`git cherry-pick` allows you to apply the changes introduced by a specific range of commits from one branch to another, enabling you to selectively incorporate features or fixes without merging entire branches.
Here's how to cherry-pick a range of commits:
git cherry-pick COMMIT_HASH1^..COMMIT_HASH2
Replace `COMMIT_HASH1` and `COMMIT_HASH2` with the actual commit hashes you want to cherry-pick.
Understanding Cherry Picking
What is Cherry Picking?
Cherry picking is a powerful feature in Git that allows developers to select specific commits from one branch and apply them to another. This is particularly useful in scenarios where you want to incorporate certain changes without merging an entire branch. Unlike merging, which combines all changes from one branch to another, cherry picking allows for a more controlled approach.
For example, if you have a feature branch that contains several commits, but only one of those commits is relevant to your production branch, you can cherry pick just that commit. This selective process is a key advantage of Git's flexibility in version control.
Key Terminology
To fully grasp the cherry picking process, it’s essential to understand some key terms:
- Commit: A snapshot of changes in your codebase; each commit has a unique identifier (SHA-1 hash).
- Branch: A pointer to a commit that allows for independent work on features.
- HEAD: The current branch reference in your Git repository.
- SHA-1 Hash: A 40-character string that uniquely identifies each commit.
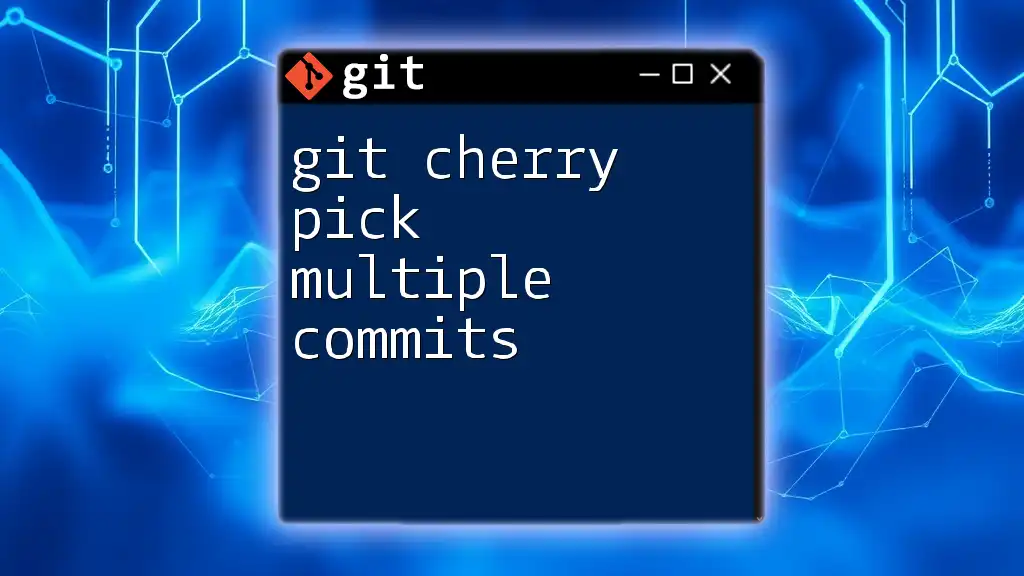
The Basics of Git Cherry Pick
How Cherry Picking Works
When you cherry pick a commit, Git applies the changes introduced in that specific commit onto your current branch. This is done by creating a new commit that contains the applied changes. The command essentially "replays" the changes in the selected commit, ensuring that only the alterations you want are included in your branch.
As an example, let’s say you want to take commit `abc1234` from your feature branch and apply it to your main branch. You would first switch to your main branch and run:
git cherry-pick abc1234
Syntax of the Cherry Pick Command
The basic syntax for cherry picking is straightforward:
git cherry-pick <commit_hash>
This command allows you to specify a single commit hash. If you need to pick multiple commits, you have to use a different approach, as elaborated in the next section.
