To clone a Git project into another repository, use the `git clone` command followed by the repository URL and the desired destination folder name.
git clone https://github.com/username/repo.git new-repo
Understanding Git Cloning
What is Git Clone?
Git clone is a command used to create a copy of an existing Git repository. This command not only copies the files from the repository but also retains the complete version history. Cloning is fundamental for collaborative Git workflows, enabling developers to work on the same codebase simultaneously.
When to Use Git Clone
Cloning is especially useful when you want to:
- Start working on an existing project created by someone else.
- Create a local backup of a remote repository.
- Contribute to a project by making edits in your own copy of the repository.
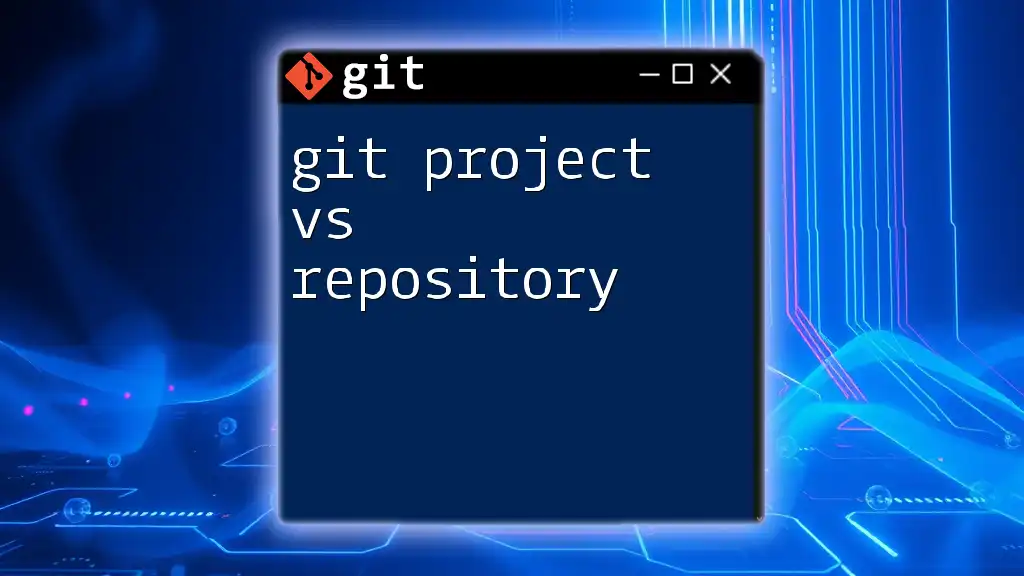
Pre-requisites for Cloning a Repository
Git Installation
Before you can clone a repository, ensure Git is installed on your system. Installation varies based on your OS. For most systems, you can follow these commands:
-
For macOS: Install via Homebrew:
brew install git
-
For Ubuntu/Linux: Use APT package manager:
sudo apt-get install git
-
For Windows: Download the installer from the official Git website.
To verify that Git is installed correctly, run:
git --version
Access to the Source Repository
It’s essential to have the appropriate permissions to clone a repository, especially if it is private. You may clone from:
- Public repositories without any authentication.
- Private repositories require authentication either through HTTPS credentials or SSH keys.
