To view the dates of the last commit on each branch in your Git repository, you can use the following command:
git for-each-ref --sort=-committerdate --format='%(refname:short) %(committerdate:relative)' refs/heads/
What Are Branch Dates?
Branch dates refer to the timestamps associated with Git branches, specifically the creation date and the date of the most recent commit. Understanding these dates is vital for effective project management, as they provide insight into when features were developed and how long they have been maintained.
Why View Branch Dates?
Viewing branch dates has multiple applications:
- Project Management: Knowing when a branch was created can help in assessing the age of features and evaluating the project timeline.
- Code Review: Awareness of the last modified date can guide reviewers in prioritizing which branches to assess first.
- Debugging: Understanding when a change was introduced helps to trace back issues that may arise during code integration.
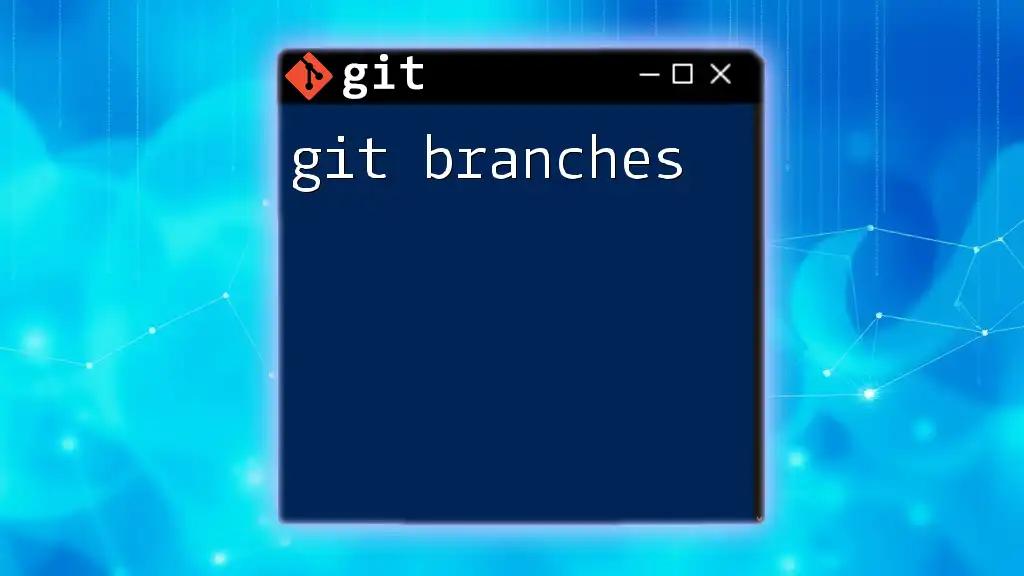
How to View Branch Dates
To check branch dates in Git, you can use several commands designed to reveal branch information and commit histories.
Checking Branch Creation Date
The `git show` command allows you to view detailed information about a branch, including its creation date. The format for retrieving the creation date involves specifying the branch name and adjusting the output format.
Example usage:
git show --format="%ai" <branch-name>
Breakdown of the Output: The command returns the creation date in the format `YYYY-MM-DD HH:MM:SS`. This timestamp represents the exact moment the branch was created and is crucial for understanding the development timeline.
Finding the Last Commit Date on a Branch
To discover the last commit date for a particular branch, the `git log` command is a powerful tool. By using this command, you can quickly locate the most recent commit and its timestamp.
Example usage:
git log <branch-name> -1 --format="%cd"
This command retrieves just the last commit, displaying its date in the standard format. The last commit date is significant as it reflects updates and changes made to the branch, which is essential for maintaining current project status.
Listing All Branches with Their Dates
When managing multiple branches, it may be necessary to get a comprehensive view of all branches along with their creation dates. The `git for-each-ref` command is suitable for this purpose, as it lists each branch and sorts them according to their creation dates.
Example command:
git for-each-ref --sort=creatordate --format='%(creatordate:short) %(refname:short)' refs/heads/
This command outputs a list of branches along with their creation dates. It is especially useful for prioritizing branches based on their age, aiding in effective project management.
