To undo a cherry-pick in Git, you can use the `git cherry-pick --abort` command if you haven't committed yet, or `git reset --hard HEAD~1` to remove the last cherry-picked commit if you've already committed it.
git cherry-pick --abort
or, if committed:
git reset --hard HEAD~1
Understanding Cherry Picking in Git
Cherry picking in Git is a powerful feature that allows you to select specific commits from one branch and apply them to another without merging all the changes. This is particularly useful when you want to include a bug fix or a specific feature that exists in one branch but not in another, without merging the entire branch.
What Does Cherry Picking Do?
The cherry-pick command takes a commit from a source branch and applies it to your current branch. It allows you to incorporate changes selectively, enabling a more granular control over the project history.
Syntax of the Cherry Pick Command
The basic command to cherry pick a commit looks like this:
git cherry-pick <commit>
In this syntax, `<commit>` is the unique identifier (SHA-1 hash) of the commit you want to cherry pick. Here’s an example of a simple cherry pick:
git cherry-pick abc1234
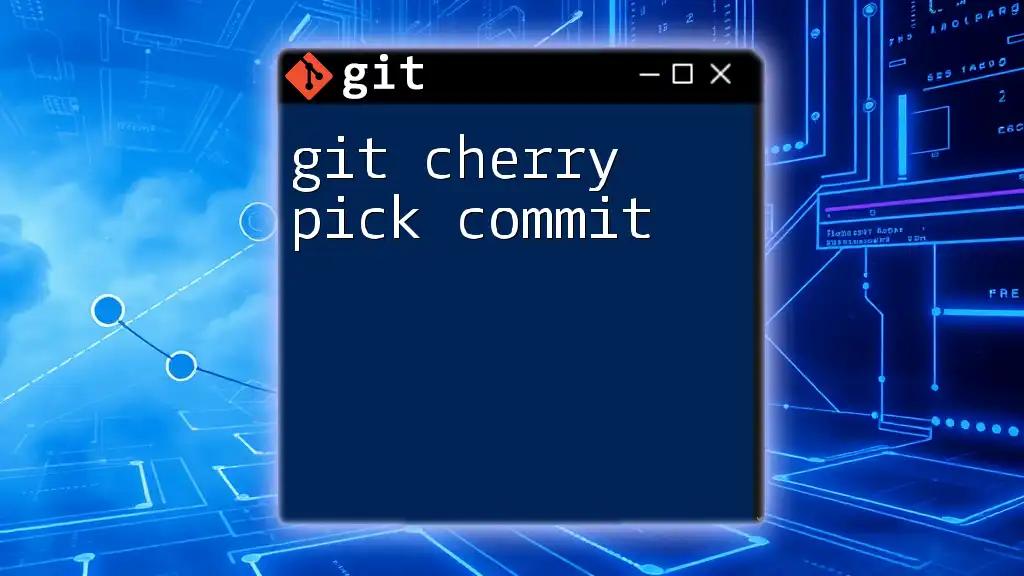
How to Undo a Cherry Pick
Identifying the Need to Undo
You may find yourself needing to undo a cherry pick for several reasons, such as:
- Merging incompatible changes from different branches.
- Accidentally picking the wrong commit, leading to conflicts or undesired changes.
- Realizing a bug was reintroduced from the cherry-picked commit.
Using Git Commands to Undo Cherry Picks
The `git cherry-pick --abort` Command
If you encounter conflicts during a cherry pick, you can abort the operation before finalizing it. This is done with the `--abort` option. This command returns your branch to the state it was in before you attempted to cherry pick.
git cherry-pick --abort
Using `git reset` to Undo Cherry Picks
If you successfully cherry pick a commit but later decide you want to revert the cherry-pick action, you can use the `git reset` command. This command alters the state of your branch and allows you to undo changes.
