You can view the complete history of changes made to a specific file in a Git repository using the following command:
git log --follow -- [file-name]
Replace `[file-name]` with the actual name of the file you want to inspect.
Understanding Git Commands for File History
Key Git Commands
To effectively explore the git history of a file, it's essential to familiarize yourself with several key commands that form the backbone of your historical analysis within a Git repository.
The `git log` Command
What is `git log`?
The `git log` command serves as the main tool for displaying the commit history of a repository or, more specifically, a file. It provides crucial insights into who made changes, when they were made, and what those changes entailed.
Basic Usage of `git log`
To view the commit history for a specific file, you can execute the following command:
git log <file_path>
This command outputs a list of commits that have affected the file located at `<file_path>`. Each entry includes the commit hash, author name, date, and commit message, providing context for the changes made.
Filtering History with `git log`
View Commit History by Author
If you want to see changes made by a specific author, utilize the `--author` option. The command looks like this:
git log --author="Author Name" <file_path>
This filtering can aid in understanding the contributions of different team members and unraveling responsibilities for particular changes.
Limit the Number of Entries
Sometimes, too much information can be overwhelming. To limit the number of entries shown, the `-n` flag can be used:
git log -n <number> <file_path>
This command serves to show only the most recent `<number>` of commits, making it easier to focus on the latest changes.
Displaying Changes with `git log -p`
For a deeper look at what changed, you can add the `-p` option, which displays the patch. The command is:
git log -p <file_path>
This means you'll not only see the history of commits but also the specific lines that were added or removed with each commit—great for precise understanding.
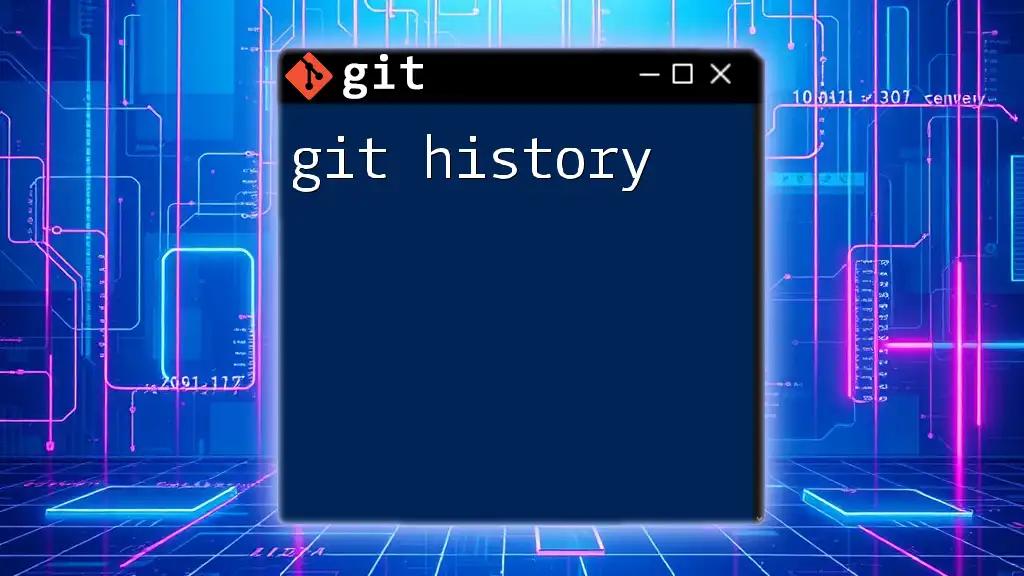
Advanced History Exploration Techniques
Using `git blame`
What is `git blame`?
The `git blame` command allows you to view the file's content alongside the last commit that modified each line. This is particularly helpful for identifying who is responsible for certain lines of code.
Example Command:
git blame <file_path>
Upon executing this command, you’ll receive output that annotates each line with the Commit hash, author, date, and the line number. This detail can clarify the lineage of code changes and improve accountability.
Using `git diff`
Comparing Changes Over Time
To compare the differences between two versions or commits of a file, the `git diff` command comes into play. It will highlight what has changed.
Example Command:
git diff <commit_hash_1> <commit_hash_2> -- <file_path>
This command will display the differences between the two specified commits for the file. Understanding these changes helps in tracing bugs or understanding enhancements.
`git show` for Specific Commits
Understanding a Specific Commit’s Changes
When you want to see the details of a specific commit pertaining to a file, `git show` is valuable.
Example Command:
git show <commit_hash> -- <file_path>
This command gives you comprehensive insights into the changes made in the specific commit relevant to your file, including the commit message and the associated changes. This is especially useful when you want to focus on one particular change without sifting through all modifications.
