You can remove the last commit in Git by using the command `git reset --hard HEAD~1`, which resets your current branch to the previous commit while discarding all changes made in the last commit.
git reset --hard HEAD~1
Understanding Commits in Git
What is a Commit?
In Git, a commit is a snapshot of your project at a particular point in time. It encapsulates all the changes made to files, capturing the state of your project. Each commit serves as a historical record that you can reference and revert to if needed. Commit messages play a crucial role in clarifying what changes were made and why, making it easier for you and your collaborators to understand the project's evolution.
Common Reasons to Remove the Last Commit
There are several scenarios where you might find yourself in need of removing the last commit:
- Unintended Changes Included: Perhaps you accidentally added files or made modifications you didn't intend to.
- Mistaken Commit Message: You might have realized that your commit message does not accurately reflect the changes made.
- Need to Revert to a Previous State: Sometimes, changes don’t work as expected, and you wish to eliminate the last commit to return to a functioning state.
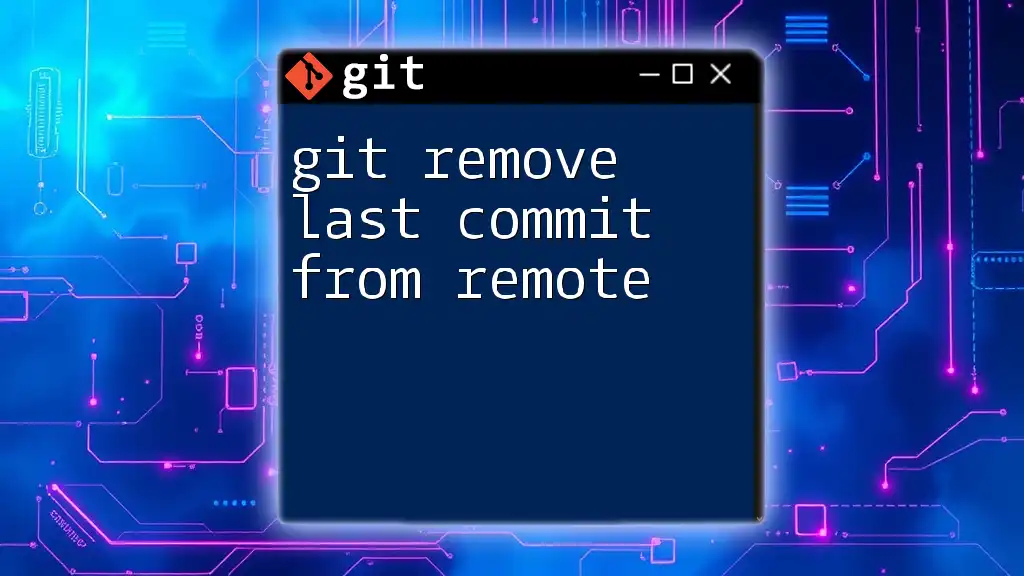
Methods to Remove the Last Commit
Soft Reset
What is a Soft Reset?
A soft reset in Git allows you to remove the last commit while preserving the changes made in the staging area. This means that you can modify your files or commit message without losing any work.
How to Perform a Soft Reset
To perform a soft reset, use the following command:
git reset --soft HEAD~1
Explanation: This command effectively moves the current branch pointer back by one commit (HEAD~1), removing the last commit while keeping the changes in the staging area. This gives you a chance to rectify what went wrong and recommit the changes with a corrected commit message or additional modifications.
Mixed Reset
What is a Mixed Reset?
A mixed reset differs from a soft reset in that it removes the last commit and un-stages the changes, returning them to your working directory. This is useful if you want to modify the changes before committing again.
How to Perform a Mixed Reset
To execute a mixed reset, run this command:
git reset HEAD~1
Explanation: This command removes the last commit much like a soft reset but leaves changes in your working directory while un-staging them. You'll find your files modified but not ready to be committed. You can make adjustments or stage the files again as needed.
Hard Reset
What is a Hard Reset?
A hard reset is the most drastic method. It not only removes the last commit but also deletes all changes made to your working directory. This means any changes that were part of the commit are permanently lost, so caution is necessary.
How to Perform a Hard Reset
To perform a hard reset, use:
git reset --hard HEAD~1
Explanation: This command is very powerful and should be used with care. It resets the current branch to the commit just before the last one and clears all changes in the working directory. This operation is not reversible via standard Git commands, so ensure you have backups or are certain of your decision when using this command.
Reverting the Last Commit
What Does It Mean to Revert a Commit?
Reverting a commit is different from resetting. When you revert, you create a new commit that negates the changes introduced by the last commit. This method keeps the project history intact, which is crucial for projects involving collaboration.
How to Revert the Last Commit
To revert the last commit, follow this command:
git revert HEAD
Explanation: This command generates a new commit that reverses the effects of the last commit. It’s a safe alternative to removing a commit and ensures that collaborators are aware of the changes that have occurred without erasing history.
