To view the changes made in your local branch compared to the last commit, you can use the `git diff` command followed by the name of the branch or leave it empty for the current branch.
git diff
Understanding Git Branches
What are Git Branches?
In Git, a branch serves as a diverging line of development, allowing you to work on changes without affecting the main project immediately. Branches facilitate parallel development, making it easier to manage features, fixes, or experiments.
Local vs. Remote Branches
- Local branches exist on your machine. They are where you make changes and commit them before pushing to a remote repository.
- Remote branches are hosted on a server (like GitHub, GitLab, or Bitbucket), serving as snapshots of your branches at a particular point in time.
Understanding the distinction between local and remote branches is crucial for effective collaboration and version control. Keeping your local branches in sync with remote branches ensures consistent project states among team members.

Checking the Status of Your Branch
Using `git status`
Before diving deeper, it's essential to check the current state of your local branch. The `git status` command provides vital information:
git status
When you run this command, you will see:
- The current branch you are on
- Changes that are staged for commit
- Changes that are not staged
- Untracked files
Being familiar with the output of `git status` allows you to understand precisely what modifications have been made and whether anything is ready for committing.
Importance of Keeping Track of Your Changes
Regularly checking the status of your branch helps maintain a neat and organized development workflow. By staying abreast of your changes, you can avoid conflicts and ensure smooth synchronizations with remote branches.
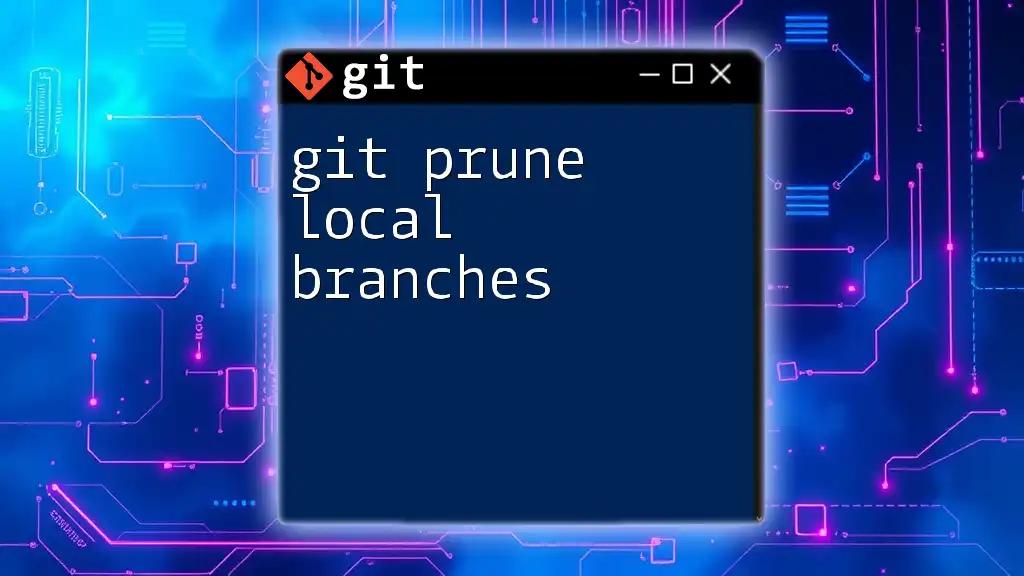
Viewing Changes in Local Branch
Using `git diff`
To see the actual differences between your current working directory and the last committed state, you can use the `git diff` command. This command is incredibly handy to identify what has changed:
To see unstaged changes, use:
git diff
If you want to view staged changes (those ready to be committed), you can run:
git diff --cached
Understanding how to use `git diff` is crucial because it highlights specific changes made to files. This gives you a clearer image of what you’re about to commit.
Exploring Differences Between Branches
You may also want to compare your current local branch with the remote branch to see what commits or changes you have made. To achieve this, use:
git diff origin/main..HEAD
In this command, `origin/main` represents the main branch on your remote repository, while `HEAD` points to your current branch. This way, you can quickly assess what changes exist in your local branch compared to what is remotely available.
Viewing Changes to a Specific File
If you wish to check changes made to a particular file, you can specify the file path directly in the command:
git diff <file_path>
This allows you to focus on a single file, which can be especially helpful when working with large codebases.
