The command `git branch --merged master` lists all branches that have been fully merged into the master branch, helping you identify branches that can be safely deleted.
git branch --merged master
What is a Branch in Git?
A branch in Git is a lightweight movable pointer to a commit. By default, Git assigns a branch named `master` (or `main` in recent practices) when you create a repository. Branches allow you to diverge from the main line of development and continue to work separately without affecting the main codebase. This enables developers to experiment with new features or fix bugs in isolated environments.
Branches are essential for collaborative development. Various feature branches can be created for individual tasks while ensuring the `master` branch remains stable and release-ready.
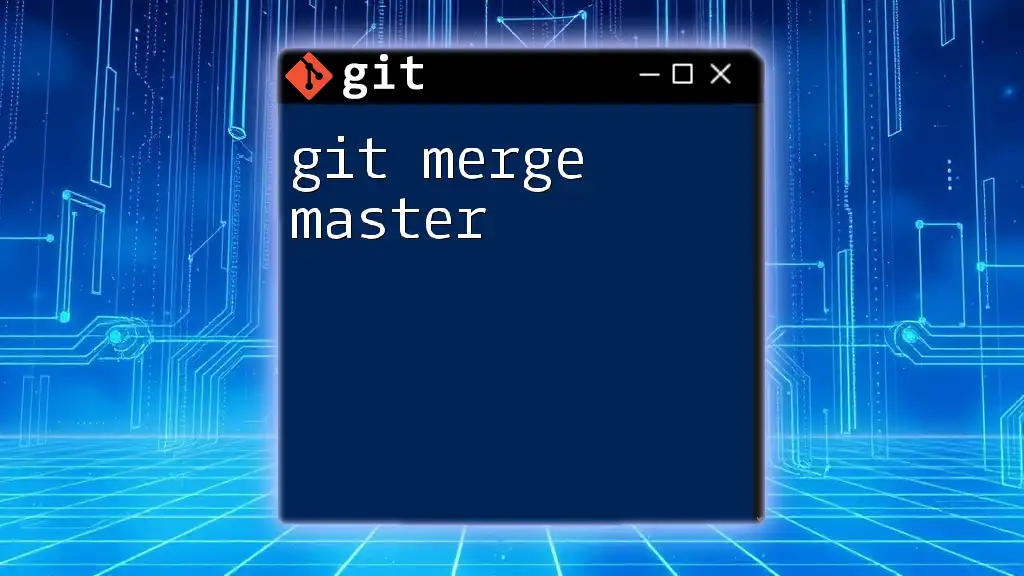
Understanding the Master Branch
The `master` branch, now commonly referred to as the `main` branch, serves as the primary branch in many repositories. It is the default working branch where the stable version of your code resides.
Historically, many workflows relied heavily on the `master` branch for production-ready code. As we evolve in our understanding of inclusive language, the transition from `master` to `main` reflects our commitment to sensitivity in terminology. Regardless of the naming convention, the principles remain the same.
Common practices for using the master/main branch involve merging it exclusively after thorough testing and reviewing changes in feature branches.
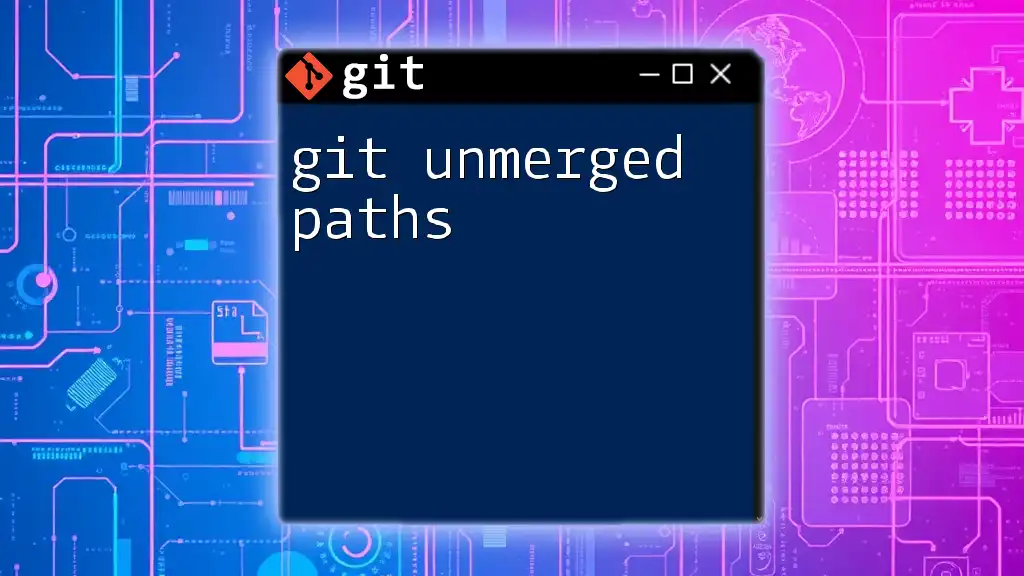
The Process of Merging Branches
What does Merge Mean?