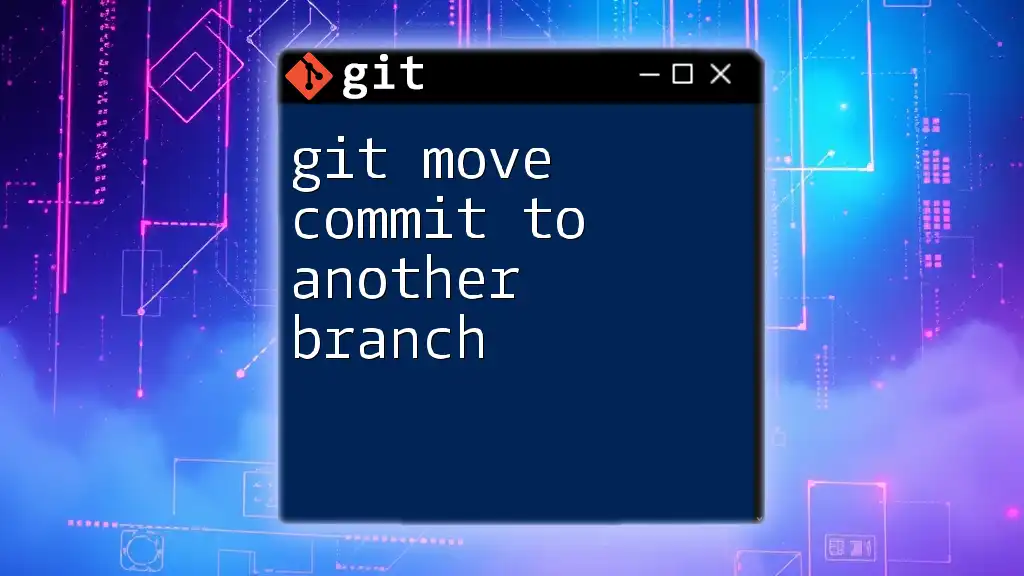
How to Clone a Repository
Basic Git Clone Command
The basic syntax for the `git clone` command is:
git clone <repository-url>
Cloning the Repository
To clone a repository, execute the following steps:
- Open your terminal or command prompt.
- Use the `git clone` command followed by the repository URL. For example, to clone a public GitHub repository:
git clone https://github.com/username/repo.git
This command creates a local copy of the repository, making it ready for you to work on.
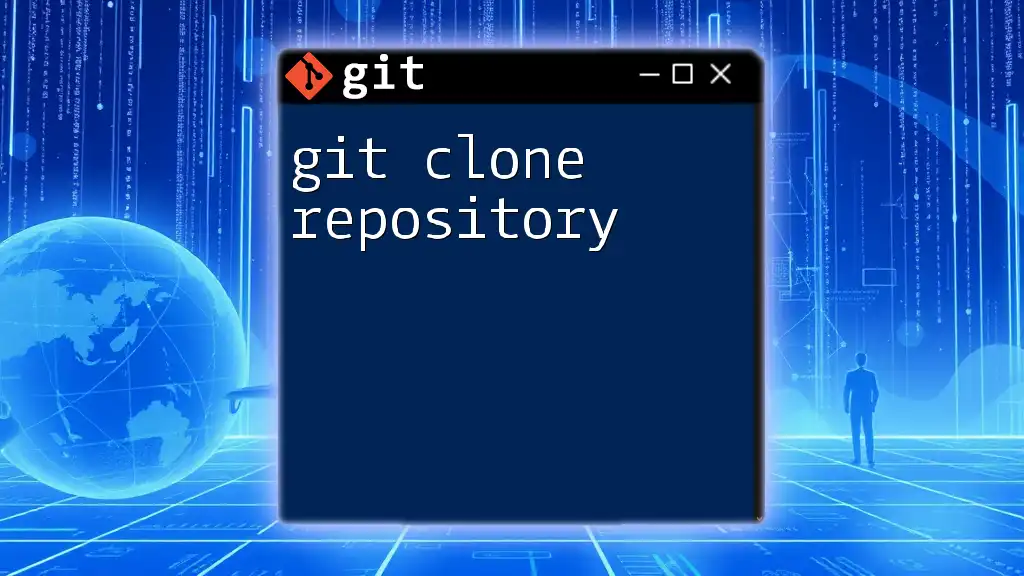
Cloning to a Specific Directory
Default Directory Behavior
When cloning a repository, Git creates a directory with the same name as the repository by default. For instance, using:
git clone https://github.com/username/repo.git
will create a folder named `repo`, containing all the files and history.
Specifying a Directory
If you want to clone the repository into a specific directory, you can simply add the desired directory name at the end of the command. For instance:
git clone https://github.com/username/repo.git my-project
This command will clone the repository into a folder named `my-project` instead of the default `repo`.
