"PHP Git" refers to the integration of Git version control commands within the context of PHP development to manage source code efficiently. Here's a code snippet to demonstrate how to initialize a Git repository in a PHP project:
git init
What is Git?
Git is a powerful version control system that allows developers to track changes in their code, collaborate with others, and manage project histories effectively. It provides a systematic way to keep versions of a project, enabling developers to work with files and directories seamlessly.
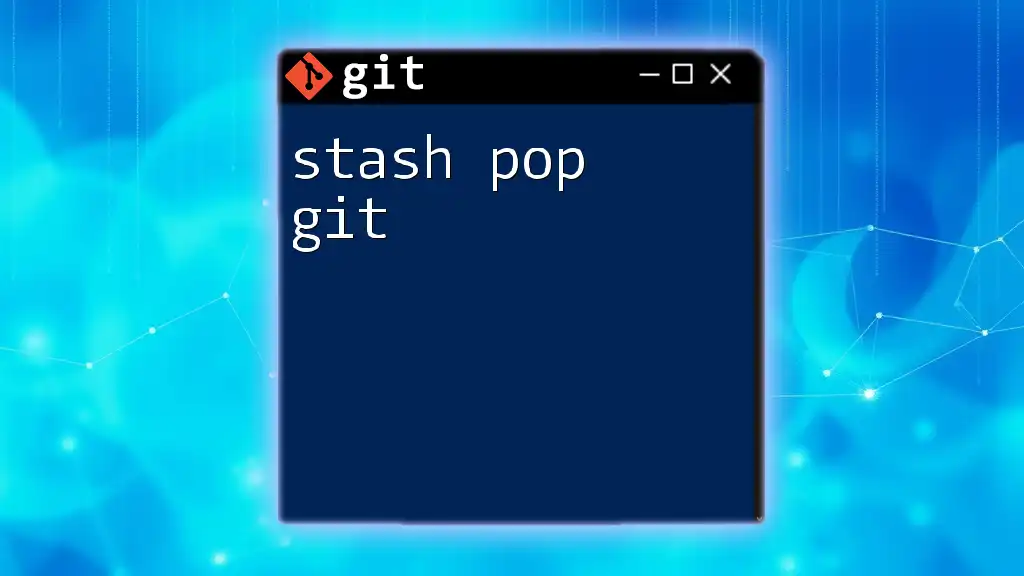
Why Use Git with PHP?
Using Git with PHP projects brings numerous advantages, including:
- Collaboration: Multiple developers can work on the same project and merge changes without overwriting one another's work.
- Version History: You can track every change made to your project, which is invaluable for debugging and understanding project evolution.
- Branching: Git allows you to create branches for new features or fixes, meaning you can develop in isolation and merge only when ready.
Common PHP frameworks like Laravel and Symfony also benefit from Git's capabilities, making it essential for modern PHP development.
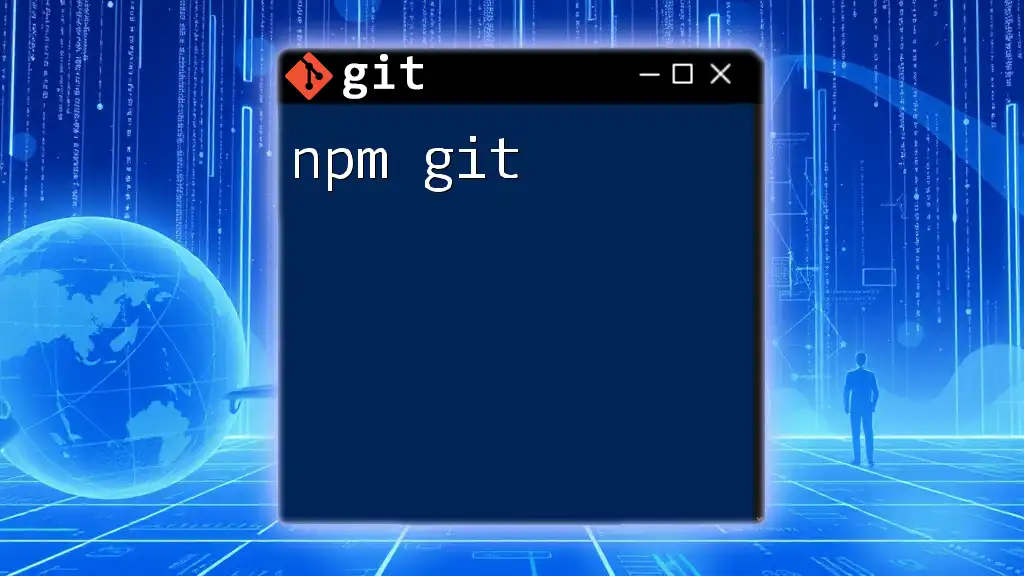
Setting Up Your Git Environment for PHP
Installing Git
To get started, you first need to install Git.
