To cherry-pick a specific commit from another Git repository, you first need to add that repository as a remote, fetch its commits, and then apply the desired commit using its hash.
git remote add other-repo https://github.com/username/other-repo.git
git fetch other-repo
git cherry-pick <commit-hash>
What is Git?
Git is a powerful version control system that allows you to track changes in your code and collaborate with others efficiently. It is fundamental in managing projects, particularly in environments where multiple developers are working on the same codebase. By using Git, teams can work simultaneously without overwriting each other's contributions.
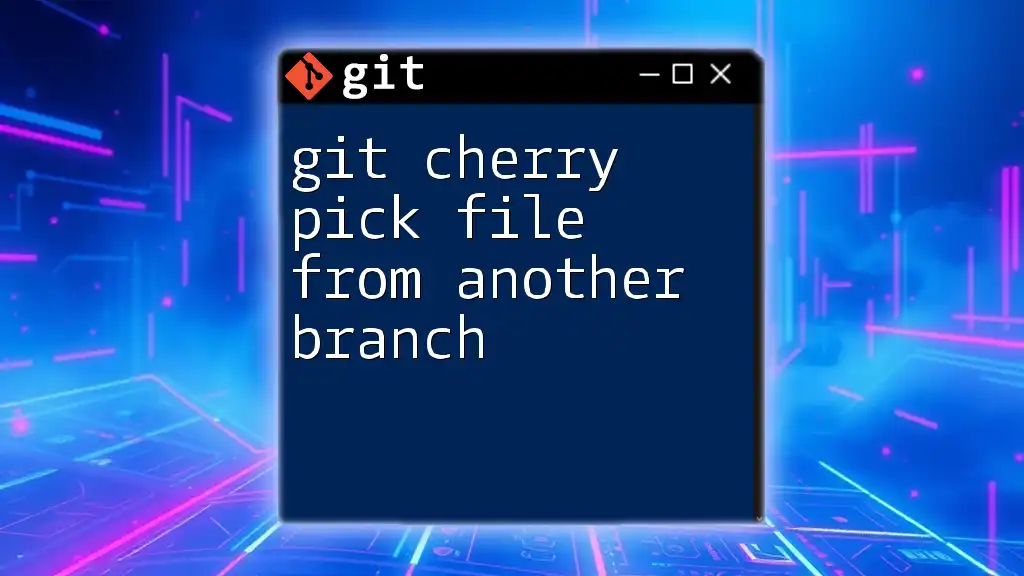
What is `git cherry-pick`?
`git cherry-pick` is a command that enables you to select specific commits from one branch and apply them to another. This technique is particularly useful in scenarios where you want to introduce changes from a different line of development without merging the entire branch. Common situations that benefit from cherry-picking include:
- Bringing bug fixes from a development branch to a production branch.
- Selectively applying features or changes when not all commits are relevant or ready for the destination branch.
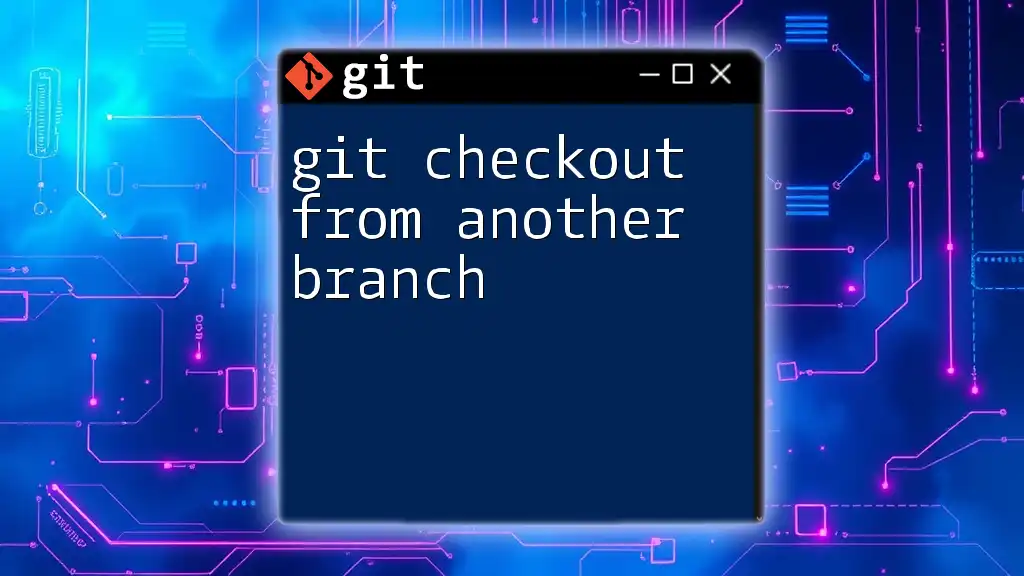
Understanding Git Repositories
What are Git Repositories?