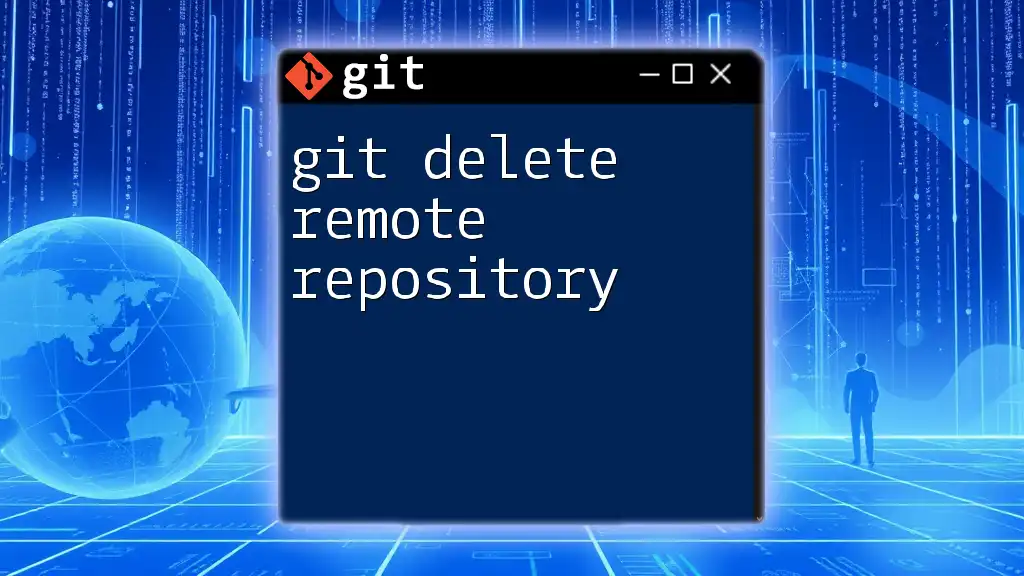
Cloning with SSH
Setting Up SSH Keys
Using SSH for cloning is recommended as it provides a more secure method of accessing private repositories. To set up SSH keys:
- Generate a new SSH key if you don't have one:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
- Copy the public SSH key using:
cat ~/.ssh/id_rsa.pub
- Add this key to your GitHub account under Settings > SSH and GPG keys.
Cloning via SSH
After setting up your SSH key, you can clone repositories with the SSH URL, ensuring a secure connection. The command looks like this:
git clone git@github.com:username/repo.git
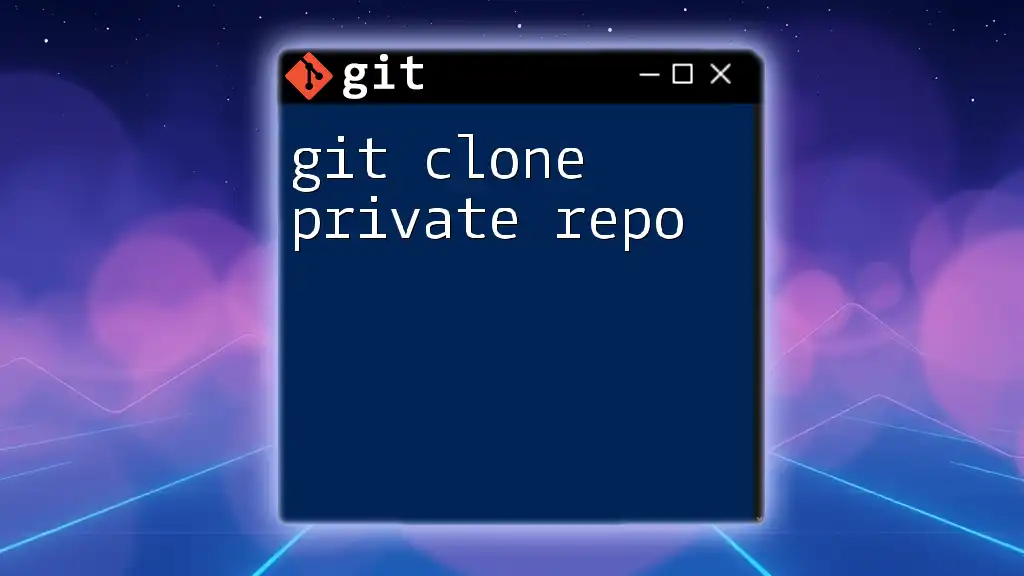
Understanding Git Clone Options
Cloning with Depth
When you clone a repository, you might not need the entire history of commits. The `--depth` option allows you to create a shallow clone, which includes only the most recent commits. This is useful for minimizing download size. Here’s how to do it:
git clone --depth 1 https://github.com/username/repo.git
Cloning a Specific Branch
If you only want to work on a specific branch instead of the master or main branch, use the `-b` option:
git clone -b branch-name https://github.com/username/repo.git
This command will clone only the specified branch, saving time and space.