Step-by-Step Installation Guide
-
Windows: Download and install Git from the official [Git website](https://git-scm.com/download/win).
-
macOS: You can install Git through Homebrew using the following command:
brew install git
-
Linux: On Ubuntu, use the command:
sudo apt-get install git
Verifying Your Installation
To confirm that Git is installed correctly, open your terminal and run:
git --version
This command will display the installed version of Git.
Configuring Git
Once installed, you should configure Git to use your identity.
Setting Up User Information
Set your username and email to link your commits to your identity:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Configuring Other Settings
You can set a default text editor for commit messages and configure color settings for your Git output. For example:
git config --global core.editor "code --wait" # For Visual Studio Code
git config --global color.ui auto # For colored output
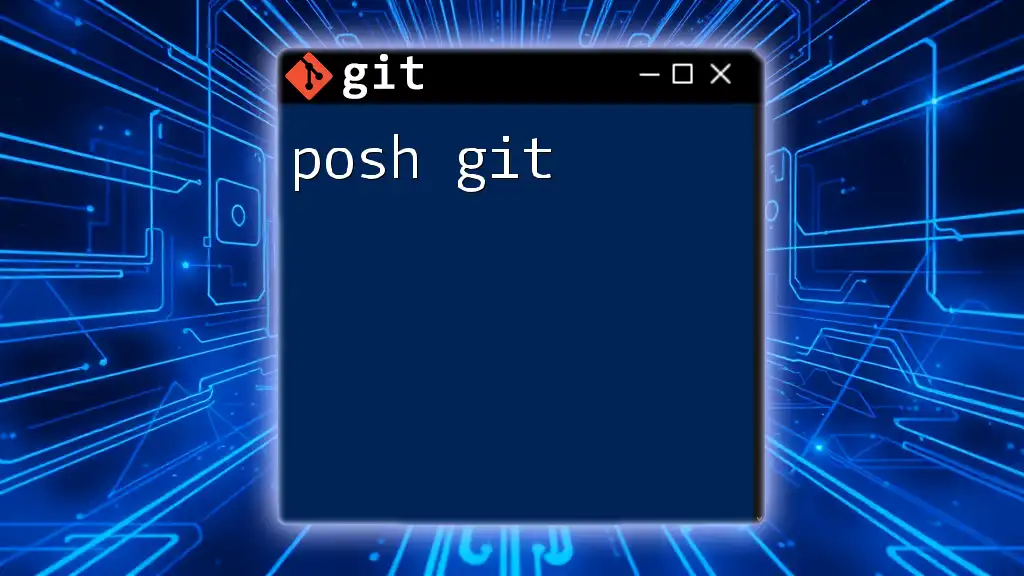
Creating a New PHP Project with Git
Initializing a Git Repository
To create a new PHP project and set up Git:
Creating a New Directory
First, create and navigate to your project directory:
mkdir my-php-project
cd my-php-project
Initializing Git
Now, initialize your new Git repository:
git init
You'll now have a `.git` directory in your project folder, establishing it as a Git repository.
Adding Your PHP Files
Creating a Sample PHP File
Let's create a simple PHP file, `index.php`, that will serve as your project's starting point:
<?php
echo "Hello, World!";
?>
Tracking Your Changes
After creating your file, you'll need to stage it for tracking. Use the following command:
git add index.php
This tells Git to include this file in the next commit.
