To copy a file in a Git repository, you can use the `cp` command followed by the source file and the destination path in your terminal.
Here's the syntax in a code snippet:
cp source_file.txt destination_directory/
Understanding Git and File Management
What is a Repository?
A Git repository is essentially a storage space for your project. It tracks changes in your files over time, allowing you to revert to earlier versions or collaborate with others seamlessly. Essentially, every Git repository contains a `.git` directory that holds all the metadata and version history associated with your project.
The Role of Files in Git
In Git, files can be categorized into two types: tracked and untracked. Tracked files are those that Git knows about—they are either staged for commit or have been committed previously. Untracked files, on the other hand, are new files that Git does not recognize until you explicitly add them.
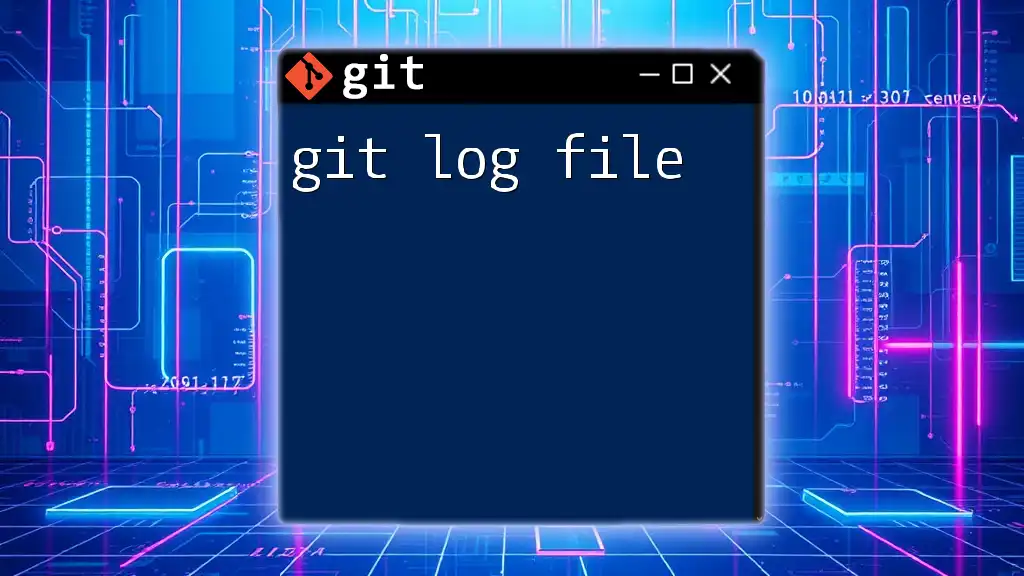
Copying Files in Git
Why Copy Files in Git?
Copying files is often necessary in various scenarios, such as:
- Backing up configurations before making changes.
- Creating a branch based on an existing file structure.
- Duplicate specific code snippets for quick experimentation.
Basic File Copying Commands
Using the `cp` Command
The `cp` command is a fundamental command used in Unix-based systems for copying files. Its syntax is straightforward:
cp [options] <source> <destination>
For instance, if you want to create a backup of a file named `myfile.txt`, you would run:
cp myfile.txt myfile_backup.txt
This command simply copies the content of `myfile.txt` to a new file called `myfile_backup.txt`. After executing this, both files will exist independently.
Copying Files Within a Git Repository
When copying files within a repository, it's vital to ensure that the newly copied file is tracked by Git. You could copy a configuration file using the following command:
cp src/config.json backup/config.json
After executing this command, you will want to verify that the `backup/config.json` is now recognized by Git. To do that, simply run:
git status
If the file doesn't appear under "Changes not staged for commit," you will need to add it using:
git add backup/config.json
Copying Files in Different Contexts
Copying Files Across Branches
You can copy files from one branch to another using the `git checkout` command. This technique lets you keep your current workspace clean while accessing files from a different branch.
For example, if you need a specific file from a branch called `feature-branch`, you can execute:
git checkout feature-branch -- path/to/file.txt
This command pulls the version of `file.txt` from `feature-branch` and places it in your current working directory. Note that if you have unsaved changes in `file.txt`, it may lead to conflicts, so make sure to manage those beforehand.
Using Git Commands to Duplicate Changes
Stashing Changes
In some cases, you might want to copy changes but are not ready to commit them. Here, `git stash` comes into play. You can temporarily save changes in your working directory using the command:
git stash
This command clears your working directory, allowing you to copy files without worrying about losing your modifications. Once the copies are made, you can apply stashed changes back into your working environment:
git stash apply
Advanced File Copying Techniques
Getting a Specific Version of a File
If you need to retrieve an earlier version of a file, you can accomplish this using the `git checkout` command with the commit ID. First, locate the commit ID using:
git log
Once you have the necessary commit ID, run the following command:
git checkout <commit_id> -- path/to/file.txt
This command retrieves the state of `file.txt` from the specified commit, allowing you to access previous versions easily.
