The `ssh -t git` command is used to initiate an SSH connection with a Git remote repository, allowing you to execute Git commands securely over the network.
ssh -t git@github.com
Understanding SSH
What is SSH?
SSH, or Secure Shell, is a cryptographic network protocol that enables secure communication between two networked devices. It allows users to access remote computers securely, ensuring that any data sent over the network is encrypted, thereby protecting against eavesdropping and other attacks.
Why Use SSH with Git?
Using SSH with Git offers several advantages, especially when it comes to security and convenience:
- Security Benefits: SSH employs strong encryption methods, which means that your authentication credentials and the data you're transmitting are secure. This is critical when dealing with sensitive code or project files.
- Convenience of SSH Keys: Instead of entering your username and password for every push or pull operation, SSH allows you to set up SSH keys. This not only simplifies the authentication process but also enhances security, as keys can be configured to be more robust than traditional passwords.
SSH plays a crucial role in maintaining the integrity and confidentiality of your version control operations, especially in collaborative environments where multiple users access shared repositories.
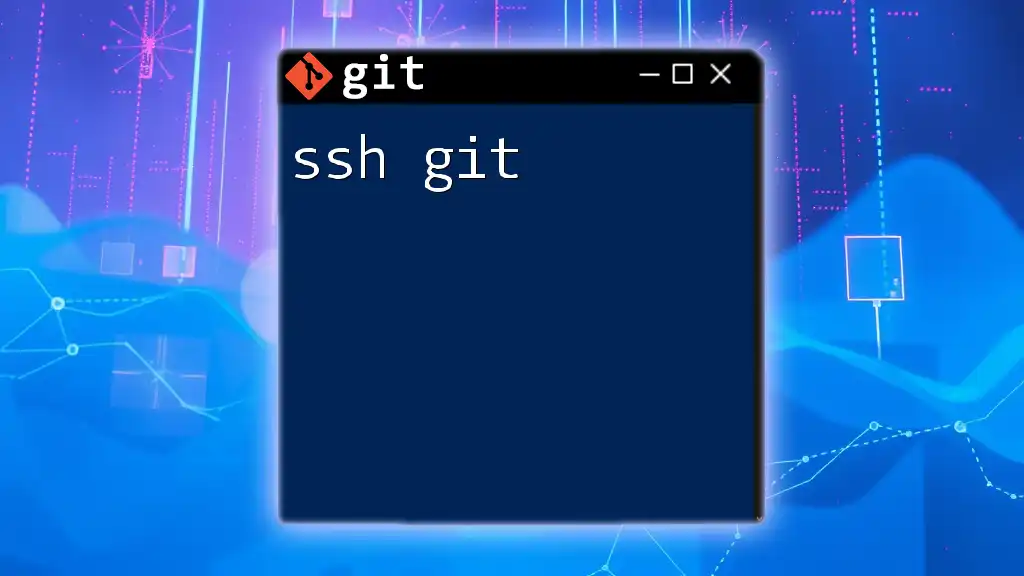
Introduction to Git Commands
Overview of Git
Git is a widely used version control system that helps developers track changes in their code over time. It allows for collaboration among multiple contributors and provides features like branching, merging, and commit history tracking.
Some key Git commands include:
- clone: Copies a repository from a remote server to your local machine.
- commit: Saves changes to the local repository with a message describing the update.
- push: Uploads local commits to a remote repository, making them available to others.
- pull: Fetches changes from a remote repository to your local machine.
Connecting Git with SSH
To use Git over SSH, you first need to set up SSH keys:
-
Generate SSH Keys: This is done on your local machine using the command:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This command creates a public and private key pair.
-
Add Your SSH Key to Your Git Account: You need to copy the contents of your public key (usually found in `~/.ssh/id_rsa.pub`) and add it to your Git hosting service account, such as GitHub or GitLab.
