You can use `git cherry-pick` without committing by applying the changes from a specific commit to your working directory using the `--no-commit` option, allowing you to review or modify them before finalizing the commit.
Here's the command you can use:
git cherry-pick --no-commit <commit-hash>
Understanding Git Cherry Pick
What is Cherry Picking?
Cherry picking in Git is a powerful feature that allows developers to select specific commits from one branch and apply them to another. This is particularly useful in collaborative development environments where multiple features or hotfixes are being developed in parallel. By cherry picking, you can integrate only the changes you need without merging an entire branch, thus keeping your main branch clean and focused.
When to Use Cherry Pick
Using cherry pick is beneficial in various scenarios, such as:
- Hotfixes: If a bug needs to be fixed in multiple branches, cherry picking allows you to apply the same fix across those branches without merging other changes unintentionally.
- Feature Porting: When a feature is refined in one branch and you want to apply that specific change to another branch, cherry picking is an efficient way to do so.
- Selective Updates: When you only want to bring over certain improvements or changes without affecting other parts of the codebase.
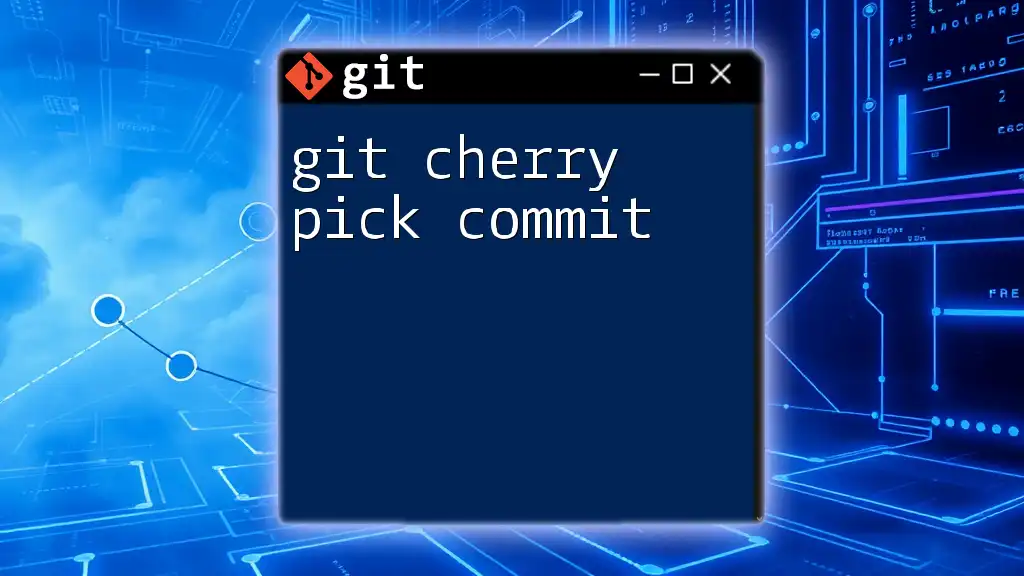
Cherry Picking Without Commit: A Deeper Dive
What Does "Without Commit" Mean?
By default, when you use the `git cherry-pick` command, Git automatically commits the changes you selected. However, there are situations when you may prefer to stage the changes without committing them immediately. Using `--no-commit` allows you to inspect and modify the cherry-picked changes before finalizing them.
Why Cherry Pick Without Committing?
There are several reasons you might want to cherry pick without committing:
- Reviewing Changes: It gives you the opportunity to examine the code changes, ensuring they fit seamlessly into your current branch.
- Making Adjustments: You might find that while the cherry-picked changes are useful, they require minor tweaks to align with the current code context.
- Staging Multiple Changes: If you are cherry picking multiple commits, you might prefer to collect all changes and then commit them together, streamlining your commit history.