-
Soft Reset: This command moves the HEAD to the previous commit but keeps changes in the staging area.
git reset --soft HEAD~1
-
Mixed Reset: This command resets the index but preserves the working directory, allowing you to modify files freely.
git reset HEAD~1
-
Hard Reset: This command resets both your index and working directory to the specified commit, effectively discarding all changes made since that commit. Use this with caution.
git reset --hard HEAD~1
Keep in mind that using `git reset` can lead to loss of uncommitted changes, so ensure you are okay with discarding these changes before proceeding.
Using `git reflog` to Recover Lost Commits
If you inadvertently lose commits after a cherry pick, you can use Git’s reflog to find and recover them. The reflog indicates where your HEAD has been over time, making it ideal for recovering lost commits.
git reflog
You can locate the commit hash from the reflog and check it out:
git checkout <commit_id>
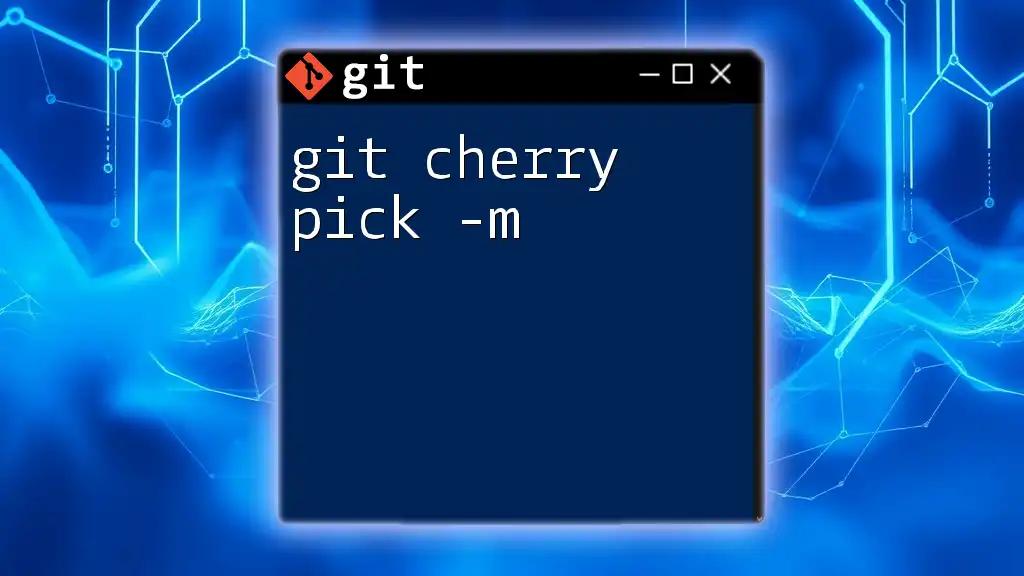
Handling Conflicts During a Cherry Pick
Understanding Cherry Pick Conflicts
Conflicts during a cherry pick can arise when the changes in the commit you're cherry picking overlap with changes in your current branch. Git may not be able to resolve these changes automatically, thus requiring human intervention.
How to Abort a Cherry Pick During a Conflict
In the event of a conflict, you can immediately abort the cherry pick by using:
git cherry-pick --abort
This command discards the ongoing cherry pick operation and returns you to the state prior to the cherry pick.
