To view the commit history of a deleted file in Git, you can use the `git log` command with the `--` option followed by the file path to see its history before it was removed.
git log -- path/to/deleted_file.txt
Understanding Git Commits
What is a Commit?
In Git, a commit is a snapshot of your project at a specific point in time. Each commit contains an extensive record of the changes made, including metadata like the author, date, and a unique identifier (hash). Understanding how commits work is fundamental to navigating the Git history effectively, especially when searching for actions like a file's deletion.
Importance of Git History
The history captured in Git allows developers to track changes, understand project evolution, and recover from mistakes. Reviewing deleted files can provide crucial insights, especially if the deletion was unintentional or if you need to track changes over time.
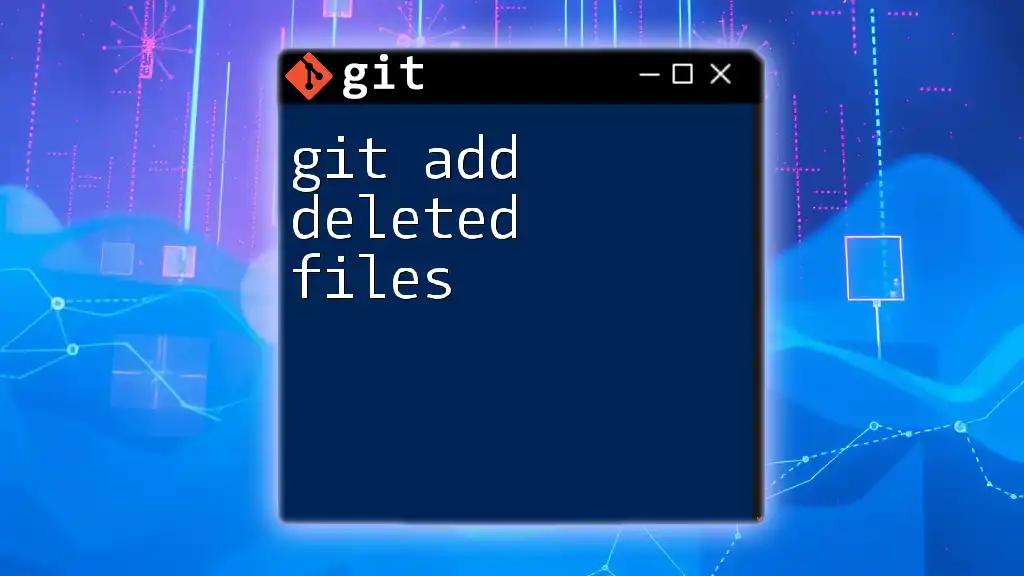
The `git log` Command
Overview of `git log`
The `git log` command is one of the most powerful tools in Git for examining the commit history. It provides a comprehensive overview of all commits in the repository, showcasing relevant information such as commit messages, authors, and dates.
Basic Usage
At its most fundamental level, you can run the command simply as follows to view the commit history:
git log
This command will output the list of commits in reverse chronological order, allowing you to see the most recent changes first.
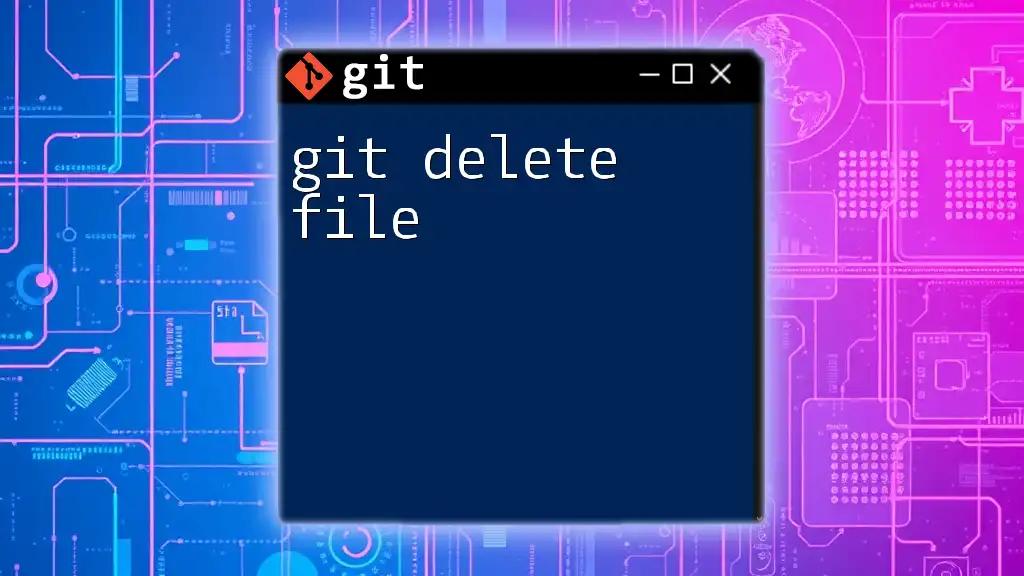
Identifying Deleted Files with `git log`
What Happens When a File is Deleted?