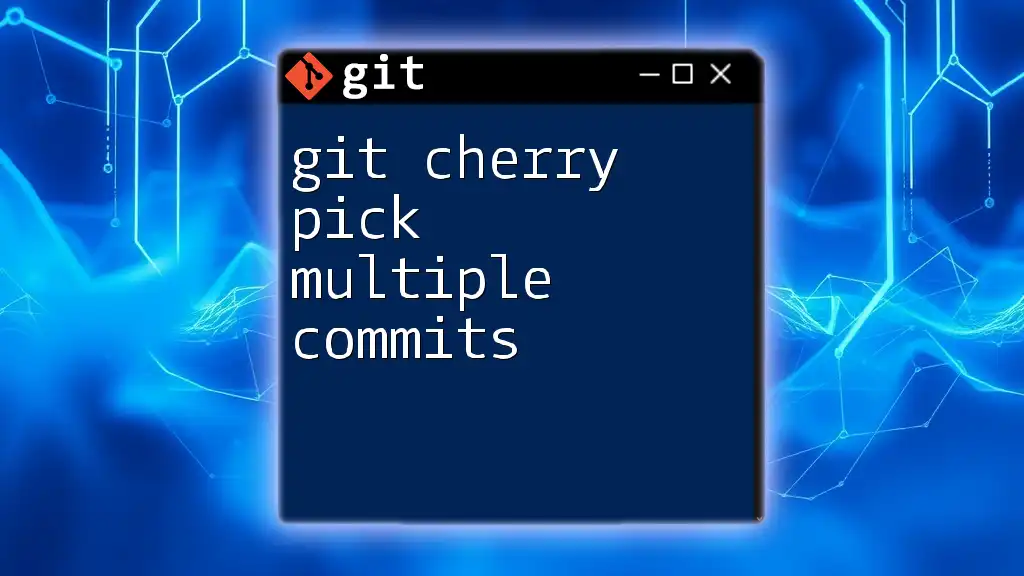
Steps to Cherry Pick Without Commit
Step 1: Identify the Commit
To cherry pick a commit, first, you need to identify its hash. You can view the commit history using:
git log
This command displays a list of commits along with their hashes. Note down the hash of the commit you wish to cherry pick.
Step 2: Using git cherry-pick with --no-commit
Now that you have the commit hash, you can cherry pick the changes without committing them by using the following command:
git cherry-pick <commit-hash> --no-commit
This command applies the changes from the specified commit to your working directory and stages them for review without making an immediate commit.
Step 3: Review Changes
After executing the cherry-pick command, it’s important to review the changes. To see what has been staged, you can use:
git status
To view the specific changes, you can use:
git diff --cached
These commands help you confirm that the changes have been applied correctly before committing them.
Step 4: Making Modifications (Optional)
If you find the changes need adjustments, you can edit the files as necessary. For example, if you need to add or remove lines, make your edits in the relevant files.
Once you have made the changes, uphold the staging area by adding the modified files:
git add <changed-file>
This command stages the updated file, preparing it for the final commit.
Step 5: Committing the Changes
Once you're satisfied with the changes, finish the process by committing them. Use:
git commit -m "Cherry picked changes from <commit-hash>"
Be sure to create a meaningful commit message that summarizes the changes effectively, as this aids in maintaining a clean project history.