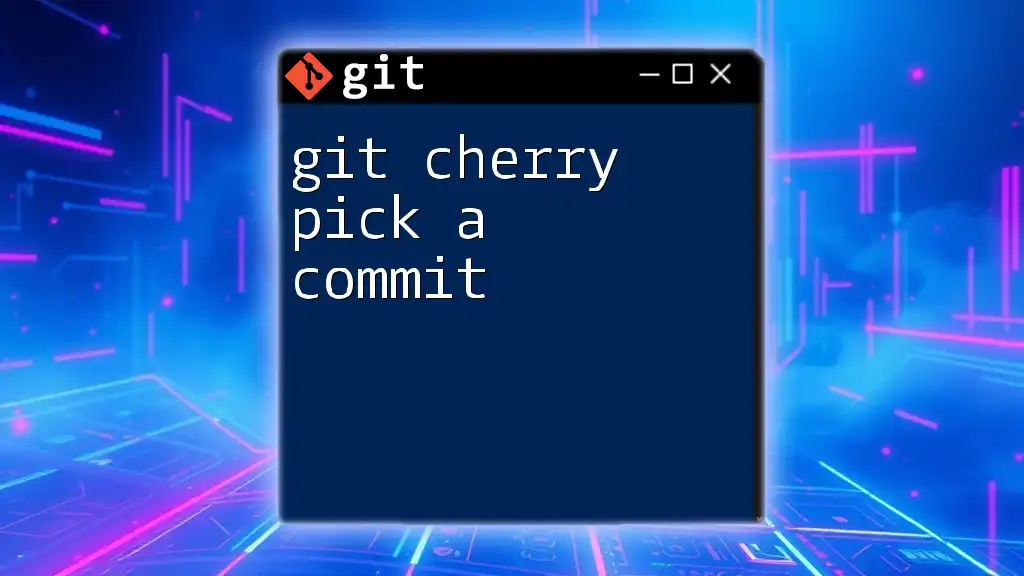
Cherry Picking a Range of Commits
Overview of Cherry Picking a Range
Cherry picking isn’t limited to single commits. You can also cherry pick a range of commits, making it easier to apply several changes at once. For instance, when developing a feature, a developer might create multiple commits. Depending on the situation, you may need to pick several at a time, rather than applying them one by one.
Syntax for Cherry Picking a Range
When cherry picking a range of commits, the syntax allows you to specify both the starting and ending commits. This is done using the `^` and `..` operators.
Here’s how it looks:
git cherry-pick <starting_commit_hash>^..<ending_commit_hash>
Important Note: The caret symbol (`^`) is used to include the starting commit in the selection.
Step-by-Step Guide
Gathering Your Commits
To cherry pick a range of commits, you first need to identify the commit hashes. You can view your commit history using:
git log --oneline
This command presents a succinct view of the commit history, allowing you to easily find the hash of the commits you wish to select.
Executing the Cherry Pick
Once you've identified the range of commits, you can proceed with the cherry pick. For example, if you want to apply all changes from commit `abc1234` to commit `def5678`, the command would be:
git cherry-pick abc1234^..def5678
Executing this command will apply all changes made in the specified range, from `abc1234` to `def5678`, onto your current branch.
Handling Merge Conflicts
During the cherry picking process, you might encounter merge conflicts if the changes conflict with the current state of your branch. When this happens, Git will halt the cherry pick and notify you.
To resolve conflicts:
- Use `git status` to check which files are conflicted.
git status
- Open the conflicted files and resolve the issues manually.
- After resolving, mark the conflicts as resolved using:
git add <resolved_file>
- Finally, complete the cherry pick with:
git cherry-pick --continue
This allows Git to finish applying the cherry-picked commits after all conflicts have been handled.
