Git cherry-pick is a command that allows you to apply a specific commit from one branch to another branch quickly.
git cherry-pick <commit-hash>
Understanding Git Cherry-Pick
What is Cherry-Picking?
Cherry-picking in Git is the process of selecting a specific commit from one branch and applying it to another. This command allows you to apply changes introduced by a commit without merging or rebasing the entire branch. It's often used to extract bug fixes or features that aren’t part of the current development focus or branch.
How Cherry-Picking Works
When you cherry-pick a commit, Git takes the changes from that specific commit and creates a new commit in your current branch with those modifications. This can help in situations where you might want to incorporate changes from a feature branch into your main branch without integrating every change made to that feature branch.
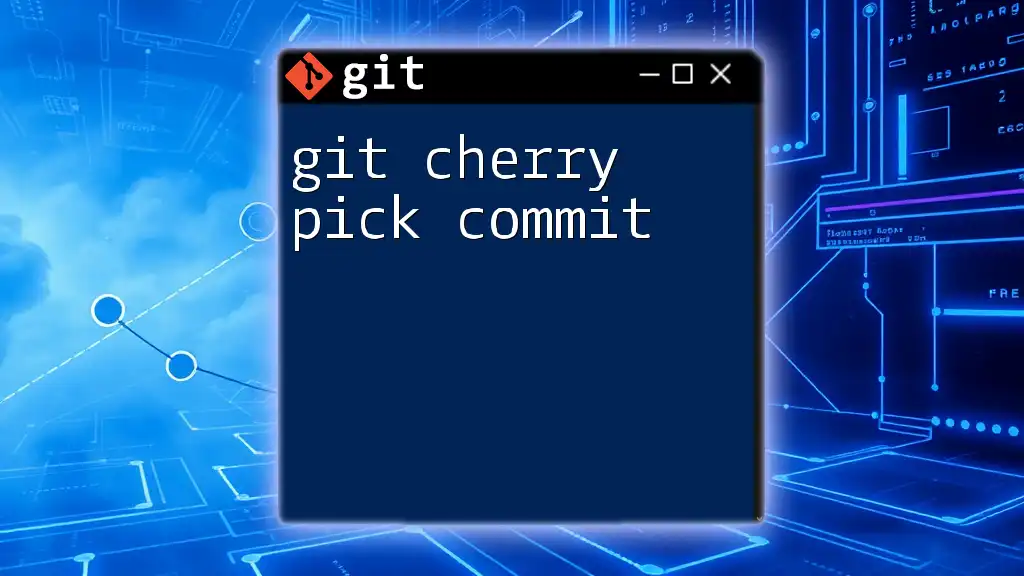
Prerequisites
Basic Git Knowledge
To effectively use `git cherry-pick`, it's vital to have a good understanding of basic Git commands and repository management. Familiarize yourself with commands like `git clone`, `git checkout`, and `git commit`, as well as understanding how branches and commits function within a Git repository.
Setting Up Your Environment
Before diving into cherry-picking, set up a sample Git repository to practice. Here’s how:
- Open your terminal and create a new directory for your project:
mkdir my-git-sample cd my-git-sample
- Initialize a new Git repository:
git init
- Create a sample file, make a commit, and create branches to experiment with cherry-picking:
touch sample.txt echo "Initial content" > sample.txt git add sample.txt git commit -m "Initial commit" git checkout -b feature-branch echo "Feature content" > feature.txt git add feature.txt git commit -m "Add feature"
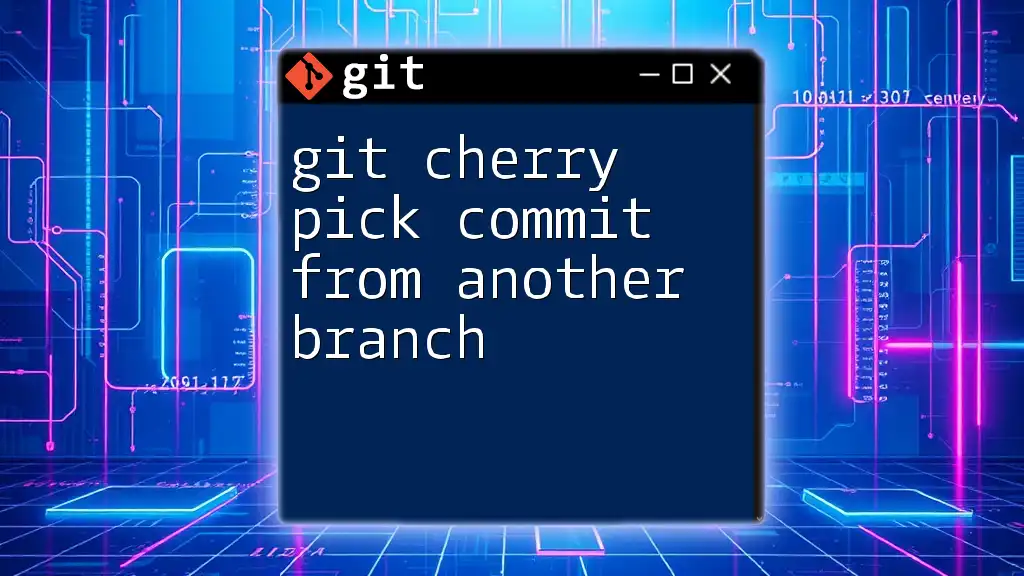
Cherry-Picking a Commit
The Basics of the Cherry-Pick Command
To use `git cherry-pick`, the syntax is straightforward:
git cherry-pick <commit-hash>