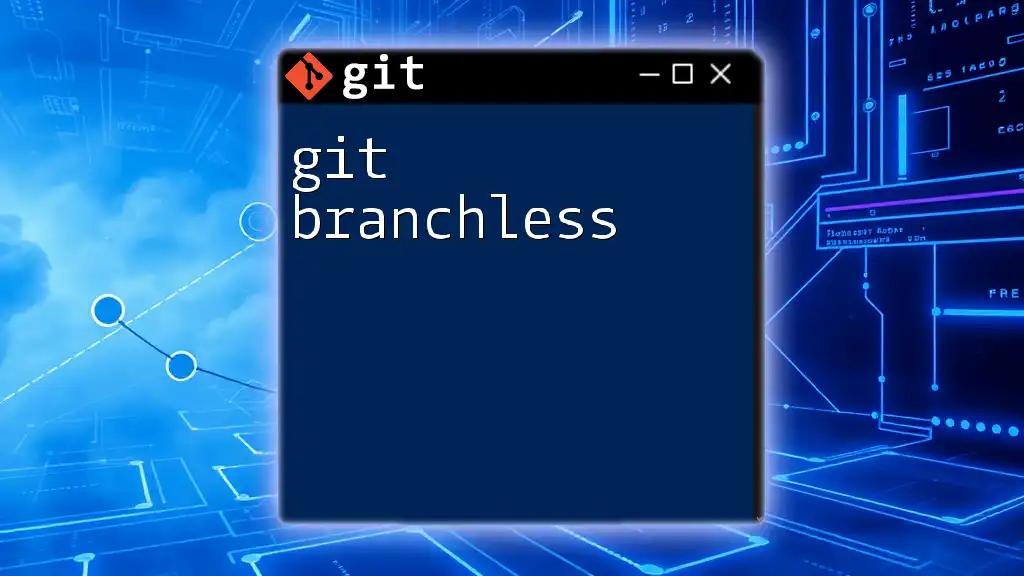
Understanding the Output
Interpreting Date Formats
When using Git commands to retrieve dates, it’s important to be able to interpret the formats provided. Git generally uses the ISO 8601 format, which looks like `YYYY-MM-DD HH:MM:SS ±HHMM`. The breakdown of the output reveals both the date and the time, making it easy to ascertain when actions were taken.
Time Zones and Date Representation
Git handles time zones explicitly in its output; by default, the timestamps are shown in UTC. It’s essential to keep this in mind, especially when teams are distributed across various time zones. Understanding the time representation allows you to coordinate contributions and changes more effectively.
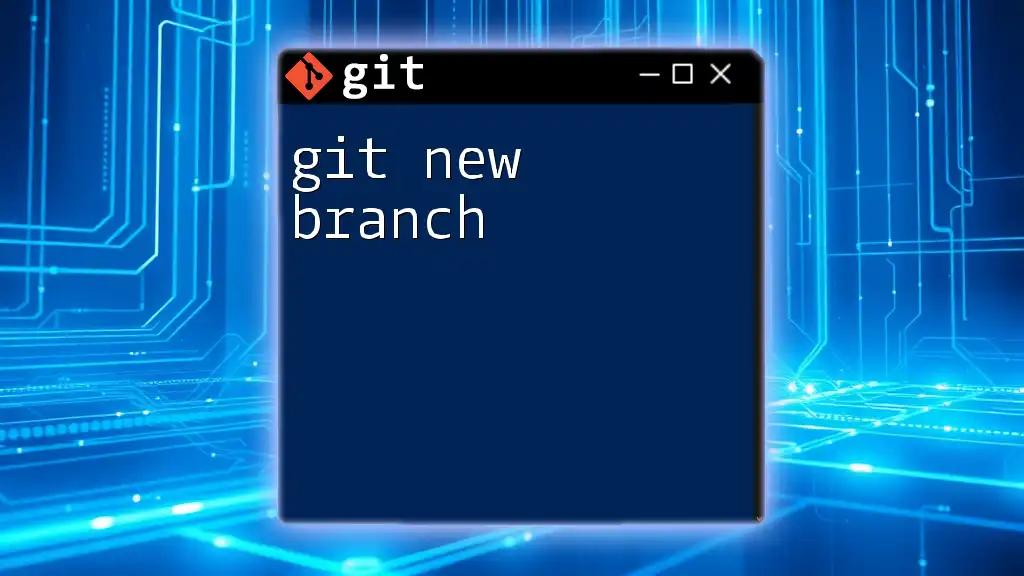
Advanced Techniques
Checking Differences between Branch Dates
In some cases, you may want to trace back history or differences between branch dates. The `git reflog` command can show you a chronological order of all operations associated with a branch, including commits and checkouts.
