The `git reset --soft HEAD~1` command is used to undo the last commit while keeping changes staged in the index, allowing you to modify or amend it before re-committing. Here's the command in a code snippet:
git reset --soft HEAD~1
Understanding Git Basics
What is Git?
Git is a distributed version control system designed to manage code changes in a collaborative environment. Its core purpose is to allow multiple developers to work on a project simultaneously without interference, keeping track of every modification made to files.
The importance of version control in software development cannot be overstated. It provides a safety net for changes, allowing developers to revert to earlier versions when necessary, compare revisions, and collaborate efficiently.
Core Concepts of Git
To grasp the mechanics of git reset to main head soft, it’s essential to familiarize yourself with some core concepts of Git, including:
- Repositories: A repository contains all files associated with a specific project, including its full history.
- Commits: A snapshot of changes made to the repository at a certain moment, each identified by a unique hash.
- Branching: The ability to diverge from the main line of development to achieve various goals without disrupting the main codebase.
- The Concept of HEAD: HEAD is a pointer that indicates the current branch reference or the most recent commit.
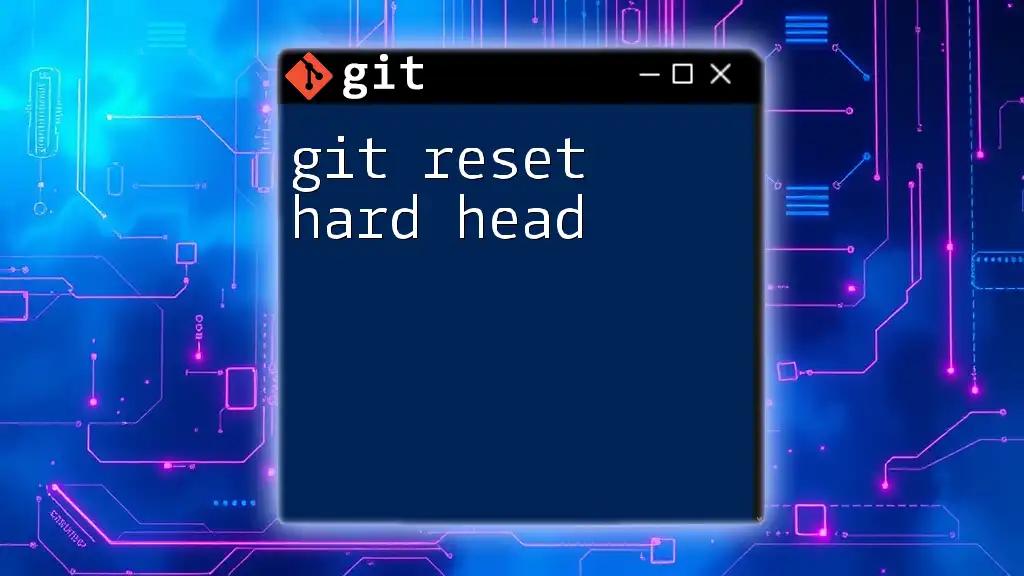
What is `git reset`?
Definition of `git reset`
The `git reset` command is one of the most powerful commands in Git, allowing you to rewind the commit history of your repository. It serves to undo commits, and its effects can vary depending on the options you provide. It's important to distinguish `reset` from other commands like `revert` and `checkout`, which serve different purposes in maintaining code history.
Types of Reset
Understanding the variations of `git reset` is critical:
- Soft: A soft reset keeps your changes in the staging area (index), ready for another commit. It only alters the commit history.
- Mixed: The default option when no argument is provided. It resets the index but not the working directory, leaving changes unstaged.
- Hard: A hard reset resets the index and working directory to match the specified commit. This discards changes permanently.