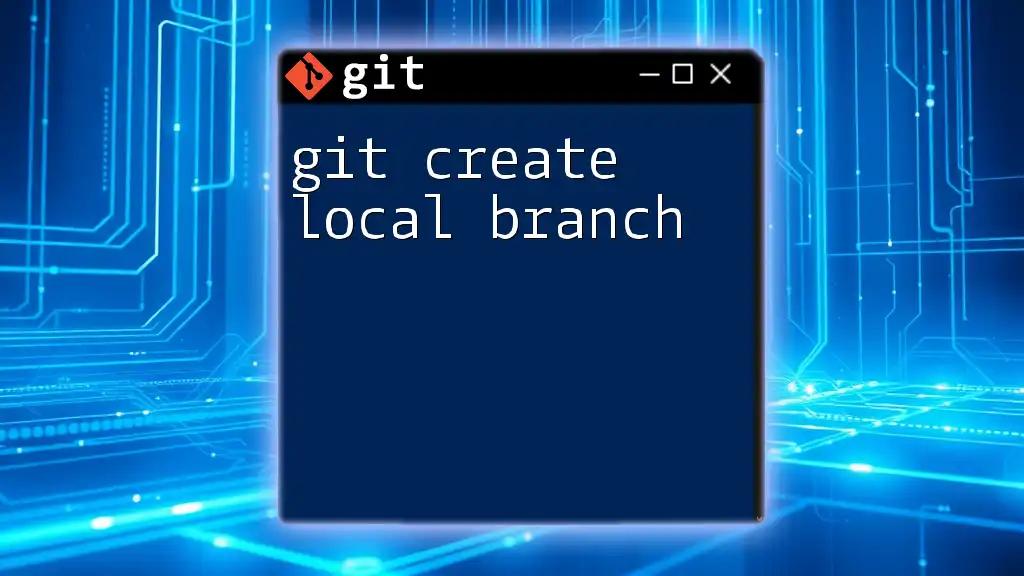
Getting a Summary of Changes
Using `git log`
To display the history of changes made in your local branch, the `git log` command is indispensable. When executed, it shows a list of recent commits:
git log
The `git log` output includes:
- Commit hash
- Author name
- Date of the commit
- Commit message
Understanding this information is vital for tracing back through your project's history and understanding how it evolved over time.
Utilizing `git log --stat`
For a more generalized summary of changes, you can use the `--stat` option:
git log --stat
This command displays each commit along with a summary of the number of insertions and deletions made in each file, providing a quick overview of what has changed without diving into the details of every commit.
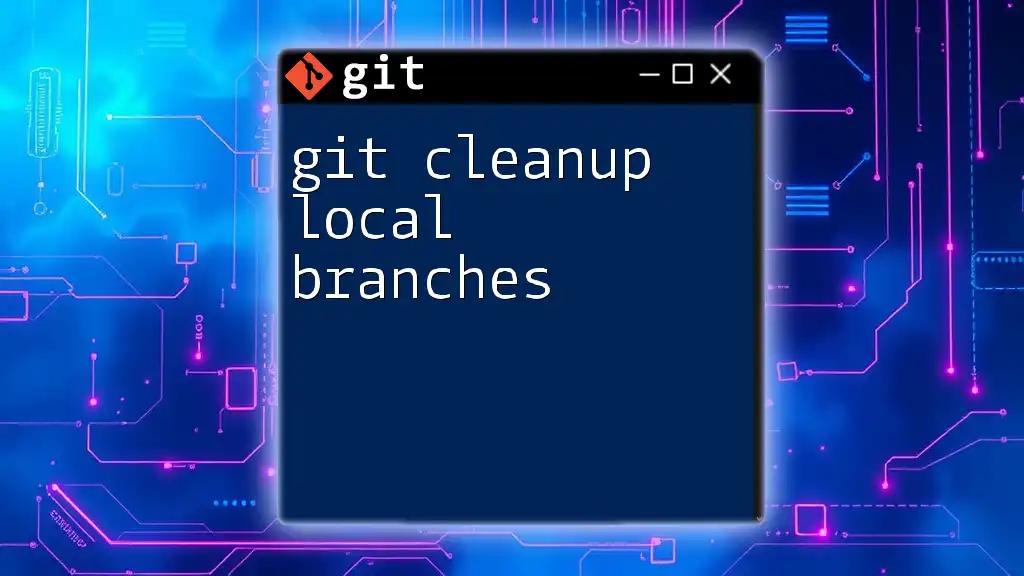
Displaying Changes in a More Visual Format
Using `git diff` with color
Enhancing the readability of output can aid in quicker comprehension. You can instruct Git to display diffs with color:
git diff --color
Color-coding the output helps you quickly identify additions (usually in green) and deletions (often in red).
Graphical Tools for Visualizing Changes
Beyond command-line tools, many graphical user interface (GUI) applications can visually represent branches and changes. Tools like Sourcetree or GitHub Desktop provide an intuitive interface where you can see the differences between branches, visualize commit history, and manage your local and remote branches more efficiently.