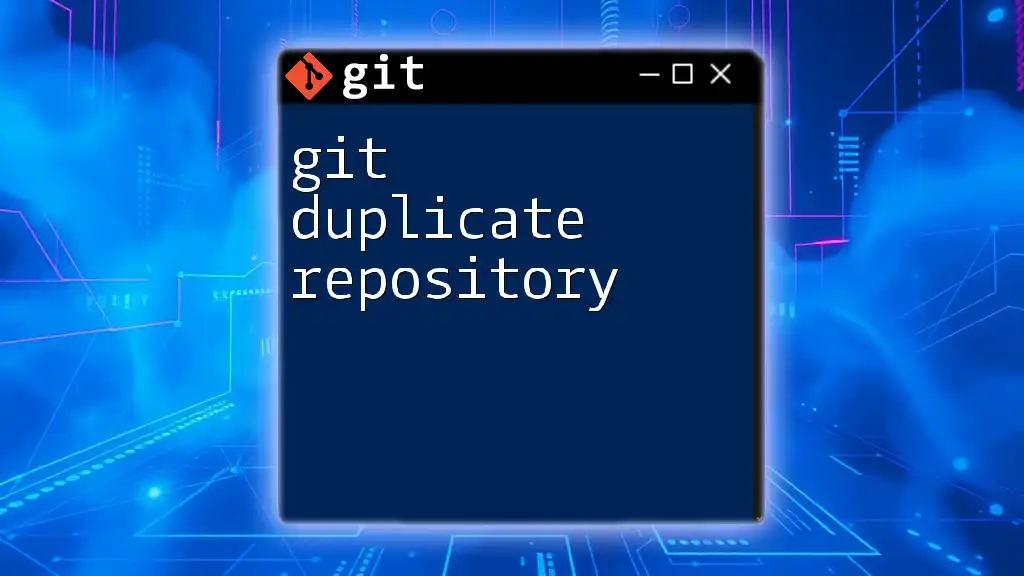
Post-Clone Operations
Verifying the Clone
After cloning, it’s critical to verify that everything cloned correctly and that your environment is set up properly. You can change directories into the newly cloned repository:
cd repo
And check the status:
git status
This makes sure you are in the right directory and that there are no pending changes.
