To cherry-pick multiple commits in Git, you can use the following command to apply the changes from specific commits to your current branch:
git cherry-pick commit1 commit2 commit3
Replace `commit1`, `commit2`, and `commit3` with the actual commit hashes you wish to cherry-pick.
Understanding Git Cherry Pick
What is Git Cherry Pick?
Git cherry-pick is a powerful command that allows you to select specific commits from one branch and apply them to another branch. This is particularly useful when you want to integrate changes from one context without merging an entire branch. By cherry-picking commits, you can maintain a clean project history while selectively bringing in features, bug fixes, and other changes that may be beneficial to another branch.
When to Use Cherry Pick
You may want to cherry-pick when:
- A commit contains a critical bug fix that needs to be applied to the production branch but isn't part of the current feature branch.
- You need to apply a change from a feature branch to another feature branch without merging the entire feature branch.
- You want to incorporate changes from a development branch into a release branch without altering the state of the development branch.
However, it’s essential to consider potential drawbacks, such as creating duplicate commits and complicating history if done excessively.
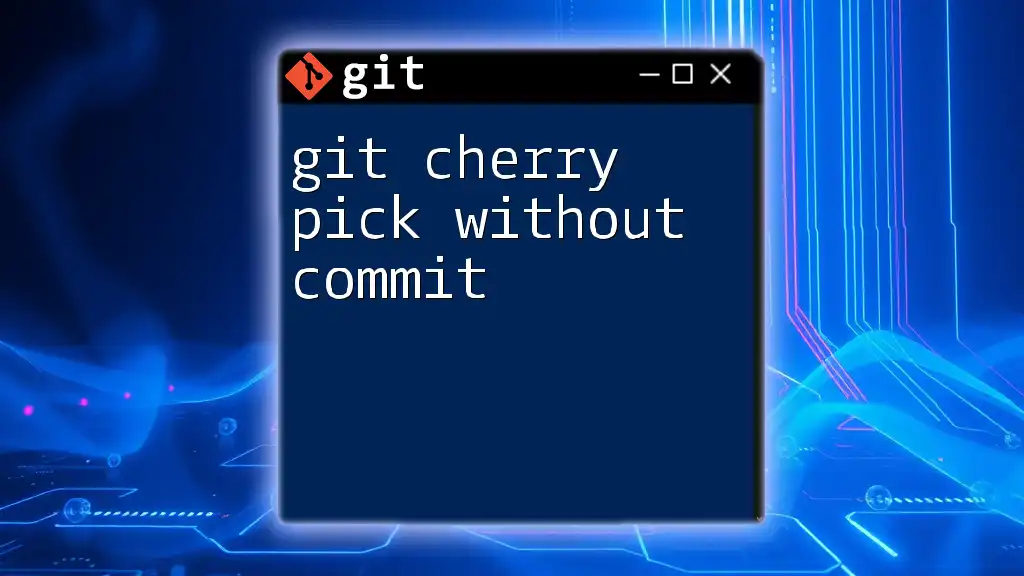
Understanding Commit References
What is a Commit Reference?
In Git, each commit is identified by a unique SHA-1 hash. A commit reference can be a full SHA, a shortened SHA, or human-readable concepts like `HEAD`, which refers to the current branch's latest commit. Understanding how to reference commits is crucial for effectively using cherry-pick.
How to Find Commit References
You can find commit references using commands such as:
- `git log`: This command will show you the commit history, allowing you to see the SHA hashes, commit messages, and respective authors.
- `git reflog`: This command provides a record of all your actions in the repository, including commits, branches, and resets. It's beneficial for recovering lost commits or identifying recent activity.
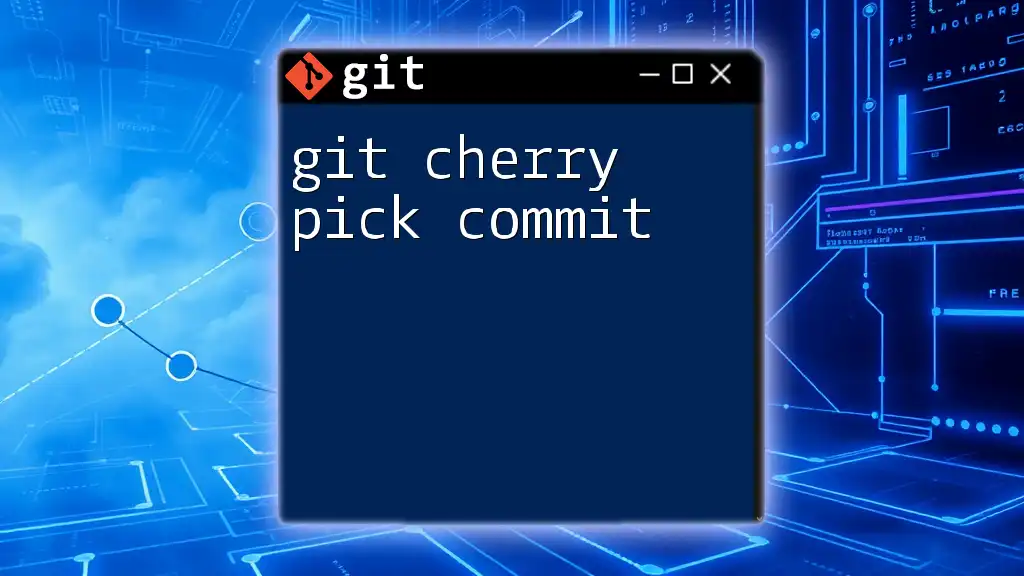
The Basics of Cherry Picking a Single Commit
Executing a Single Cherry Pick
The basic syntax for cherry-picking a single commit is as follows:
git cherry-pick <commit>
For example, if you want to cherry-pick a commit with hash `a1b2c3d`, you would run:
git cherry-pick a1b2c3d
This command applies the changes from the specified commit onto your current branch.
