To recover a deleted Git branch, you can use the `git reflog` command to find the commit where the branch was last pointed and then create a new branch from that commit using `git checkout -b`.
git reflog
git checkout -b <branch-name> <commit-hash>
Understanding Git Branches
What is a Git Branch?
A Git branch serves as a pointer to one of the commits in your project's history, allowing you to develop features, fix bugs, or experiment in isolation without affecting the main codebase. Branches are essential for maintaining different lines of development, making concurrent work possible in collaborative environments.
Why Branches Can Be Deleted
Branches can be deleted as a part of standard workflow processes. Common scenarios include:
- Merging: When a feature branch is merged into the main branch, it can be deleted to keep the repository clean.
- Clean-Up: Outdated or unused branches are often deleted during routine repository maintenance.
Accidental deletions also occur, often due to command misuse or changes in project direction. Understanding how to recover deleted branches becomes crucial for maintaining workflow continuity.
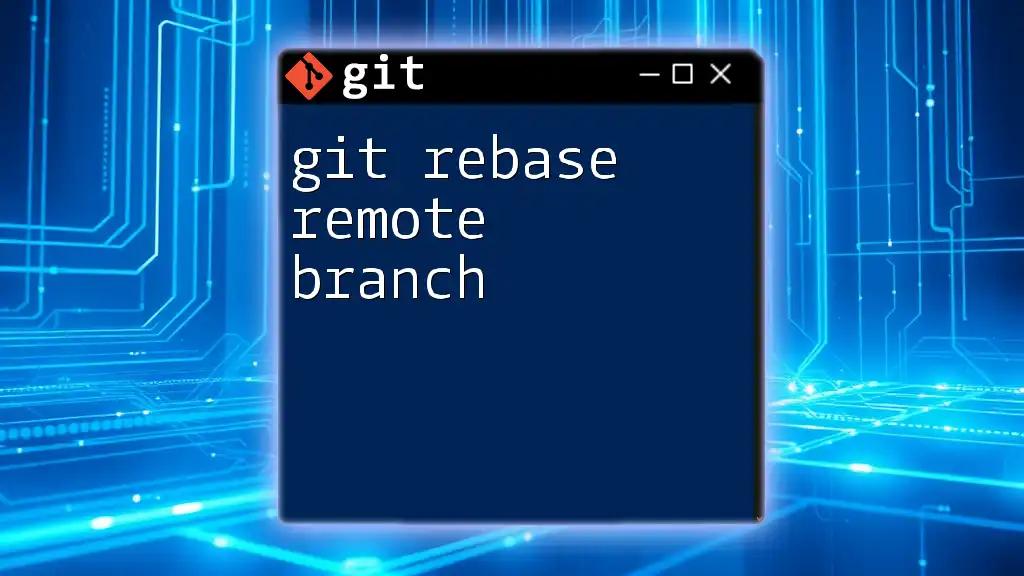
The Importance of Recovery
Why You Might Need to Recover a Deleted Branch
There are several scenarios where you might find yourself needing to recover a deleted branch:
- Lost Work: If you accidentally delete a branch that contains important changes, recovery can prevent loss of significant efforts.
- Errors: Sometimes, changes might not go as planned, causing a need to revert to previous states.
- Collaboration: Teams often rely on branches for different features. Losing one can disrupt the workflow among team members.
In collaborative environments, understanding how to execute `git recover deleted branch` is paramount in preventing disruptions and ensuring that valuable work is not permanently lost.
Consequences of Deleting a Branch
Deleting a branch is not just about removing references; such actions can affect project history significantly. A deleted branch might mean losing access to unique development paths, possibly leading to confusion within the team. Immediate recovery reduces the risk of data loss and allows your team to recover quickly from such events.