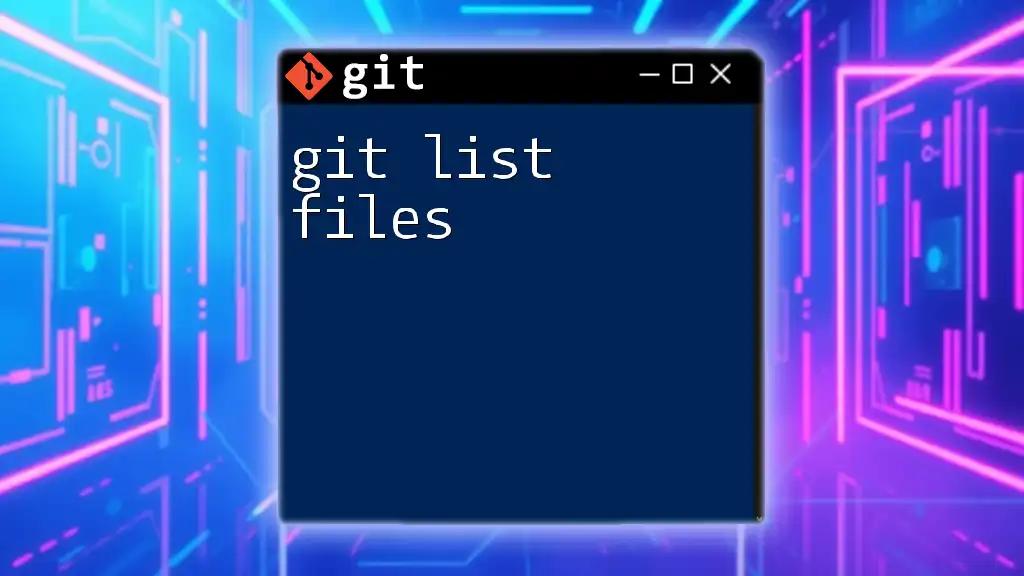
Visualizing File History with Git GUI Tools
Introduction to GUI Tools
While command-line tools are powerful, sometimes a visual approach makes understanding file histories more intuitive. Graphical user interfaces (GUIs) can complement the command line by making complex structures easier to comprehend.
Popular Git GUI Tools
-
GitKraken: A visually appealing tool that helps manage Git repositories effectively while allowing for easy visual exploration of the commit history.
-
SourceTree: Offers rich features for both beginners and advanced users, providing a straightforward visualization of file histories and commit graphs.
-
GitHub Desktop: Ideal for those working primarily with GitHub repositories, making it easy to navigate commits and file changes with a user-friendly interface.
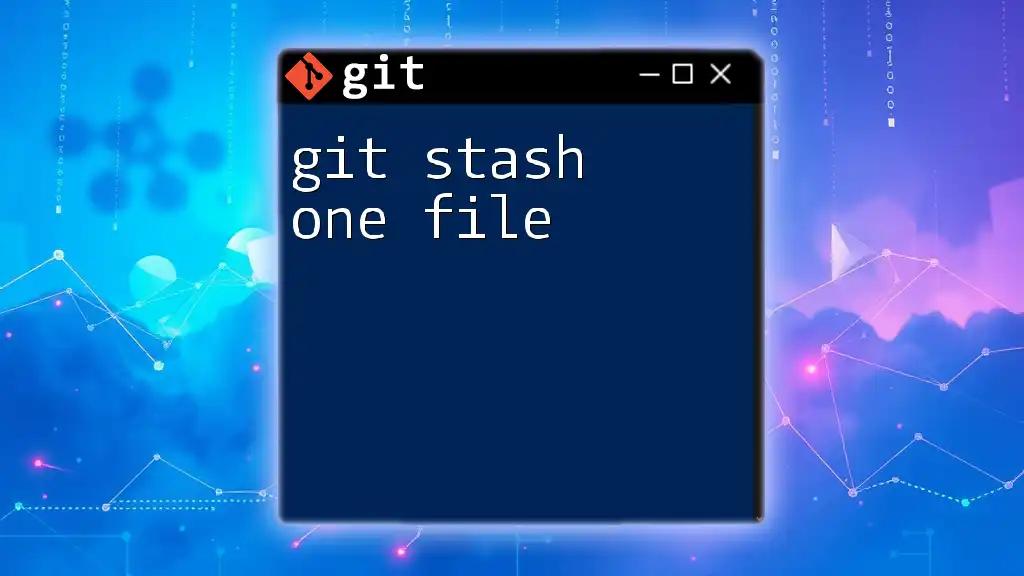
Practical Use Cases of File History Analysis
Debugging and Error Resolution
The git history of a file can be crucial in debugging. By reviewing past commits, you can pinpoint when a bug was introduced by examining the changes leading up to the issue. This helps to isolate and resolve problems swiftly.