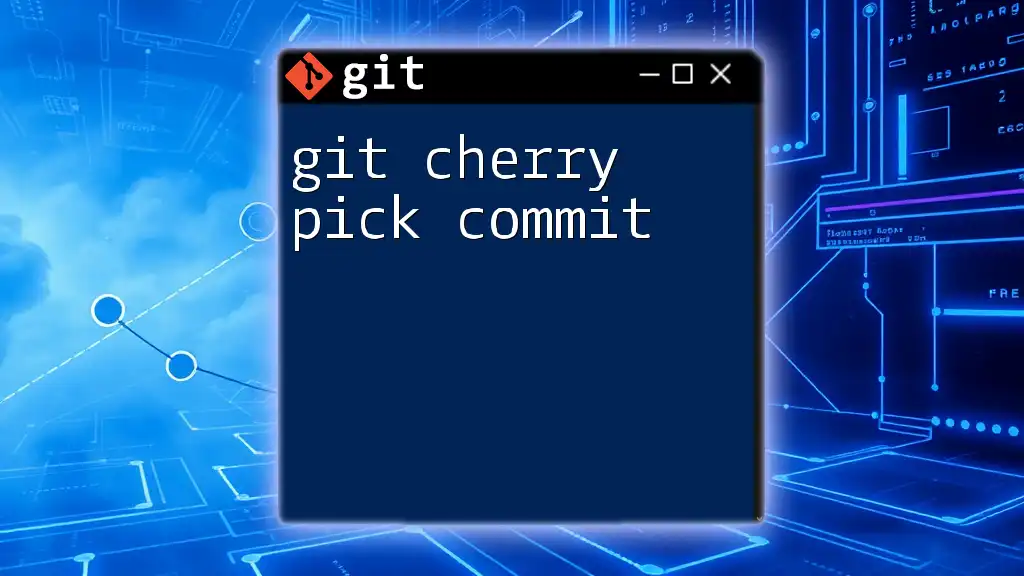
Best Practices for Cherry Picking
When to Use Cherry Picking
Cherry picking can be a great tool in a developer's toolkit. Use it effectively during situations like:
- Hotfixes: Quickly apply urgent patches from a feature branch to the main branch without waiting for the complete feature development to finish.
- Feature Branches: When a feature branch contains experimental changes, you might want to cherry pick only the stable commits.
Avoiding Common Pitfalls
While cherry picking is highly beneficial, avoid common mistakes. Always double-check which commits you are picking to prevent accidentally introducing unstable or unwanted changes. Additionally, ensure thorough testing after cherry picking to verify the integrity of your codebase.
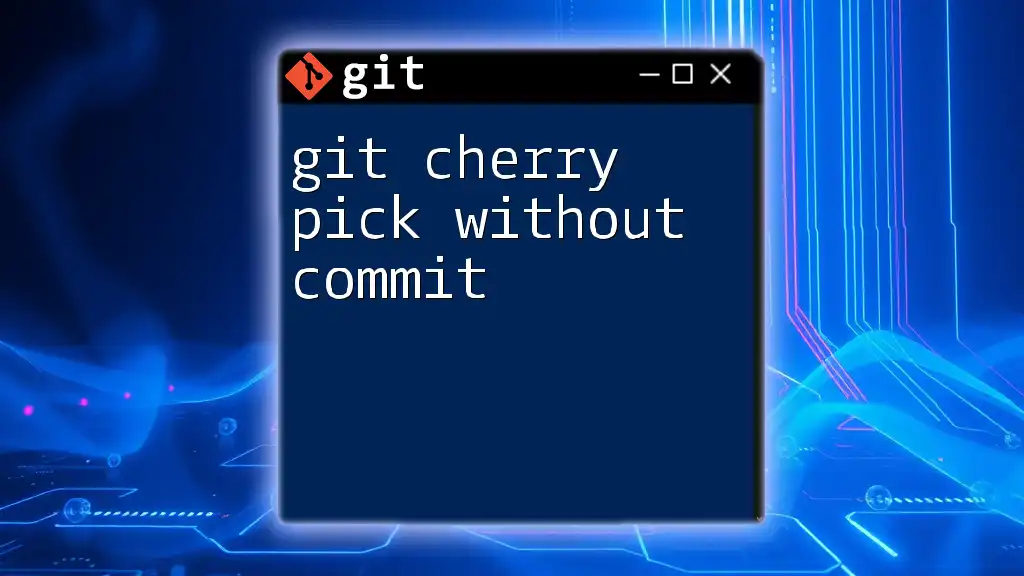
Advanced Cherry Picking Techniques
Interactive Cherry Picking
For advanced users, interactive rebase can work in tandem with cherry picking. Interactive rebase allows you to pick and reorder multiple commits with more control.
