The command `git stash pop` is used to reapply the changes stored in your most recent stash and then remove that stash entry from the stash list.
git stash pop
What is Git Stash?
In Git, a stash represents a temporary storage area where you can save uncommitted changes in your working directory. When you stash your changes, Git essentially takes a snapshot of your modifications and puts them aside so that you can return to a clean state. This functionality is invaluable for developers who need to switch contexts quickly without committing unfinished work.
Benefits of Using `git stash`
- Keeping Your Working Directory Clean: The stash allows you to save your local modifications without cluttering your history with incomplete commits. It helps maintain a clear and organized project history.
- Branch Switching: When working on a project, you might need to switch branches frequently. If you have uncommitted changes, you can stash them and easily switch to another branch, all while ensuring that your changes are not lost.
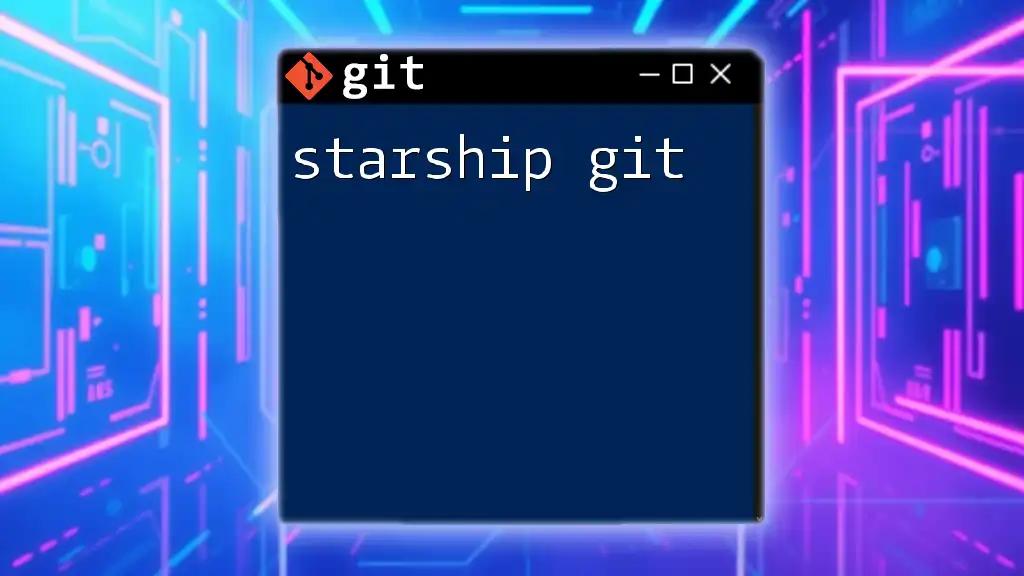
How to Use Git Stash
Before diving into `stash pop git`, it's essential to understand the basic commands associated with stashing changes in Git.
Basic Commands Related to Stashing
-
Creating a stash: When you are ready to stash your changes, simply run the following command:
git stash
This command saves your modified tracked files and the staging area, essentially storing your work safely.
-
Listing stashes: You can view all stashes created using:
git stash list
This command displays a list of your stashed changes, each identified by its index.
-
Viewing a stash: To inspect the changes in a specific stash, run:
git stash show stash@{0}
This command allows you to review the contents before deciding to apply them.
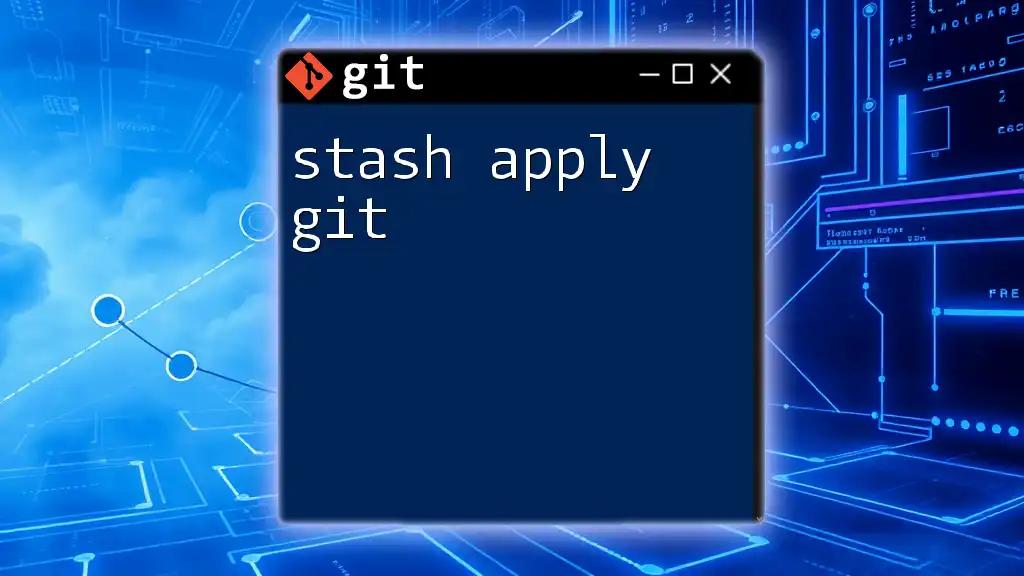
Understanding `git stash pop`
Definition of `git stash pop`
The command `git stash pop` is a powerful utility that restores the most recent stash and removes it from the stash list. This command is particularly useful when you want to reapply your stashed changes and continue working without leaving remnants in your stash.
