To discard local changes and pull the latest version from the remote repository, use the following commands:
git reset --hard
git pull origin main
Make sure to replace `main` with your specific branch name if necessary.
Understanding Local Changes in Git
What Are Local Changes?
In Git, local changes refer to modifications made to files in your local working directory. These changes can be categorized into:
- Tracked Changes: Modifications made to files that are already being monitored by Git.
- Untracked Changes: New files that have been added to your working directory but haven’t been staged for commit yet.
Understanding these differences is crucial when deciding whether to keep or discard changes, as well as how to proceed with pulling updates from a remote repository.
Why Discard Local Changes?
There are several situations where you might want to discard local changes:
- Experiment Gone Wrong: If you tried something new and it didn’t work out, you might prefer to revert to the last stable version.
- New Updates from Remote: When you need to pull the latest changes from the remote repository and your local changes could cause merge conflicts.
- Commit Mishaps: If you’ve made changes that you realize don’t align with your project goals.
Important Note: Always remember that discarding changes is permanent! Once discarded, you cannot retrieve those changes unless you previously stashed or committed them.
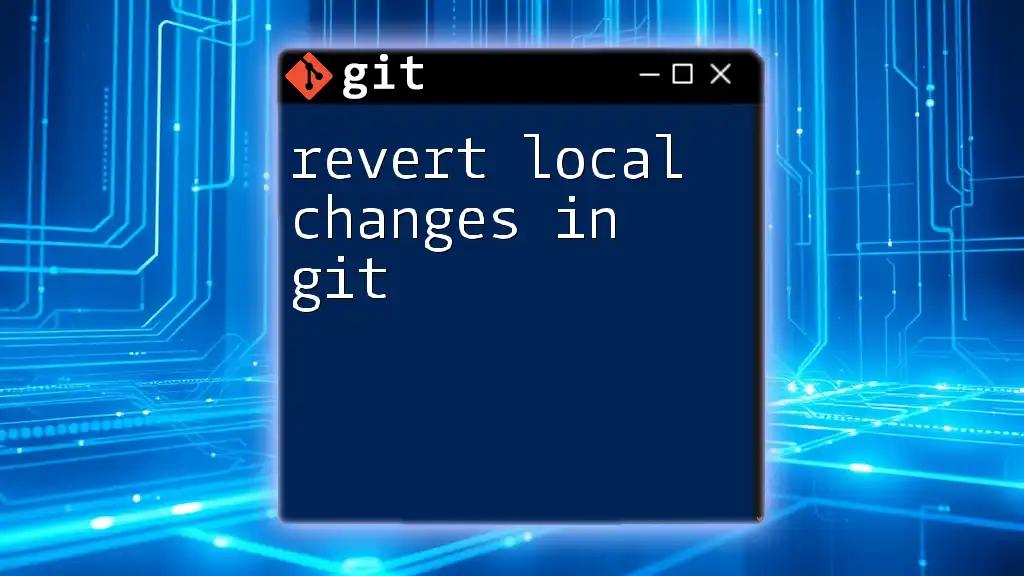
Preparing to Discard Local Changes
Evaluating Your Current Status
Before discarding any local changes, it’s vital to evaluate what has been modified. Running the following command shows the status of your local repository, highlighting modified, staged, and untracked files:
git status
By reviewing this output, you can clearly identify the changes you might need to discard.
Stashing Changes (Optional)
If you’re not entirely sure about applying changes yet, consider stashing them instead of discarding. Stashing allows you to save your modifications temporarily:
git stash
Later, if you want to retrieve those changes, simply use:
git stash pop
This command applies your stashed changes back into your working directory. This can be handy if you have uncommitted changes but need to switch contexts momentarily.

Discarding Local Changes
Discarding Unstaged Changes
When local changes are currently unstaged and you want to revert them, you can use this command:
git checkout -- <file>
Replace `<file>` with the name of the file you want to discard. This command discards all modifications and returns the file to its last committed state. Exercise caution, as this action cannot be undone.