Handling Merge Conflicts
When cherry-picking, it’s possible to encounter merge conflicts. These arise when the changes in the selected commit contradict changes in your current branch. In this case, follow these steps:
- Identify the files with conflicts. Git will mark these in your working directory.
- Open the files and resolve the conflicts by choosing between changes or integrating them.
- After resolving conflicts, use:
git add <filename>
- Finally, complete the cherry-pick process by committing the changes:
git cherry-pick --continue
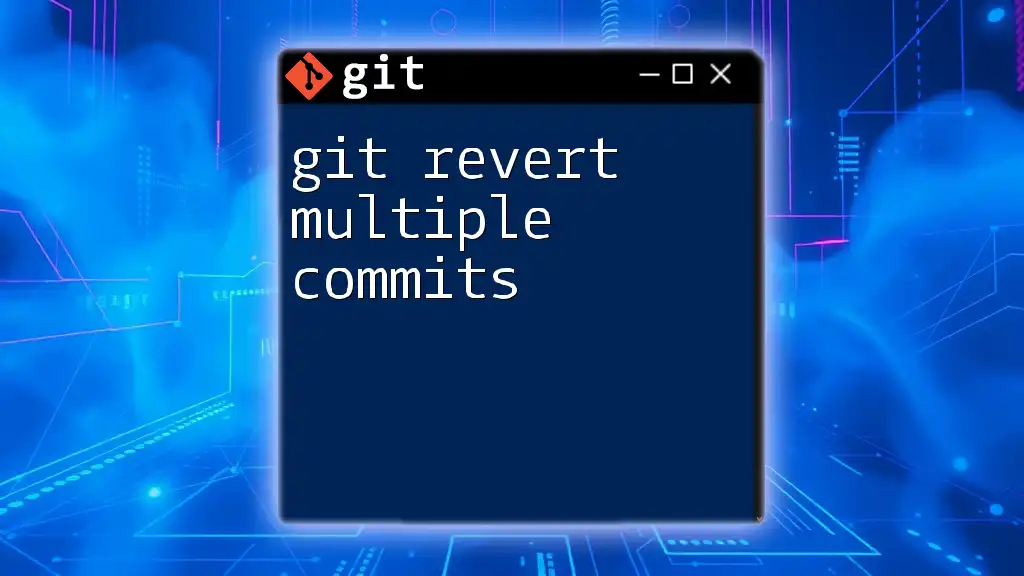
Cherry Picking Multiple Commits
How to Cherry Pick Multiple Commits
To cherry-pick multiple commits in one go, you can list them all in the command:
git cherry-pick <commit1> <commit2> ...
For example, to cherry-pick commits with hashes `a1b2c3d` and `e4f5g6h`, you would execute:
git cherry-pick a1b2c3d e4f5g6h
This command will sequentially apply each listed commit to the current branch.
Cherry Picking a Range of Commits
If you want to cherry-pick a range of commits, the syntax is slightly different:
git cherry-pick <start_commit>^..<end_commit>
Here, `start_commit` is the first commit to include, and `end_commit` is the last. For example:
git cherry-pick a1b2c3d^..e4f5g6h
This command applies all commits between the specified start and end commits, inclusive.
Cherry Picking with the `-n` Option
Sometimes, you might want to cherry-pick changes without immediately committing them. In this case, you can use the `-n` (or `--no-commit`) flag:
git cherry-pick -n a1b2c3d e4f5g6h
This option allows you to review and modify the changes before finalizing the commit, giving you greater control over your history.