Comparison with `git stash apply`
While both commands restore changes, the main difference is that `git stash apply` applies the changes but leaves the stash intact for potential later use. If you are confident that you no longer need the stash, `git stash pop` is the way to go.
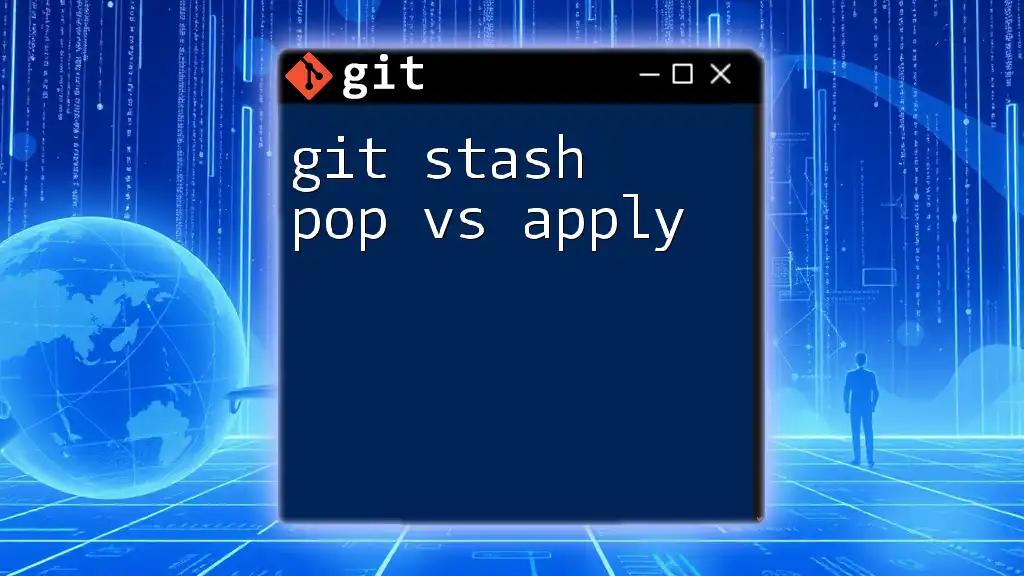
When to Use `git stash pop`
Common Scenarios
- Preparing for a Git Pull or Fetch: If you are about to pull updates from a remote repository and have uncommitted changes, it's best to stash them first using `git stash` before performing the pull operation.
- Multitasking: If you find yourself switching between multiple features or branches, stashing allows you to put your work on hold without losing anything.
- Rapid Bug Fixes: Sometimes, a critical bug may arise while you are in the middle of developing a new feature. Stashing your current ground allows you to switch to a hotfix branch and resolve the issue quickly.
