Git is a powerful version control system that enables developers to track changes in their code, collaborate seamlessly, and maintain a history of their projects to enhance productivity and teamwork.
Here's a basic example of how to clone a repository using Git:
git clone https://github.com/username/repository.git
Version Control
Understanding Version Control
Version control is a systematic way of managing changes to documents, programs, and other information stored as computer files. It primarily serves the software development community, allowing developers to track modifications made to code over time. The importance of version control in software development cannot be overstated: it provides a safety net for developers, ensures code integrity, and facilitates collaboration among team members.
Using Git as a version control system offers several key advantages:
- Backup: Git stores your project’s history, allowing you to revert to previous states if necessary.
- Collaboration: Multiple developers can work on different features simultaneously without overriding each other's work.
Examples of Version Control in Git
Git makes it easy to manage and navigate through versions. Here are some essential commands that highlight Git's effectiveness in version control:
-
Creating a new repository:
git init my-project
This command creates a new directory with a `.git` subdirectory—in effect initiating a new Git repository.
-
Committing changes:
git add . git commit -m "Initial commit"
The `git add .` command stages changes for the next commit, while `git commit -m "Initial commit"` records those changes in the repository history with a descriptive message.
-
Viewing history:
git log
This command displays a chronological list of all commits, along with details like the author's name, date, and commit message.
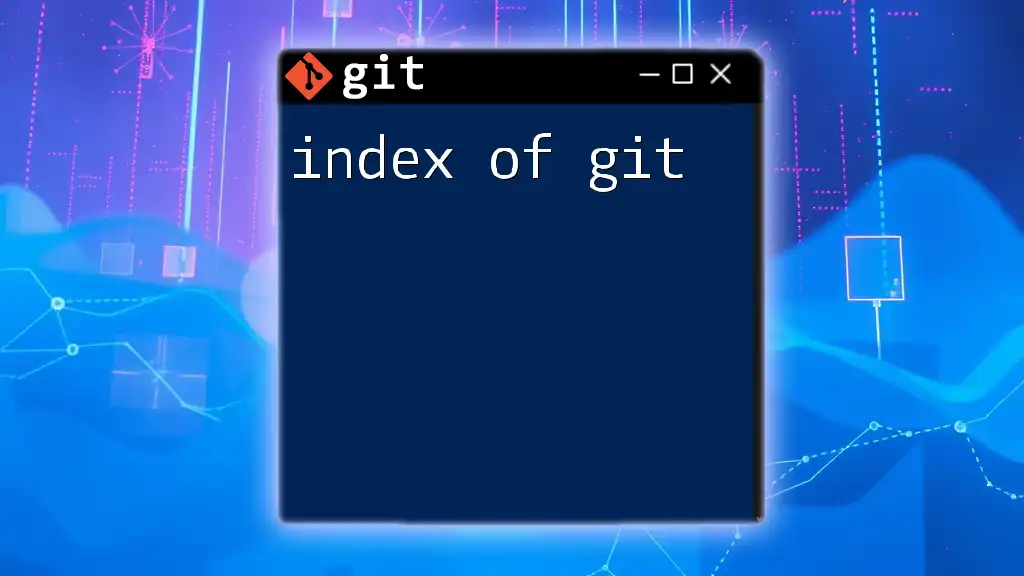
Collaboration
How Git Enables Team Collaboration
One of the standout features of Git is its ability to enhance collaboration among multiple developers. This is largely due to its branching strategy. When developers work on new features or bug fixes, they can create separate branches that allow them to experiment without affecting the main codebase.
Using Branching in Git
Branches are integral to effective collaboration. They allow developers to work independently and manage changes flexibly. Here’s how to use branching:
-
Creating branches for features:
git checkout -b feature-branch
This command creates a new branch named `feature-branch` and switches your working directory to that branch, enabling you to develop new features without any interruptions to the main branch.
-
Merging branches:
git checkout main git merge feature-branch
After developing on your feature branch, you can merge it back into the main branch, incorporating your changes into the primary codebase.
Avoiding Merge Conflicts
Merge conflicts can occur when two branches have competing changes to the same line of code. To minimize the potential for these conflicts, consider following these strategies:
- Frequent communication: Keep lines of communication open among team members regarding who is working on what.
- Regular merging: Frequently merge branches to the main repository to minimize discrepancies.
