To set and get user values in your Git configuration using the command line, you can utilize the `git config` command alongside cron jobs to automate this process; here's how you can set your username and email, and retrieve them:
# Set Git username and email
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
# Get Git username and email
git config --global --get user.name
git config --global --get user.email
To automate the retrieval of these values using crontab, you could add a cron job like this:
# Edit the crontab file
crontab -e
# Add a cron job to get the git user values every hour
0 * * * * git config --global --get user.name >> /path/to/output.txt && git config --global --get user.email >> /path/to/output.txt
Understanding Git Config
What is `git config`?
`git config` is a powerful command used in Git to set configuration values that affect how Git behaves on a system. The configurations can include user identification (like your name and email), preferences for merge behavior, and more. By configuring these settings, users ensure that their commits and other Git operations reflect their identity and intentions.
Types of Git Configuration Levels
Git configurations can be set at three different levels, each affecting how and where the configurations apply.
-
System Level: Configuration at this level affects all users on the system. It is stored in a global configuration file located at `/etc/gitconfig`. Use this for system-wide settings.
-
Global Level: This configuration applies to a single user across all repositories on a system. It is typically found in the user’s home directory, in the file `~/.gitconfig`. This is where individual preferences, such as user identity, are primarily set.
-
Local Level: Local configurations affect only a specific repository. They are saved in the `.git/config` file within that repository. This level is perfect for settings that are only relevant to the current project.
Commonly Configured User Values
Two of the most commonly configured user values in Git are the user name and user email. These values help identify the author of commits and ensure that commits are associated correctly with the author's identity:
- User Name: Sets the name that will appear in commits.
- User Email: Sets the email that will appear alongside the user name in commits.

Setting User Values with Git Config
Using `git config` to Set User Values
Setting your user information is simple and can be done using the `git config` command. Employing the global flag as shown below ensures that your configurations are applied universally across all Git repositories on your system.
To set your Git username and email, use the following commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Practical Examples
For example, to set your user name as "John Doe" and your email as "john@example.com", run the following:
git config --global user.name "John Doe"
git config --global user.email "john@example.com"
Explanation of the Command
In these commands:
- The `--global` option indicates that the setting should apply to the user’s global Git configuration.
- The first command specifies the name that will be recorded in each commit you make.
- The second command sets the email associated with your Git commits, which is crucial for collaboration and for sign-offs.
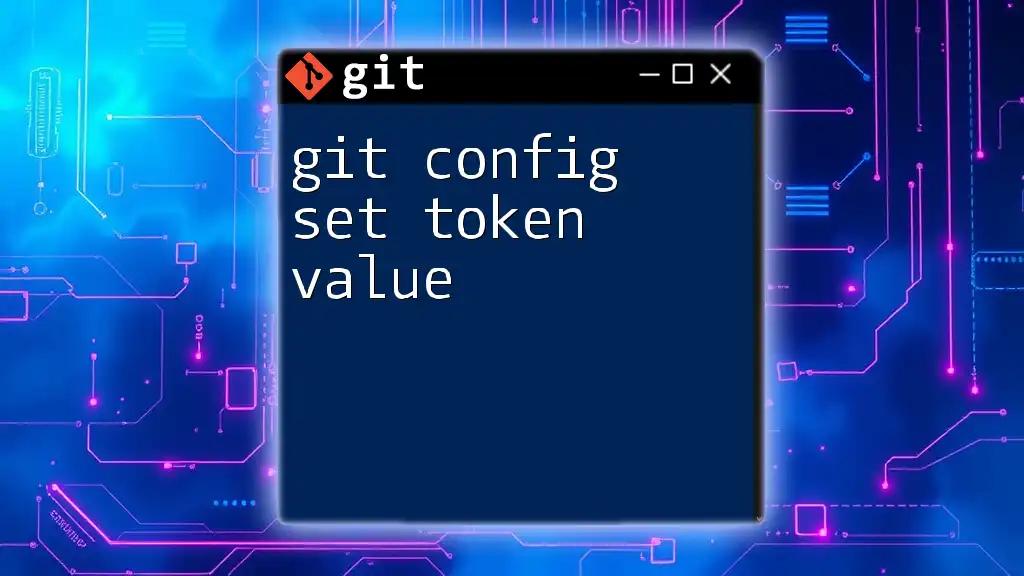
Getting User Values with Git Config
Using `git config` to Get User Values
If you need to check which user name and email are currently configured, you can retrieve these values using the following commands:
git config --global user.name
git config --global user.email
Practical Examples
To view your configured user name, you would execute:
git config --global user.name
Explanation of the Command
These commands retrieve and print the current configurations. This is particularly useful for verification purposes or troubleshooting any identity-related issues in commits.