Merging is the process of integrating changes from one branch into another. This typically involves merging feature branches back into the `master` to include the new functionality or fixes developed in that branch.
An important distinction to make is between merging and rebasing. While merging creates a new merge commit that ties together the two branches, rebasing reapplies your changes over the base branch, resulting in a linear history. Each approach has its benefits, and the choice often depends on the team's workflow.
Types of Merges
-
Fast Forward Merge: This occurs when the `master` branch has not diverged from the feature branch. Essentially, Git will simply move the `master` branch pointer forward to point to the current feature branch commit.
-
Three-Way Merge: If the two branches have diverged, Git performs a three-way merge, using the most recent commit common to both branches to create a new merge commit.
Understanding the type of merge that will occur is crucial for effective version control.
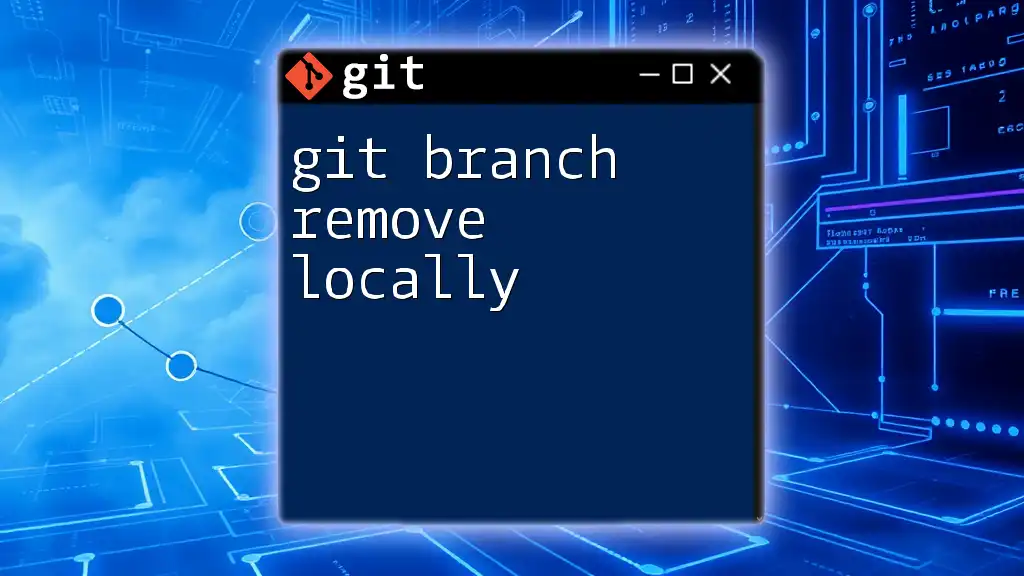
Preparing to Merge: Best Practices
Before merging, it's important to adhere to some best practices:
- Ensure your `master` branch is up-to-date to avoid unnecessary conflicts.
- Review the changes in your feature branches and confirm they work as expected.
- Testing your code is vital. Using Continuous Integration (CI) systems allows automated tests to ensure code integrity before merges.