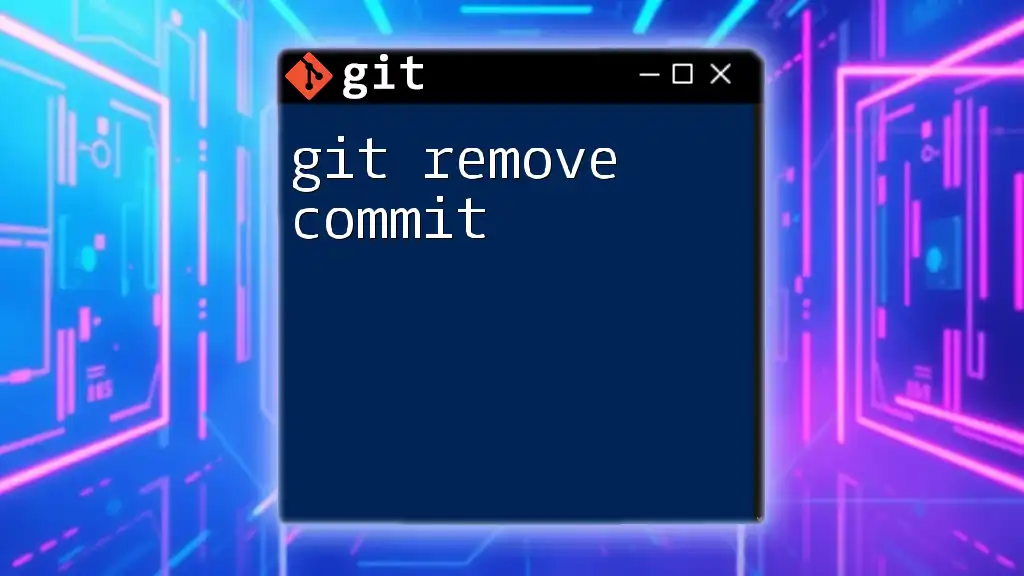
Situations to Consider
Collaborating with Others
When working in a team, altering commit history—especially after sharing changes with collaborators—can cause confusion. Ensure clear communication with your team about any alterations to commits. Discuss the decisions before proceeding with resets or reverts that affect the collective repository.
Undoing Changes After Pushing
If you've already pushed your last commit to a remote repository, be mindful of your actions. If you use `git reset` in this situation, you'll need to force-push with:
git push origin <branch-name> --force
However, be cautious—force-pushing can lead to complications for others working on the same branch. It’s often better to use `git revert` in collaborative environments, where it’s critical to preserve the shared history.
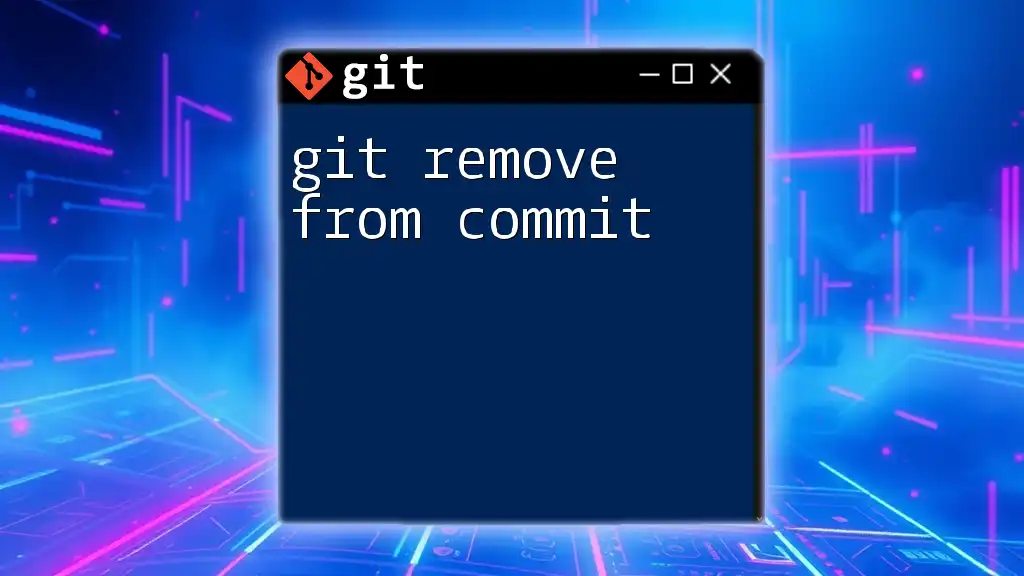
Conclusion
Removing the last commit in Git can be done via various methods—soft reset, mixed reset, hard reset, and reverting. Each method serves a different purpose and has its implications on your commit history and working directory. Understanding these options is vital for anyone looking to master Git and manage their code effectively.
