To check out a remote branch and set up tracking for future pulls and pushes, use the following command in your terminal:
git checkout -b <branch-name> origin/<branch-name>
Understanding Remote Branches
What is a Remote Branch?
A remote branch represents the state of a branch on a remote repository, such as GitHub or GitLab. When you clone a repository, Git creates local copies of these remote branches. The remote branches are crucial for collaboration, as they allow multiple developers to work on different features without conflicts. Unlike local branches that exist only on your machine, remote branches reflect the project's state on a server.
In essence, understanding the relationship between local and remote branches is vital. Local branches are your working branches where you make changes, while remote branches serve as the shared reference points for collaboration.
Why Track a Remote Branch?
Tracking a remote branch means that your local branch is set to follow a specific remote branch. This setup simplifies workflows by allowing you to perform commands like `git pull` and `git push` without specifying the remote branch name each time.
The benefits of tracking include:
- Automatic Updates: When you pull changes, your local branch automatically integrates updates from the tracked remote branch.
- Easier Collaboration: Knowing which branches track one another fosters clear communication among team members.
- Simplified Merging: You reduce the complexity of merging changes across branches when you consistently work with tracked branches.
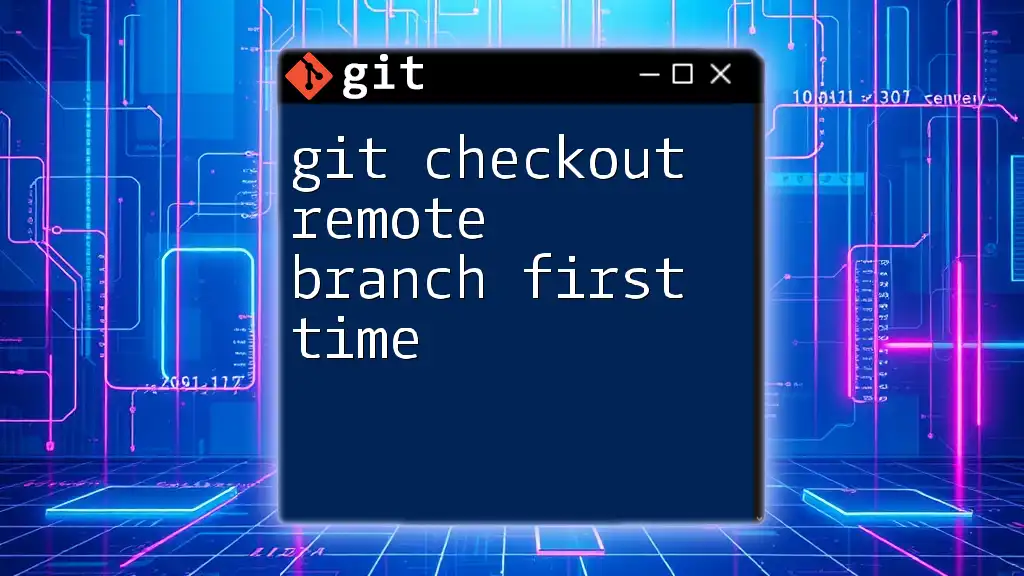
Prerequisites
Basic Understanding of Git Commands
Before diving into checking out remote branches, it's essential to have a grasp of basic Git commands. Familiarity with `git clone`, `git fetch`, and `git pull` will significantly ease your learning path. Git terminology, such as repository, commit, and branch, will also help clarify concepts discussed in this article.
Setting Up Your Environment
To use Git effectively, ensure it is installed and properly configured on your machine. Start by installing Git from [git-scm.com](https://git-scm.com), and then set up your user information:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
This configuration allows Git to associate your commits with your identity.