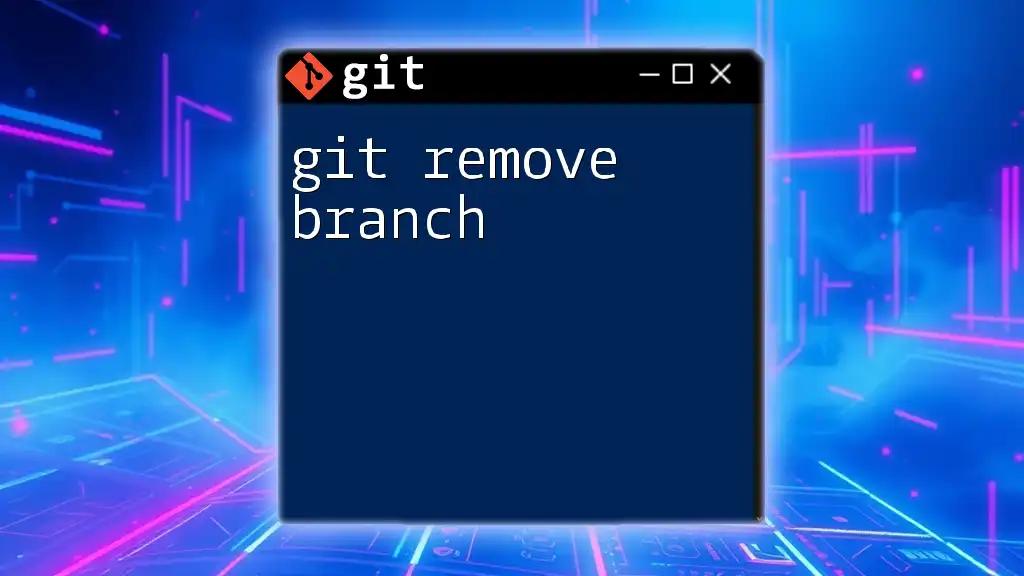
How Git Stores Branches
Understanding Refs and Commits
In Git, branches are stored as pointers (or references) to commits. When you create a branch, Git assigns a reference to the latest commit on that branch. This structure allows you to traverse your project’s history effectively. Understanding this helps grasp how deletions work and the potential for recovery.
The Reflog: Your Best Friend in Recovery
The reflog is a powerful feature in Git that keeps track of changes made to your branches, even when they are deleted. Every time you move your HEAD (for instance, through checkouts or commits), Git records this action in the reflog. To view the reflog, use the following command:
git reflog
This command will output a list of actions taken within the repository, showing a history that includes deleted branches, making it an invaluable tool for recovery.
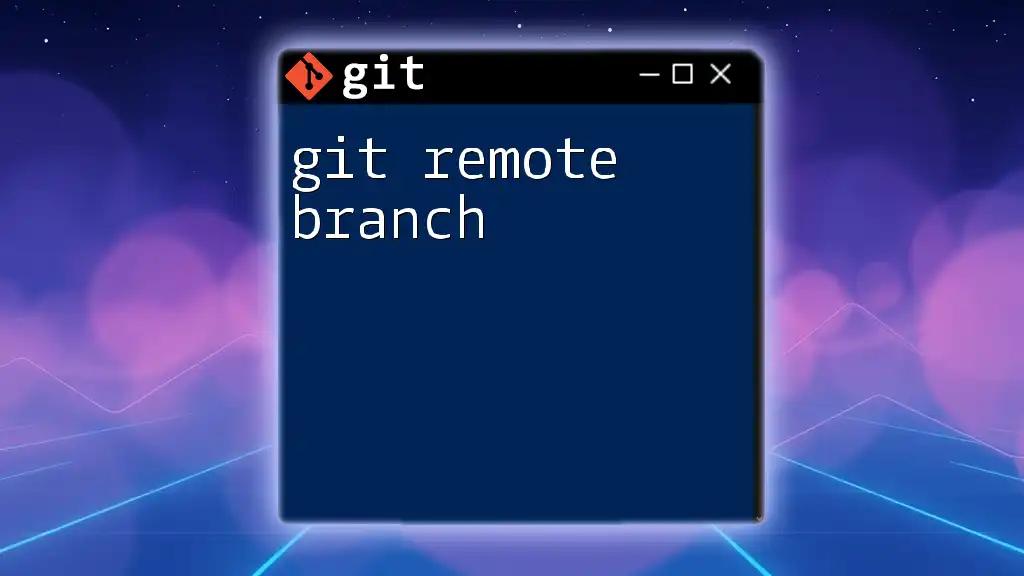
Steps to Recover a Deleted Branch
Checking the Reflog
To begin your recovery process, first check the reflog. You might see an output similar to:
abc1234 HEAD@{0}: checkout: moving from feature-branch to main
abc2345 HEAD@{1}: commit: Completed changes on feature-branch
abc3456 HEAD@{2}: checkout: moving from main to feature-branch
Each entry consists of a commit reference followed by a description of actions taken. Look for the entry that corresponds to the last commit made on the deleted branch.
