To unstage all changes in Git, you can use the command `git reset`, which reverts the staged changes back to your working directory without affecting the actual files.
Here's the command in markdown format:
git reset
Understanding Staging in Git
What is Staging?
In Git, staging refers to the process of preparing files for a commit. This staging area acts as an intermediate step, allowing you to decide which changes you want to include in the next commit. When you make changes to files in a working directory, those changes initially exist only locally. By staging the changes, you indicate which modifications should be included in your next snapshot of the project.
The staging area is crucial as it allows for a finer degree of control over what gets committed. You can choose individual files or even parts of files to stage, giving you flexibility in managing your changes.
The Need to Unstage
There are various scenarios where you might need to unstage files after adding them to the staging area:
- Mistakenly Added Files: You might accidentally stage files that you didn’t intend to include.
- Partial Commits: Sometimes, you may want to commit only certain changes from a file while keeping others staged for later.
- Working with Multiple Changes: You may decide to investigate or refine specific changes before finalizing a commit.
In these situations, it becomes essential to know how to unstage changes effectively.
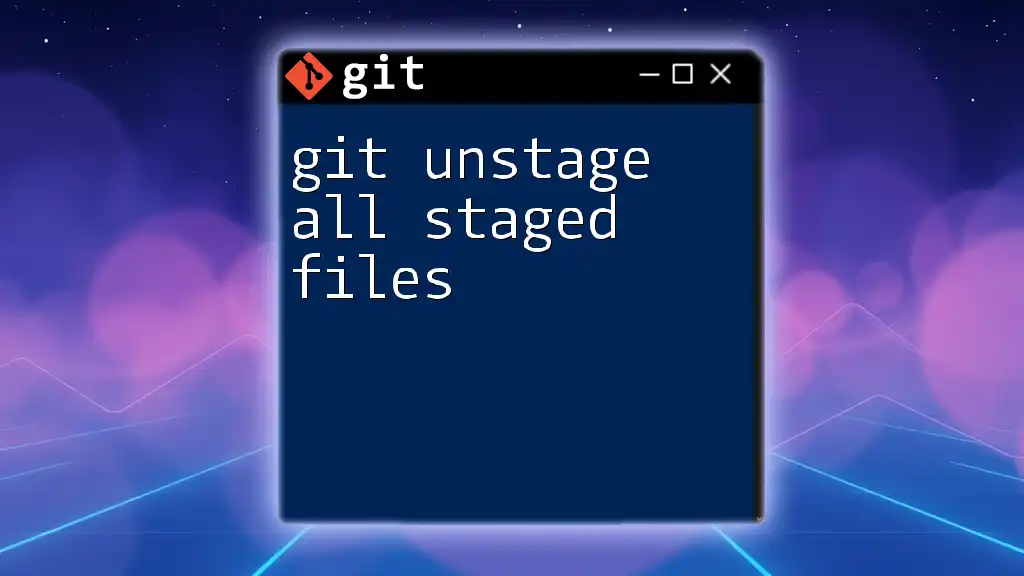
The `git reset` Command
Overview of `git reset`
The `git reset` command is a versatile tool in Git that can change the current HEAD to a specified state. It is primarily used to execute three modes: Soft, Mixed, and Hard. For most unstaging needs, Mixed mode is the most appropriate because it leaves your working directory untouched while modifying the staging area.
Using `git reset` to Unstage All Files
To unstage all files at once, you can use the following command:
git reset
This command will unstage all the files currently in the staging area, moving them back to the working directory. The outcome is that all your changes remain intact but are no longer flagged to be committed. You can review the changes as needed before deciding to stage again.