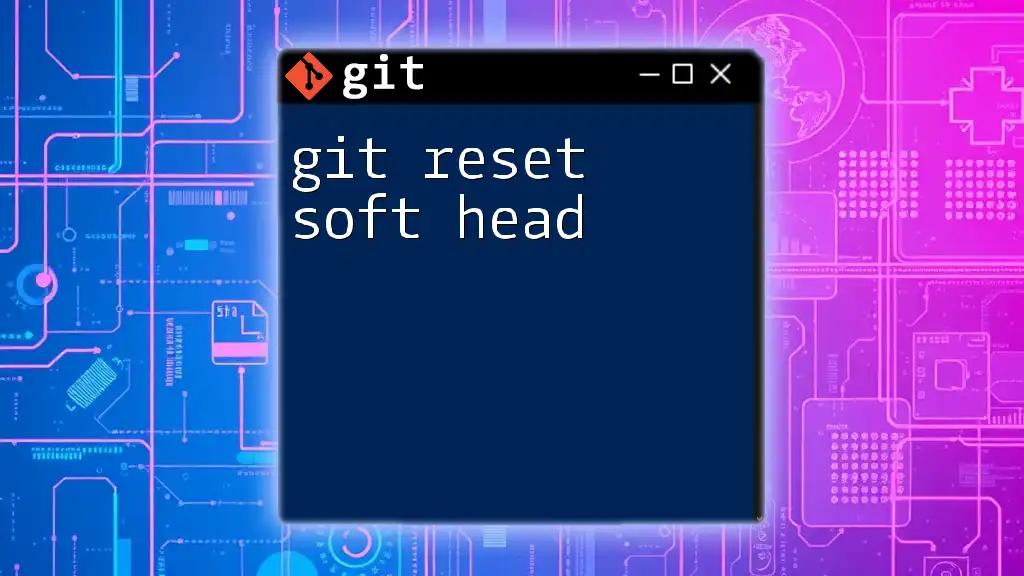
The Concept of HEAD in Git
What is HEAD?
HEAD in Git is a term used to denote the current commit your repository is operating on. It usually points to the latest commit in the current branch, indicating your current location in the project's history.
Main vs. Current HEAD
Every Git repository usually has a main branch (commonly named `main` or `master`). Understanding how HEAD interacts with this and other branches is integral to using Git effectively. The main branch is typically where the stable code resides, while other branches may contain work in progress or experimental features.
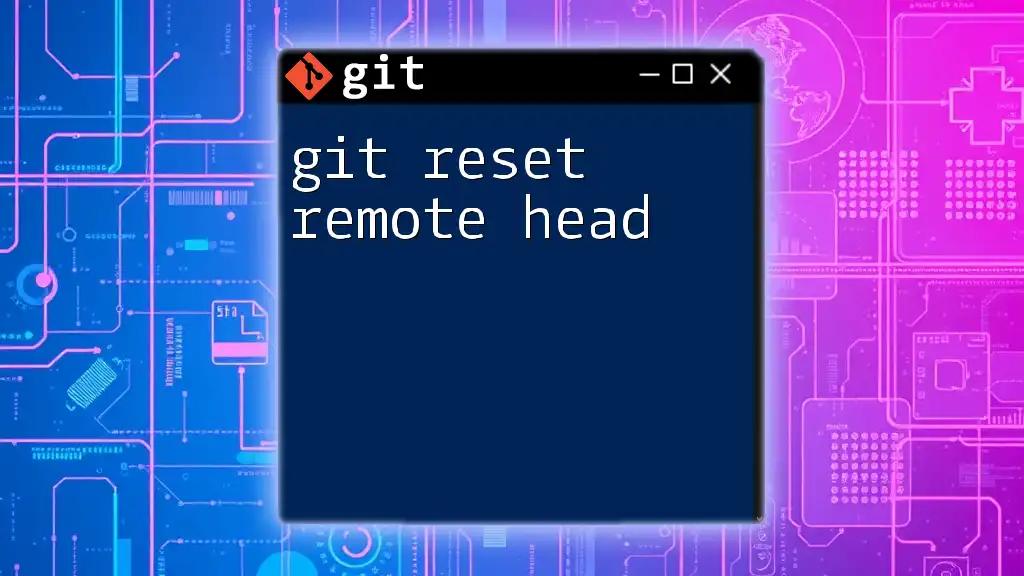
The `git reset HEAD --soft` Command
What Does `git reset HEAD --soft` Do?
Using `git reset HEAD --soft` effectively takes your repository’s HEAD back to the last commit while retaining all of your changes in the staging area. This means that your modifications are not lost; you can simply commit them again or make further adjustments. This feature is beneficial for cases where you need to modify your commit.
Use Cases
There are several scenarios where `git reset HEAD --soft` can prove beneficial:
- Undoing the Last Commit: If a commit was made too hastily, this command allows you to revert to the state just before the commit, while still keeping the changes in your staging area for further modifications.