Integrating Git Config with Crontab
Why You Might Want to Use Git Commands in Crontab
Scheduling Git commands using crontab can be highly efficient for automating routine tasks. For instance, if you want to ensure that your Git user configuration is updated regularly or if there are periodic tasks wearing the learner’s hat within collaborative environments, using crontab can save you both time and hassle.
Setting Up Crontab
To create or edit a crontab file, you need to use the command:
crontab -e
This opens the crontab editor, where you can add your scheduled tasks.
Example of a Crontab Entry to Set Git User Config
Let’s say you want to ensure that your Git user name is consistently updated every hour. You could add the following entry in your crontab:
0 * * * * git config --global user.name "Scheduled User"
Explanation of the Crontab Entry
In this entry:
- `0 * * * *` means the command will run at the top of every hour.
- The command will set the user name to "Scheduled User" every hour, ensuring there's a quick change if needed.
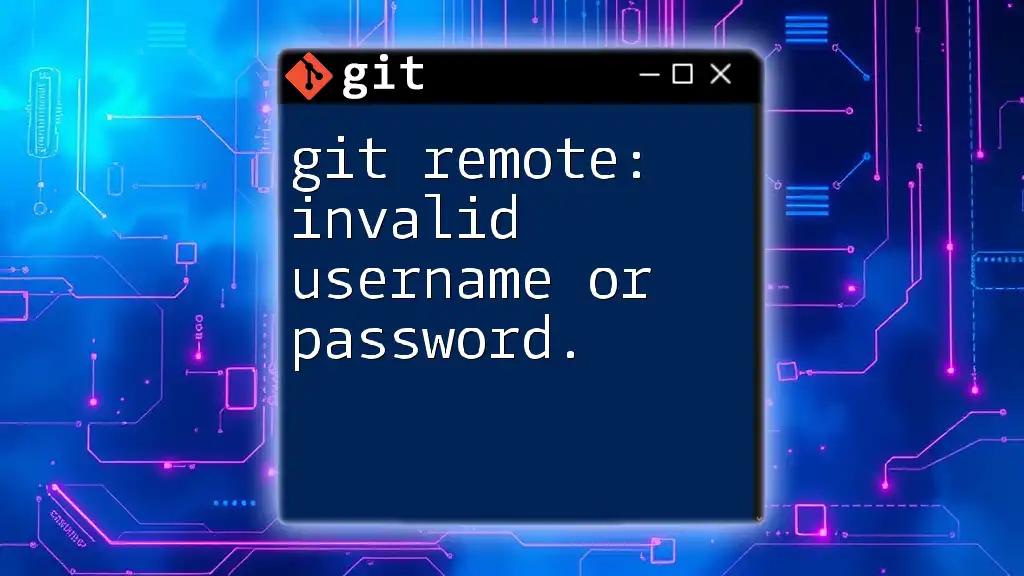
Common Use Cases
Automating Repository Maintenance
You might want to schedule tasks like cleaning up local repositories, pulling the latest changes, or pushing committed changes automatically using Git commands in crontab.
Regular User Updates
In teams, it’s crucial to have the latest configurations. Regularly updating user values in commits ensures proper tracking of contributions and maintains data accuracy.