Where `<commit-hash>` is the identifier of the commit you want to cherry-pick. Understanding how to find this hash will be important, which you can do using:
git log
Step-by-Step Example
Creating a Commit to Cherry-Pick
Assuming you have two branches, one of which has additional features or fixes, you can create a commit in your `feature-branch` to cherry-pick later. Here’s how you can execute it:
-
Switch back to the main branch:
git checkout main
-
Use `git log` to find the commit hash of the commit you want from `feature-branch`:
git log feature-branch
-
Note the hash (e.g., `abcd1234`), and now cherry-pick that specific commit:
git cherry-pick abcd1234
This command applies the changes from the commit `abcd1234` directly to your `main` branch.
Executing the Cherry-Pick Command
When you run the cherry-pick command, Git will apply the changes introduced by that specific commit. You should see the output showing that the commit has been successfully applied in your current branch.
Dealing with Conflicts
What Happens During a Conflict?
If the changes you are cherry-picking conflict with changes already made in your current branch, Git will not automatically merge them. Instead, it will halt the process and inform you of the conflicts that must be resolved.
Resolving Conflicts
To resolve conflicts during a cherry-pick operation, follow these steps:
-
Check the status to see which files are conflicting:
git status
-
Open the conflicted files in your favorite text editor and manually resolve the conflicts by choosing which changes to keep.
-
Once resolved, mark the conflicts as resolved, and finalize the cherry-pick:
git add <resolved-file> git cherry-pick --continue
Git will then finalize the commit and continue the process.
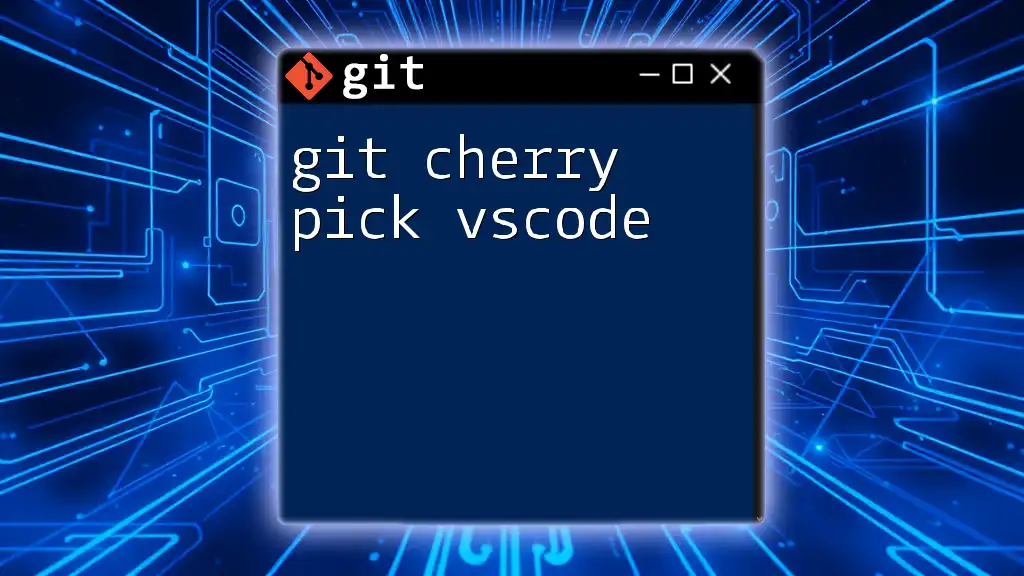
Advanced Cherry-Picking Techniques
Cherry-Picking Multiple Commits
To cherry-pick a range of commits, you can specify the commit range using two commit hashes:
git cherry-pick A..B
This command will cherry-pick all the commits from commit `A` to commit `B`, inclusive. It's crucial to check the order of commits to ensure you are selecting what you intend.
