To compare the differences in a specific file between two commits in Git, you can use the `git diff` command followed by the commit hashes and the file path.
git diff <commit1> <commit2> -- path/to/your/file.txt
What is `git diff`?
`git diff` is a command that allows you to view the changes between different states of your Git repository. It highlights the differences between various commits, branches, or even the working directory against the last commit.
Understanding `git diff` is crucial for developers working in teams, as it facilitates code reviews and helps in debugging by showing what has changed over time.
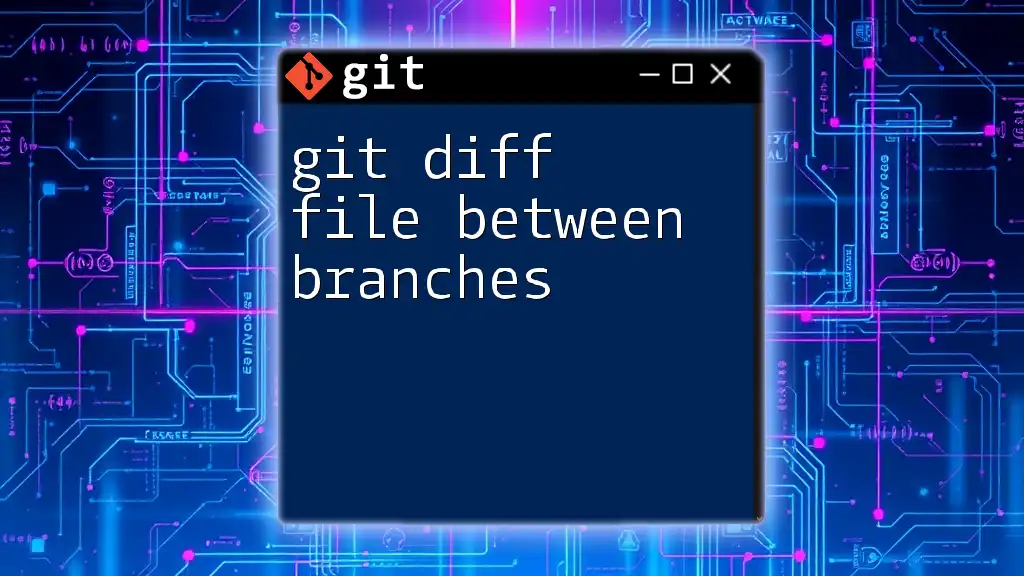
Understanding Commits in Git
What is a Commit?
A commit in Git represents a snapshot of your project at a specific point in time. Each commit captures the current changes and includes metadata like the author, date, and a message describing the changes.
Good commit messages are essential for collaboration and version control, as they provide context about what was changed and why. An example of a good commit message is:
Fix issue with user authentication by validating input fields
How Commits are Linked
Git uses unique identifiers called commit hashes to link and track commits. Each commit is associated with a hash (a long string of characters) that serves as its identifier.
You can view the history of commits in your repository by using the command:
git log
This command will display a list of commits, showing their hashes, authors, dates, and messages.
