You can view the commit history between two specific commits in Git by using the `git log` command with the commit hashes specified as the range in the following format:
git log commit1..commit2
Replace `commit1` and `commit2` with the actual commit hashes you want to examine.
Understanding Commits in Git
What is a Commit?
A commit in Git is a snapshot of the changes made to your files at a particular point in time. Think of it as a milestone in a project where all modifications to the codebase are packaged together. Each commit is represented by a unique commit message, which describes the changes made. Here’s an example of a commit message:
Add user authentication feature
Commits are significant because they allow developers to track changes, review history, and revert to earlier states if needed.
The Concept of Commit Hashes
Every commit in Git is identified by a unique string known as a commit hash. These hashes are typically SHA-1 hashes that Git generates to differentiate each commit. You can view the commit hashes by using the `git log` command. Below is an example output showing commit hashes:
commit 7a7f4d8f8ab5eb2071ce3a30082dcd16ae29d2e4
Author: Alice <alice@example.com>
Date: Mon Oct 1 12:34:56 2023 -0700
Add user authentication feature
In this output, `7a7f4d8f8ab5eb2071ce3a30082dcd16ae29d2e4` is the commit hash.
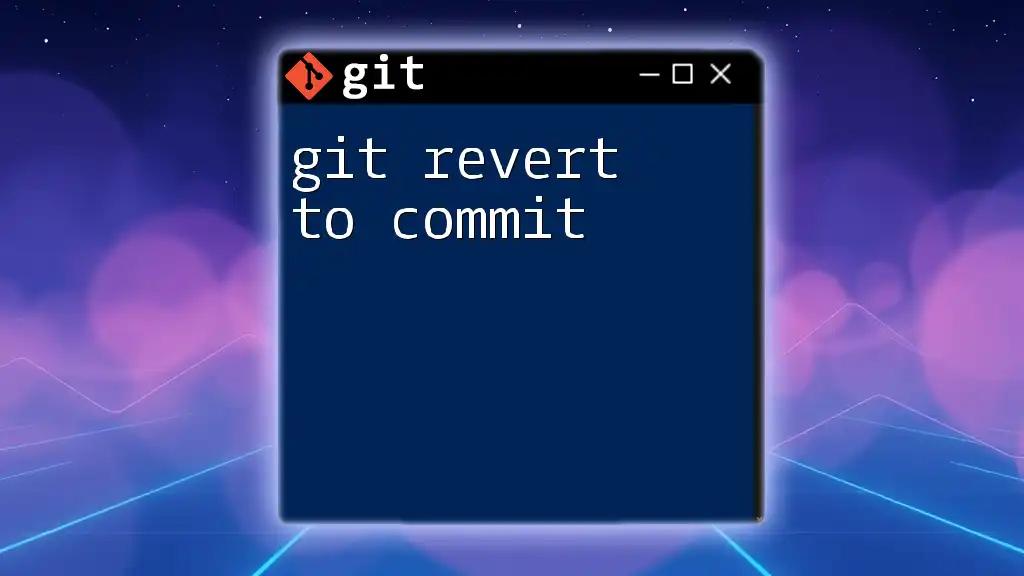
The `git log` Command Overview
Basic Syntax of `git log`
The `git log` command is essential for exploring the commit history. To use it, simply type:
git log
This command provides a detailed list of commits in the current branch, displaying each commit alongside its hash, author details, date, and message.
Options and Modifiers for `git log`
Git provides various options to enhance the `git log` command's output. Some commonly used options include:
- `--oneline`: Displays each commit on a single line to make the output concise.
- `--graph`: Visually represents the branch and merge structure.
- `--pretty`: Allows for custom formatting of the log output.
For example, to view a more concise list, you might run:
git log --oneline
This modification makes it easier to scan through multiple commits quickly.
