To delete a specific commit in Git, you can use the `git rebase` command followed by the commit hash you want to remove, which allows you to interactively edit your commit history.
git rebase -i <commit-hash>^
In the interactive editor, change 'pick' to 'drop' next to the commit you want to delete, save, and exit.
Understanding Git Commits
What is a Git Commit?
A Git commit serves as a snapshot of your project's repository at a particular time. Each commit captures the state of files and directories in your project, allowing you to track changes, revert to previous versions, or understand the evolution of your codebase. Understanding the structure of commits is crucial, as they represent your project's history.
Why Might You Want to Delete a Commit?
There are various scenarios where you may need to delete a specific commit. Common reasons include correcting mistakes made in commits, such as typos or incorrect code, eliminating commits that shouldn't have been pushed, and managing commit messages for cleaner history. Deleting a commit can significantly alter your commit history, making it vital to approach this task with caution.
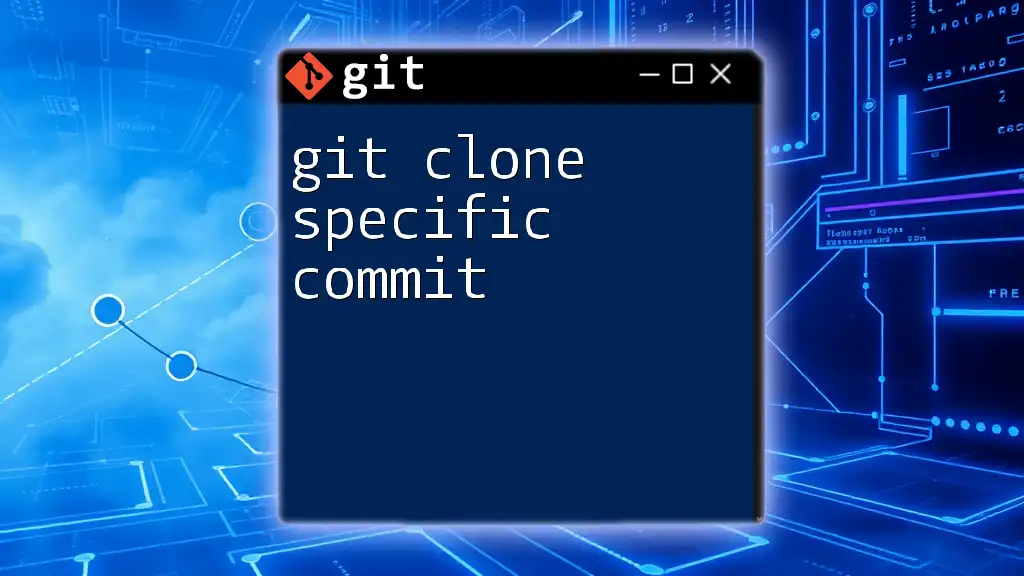
Viewing Your Commit History
Using `git log`
Before deleting any commit, it is essential to visualize your commit history. The `git log` command is instrumental in displaying a list of all commits in the current branch, including their hashes, messages, and timestamps. This data is crucial for identifying the specific commit you wish to delete.
To see a concise view of your history, use the command:
git log --oneline
This command outputs a simplified list of commits, each represented by its abbreviated hash and commit message. Analyzing this output will help you accurately target the commit for deletion.