Interpreting Reflog Output
Once you identify the commit corresponding to the deleted branch, note the commit hash (e.g., `abc2345`). This is crucial for recreating the branch. If unsure which entry corresponds to the deleted branch, look for recent checkout or commit actions related to it.
Creating a New Branch from the Lost Commit
Now that you have the commit hash, you can restore the deleted branch. Use the following command:
git checkout -b <branch-name> <commit-hash>
Replace `<branch-name>` with the name you want for your restored branch and `<commit-hash>` with the hash obtained from the reflog. For example:
git checkout -b feature-branch abc2345
This command creates a new branch that starts at the commit where your deleted branch last pointed.
Verifying Recovery
To confirm that your branch has been successfully restored, list your branches with:
git branch
You should see your newly recreated branch among the list. Double-check the branch's state by inspecting its recent commits:
git log --oneline
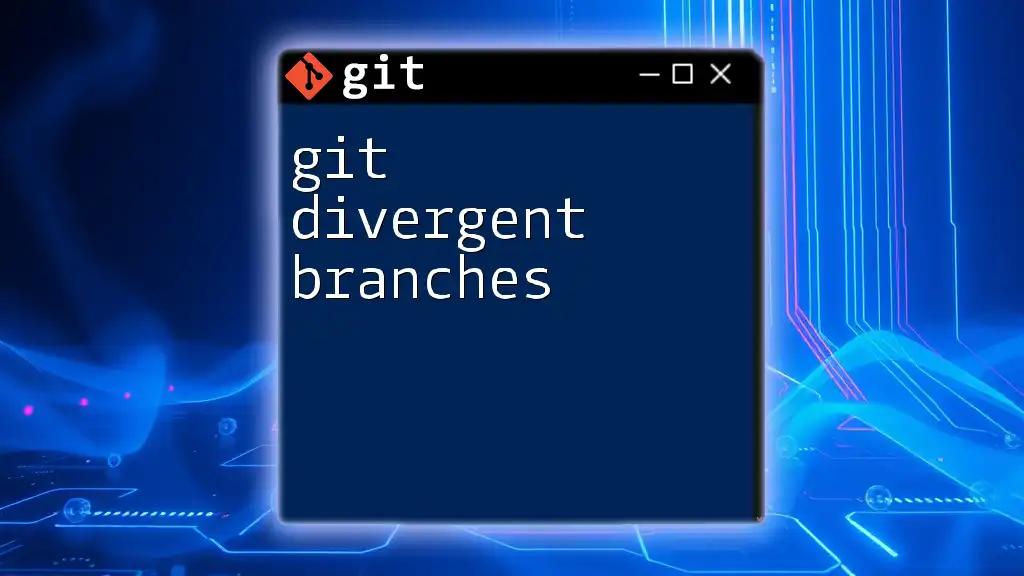
Alternative Recovery Methods
Recovering from a Remote Repository
If your deleted branch was pushed to a remote repository, it may still exist. In such cases, you can retrieve the branch using:
git fetch origin
git checkout <deleted-branch-name>
This will recreate the branch based on the latest push from the remote repository, allowing you to recover work that may have been unintentionally lost.