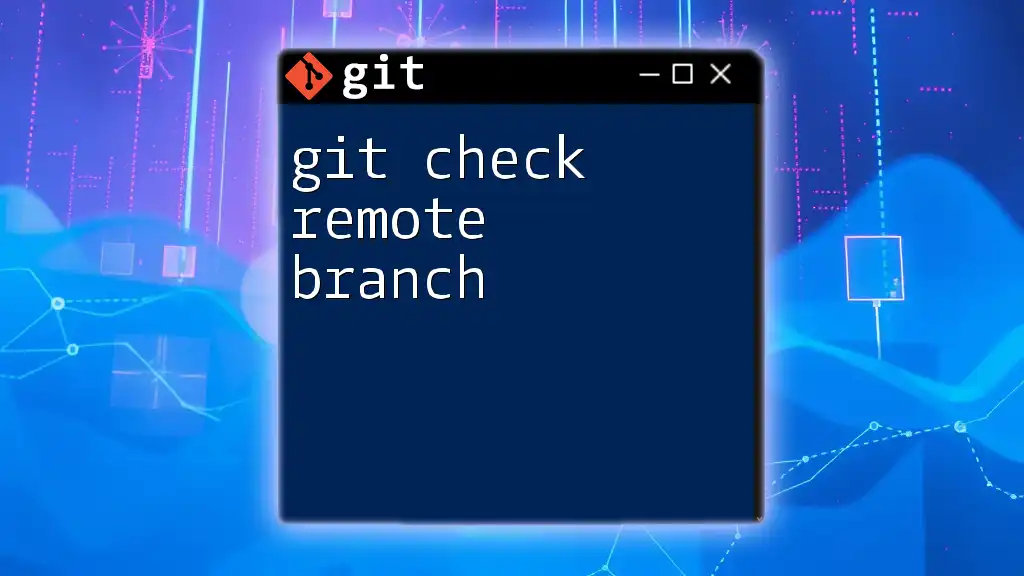
Checking Out a Remote Branch
Fetching Remote Branches
To work with a remote branch, you must first update your local repository's information. This is where the `fetch` command comes into play. Running this command ensures you have the latest references of remote branches:
git fetch origin
This command fetches all branches from the remote `origin` but does not merge or checkout any branches. It's a crucial step before checking out a specific branch.
Creating a Local Tracking Branch
Once you've fetched the remote branches, you can create a local tracking branch by using the `checkout` command combined with the `--track` option. This process links your local branch directly to the remote one, enabling seamless synchronization:
git checkout --track origin/branch-name
In the above command, replace `branch-name` with the name of the remote branch you want to check out. By using `--track`, your local branch is now set to track the specified remote branch.
What Does `--track` Do?
The `--track` option establishes a relationship between your local branch and the remote branch. This configuration ensures that when you run commands like `git pull` or `git push`, Git knows which remote branch to interact with.
For example, if you want to pull the latest changes from the remote branch after setting it up, you can simply run:
git pull
This command automatically pulls updates from the corresponding remote branch without needing to specify it.
Working with Remote Branches after Checkout
Viewing Your Current Branch
After checking out a remote branch, it's a good idea to verify which branch you are currently on. Use the following command to list all branches and highlight your current branch:
git branch
The current branch will be preceded by an asterisk (*), making it easy to identify.
Pulling Changes from the Remote Branch
Keeping your local branch updated with the latest changes from the remote branch is essential for seamless collaboration. Use the pull command as follows:
git pull
This command integrates changes from the remote branch into your local branch, allowing you to stay up-to-date with the latest developments from your team.
Switching Between Local and Remote Branches
It's common to work with multiple branches in a project. If you need to switch back to a different local branch, you can use:
git checkout local-branch-name
This flexibility allows you to manage various features and collaborate effectively without losing track of your work.
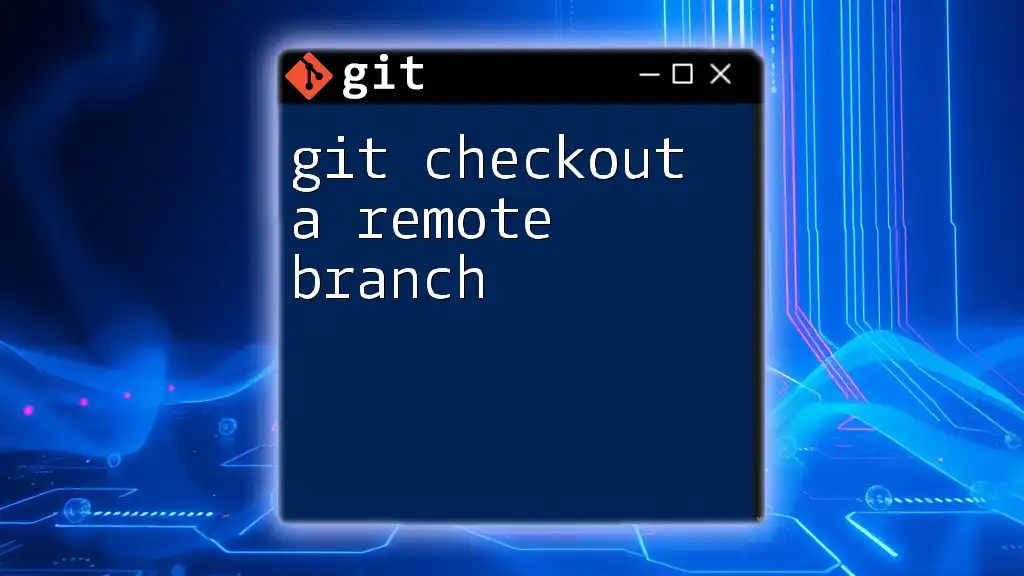
Troubleshooting Common Issues
Common Errors When Checking Out Remote Branches
While working with remote branches, you may encounter several issues, such as "branch not found" errors. This typically occurs when the specified branch does not exist on the remote repository. To troubleshoot, ensure you’ve fetched the latest branches using `git fetch origin`.
