The `git cherry-pick` command allows you to apply the changes from a specific commit in another branch to your current branch.
git cherry-pick <commit-hash>
Introduction to `git cherry-pick`
What is `git cherry-pick`?
`git cherry-pick` is a powerful Git command that allows developers to apply the changes introduced by one or more existing commits from one branch into another branch. Instead of merging entire branches, which can introduce unwanted changes, cherry-picking enables a more selective approach, making it ideal for situations where you need specific bug fixes or features without the bulk of additional history.
Benefit of Using `git cherry-pick`
The flexibility provided by `git cherry-pick` is one of its most significant advantages. It allows developers to maintain cleaner branches by pulling in just what’s needed, which is especially useful in large projects with complex branching strategies. By cherry-picking, developers can effectively control their commit history, ensuring that only relevant changes are included in a particular codebase.

Understanding the Basics of Cherry Picking
How Git Works with Commits
To fully grasp the concepts behind cherry-picking, it's essential to understand how Git structures commits. Each commit in Git represents a snapshot of changes at a given point in time. Commits are organized in a directed acyclic graph (DAG), with each commit linked to its parent. This structure allows you to traverse through history, enabling commands such as cherry-pick to pinpoint and extract specific changes.
When to Use `git cherry-pick`
Using `git cherry-pick` is particularly beneficial in scenarios where you need to:
- Isolate a bug fix made in one branch and apply it to production without merging the entire set of changes.
- Craft a feature branch by selectively integrating specific commits from various branches. In some cases, cherry-picking can be a better option than merging or rebasing when you want to avoid the inclusion of extra commits and maintain a cleaner project history.
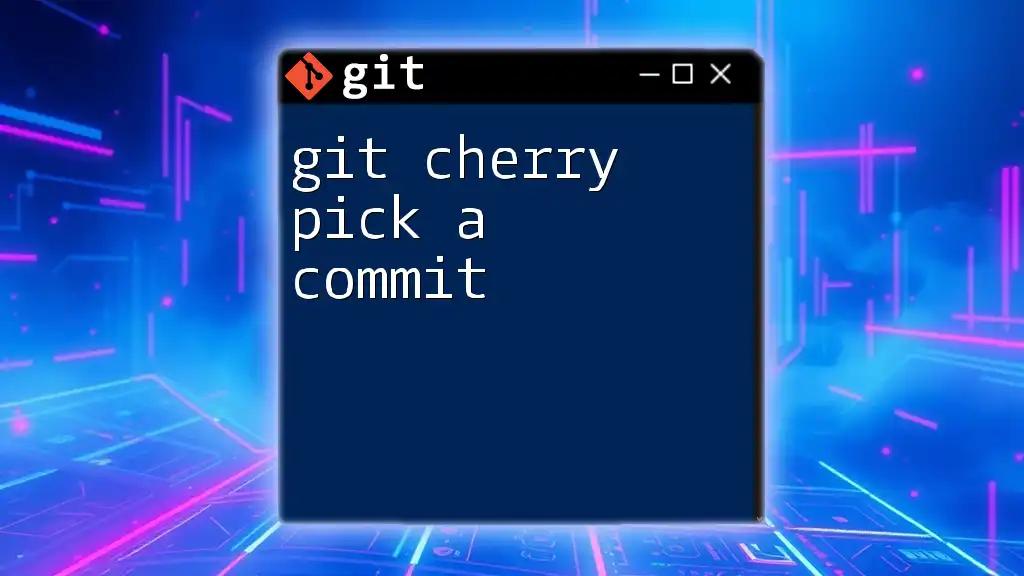
The Syntax of `git cherry-pick`
Basic Command Structure
The basic syntax of `git cherry-pick` is quite straightforward:
git cherry-pick <commit_hash>
Here, `<commit_hash>` refers to the unique hash identifier of the commit you want to cherry-pick.
