The `git diff` command allows you to view the differences between commits, branches, or files, and can be used to create a patch file that captures those changes with the following command:
git diff > changes.patch
Understanding Git Diff
What is Git Diff?
`git diff` is a fundamental command in Git, designed to show differences between various states of files in a repository. Whether you're comparing changes between commits, working directories, or specific files, `git diff` provides a clear visualization of what has changed. It's essential for reviewing updates made to your code and ensuring everything aligns with your expectations before integration.
Basic Usage of Git Diff
The command syntax for `git diff` is straightforward. Here are a few common usages:
- To see changes between your working directory and the index (staging area), execute:
git diff
- If you want to compare changes added to the staging area with the last commit, use:
git diff --cached
- To compare changes made in a specific commit against your current state, you can specify the commit hash:
git diff <commit>
By using these commands, you gain the insights needed to make informed decisions about your code before proceeding with commits or further modifications.
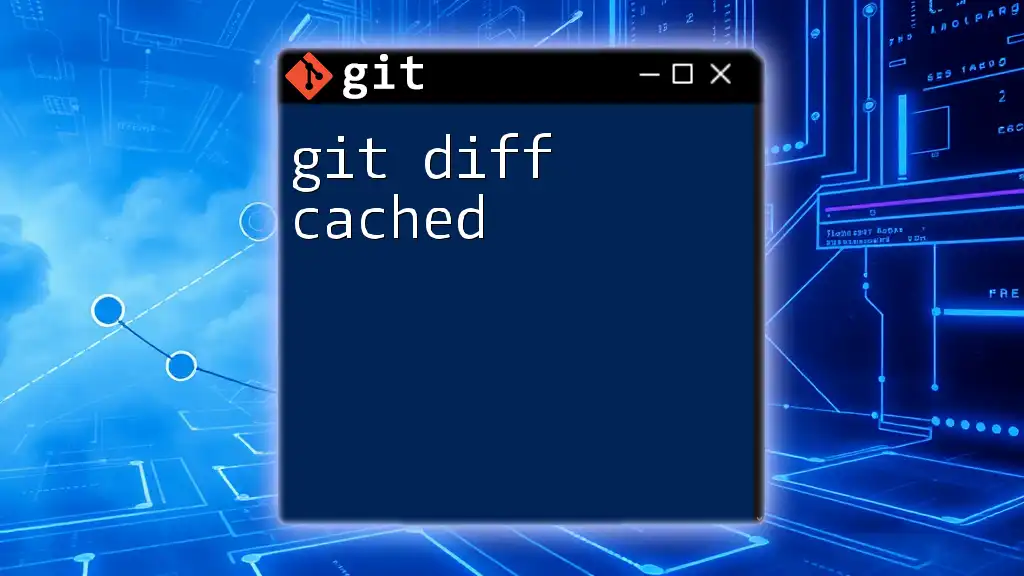
Creating Patches with Git Diff
What is a Patch?
In the context of version control, a patch is a file that contains differences between two sets of files. Patches are beneficial because they allow developers to share changes without directly accessing the repository. This is particularly useful for code reviews or when collaborating across different environments.
Generating Patches using Git Diff
You can easily generate patches using the `git diff` command.
To create an uncommitted patch from your current changes, run:
git diff > my_changes.patch
This command creates a patch file named `my_changes.patch` that contains the differences in your working directory.
For those looking to create a patch from committed changes, you can specify a range of commits. To create a patch reflecting changes between the current HEAD and the previous commit, use:
git diff HEAD~1 HEAD > my_patch.patch
This command produces a patch file that shows all differences introduced in the latest commit.
Formatting the Patch Output
Patches can be generated in various formats. The default output is the "unified" format, which provides a concise overview of changes. You can control the number of context lines shown (lines before and after the change) with the `-U` option. An example would be:
git diff -U3 > my_context_patch.patch
This command generates a patch file with three lines of context, giving you a better visual surrounding the changes.