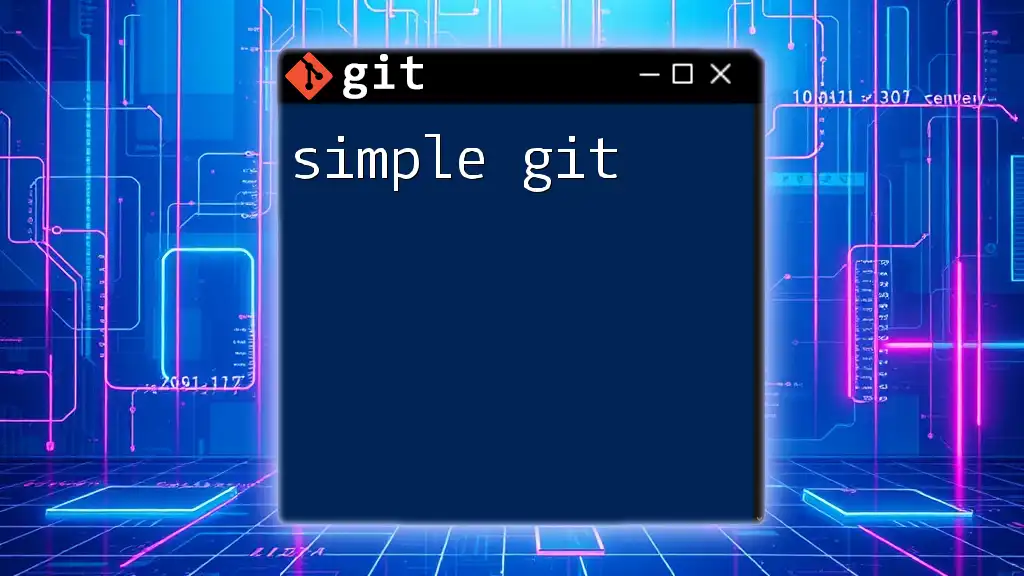
Committing Changes in Your PHP Project
Making Your First Commit
Understanding the Commit Process
Committing is crucial in Git as it records your changes and provides a snapshot of your project. A well-crafted commit message enriches the history of your project.
Making a Commit
To commit the staged file, run:
git commit -m "Initial commit: Created index.php"
This command saves the current state of your project. It’s important to use descriptive messages for future reference.
Viewing Commit History
To see the history of your commits, you can use:
git log
This command will display an ordered list of all commits you've made, including their messages and timestamps.
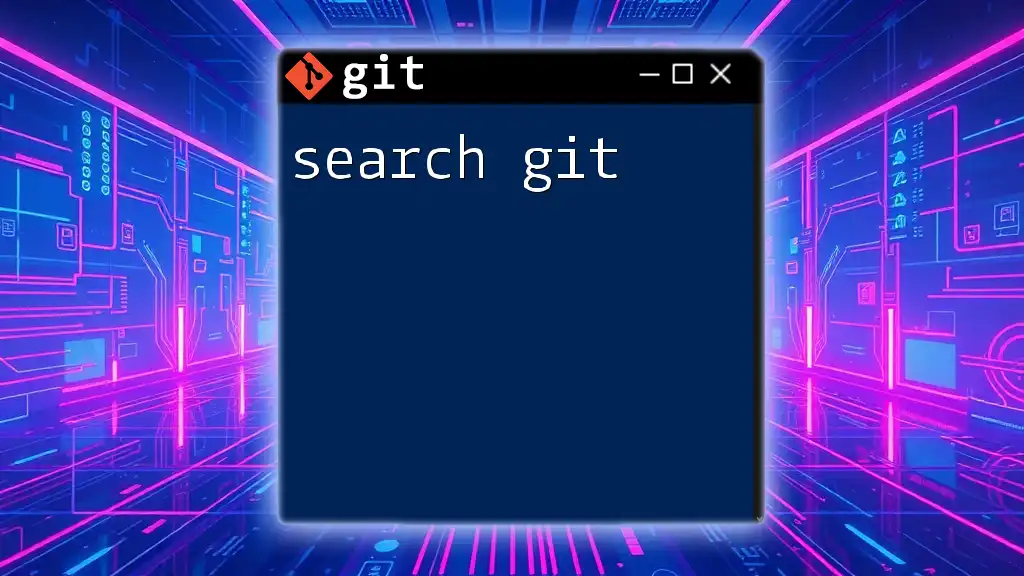
Branching and Merging in PHP Projects
What are Git Branches?
Branches are fundamental to Git, enabling you to develop new features or fix bugs without affecting your main codebase.
Understanding Branching
Branching allows for parallel development. A branch can diverge from the main line of development and be merged back later.
Working with Branches
Creating a New Branch
To create and switch to a new branch:
git branch new-feature
git checkout new-feature
Merging Branches
Once you've developed your feature and are ready to integrate it back into the main line, switch back to the main branch and merge:
git checkout main
git merge new-feature
This action brings your changes from the `new-feature` branch into the main branch.