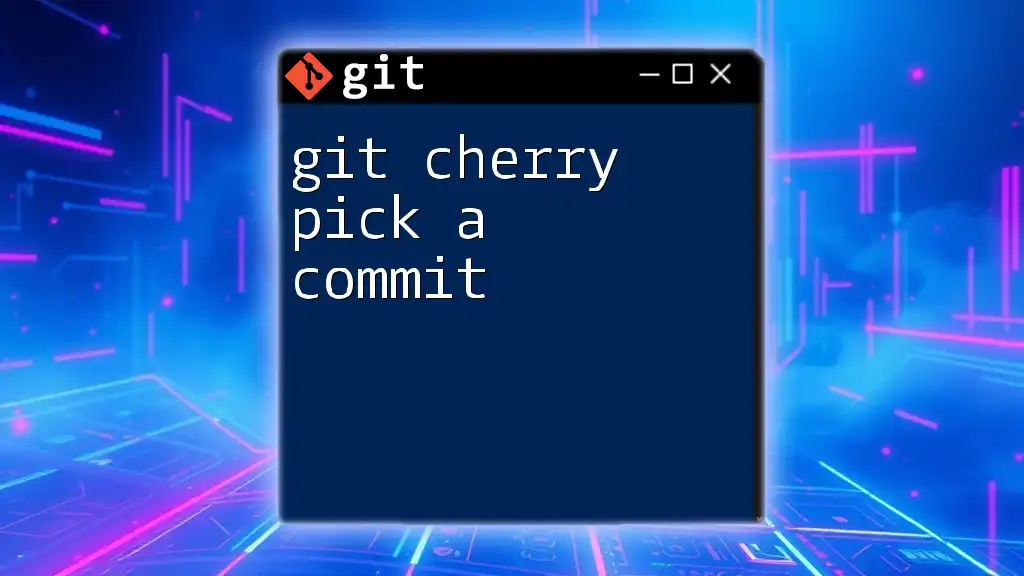
Handling Conflicts During Cherry Picking
What are Merge Conflicts?
Merge conflicts may arise during cherry picking if the changes you're trying to apply clash with existing changes in your current branch. Git will mark these conflicts, preventing the automatic cherry pick process from completing successfully.
Resolving Conflicts
If you encounter conflicts, Git will inform you which files are in conflict. To see the status, use:
git status
Resolve the conflicts in each file as necessary, and then mark the conflicts as resolved by adding the modified files:
git add <resolved-file>
Once all conflicts are resolved, complete the cherry-pick process by:
git cherry-pick --continue
Using Abort and Retry
If you decide that the cherry picking operation is not worth pursuing, or if conflicts are too complicated, you can abort the process:
git cherry-pick --abort
This command will return your branch to the state it was in before the cherry pick began, allowing you to retry later if needed.
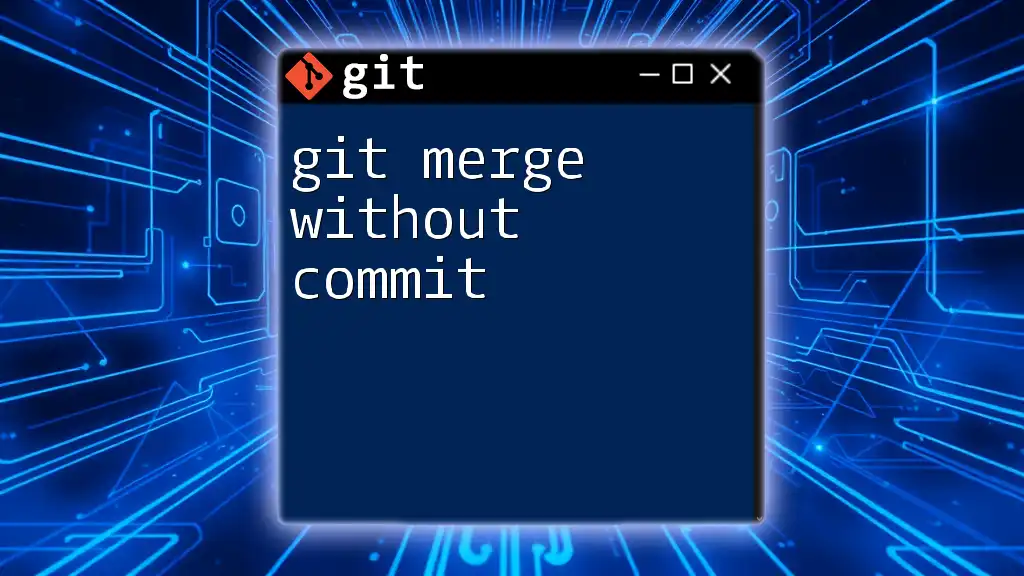
Best Practices for Cherry Picking
When to Avoid Cherry Picking
While cherry picking can be useful, there are instances when it may be best to avoid it. Consider avoiding cherry picking if:
- The commits you’re trying to cherry pick introduce complex changes that may not work well within the context of your current branch.