Discarding Staged Changes
If you’ve accidentally staged changes but wish to discard them, you first need to unstage them, and then check out the version from the last commit. Here’s how:
git reset HEAD <file>
git checkout -- <file>
The first command un-stages the changes, while the second one discards the changes to revert the file.
Resetting the Entire Local Branch
If you've decided that you want to discard all local changes, you can reset your entire local branch using:
git reset --hard
This command will erase all local changes (tracked and untracked) and revert the current branch to match the remote. Be very careful with this command, as you cannot recover discarded changes.
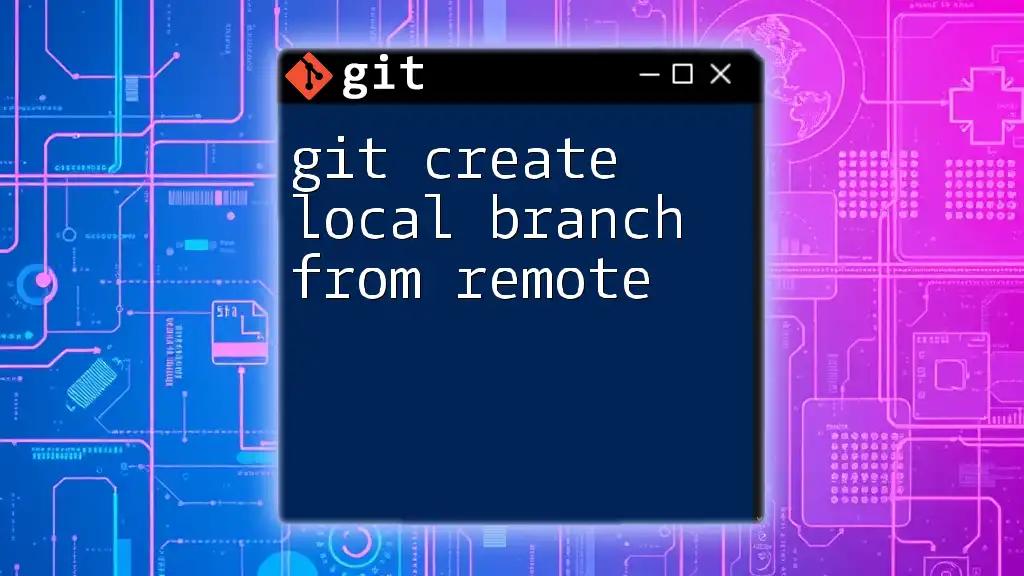
Pulling from the Remote Repository
Understanding Git Pull
The `git pull` command is frequently used to fetch and integrate changes from a remote repository into your local branch. This command simplifies the fetching of changes and merging them into your current working state.
Pulling After Discarding Changes
Once you’ve wisely discarded unnecessary local changes, you can pull the latest updates from the remote repository. Use the following command:
git pull origin <branch_name>
Replacing `<branch_name>` with the active branch you’re working on. This pulls in updates while ensuring that your local environment reflects the latest changes from the remote source.
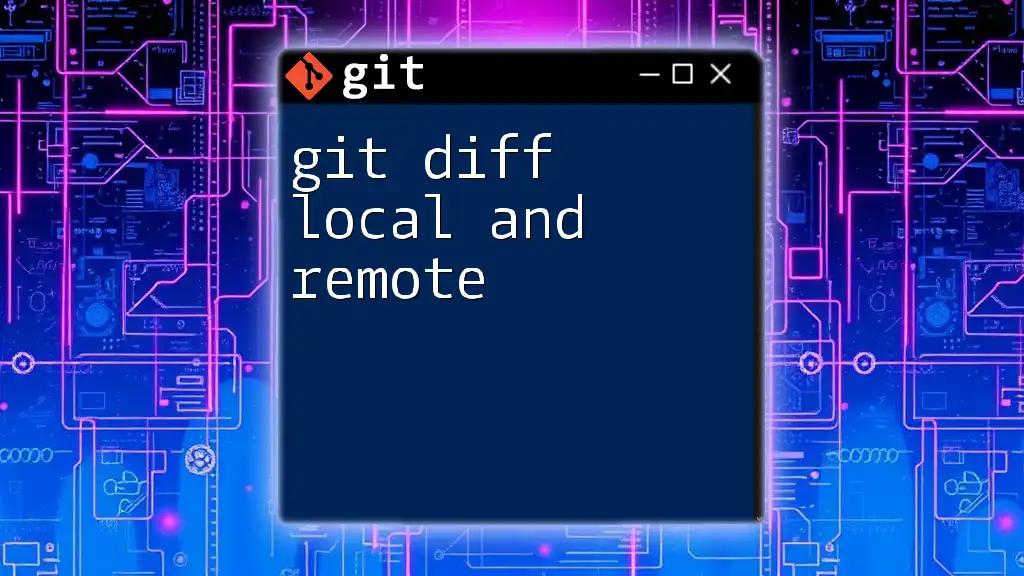
Best Practices for Managing Local Changes
Regularly Commit Changes
Making frequent commits is crucial when working with Git. Regular commits not only serve as checkpoints but also reduce the potential need for drastic changes. Maintain a habit of committing often; it makes managing your local changes a straightforward process.