Understanding Feature Development
Tracking the changes made during feature enhancements allows developers to grasp the evolution of code more effectively. Knowing how features have transformed over time can guide new feature implementations.
Conducting Code Reviews
The history of a file can play an essential role in code reviews. When evaluating changes, understanding who made alterations and the rationale behind them can provide context and improve the overall quality of the code collaboration process.
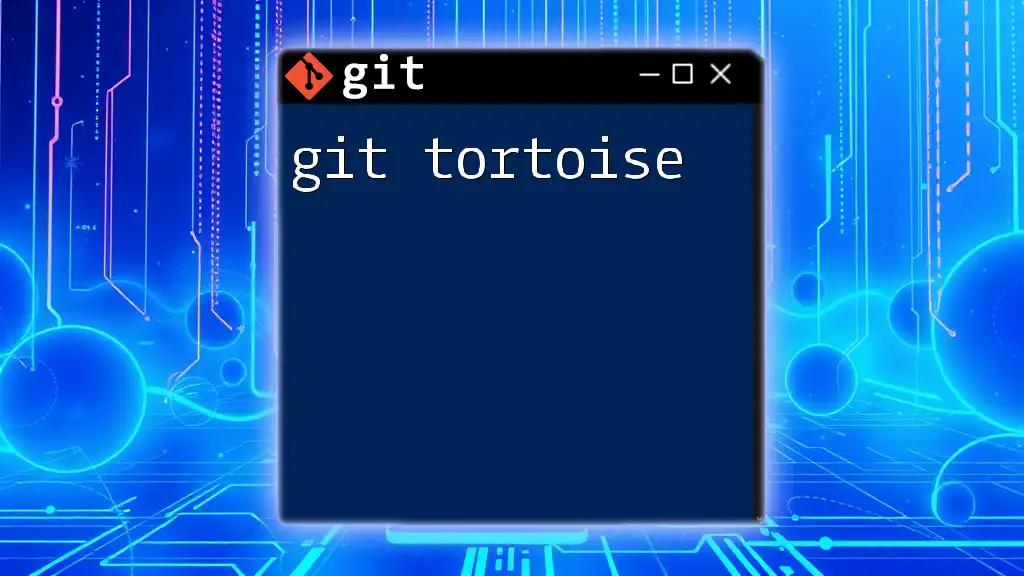
Common Mistakes and Best Practices
Mistakes to Avoid
A common pitfall is neglecting to filter your logs and blame outputs, leading to overloads of information that can confuse rather than clarify. Always start with concise commands and progressively add options as needed.
Best Practices for Using Git History Commands
- Be Specific: Utilize the commands with precise filters (e.g., by author or date) to get relevant results.
- Combine Commands: Use a combination of `git log`, `git blame`, and `git diff` to gain a comprehensive understanding of changes.
- Regular Review: Make it a habit to review file histories regularly, as this fosters better awareness of the codebase’s evolution.
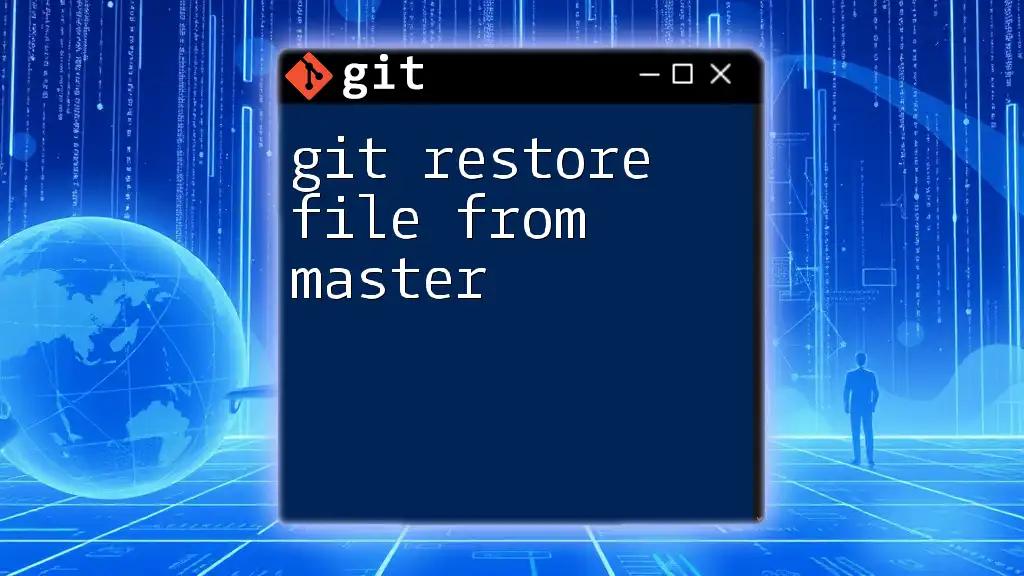
Conclusion
Understanding the git history of a file is not just about seeing the past—it’s about leveraging that knowledge to improve the present and guide future development. Exploring the history enables better collaboration, aids in troubleshooting, and enriches the overall development experience. Utilize the commands and techniques discussed here to gain profound insights into your files' histories, enabling you to make informed decisions in your projects.
