To create a new branch from a specific Git tag, use the following command, which will check out the tag and create a new branch based on it:
git checkout -b new-branch-name tags/tag-name
Understanding Git Tags
What are Git Tags?
In Git, a tag is a reference to a specific point in your repository's history. Tags are often used to mark releases, helping developers identify key milestones in their projects. There are two primary types of tags:
- Lightweight tags: These are essentially bookmarks to a specific commit. They don't contain any extra information apart from the commit itself.
- Annotated tags: These are full objects in Git's database. They contain a tagger name, email, date, and a tagging message, making them ideal for capturing essential release details.
Using tags allows teams to communicate clearly about versions, making it easier to coordinate and recall changes.
Benefits of Using Tags
There are several advantages to utilizing tags in your Git workflow:
- Marking specific points: Tags serve as landmarks in your project's development, enabling you to navigate easily to milestones like version releases.
- Easy identification: By tagging releases, you can quickly find and differentiate between various project states, improving the clarity of your versioning strategy.
- Facilitating collaboration: Teams can reference tags for collaboration, ensuring everyone is on the same page regarding release states and features.
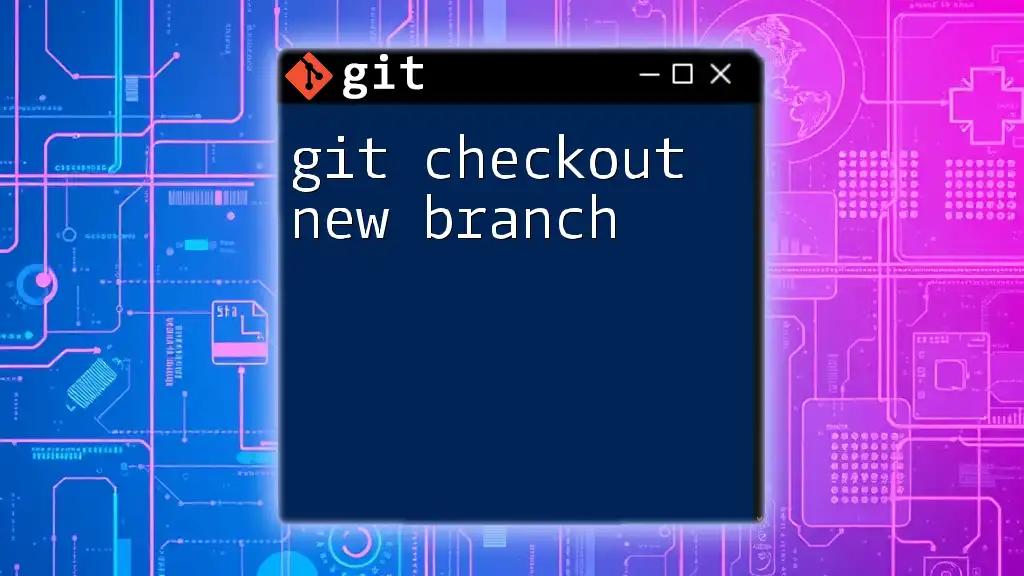
The `git checkout` Command
Overview of `git checkout`
The `git checkout` command is pivotal in navigating branches and restoring files in Git. It allows you to switch branches or retrieve a previous version of files.
