The command `git checkout -b branch` creates a new branch called `branch` and immediately switches to it in your Git repository.
git checkout -b branch
Understanding `git checkout`
What is `git checkout`?
The `git checkout` command is a fundamental part of Git that allows users to navigate between different branches in a repository. It plays a crucial role in the version control system, enabling developers to easily switch context based on the task they’re working on.
Common Use Cases
- Switching between branches: Moving from one branch to another to work on different features or fixes.
- Restoring files: Retrieving a specific version of a file or directory from the repository.
Syntax Overview
The basic syntax for the `git checkout` command is:
git checkout [options] <branch>
Among its various options, the `-b` flag specifically indicates that a new branch should be created.
Common Options
- `-b`: This flag creates a new branch and switches to it.
- `-f`: Used to force the checkout, helpful if there are uncommitted changes.
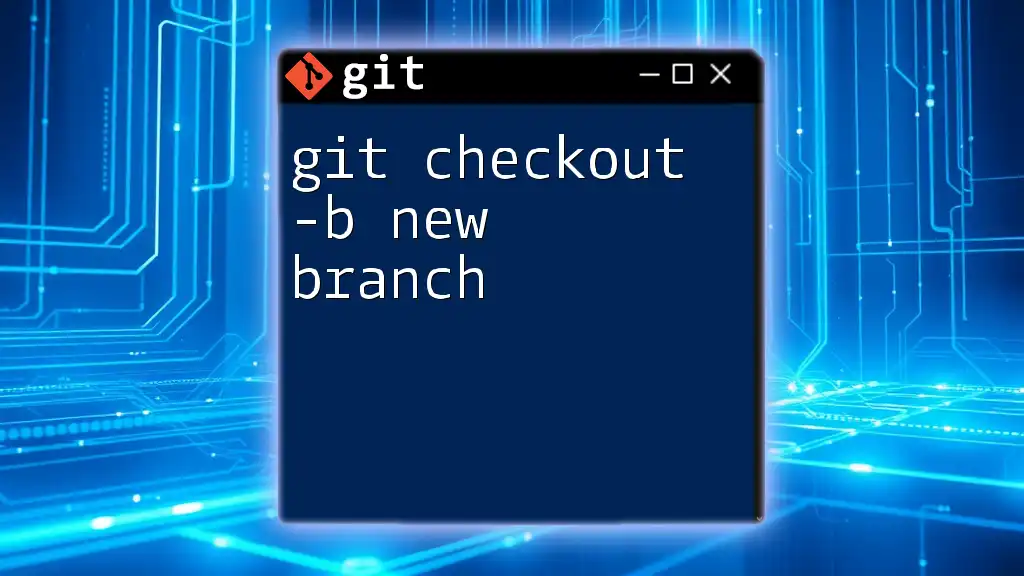
The `-b` Flag
What Does `-b` Mean?
The `-b` flag in the `git checkout` command is a powerful tool that allows users to create a new branch and switch to it simultaneously. This command combines two actions into one, enhancing workflow efficiency.
Benefit of Using `-b`
Using `-b` simplifies the process of branch creation. Instead of having to create a branch and then switch to it in two separate commands, you can achieve both actions with a single line of code, saving you time and effort.
How to Use `git checkout -b branch`
The syntax for creating a new branch with `git checkout -b` is:
git checkout -b <branch-name>
For example, if you want to create and switch to a branch for implementing a new login feature, you can execute:
git checkout -b feature/login
This command not only creates a new branch named `feature/login`, but it also immediately switches to that branch, allowing you to start development right away.
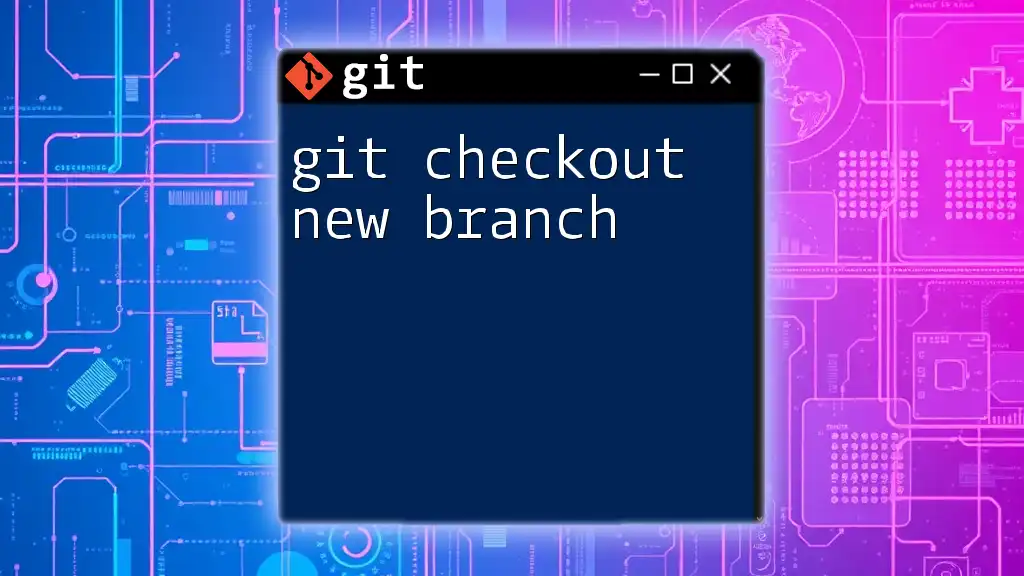
Step-by-Step Guide to Using `git checkout -b branch`
Prerequisites
Before using the `git checkout -b` command, ensure that:
- You have a basic understanding of how Git operates.