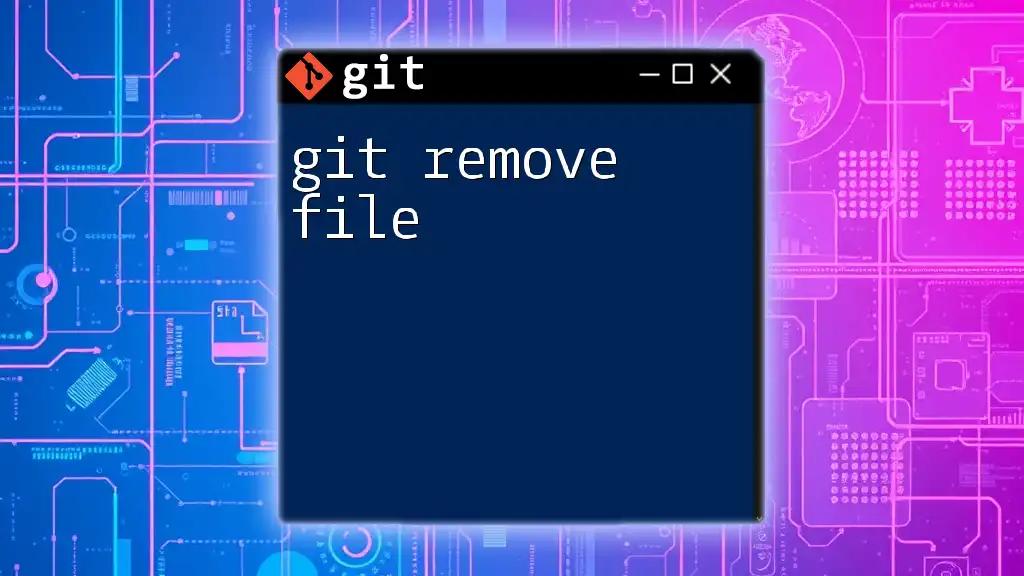
Further Reading and Resources
For additional information and in-depth learning, refer to the official Git documentation and explore online tutorials dedicated to mastering Git. As you learn, remember that practice is key to proficiency, so don’t hesitate to apply what you've learned in real projects.
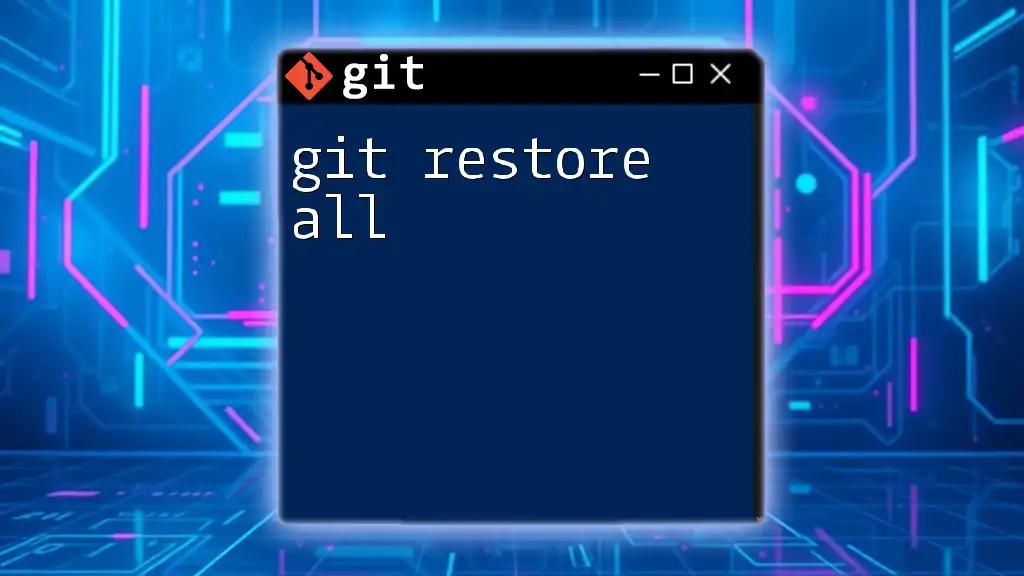
Call to Action
Join our course to deepen your understanding of Git commands and enhance your version control skills! Whether you are a beginner or looking to polish your expertise, we provide concise and practical guidance for all levels.