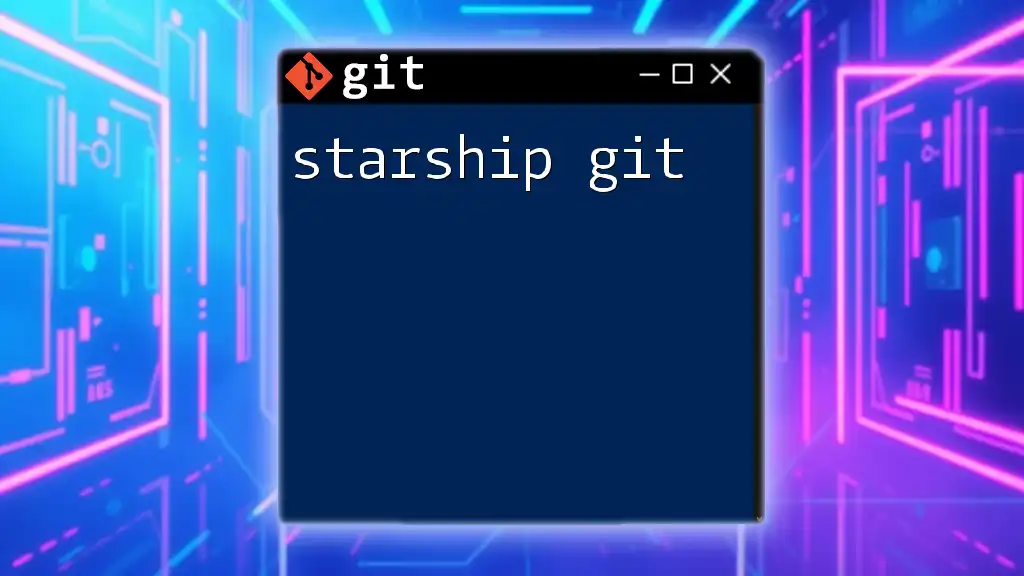
Collaborating on PHP Projects using Remote Repositories
Setting Up a Remote Repository
Using platforms like GitHub or GitLab allows your projects to be hosted online, facilitating collaboration with others.
Creating a New Repository
On GitHub, follow the prompts to create a new repository. Once created, you’ll receive the repository’s URL.
Pushing Local Changes to Remote Repository
Adding a Remote URL
Link your local repository to the created remote repository:
git remote add origin https://github.com/username/my-php-project.git
Pushing Changes
To push your local commits to the remote repository for the first time, use:
git push -u origin main
This command uploads your changes and establishes a link between your local `main` branch and the remote branch.
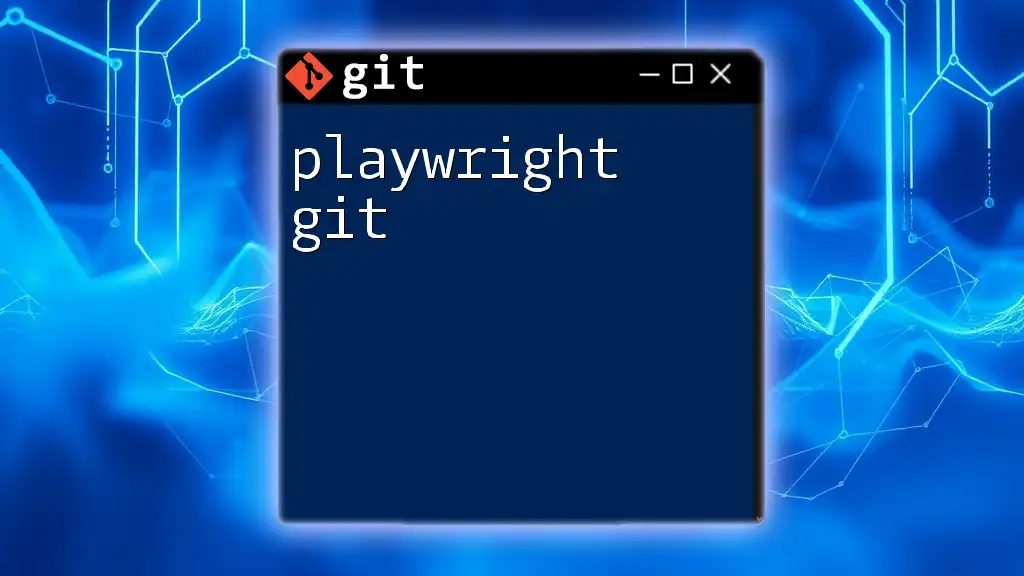
Best Practices for Using Git with PHP
Writing Effective Commit Messages
The effectiveness of commit messages cannot be understated. Use the imperative mood (e.g., "Add feature") and keep it concise yet descriptive. Consistent and informative commit messages help maintain clarity in project history.
Managing .gitignore Files
Understanding .gitignore
Every PHP project should have a `.gitignore` file to prevent unnecessary files from being tracked by Git. It helps keep your repository clean and free from sensitive data or temporary files.