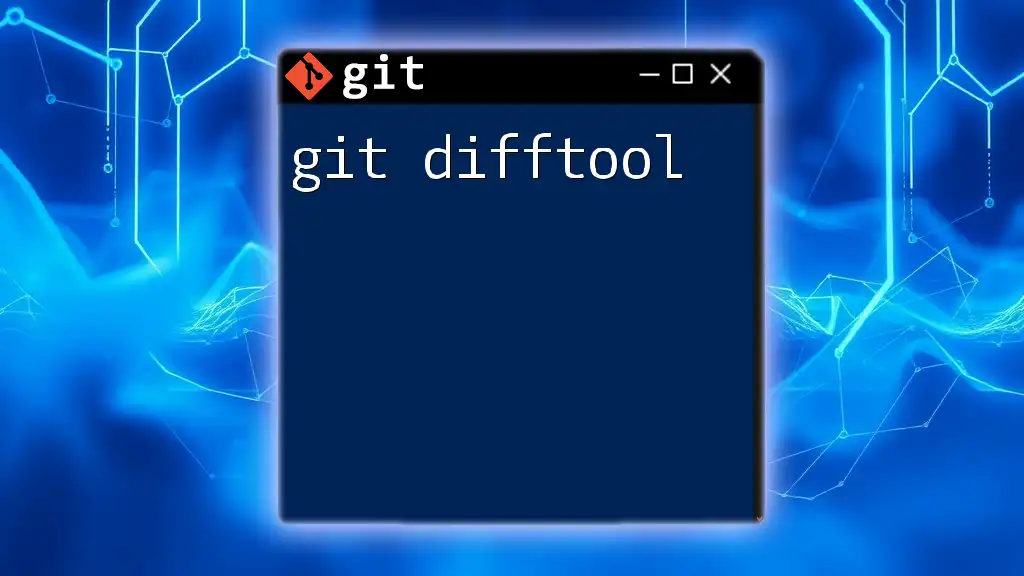
Applying Patches with Git
How to Apply a Patch
Once you have a patch file, you can apply it to your working directory using `git apply`. To do this, run:
git apply my_patch.patch
This command takes the changes outlined in `my_patch.patch` and applies them directly to your current working files.
Checking for Potential Issues
Before applying a patch, it’s wise to ensure that it can be applied cleanly. You can do this with:
git apply --check my_patch.patch
This command verifies whether applying the patch will result in any conflicts or errors, allowing you to troubleshoot before making changes.
Managing Patch Application Conflicts
Sometimes, applying a patch can lead to conflicts, particularly if other modifications have been made since the patch was created. In such cases, you can run:
git status
This command helps diagnose and resolve any issues by showing you which parts of your codebase are affected. Always ensure that the changes from the conflicting patch are correctly integrated.
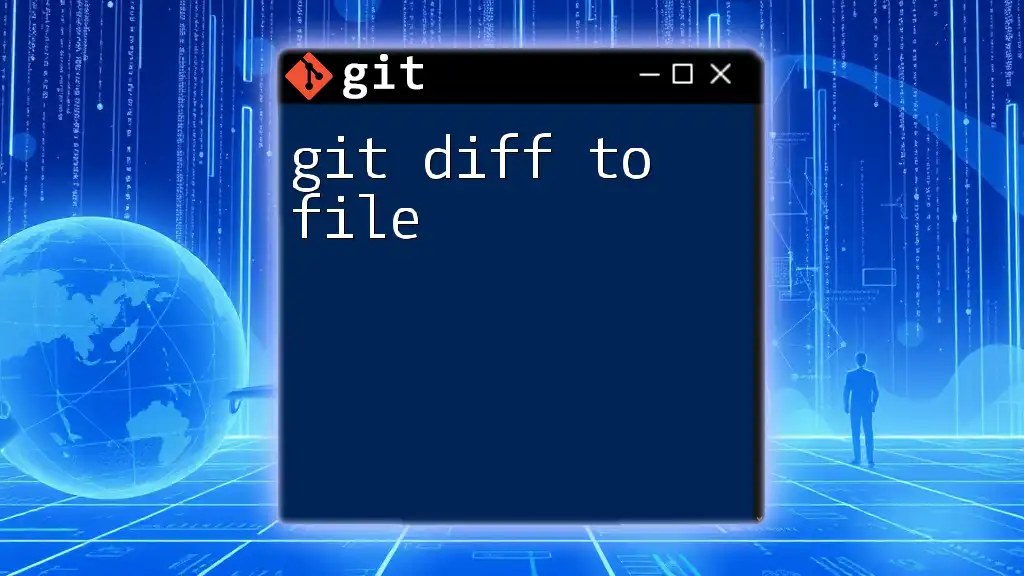
Viewing and Inspecting Patches
Reviewing Patch Content
Before applying a patch, it’s prudent to review its contents. You can use standard Unix commands like `cat`, `less`, or `more` to check:
cat my_patch.patch
This command will display the entire contents of the patch in your terminal, allowing you to assess the proposed changes.