- Preparing for a Rework: If you realize your last few commits should be restructured or split into multiple parts, this command lets you reset your commit history without losing your work.
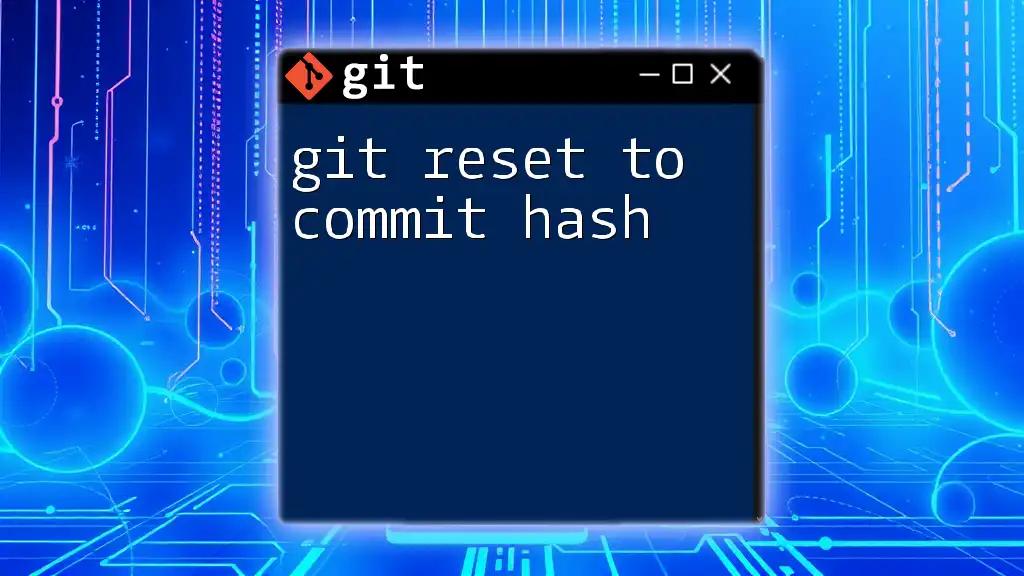
Step-by-Step Usage of `git reset HEAD --soft`
Step 1: Open Your Terminal or Command Line
First, ensure that you are in an environment where you can run git commands. If you are new to git, consider using a Bash terminal or any command line interface that supports Git commands.
Step 2: Navigate to Your Git Repository
Change your directory to the local repository where you want to execute the reset command. Use the following command:
cd path/to/your/repo
Step 3: Check Current Status
Before making any changes, check the current status of your repository to understand where you stand:
git status
This will help you see what has been staged and what commits exist.
Step 4: Execute `git reset HEAD --soft`
When you're ready to reset, you can use the following command. This will take you back to the last commit while keeping your changes staged.
git reset HEAD --soft
Step 5: Verifying Changes
After the reset, you can verify what remains staged and what has changed:
git status
This command will show you the files that are still in the index, ready to be re-committed.
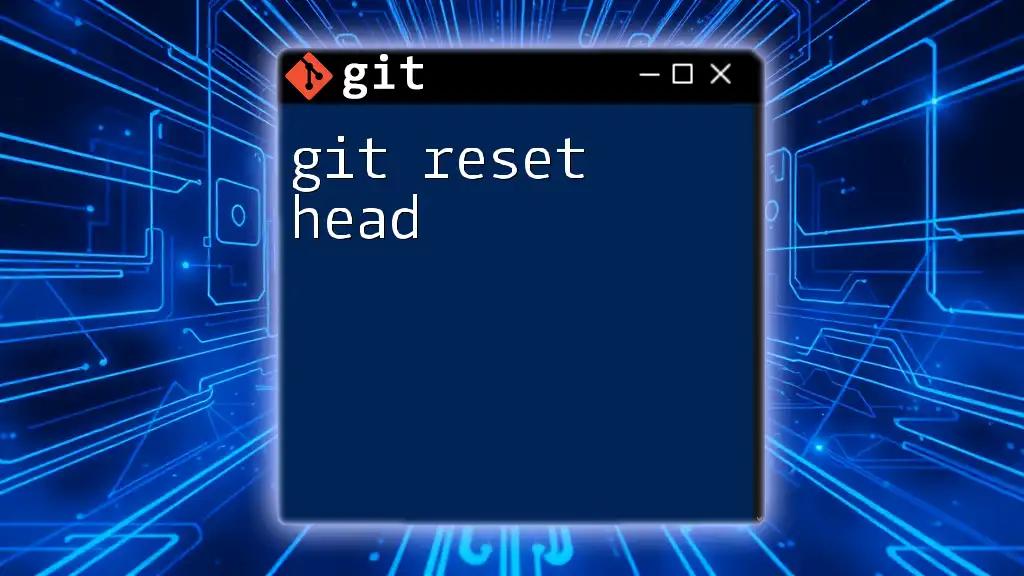
Understanding Side Effects
What Happens After Using `git reset --soft`?
