In Git, local branches are separate lines of development that allow you to work on different features or fixes without affecting the main codebase, and you can create a new branch using the following command:
git checkout -b new-branch-name
Introduction to Git Branching
A Git branch represents a separate line of development within a Git repository. Branching is essential for managing different features, bug fixes, or experiments in isolation without affecting your main codebase. When we talk about git branches on my local, we refer to branches created and managed directly on your local machine rather than on a remote repository like GitHub or GitLab.
The difference between local and remote branches is crucial to understand. Local branches are those created in your own workspace, meaning only you can see and interact with them until you push them to a remote repository. This separation allows developers to work independently without disrupting the main project until they're ready.
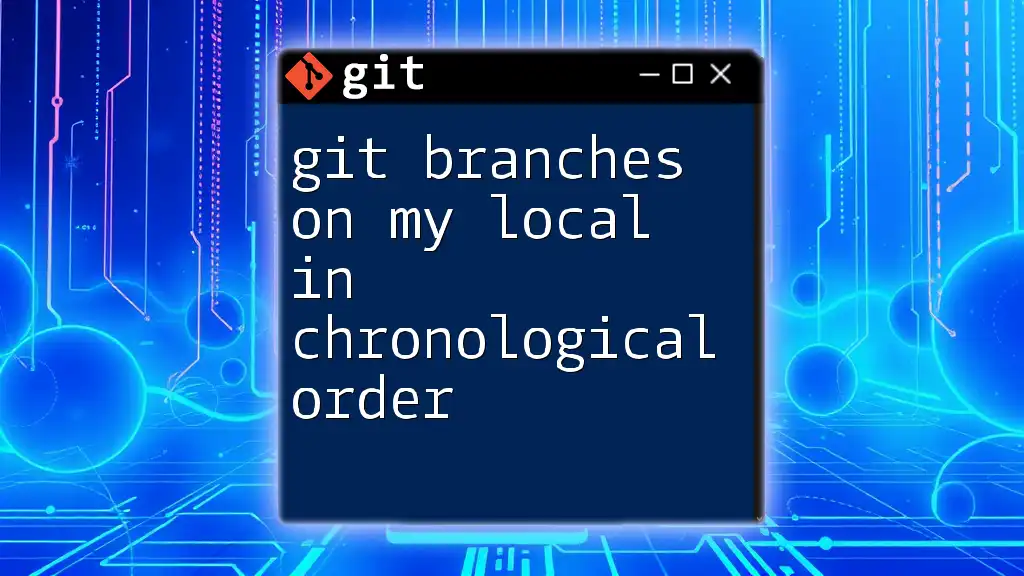
Creating a New Local Branch
Using the `git branch` Command
To create a new local branch, you can use the `git branch` command followed by the name you want for the new branch:
git branch <branch_name>
For example:
git branch feature/login
After executing this command, a branch named `feature/login` will be created. However, you won't automatically switch to this branch; you will still be on your current branch. This command is useful when you want to prepare your branches without affecting your current work.
Creating and Switching with `git checkout`
For most scenarios, you will want to create and switch to a new branch simultaneously. You can do this with the `git checkout` command using the `-b` option:
git checkout -b <branch_name>
For instance:
git checkout -b feature/signup
This will create the `feature/signup` branch and immediately switch you to it. Doing this in one command saves time and keeps your workflow efficient.