Options and Flags
- `-n / --no-commit`
This option allows you to apply changes without creating a new commit immediately. It’s useful for further modifications before finalizing the commit:git cherry-pick -n <commit_hash>
- `-e / --edit`
This flag opens the commit message in an editor, allowing you to modify the default message associated with the commit you'd like to cherry-pick:git cherry-pick -e <commit_hash>
- `--continue`, `--abort`, `--quit`
These commands are useful when there are conflicts during cherry-picking. After resolving any conflicts:
Use `--abort` to cancel the cherry-pick and return to the state before the command was issued, or `--quit` to exit the cherry-pick process without changes.git cherry-pick --continue
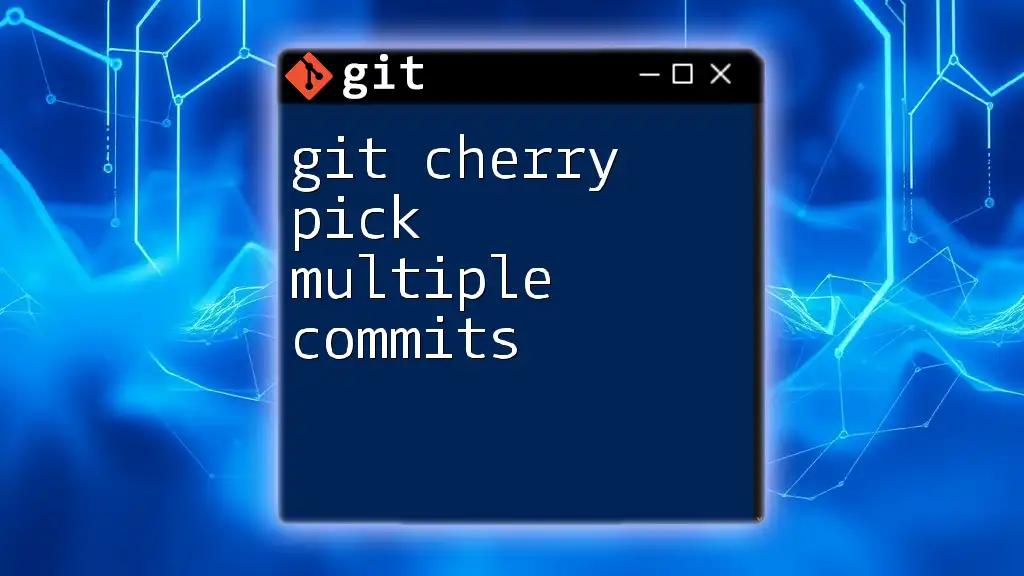
Step-by-Step Guide to Using `git cherry-pick`
Setting Up Your Git Environment
Before executing a cherry-pick, ensure that your working directory is clean. Check for staged or modified files. If necessary, create a new branch where you want to apply the changes:
git checkout -b new-feature-branch
Executing a Basic Cherry-Pick
To cherry-pick a specific commit from another branch, first, identify the commit hash you want to apply. For demonstration:
git checkout feature-branch
git cherry-pick abc1234
In this example, the `abc1234` commit from `feature-branch` is applied to the current branch.
Cherry-Picking Multiple Commits
To apply multiple commits at once, simply list their respective hashes:
git cherry-pick commit1 commit2 commit3
This command allows you to bring in several changes in one go, streamlining your workflow.