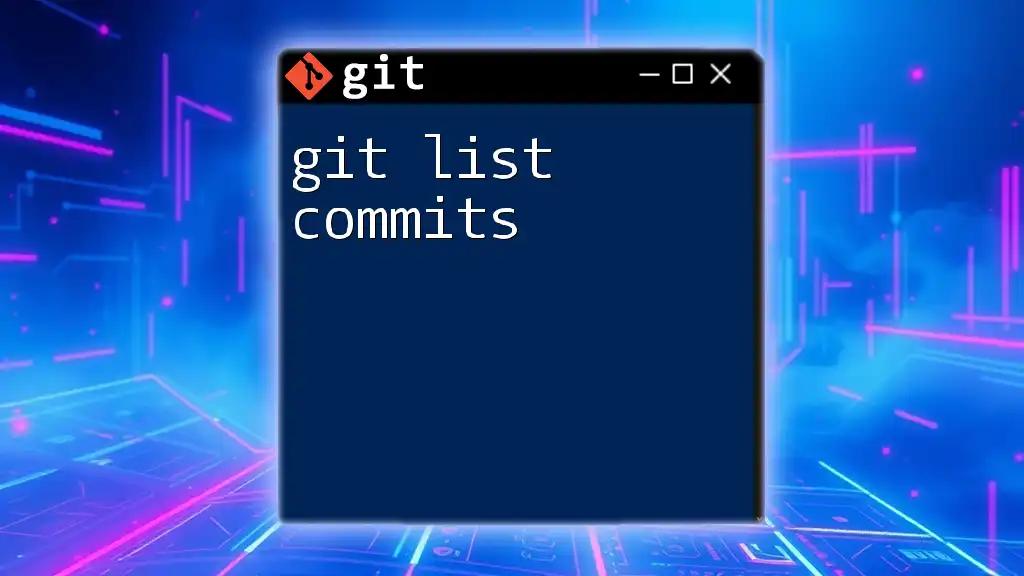
Using `git log` to View Logs Between Two Commits
How to Specify Two Commits
To view the log between two specific commits, you need to specify the commit references in the command. You can use commit hashes directly or branch names. The basic syntax looks like this:
git log <commit1>..<commit2>
Examples of the Command in Action
Here are examples of specifying two commits using hashes and branch names:
- Using commit hashes:
git log abcdef1..abcdef2
- Using branch names:
git log master..feature-branch
These commands display only the commits that exist in `<commit2>` but not in `<commit1>`, providing a clear picture of the changes made within that range.
Interpreting the Output
Understanding the Log Output
When you run the `git log` command between two commits, you will see a list of commits with information like commit hashes, authors, dates, and commit messages. This data illustrates the evolution of the code between the two specified points.
Filtering the Log Output
To focus on specific changes, you can filter the log output using options like `--author`, `--since`, and `--until`. For instance, if you're interested in commits made by a specific author during the range, you can use:
git log --author="Alice" abcdef1..abcdef2
This command will only show the commits authored by Alice in the specified range, helping you narrow down the results effectively.
Formatting the Output
Customizing Log Output Formats
Another powerful feature of `git log` is the ability to format the output. You can customize how each commit entry is displayed using the `--pretty` option. Here are some examples:
To display a concise one-liner format, use:
git log --oneline abcdef1..abcdef2
For a more detailed view, you might prefer a format like this:
git log --pretty=format:"%h - %an, %ar : %s" abcdef1..abcdef2
In this format, `%h` represents the shortened commit hash, `%an` denotes the author’s name, `%ar` shows the relative time, and `%s` displays the commit message.
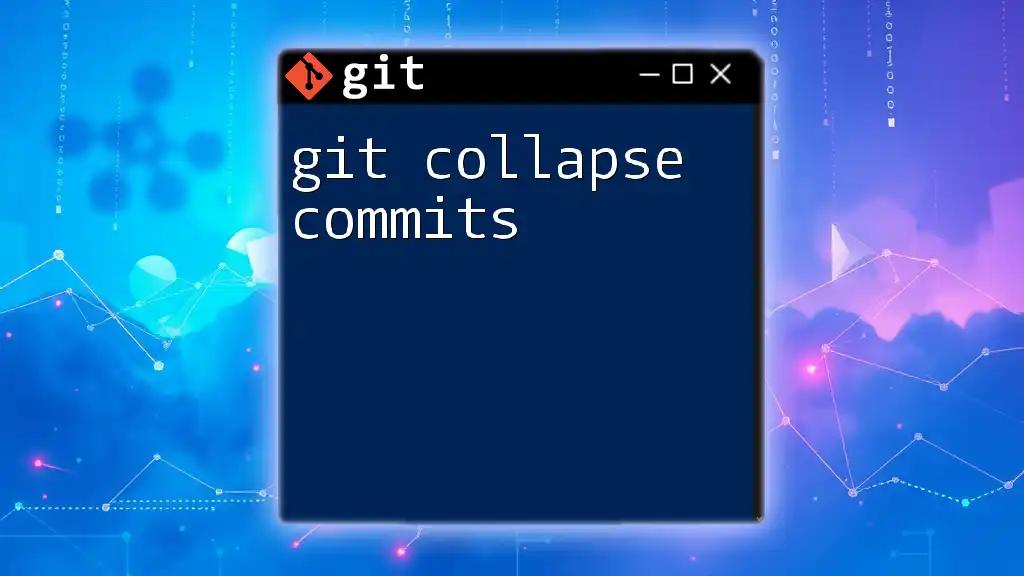
Practical Usage Scenarios
Debugging and Tracking Changes
Using `git log between two commits` is instrumental in debugging and tracking changes. If a new bug arises, this command helps identify when specific changes were introduced. For instance, if you suspect a bug was added between two significant releases, you can compare logs between those commits to pinpoint the modification responsible for the issue.