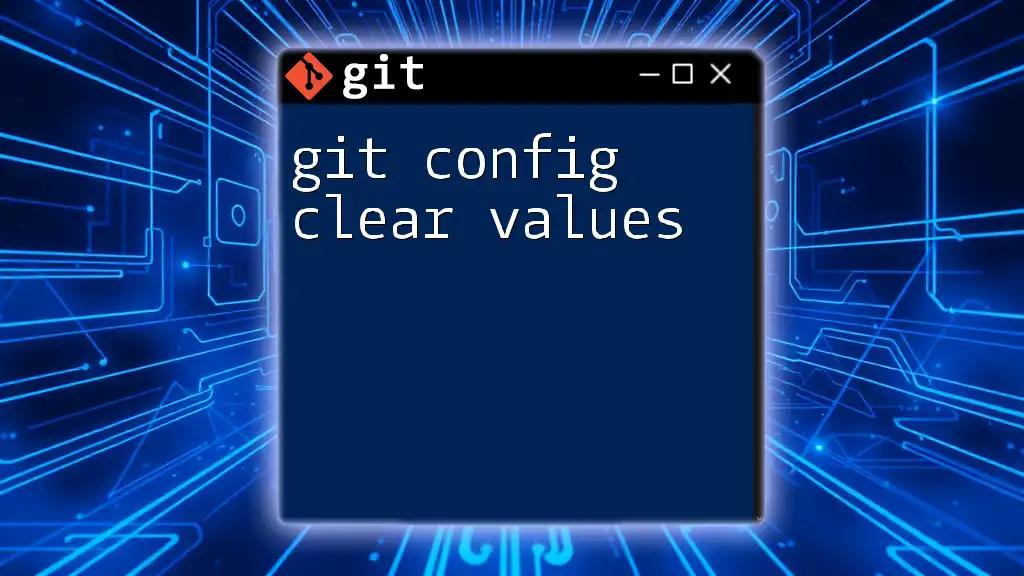
Troubleshooting
Common Issues with Git Config in Crontab
When utilizing Git commands in crontab, you may encounter permission errors or environment variable issues. To resolve these, ensure that the user under which the crontab is running has the required permissions to execute Git commands.
Best Practices for Using Git with Crontab
- Always log outputs of your scheduled commands to a file so you can check for errors.
- Be cautious with frequent changes; excessive writing to configuration files can clutter them and make troubleshooting complex.
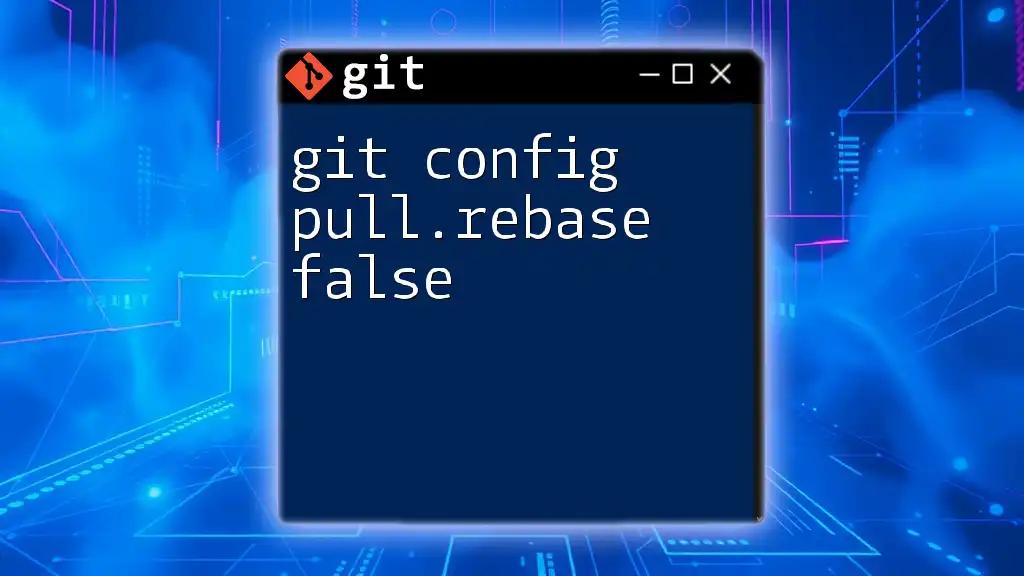
Conclusion
In this guide, we explored how to effectively use `git config` to set and get user values, integrate these capabilities with crontab, and automate routine Git tasks. By leveraging these powerful tools together, you can streamline your workflow and reduce the chances of human error.