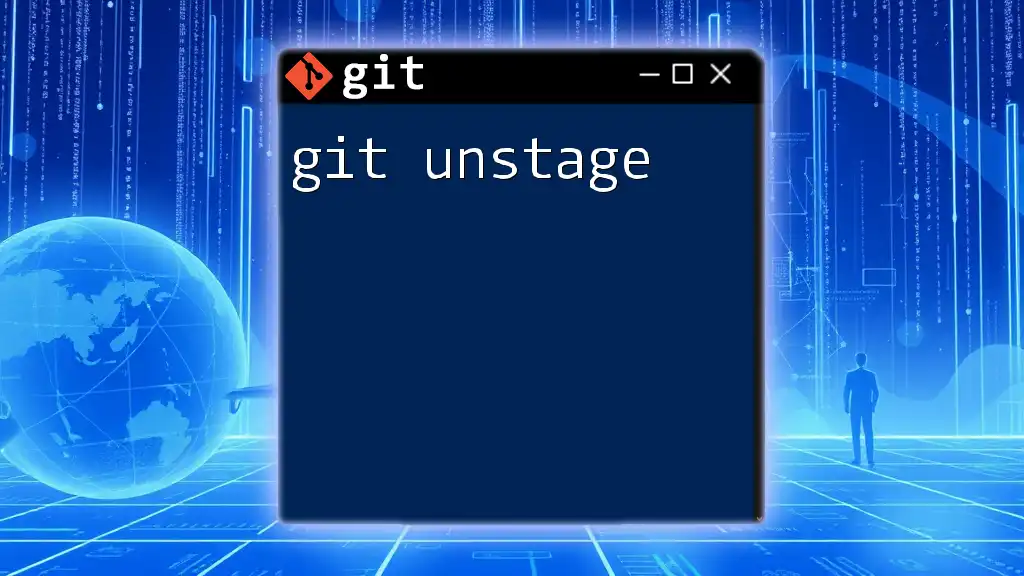
How to Unstage Specific Files
Unstaging Specific Files Using `git reset`
If you prefer to unstage specific files rather than all of them, you can specify the files explicitly. For instance, if you want to unstage `file1.txt` and `file2.txt`, you would run:
git reset file1.txt file2.txt
This command moves only the specified files from the staging area while keeping other staged changes intact. This fine-grained control is particularly useful when working with selective commits.
Alternative: `git restore` Command
With recent updates in Git, the `git restore` command provides a more pronounced way to handle the staging area. To unstage all changes, you can utilize the following command:
git restore --staged .
This command effectively unstages all files in the current directory without affecting the changes in the working directory. While `git reset` has been the traditional route, `git restore` offers an alternative that is often considered more intuitive, especially for beginners.
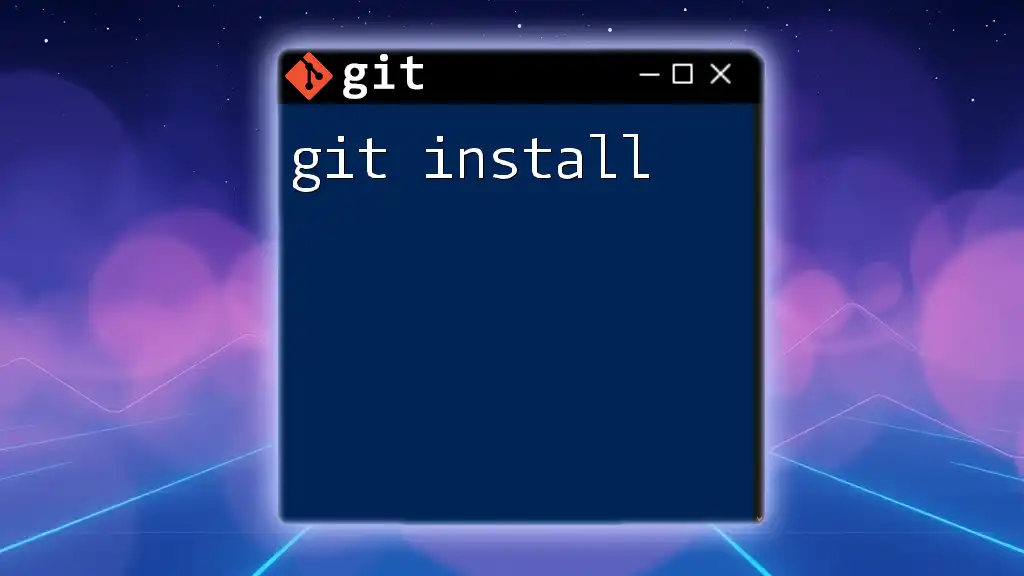
Practical Examples
Example Scenario: Unstage Changes After Mistakenly Adding Files
Imagine you are working on a project, and you accidentally staged a set of configuration files that shouldn't be part of your commit. You can resolve this issue quickly by using the `git reset` command to unstage all files:
git reset
After executing this command, run `git status` to verify that the staging area is clear, while your changes remain safe in the working directory. You can now selectively re-add files you actually want to commit.