To start an interactive rebase, use:
git rebase -i <base_commit_hash>
In the interface that opens, you can choose which commits to pick and rearrange as needed.
Cherry Picking Across Different Branches
It’s also possible to cherry pick commits from different branches. Simply check out to the branch you wish to apply changes to and then use the cherry pick command:
git cherry-pick <commit_hash> --strategy-option theirs
This ensures that if there are conflicts, the current branch changes are preferred, effectively letting you take the necessary changes from the source branch.
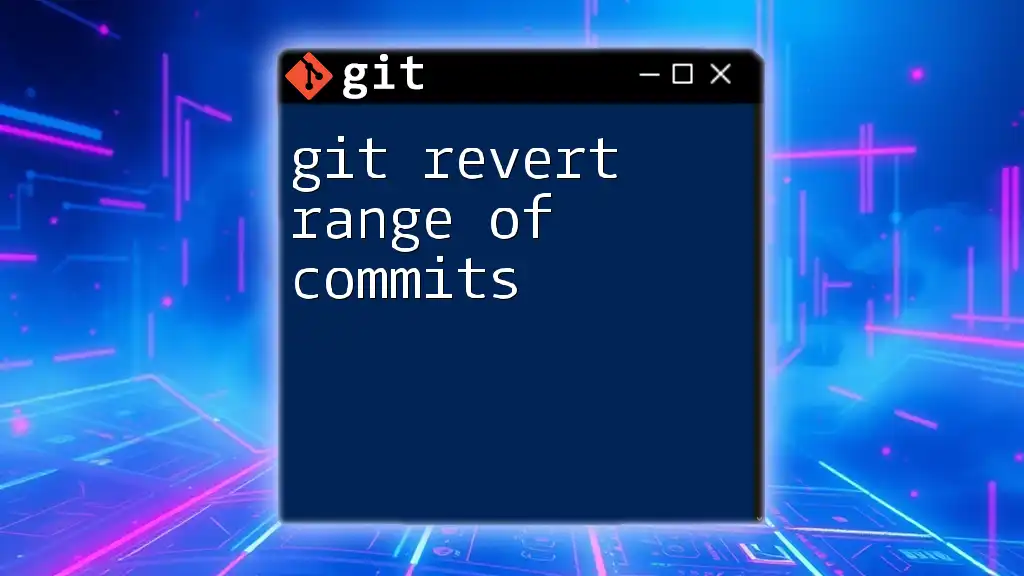
Conclusion
Cherry picking a range of commits is a valuable skill in Git, providing developers with the ability to fine-tune their codebases efficiently. Understanding the process, syntax, and the potential for conflicts is crucial for mastering this technique. By adhering to best practices and knowing when to use cherry picking, you can enhance your workflow and maintain a clean project history.