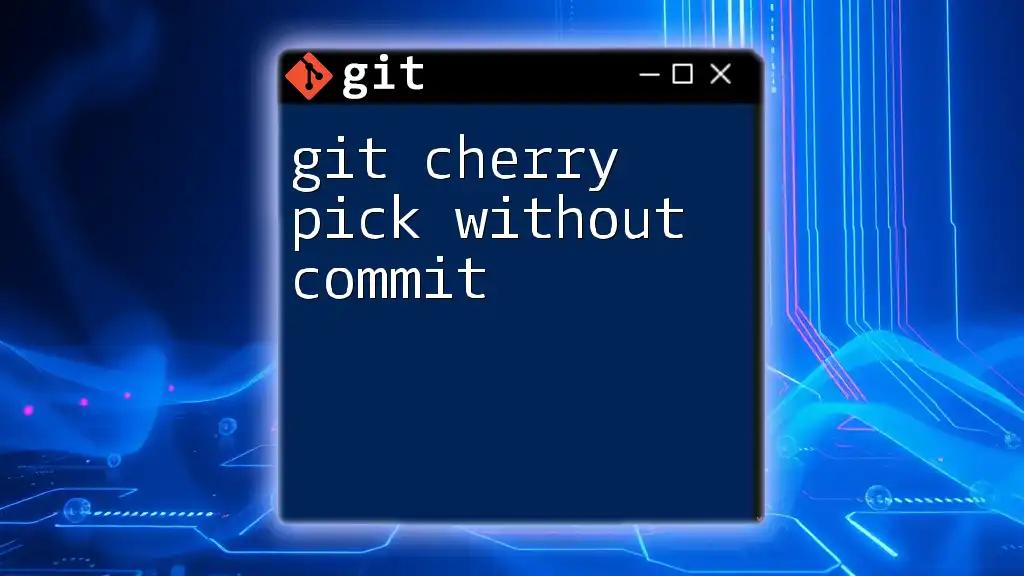
Handling Conflicts During Cherry-Pick
Understanding Merge Conflicts
Merge conflicts occur when changes in the cherry-picked commit overlap with changes in the target branch. Git cannot merge the differences automatically and requires user intervention. It’s crucial to carefully review the conflicting files and resolve them efficiently.
Resolving Conflicts
Once you encounter a conflict during cherry-picking, Git will pause the process and indicate the file(s) that need to be resolved. You can use:
git status
This command helps you identify which files are in conflict. Open these files, look for conflict markers (e.g., `<<<<<<<`, `=======`, and `>>>>>>>`), and manually adjust the code to resolve the issue. After making changes, stage the resolved files:
git add <resolved_file>
Finally, resume the cherry-pick process:
git cherry-pick --continue
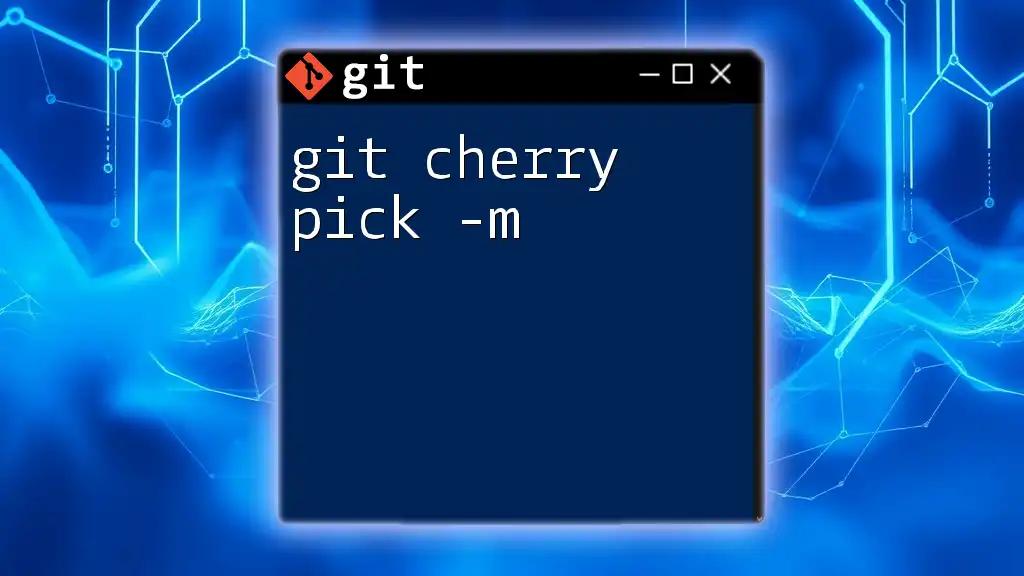
Best Practices for Cherry-Picking
When Not to Use `git cherry-pick`
Though powerful, `git cherry-pick` may not always be the best choice. Avoid using it if:
- You need to merge entire histories or branches, as `git merge` or `git rebase` may be more appropriate.