Additionally, if you face merge conflicts after pulling changes, Git will prompt you to resolve these conflicts before finalizing the merge. Carefully review the files with conflicts and make the necessary adjustments.
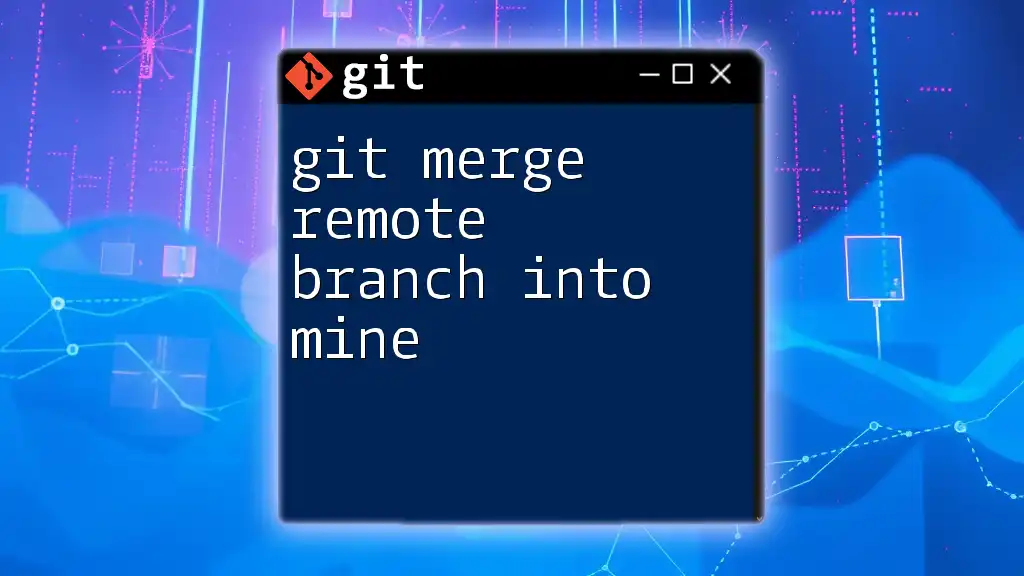
Best Practices for Using Remote Branches
Creating Descriptive Branch Names
When collaborating with others, it's crucial to use descriptive branch names. Meaningful names make it easier for your team to understand the purpose of each branch. For instance, using `feature/login-system` is more informative than simply calling it `feature1`.
Regularly Syncing Your Branch
To maintain harmony in collaboration, regularly sync your branch with the remote one. Use `git fetch` to check for updates and `git pull` to integrate changes frequently. This habit minimizes the risk of significant merge conflicts later.
Keeping Your Local Repository Clean
To keep your repository clean and organized, it’s a good practice to delete branches that have been merged successfully. Use the following command to delete a merged branch:
git branch -d branch-name
This cleanup helps maintain a tidy workspace, making it easier to focus on active development efforts.
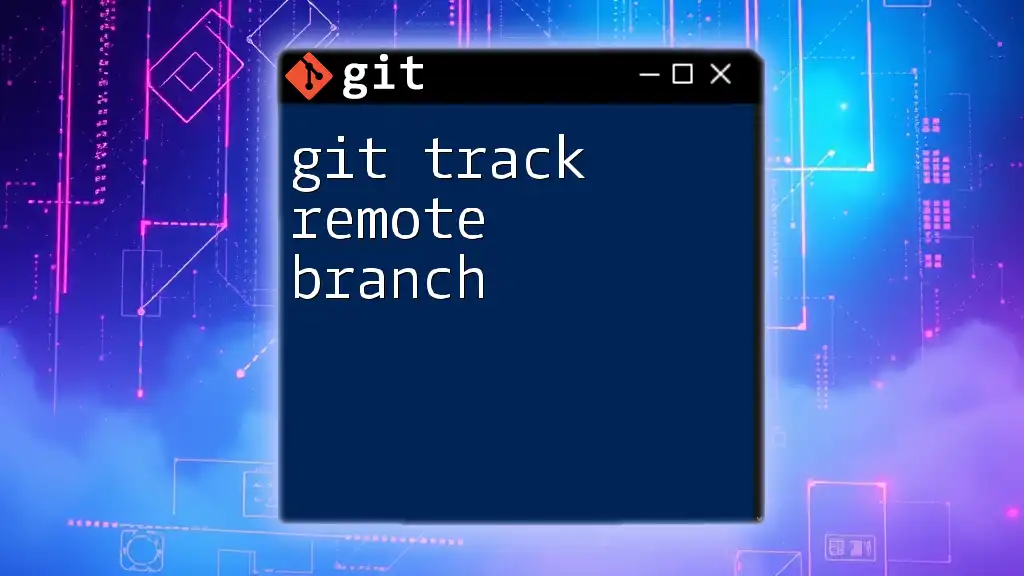
Conclusion
Understanding how to git checkout remote branch with tracking is an invaluable skill for any developer working in a collaborative environment. By establishing tracking relationships and keeping branches updated, you can ensure smooth workflows and reduce the complexity of collaborating with your team. We encourage you to practice these commands and reinforce your learning, as mastering Git will undoubtedly enhance your development experience.