Example Scenarios
Example 1: Stash Changes Before Pulling Updates
When you want to pull the latest changes from the remote repository but have local modifications:
git stash # Stash the current changes
git pull # Pull the updates from remote
git stash pop # Restore your stashed changes
Example 2: Switching from a Feature Branch to a Hotfix Branch
Suppose you are working on a new feature and need to address a bug in another branch:
git stash # Save your changes to the stash
git checkout hotfix-branch # Switch to the bug fix branch
# Fix the bug, then commit the fix
git checkout feature-branch # Return to the feature branch
git stash pop # Apply your previously stashed changes
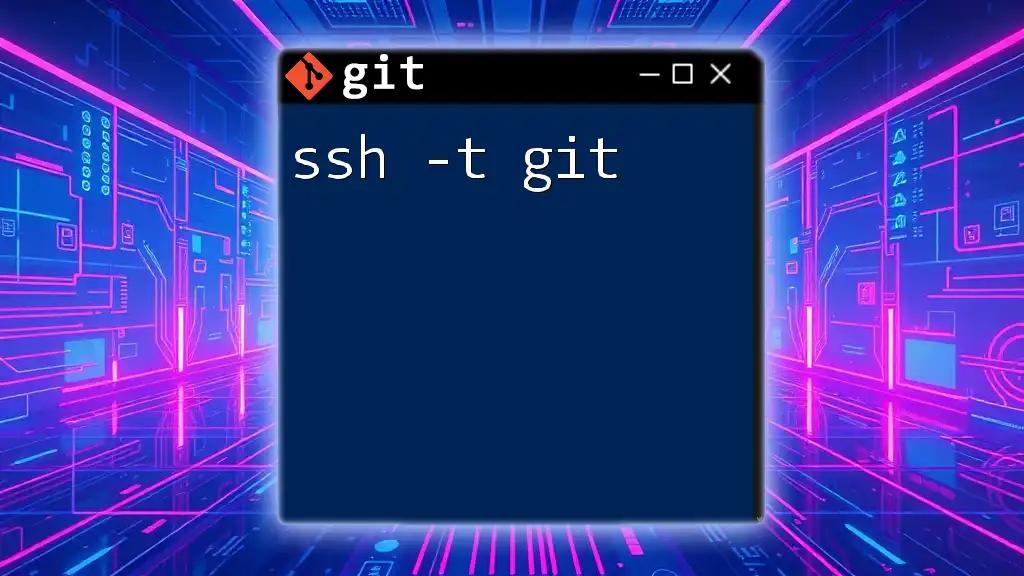
Executing `git stash pop`
Step-by-Step Guide
- Ensure your working directory is clean and ready to accept changes. Run `git status` to confirm.
- Execute the `git stash pop` command:
git stash pop
- If there are no conflicts, your changes will be reapplied to the working directory.
- Handling conflicts: If there are merge conflicts, Git will notify you. You will have to resolve these conflicts manually by following the prompts given by Git.
Code Snippet Example
To restore your latest stash:
git stash pop
Be attentive, as this action will clear the latest stash when completed successfully.
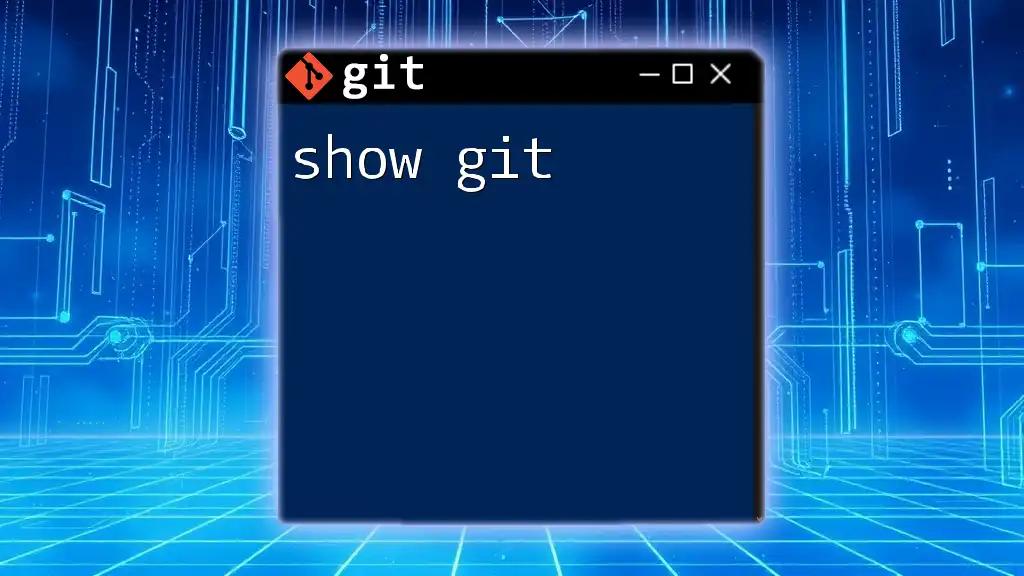
Handling Merge Conflicts
When you execute `git stash pop`, there may be instances where there are conflicting changes between your stashed modifications and the current state of the branch. In such cases, you should handle the conflicts as follows:
- Identify Issues: Use the `git status` command to identify which files are in conflict.
- Resolve Conflicts: Open each conflicted file and decide how to merge the changes. Git will mark the areas where conflicts exist.