- You are cherry-picking frequently, given it can lead to inconsistencies or duplicated changes across branches.
Maintaining Commit History
Keeping your project history clean is essential. Whenever possible, maintain the original commit message when cherry-picking to ensure context is preserved. Consider documenting the reason for the cherry-pick in the commit message to enhance clarity for future developers.
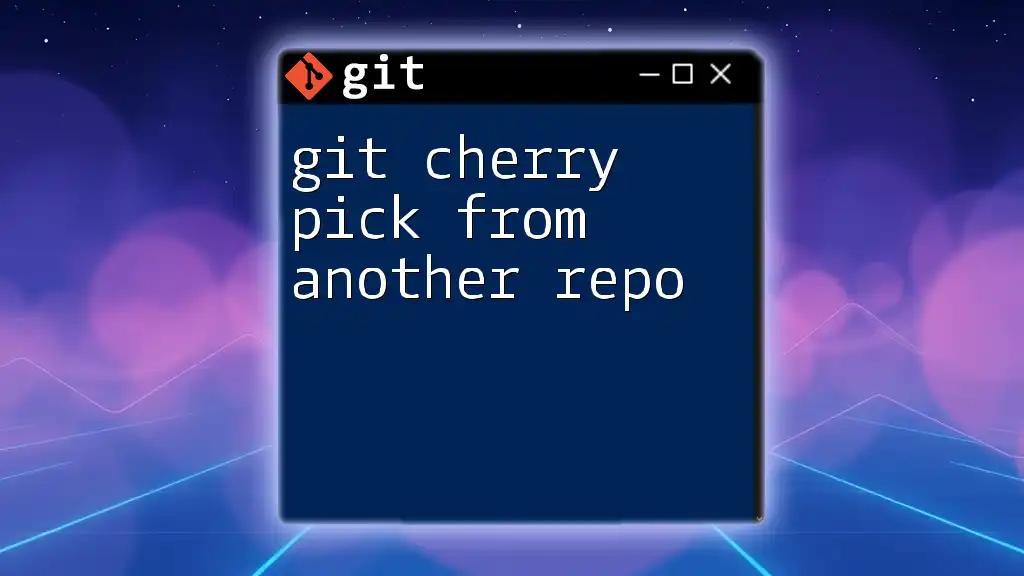
Real-World Example
Case Study: Fixing Bugs Across Branches
Suppose you have a `development` branch where a significant bug was fixed with a commit (let's say the commit hash is `def5678`). You want to apply this fix to the `production` branch. Here’s how to do it:
-
Checkout the Production Branch:
git checkout production
-
Cherry Pick the Commit:
git cherry-pick def5678
-
If Conflicts Arise, Resolve Them as Explained Above.
This process helps ensure that the bug fix gets into production without merging all the ongoing changes in the `development` branch.