Example Scenario: Working with Multiple Branches
Let’s say you’ve been experimenting on a feature branch and staged multiple changes, but now you need to switch to the main branch to address some urgent issues. Instead of committing half-finished code, you can unstage everything with:
git reset
Then, you can switch branches safely without carrying over unintended changes.
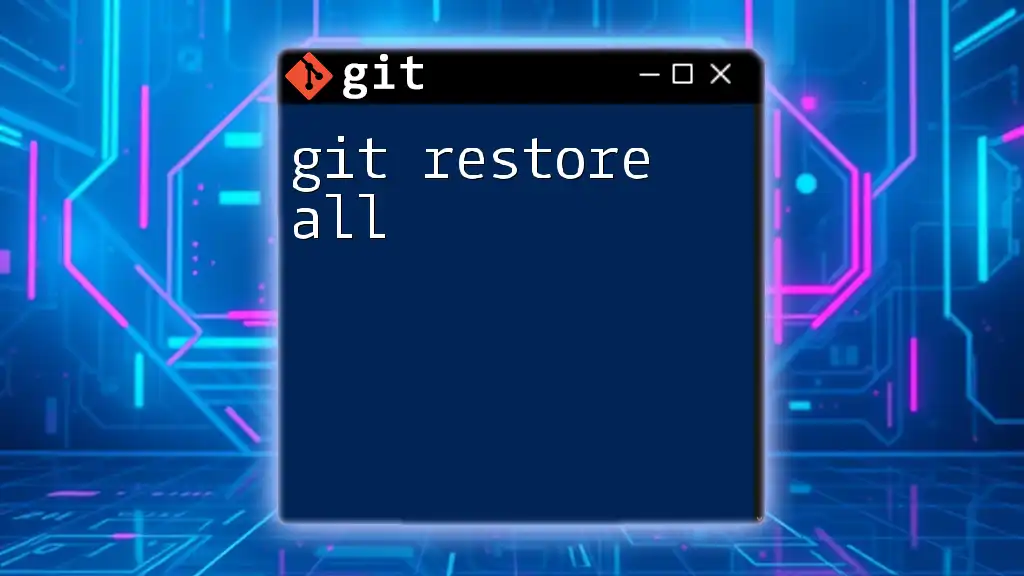
Common Mistakes and How to Avoid Them
Mistakenly Using `git reset --hard`
A frequent pitfall when attempting to manage staging and commits is mistakenly using `git reset --hard`. This command irreversibly clears your changes from the working directory as well as the staging area. If your goal is to unstage files only, the Mixed mode (`git reset`) is the way to go.
Forgetting to Check Current Status
Before unstaging files, it's essential to check the current state of your working directory and staging area. This can be done using:
git status
The output will inform you of which files are staged, unstaged, or untracked, preventing confusion and ensuring you're unstaging the right files.