Syntax of `git checkout`
The typical syntax for `git checkout` is:
git checkout [options] <branch|tag|commit>
For example, you might use it to switch to a branch:
git checkout <branch-name>
Alternatively, you can check out a specific tag, which puts you in a detached HEAD state:
git checkout <tag-name>
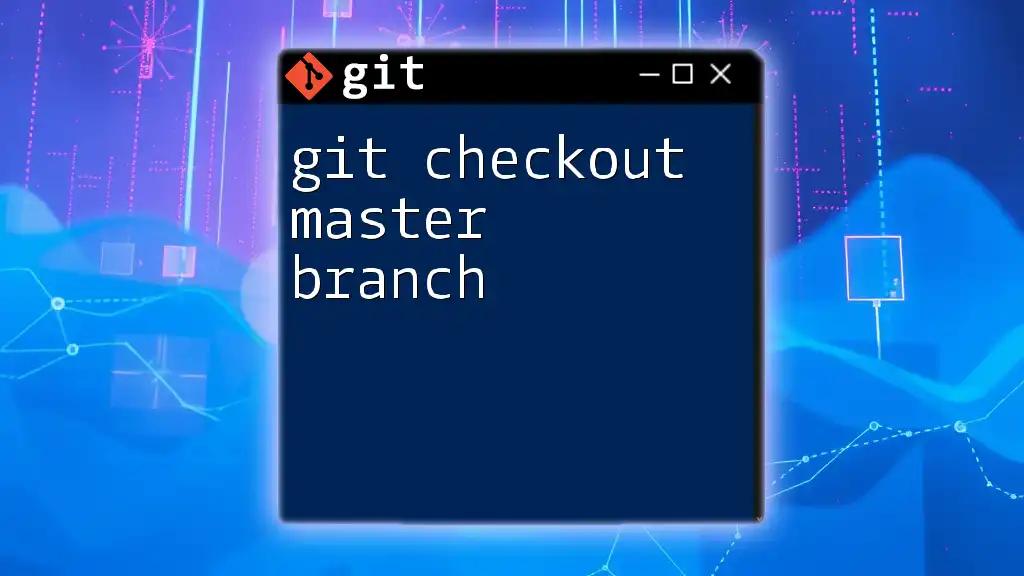
Checking Out a Tag as a New Branch
Why Create a Branch from a Tag?
Creating a new branch from a tag is beneficial for several reasons. It allows you to:
- Develop new features or fixes based on a stable version marked by a tag, without disrupting the main project.
- Capture specific changes which were carefully labeled in your project history, aiding in both development and backtracking.
Step-by-Step Guide to Checkout a Tag as a New Branch
Finding Available Tags
First, you need to know which tags are present in your repository. You can list all available tags using the following command:
git tag
This command will display all tags, helping you identify the one you wish to work from.
Checking Out a Tag
Once you've found the desired tag, you can check it out with:
git checkout <tag-name>
This puts you in a detached HEAD state, meaning you're no longer on a branch, but rather at a specific commit associated with that tag.
Creating a New Branch from the Checked-Out Tag
The next step is to create a new branch based on the checked-out tag. You can accomplish this with the following command:
git checkout -b <new-branch-name> <tag-name>
This command creates a new branch and switches you to it in one go. For example:
git checkout -b feature/awesome-feature v1.0
This command does two things: it creates a new branch named `feature/awesome-feature` from the `v1.0` tag and switches you to that new branch.
Example Walkthrough
Let’s consider a practical situation where you want to develop a feature based on an existing release termed `v1.0`. You would execute:
git checkout -b feature/awesome-feature v1.0
Upon execution, you will find that Git has:
- Created a new branch: `feature/awesome-feature`
- Checked out to this new branch, ready for development
You are now free to introduce changes, add features, and commit without affecting the original tag's state.
Best Practices When Working with Tags and Branches
To maximize the efficiency of working with tags and branches, consider the following best practices:
- Naming conventions: Make sure your branch names follow a consistent naming convention for clarity and organization. A good format is to prefix features and fixes, like `feature/` or `bugfix/`.
- Regularly update tags: As your project evolves, keep the tagging system updated to reflect new releases. This will prevent confusion and enhance collaboration.
- Manage branches effectively: After creating branches from tags, remember to regularly review and clean up old branches to maintain a tidy repository.