Formatting and Context in Patches
When examining patch files, you'll see lines prefixed with `+` indicating additions and `-` representing deletions. Understanding this format is crucial for comprehending how the changes interplay with existing code.
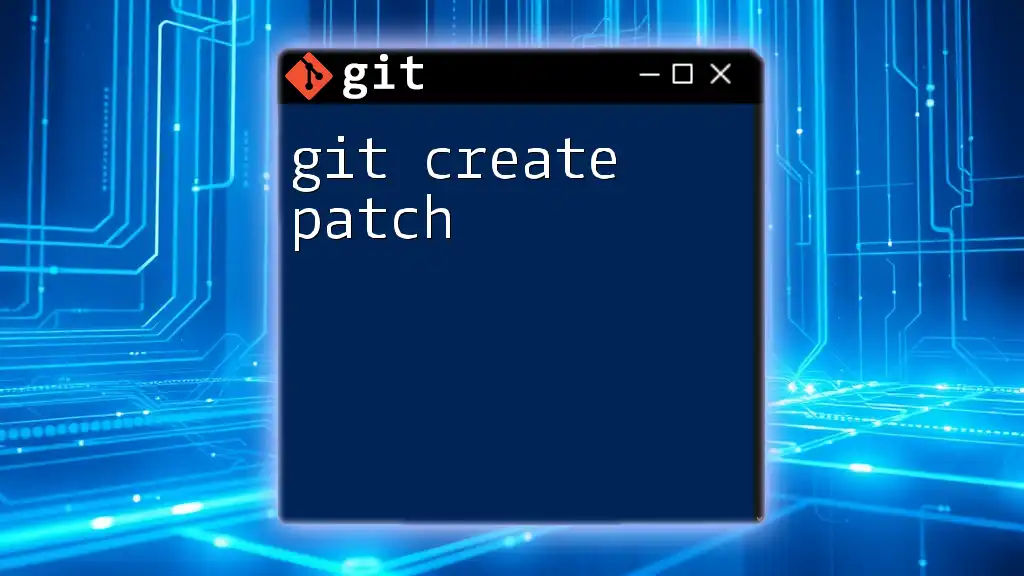
Best Practices for Using Git Diff to Patch
Consistency in Patching
To maintain order in your version control process, it’s essential to adopt consistent naming conventions for your patch files. Consider appending the date or a brief description to ensure clarity, such as:
feature_update_2023-10-01.patch
This practice aids in tracking changes over time, especially when working within larger teams or multiple projects.
Documentation and Comments
Adding comments to your patches is a powerful way to enhance communication among developers. When generating patches, consider including a documentation file that explains what changes are incorporated and why they are necessary. This could be in the form of a README or CHANGES file located alongside the patch.
