To find out when a file was deleted in a Git repository, you can use the `git log` command with the `--diff-filter=D` option and specify the file name to see the commit history that included the deletion.
git log --diff-filter=D -- [filename]
Understanding Git's Tracking Mechanism
What is Git?
Git is a distributed version control system that allows developers to track changes in their source code during software development. It helps teams collaborate efficiently, manage multiple versions of a codebase, and recover previous versions if needed. By enabling more straightforward branching and merging, Git allows developers to experiment and innovate without the fear of losing their work.
How Git Tracks Changes
Git tracks changes through a model where every change is recorded in "commits." Each commit acts like a snapshot of your project at a specific point in time. Essentially, these snapshots capture the state of your entire project, making it easy to review past changes. Files may be classified as "tracked" or "untracked"; tracked files are being monitored for changes while untracked files are new additions not yet committed to the repository.
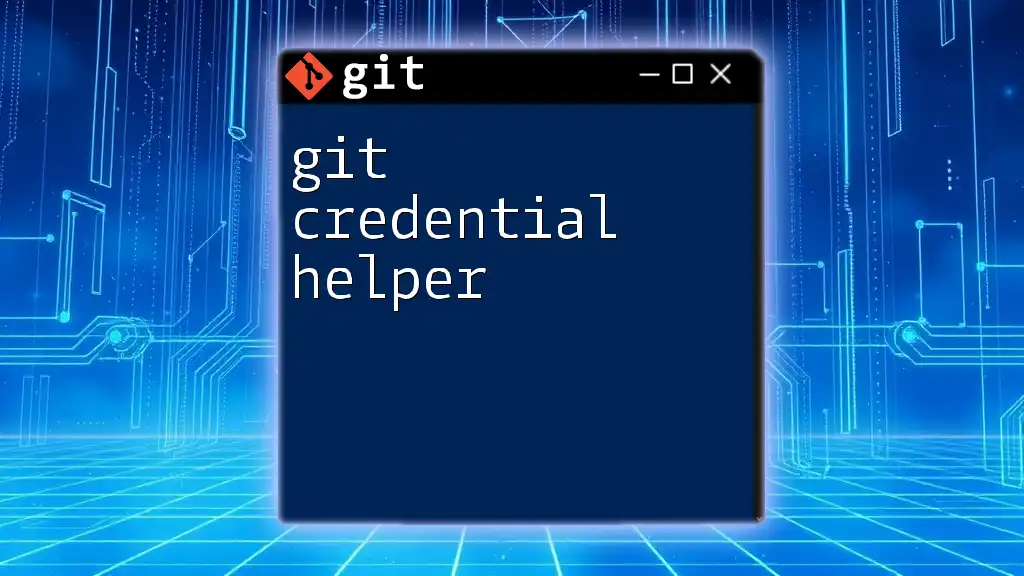
Identifying Deleted Files in Git
How Does Git Handle Deleted Files?
When files are deleted in Git, they aren't immediately removed from the history. Instead, the file is marked as deleted in the commits where those changes occurred. Git maintains a comprehensive history of all changes, including deletions, enabling you to review when and where a file was removed.
Finding Deleted Files
Using `git log` Command
To find out when a file was deleted, you can leverage the `git log` command with the path to that specific file. This command will show you the commit history related to the file, including any deletions.
Command Syntax:
git log -- <file-path>
Example:
git log -- path/to/your/file.txt
This command will return a list of commits that affected the specified file, including the one indicating its deletion. Each entry will show the commit hash, author, date, and commit message, allowing you to determine when and perhaps why the file was removed.
Using `git rev-list` and `git grep`
To pinpoint the last commit that affected a file, you can use `git rev-list` along with `git grep`. This approach gives you a more focused look into all revisions of the file.
Command Syntax:
git rev-list -n 1 --all -- <file-path>
Example:
git rev-list -n 1 --all -- path/to/your/file.txt
The command returns the commit hash of the last modification, letting you delve deeper into the commit history.
