When using `git cherry-pick` to apply a commit, it's important to be cautious with "bad revisions"—commits that introduce errors or undesirable changes—since they can negatively impact your codebase if not properly addressed.
git cherry-pick <commit_hash> # This applies the specified commit to your current branch.
Understanding Git Cherry-Pick
What is Git Cherry-Pick?
Git cherry-pick is a powerful command used to apply specific commits from one branch onto another branch. This command is particularly useful when you want to introduce changes that exist in one branch without merging the entire branch. For instance, if you have a hotfix in your development branch that needs to be applied to your production branch, you can cherry-pick just that hotfix instead of merging all changes.
When it comes to cherry-picking, it's important to use it wisely, particularly with bad revisions that might lead to issues in your codebase. Understanding the implications of choosing specific commits is crucial for maintaining a clean and functional project.
How Cherry-Picking Works
The cherry-picking process involves selecting a commit by its hash and applying it to your current branch. Unlike merging, which combines the entire work of a branch, cherry-picking can be seen as a selective copy operation.
It is essential to remember the differences between cherry-picking and other Git commands:
- Merge combines entire branches, along with all their changes.
- Rebase moves an entire series of commits to a new base commit, keeping changes in a linear sequence.
- Cherry-pick allows you to grab just the commits that you want from a branch.
This selectivity can be powerful, especially when working with a commit that you know is beneficial, but it can also introduce risks when dealing with bad revisions.
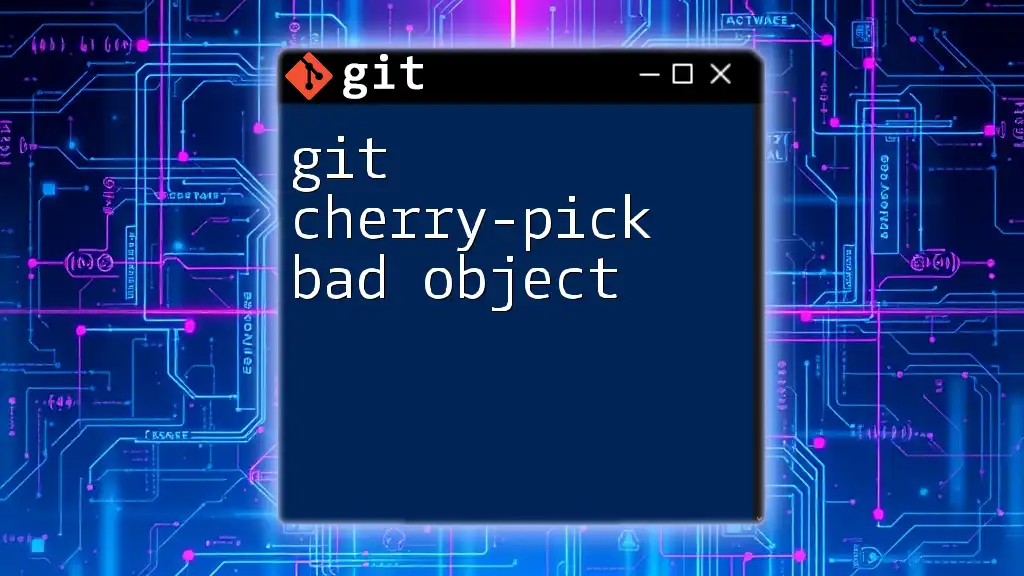
Identifying Bad Revisions
What Constitutes a Bad Revision?
A bad revision is typically characterized by defects such as broken code, syntax errors, poor implementation logic, or incomplete features. These issues can disrupt the stability of your application and lead to larger problems down the line.
Consider the following examples:
- A commit that introduces a major bug which causes functionality to fail.
- A change that doesn’t fulfill the intended feature requirements.
Understanding what a bad revision looks like is the first step towards being able to handle it appropriately when using `git cherry-pick`.
How to Identify Bad Revisions in Your Repository
Identifying bad revisions involves using some Git tools and commands:
- Use `git log`: This command provides a historical view of changes made to the repository, allowing you to scrutinize commit messages and changes.
- Use `git diff`: This command helps to see the differences between commits, giving insight into what has changed and whether those changes are problematic.
By effectively using these tools, you can examine your commit history and catch bad revisions before they become a larger issue.