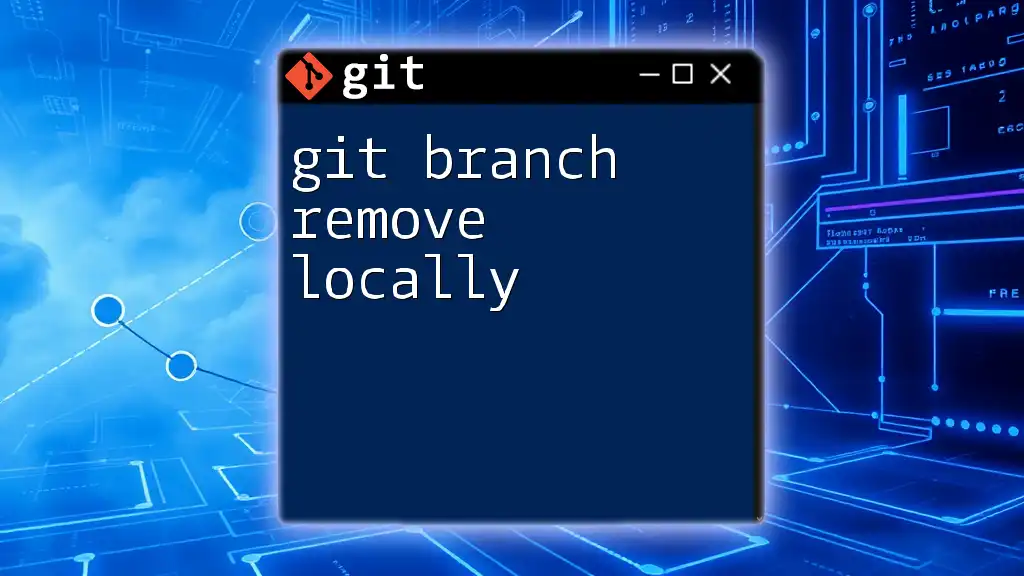
Listing Local Branches
Showing Available Branches
To see a list of all your local branches, simply use the following command:
git branch
This command will generate a list that indicates your current branch with an asterisk (*) next to it. The output might look something like this:
* main
feature/login
feature/signup
Understanding this output is crucial. The asterisk identifies which branch you are currently working on, helping you keep track of your current development context.
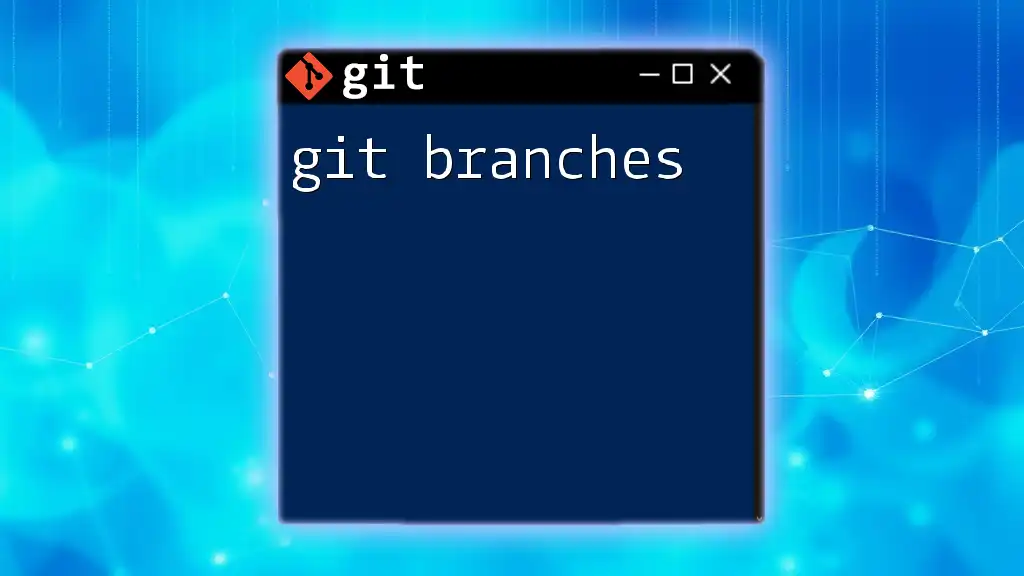
Switching Between Local Branches
Using `git checkout`
Switching between branches is easy with the `git checkout` command:
git checkout <branch_name>
For example, if you want to move to a branch named `develop`, you would enter:
git checkout develop
It's important to note that you may encounter merge conflicts when switching branches if there are uncommitted changes in your working directory. Git will alert you if these exist, preventing the switch until you resolve the issues.
Using `git switch` (Newer Command)
A newer and more intuitive command for switching branches is `git switch`:
git switch <branch_name>
For example:
git switch feature/update-UI
The `git switch` command simplifies the process, making it more user-friendly, especially for those new to Git. This command's introduction is part of the Git team's effort to enhance the clarity of commands in version control.
