Git branching allows users to create separate lines of development to work on features or fixes independently, and merging combines these branches back into the main line while integrating changes. Here's a basic example of how to create a branch, switch to it, and then merge it back to the main branch:
# Create a new branch called 'feature-branch'
git branch feature-branch
# Switch to the new branch
git checkout feature-branch
# After making changes, add and commit them
git add .
git commit -m "Added new feature"
# Switch back to the main branch (usually 'main' or 'master')
git checkout main
# Merge changes from 'feature-branch' into 'main'
git merge feature-branch
Understanding Git Branches
What is a Branch?
In Git, a branch serves as an independent line of development. It allows you to diverge from the main line of code, enabling parallel work on features, bug fixes, or experiments without affecting the main codebase. By creating branches, developers can work on different features simultaneously without interfering with each other’s progress.
Creating a Branch
To create a new branch, use the following command:
git branch <branch_name>
For example, to create a new feature branch called "new-feature," you would run:
git branch new-feature
This command simply creates the branch. It does not switch you to that branch. You now have diverged from the current state; however, changes made in this new branch won’t affect others until you merge them back.
Switching Branches
To switch to a different branch, you can use:
git checkout <branch_name>
Switching to your newly created branch would look like this:
git checkout new-feature
When you execute this command, Git updates the files in your working directory to match the state of the `new-feature` branch. It’s crucial to frequently switch back and forth between branches to ensure you are working on the correct version of your project.
Viewing Branches
You can view all branches in your local repository by executing:
git branch
This command will display a list of all branches, with an asterisk (*) next to the currently active branch. Understanding the state of your branches is essential for maintaining clarity, especially in larger projects.
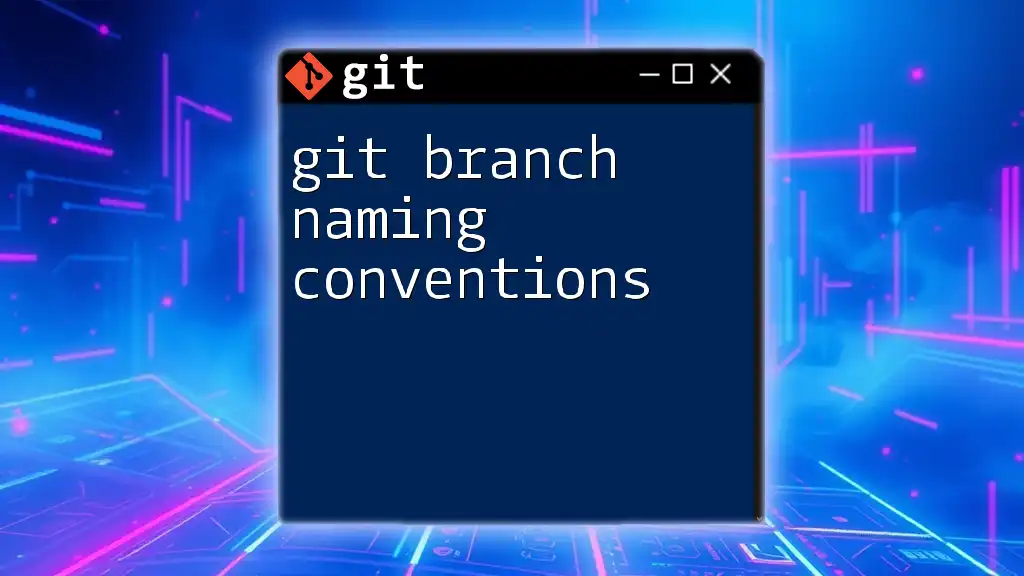
The Branching Model
Main Branch (main/master)
The main branch, often referred to as `main` or `master`, is considered the stable branch of your repository. This branch should always have a production-ready state, implying that it contains code known to be functional and bug-free.
Feature Branching
Feature branching involves creating a new branch specifically for the development of a new feature or a fix. This method allows the developer to work in isolation. For example, when beginning work on a new feature, you might follow this workflow:
- Create a feature branch (e.g., `new-feature`).
- Implement the new feature.
- Test to ensure that everything works as intended.
- Merge the feature branch back into the main branch upon completion.
When naming feature branches, consider adopting a meaningful naming convention that describes the purpose (e.g., `feature/user-authentication`).