After executing `git reset HEAD --soft`, the commit you targeted will be removed from your history in the current branch, but any modifications you made will still be in the staging area. This balance allows for real-time adjustments without losing any work.
You can check the commit history even after a reset using:
git reflog
This command shows you a log of changes made, including those removed commits, allowing you to recover if needed.
Potential Pitfalls
Using `git reset HEAD --soft` can be powerful, but it also requires caution. If you aren't aware of what changes you are making or if you misuse the command, you might end up in a confusing state. Understanding the implications of the reset is crucial, and if mistakes occur, you can typically find recovery options through `git reflog`.
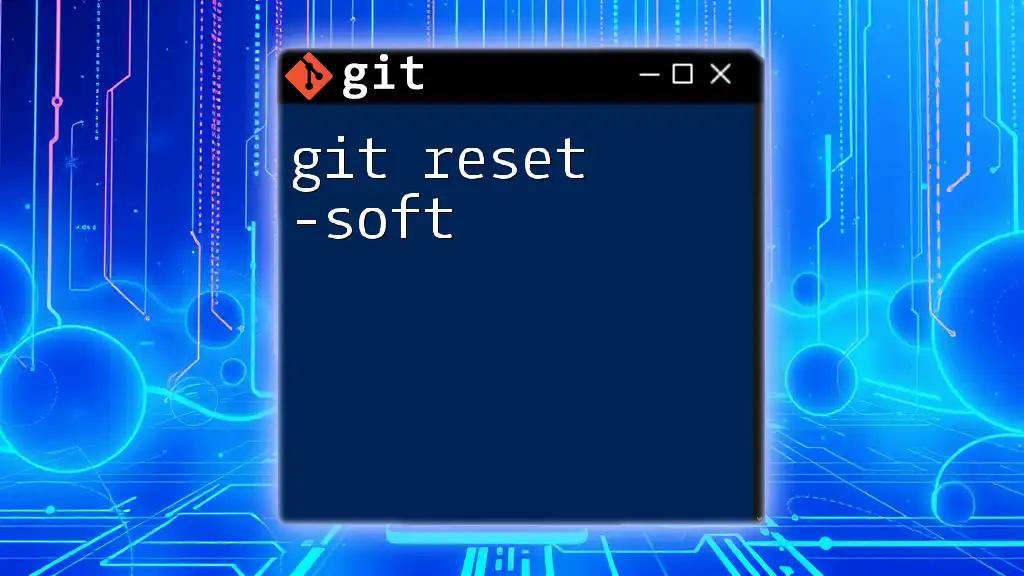
Practical Examples
Example 1: Removing the Last Commit
Let’s say you accidentally committed without including a necessary change. You can undo this last commit while keeping changes:
git reset HEAD~1 --soft
This command takes you back one commit and retains all changes in your staging area, allowing you to fix and re-commit as needed.
Example 2: Combining Changes
You can also use a soft reset to consolidate multiple commits into one. After performing several commits that should logically be grouped, reset to the earliest of those commits and stage the changes again:
git reset HEAD~3 --soft
This will bring everything back three commits but keep all changes ready for a single, improved commit.