- Git is installed on your machine.
- You are inside a Git repository, which ensures the context in which you can create or switch branches.
Creating a New Branch
Before creating a new branch, it's important to understand the state of your current branch. You can verify your current branch by running:
git branch
This command lists all branches and highlights the active one. Naming your new branch descriptively can help maintain a clear focus on the task.
Executing the Command
To create a new branch, type the following command:
git checkout -b <new-branch-name>
For instance:
git checkout -b feature/login
This command adds clarity to your workflow; as soon as you execute it, you are on the new branch `feature/login`, ready to make changes.
Confirming the Branch Creation
After executing the command, it’s good practice to confirm that the new branch has been created and you are on it. Use the following command:
git branch
This will display all available branches, with your newly created branch highlighted. It’s a great way to ensure you are on track.
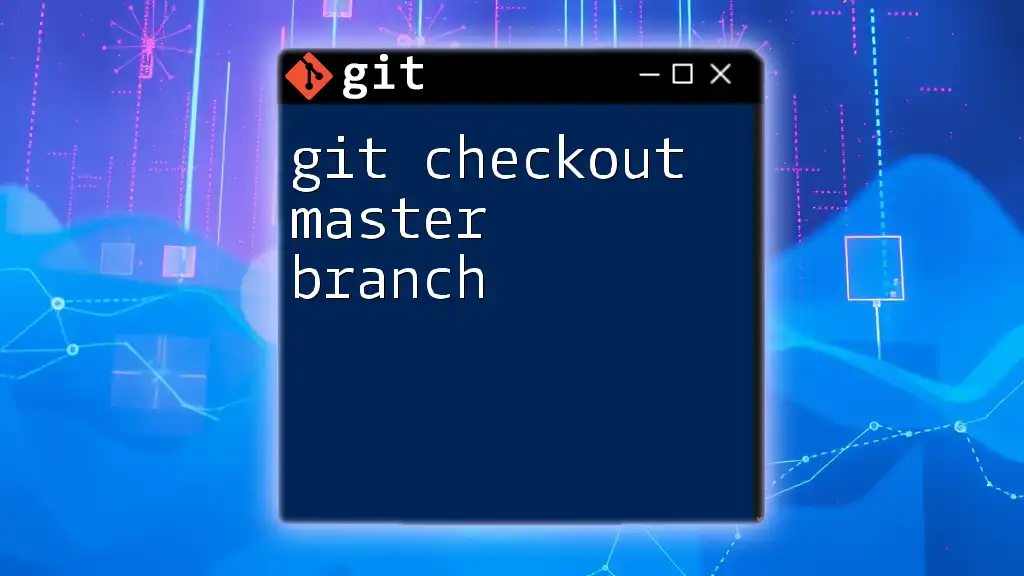
Common Scenarios for Using `git checkout -b branch`
Feature Development
Creating branches for new features is an essential practice in Git. It helps to isolate development work, making it easier to manage changes without affecting the main codebase. For instance, when starting a new feature, create a branch:
git checkout -b feature/<feature-name>
Bug Fixes
Similarly, when you need to address a bug, it's beneficial to create a dedicated fix branch. By using:
git checkout -b bugfix/<issue-number>
you can resolve issues without interfering with ongoing development tasks. This keeps your main branch cleaner and reduces the chances of introducing bugs.
Collaboration with Team Members
When working in teams, creating separate branches for different tasks helps maintain a streamlined workflow. Each member can create branches for their respective features or fixes while collaborating effectively. This not only enhances productivity but also makes it easier to integrate changes later.