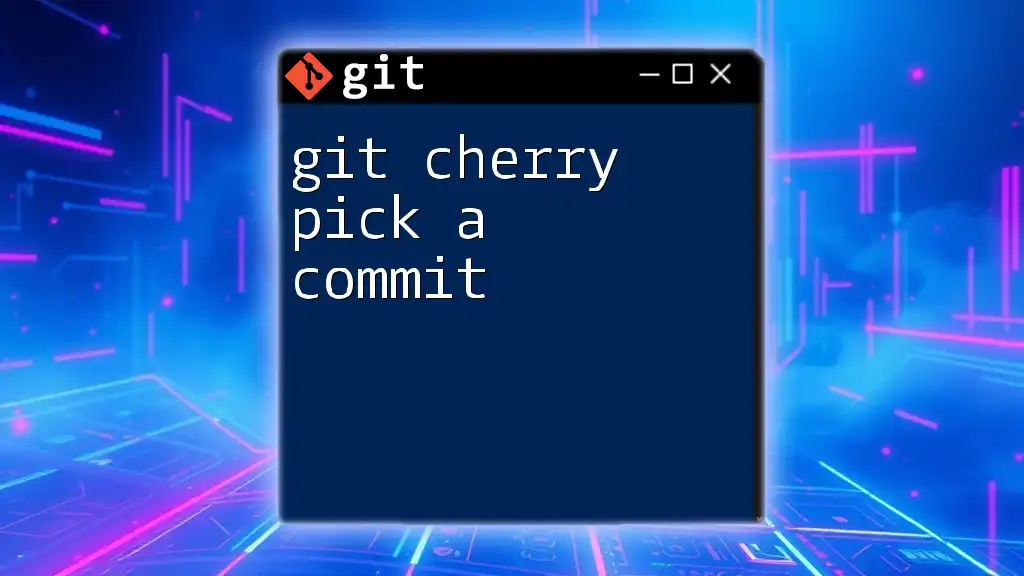
Best Practices for Cherry Picking
Commit Scope and Context
When deciding which commits to cherry-pick, consider the scope and context of each change. Ensure that the selected commits logically fit into your current branch's purpose and don't introduce unrelated changes. This practice helps maintain a clear and understandable project history.
Testing After Cherry Picking
After cherry-picking commits, thorough testing is crucial to ensure that the applied changes work as expected. Set up a testing framework, run automated tests, and manually check any critical paths affected by the new commits. This safeguard will help prevent introducing bugs or regressions into your codebase.
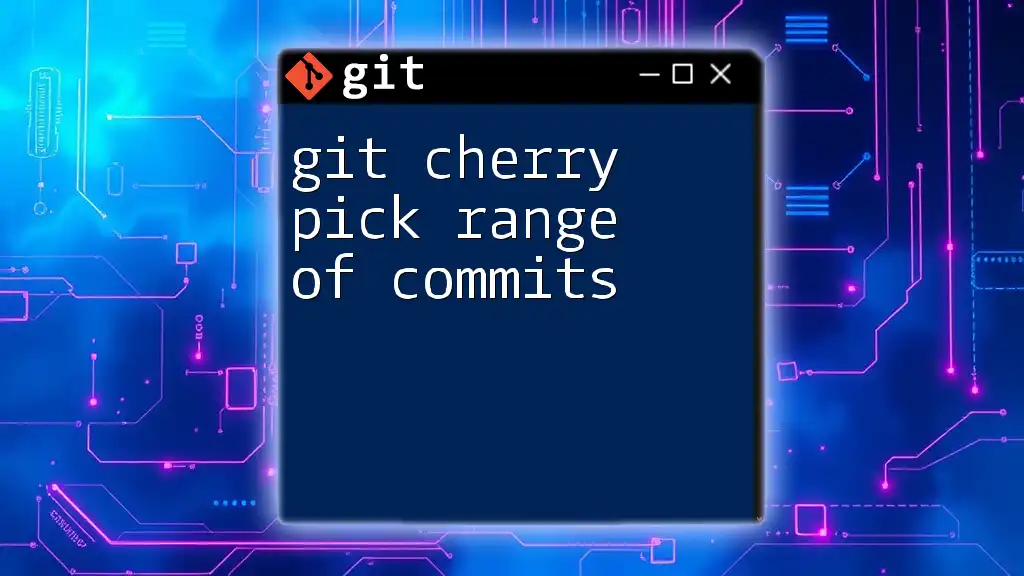
Troubleshooting Common Issues
Merge Conflicts Revisited
As previously mentioned, merge conflicts can occur during cherry-picking. Common causes include changes to the same lines of code in the original commit and your current branch. If you encounter a conflict, remember to resolve it carefully, maintaining the integrity of your code.
Cherry Pick Fails
Sometimes a cherry-pick may fail due to conflicts or other issues. If you find yourself in this situation, you can abort the cherry-pick process using:
git cherry-pick --abort
This command will revert any changes made during the cherry-pick, allowing you to start over.