- Multiple related changes are present in the commits you're considering. In these cases, a full merge may yield a cleaner and more coherent result.
Maintaining a Clean Commit History
As a best practice, strive to keep a clean commit history. This involves:
- Writing clear, concise commit messages that describe what changes were made and why.
- Regularly reviewing and cleaning up your commit history to eliminate any unnecessary or redundant commits.
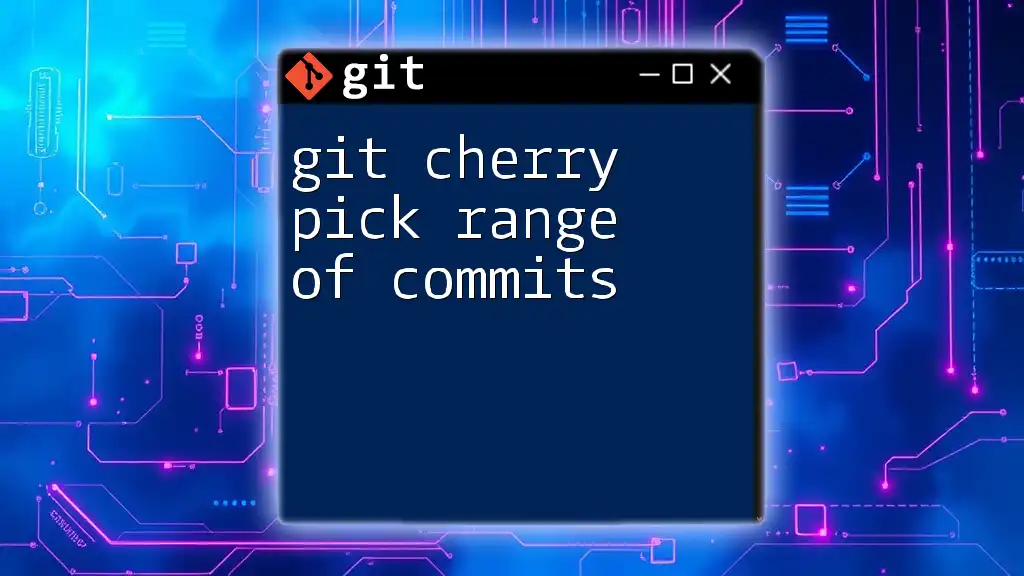
Common Pitfalls
Forgetting to Commit
One common mistake is failing to commit changes after cherry picking. This can lead to confusion and unintentional modifications in your working directory. Always ensure to commit or abort your changes after cherry picking to avoid leaving your branch in an ambiguous state.
Mixing Changes from Different Commits
If you cherry pick multiple unrelated changes without careful tracking, you can create confusion in your commit history. Be mindful about mixing different changes together; aim to scope cherry picks narrowly, keeping related changes together to maintain clarity.