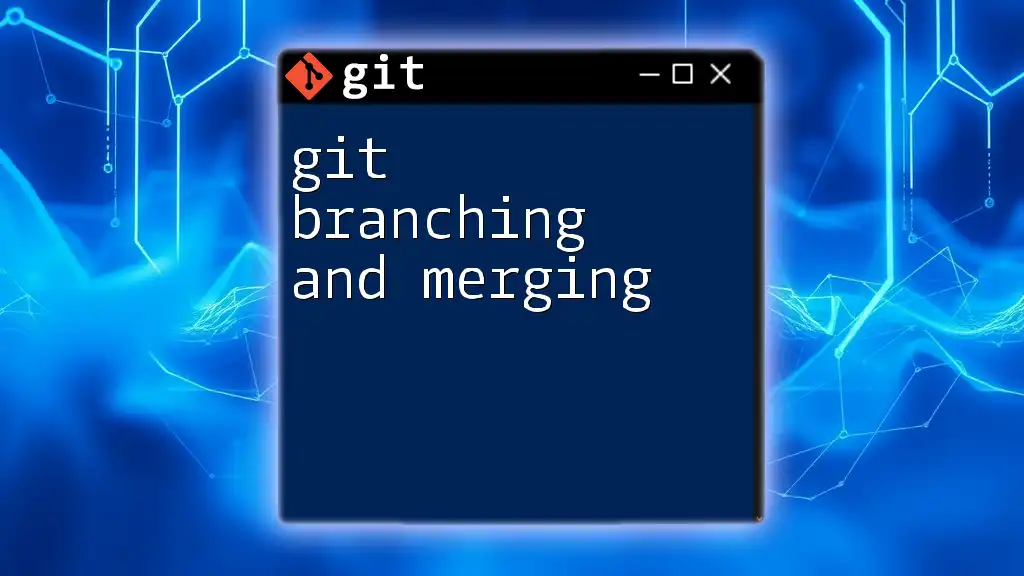
Using the `git merge` Command
Syntax of the Command
Merging branches in Git is performed using the `git merge` command. The basic structure is simple:
git merge [branch-name]
For example, to merge a feature branch named `feature-xyz` into the `master` branch, follow these commands:
# Check out the master branch
git checkout master
# Merge the feature branch into master
git merge feature-xyz
Merge Commit
When merging branches, Git creates a new merge commit. This commit serves as a connection point between the merged branches. You can view the merge history using:
git log --graph --oneline
This command displays a visual representation of your branch commits and merges.
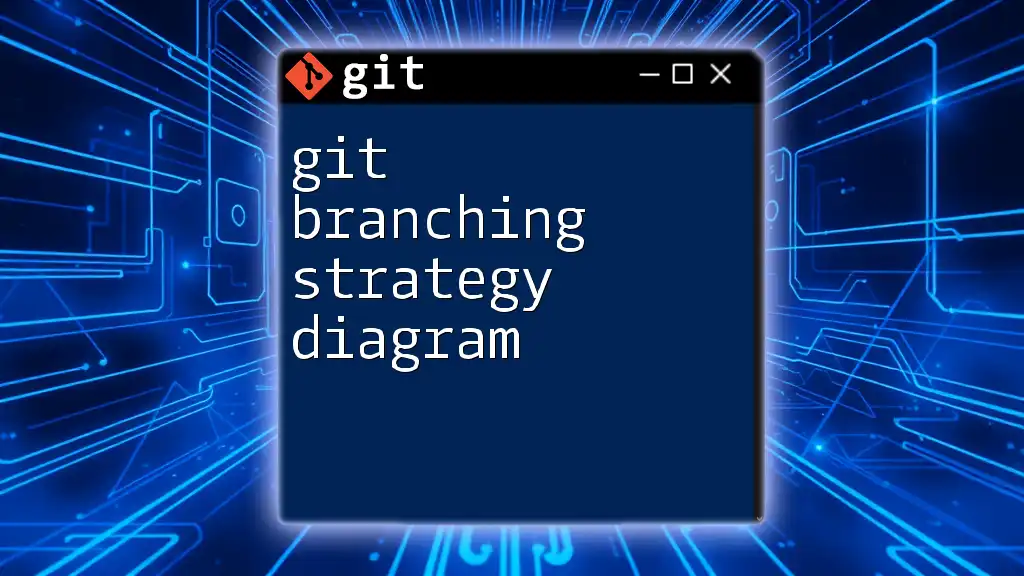
Handling Merge Conflicts
What are Merge Conflicts?