Using Aliases for Quick Copying
Creating a custom alias can significantly speed up your workflow. You can set up an alias in your Git configuration that allows you to copy files quickly. For example:
git config --global alias.copy '!f(){ cp "$1" "$2"; git add "$2"; }; f'
With this alias, you can execute the following command to copy a file and stage it at the same time:
git copy path/to/source.txt path/to/destination.txt
This streamlines the often-repeated steps involved in file copying and staging.
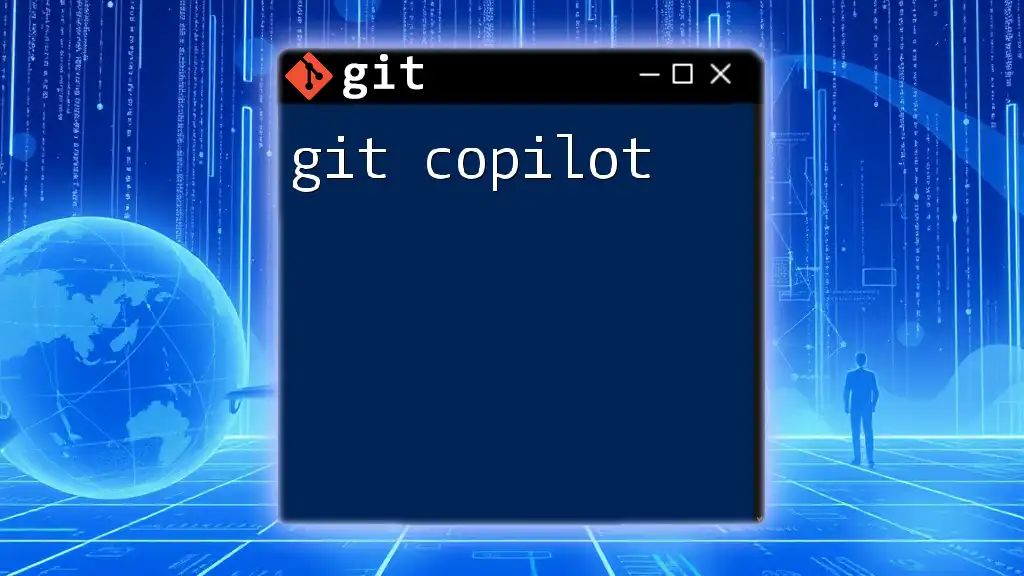
Common Issues When Copying Files in Git
Not Tracking the Copied File
One common pitfall when copying files is forgetting to track the new file. After copying your file, it's vital to stage it to ensure that Git recognizes it. You can do this by running:
git add path/to/copied_file.txt
Neglecting this step can result in a missed commit.
Handling Merge Conflicts When Copying
If you’re working across branches and copy files that are also modified in the destination branch, merging becomes tricky. Git may throw conflicts your way, making it crucial to resolve these before proceeding with any staging or commits.
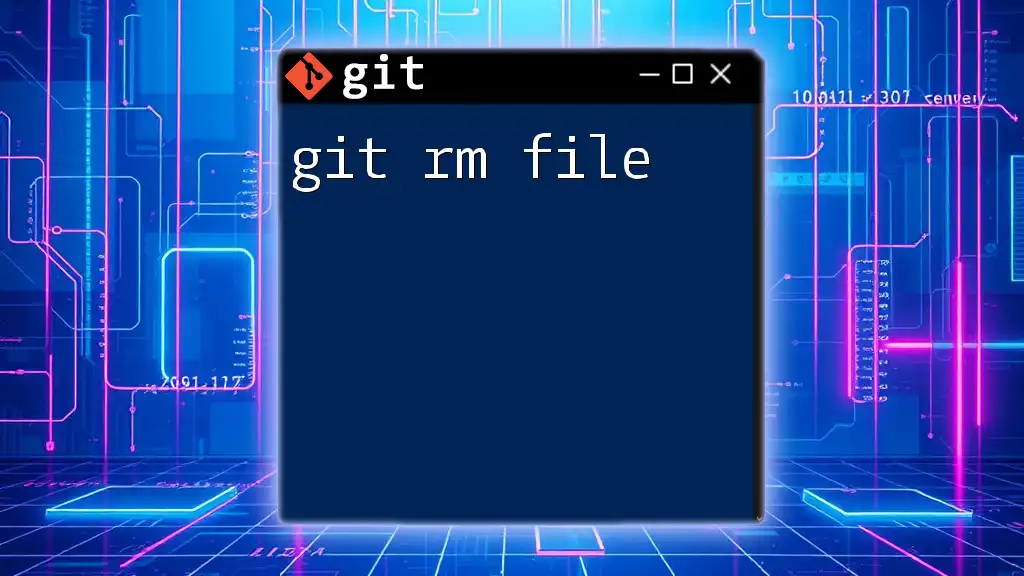
Best Practices for Copying Files in Git
Keep Commits Meaningful
Using clear and descriptive commit messages when copying files can be highly beneficial. It allows you and other collaborators to understand the context behind changes and quickly assess the relevance of past operations.