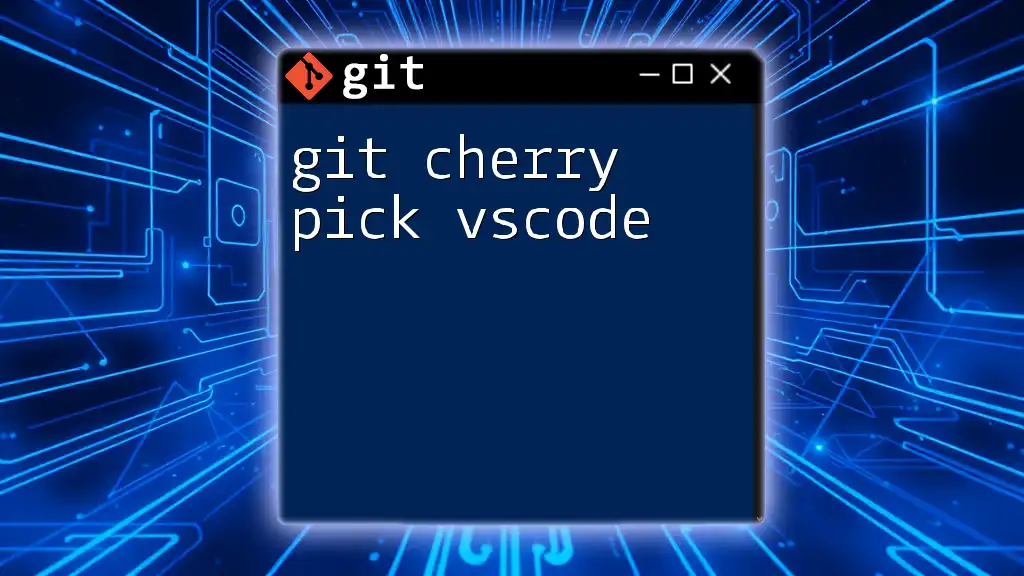
Using Git Cherry-Pick with Bad Revisions
The Basic Cherry-Pick Command
Chery-picking a commit is straightforward, using the basic syntax:
git cherry-pick <commit-hash>
This command applies the changes made in the specified commit to your current branch.
However, when cherry-picking a bad revision, you must proceed with caution, as it can lead to complications in your codebase.
What Happens When You Cherry-Pick a Bad Revision?
When you cherry-pick a bad revision, several potential problems can arise:
- Code Conflicts: If the codebase has changed since the bad commit was made, conflicts may occur, necessitating resolution.
- Cascading Errors: A bad commit can introduce errors that may not surface until runtime, complicating debugging.
Understanding these risks is crucial for maintaining the integrity of your projects, especially when cherry-picking questionable revisions.
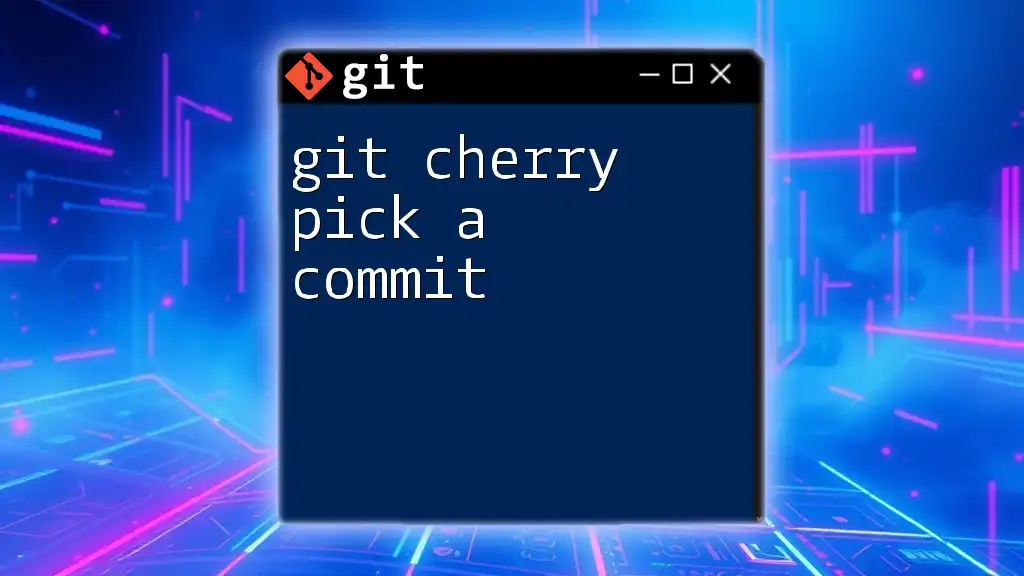
Steps to Cherry-Pick a Bad Revision Safely
Backup Your Current Branch
Before attempting to cherry-pick a potentially harmful commit, backing up your current branch is essential. This precaution ensures that you can easily roll back to a stable state if needed. To create a backup branch, you can use the following commands:
git branch backup-branch-name
git checkout backup-branch-name
This way, you can experiment with cherry-picking without the fear of losing your progress.