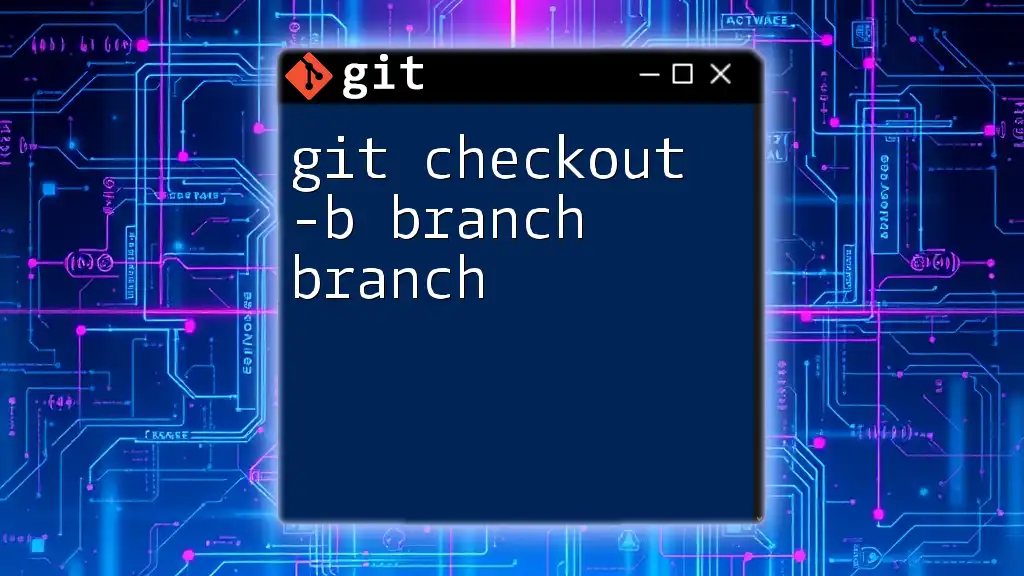
Additional Resources
For further exploration, visit the official Git documentation, which provides in-depth explanations of commands and features. You might also consider online courses or tutorials focusing on Git best practices to deepen your understanding.
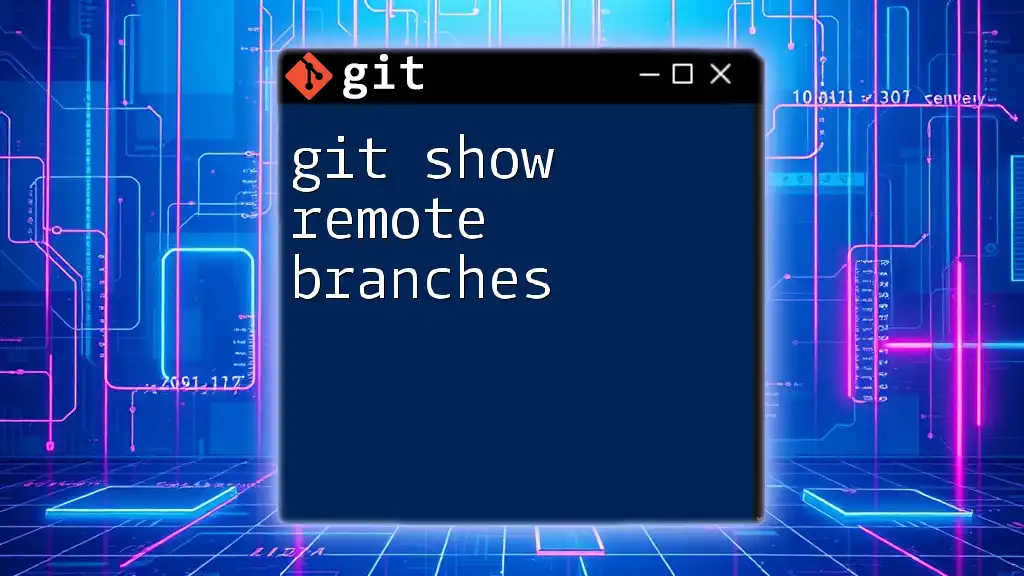
Call To Action
Have experiences or tips about working with remote branches? Feel free to share them in the comments below! Your insights will help fellow developers enhance their Git skills and collaborative efforts.