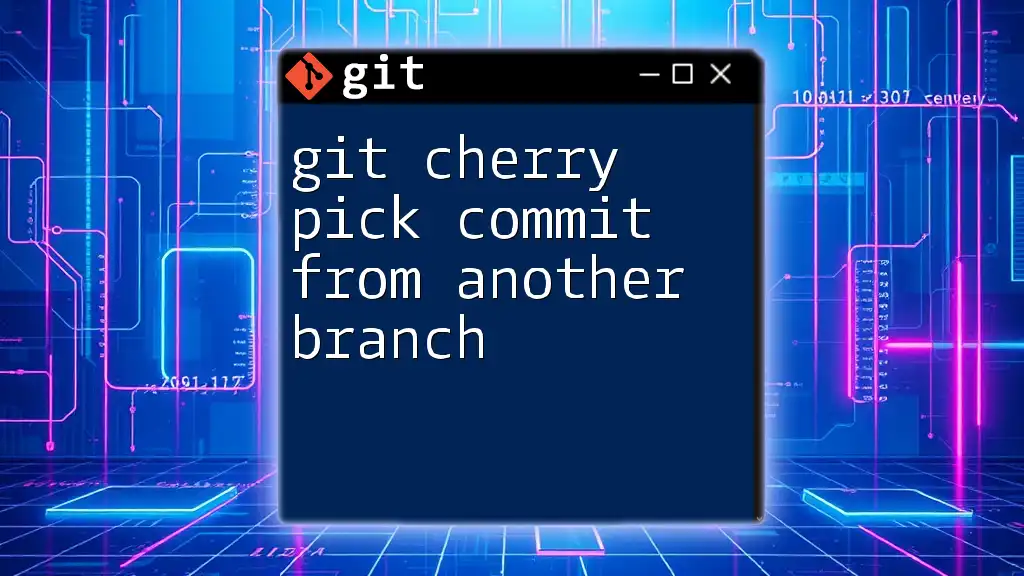
Conclusion
By understanding how to git cherry pick without commit, you can gain more control over your development workflow. This approach allows you the flexibility to review and modify changes before integrating them into your codebase, ensuring everything fits just right. Practice these commands regularly, and you'll be well on your way to mastering Git cherry picking and enhancing your collaboration efforts. Explore additional Git techniques to further streamline your version control processes—and happy coding!
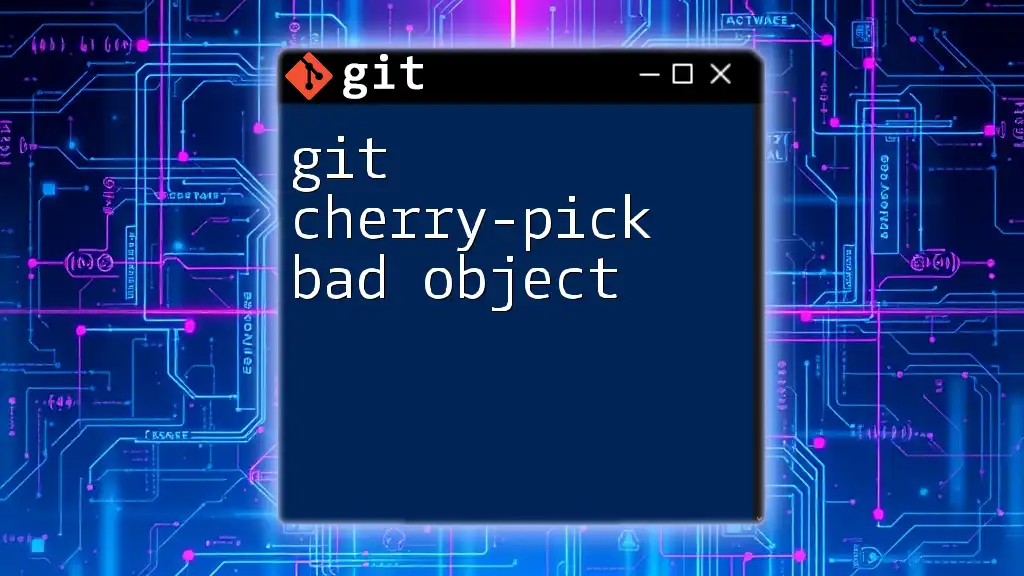
Further Reading
For more detailed information about Git commands, refer to the official Git documentation. This resource, along with additional tutorials on Git commands, will help you expand your knowledge and proficiency in using Git effectively.