Performing the Cherry-Pick Command
Once you have a backup, you can execute the cherry-pick command on the bad revision. For instance:
git cherry-pick <commit-hash>
By executing this command, you apply the changes associated with the bad revision to your current branch.
Handling Conflicts
Conflicts may arise during the cherry-pick if the changes in the selected commit clash with changes in your current branch. Here's how to handle conflicts:
- After the command fails, use `git status` to identify which files are in conflict.
- Launch your merge tool using:
git mergetool
- Resolve the conflicts manually in the affected files.
- Once resolved, stage the changes:
git add <resolved-file>
- Finally, continue the cherry-pick process:
git cherry-pick --continue
By following these steps, you can effectively manage conflicts introduced by a bad revision.
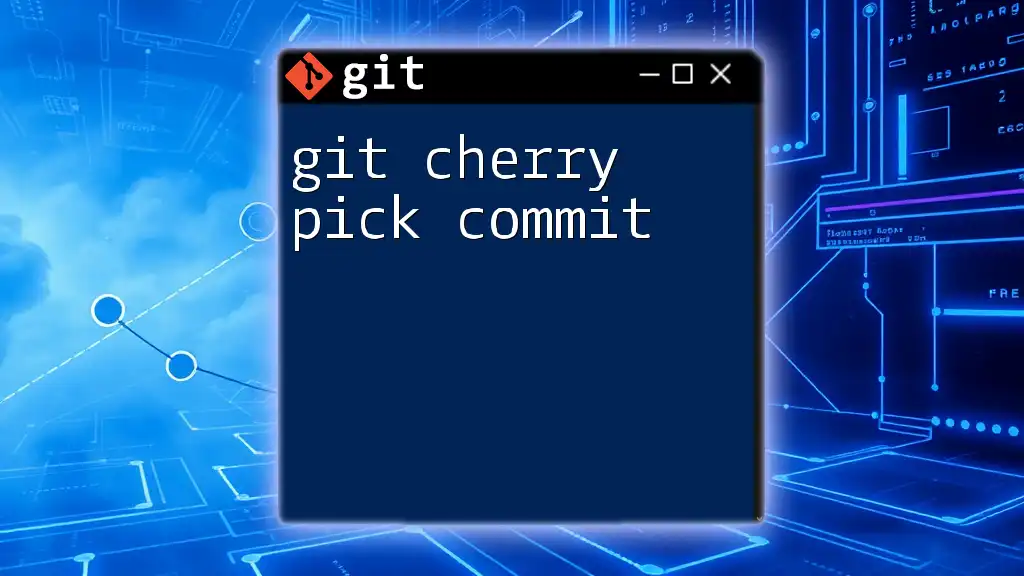
Best Practices for Cherry-Picking Bad Revisions
Cherry-Pick with Caution
Always exercise caution when cherry-picking commits, especially bad revisions. If possible, consider avoiding cherry-picking entirely. Alternatives like reverting the commit can sometimes provide a cleaner solution without introducing problematic changes.
Document Your Changes
Documentation is crucial when cherry-picking bad revisions. Maintain clear commit messages that describe why the commit was selected and any implications it may have on the codebase. This practice aids future team members in understanding the context of decisions made during development.
Build and Test After Cherry-Picking
After cherry-picking a commit, it's imperative to build and test your application thoroughly. This step ensures that no hidden issues arise from the newly applied changes. Ensure you have a robust testing framework in place to catch any potential errors as part of your regular workflow.