Using Cherry-Pick with Options
You can modify how cherry-picking behaves using certain flags:
- No commit option (`-n`): Use this flag if you want to apply the changes but not create a commit right away. This can be useful if you want to make further modifications.
git cherry-pick -n abcd1234
- With message option (`-x`): Including this option appends a note to your commit message indicating where the changes were cherry-picked from. This helps maintain a record of the source:
git cherry-pick -x abcd1234
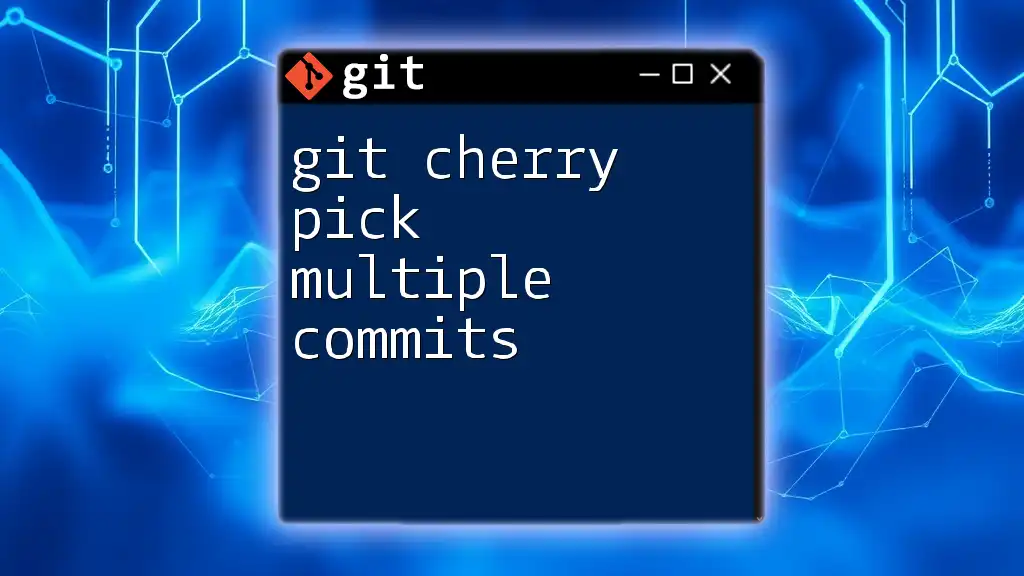
Best Practices for Cherry-Picking
When to Use Cherry-Pick
Cherry-picking is best used for specific, targeted changes you want to bring across branches without merging everything. It’s particularly useful for applying bug fixes or small updates from a development branch to a stable branch.
Alternatives to Cherry-Picking
While cherry-picking is a powerful tool, it’s essential to know when not to use it. In scenarios requiring the integration of multiple changes or when maintaining an up-to-date branch would be easier, consider using merging or rebasing as alternatives. Each of these methods comes with its pros and cons, so choose them based on the context of your changes.
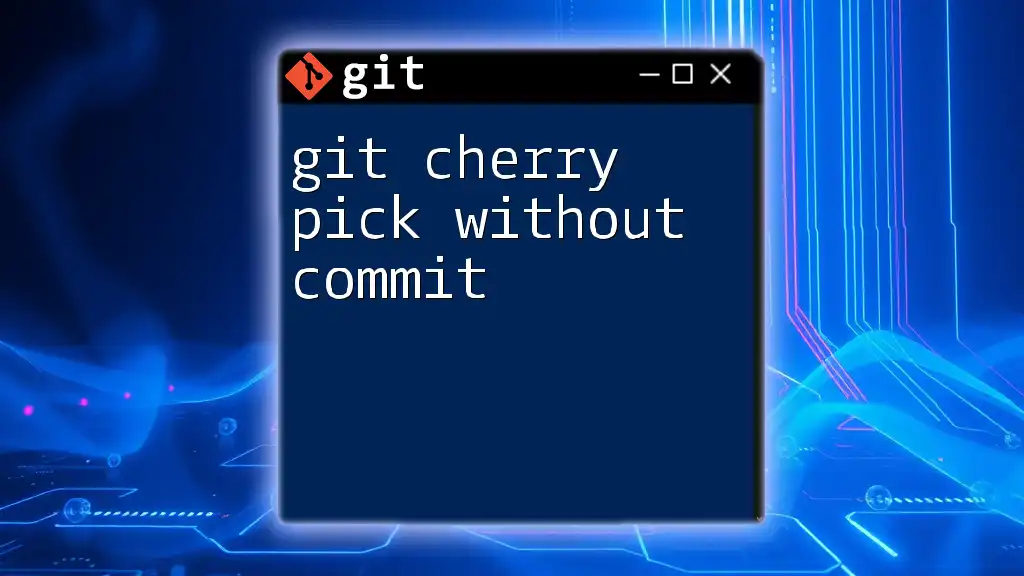
Conclusion
Mastering how to git cherry-pick a commit is crucial for efficient version control within your projects. Not only does it allow for targeted changes, but it also promotes better collaboration by giving teams the ability to isolate features, fixes, and updates.