When you delete a file and create a commit, Git registers that action. The deleted file is still preserved in the project history, meaning you can recover it if necessary. Understanding how deletions are recorded is critical for any developer dealing with files that may need to be restored.
Using the `--diff-filter` Option
To specifically view deleted files, you can utilize the `--diff-filter` option with `git log`. The `D` filter stands for deletions, allowing you to isolate commits that resulted in deleted files in your project.
Example Command
To find the history of a deleted file, use the following command syntax:
git log --diff-filter=D -- [file-path]
Replace `[file-path]` with the path of the file you are interested in. This command will return a list of commits that deleted the specified file.
Viewing Detailed Changes
Using the `-p` Flag
The `-p` flag with `git log` allows you to see the patch of changes made in each commit, making it easier to understand what led to a file's deletion and how the repository’s state changed.
Example Command
You can view the detailed changes alongside the deletions by executing:
git log -p --diff-filter=D -- [file-path]
This command will not only show you all the commits that deleted the file but also the actual differences in the content, giving you better context around the deletions.
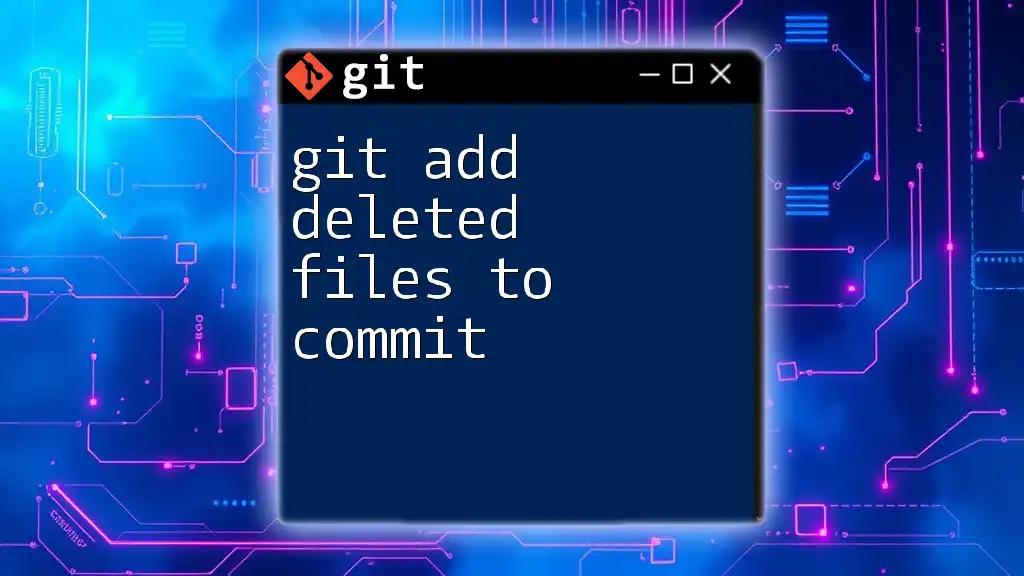
Exploring the Commit History for Deleted Files
Reverting Changes
One of the strengths of Git is its ability to revert changes, including file deletions. If you discover that a file was deleted unintentionally, you can effectively use the commit history to restore it.