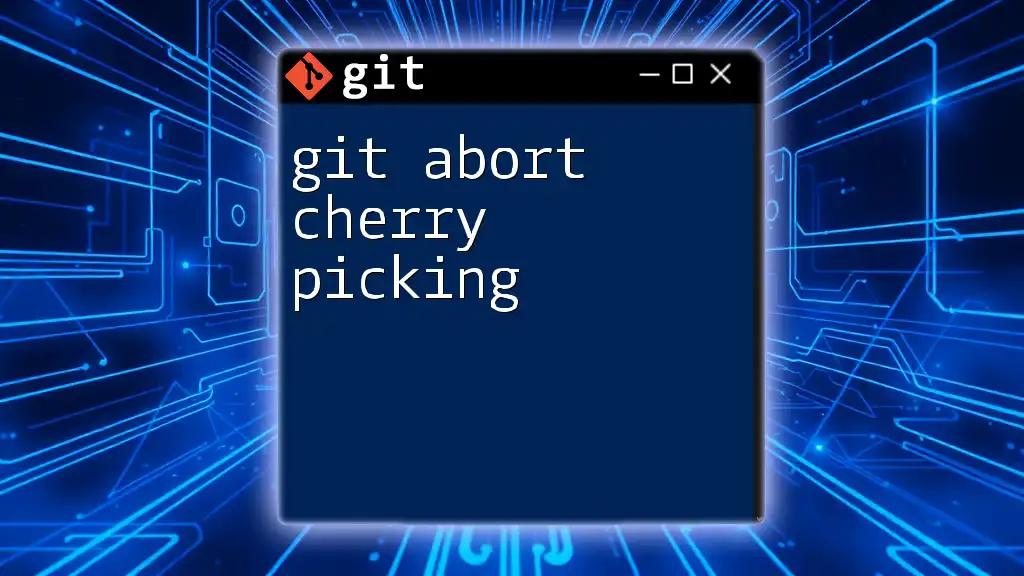
Best Practices for Using Cherry Pick
When to Cherry Pick
Cherry picking can be quite useful, but it's important to use it judiciously. Here are some recommended scenarios:
- Incorporating crucial bug fixes from one branch to another.
- Delivering specific features without merging entire branches.
Alternatives to Cherry Picking
While cherry picking is effective, it may not always be the best option:
- Merging: Merging is ideal when you want to incorporate a complete set of changes from another branch.
- Rebasing: Rebasing helps integrate changes in a cleaner way by building a new commit on top of your current branch.
By understanding these alternatives, you can make an informed decision on how to integrate changes into your project.
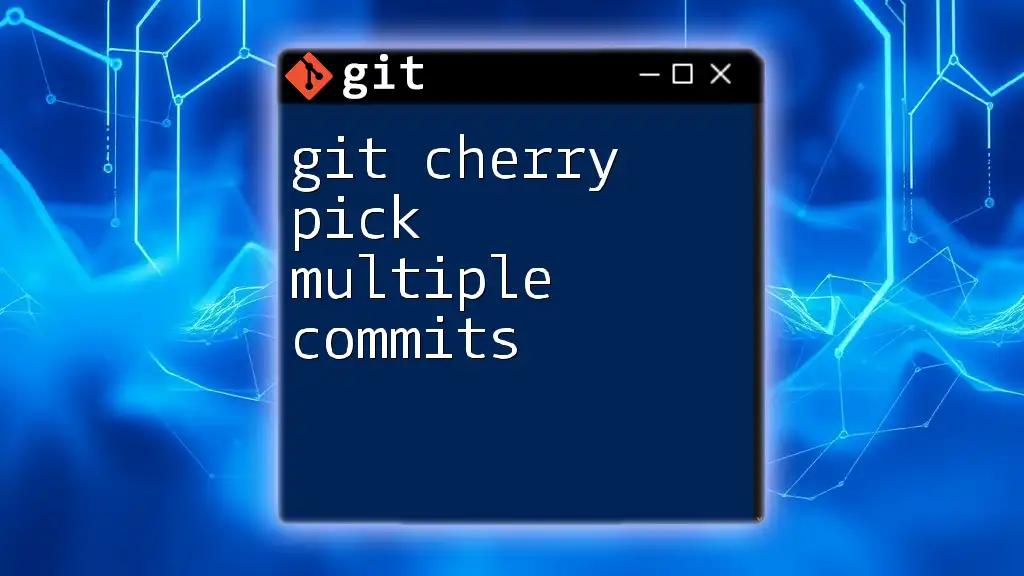
Conclusion
In summary, knowing how to use `git undo cherry pick` is essential for any developer working with version control. Whether you need to abort a cherry pick, reset your branch, or recover lost commits, the steps outlined above can help you manage your Git workflows effectively. Remember that practice makes perfect—experimentation with these commands in a safe environment can enhance your understanding and proficiency.
For further learning, consult the official Git documentation, engage with tutorials, and don’t hesitate to reach out for assistance as you refine your Git skills.