A Git repository is a storage space for your project, where you can keep track of versions of your code. There are two main types:
- Local Repositories: These reside on your local machine, allowing you to work offline.
- Remote Repositories: Hosted on platforms like GitHub or GitLab, enabling collaboration among multiple users.
Cloning a Repository
To work on a remote repository, you first need to clone it to your local environment. Use the following command:
git clone https://github.com/username/repo.git
This command copies the entire repository, including its history, to your local machine, allowing you to work on it.
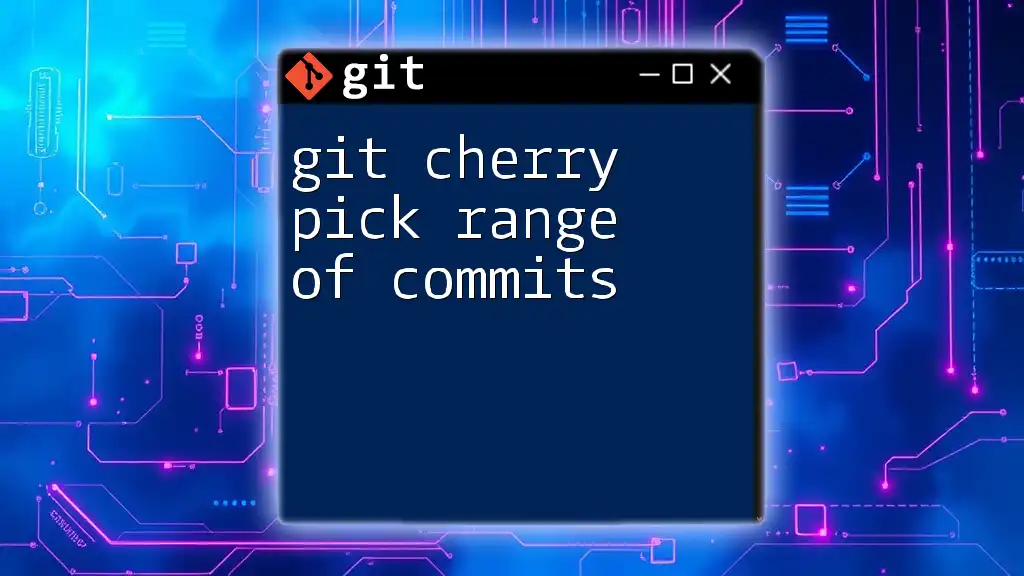
Preparing for Cherry-Picking
Setting Up Your Environment
Before you can cherry-pick commits, you need to switch to the branch where you want the commits applied. Use:
git checkout target-branch
This command ensures you are working on the correct context within your project.
Fetching Changes
To ensure you have the most recent changes from the upstream remote repository, run the fetch command:
git fetch upstream
Fetching will update your references to commits without merging, keeping your workspace clean while incorporating changes made by others.