Organize Directories Thoughtfully
A well-structured directory can make file management much easier. Consider grouping related files together and maintaining a clean organization to minimize confusion when copying and accessing files across various parts of your project.
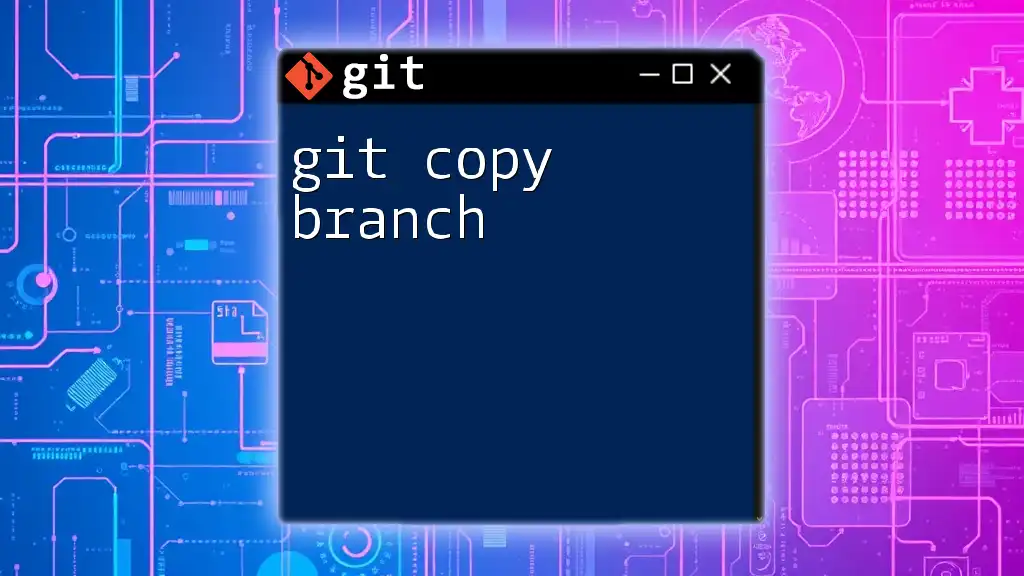
Conclusion
In summary, understanding how to efficiently git copy file is crucial for effective file management within your Git repositories. Armed with practical techniques and knowledge, you'll be able to copy files seamlessly, ensuring a more organized and productive environment. Dive into practicing these commands and see how they can simplify your workflow!
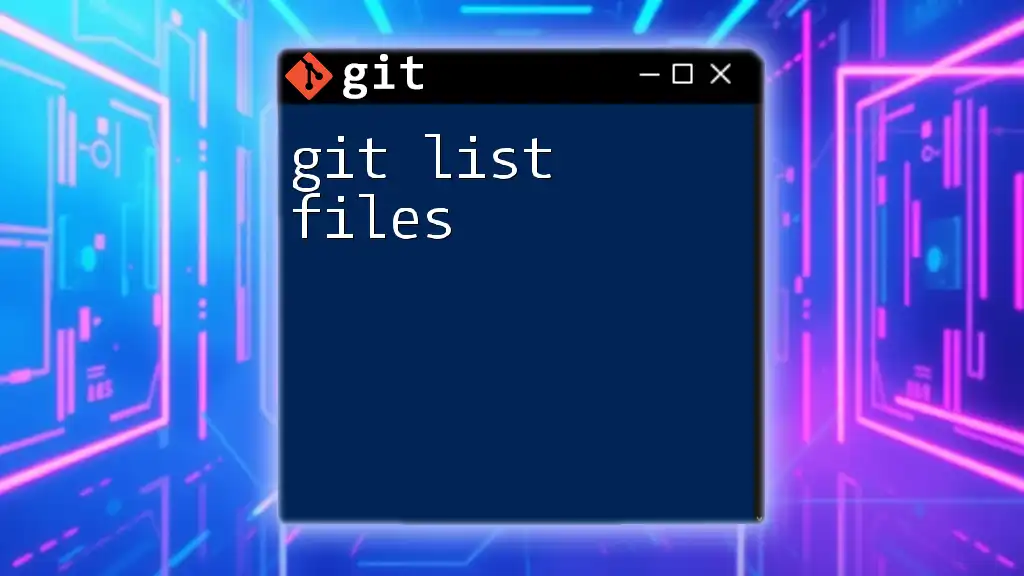
Additional Resources
To further enhance your understanding and implementation of Git, consider checking out the official Git documentation and explore various tutorials and courses available online.