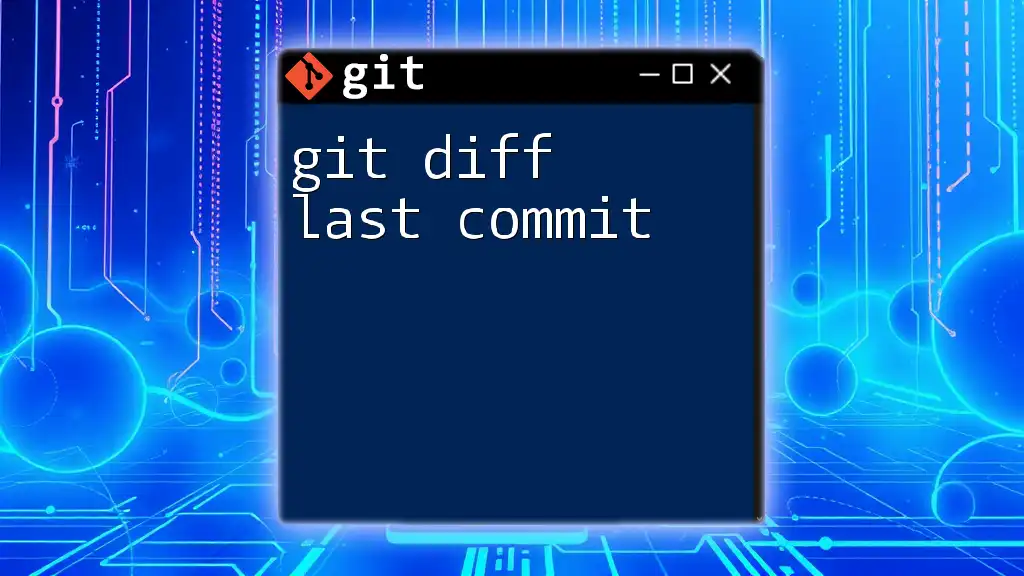
Using `git diff` Between Commits
Basic Syntax
The basic syntax for comparing files between two commits is:
git diff <commit1> <commit2>
In this command, `<commit1>` and `<commit2>` can be commit hashes, branch names, or other references.
Examples of Basic Usage
Example 1: Compare Two Specific Commits
To see the changes between two specific commits, you would use:
git diff abc123 def456
Here, `abc123` and `def456` are the hashes of the commits you want to compare. The output will show the differences between these two states of the repository.
Example 2: Compare the Latest Commit with Its Previous
Another useful command is comparing the latest commit with its immediate predecessor, which can be done as follows:
git diff HEAD HEAD~1
In this example, `HEAD` represents the most recent commit, and `HEAD~1` represents the commit just before it. This command is especially useful for quickly checking what changes you have made in your latest commit.
Viewing Changes in a Specific File
If you only want to view the changes in a specific file between two commits, you can specify the file as follows:
Single File Comparison
git diff <commit1> <commit2> -- <file-name>
This command displays the changes made specifically in `<file-name>` between the two commits.
Example:
git diff abc123 def456 -- src/main.py
The output will show additions, deletions, and any modifications in `main.py` that occurred between the specified commits.
Multiple Files Comparison
You can use a similar command to compare all changes in a specific directory:
git diff <commit1> <commit2> -- <directory-name>
This comparison will provide you with a unified view of changes across multiple files within the indicated directory.
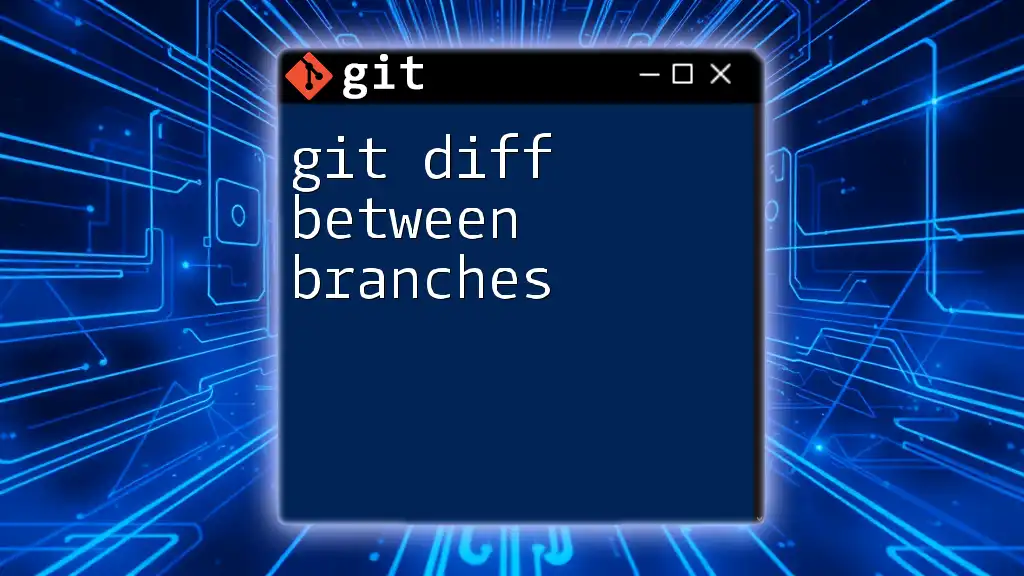
Advanced Options for `git diff`
Comparing Commits with Branches
You can also compare a commit to a branch using the following syntax:
git diff <branch-name> <commit>
This is particularly helpful when you want to check the differences between your current branch and one that has received updates from your collaborator.
