Express.js is a minimal and flexible Node.js web application framework that simplifies the process of building web applications, and using Git with Express allows developers to efficiently manage changes and collaborate on their projects.
Here’s a simple Git command to initialize a new Express.js project:
git init
npm install express
Getting Started with Express.js
What is Express.js?
Express.js is a web application framework for Node.js designed for building web applications and APIs with ease. Its minimalist structure allows developers to create robust applications without having to write repetitive boilerplate code. Express is known for its speed and flexibility, making it a popular choice among developers for building RESTful APIs.
Setting Up Express.js
To get started with Express.js, you'll need to have Node.js installed on your machine. Once that’s confirmed, you can easily install Express using npm. Here’s how:
- Open your terminal.
- Navigate to your project directory.
- Run the following command:
npm install express --save
The `--save` flag indicates that Express.js will be added as a dependency in your `package.json` file.
Creating Your First Express App
To create a basic Express app, follow along with this straightforward example:
- Create a new file named `app.js`.
- Add the following code to set up a basic server:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
In this example, we are importing the Express module, then setting up a GET route that responds with "Hello, World!" when the root URL is accessed. The server listens on a port specified by the environment variable or defaults to 3000.
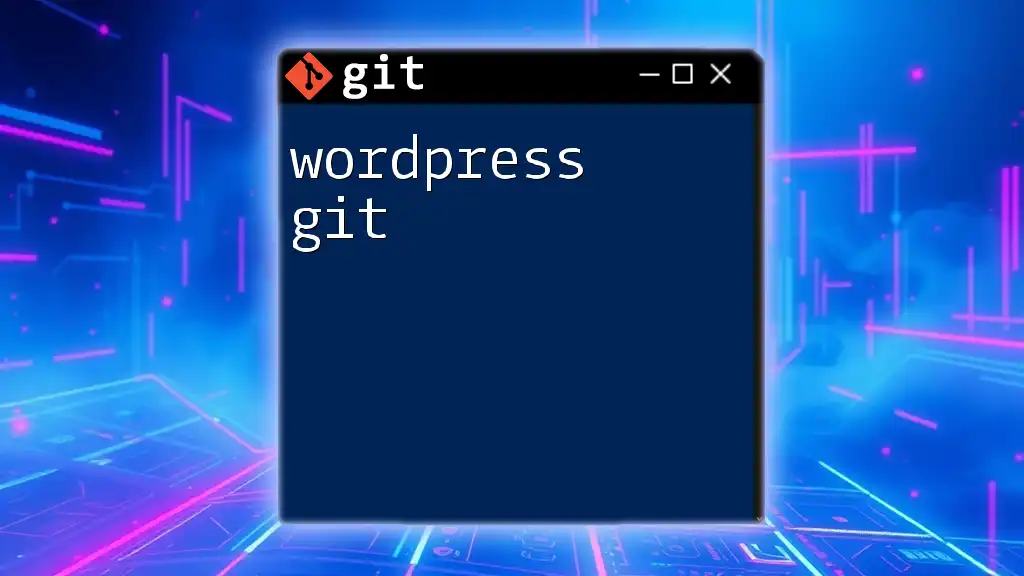
Introduction to Git
What is Git?
Git is a version control system that allows developers to track changes in their code, collaborate with others, and manage multiple versions of their projects. It simplifies the process of maintaining and modifying codebases, making it crucial for modern software development.
Setting Up Git
To start using Git, you’ll need to have it installed on your system. Here’s a quick guide for installation:
- Windows: Download the installer from [Git's official website](https://git-scm.com/download/win) and follow the installation wizard.
- macOS: Use Homebrew by running `brew install git` in the terminal.
- Linux: Install via the package manager, for example, using `sudo apt-get install git`.
Once Git is installed, it's essential to set up your configuration by running:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
This configuration allows Git to track who made changes to the code.