Setting Up the Remote Repository
After cloning, verify the remote connection using:
git remote -v
This command lists all remote repositories associated with your local copy. If needed, you can change the remote URL with:
git remote set-url origin <new-repo-url>
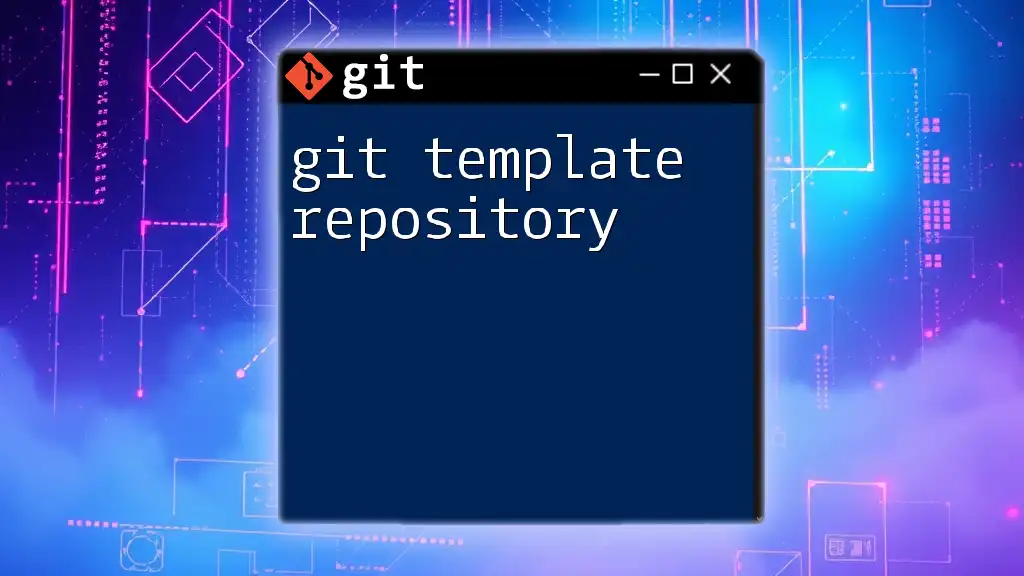
Troubleshooting Common Cloning Issues
Common Errors When Cloning
While cloning a repository, you may encounter several potential errors:
- 403 Forbidden Error: Often due to insufficient permissions, especially for private repositories.
- Repository Not Found: This usually arises from a typo in the repository URL.
Fixing Issues
To troubleshoot these issues:
- Verify URL: Check for typos in the repository URL.
- Check Permissions: Ensure you have adequate access rights for the repository. If using SSH, confirm that your SSH key is configured correctly.
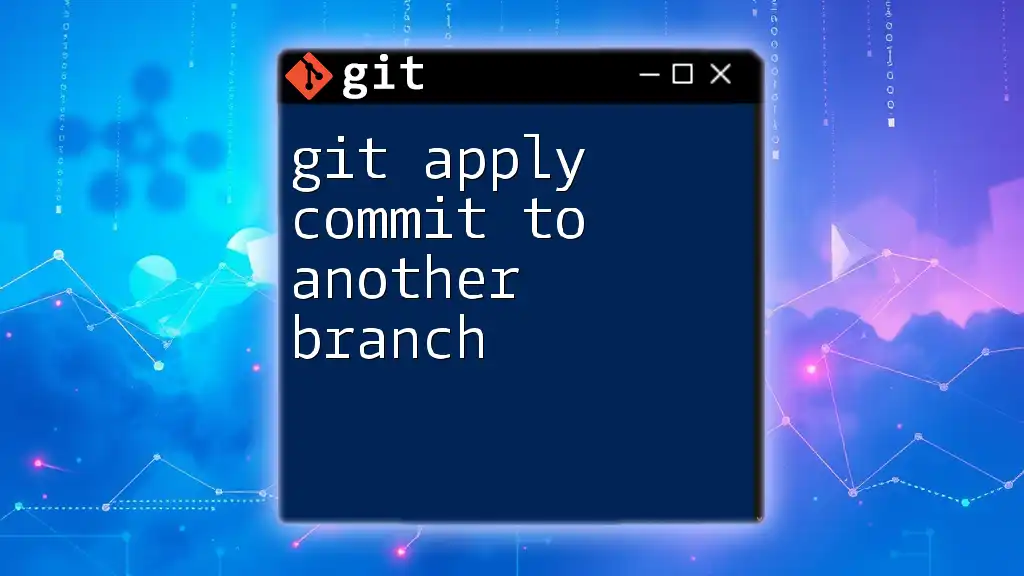
Conclusion
Mastering `git clone project to another repository` is a fundamental skill for any developer or professional working with version control systems. Understanding how to effectively clone, including directory management and handling SSH configurations, ensures you can navigate Git with ease. With practice, you’ll be able to streamline your workflow and collaborate efficiently with others.