Creating Feature Branches
Working in feature branches can significantly mitigate issues related to local changes. Instead of making modifications on the main branch, create a separate branch for new features:
git checkout -b feature-branch
Whenever you're ready to merge your feature into the main branch after your changes are vetted, you can do so without affecting the state of the primary project.
Utilizing Stashes for Work in Progress
Stashing is your safety net for ongoing work. If halfway through making changes, you suddenly need to deal with a critical issue, stash your changes. This practice results in less stress as you can confidently pull from the remote repository without worrying about losing track of your current work.
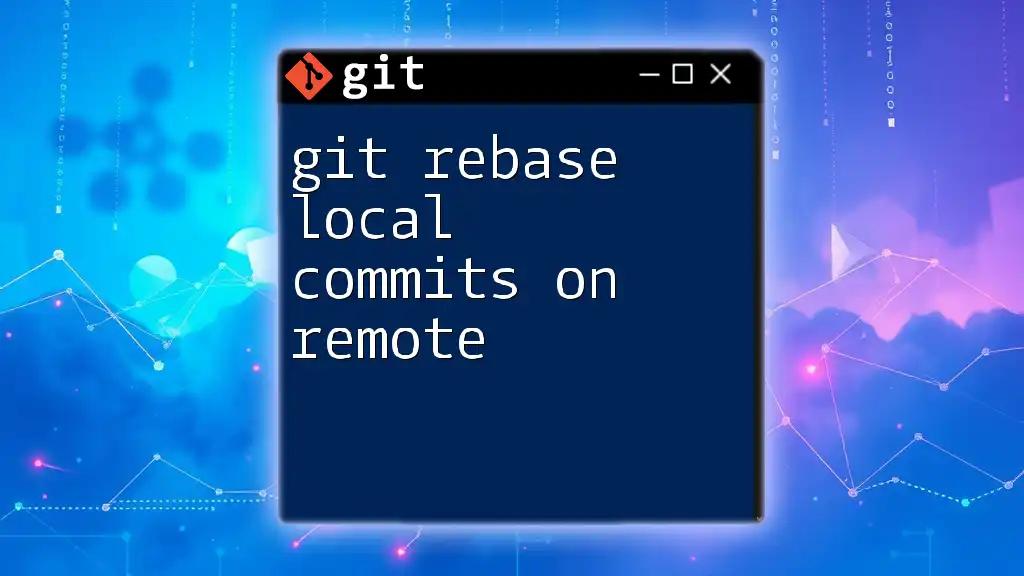
Conclusion
Understanding how to git discard local changes and pull from remote is fundamental for effective version control. Always assess your current state, and don’t hesitate to employ stashes where necessary. By regularly committing changes and branching properly, you can minimize risks associated with local changes and streamline your development workflow.