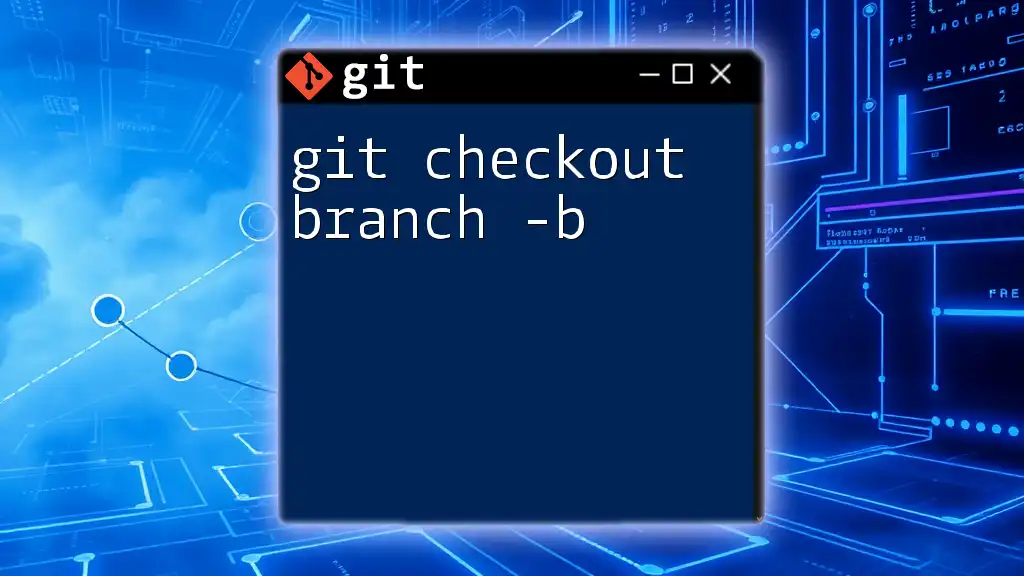
Troubleshooting
Common Issues
While using `git checkout -b`, you might encounter some common errors, such as:
- Error: branch already exists: This occurs if you attempt to create a branch that has already been created. Ensure to check existing branches before creating a new one.
Solutions and Fixes
If you face errors or discrepancies, the first step is to run:
git status
This command will provide insights into your current branch status and any uncommitted changes or merges needed. Understanding these details is crucial for successful branch management.
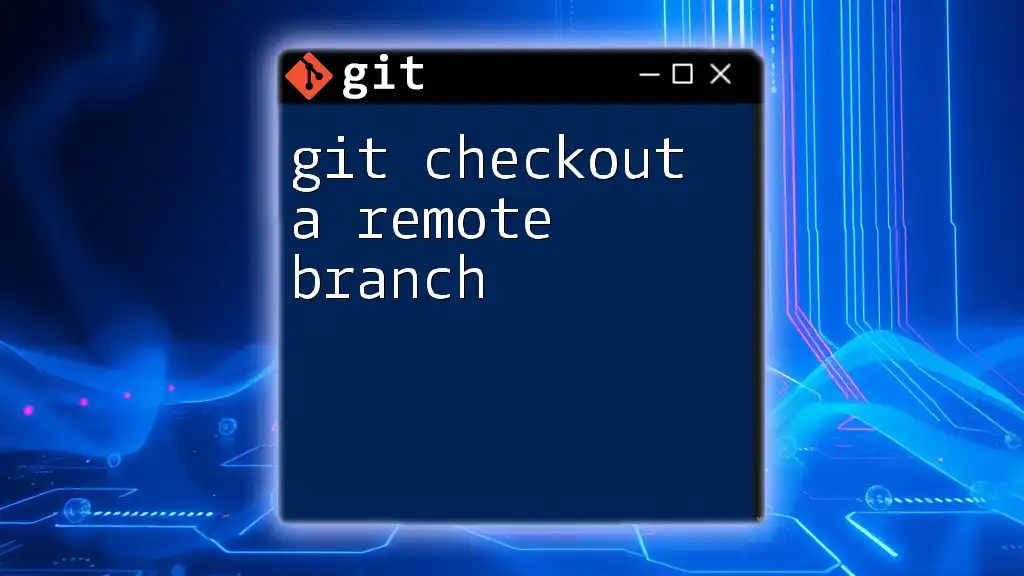
Conclusion
Recap of Key Points
In this guide, we have examined the `git checkout -b branch` command in depth, emphasizing its role in creating and switching branches rapidly. This command is a cornerstone for effective branching strategies in Git, enhancing both individual and collaborative development efforts.
Additional Resources
For further reading, consider exploring Git’s official documentation or reputable books on version control systems. These resources can deepen your understanding and improve your usage of Git commands.