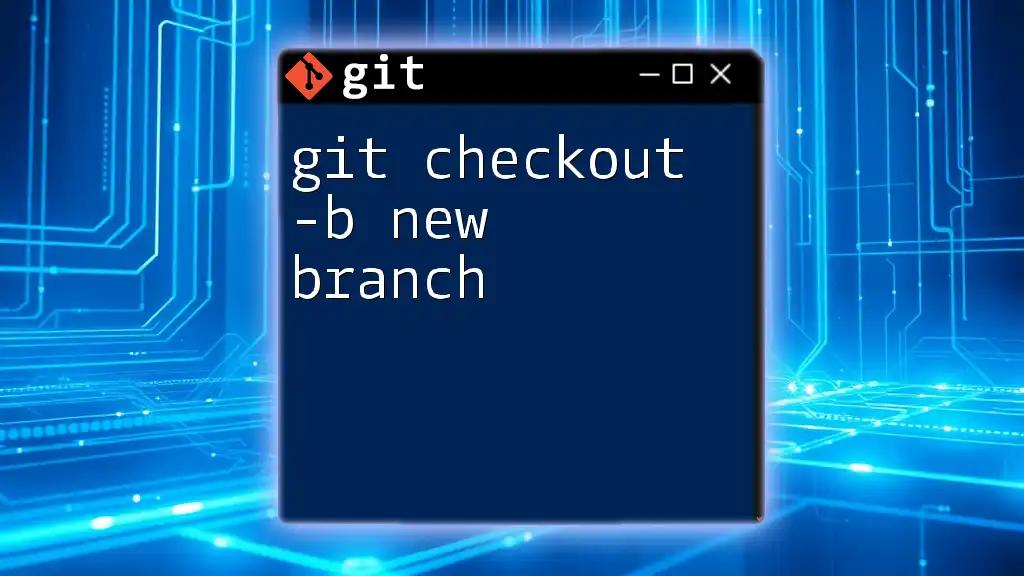
Conclusion
In summary, learning how to use the `git checkout tag as new branch` command significantly enhances your ability to manage your projects efficiently. By tapping into tags, you can develop new features or fixes based on specific, stable versions of your codebase. Practice these commands in your projects, and consider diving deeper into Git to discover its vast capabilities.
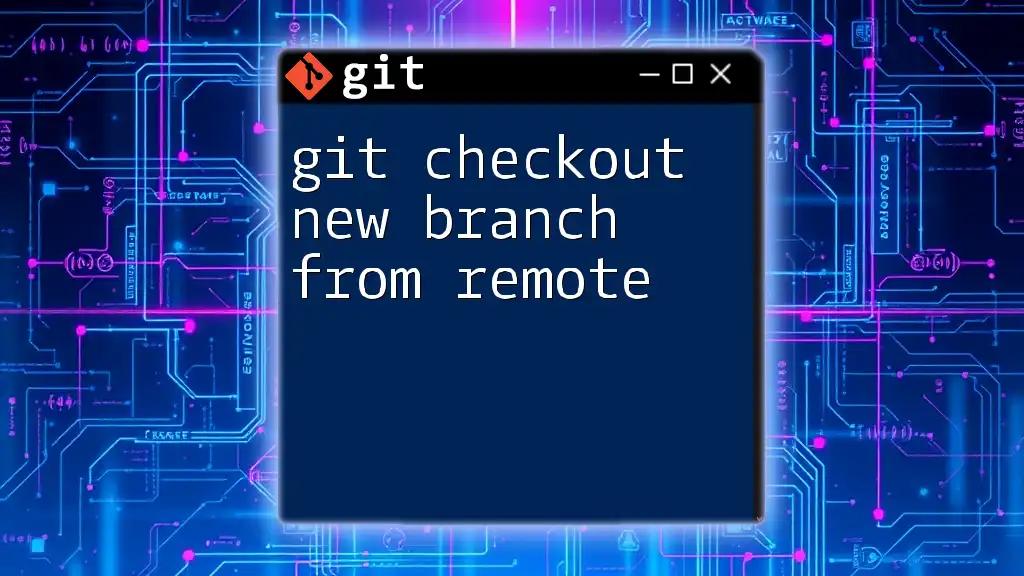
Additional Resources
For those interested in further enhancing their Git skills, consider exploring the official Git documentation or seeking out additional tutorials on Git commands and workflows. The more familiar you become with Git, the more proficient you will be in managing and collaborating on your projects!