For instance:
git diff main feature-branch
This command will show you the differences between the `main` branch and the `feature-branch`, helping you understand what has changed before merging.
Comparing Against the Working Directory
When working on updates, you might want to see what you're about to commit as compared to the last commit. You can achieve that by running:
git diff <commit>
This command will allow you to inspect changes in your working directory against the specified commit. It’s an excellent way to review modifications before staging them.
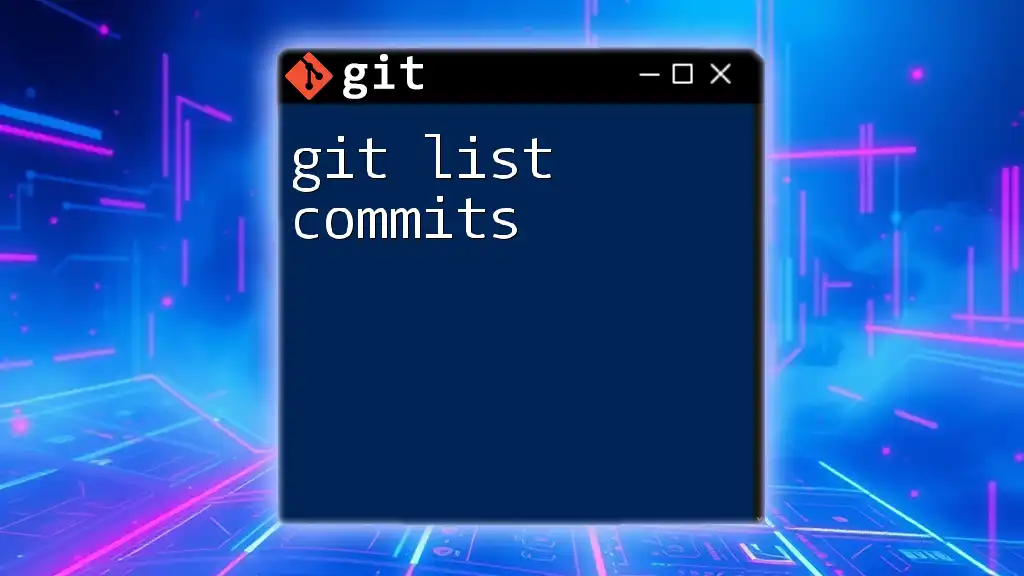
Best Practices for Using `git diff`
Check Before Committing
Always use `git diff` to review your changes before finalizing a commit. This helps in preventing unintended changes and ensures that you are only including relevant updates.
Use Descriptive Commit Messages After `git diff`
After performing `git diff`, consider writing informative commit messages that reflect the changes you've reviewed. This practice enhances the readability of your commit history and makes collaboration smoother.