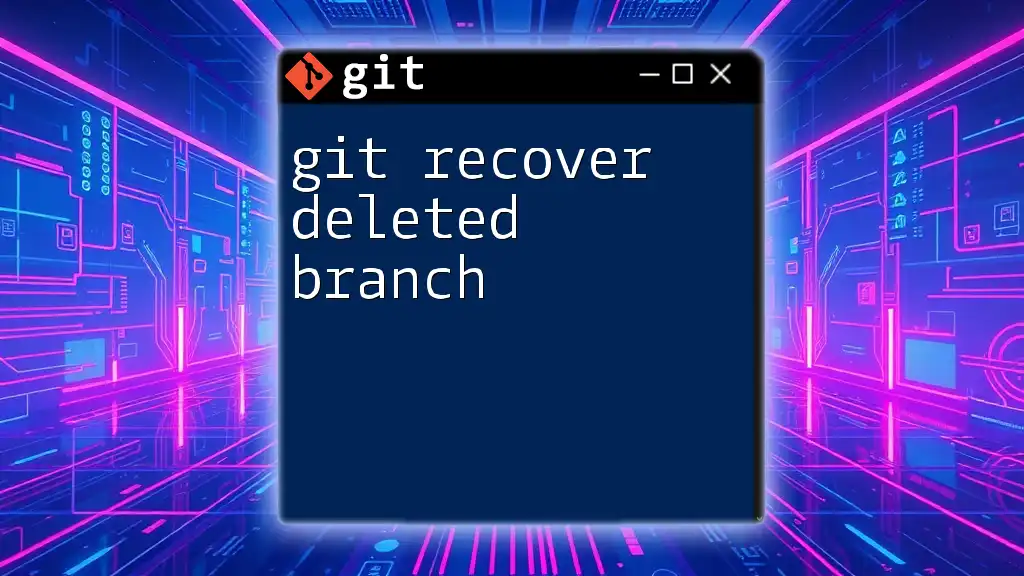
Locating the Exact Commit of Deletion
Using `git blame`
The `git blame` command provides a way to investigate line-by-line changes in a file, revealing who last modified each line. By employing specific flags, you can also track moves and copies.
Command Syntax:
git blame -C -C -M <file-path>
Example:
git blame -C -C -M path/to/your/file.txt
This command will list the commits associated with each line of the file, giving you insight into when and why lines were altered or the file was deleted, if applicable.
Using `git diff`
To gain insights into changes made between two commits, including deletions, you can utilize the `git diff` command. This command compares the state of a file in different commits to show what exactly has changed.
Command Syntax:
git diff <commit-id>^ <commit-id> -- <file-path>
Example:
git diff a1b2c3d^ a1b2c3d -- path/to/your/file.txt
In this example, replace `a1b2c3d` with the actual commit ID where the file was believed to be deleted. The output will highlight changes, including deletions, between the two commits.
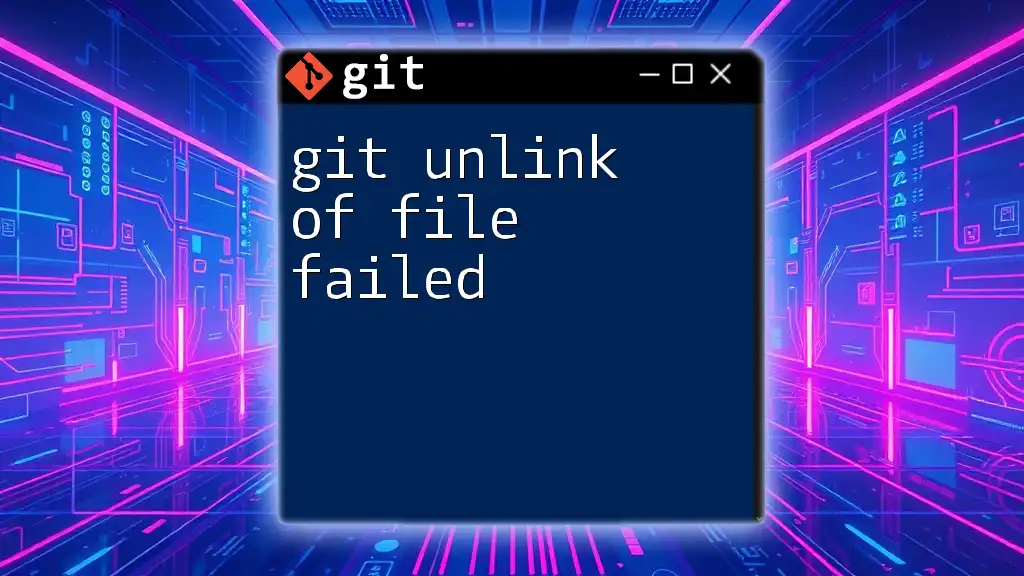
Viewing the Commit History
Using Git Log with Options
Sometimes, you may want to view a comprehensive history of a file, including all modifications. You can achieve this by expanding your `git log` query with specific options.
Command Syntax:
git log --all --full-history -- <file-path>
Example:
git log --all --full-history -- path/to/your/file.txt
This command provides a full history of the specified file, detailing all commits, including deletions. It allows you to trace back through time to understand the evolution of your file.
Checking the History of Deletion
Interpreting the output of the `git log` and other commands for deletions will help you grasp the context surrounding the file's removal. Focus on the commit messages; often, developers will annotate their changes with explanations that can clarify the rationale for deleting a file.