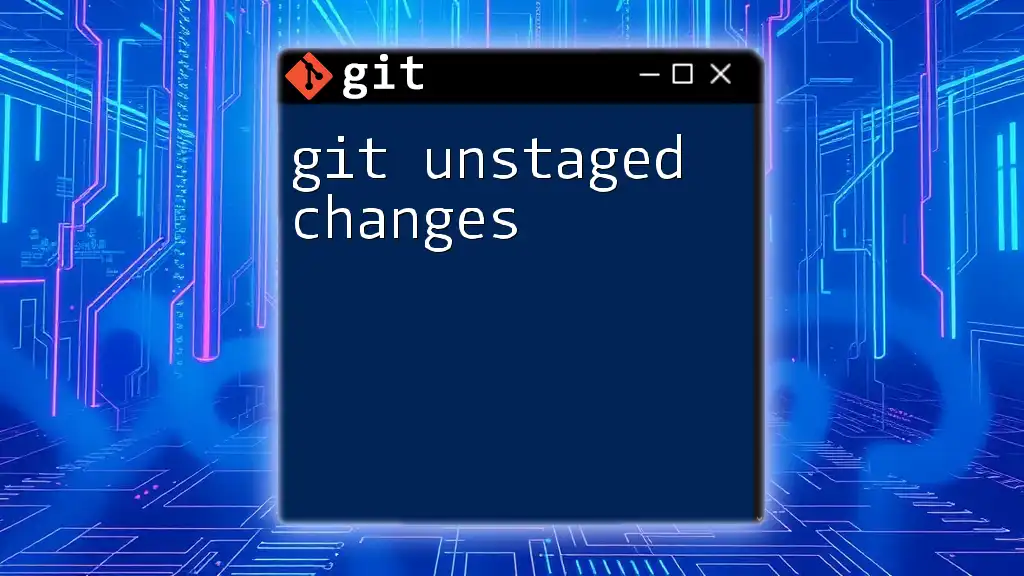
Conclusion
In this article, we've explored the critical Git command git unstage all, offering insights into unstaging files efficiently. Knowing how to manipulate the staging area is vital for effective version control, preventing mistakes, and maintaining a well-organized commit history. Practice these commands in your everyday workflow to become more confident in managing your projects with Git.
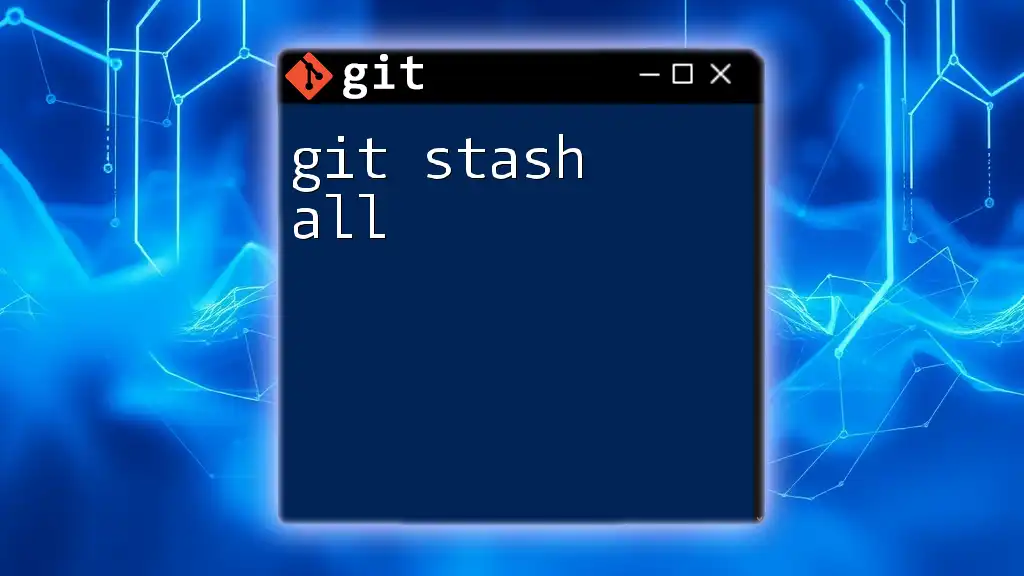
Additional Resources
Recommended Reading
For further learning, you can explore the official Git documentation, which provides detailed commands and their uses. Additionally, numerous tutorials exist online to extend your knowledge of Git commands.
Join Our Community
We invite you to join our forum for ongoing discussions, tips, and support as you advance your understanding of Git commands. Staying connected can enhance your skills and knowledge while learning from others in the community.