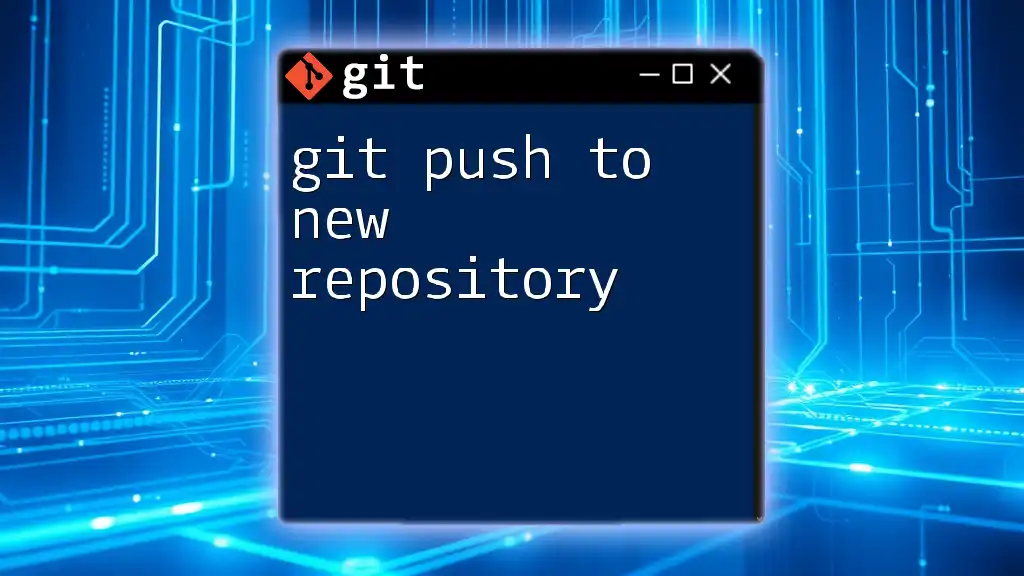
Additional Resources
- Official [Git documentation on cloning](https://git-scm.com/docs/git-clone)
- Online tutorials and courses for further learning in Git functionalities.
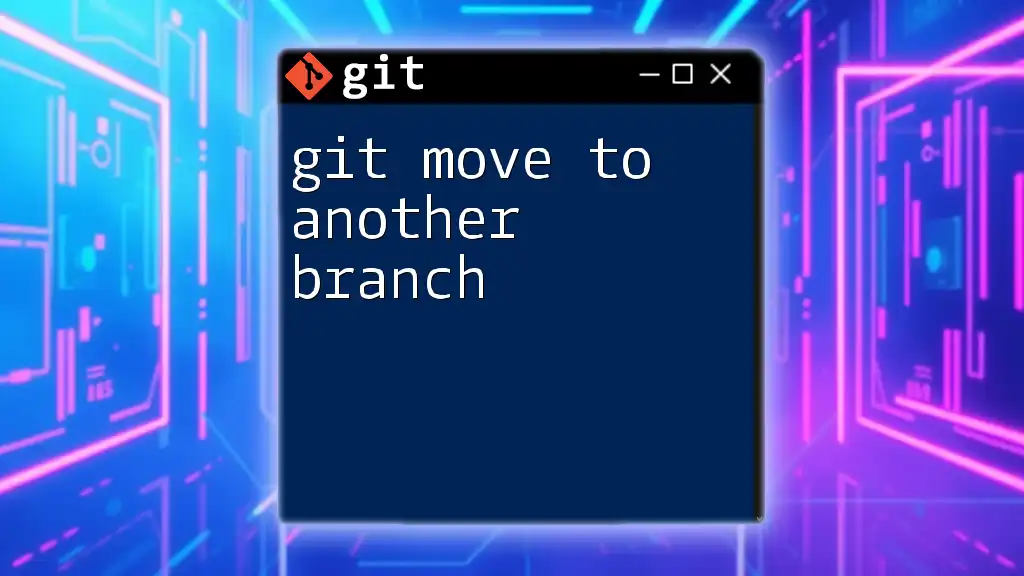
Call to Action
Don’t hesitate to dive into cloning your own repositories today! Explore new projects, contribute, and share your journey with the community. If you have any questions, feel free to ask!