Using `git fsck`
If reflog is unavailable, you can utilize `git fsck` to discover dangling commits (unreachable commits). Running this command allows you to view objects and references still present in your Git directory, which may include the lost commit. Simply execute:
git fsck --full
Inspect the output for any dangling commits and note their hashes for potential recovery.
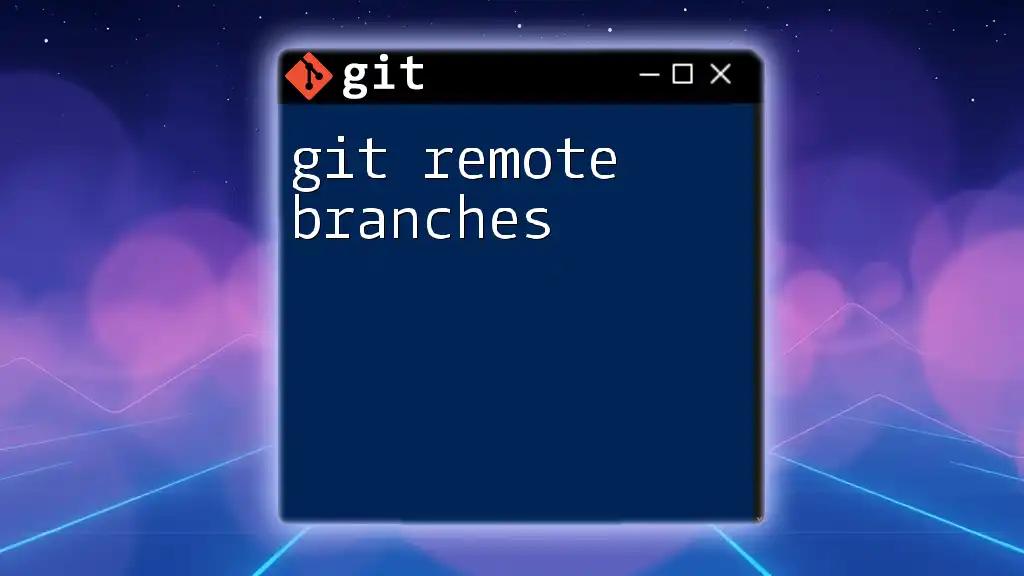
Best Practices to Avoid Deleting Important Branches
Naming Conventions
Use clear and descriptive names for branches to minimize confusion. An effective naming strategy, such as using feature prefixes or task IDs, can significantly reduce the chances of accidental deletions.
Regular Backups
Consider implementing regular backups for your Git repositories. You can use tools like Git hooks to automate snapshots or employ third-party solutions to back up your entire codebase periodically.
Team Communication
Fostering a culture of communication within your team can prevent accidental deletions. Establish practices that encourage team members to inform one another about branch deletions and merges, enhancing collaborative workflow.