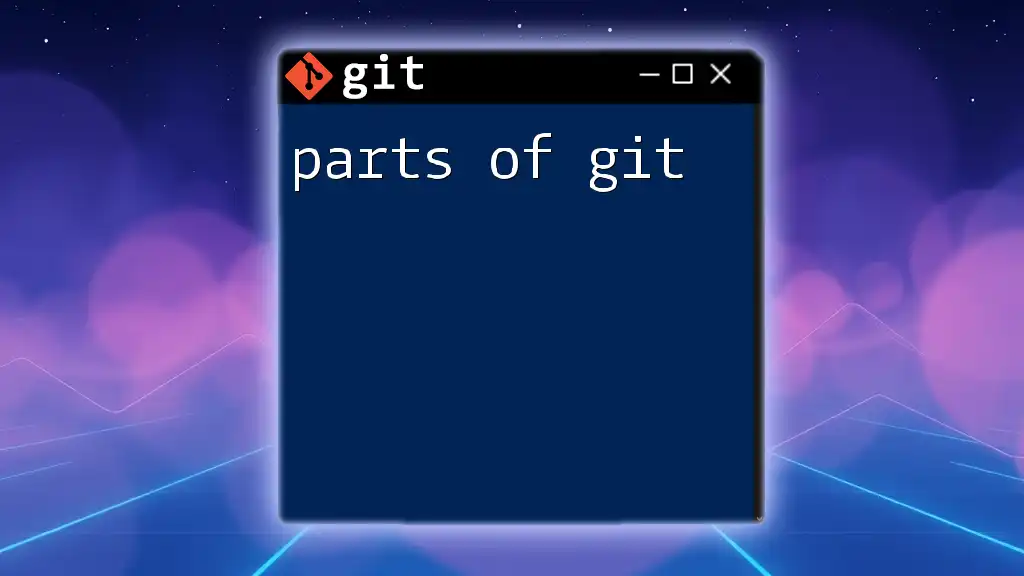
Code Review and Quality Assurance
Importance of Code Reviews in Team Projects
Code reviews are a crucial part of the software development lifecycle. They ensure that the code meets project standards and identifies potential bugs or vulnerabilities early in the development process. Git simplifies the code review process through its branching and merging capabilities.
Using Pull Requests (PRs)
Pull requests are a primary tool in collaborative Git usage. They facilitate discussion around code changes before integration, allowing team members to review and suggest modifications.
- Creating a pull request in GitHub: After pushing your changes to a new branch, you can create a pull request in GitHub by navigating to the repository and clicking the "New pull request" button. This enables code review and discussion around the proposed changes before they are merged.
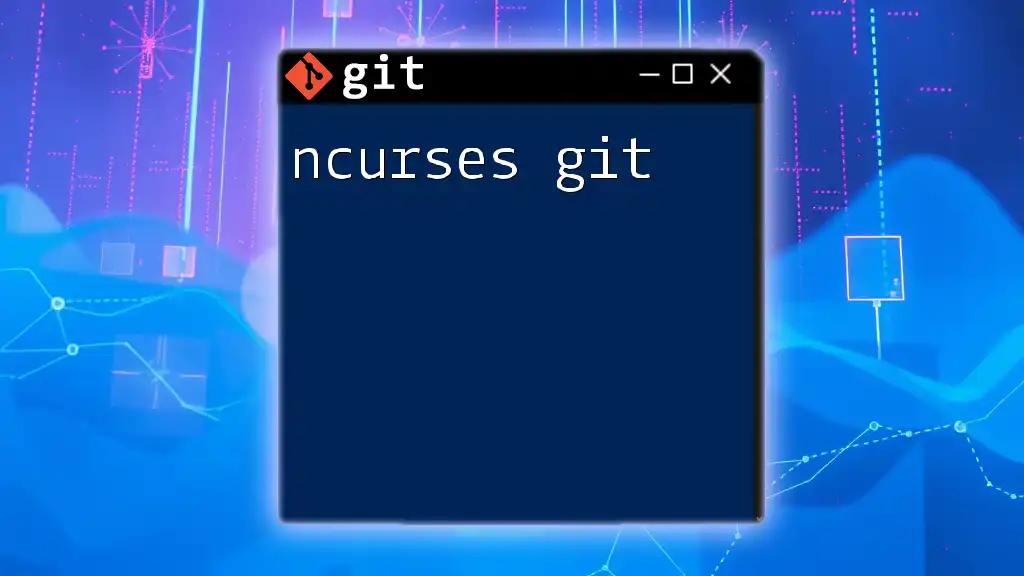
Continuous Integration and Deployment (CI/CD)
Linking Git with CI/CD Tools
Continuous Integration and Deployment (CI/CD) are practices that encourage frequent integration of code changes and automatic deployment to production environments. Utilizing Git with CI/CD tools streamlines this process, ensuring your code is always in a deployable state.
Automating Deployment Processes
Git hooks are powerful scripts that Git executes at certain points in the version control process. They can be used to automate deployment upon successful commits.
- Example of a post-commit hook:
This script would run automatically after every commit, allowing you to trigger deployments seamlessly.# .git/hooks/post-commit #!/bin/bash echo "Deploying application..."