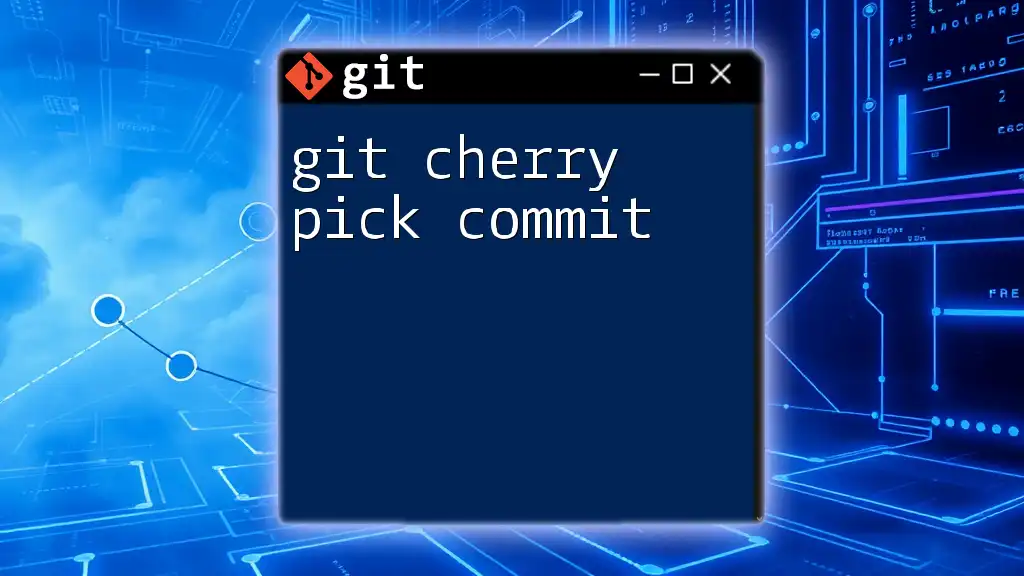
Executing `git cherry-pick`
Basic Syntax of `git cherry-pick`
The basic form of the cherry-pick command is structured as follows:
git cherry-pick <commit-hash>
Here, `<commit-hash>` is the unique identifier for the commit you wish to apply.
Identifying the Commit Hash
To cherry-pick a commit, you first need to find its hash. Use the following command to view the commit history:
git log --oneline
This command displays a simplified history of commits, showing the short commit hash along with the commit message, making it easy to identify the changes you want to apply.
Cherry-Picking from Another Repository
Understanding Cross-Repo Cherry-Picking
Cross-repo cherry-picking means selecting commits from one repository while working in another. It’s crucial to understand how different repositories can have different configurations and setups.
Steps to Cherry-Pick from Another Repo
-
Fetch the Desired Repository
If you haven’t added the remote repository from which you want to cherry-pick, do so with this command:
git remote add upstream https://github.com/other-repo.git git fetch upstream
This command links the other repository and updates your local reference to it.
-
Performing the Cherry-Pick
Now, you’re ready to cherry-pick. Execute the command with the commit hash from the other repository:
git cherry-pick <upstream_commit_hash>
Replace `<upstream_commit_hash>` with the actual commit hash you identified earlier. This command will apply the changes from the specified commit to your current branch.
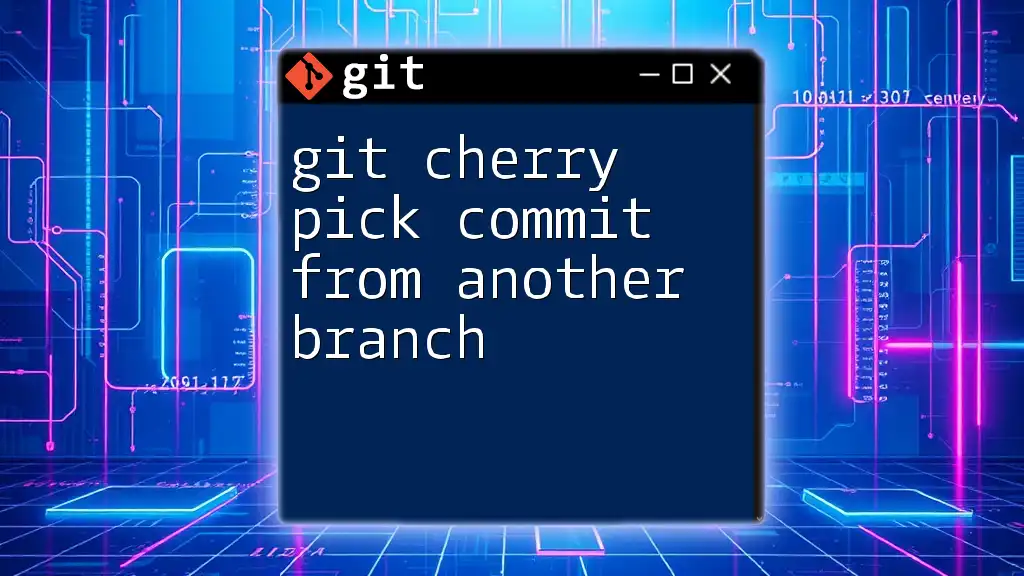
Handling Conflicts
What to Do When Conflicts Arise
Conflicts can occur during cherry-picking if the changes in the selected commit overlap with existing changes in your branch. Git will alert you to these conflicts, and you must resolve them before continuing.