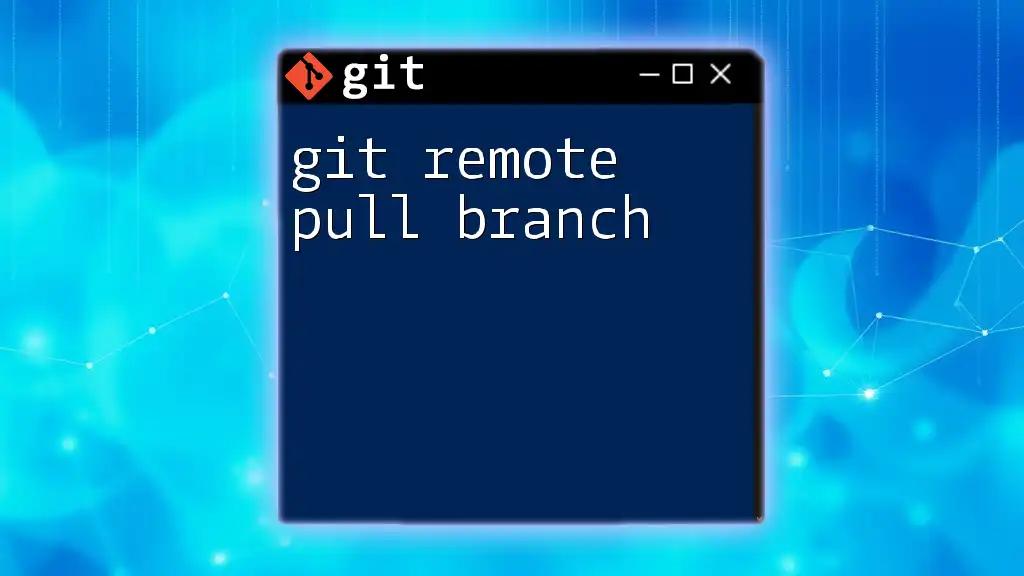
Conclusion
Recovering a deleted branch with Git is a critical skill in the life of a developer. By understanding the mechanics of branch management, leveraging the reflog, and following best practices, you can ensure your workflow remains productive even in the face of errors. Practice these techniques within a controlled environment to gain confidence in utilizing Git commands effectively, ensuring you are equipped to handle branch recovery when necessary.
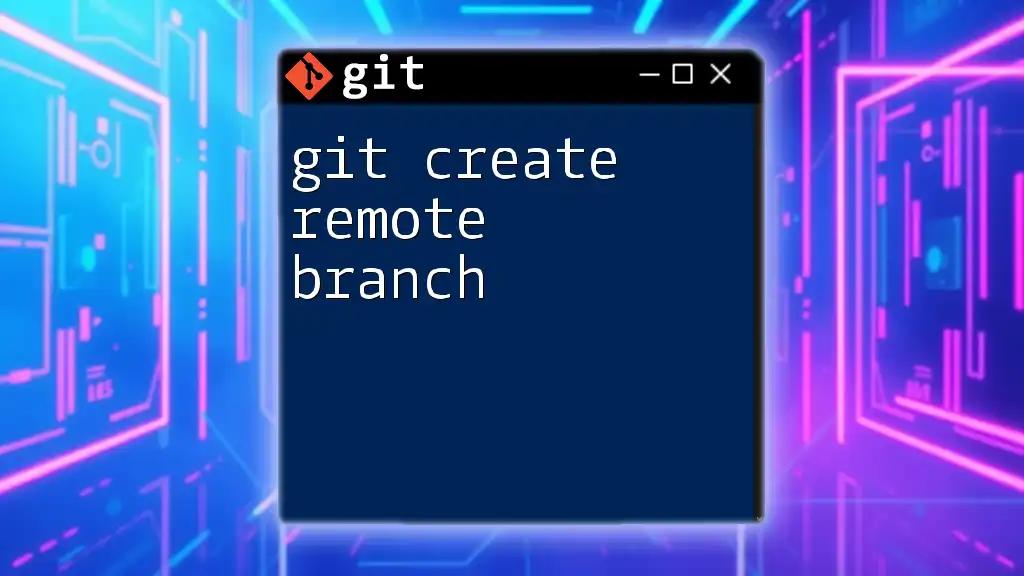
Frequently Asked Questions
Common Issues when Recovering Deleted Branches
What if the reflog is not available? If the reflog is unavailable due to repository settings or recent garbage collection, recovery may become increasingly challenging. Consider other methods, such as `git fsck`, or look for backups if you have them.
Additional Resources
For a deeper understanding of Git's architecture and commands, refer to the official Git documentation or explore community resources and tutorials. Staying informed will strengthen your grasp of version control and empower your development journey.