As you practice and incorporate cherry-picking into your workflow, you’ll find it an indispensable skill in your Git toolkit.
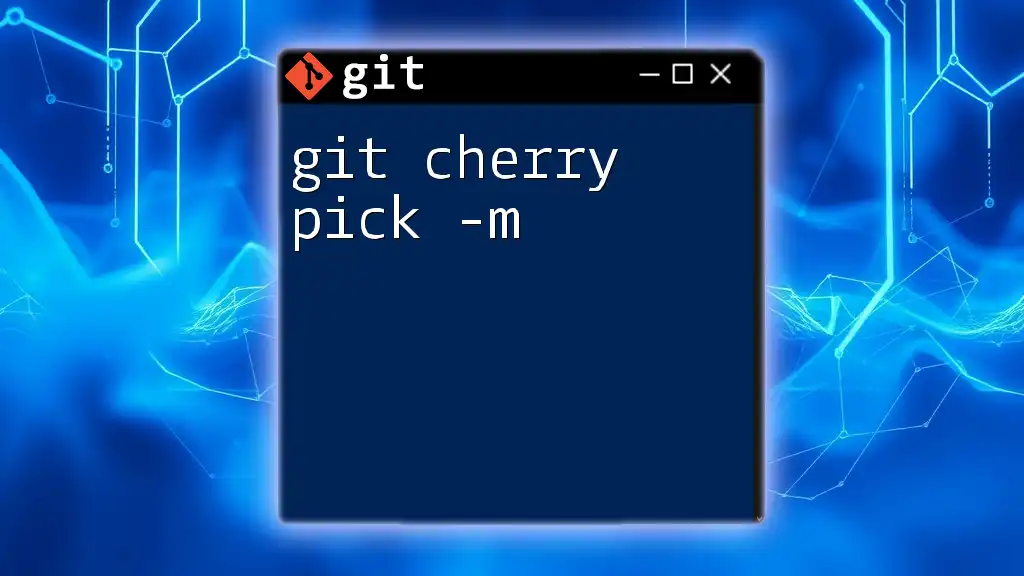
Additional Resources
To further enrich your understanding of cherry-picking and other Git commands, explore official Git documentation, tutorials, and interactive coding platforms. An active practice environment, such as GitHub, can greatly enhance your skills and confidence in using Git.
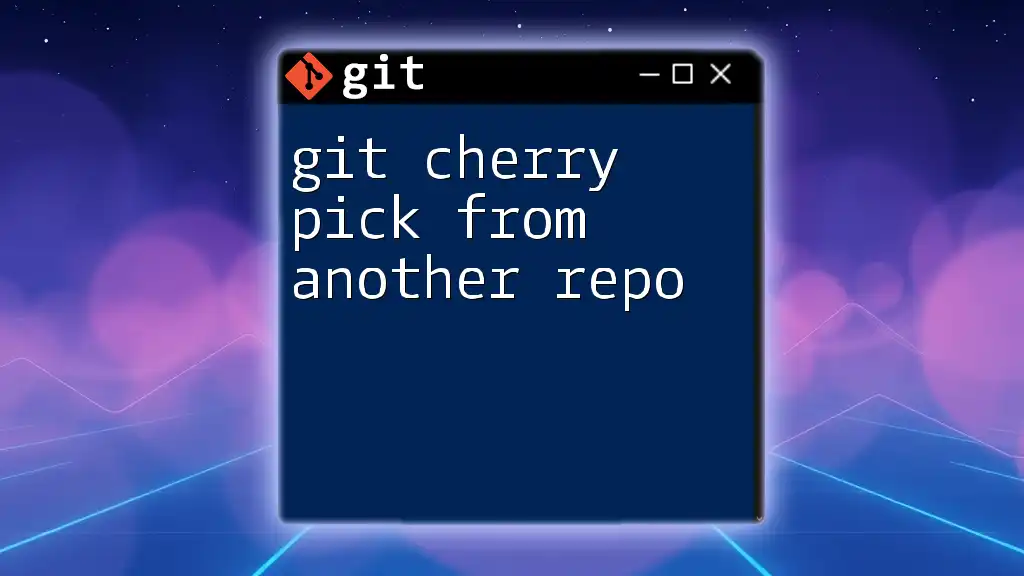
Call to Action
We encourage you to share your experiences with cherry-picking in the comments. What challenges have you faced? What victories have you celebrated? Join the conversation and subscribe for more concise Git tutorials and updates from our company!