"Git cherry-pick in VSCode allows you to apply specific commits from one branch to another easily using the command line or built-in Git tools."
Here's a code snippet that demonstrates how to perform a cherry-pick:
git cherry-pick <commit-hash>
Understanding Git Cherry Picking
What is Git Cherry Picking?
Git cherry-picking is a powerful feature that allows users to apply specific commits from one branch onto another without merging the entire branch. This means you can take a single change from a different line of development and integrate it into your current working branch. This is particularly useful in scenarios where you need to apply a bug fix or a specific functionality without bringing in other changes that may not be relevant.
Benefits of Using Cherry Picking
The cherry-picking approach offers several advantages:
- Selective Integration of Changes: You can integrate only those commits you need, maintaining finer control over what gets merged into your branch.
- Keeping the Commit History Clean: By cherry-picking, you avoid unnecessary commits that could otherwise clutter your project’s history.
- Facilitating Bug Fixes: If a bug is fixed in another branch, cherry-picking allows you to quickly apply that fix to your current working branch.
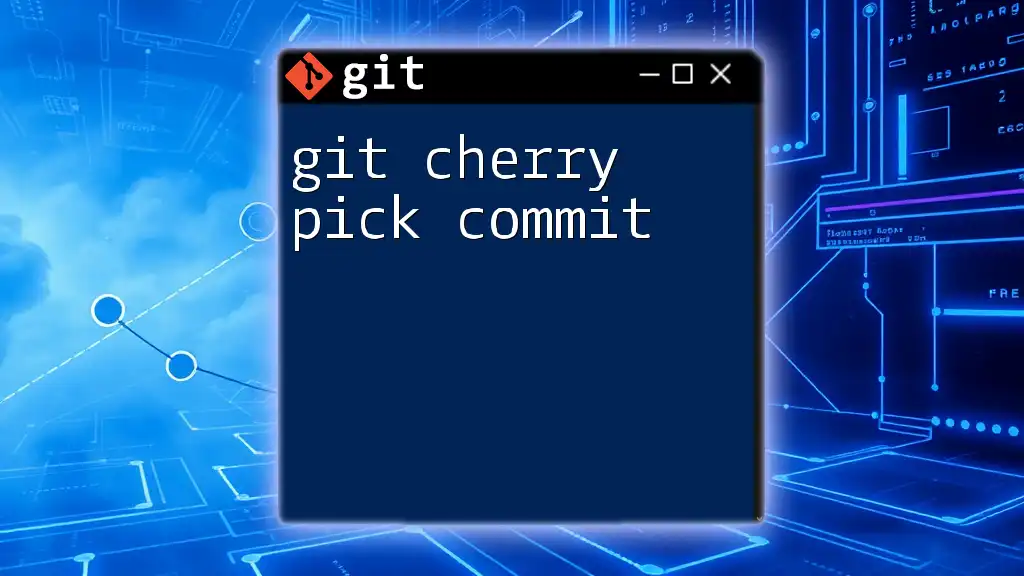
Setting Up VSCode for Git Cherry Picking
Installing VSCode and Git
Before you can start using cherry-picking in VSCode, you need to set up your environment properly. Here’s how:
- Installing VSCode: Download and install Visual Studio Code from the official website. It is available for Windows, macOS, and Linux.
- Setting up Git: Make sure you have Git installed. You can download it from the official Git website and follow the installation instructions relevant to your operating system.
Configuring VSCode for Version Control
Visual Studio Code comes with built-in support for Git, but there are steps you can take to enhance your experience:
- Extensions for Git in VSCode: Install extensions like “GitLens” to gain additional insights into your commits and branches.
- Source Control Interface Overview: Familiarize yourself with the Source Control panel, which allows you to manage your version control activities directly from the editor.
- Customizing Settings: Go to File → Preferences → Settings and look for Git-specific settings to optimize your workflow in VSCode.