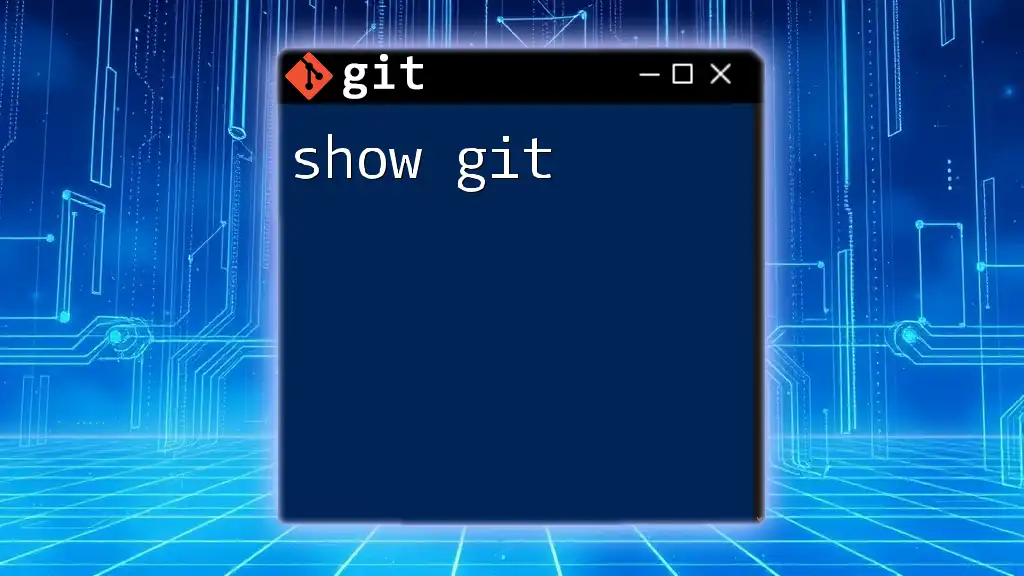
The `ssh -t` Flag Explained
What does `ssh -t` do?
The `-t` option in the SSH command is specifically used to allocate a pseudo-terminal. This is important when you need to execute commands that require a terminal environment, such as running interactive commands remotely. When using Git, certain operations may require this option to function correctly.
Common Use Cases for `ssh -t git`
Using `ssh -t` is particularly useful when you need to run interactive Git commands on a remote server. For example, if you're managing a repository that is hosted on a remote machine and you wish to initiate a Git operation that requires interaction, `ssh -t` simplifies that process.
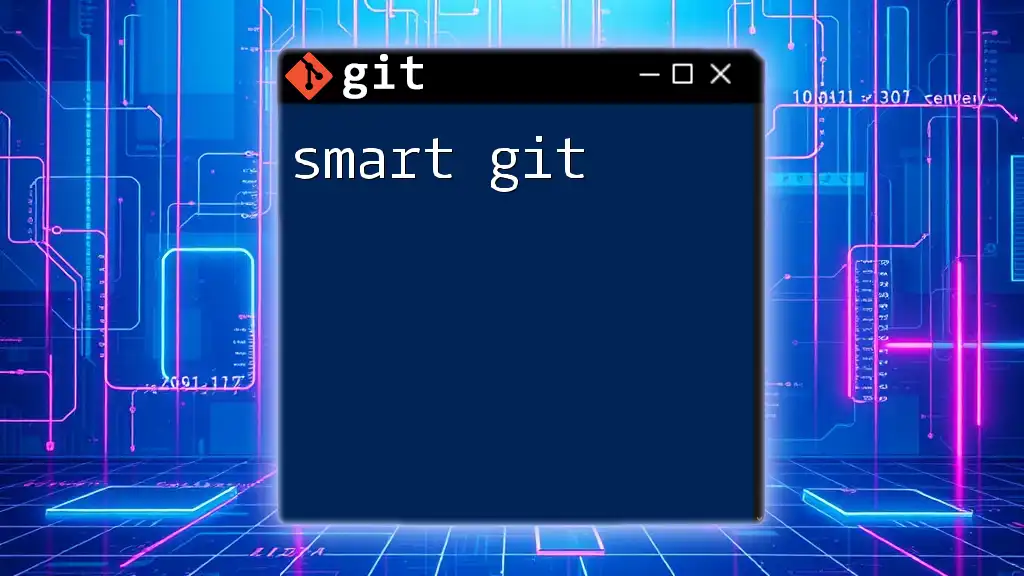
Implementing `ssh -t git`
Basic Syntax and Command
The general format for using `ssh -t` with Git commands is as follows:
ssh -t [user@]hostname git [command]
Replace `[user@]` with your SSH username and `hostname` with the remote server's address.