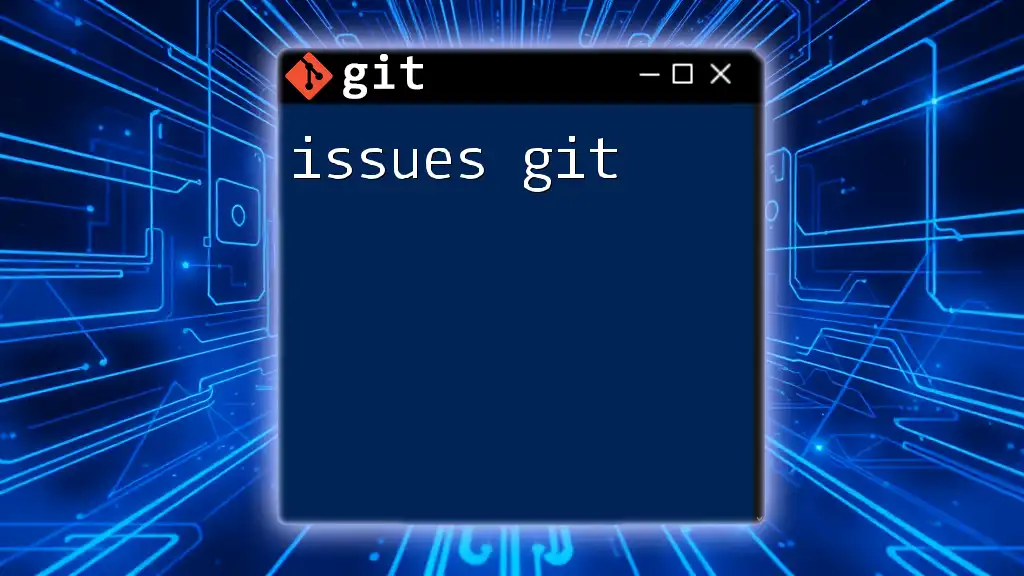
Backup and Recovery
How to Use Git for Backups
Keeping backups of your code is vital for any project. Git allows you to clone repositories, which helps ensure that your code is safe and retrievable.
- Cloning a repository for backup:
This command duplicates the repository to your local machine, allowing you to retain a copy of your project.git clone https://github.com/username/my-project.git
Recovering from Mistakes
Git offers several functionalities that help you recover from mistakes, making it easier to navigate tricky situations.
-
Discarding changes in the working directory:
git checkout -- filename
This command allows you to revert uncommitted changes to a file, ensuring you don't accidentally keep unwanted modifications.
-
Reverting a commit:
git revert <commit-hash>
If you need to undo a committed change, `git revert` helps you safely create a new commit that negates the effects of the specified commit.
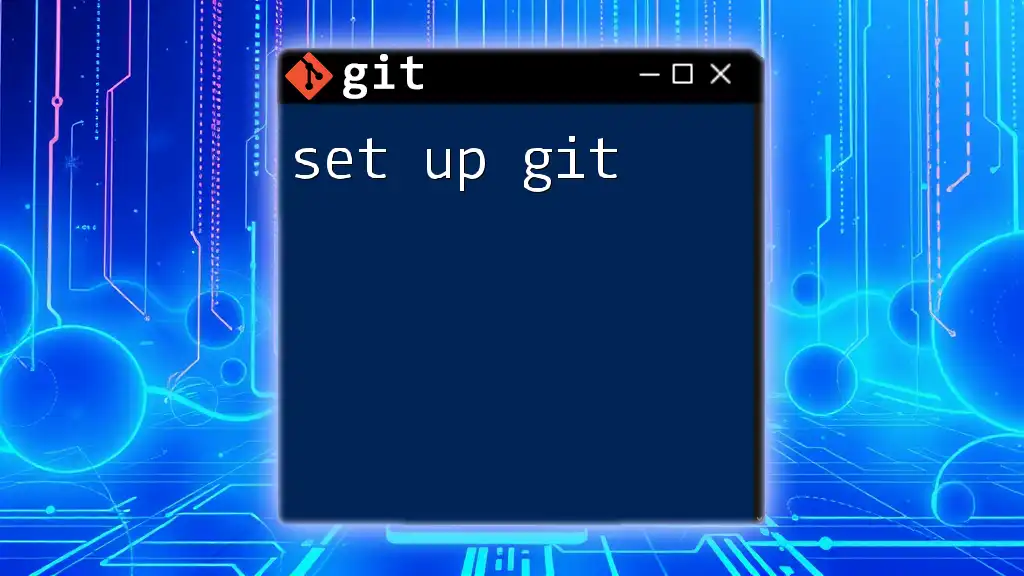
Cross-Platform Compatibility
Git’s Versatility
Git’s design allows it to function seamlessly across various operating systems, including Windows, macOS, and Linux. This cross-platform compatibility ensures that development teams can collaborate effectively, regardless of their choice of environments. Moreover, Git can be integrated into various Integrated Development Environments (IDEs) and platforms, facilitating more intuitive workflows for developers.
In summary, Git is an extraordinarily powerful tool within the software development realm, offering a suite of features that cater to version control, collaboration, code quality, deployment, and recovery. By learning the uses of Git, individuals and teams can significantly enhance their development processes and project outcomes.