You can either use `git checkout` or `git restore` to revert to a previous version before the file was deleted.
Example Command
To restore a deleted file from a previous commit, run:
git checkout <commit-hash> -- [deleted-file-path]
Replace `<commit-hash>` with the hash of the commit where the file still exists. This action retrieves the file from that specific commit into your working directory, effectively restoring it.
Recovering Deleted Files
Successfully recovering deleted files requires an understanding of the commit history. By locating the commit before the deletion occurred, you can bring back the files you need without losing other subsequent changes.
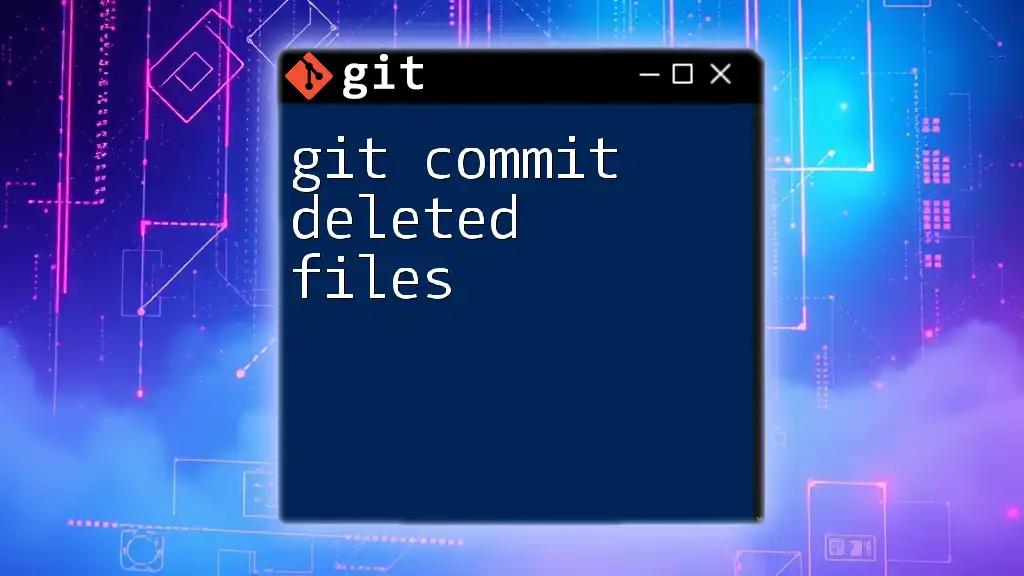
Advanced Usage of `git log`
Custom Formatting with `--pretty`
To customize how `git log` displays information, you can use the `--pretty` option. This option allows you to format commit messages and other metadata, making the output easier to read and more useful.
Example Command
Utilize the following command to see a concise format that lists deleted files:
git log --diff-filter=D --pretty=oneline
This command provides a one-line summary of each commit that deleted a file, making it quicker to scan through history.
Combining Multiple Options
For a more tailored view, combining various options can yield precise outcomes. For example, you might want to see a detailed patch for only deleted files in a compact format:
git log --diff-filter=D -p --pretty=medium
This command will show the deleted files with a medium-format commit overview, along with the changes made in each relevant commit.