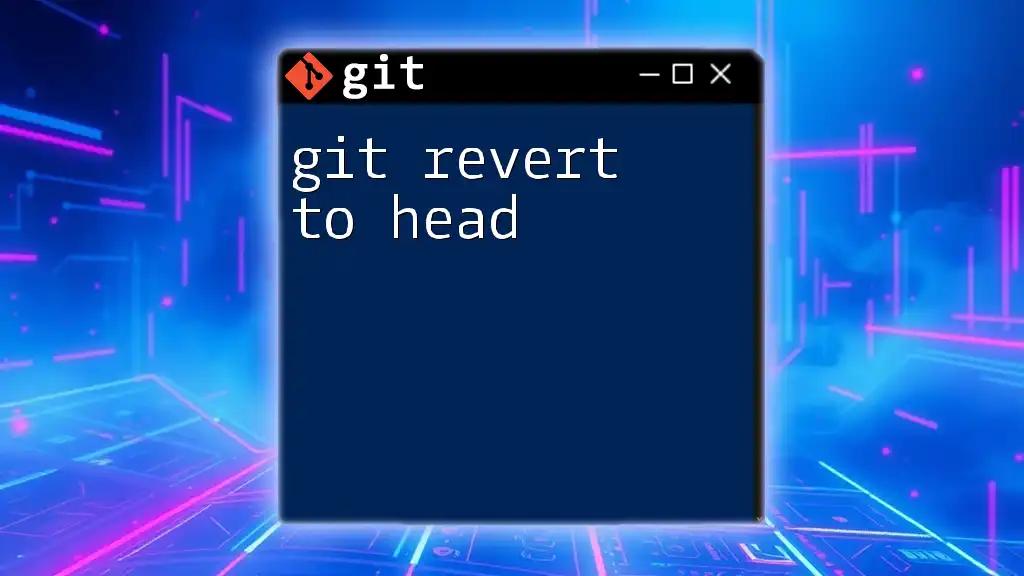
Conclusion
Summary of Key Points
In this guide, we explored how to use the command `git reset to main head soft`, understanding its importance and utility within the Git environment. This command provides a way to undo commits while preserving your modifications in the staging area, enabling you to make the necessary edits or reconsolidate your commit history.
Next Steps
For those looking to deepen their knowledge, consider exploring advanced Git commands or pursuing training classes on Git functionalities.
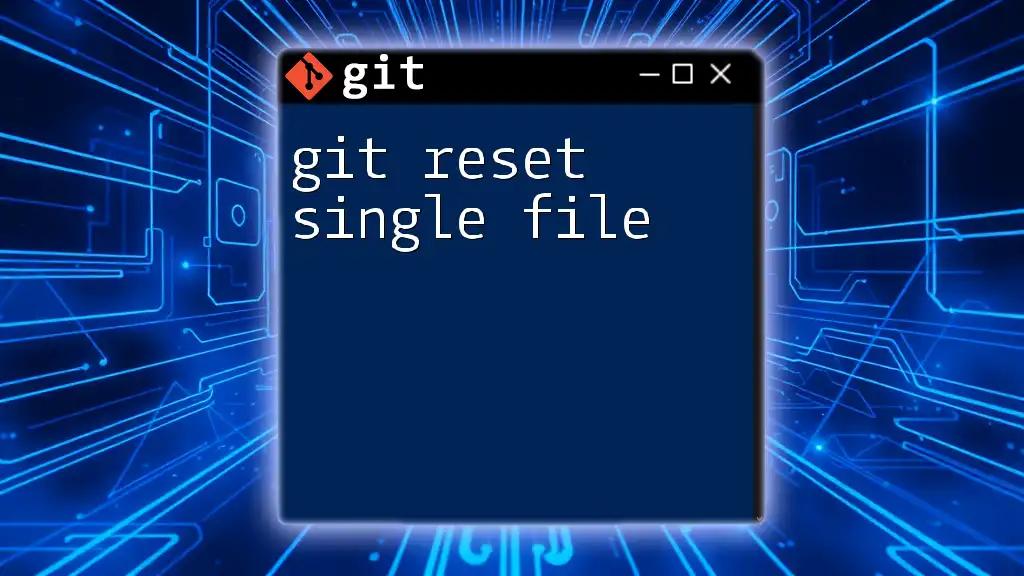
Additional Resources
Explore further learning through the official Git documentation and join online communities or forums where you can engage with other Git enthusiasts.
Call-to-Action
We encourage you to practice `git reset HEAD --soft` in your own repositories to gain a hands-on understanding of how this command can enhance your workflow. Follow our company for more quick Git tips and tricks!