Example of Conflict Resolution
To check the status of your repository and see which files are in conflict, use:
git status
Next, you can use a merge tool to help resolve conflicts:
git mergetool
After resolving the conflicts, you can continue with the cherry-pick process by running:
git cherry-pick --continue
This command finalizes the application of the commit after conflicts have been resolved.
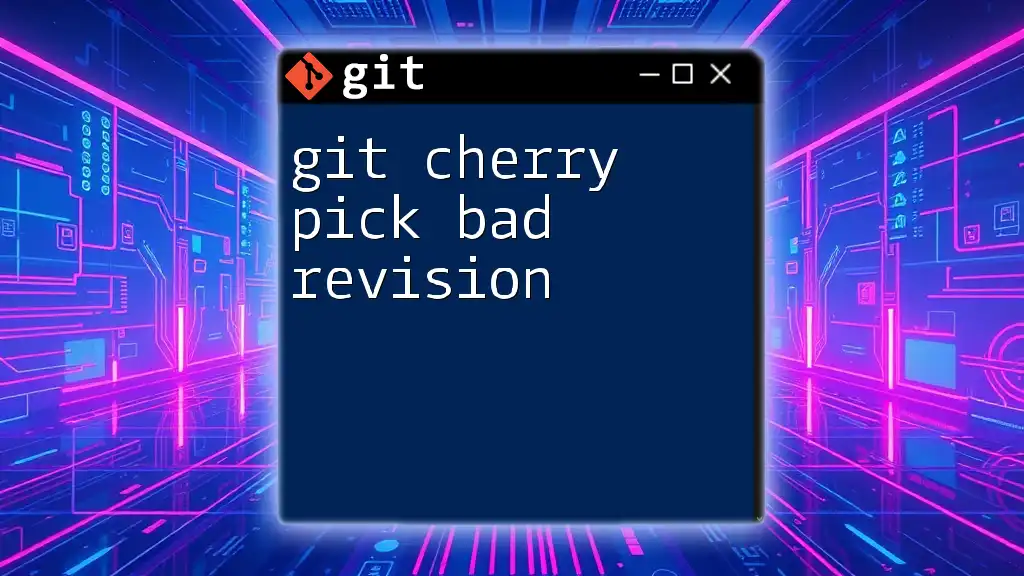
Best Practices for Cherry-Picking
When to Cherry-Pick
While cherry-picking is useful, it’s essential to use it judiciously. It works best when:
- You only want specific changes from another branch without merging or pulling all commits.
- You need to backport a bug fix to a production branch without integrating other newer changes.
Alternatives to Cherry-Picking
In some cases, merging or rebasing might offer a cleaner approach. Merging integrates all changes from one branch to another while maintaining the history, while rebasing allows you to pick a branch and replay its commits on another branch. Choosing the right strategy depends on your project’s needs and collaboration style.