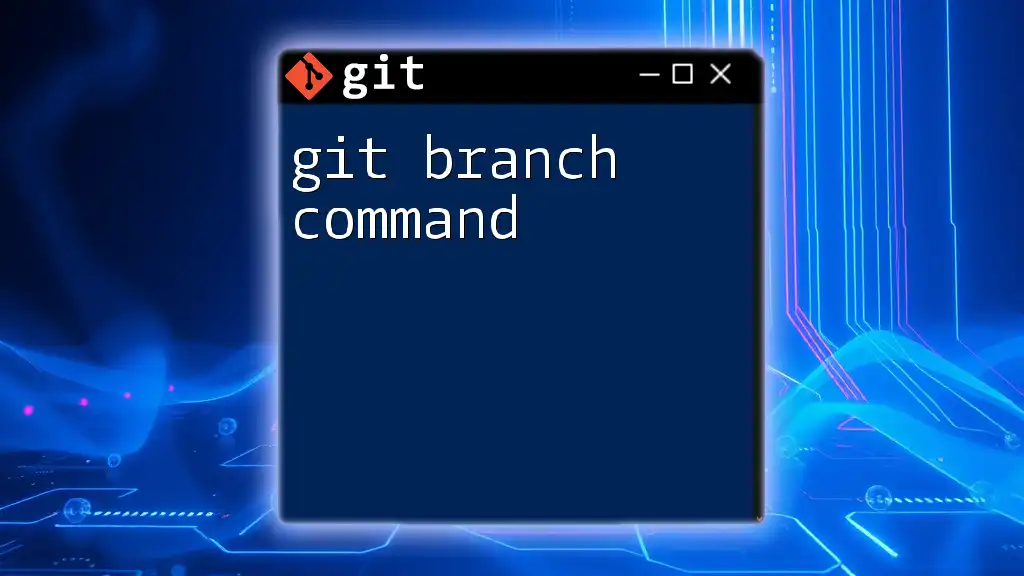
Deleting Local Branches
When to Delete a Branch
It's important to clean up branches that are no longer needed. This typically includes branches that have been merged or are no longer relevant to your project.
Using `git branch -d` Command
To delete a branch that you've already merged, use:
git branch -d <branch_name>
For instance:
git branch -d feature/old-feature
This command safely deletes the branch, ensuring you don't accidentally lose unmerged changes. If you attempt to delete a branch that has unmerged changes, Git will prevent the deletion. In such cases, you can use the `-D` option to force the deletion:
git branch -D feature/old-feature
While this command can be handy, use it with caution, as it can lead to unintended data loss.
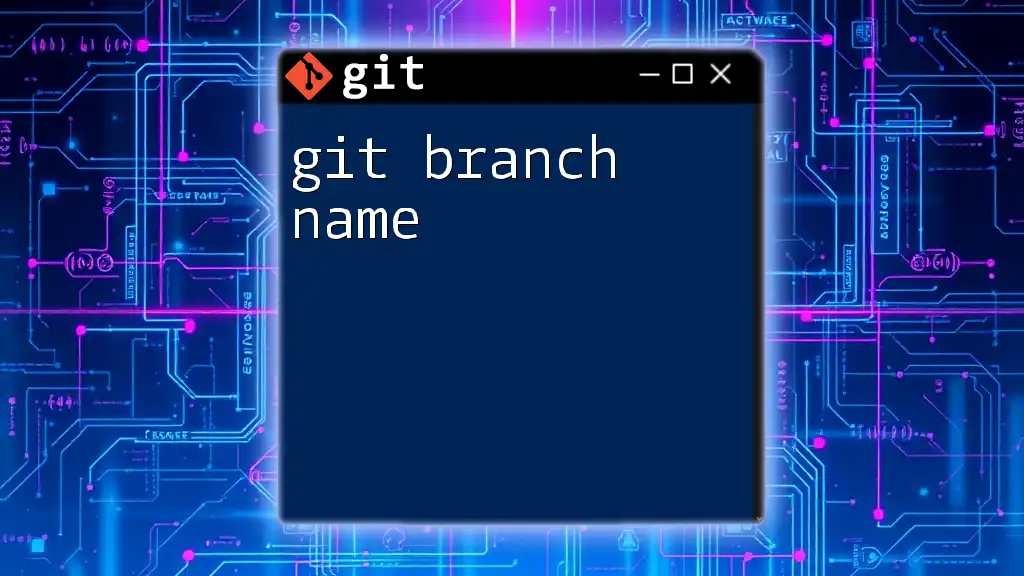
Renaming Local Branches
Changing a Branch Name
Renaming branches is simple and can help maintain clarity within your Git repository. To rename a branch, use the `-m`:
git branch -m <old-branch-name> <new-branch-name>
For example:
git branch -m old-branch new-branch
Renaming branches can be especially important for keeping your workflow organized, especially as a project evolves and the significance of branches changes.