- Standby Changes: After resolving the conflicts in the files:
git add <file_name> # Add the resolved files to the staging area git commit # Commit the resolution
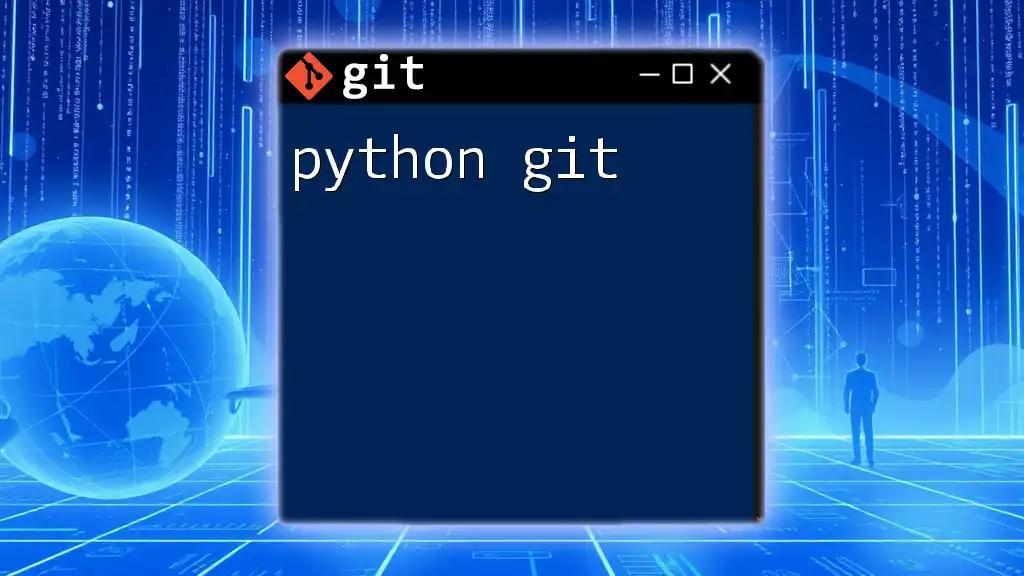
Advanced Usage of `git stash pop`
Options and Flags
-
Using a Specific Stash: If you want to pop a specific stash rather than the most recent one, identify it by specifying the index:
git stash pop stash@{1}
-
Combining with Other Git Commands: You can chain commands to streamline your workflow. For instance:
git stash pop && git push
This command sequence pops your changes and then pushes the latest commits to your remote repository.
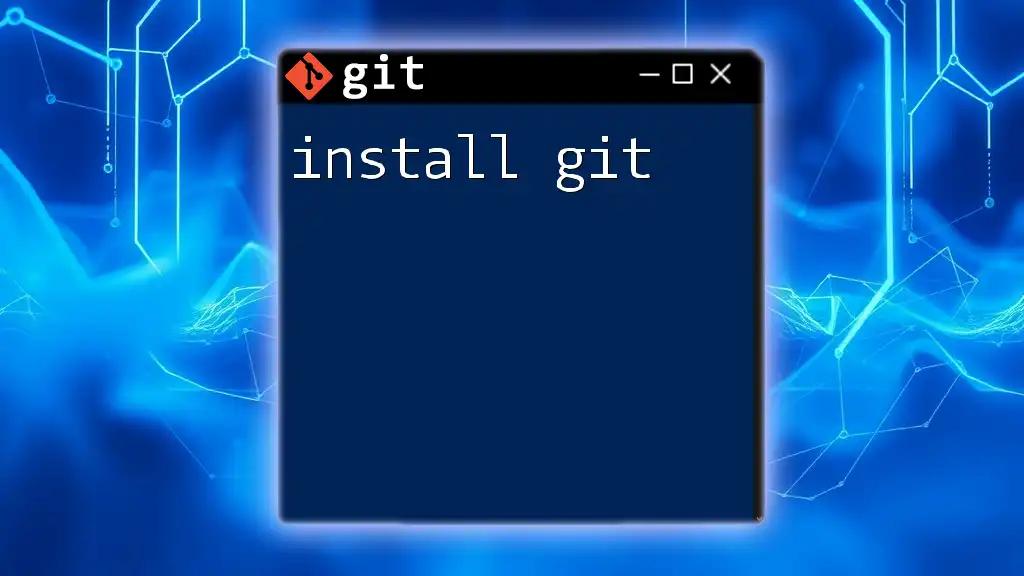
Conclusion
Understanding how to use `git stash pop` effectively enhances your development workflow by maintaining a clean working directory while allowing you to juggle multiple changes easily. It empowers you to be agile in your coding practices and minimizes the risk of losing your work.
To truly master the intricacies of Git stashing, practicing the commands and reflecting on different use cases in real projects will build your proficiency. As you continue your journey in version control, remember that the flexibility provided by Git is at your fingertips!
For further learning, explore the official Git documentation, engaging tutorials, and our recommended courses to deepen your knowledge of Git and improve your development skills.