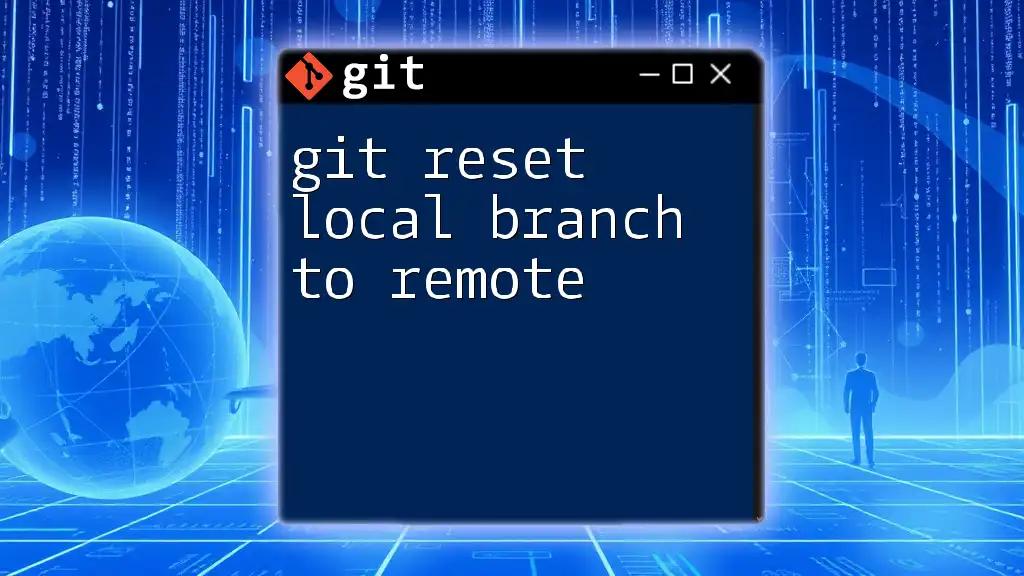
Additional Resources
For further reading and enhancement of your Git skills, you can refer to the official [Git documentation](https://git-scm.com/doc) and explore various online platforms offering Git tutorials and hands-on exercises.
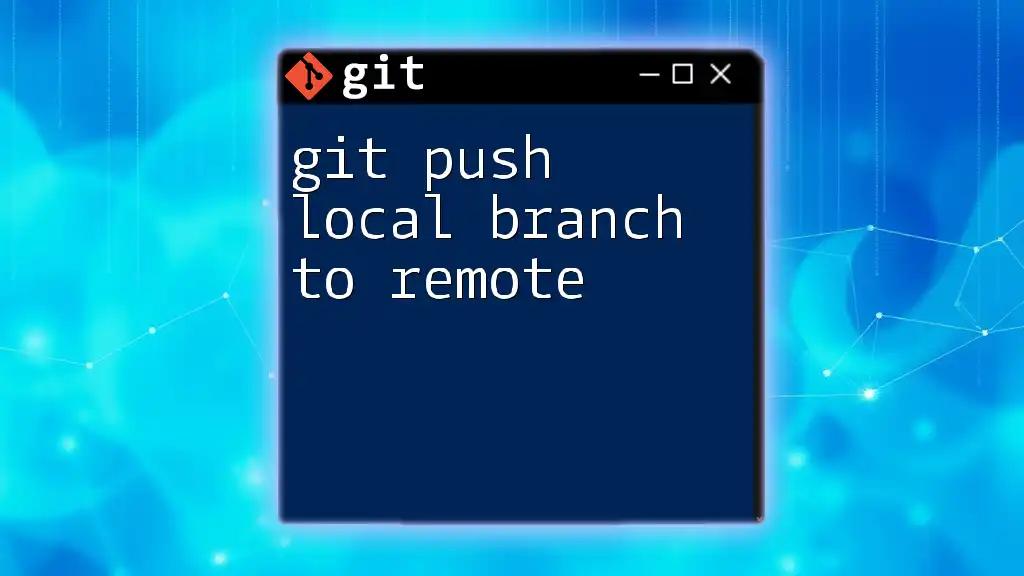
Call to Action
Stay updated with our blog for more insightful tips and tricks about using Git effectively. Share your experiences or questions in the comments below; we’d love to hear from you!