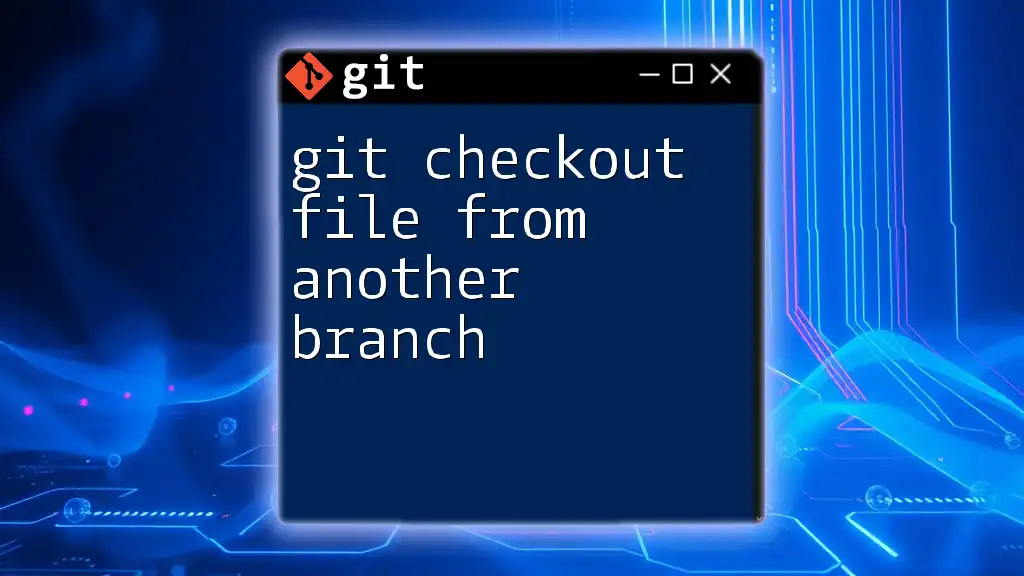
Conclusion
In this guide, we explored the process of using `git cherry-pick` from another repository. We discussed its purpose, preparation steps, execution, conflict resolution, and best practices. With a solid understanding of cherry-picking, you can effectively manage your versioning and collaboration in Git, ensuring that you apply only the changes that matter to your project.
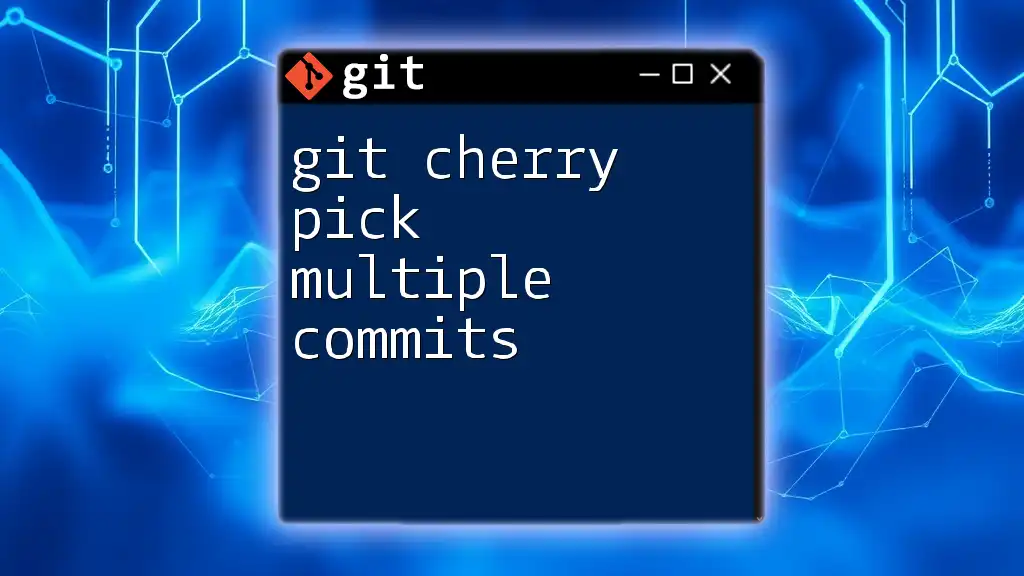
Questions About `git cherry-pick`
For quick reference, here are some common questions:
- What happens if the cherry-pick results in conflicts? You will need to resolve the conflicts before proceeding.
- Can I cherry-pick multiple commits? Yes, you can cherry-pick multiple commits by specifying their hashes in succession or using ranges.
- Is cherry-picking the same as merging? No, cherry-picking applies individual commits, while merging combines entire branches and histories.
Feel free to explore further and experiment with cherry-picking in your own projects!