Using these tools can streamline your workflow and enhance your understanding of how changes correlate between different branches.
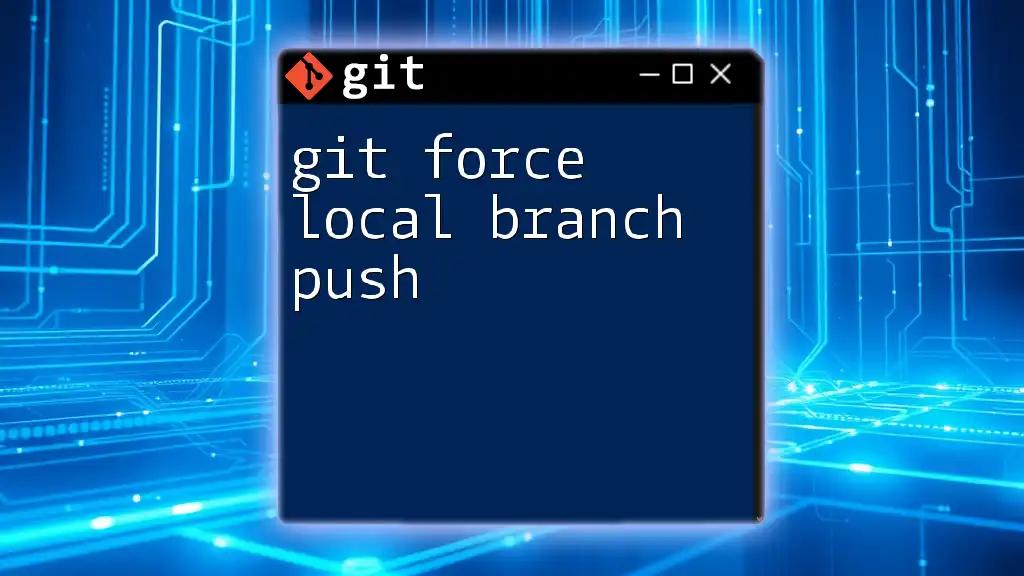
Conclusion
Mastering how to git show changes in local branch is critical for effective version control and collaboration. By utilizing commands like `git status`, `git diff`, and `git log`, you gain valuable insights into your project, enabling you to make informed decisions about your code changes.
Regular practice with these commands will empower you to navigate Git with confidence. Consider diving deeper into additional Git commands and workflows to enhance your skill set further. You’re now equipped with the essential tools to manage your local branch changes seamlessly!
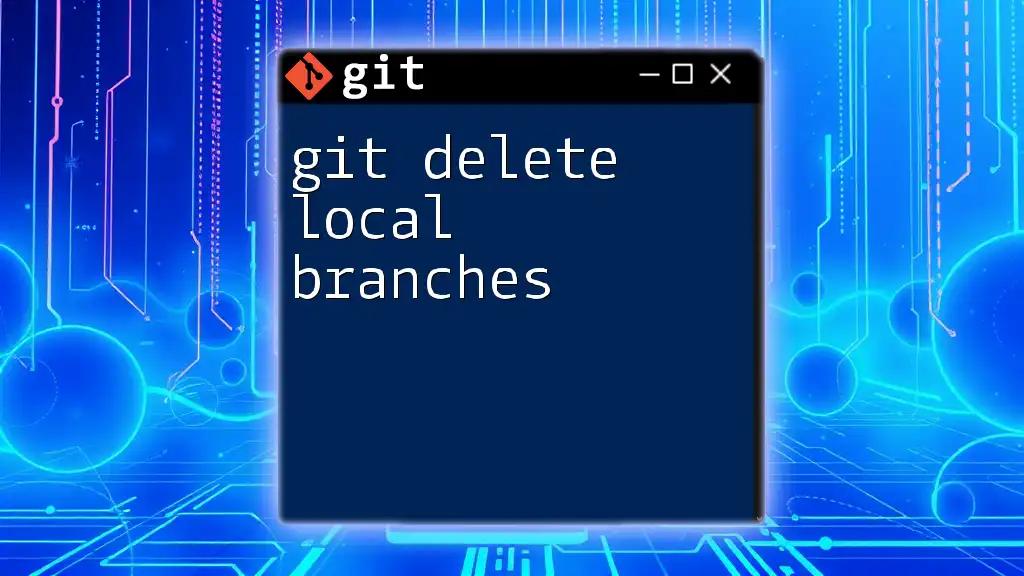
Additional Resources
For more extensive learning, check out the official Git documentation and various online tutorials. Engaging with community forums can also provide additional insights and tips to refine your Git proficiency as you continue your journey in mastering version control.