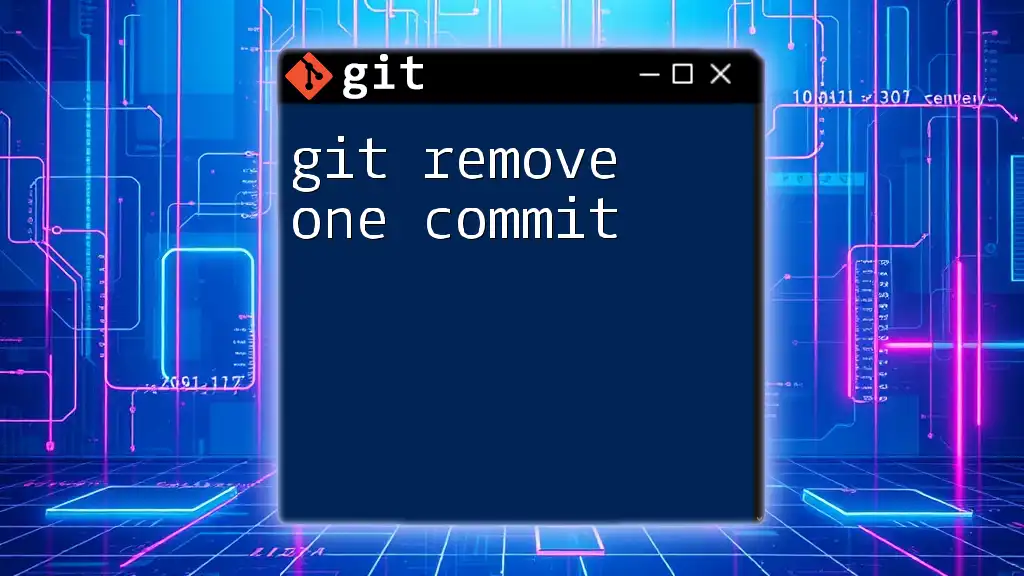
Additional Resources
For those eager to expand their Git knowledge, consider exploring additional tutorials and courses. Resources that delve deeper into Git commands and branching strategies will prove invaluable.
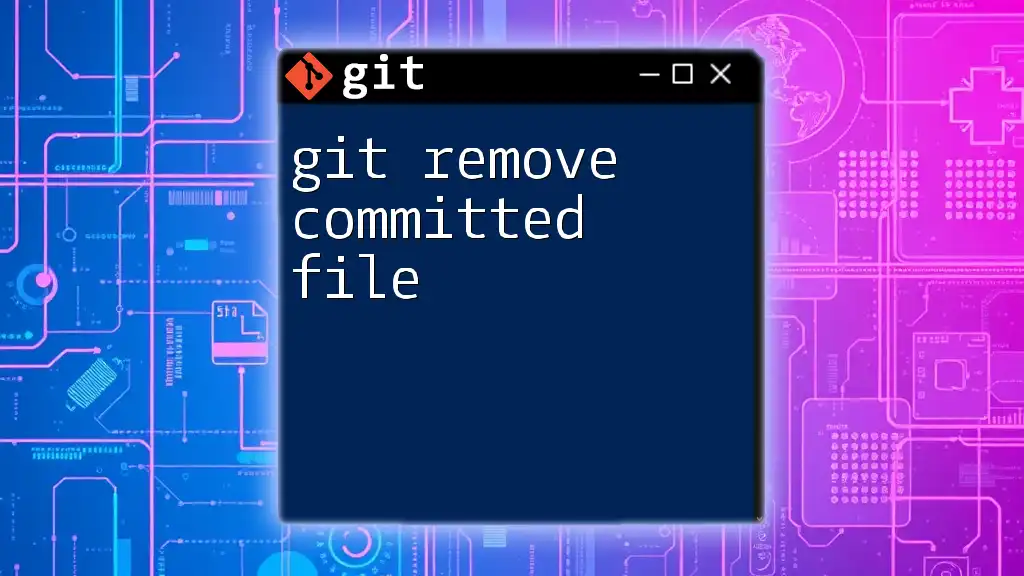
FAQs
Can I remove multiple commits at once?
Yes, you can remove multiple commits using the `HEAD~n` syntax in your reset command, where `n` is the number of commits to go back. For example:
git reset --hard HEAD~3
What happens if I remove a commit that others rely on?
Removing a shared commit can lead to significant confusion and should be avoided without consensus. Ensure that everyone is aware of such changes to prevent disruptions in collaborative workflows.
Is it possible to recover a removed commit?
Yes, Git keeps a log of all actions performed in your repository called reflog. To view it, run:
git reflog
This allows you to identify and recover lost commits in certain scenarios.
Understanding how to git remove the last commit is critical for keeping your project under control and making necessary adjustments when mistakes happen.