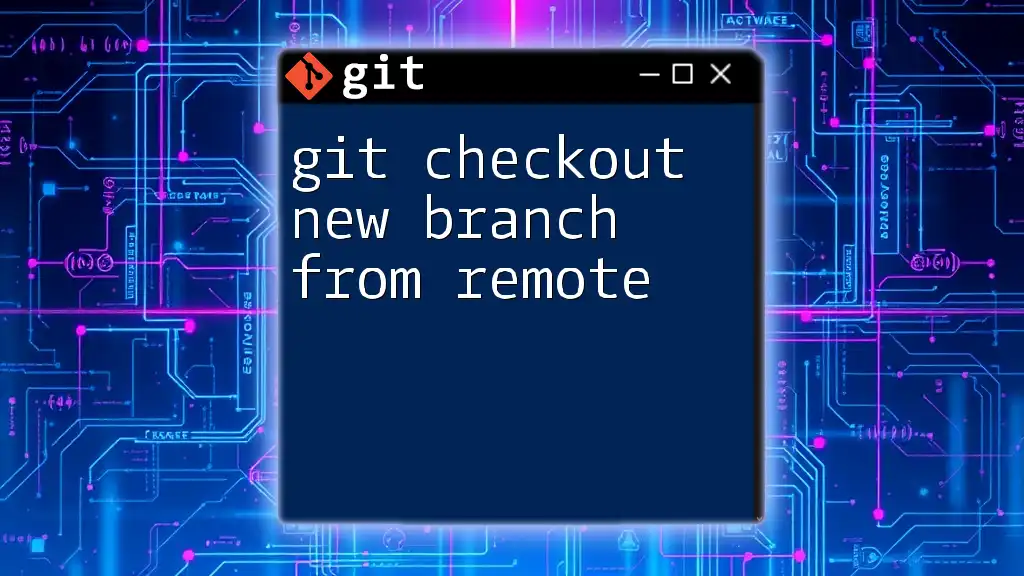
Call to Action
Now that you’re equipped with the knowledge on `git checkout -b branch`, take a moment to practice it in real-world scenarios! Experiment with branching strategies and see how they can enhance your workflow. If you have questions or seek more tutorials, feel free to reach out!
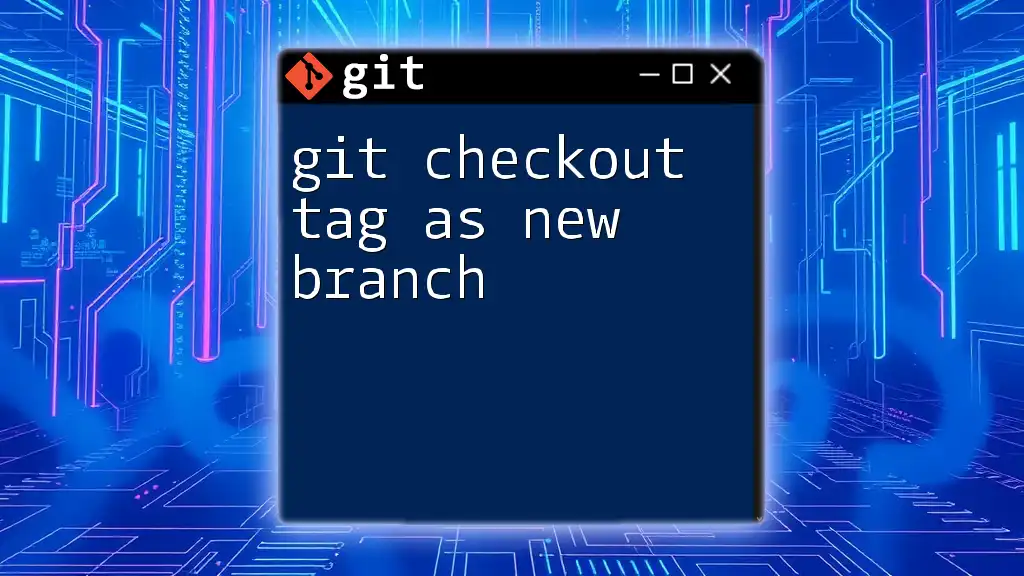
FAQs
What happens if you switch to a new branch without committing changes?
Switching branches with uncommitted changes can lead to complications. Git will hold your changes, but if those changes conflict with the files on the target branch, you might encounter merge conflicts that need resolution.
Can you create a branch from a specific commit?
Yes, you can create a branch based on a specific commit using:
git checkout -b <new-branch-name> <commit-hash>
This allows you to branch off from any point in the history, rather than just the latest commit on your current branch.
How does `git checkout -b` differ from `git switch -b`?
The `git switch` command, introduced in Git 2.23, specifically caters to branch switching and creation, providing a clearer intent. While `git checkout` can perform multiple functions, `git switch -b` is dedicated solely to branch management, improving user experience and reducing command-line confusion.