Example command:
git reflog show <branch-name>
The output lists commits along with their timestamps. This detailed history is invaluable for tracking changes and understanding the evolution of your codebase.
Custom Scripts for Branch Date Management
For enhanced usability, you can develop a simple Bash script that iterates over branches and outputs their creation dates. Here’s an example of such a script:
#!/bin/bash
for branch in $(git branch --format='%(refname:short)'); do
echo "$branch: $(git show -s --format=%ci $branch)"
done
This script lists each branch followed by its creation date. Custom scripts like this one can be adjusted for specific needs, allowing for consistent and efficient management of branch date information.
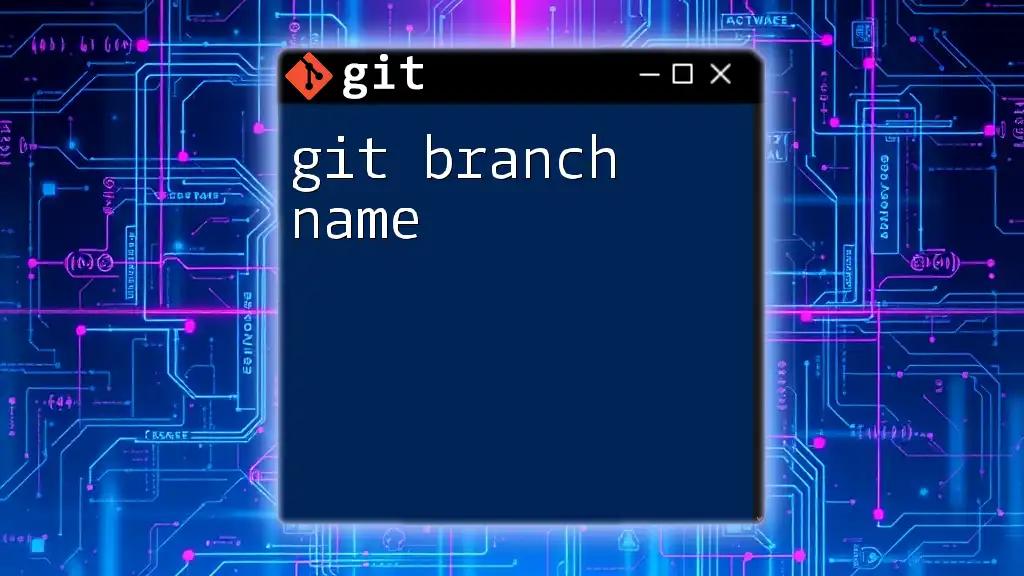
Common Issues and Troubleshooting
Common Errors when Retrieving Dates
While viewing branch dates, users might encounter errors such as “branch not found.” This can commonly occur if the branch name is misspelled or if you are in a repository that has not been properly initialized.
How to Resolve Output Format Issues
If you encounter confusion over date output formats, you can specify different formats using the `--date` option available in many git commands. For example, to view relative dates, you could use:
git log <branch-name> --date=relative
This flexibility in date formats allows you to adapt the output to suit your project’s needs and your team’s understanding.