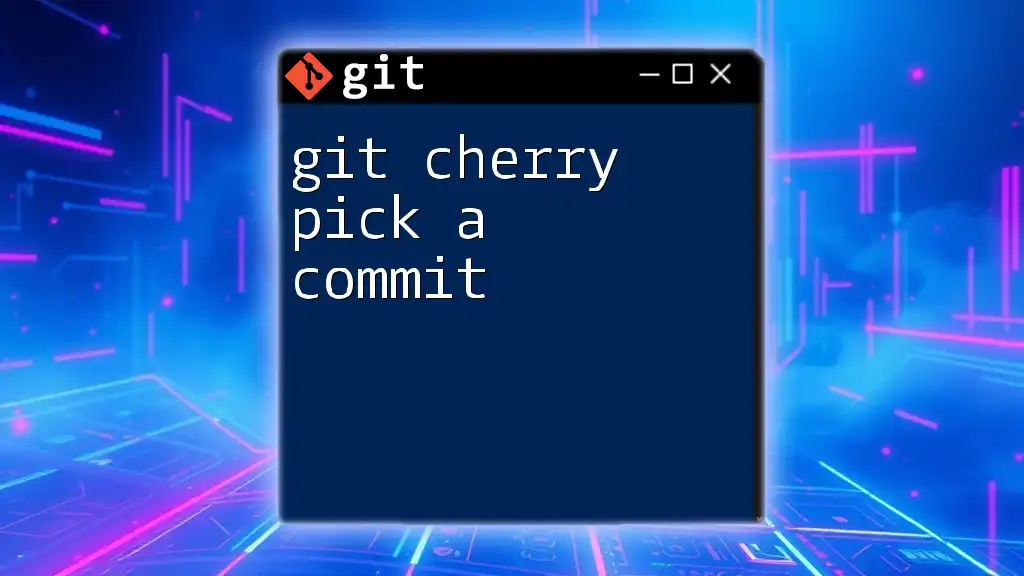
Performing Cherry Picking in VSCode
Step-by-Step Guide to Cherry Picking
Viewing Commit History
Before cherry-picking, you need to view the commit history to identify the specific commit you want. You can do this directly within VSCode:
- Navigate to the Source Control Panel and click on the three dots for more options.
- Select View History to see a list of commits on the current branch.
Alternatively, in the terminal, you can use the following command to view commit history:
git log
Selecting a Commit to Cherry Pick
Once you have the commit history, you can select the commit you want to cherry-pick. Make sure you note its commit hash, which is a unique identifier for that commit. This identifier is essential for the cherry-picking command to work properly.
Executing the Cherry Pick Command
Cherry Picking via the Command Palette
One of the simplest ways to cherry-pick in VSCode is through the command palette:
- Open the command palette by pressing `Ctrl + Shift + P` (or `Cmd + Shift + P` on macOS).
- Type Git: Cherry-Pick Commit and select it.
- Enter the commit hash you noted earlier and hit Enter.
VSCode will attempt to apply the changes associated with that commit to your current branch.
Cherry Picking with the Terminal
If you prefer using the command line, you can also execute the cherry-pick command directly in the terminal integrated into VSCode:
git cherry-pick <commit-hash>
Replace `<commit-hash>` with the actual hash of your desired commit.
Handling Merge Conflicts
Identifying Conflicts
During the cherry-picking process, it's possible that conflicts may arise if the changes in the selected commit conflict with the current state of your branch. VSCode will highlight such conflicts in the UI, making it easy to identify which files are affected.
Resolving Conflicts in VSCode
Resolving conflicts is a critical part of version control. To handle merge conflicts in VSCode:
- Locate the file(s) with merge conflicts, which will be marked with highlighted sections in the editor.
- Use the built-in merge editor to choose between the incoming changes and the current branch changes, or combine them as necessary.
- After resolving the conflicts, stage your changes:
git add <file>
- Finally, to complete the cherry-pick operation after resolving conflicts, run:
git cherry-pick --continue
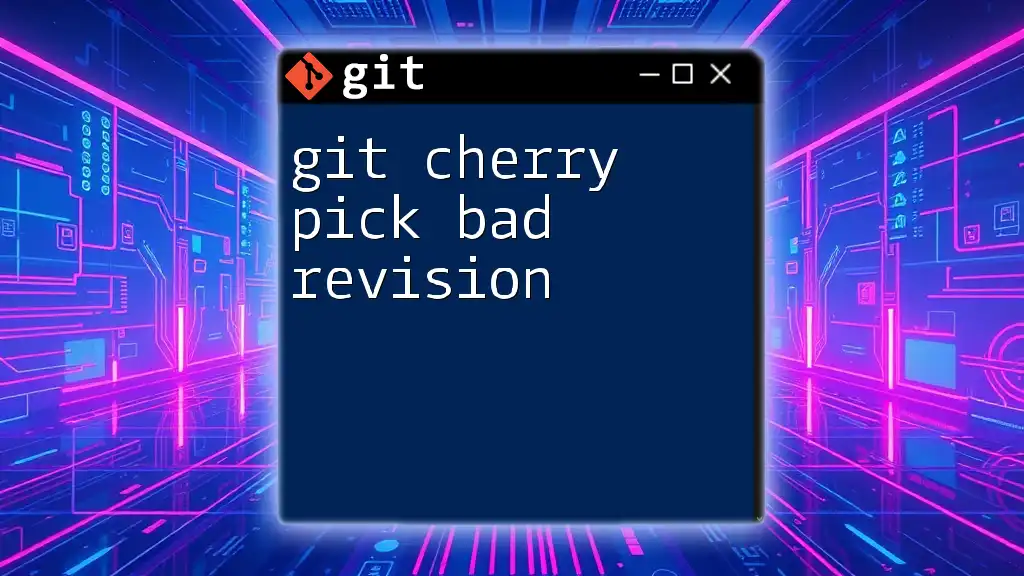
Best Practices for Cherry Picking
When to Avoid Cherry Picking
While cherry-picking can be helpful, there are times you might want to avoid this technique:
- Complex Changes: If a set of changes affects multiple commits and is interdependent, cherry-picking may lead to inconsistencies.