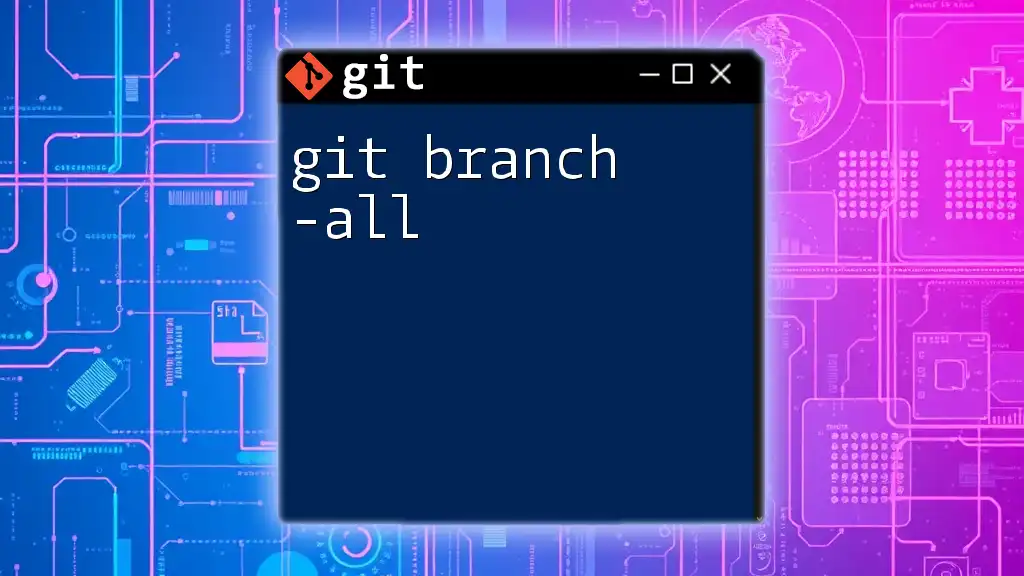
Merging Local Branches
Basic Merge Process
To integrate changes from one local branch into another, we use the `git merge` command. First, switch to the branch you want to merge into, often the main or development branch:
git checkout main
Then merge the feature branch:
git merge feature/add-payment
After executing this command, Git will attempt to combine the changes. If there are conflicts, they will need to be resolved before the merge can complete. Conflict resolution involves editing the files to resolve discrepancies, adding the resolved files, and then completing the merge with:
git commit
Understanding merging is essential in collaborative environments, where multiple developers may work on different features simultaneously.
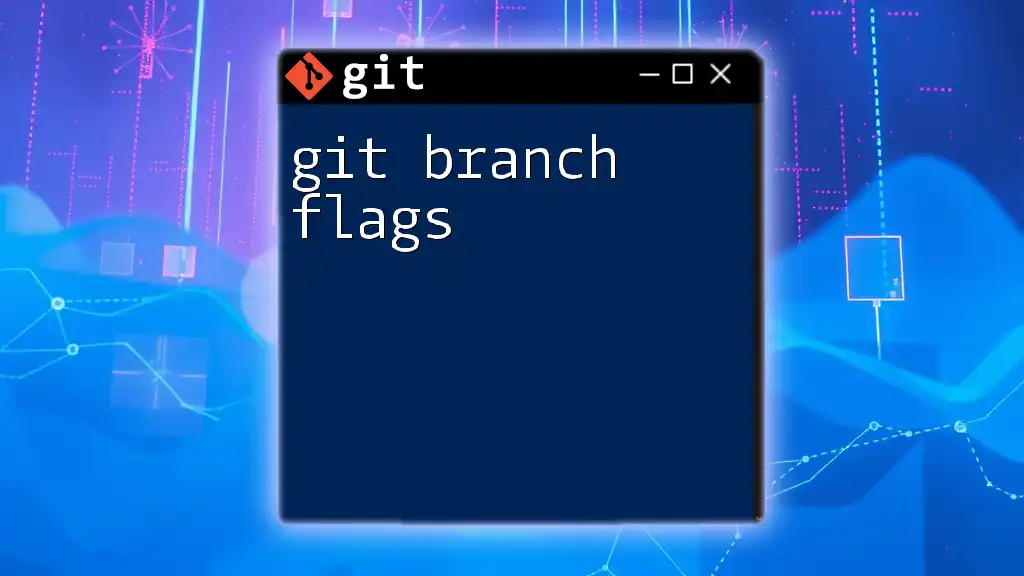
Conclusion
In conclusion, mastering git branches on my local allows for effective management of features, bug fixes, and experimental work by providing a structured approach to development. Each command and concept discussed enhances your ability to maneuver within a Git repository, ensuring that your codebase remains clean and organized.