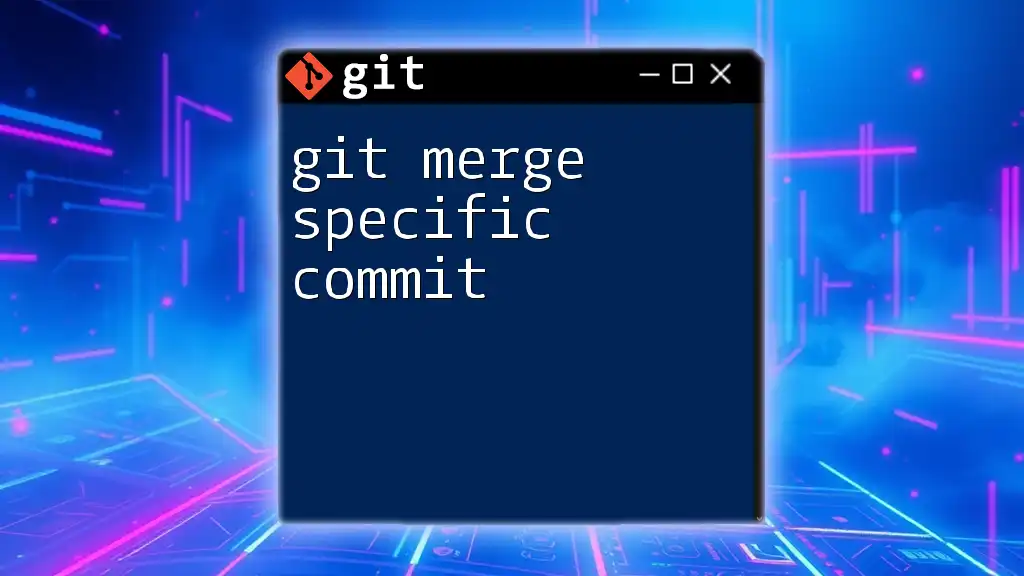
Choosing the Right Method to Delete a Commit
Different Approaches to Deleting Commits
When it comes to deleting specific commits in Git, there are two primary methods: reverting and resetting. The choice between these approaches hinges on whether you want to keep your commit history intact or rewrite it.
Reverting a Commit
What is a Revert?
Reverting a commit involves creating a new commit that negates the changes made by the target commit. This method is beneficial for maintaining commit history while effectively undoing the impacts of a previous commit.
When to Use Revert
Revert is ideal for situations where you want to keep your project's history transparent — particularly in shared branches, as it records your decision-making process, allowing others to see the changes made.
How to Revert a Specific Commit
To revert a commit, execute the following command, replacing `<commit_hash>` with the hash of the commit you wish to negate:
git revert <commit_hash>
After running this command, Git will create a new commit that reverses the specified commit’s effects. If there are conflicts, Git will explain what has to be resolved before you can complete the revert process.
Example: Reverting a Commit
For instance, if you want to revert a commit with the hash `abc1234`, the command will look like this:
git revert abc1234
Once executed, Git will apply the necessary changes and create a new commit with the revert message, effectively negating the original changes.
Resetting to Remove a Commit
What is a Reset?
The reset command in Git shifts the current branch pointer to a specified commit, effectively erasing commits that come after it. This method rewrites history, which makes it powerful but potentially dangerous in collaborative environments.
When to Use Reset
Resetting is suitable when you want to eliminate local changes that haven't been pushed to a remote repository yet. It allows for a clean slate when dealing with mistakes before sharing your work with others.
Using Soft, Mixed, and Hard Reset
There are three primary types of resets you can perform, each with different implications on your working directory:
-
Soft Reset
A soft reset keeps the changes in your working directory, un-staging them for potential modifications.git reset --soft <commit_hash>
-
Mixed Reset (default)
A mixed reset keeps the changes in your working directory but removes them from the staging area.git reset <commit_hash>
-
Hard Reset
A hard reset discards all changes following the specified commit, permanently deleting any changes made after that commit. This action cannot be undone.git reset --hard <commit_hash>
Example: Performing a Hard Reset
If you want to remove all changes and set your branch back to the state of commit `abc1234`, use:
git reset --hard abc1234
Caution: A hard reset is irreversible. It is crucial to ensure you don't have any changes you want to keep before using this command.
