To clone a specific branch of a Git repository into an already existing folder, you can use the following command with the `--single-branch` option and specify the branch name.
git clone --single-branch --branch <branch-name> <repository-url> <existing-folder>
Understanding Git Cloning
What is Git Cloning?
Git cloning is a fundamental operation in version control that allows you to create a copy of a repository from a remote server to your local machine. This includes not only the files but also the entire repository history. By cloning, you gain access to the project code, branches, and commit history, enabling you to collaborate and contribute effectively.
The Importance of Branches in Git
Branches are pivotal in Git because they allow developers to work in parallel on features, bug fixes, or experiments without affecting the primary codebase. When you want to work on a specific feature in an isolated workspace, you typically do this on a separate branch. Cloning a specific branch is especially useful when the project has multiple ongoing developments, as it helps to focus on what is relevant without cluttering your active environment with unnecessary files.
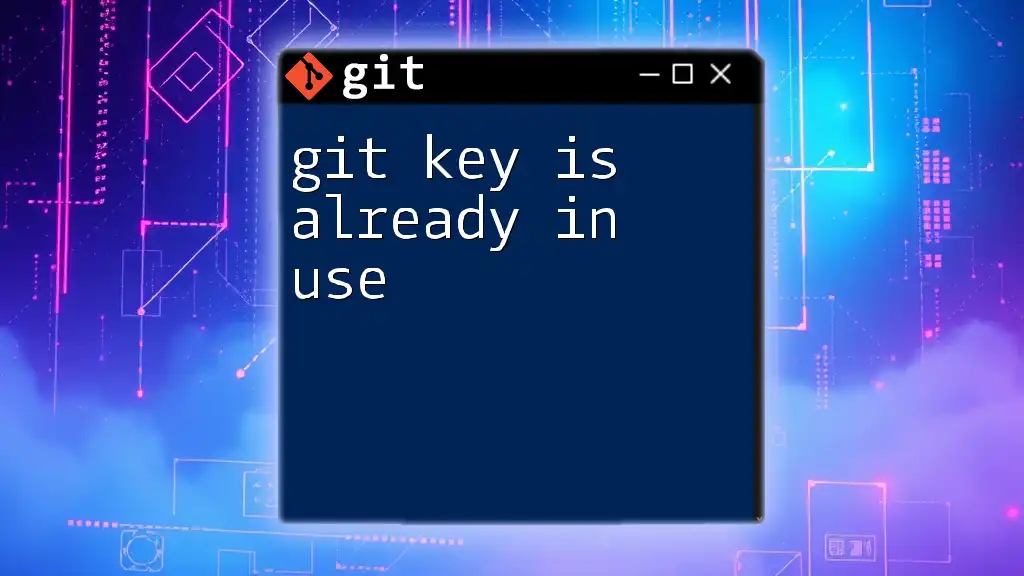
Prerequisites
Setting Up Your Environment
Before diving into the command, ensure you have Git installed on your machine. You can follow links to installation guides for your operating system. After installation, configure Git with your user name and email to keep track of your contributions effectively:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Checking for Existing Folders
It's crucial to confirm the existence of the folder where you intend to clone the branch. You can do this by navigating using the command line. To check your current directory, use:
pwd
You can also list the directories in your current location with:
ls
Ensure that the targeted directory does not contain files that may conflict with the cloning process.
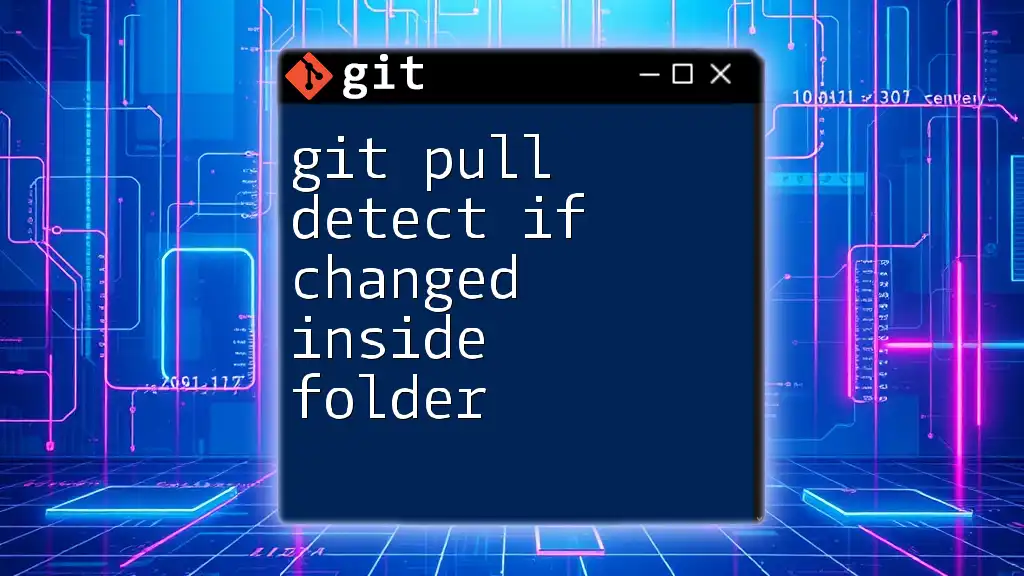
Cloning a Specific Branch into an Existing Folder
General Syntax of Clone Command
The general syntax for the `git clone` command is straightforward. Here’s how it looks:
git clone <repository> <folder-name>
However, when you particularly want to clone a branch into an already existing folder, we will use specific options.