Examples of Using `ssh -t git`
Example 1: Running Git Commands Remotely
Suppose you want to clone a repository hosted on a remote server. You could use a command like:
ssh -t user@hostname git clone https://github.com/user/repo.git
This command would securely connect to the remote server and initiate the cloning process using SSH.
Example 2: Push Changes to a Remote Repository
Here’s a detailed demonstration of pushing changes to a remote repository:
-
After making changes locally, first stage them with:
git add .
-
Commit those changes by providing a meaningful message:
git commit -m "Making changes"
-
Now, push your changes to the remote repository using:
ssh -t user@hostname git push origin master
With this command, you're ensuring a secure connection while performing the push operation.
Combining `ssh -t` with Other SSH Options
You can enhance your SSH command by combining it with other options:
-
Use `-i` to specify an alternate private key:
ssh -i ~/.ssh/another_key -t user@hostname git pull
-
Utilize `-J` to set up a jump host, which is useful for advanced networking scenarios.
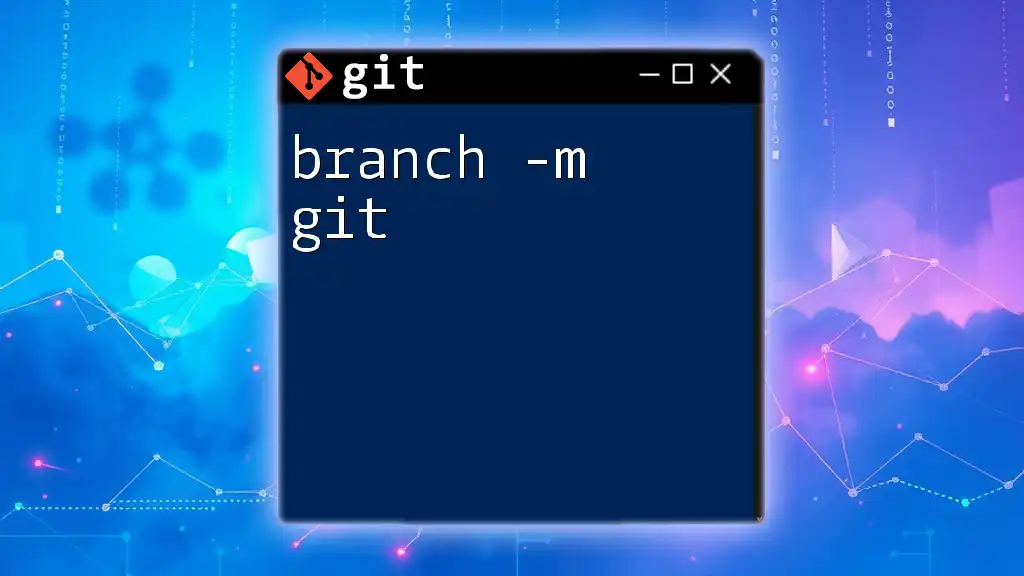
Troubleshooting Common Issues with `ssh -t git`
Connection Issues
When facing connectivity problems, consider the following:
-
Check SSH Logs: Often, connection issues can be traced through SSH logs which provide detailed information about the connection attempt.
-
Ensure SSH Agent is Running: If your SSH key is not being recognized, verify that the SSH agent is active and your keys are loaded.
Permission Denied Errors
Permission errors often arise from misconfigured keys or SSH settings. Here are common causes and solutions:
-
Key Permissions: Ensure your private SSH key has correct permissions. Use:
chmod 600 ~/.ssh/id_rsa
-
Mismatch between Key and Git Account: Confirm that the public key added to your Git account matches your local private key.