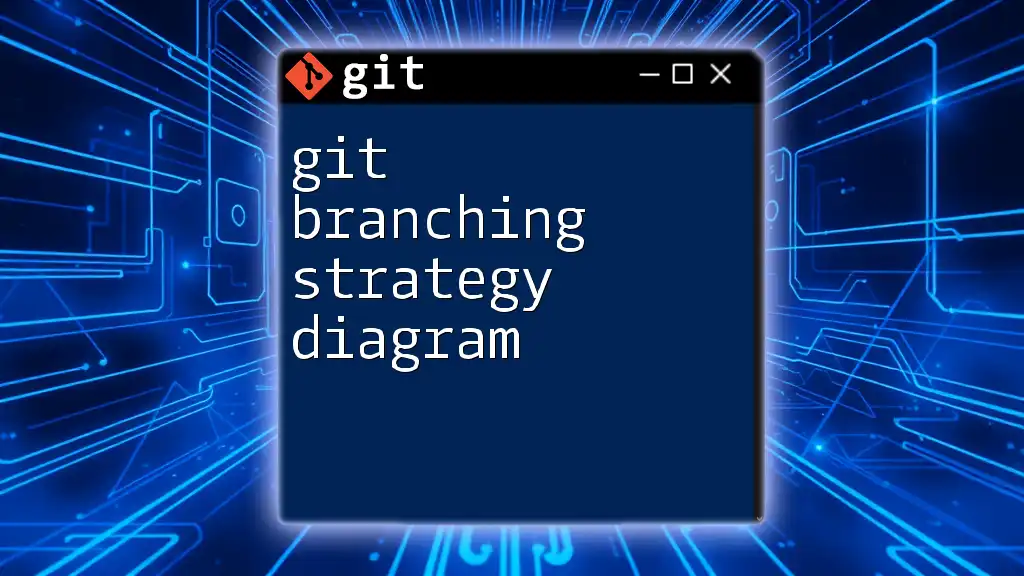
Merging Branches
What is Merging?
Merging in Git is the process of integrating changes from one branch into another. For example, once you’ve completed work on `new-feature`, you would merge it back into the `main` branch. Merging can happen in two primary ways: fast-forward merges and non-fast-forward merges.
Basic Merge Command
To merge a branch, first ensure you are on the branch you want to merge changes into (e.g., `main`):
git checkout main
Then, run the merge command:
git merge new-feature
This command will bring all changes from `new-feature` into `main`. If changes to both branches don’t conflict, this will happen seamlessly.
Handling Merge Conflicts
What is a Merge Conflict?
A merge conflict arises when changes to the same line of a file differ between two branches. For instance, if one branch modifies a line that another branch has also changed, Git will notify you of this conflict when trying to merge.
Resolving Merge Conflicts
To resolve conflicts, first run:
git status
This will highlight files that are in conflict. Then, you can manually edit these files to resolve conflicts. After editing, you must stage the resolved files:
git add <file_name>
Finally, you conclude the merge by completing it with:
git commit
Tips for resolving conflicts include employing merge tools or considering a team discussion on complex merges.
Merge Strategies
Overview of Merge Strategies
Git offers several merge strategies, including `recursive` (the default) and `resolve`. Understanding these strategies allows you to choose the most effective one for your scenario.
Specifying a Merge Strategy
If necessary, you can specify a merge strategy using:
git merge -s <strategy> <branch_name>
For example:
git merge -s resolve new-feature
Using the appropriate strategy depends on the complexity and history of the branches involved.
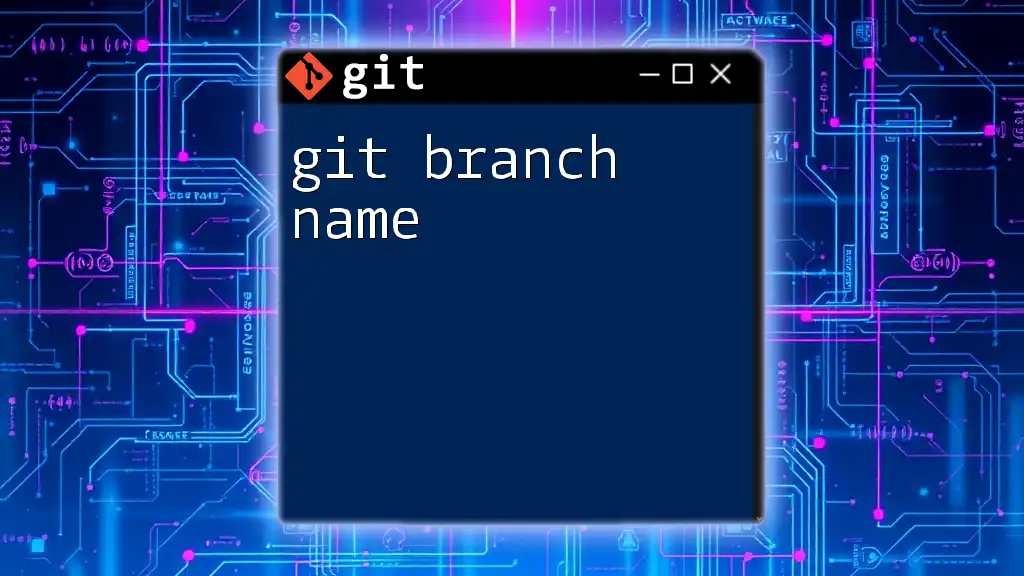
Advanced Branching Techniques
Rebasing vs. Merging
Understanding the difference between rebasing and merging is crucial for maintaining a clean project history. Rebasing replays changes from one branch onto another, whereas merging creates a new commit that includes the history of both branches. This can be demonstrated with:
git rebase <branch_name>
Rebasing might result in a linear project history, yet it can be risky if changes have already been shared with others.
Creating a Branch from a Different Commit
To create a branch based on a specific commit, you use:
git checkout -b <branch_name> <commit_id>
This technique allows you to base new work on a previous state of the project, which can be useful for hotfixes or experimenting.