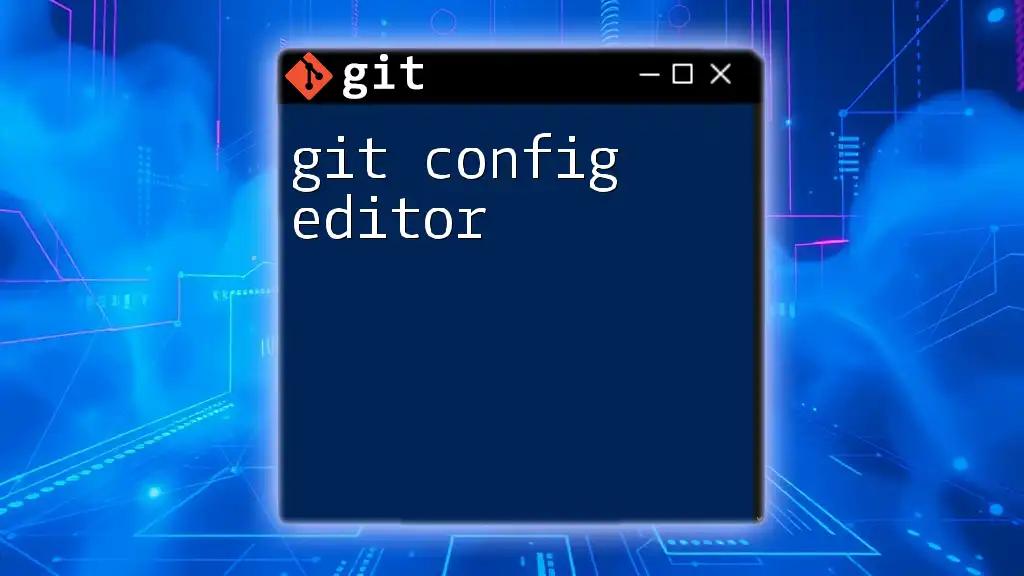
Additional Resources
To deepen your understanding, check out the official Git documentation and additional guides on crontab. Familiarizing yourself with these resources will enhance your mastery of Git and task scheduling.
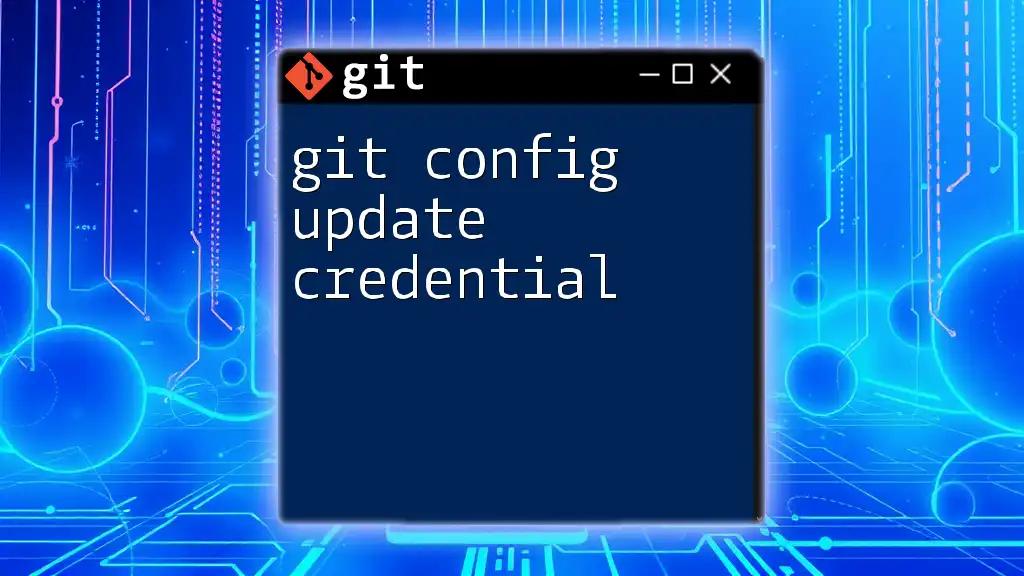
FAQs
Can I use Git commands in non-Unix systems?
Yes, while crontab is mainly a Unix tool, you can schedule tasks in a similar manner using task schedulers available on other systems, like Task Scheduler on Windows.
How often can I schedule tasks with crontab?
Tasks can be scheduled at any minute interval depending on your requirements. However, ensure that the tasks aren't too frequent to avoid resource strain.
Is it safe to automate Git commands?
While automation can enhance efficiency, always be aware of the implications of regularly running commands that can alter your Git environment. Implement safeguards and monitor output to keep integrity.