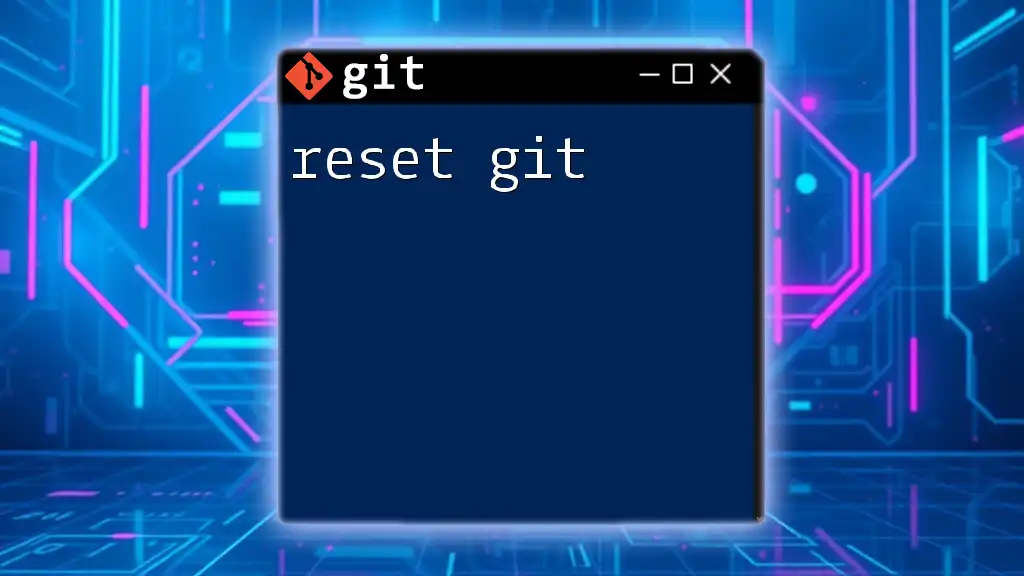
Integrating Git with Your Express.js Project
Initializing a Git Repository
Starting a new Git repository in your Express.js project is simple. Navigate to your project directory and run:
git init
This command creates a new `.git` directory, enabling Git to track changes in your project.
Creating Your First Commit
A commit is a snapshot of your code at a particular point in time. To make your first commit:
- Stage your changes with:
git add .
The `.` signifies that you want to stage all files.
- Then, commit your changes with a message:
git commit -m "Initial commit - Express.js setup"
Good commit messages help collaborators understand the history of changes.
Branching in Git
Branching allows you to develop features or fixes in isolation without affecting the main codebase. To create a new branch, use:
git checkout -b feature/new-feature
This command creates a new branch named `feature/new-feature` and switches to it.
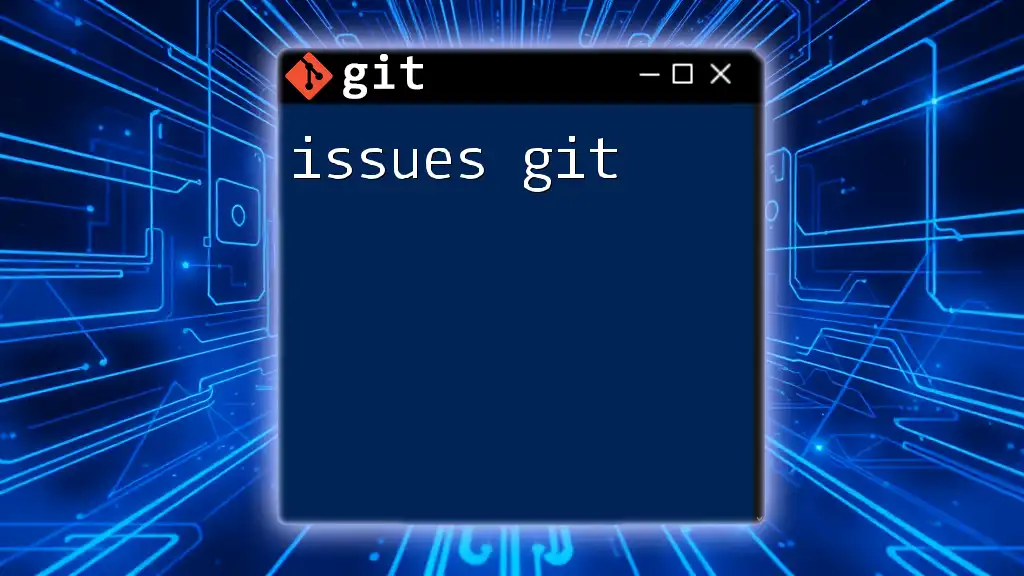
Collaborating with Git
Cloning a Repository
You may need to clone an existing Git repository, especially when collaborating with a team. To do this, you can run the following command:
git clone https://github.com/user/repo.git
Replace `https://github.com/user/repo.git` with the URL of the repository you want to clone.
Merging Branches
When you’re done working on a feature in your branch and want to integrate it back into the main codebase, you’ll need to merge it. First, switch back to the main branch:
git checkout main
Then, merge your feature branch:
git merge feature/new-feature
This command combines the changes you made in the feature branch into the main branch.
Handling Merge Conflicts
Sometimes, merging can lead to merge conflicts, which occur when changes in different branches contradict each other. To resolve a merge conflict:
-
Open the conflicting file. You’ll see both sets of changes clearly marked.
-
Edit the file to combine the changes as needed and save it.