Example .gitignore for PHP Projects
Here’s a typical structure for a `.gitignore` file in a PHP project:
/vendor
.env
.idea
*.log
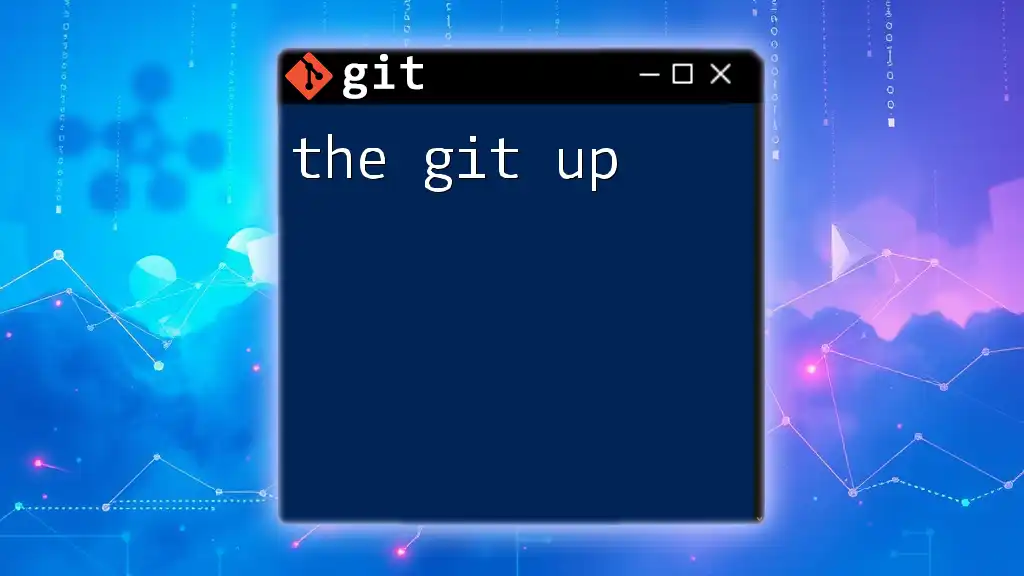
Conclusion
Mastering Git is invaluable for any PHP developer. It streamlines collaboration, ensures version control, and enhances project management. By practicing these commands and applying the knowledge of branching, merging, and working with remote repositories, you will significantly improve your development workflow.
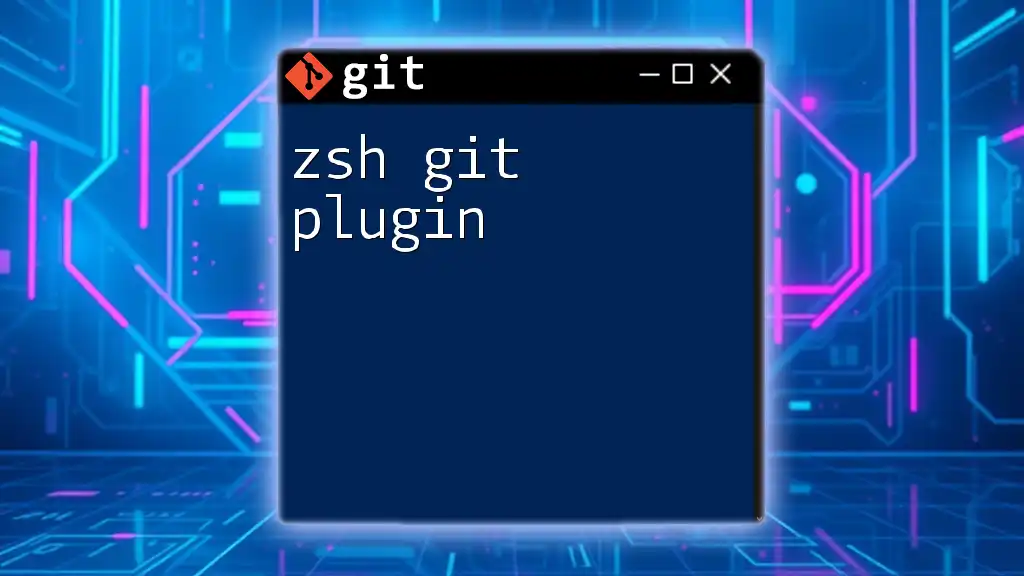
Additional Resources
For further learning, consider diving into these resources:
- Official Git documentation
- Tutorials and guides on PHP development frameworks
- Online courses that cover Git and version control systems
Joining communities and forums can also provide vital support as you enhance your skills in using Git with PHP.