Deleting Branches
Why and When to Delete Branches
Keeping your branch structure clean is vital. Over time, branches that are no longer needed can clutter your repository, making it hard to navigate.
Deleting a Local Branch
To delete a local branch, use:
git branch -d <branch_name>
You would typically delete branches that have been merged or are no longer active.
Deleting a Remote Branch
To delete a branch on the remote repository, execute:
git push origin --delete <branch_name>
This is useful when cleaning up your remote repository, ensuring that only relevant branches remain.
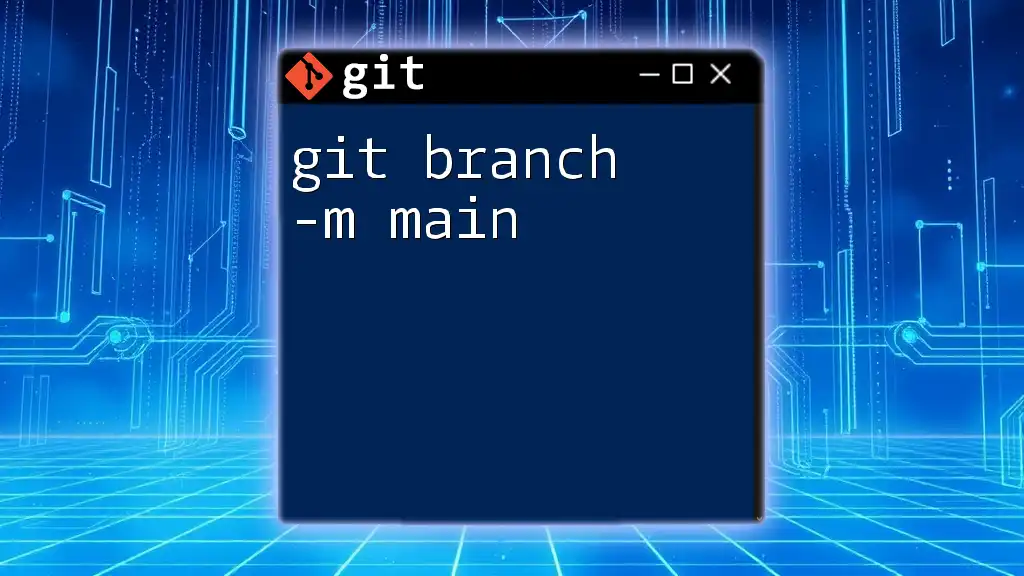
Conclusion
Mastering git branching and merging is essential for collaborative development and effective project management. Through understanding how to create, manage, and merge branches, developers can work more efficiently and with fewer integration issues. Remember to practice merging and conflict resolution in real-world projects to solidify your understanding and improve your workflow.
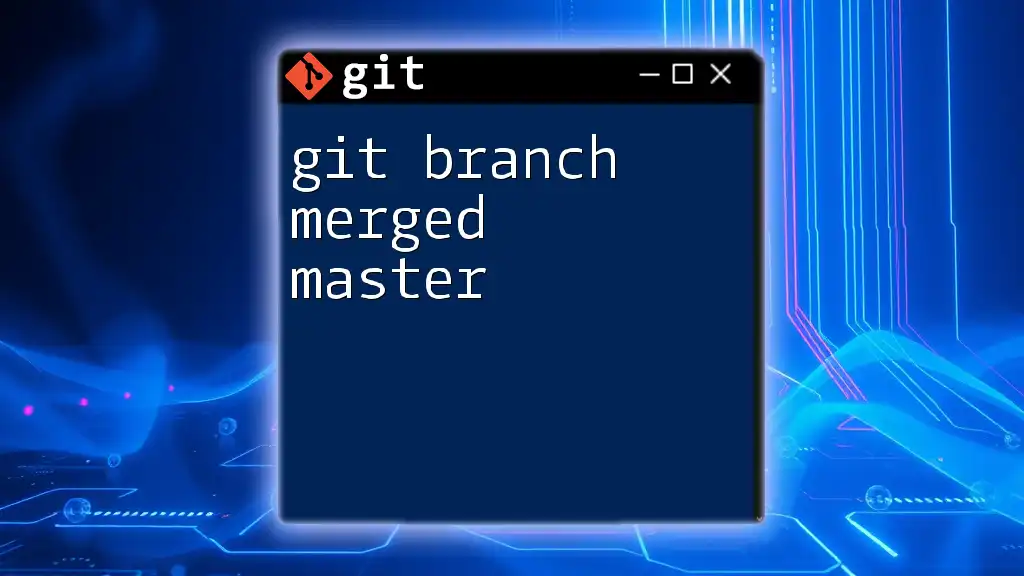
Frequently Asked Questions (FAQs)
What is the best practice for branching in a team?
Effective communication and structured naming conventions can help the team avoid conflicts and streamline branch management.
When should I merge versus rebase?
Use merging to preserve the history of how branches came together, but consider rebasing for a linear history when the branches have not been shared.
How do I manage long-lived feature branches?
Regularly sync long-lived branches with the main branch to minimize conflicts and ensure that they remain up to date.