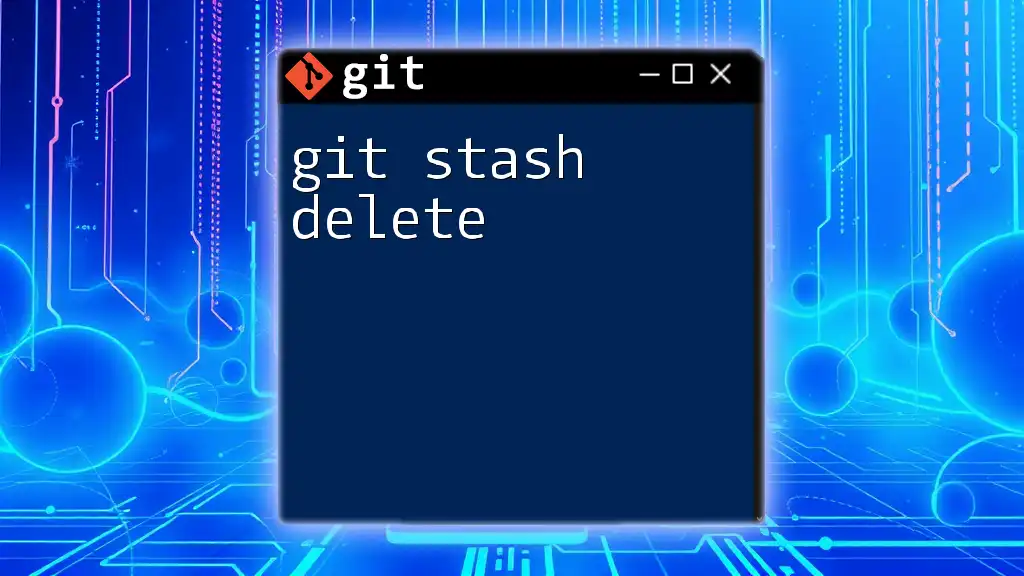
Recovering Deleted Files
Restoring a Deleted File
If you determine that a deletion was a mistake, Git allows for easy recovery of deleted files from prior commits. You can effectively restore a deleted file using the `git checkout` command.
Command Syntax:
git checkout <commit-id>^ -- <file-path>
Example:
git checkout a1b2c3d^ -- path/to/your/file.txt
This command restores the file from the commit prior to its deletion. It's crucial to understand that after recovery, the file will revert to its state at that version, and any changes made thereafter will be lost unless saved separately.
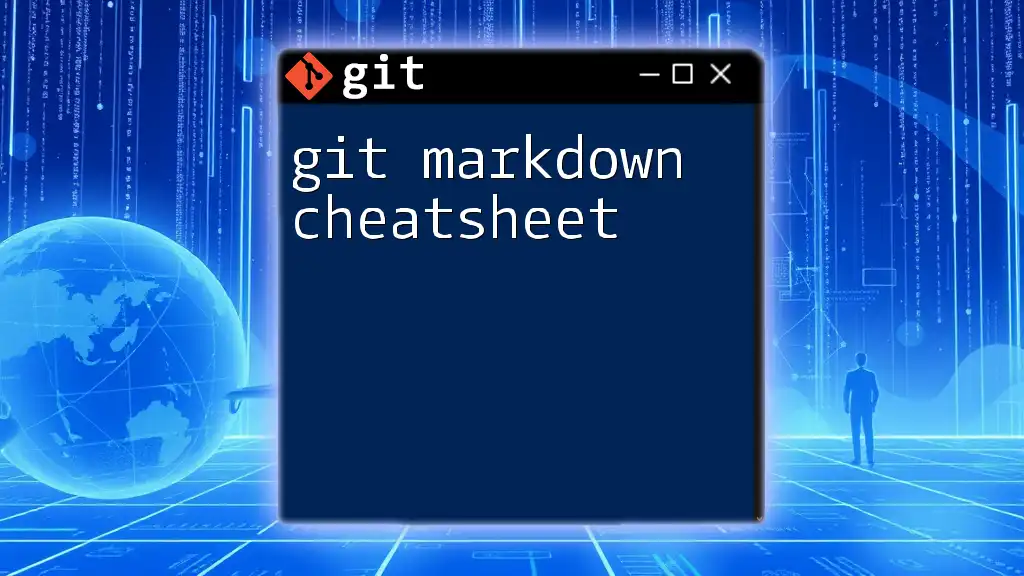
Best Practices for File Management in Git
Keeping Track of Changes
When working with Git, meaningful commit messages play a vital role in understanding the history. Clearly document your reasons for changes, especially deletions. Utilizing branching can also keep your main developments separate, allowing for safer experimentation.