-
After resolving conflicts, stage the changes:
git add filename.js
- Finally, commit the merge resolution:
git commit -m "Resolved merge conflict in filename.js"
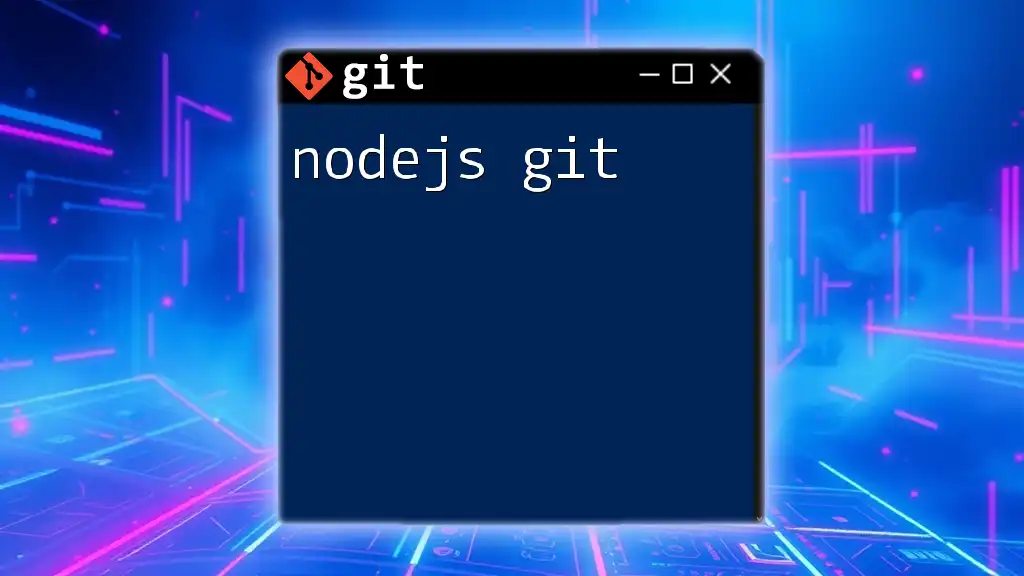
Best Practices for Using Git with Express.js
Commit Messages
Good commit messages are vital for maintaining clarity in your project. A helpful message typically starts with a brief description of what the commit does, followed by additional details in the body if necessary. Here’s a bad example:
Fixed things
And here’s a good example:
Fix user login issue by validating inputs before submission
Workflow Strategies
When working on a team, adopting a consistent workflow strategy is crucial. Some popular options include:
- Feature Branch Workflow: Each new feature is developed in its own branch, which is later merged into the main branch.
- Gitflow: A more structured workflow that separates feature development, releases, and hotfixes into different branches.
Choose a workflow based on your team size and the complexity of your project.
Documenting Your Project
Comprehensive documentation is essential in your Git repository. Utilize a `README.md` file to explain the purpose of your project, its setup, and how to contribute. Clear comments in your code can also make it easier for others (and your future self) to understand what each section does.
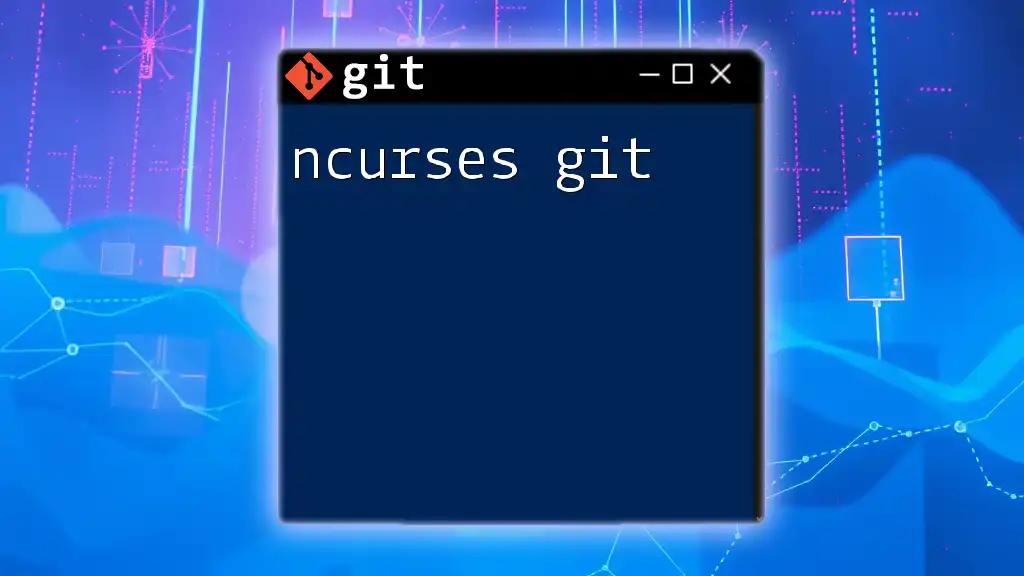
Additional Tools and Resources
Git GUI Clients
While command-line tools are powerful, many developers prefer using Git GUI clients for a more visual approach. Popular options include GitKraken, SourceTree, and GitHub Desktop, which can simplify managing repositories.