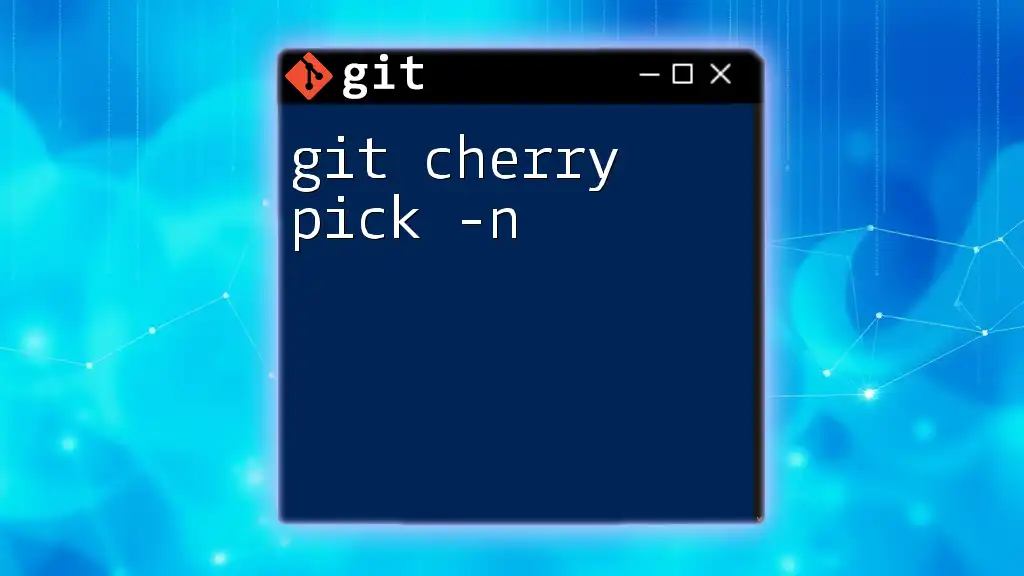
Conclusion
Recap of Key Points
To summarize, understanding how to handle git cherry-pick bad revisions is crucial for maintaining code quality. Identify bad revisions carefully, always back up your branches, and use the cherry-pick command judiciously.
Encouraging Safe Practices
The importance of safe practices cannot be overstated in the world of version control. Encourage your team to think critically about their commits and the implications of cherry-picking certain revisions. Maintaining a systematic and thoughtful approach will lead to cleaner and more manageable codebases.
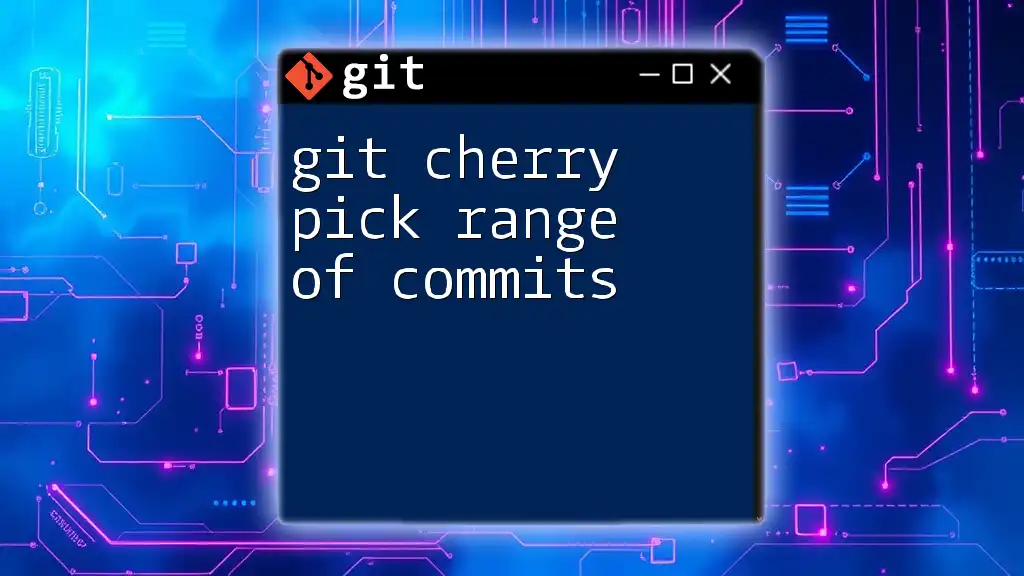
Additional Resources
Recommended Reading and Tools
For further learning and tools to enhance your Git workflow, consider exploring the following resources:
- The [official Git documentation](https://git-scm.com/doc)
- Tutorials on Git commands and workflows available online
- Tools like Git GUI clients for improved management of your repository