Steps to Clone a Branch
Step 1: Navigate to the Desired Folder
First, use the command line to navigate to the folder where you want the branch's contents placed. Use the `cd` command:
cd /path/to/existing/folder
Make sure you replace `/path/to/existing/folder` with your actual path.
Step 2: Use the --single-branch Option
To clone a specific branch into the folder, you can use the `--single-branch` option. This option ensures that Git fetches only the history for the branch you're interested in, rather than the entire repository. The command format looks like this:
git clone --single-branch --branch <branch-name> <repository-url> .
The dot (`.`) at the end signifies that you want to clone the branch's content into the current directory.
Example Use Case
Let's say you want to clone the branch named `feature-branch` from a repository located at `https://github.com/user/repo.git`. The command would be:
git clone --single-branch --branch feature-branch https://github.com/user/repo.git .
This action will copy the contents of the `feature-branch` into your currently navigated existing folder.
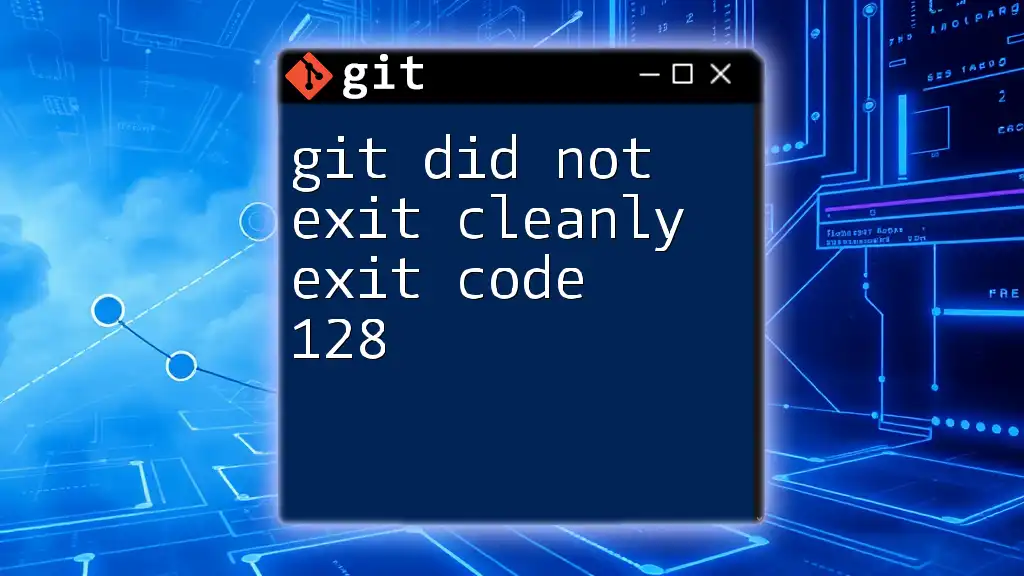
Handling Common Errors
Error: Directory Not Empty
If the target directory is not empty, Git will throw an error. This is because Git cannot clone into a directory that already contains files or folders. To resolve this, you can either clear the folder or use the above command with the dot (`.`) to indicate that you want the files added to the existing contents.
Error: Remote Repository Not Found
In some cases, you may encounter an error saying that the remote repository isn't found. This can typically happen due to a typo in the URL. To fix this, double-check the URL you’re using to ensure that it is correct and accessible.