Learning Resources
To deepen your understanding of Express.js and Git, consider exploring online courses, tutorials, and documentation. Here are a few recommended resources:
- Express.js Documentation: The official documentation provides extensive guidance and examples.
- Pro Git Book: A free online book that covers everything you need to know about Git.
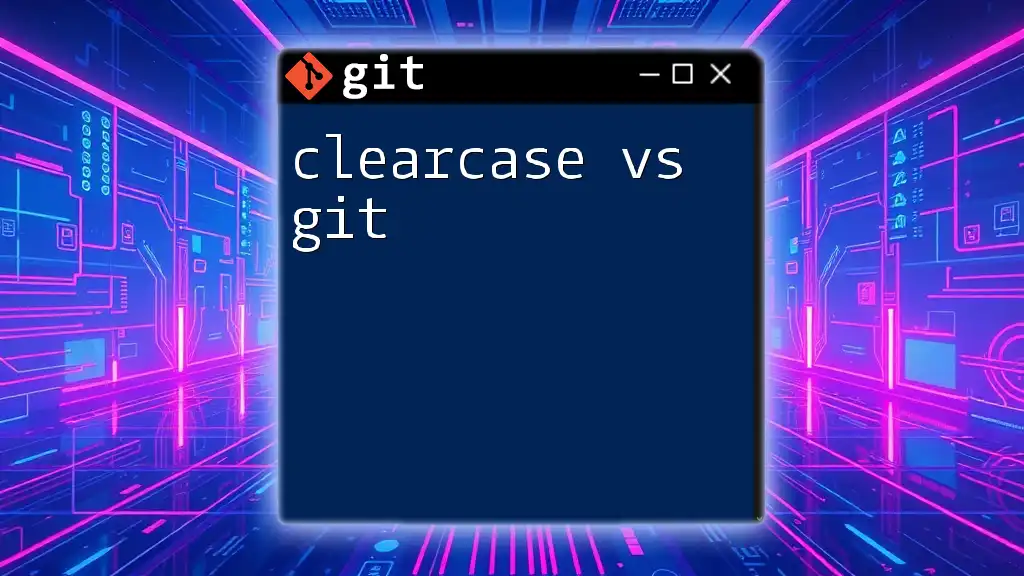
Conclusion
Using Express.js and Git together can enhance your web development workflow significantly. Understanding how to leverage Git for version control while developing your Express applications will not only improve your coding practices but also facilitate collaboration with fellow developers.
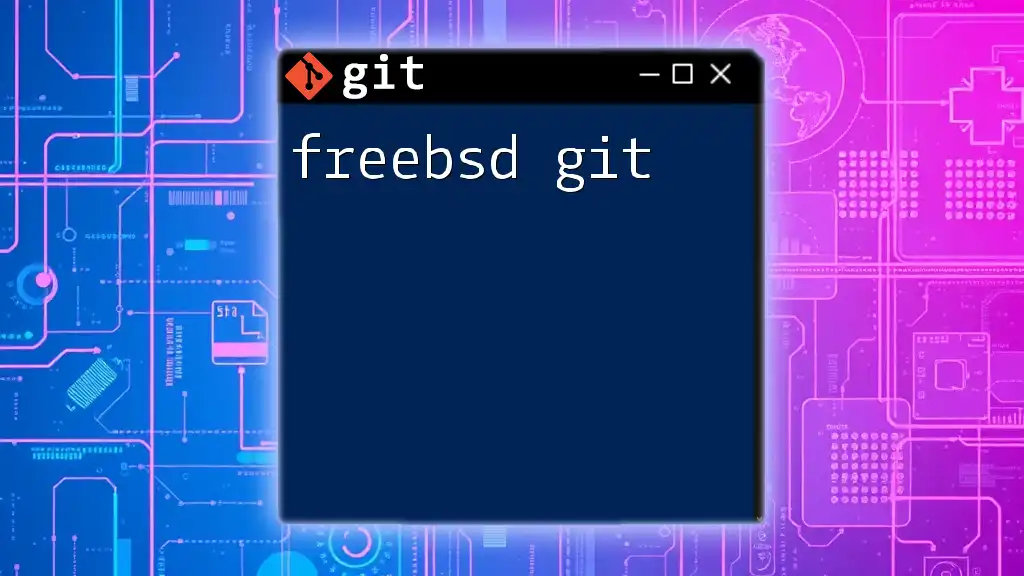
Call to Action
We encourage you to share your experiences with Express.js and Git in the comments below. If you found this article helpful, please share it on social media to help others improve their web development skills!