Merge conflicts occur when changes in two branches are incompatible. This usually happens when the same line of code is modified differently in each branch.
Such conflicts necessitate manual resolution, and understanding how to handle them is essential for efficient version control.
Steps to Resolve Merge Conflicts
When you encounter a merge conflict, Git will notify you about the conflicting files. Follow these steps to resolve:
-
Identify conflicting files:
git status
This command will show you which files are in conflict.
-
Edit the files to resolve conflicts. Open the conflicting files in your preferred text editor and look for markings that highlight the conflicting sections.
-
After resolving the conflicts, mark them as resolved with:
git add [file-name]
-
Finally, complete the merge with:
git commit
Tools to Help with Conflict Resolution
Utilizing graphical tools such as GitKraken or SourceTree, alongside command-line tools like kdiff3, can significantly ease the conflict resolution process. Example: Developers using Visual Studio Code often find its built-in merge editor helpful in visually managing conflicts.
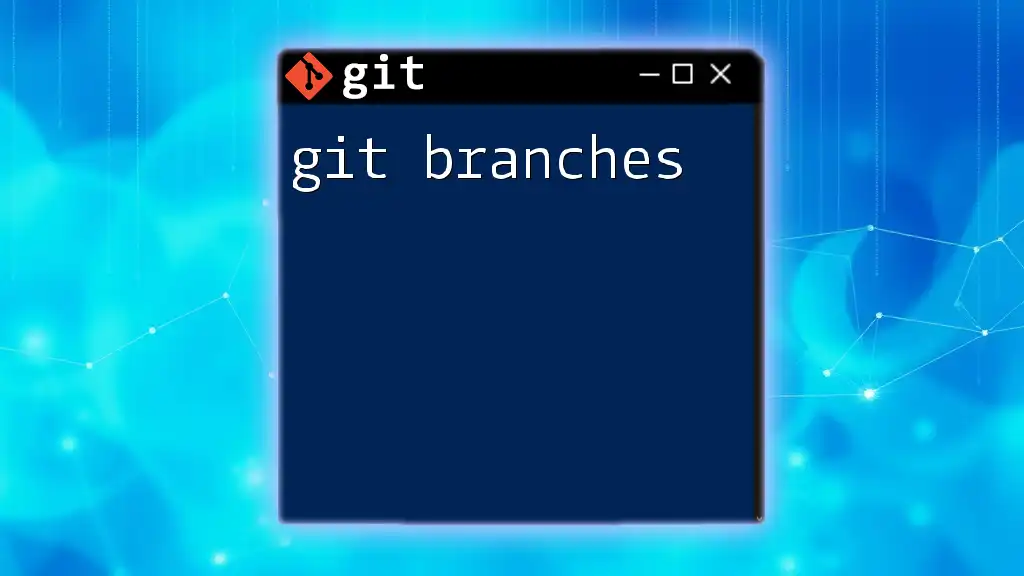
Best Practices for Working with Branches and Merging
To maintain a smooth workflow while managing branches, adhere to these practices:
-
Keep branches focused and short-lived. Aim to merge changes back into `master` frequently to avoid long-lived branches that can diverge significantly.
-
Regularly merge the latest changes from `master` into your feature branches to minimize conflicts later on.
-
Document changes with clear, descriptive commit messages to facilitate easier tracking and understanding of each change made over time.