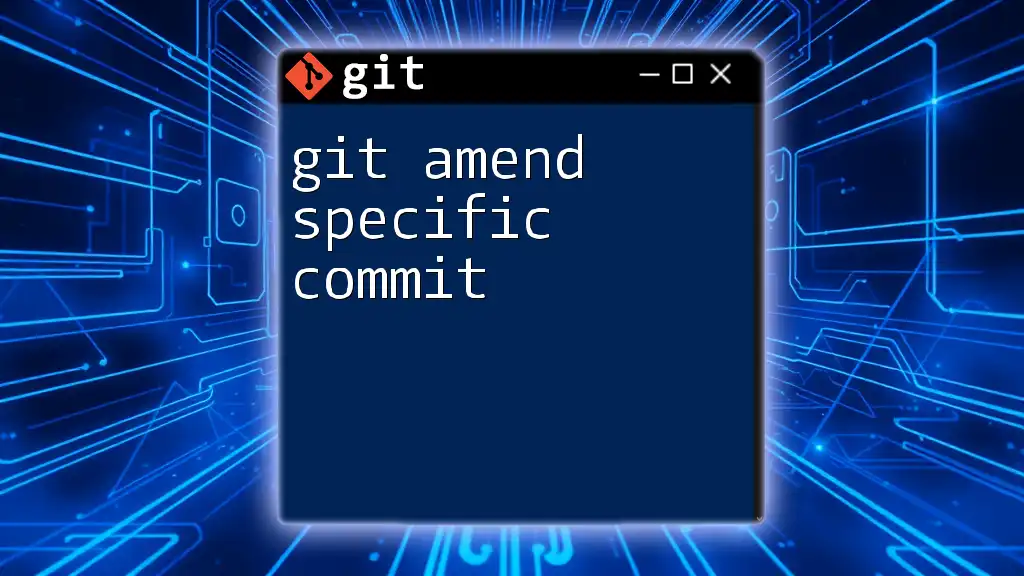
Best Practices for Deleting Commits
When Not to Delete Commits
Avoid deleting commits from shared branches, as this can confuse collaborators and break the project's history. Instead, consider using the revert approach, which maintains a clean project history.
Backing Up Before Making Changes
Always back up your repository before making significant changes, especially before resetting or deleting commits. You can achieve this by creating a new branch from your current state:
git checkout -b backup-branch
Using Branches for Experimentation
Utilize branches to test new ideas or make questionable changes. This strategy allows you to experiment freely without affecting the main codebase until you're confident with the changes.
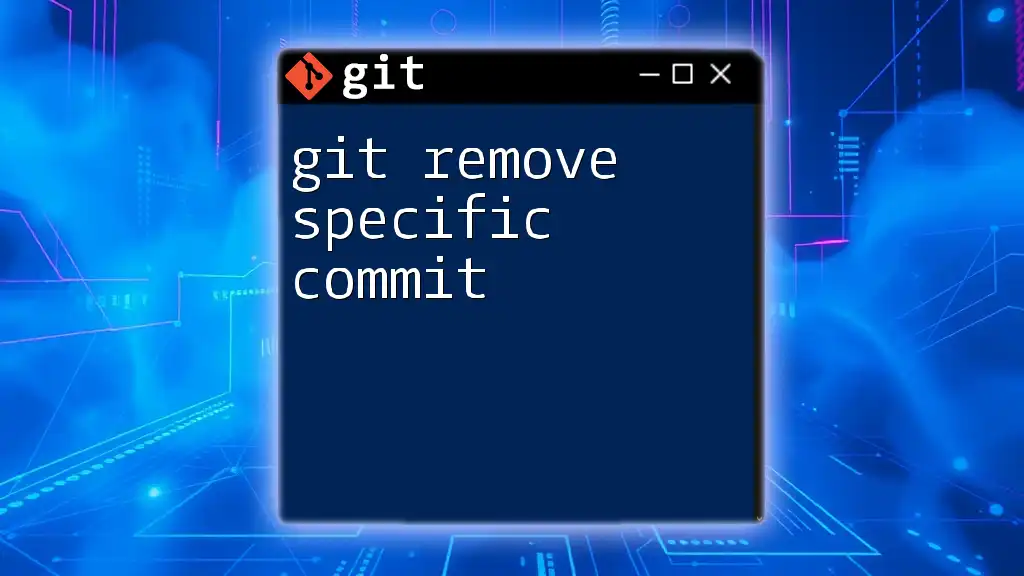
Conclusion
In summary, knowing how to effectively delete specific commits in Git is essential as it helps manage your commit history responsibly. Whether you choose to revert or reset depends on your requirements and the context of your project. Always practice caution, as the ramifications of deleting commits can be significant, especially in collaborative environments. Regularly using Git commands will enhance your proficiency and confidence in using this powerful version control system.