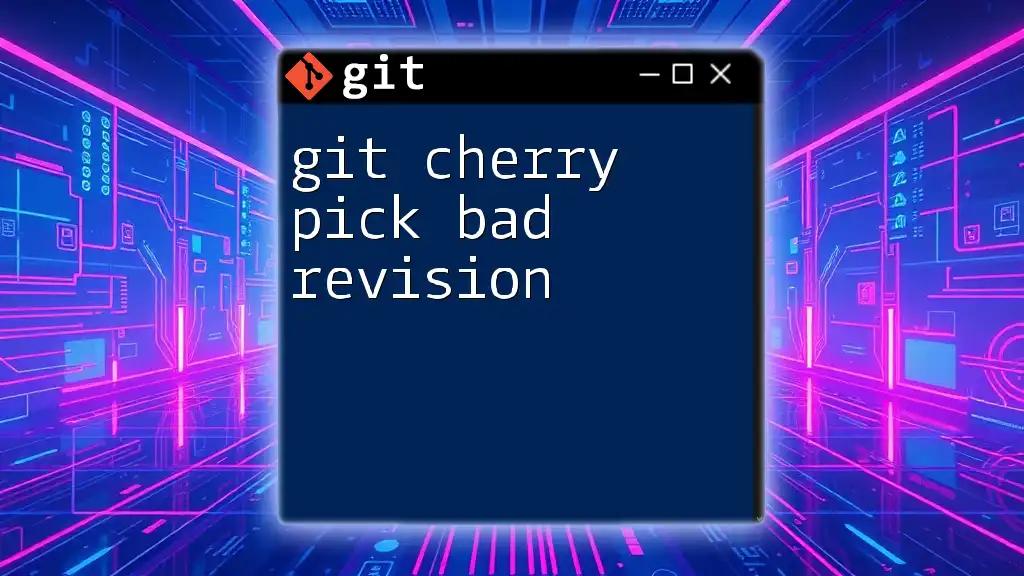
Conclusion
In conclusion, `git cherry-pick` is a valuable tool for developers looking to manage their code base's commit history effectively. By allowing you to select specific commits to apply to other branches, it provides flexibility and precision in collaboration. Understanding how and when to use this command, along with mastering conflict resolution, are key skills for any developer working with Git. Practicing cherry-picking will ensure you can maintain a clean working environment while leveraging the full power of version control.
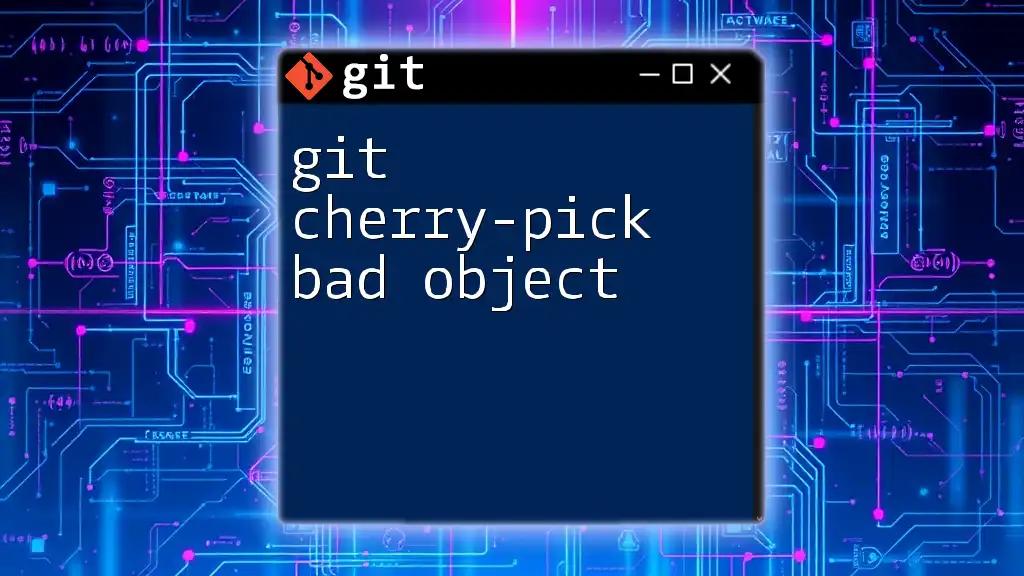
Additional Resources
For more in-depth information about Git commands and techniques, consider exploring the following resources:
- Official Git Documentation
- Recommended Books and Tutorials
- Community Forums and Support

FAQ Section
What is the difference between cherry-picking and merging?
Merging combines entire branch histories, applying all changes together, whereas cherry-picking allows for selective integration of individual commits.
Can `git cherry-pick` be undone?
Yes, you can undo a cherry-pick operation using `git cherry-pick --abort` if conflicts arise or by resetting the branch to the previous commit.
How does cherry-picking affect the project’s integrity?
While cherry-picking offers flexibility, overusing it can result in discrepancies in branch history. Maintaining clear commit messages and careful documentation is essential for project integrity.