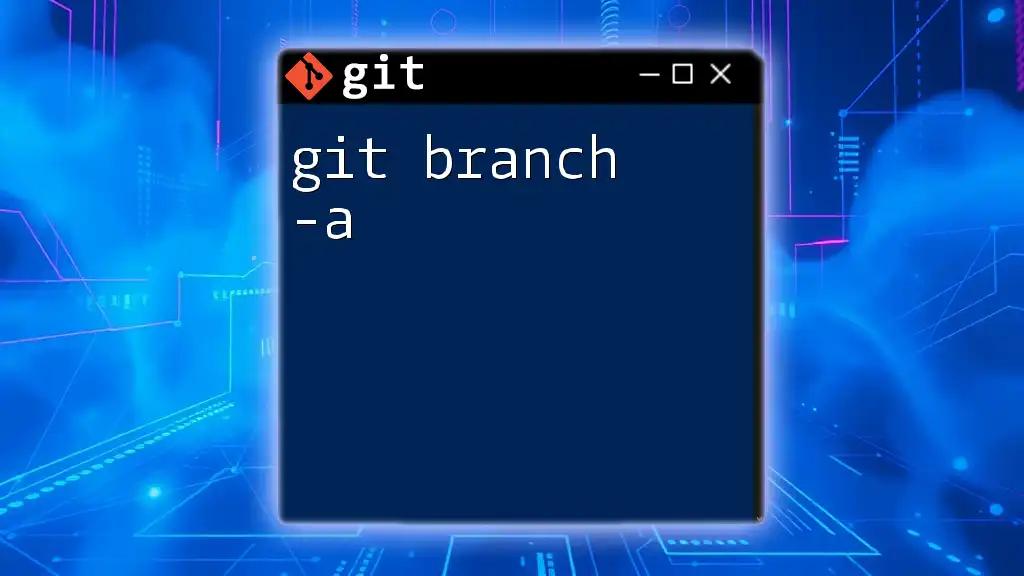
Conclusion
Managing and viewing branch dates is a fundamental aspect of leveraging Git effectively. Whether you are managing a single branch or coordinating across a large team, the ability to consult branch creation and last modified dates can significantly impact your workflow. By mastering the commands and techniques outlined in this guide, you will enhance your productivity and improve your project’s organization.
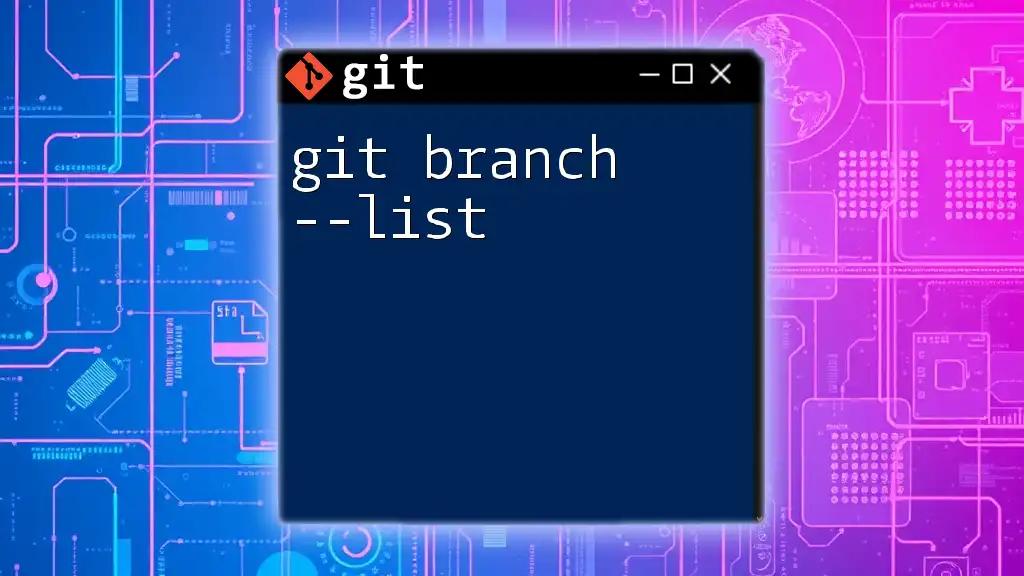
Additional Resources
For further reading on Git and branching strategies, you can refer to the official Git documentation, where you will find comprehensive guides and tutorials tailored for both beginners and advanced users. Engaging with online communities and forums dedicated to Git can also provide additional insights and best practices for your continuous learning journey.