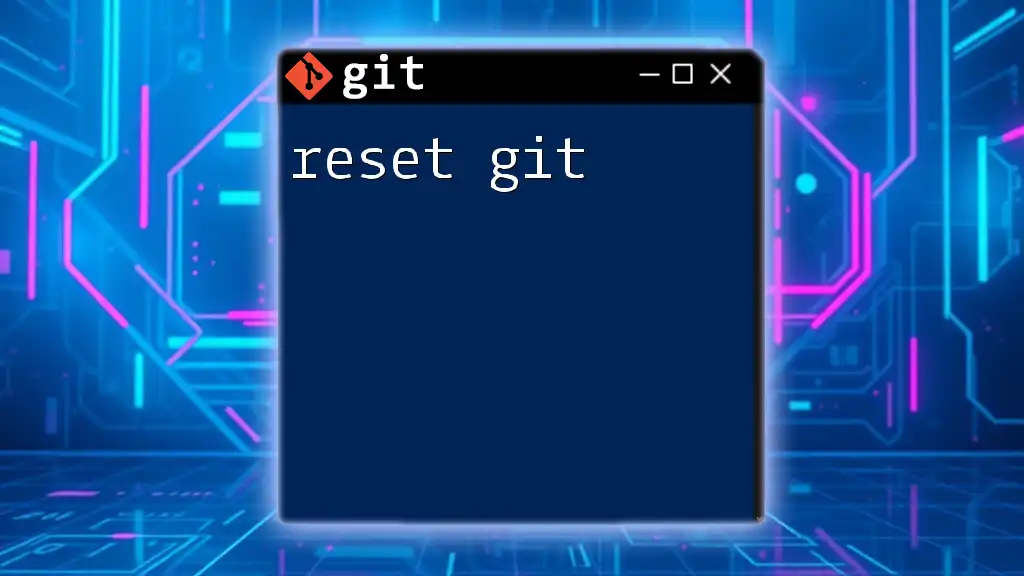
Best Practices for Using `ssh -t git`
Security Measures
To keep your SSH keys secure:
- Use Strong Passphrases: Always protect your private keys with a strong passphrase.
- Regularly Rotate Keys: Update your SSH keys periodically to mitigate risks from potential exposure.
Performance Improvements
For enhanced performance when using SSH with Git:
- Consider network optimizations, such as connecting via a wired network if possible to avoid latency.
- Use SSH multiplexing to share multiple sessions over a single connection, reducing connection overhead:
ssh -M -S /tmp/ssh-socket -f -N user@hostname
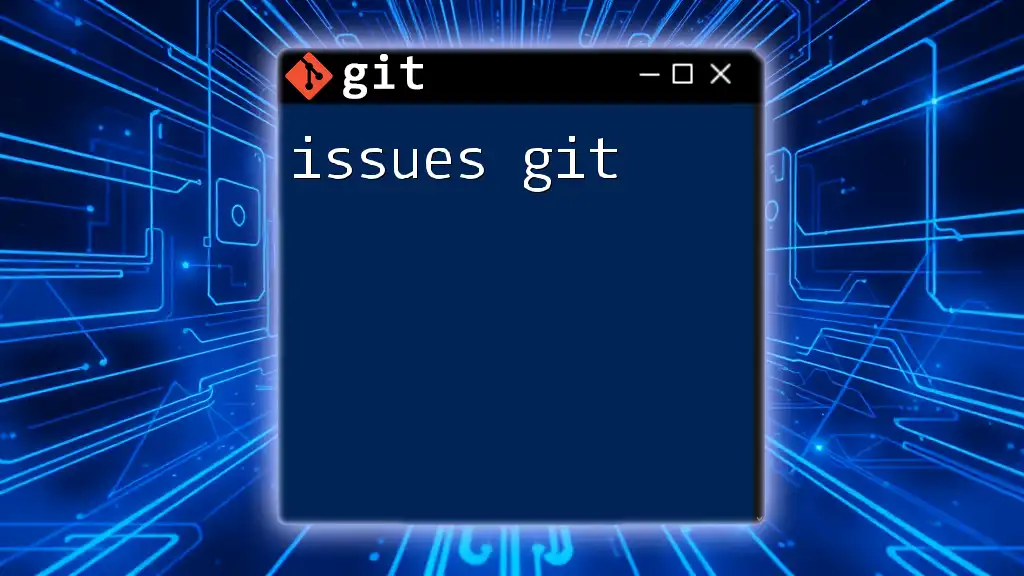
Conclusion
In summary, mastering `ssh -t git` is essential for securing and managing remote Git repositories efficiently. Understanding how to set up SSH, implement the `-t` flag, and troubleshoot common issues will significantly enhance your productivity when working with Git in collaborative environments. We encourage you to practice these commands and explore more advanced features as you continue your journey in Git.