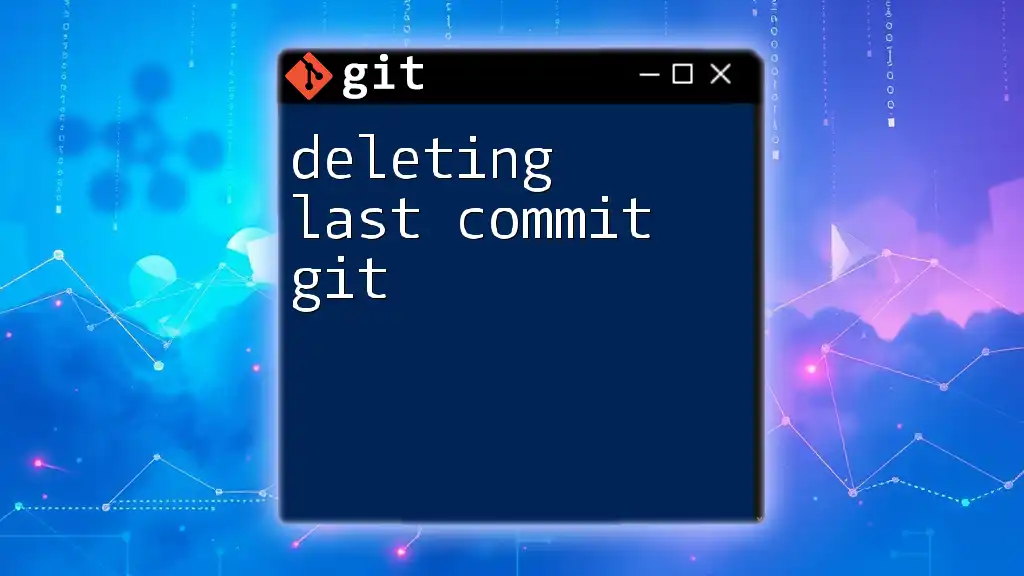
Additional Resources
To deepen your knowledge of Git commands, consider exploring the official Git documentation or online learning platforms dedicated to version control best practices.
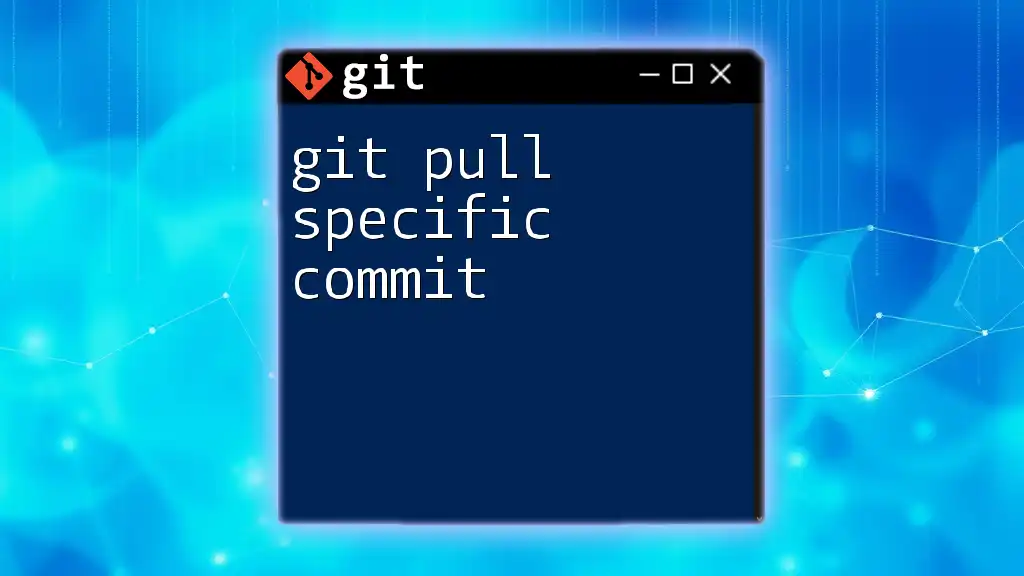
Common FAQs
Can I permanently delete a commit?
Yes, but caution is advised. Use the hard reset to remove a commit permanently.
What happens if I delete the commit that others depend on?
It can cause issues in collaboration, leading to broken histories and confusion among team members.
How can I recover a deleted commit?
If you haven't yet committed changes or pushed to a remote branch, you can often recover it using the reflog, which tracks all changes made in the repository. Use:
git reflog
to find the deleted commit's hash and check it out.