- Frequent Collaboration: In projects with many collaborators, cherry-picking commits selectively can cause confusion about the state of the code base.
Keeping Commit History Clean
To maintain a clean commit history while using cherry-picking effectively, consider these strategies:
- Ensure each cherry-picked commit is well described.
- Regularly communicate with your team to avoid duplicating efforts and to understand when and why specific commits have been cherry-picked.
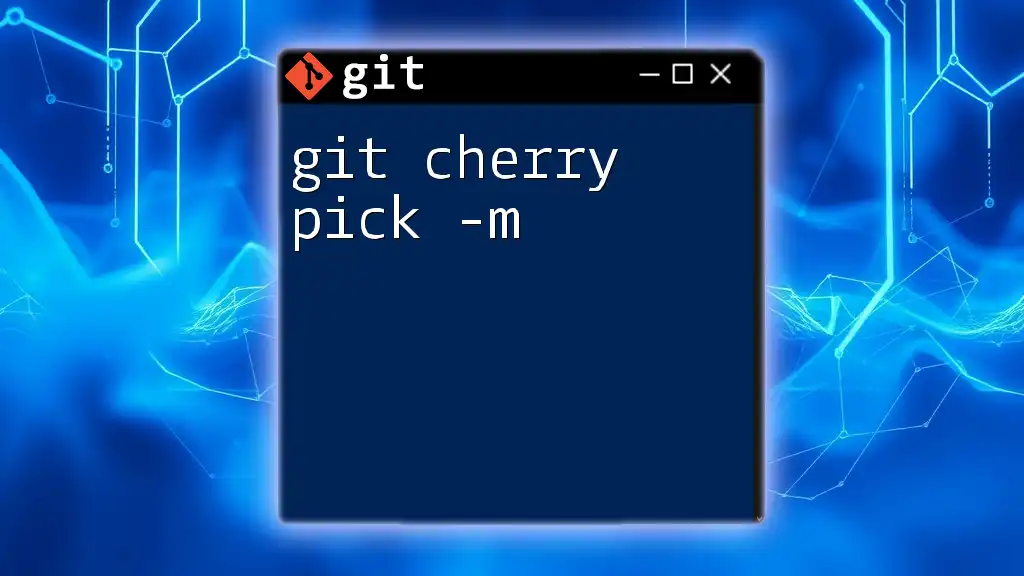
Conclusion
In conclusion, mastering git cherry pick vscode can greatly enhance your efficiency and control over version management in your projects. By selectively applying changes and maintaining a clean commit history, you can streamline your development process. Practice these cherry-picking techniques in VSCode to become a more proficient Git user and better collaborate with your team.
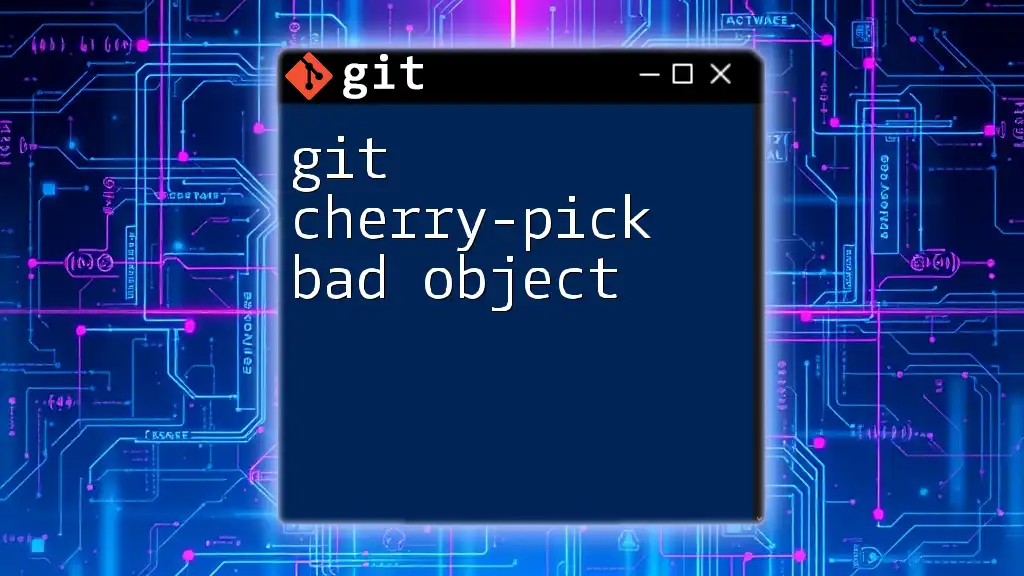
Additional Resources
Feel free to explore the official Git documentation for in-depth knowledge of Git features. Consider checking out excellent extensions for Git in VSCode to further enhance your productivity. Finally, reading more about version control best practices can help solidify your understanding and improve your project management skills.