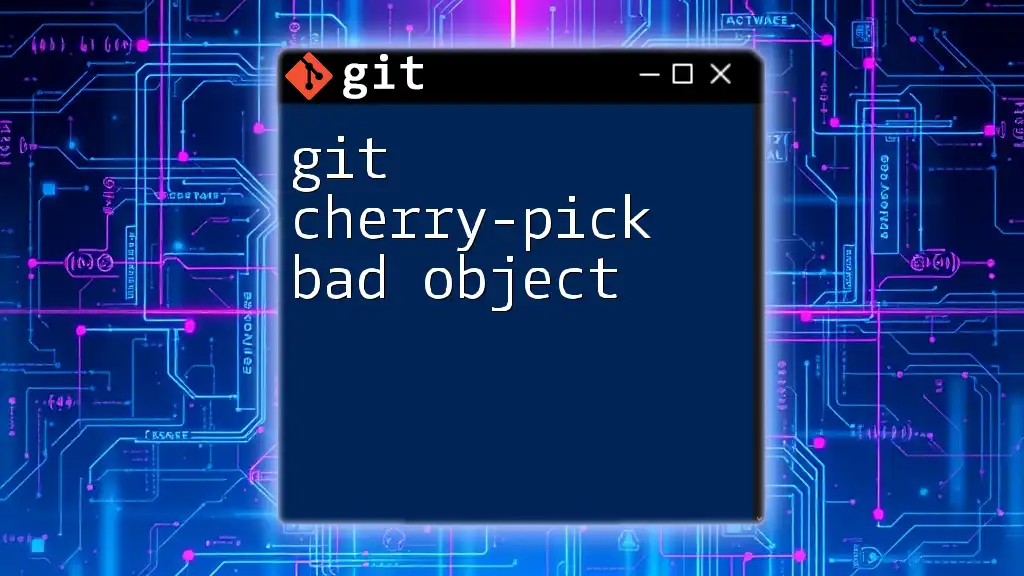
Additional Resources
For further exploration of Git and its cherry picking capabilities, consider investigating additional documentation and tools designed to streamline your version control experience.
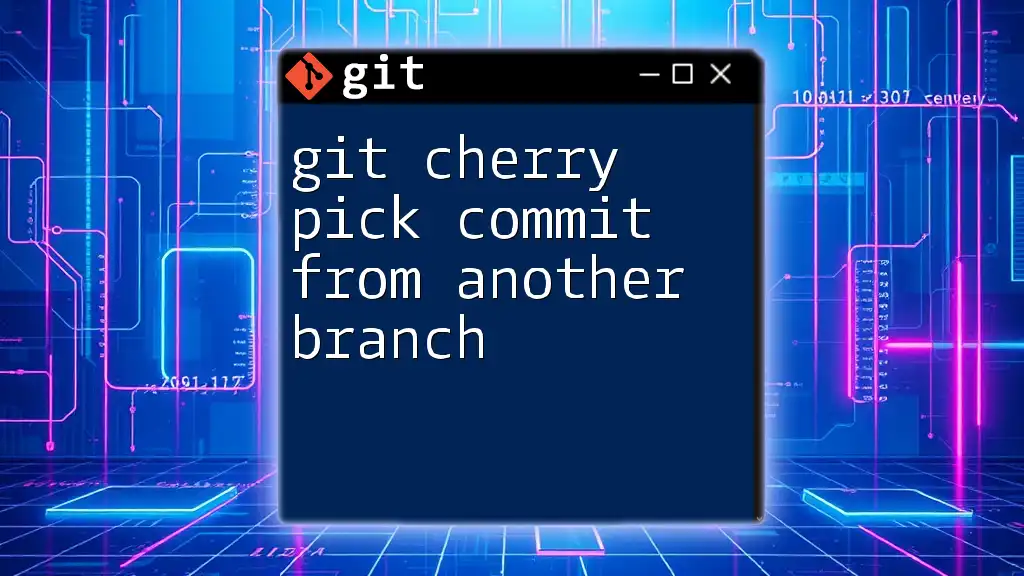
FAQs
What if I cherry pick a commit that was already applied?
If a commit has already been applied, Git will notify you about conflicts. You may need to resolve these conflicts manually.
Can I cherry pick commits from a different repository?
You cannot directly cherry pick from unrelated repositories. However, you can fetch the commits you need into a branch in your repository and then cherry pick from there.
How can I undo a cherry pick?
If you want to undo a cherry pick, you can use:
git cherry-pick --abort
This command will stop the cherry pick process and restore your branch to the previous state. If you have already committed changes, use:
git reset --hard HEAD~1
This will remove the last commit (the result of the cherry pick) and return to the previous state.