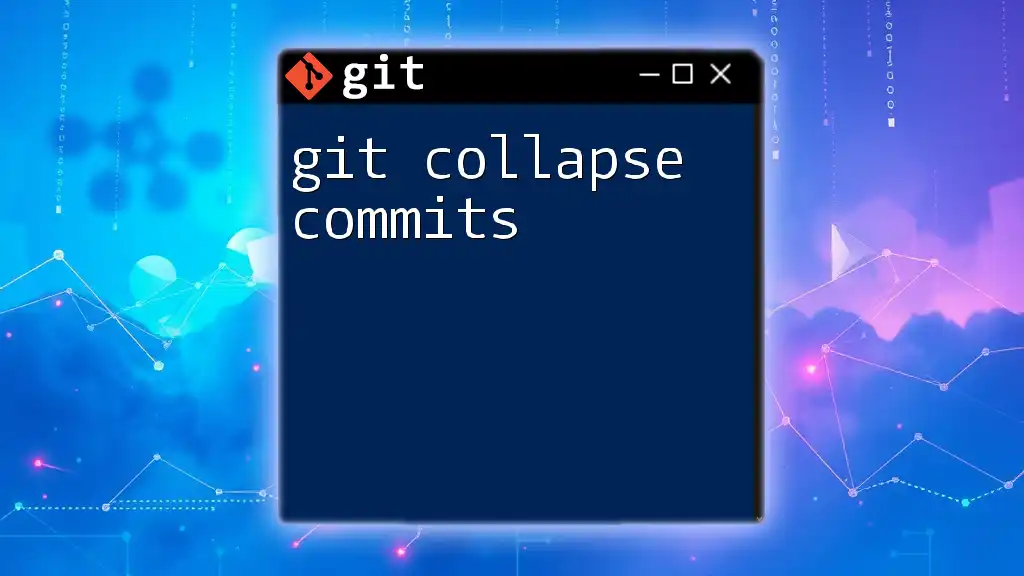
Conclusion
Mastering `git diff file between commits` is vital for effective use of Git and understanding the evolution of your codebase. By utilizing the command strategically, you can gain insights into how your project has changed over time and make informed decisions during collaboration.
As you continue to explore Git, practice using `git diff` in various scenarios to enhance your skills. The more familiar you become with its capabilities, the better equipped you'll be to manage your projects efficiently.
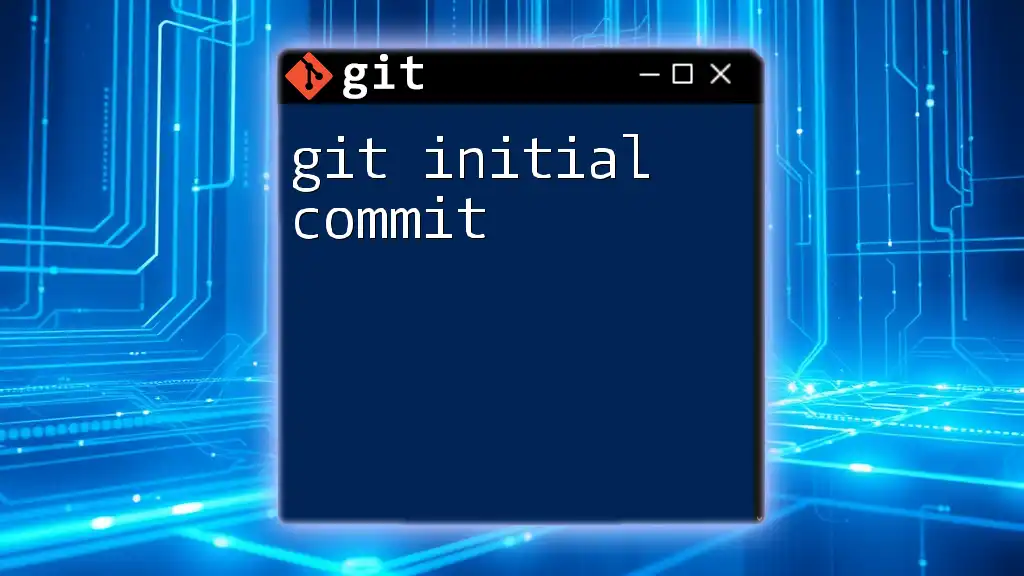
Additional Resources
For further learning, refer to the official Git documentation for comprehensive insights into all Git commands. Explore tutorials and courses that dive deeper into Git functionality, including tools that integrate with Git to optimize your workflow.
FAQs
- What if I accidentally omit a file I wanted to compare?
- Can I use `git diff` with uncommitted changes?
- How do I interpret `git diff` output?
Be sure to address these common queries as you share your newfound knowledge of `git diff` with others!