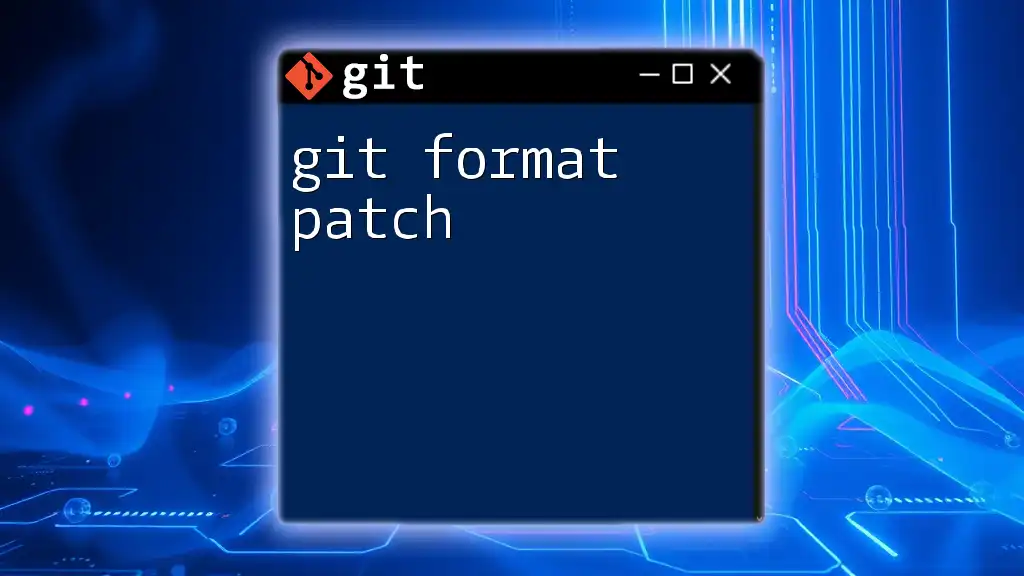
Conclusion
Navigating the intricacies of using `git diff to patch` can dramatically enhance your workflow and collaboration capabilities. By mastering the creation and application of patches, you empower yourself to share code efficiently, adhere to best practices, and ultimately improve the quality of your projects. Embrace these tools, practice with examples, and delve deeper into the robust capabilities of Git for optimal version control and collaboration.
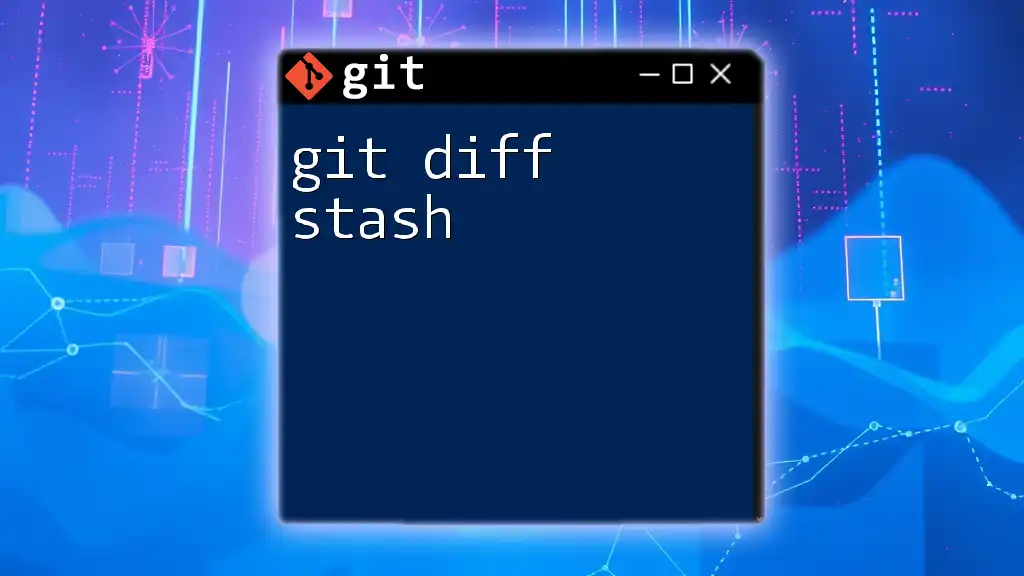
Additional Resources
For further guidance, refer to the official Git documentation related to `git diff`, look into the `git apply` command, and explore advanced patching techniques to enhance your Git proficiency.