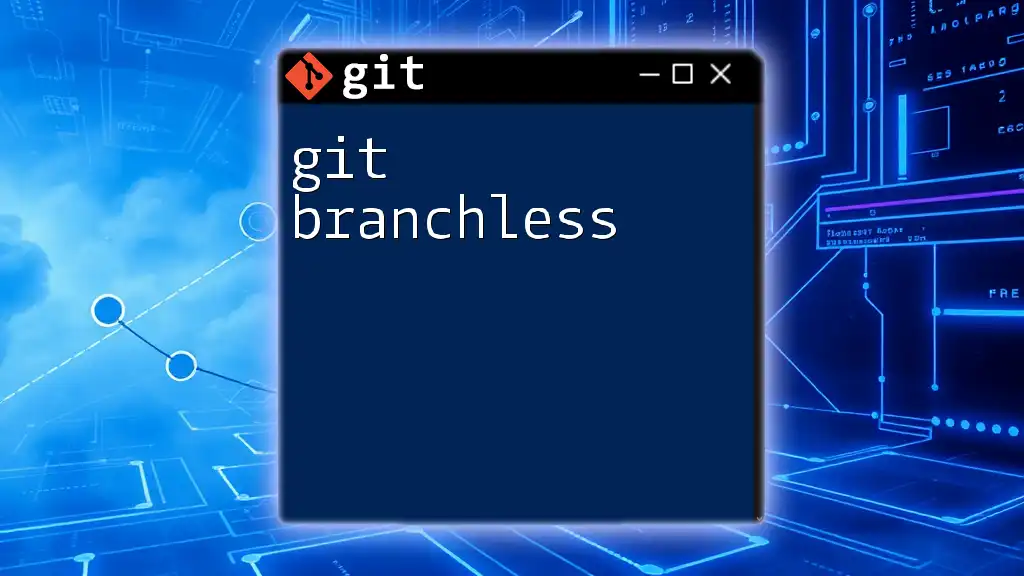
Conclusion
Merging branches in Git, particularly merging a branch into `master`, is a crucial skill for developers aiming for collaborative and efficient workflows. Understanding how to correctly use the `git merge` command, handle conflicts, and follow best practices will position you for success in any development environment.
As you delve deeper into Git, consider practicing these commands in a controlled environment to build your confidence. For more comprehensive lessons and hands-on experience, we invite you to explore our courses designed to extend your mastery of Git.
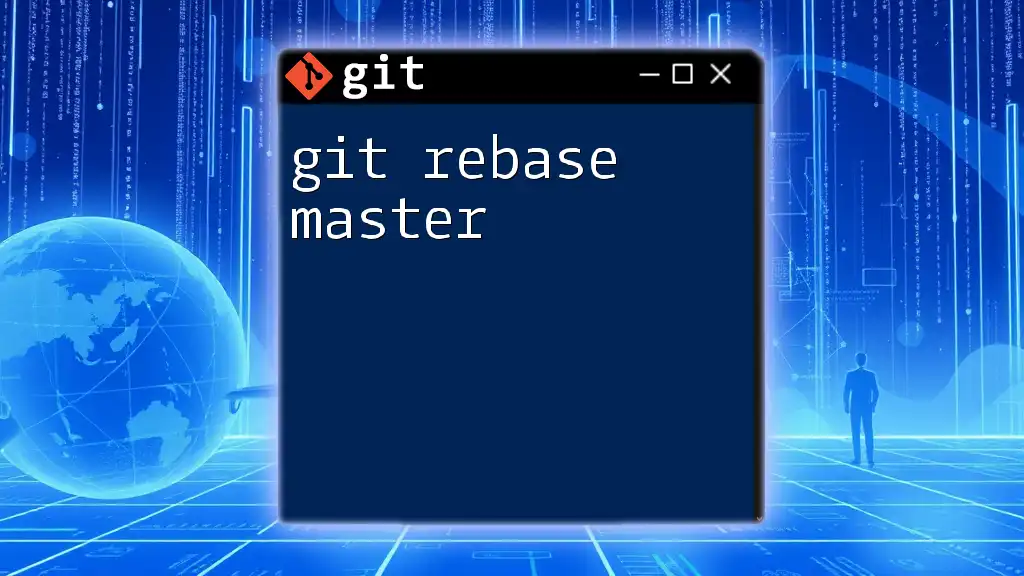
Additional Resources
For further exploration of Git, consider visiting the official Git documentation and additional reading on branching and merging strategies. Additionally, online Git playgrounds can provide practical exercises to refine your command-line skills.