Regular Backups and Syncing
To avoid issues with missed changes, regularly synchronize your local repository with the remote one by using `git pull` and `git push`. Commit often to maintain a detailed project history that reflects your development process.
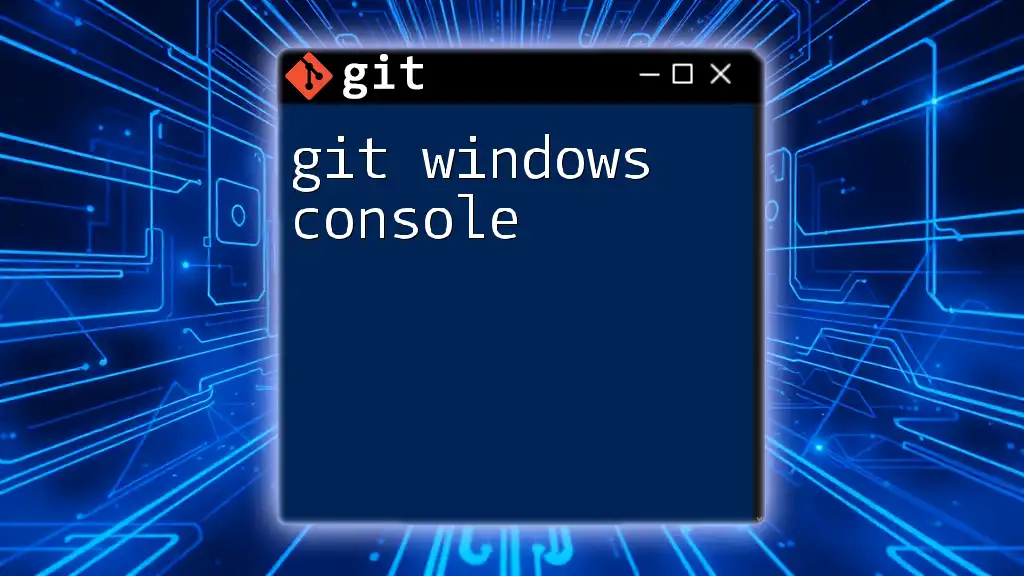
Conclusion
Knowing how to effectively use Git commands to trace the history of removed files is invaluable for any developer. Understanding when a file was deleted and the context surrounding its removal allows you to maintain a clear and focused version history. Familiarity with commands like `git log`, `git blame`, and others will empower you to utilize Git adeptly, ensuring you keep your projects organized and recoverable.
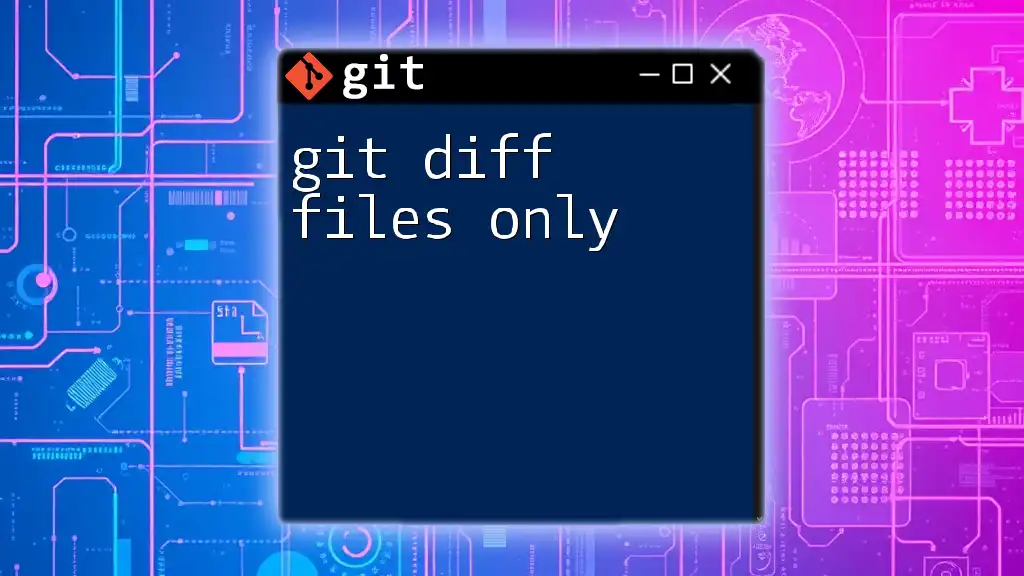
Call to Action
I encourage you to explore more about Git commands and share your experiences. Keep an eye on future tutorials where we delve into more concise and effective methods of managing your source code using Git!