As you become comfortable with these basic commands, I encourage you to delve into more advanced Git techniques such as rebasing, cherry-picking, and managing remote branches to further enhance your workflow.
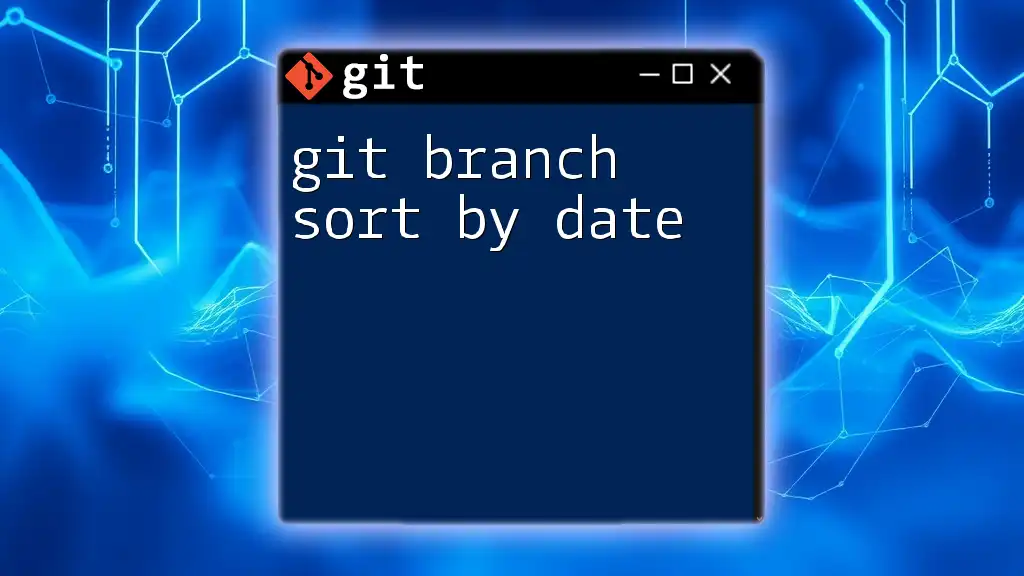
Additional Resources
To extend your learning, consider exploring the following resources:
- Git official documentation for detailed command explanations.
- Online tutorials or courses that focus on advanced Git usage.
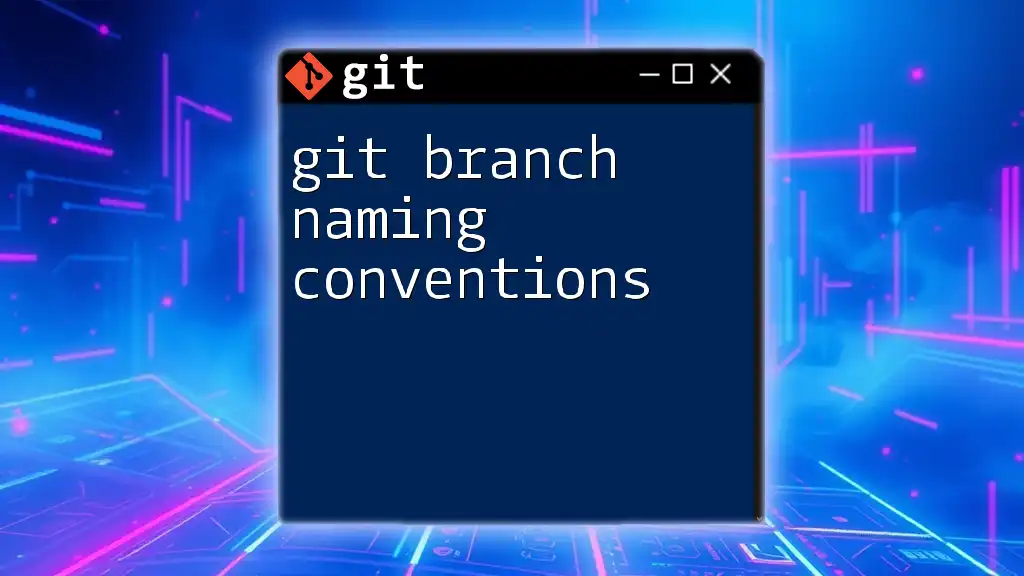
Call to Action
If you found this guide helpful, be sure to subscribe for more insightful tips on Git commands and share your experiences with git branching in the comments below!