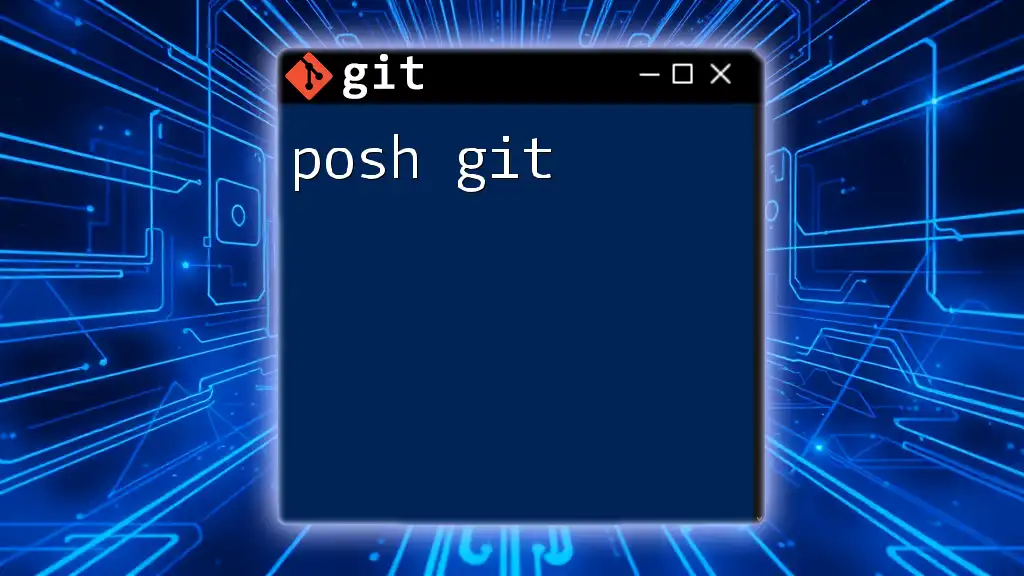
Additional Resources
For further learning, you can explore more thorough documentation on SSH and Git. Engaging with community forums can also provide valuable insights and assistance.
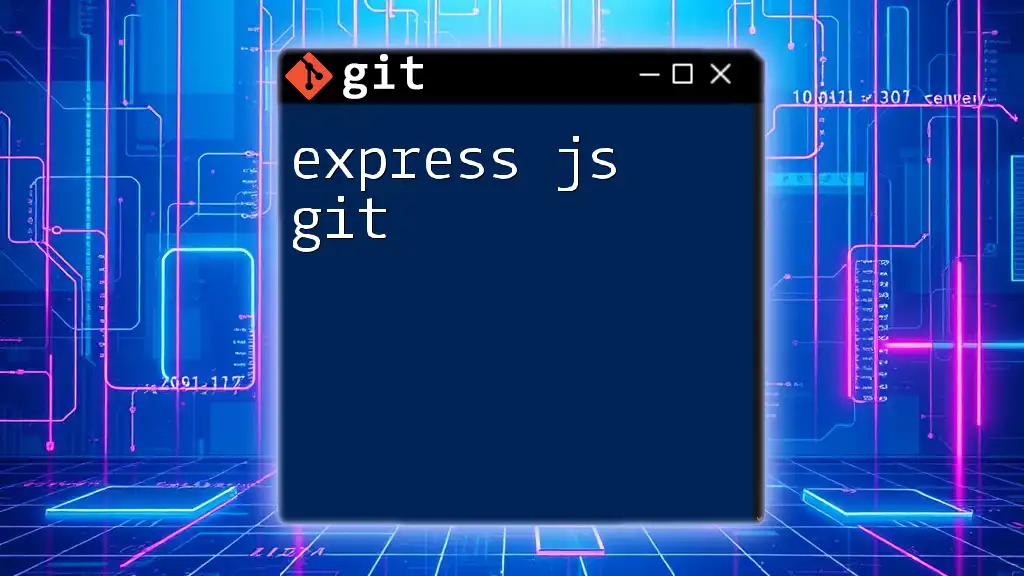
Call to Action
Continue diving into Git's fascinating array of features. Stay tuned for more in-depth content and courses designed to elevate your Git command skills!