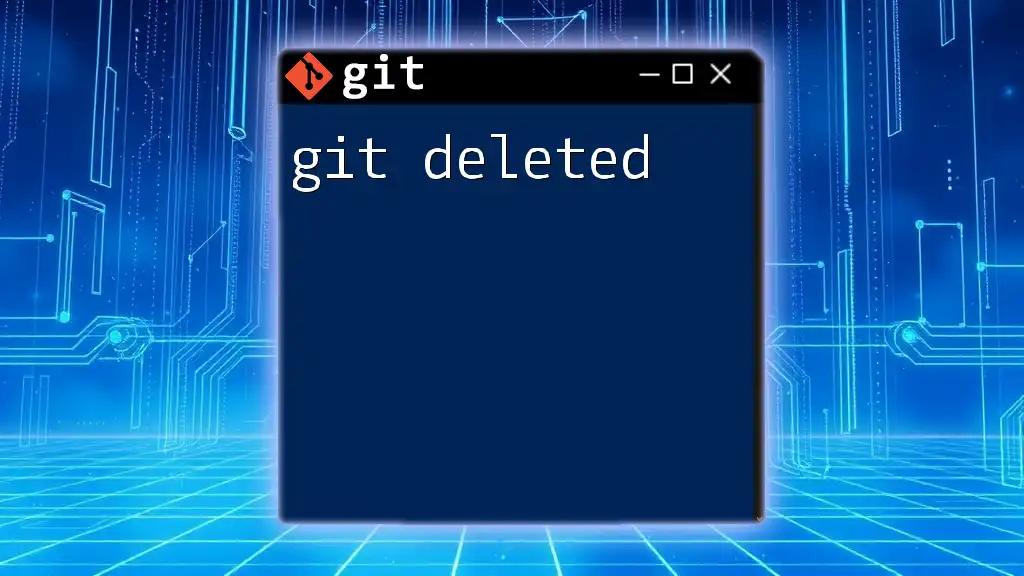
Best Practices for Managing Deleted Files in Git
Regularly Review Your Git History
Regularly reviewing your Git history, including the changes and deletions, is critical for maintaining a healthy codebase. Being proactive in examining your project's history helps prevent unintended loss of crucial files and fosters a better understanding of the project's evolution.
Documenting Changes
Whenever you make significant changes, particularly deletions, ensure that your commit messages are descriptive. Documenting the reasons behind deletions provides context for future reference, making it easier for yourself and others to understand the choices made in the project.
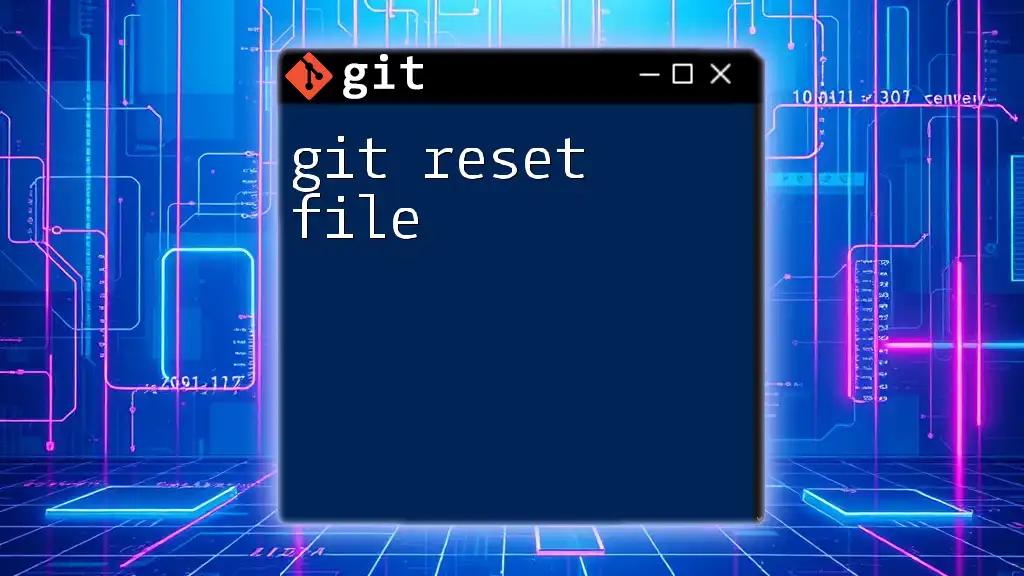
Conclusion
Utilizing the `git log` command to track deleted files offers robust capabilities to manage your project's history effectively. By employing the right commands and techniques, you can navigate your Git repository's commit history, recover deleted files safely, and maintain a thorough understanding of your project's evolution. Remember to regularly engage with the history of your repository to enhance your development workflow.