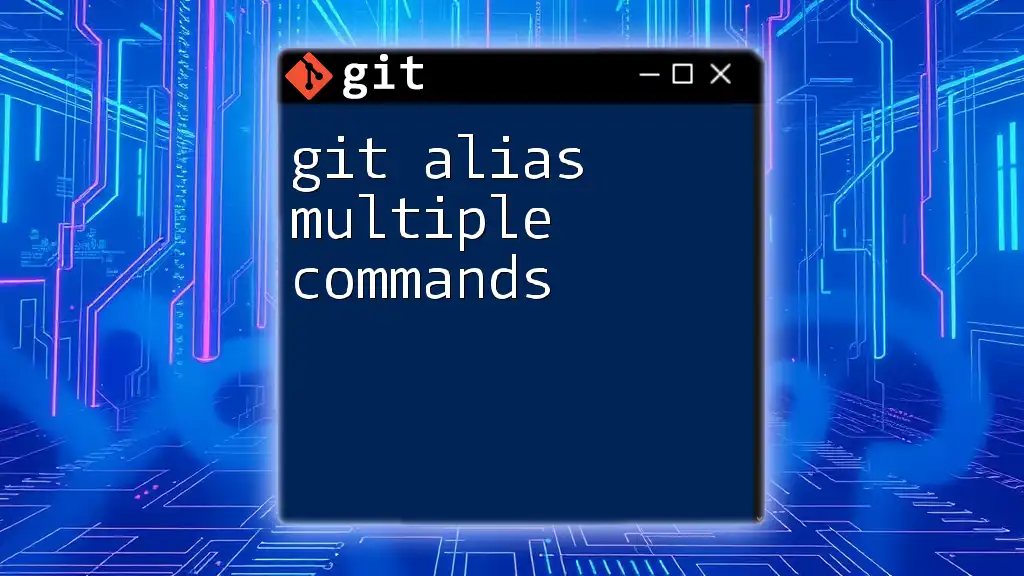
Alternatives to Cherry Picking
Rebasing vs. Cherry Picking
Cherry-picking is not the only way to bring in changes. Rebasing allows you to reap similar benefits by moving or transferring commits from one branch onto another. Consider using rebasing when you want to merge an entire set of changes while preserving commit history and relevance.
Creating a New Branch
If you frequently find yourself needing specific commits, consider creating a new branch for your feature or fix instead of cherry-picking scattered commits. This strategy helps maintain better organization, and you can merge back changes to the original branch when they are complete.
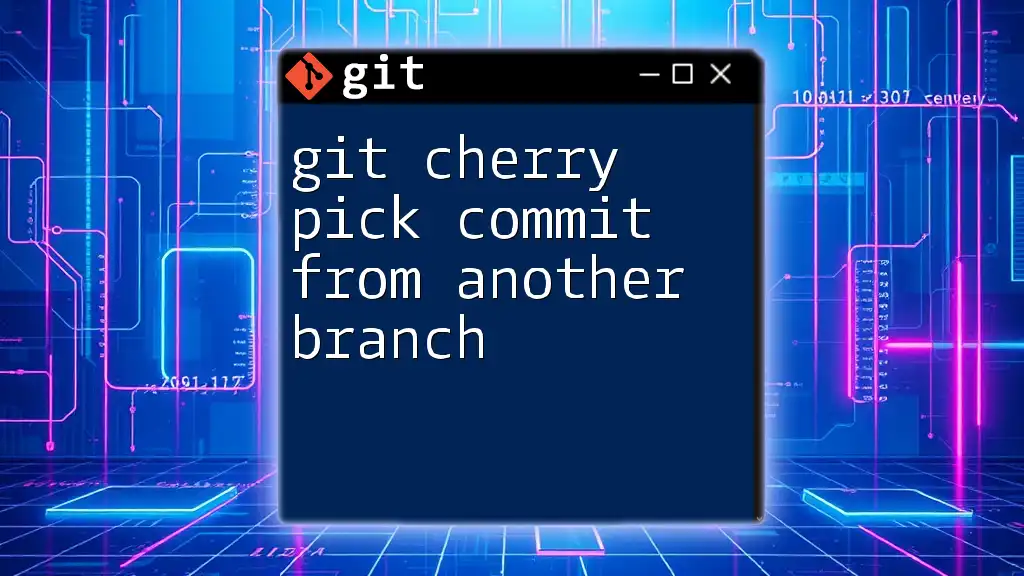
Conclusion
Throughout this article, we’ve explored various ways to effectively use git cherry pick multiple commits. By understanding the contexts in which cherry-picking is advantageous, mastering the syntax for single and multiple commits, and adhering to best practices, you can streamline your Git workflow and enhance collaboration on projects. Remember, with great power comes great responsibility—use cherry-picking judiciously to maintain a clean and logical commit history.