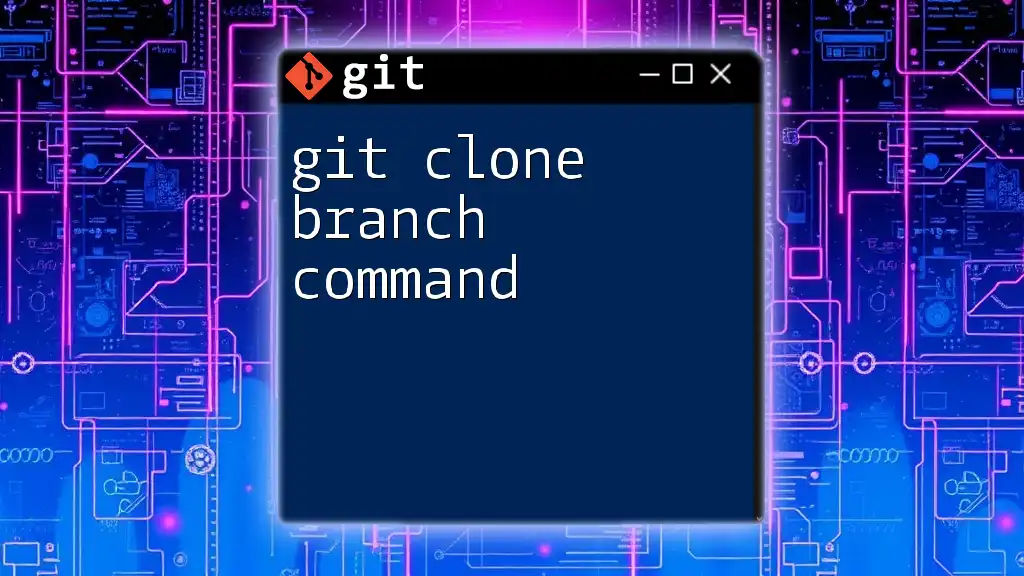
Best Practices for Cloning
Verifying the Clone
After cloning, it's a good idea to check the status of your newly cloned repository. You can do this by running:
git status
This command will give you an overview of the current state of the repository, confirming that you are on the desired branch.
Keeping Your Cloned Repo Updated
To ensure you're working with the latest changes in the cloned branch, it’s important to regularly fetch updates. You can do this simply with:
git fetch origin <branch-name>
git pull origin <branch-name>
Working With Multiple Branches
Post-cloning, you might want to switch between different branches. Use the `git checkout` command for this purpose:
git checkout another-branch
This command will switch your working directory to `another-branch`, allowing you to work in a different context without retrieving all branches unnecessarily.
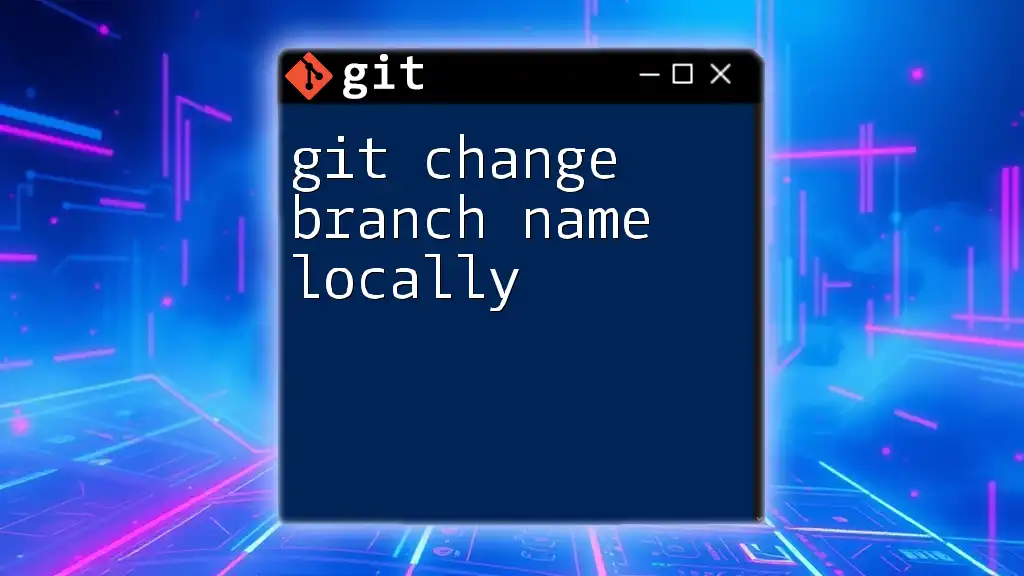
Conclusion
Cloning a specific branch into an existing folder is a practical skill that enhances your efficiency in collaborative projects using Git. By understanding how to use Git clone effectively, especially for specific branches, you can streamline your workflow and focus on what matters most in your development environment. Remember to practice and explore further Git commands to expand your proficiency in version control!
