configuration files, to enhance readability.
- Use Global Git Ignore: For files you’d like to ignore across all your repositories (like OS-specific files), consider setting up a global `.gitignore` file.
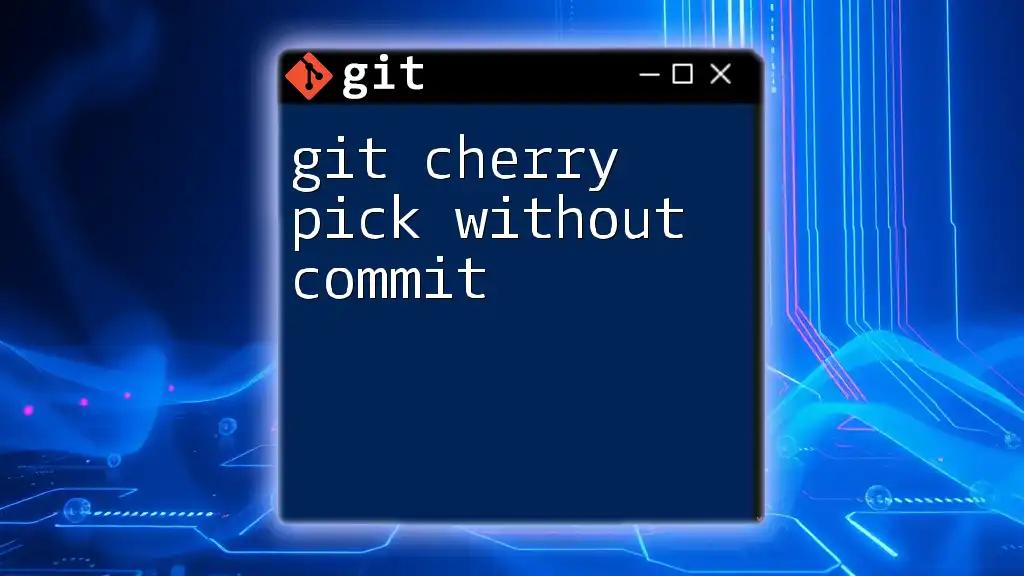
Conclusion
Untracking files without deleting them is a crucial skill when using Git, particularly when managing repositories with sensitive configuration files or transient build artifacts. By utilizing the power of the `.gitignore` file and the `git rm --cached` command, you can streamline your version control workflow while keeping your repository clean and efficient. Remember to practice these commands to gain confidence in managing file tracking effectively.
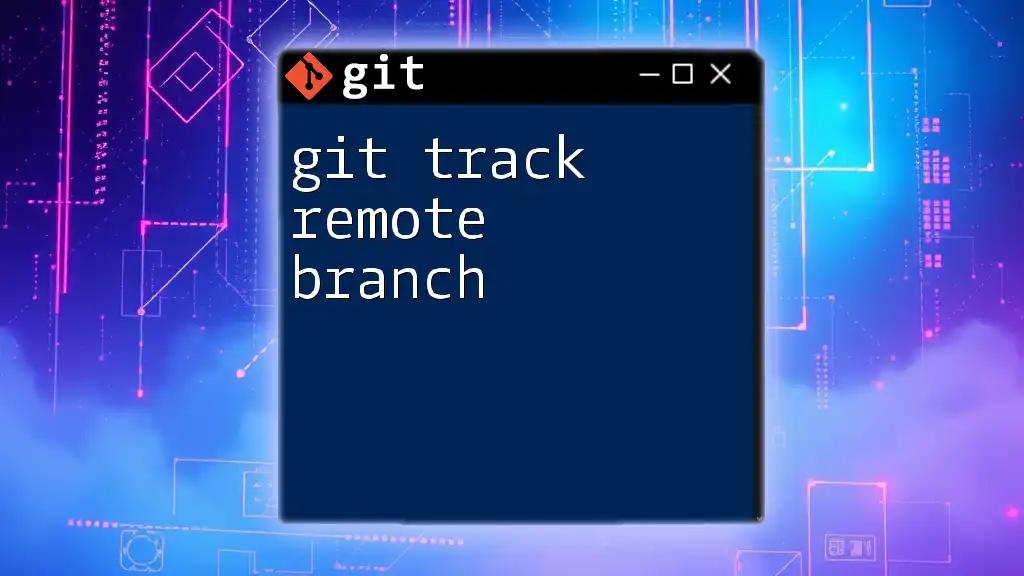
Resources and Further Reading
- Refer to the [official Git documentation](https://git-scm.com/doc) for a deeper understanding of file tracking and the various Git commands.
- Explore recommended tutorials for advanced Git usage to enhance your skills further in using Git effectively.