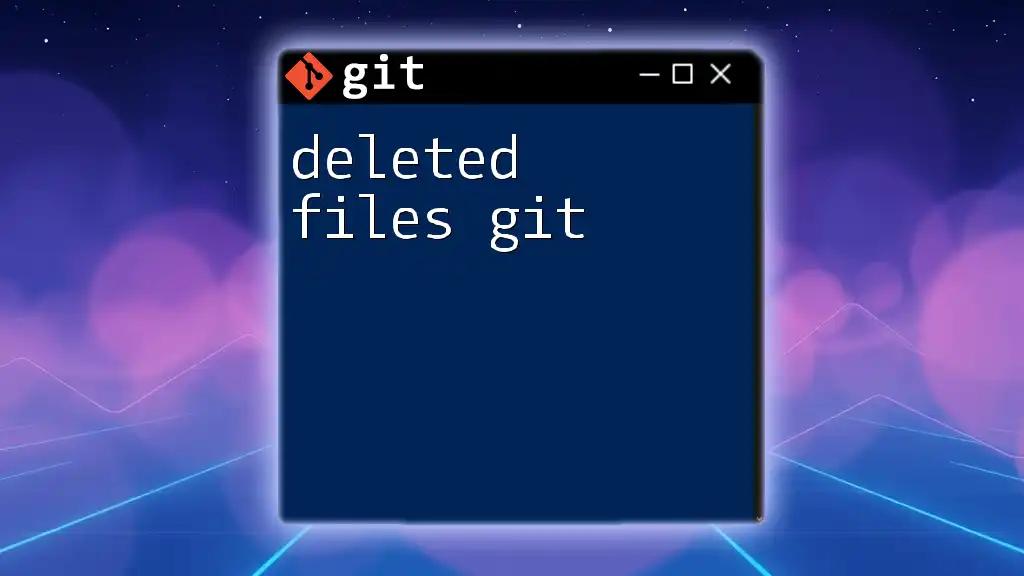
Additional Resources
For further reading, consider exploring the official [Git documentation](https://git-scm.com/doc). There, you can find valuable insights on commands, best practices, and advanced features. Additionally, look for recommended Git tutorials or courses that provide deeper explorations of important concepts within version control.
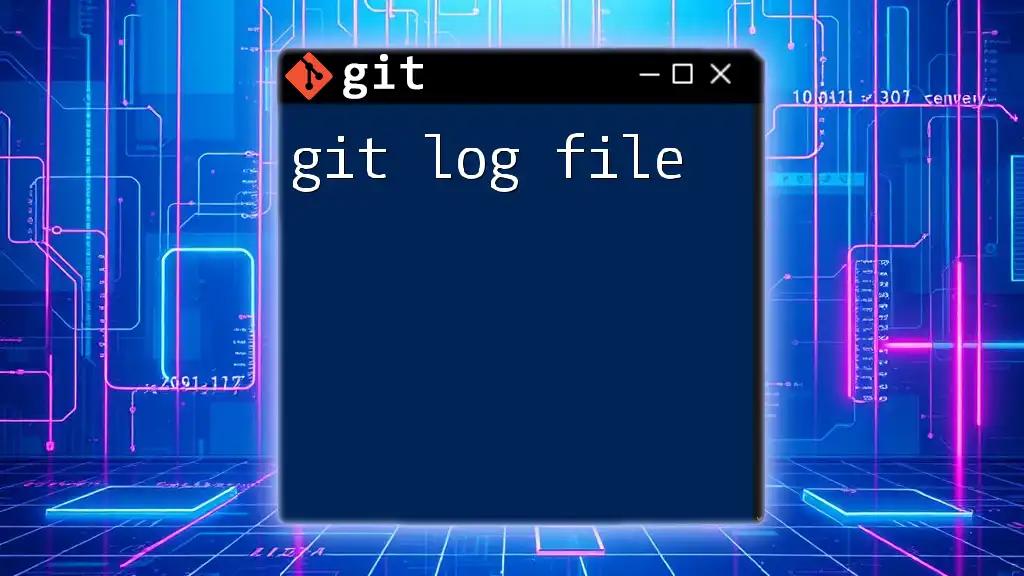
FAQs
When you encounter common questions regarding `git log` and managing deleted files, refer back to this guide to clarify your understanding and improve your fluency with Git commands.