Collaboration and Reviewing Changes
In a collaborative environment, understanding the logs between two branches or commits is vital for code reviews. When a developer opens a pull request, reviewing what has changed since the last merge can help you quickly assess the implications of those updates. For example, you might find that a new feature was added in line with the specifications or might identify areas needing adjustments.
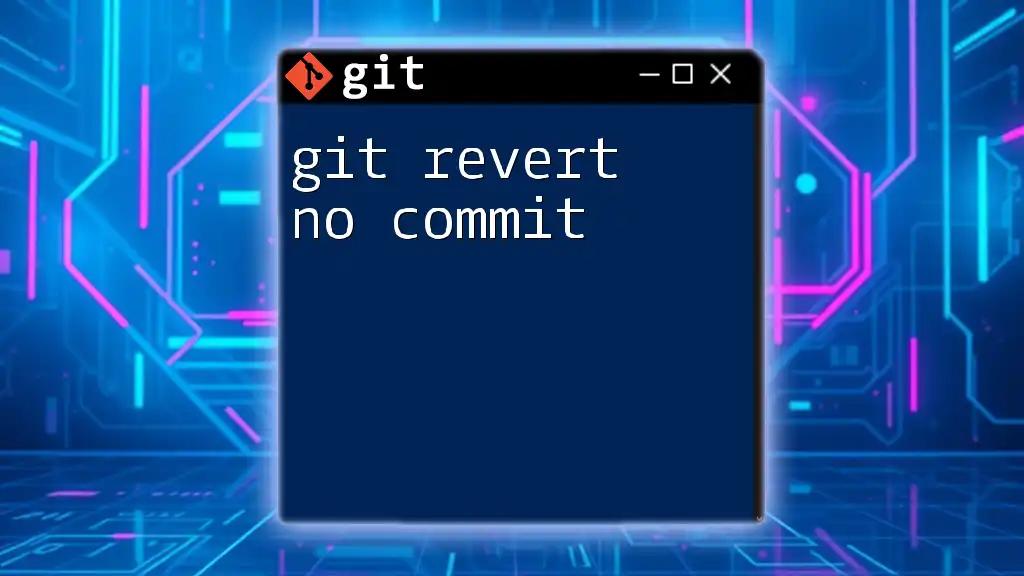
Conclusion
By mastering how to use `git log between two commits`, you enhance your version control skills and improve your efficiency as a developer. This command not only provides a detailed timeline of changes but also allows you to filter, format, and understand the evolution of your project more clearly. Practice the `git log` command frequently to become proficient in tracking your code's history and collaborating effectively with your team.
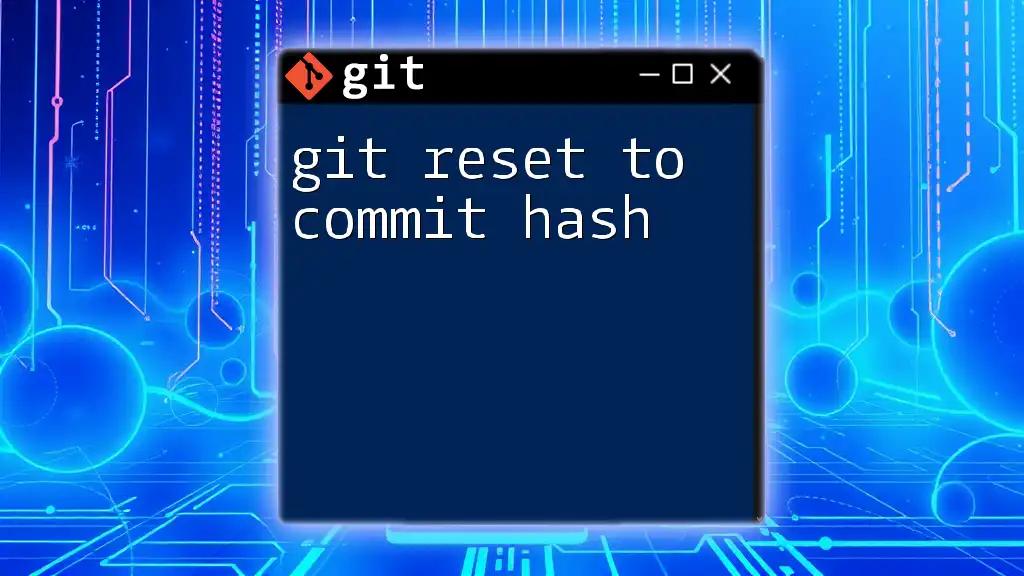
Additional Resources
For further exploration, consider reviewing the [official Git documentation](https://git-scm.com/doc). Additionally, you can look for tutorials and courses focused on Git to expand your knowledge and skills in version control. Engaging with structured learning will enable you to tackle more complex scenarios and enhance your workflow in software development.