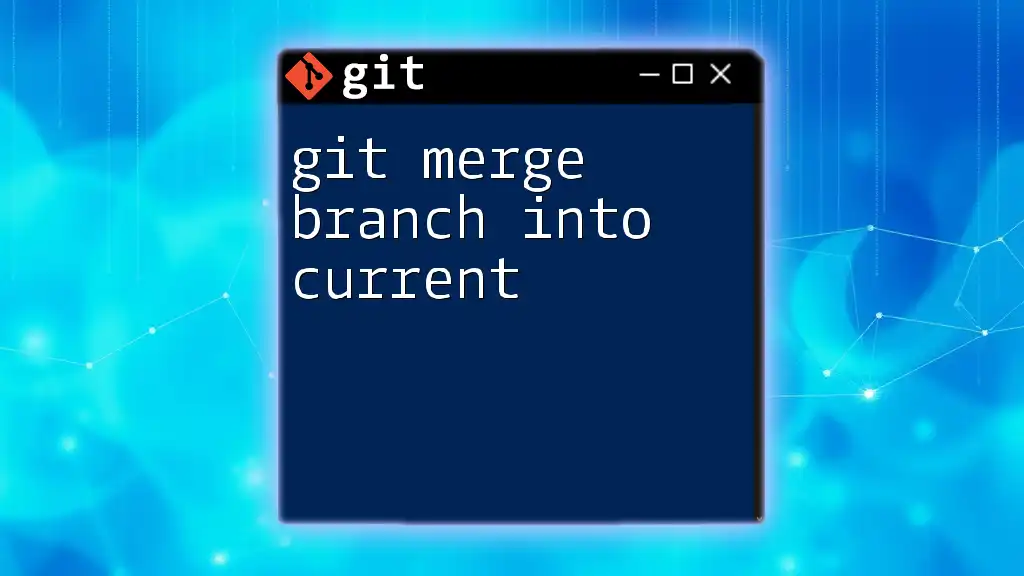
Additional Resources
For further learning, consult the official Git documentation and consider engaging in tutorials that delve deeper into Git's capabilities. Embrace the power of Git and enhance your coding journey!
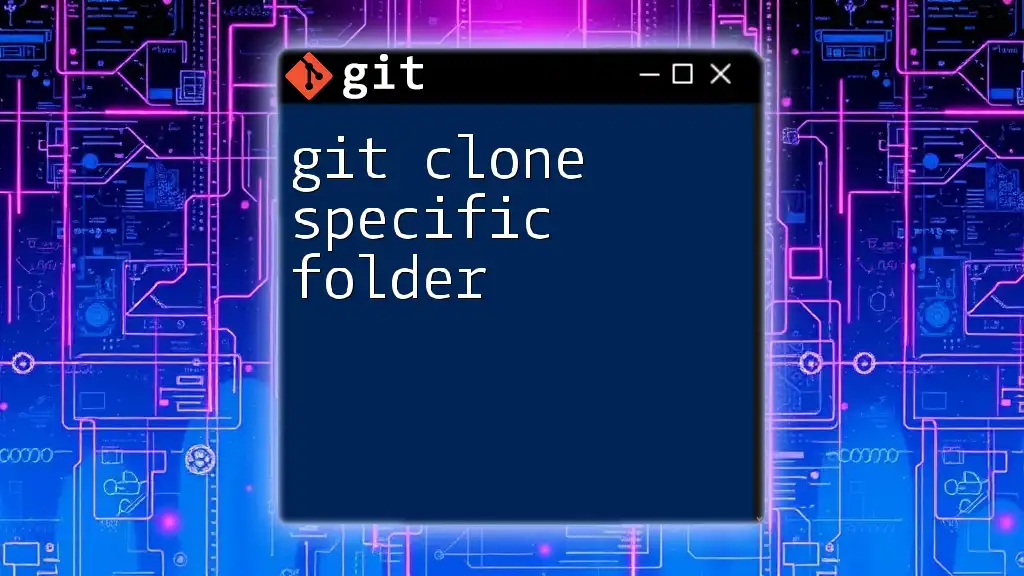
Frequently Asked Questions (FAQs)
Can I clone a repository without cloning its entire history?
Yes, you can use shallow cloning with the `--depth` option to limit the history being cloned.
What if I want to keep the existing files in the folder?
You can safely use the dot notation (`.`) in your clone command, as it will integrate the cloned content with the existing files without deletion, provided there are no conflicting files.
How can I switch back to the main branch after cloning?
You can switch back to the main branch easily using:
git checkout main
This command allows you to return to your main working branch, facilitating easier access to your main codebase after feature work or experiments.
Play around with these commands and become skilled in managing your Git repositories effectively!