To stage modified files in Git for the next commit, you can use the `git add` command followed by the `.` (dot) to add all changes in the current directory, or specify individual files.
git add .
# or for a specific file
git add <filename>
Understanding Git's Staging Area
The staging area in Git, often referred to as the index, is a critical component of the version control process. It serves as a middle ground where changes can be prepared before committing them to the repository. Understanding how the staging area works is essential for effective version control.
When you make modifications to files in your working directory, they are untracked until you explicitly stage them using `git add`. The staging area allows you to review and select which changes you want to include in your next commit.
Difference between working directory, staging area, and repository:
- Working Directory: This is where you make changes to your files. Any modification is not yet tracked by Git until it is staged.
- Staging Area: The intermediate space for your changes. Here, files are prepared before being committed to the repository.
- Repository: The final storage of your project's history and versions. Only staged changes can be committed and pushed here.
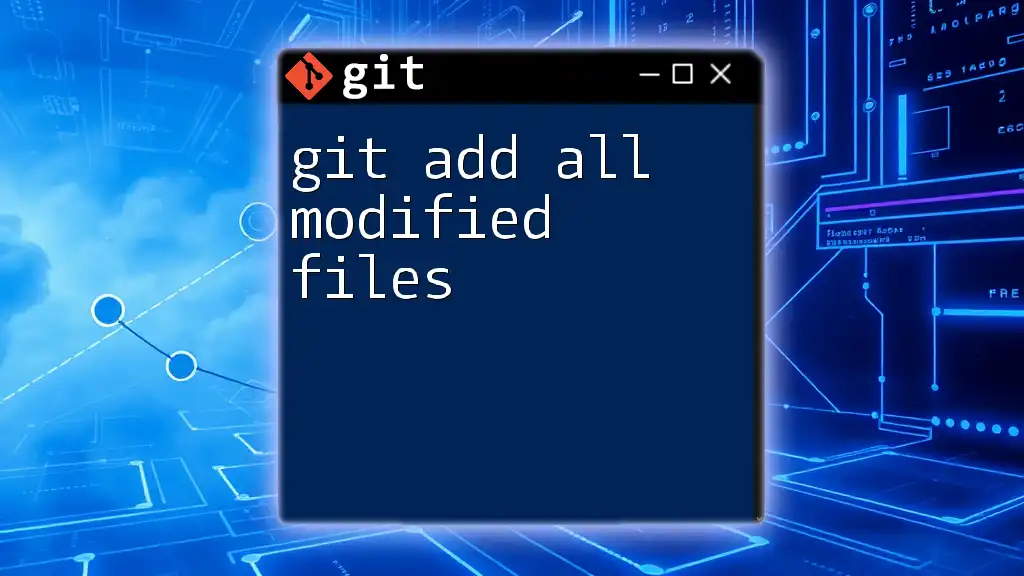
The Syntax of Git Add
The basic command structure for adding modified files is as follows:
git add [options] [file...]
In this syntax:
- options: These can modify how `git add` behaves.
- file: This can be the name of a single file, multiple files, or even directories.
Understanding these options helps tailor the staging process to your needs.
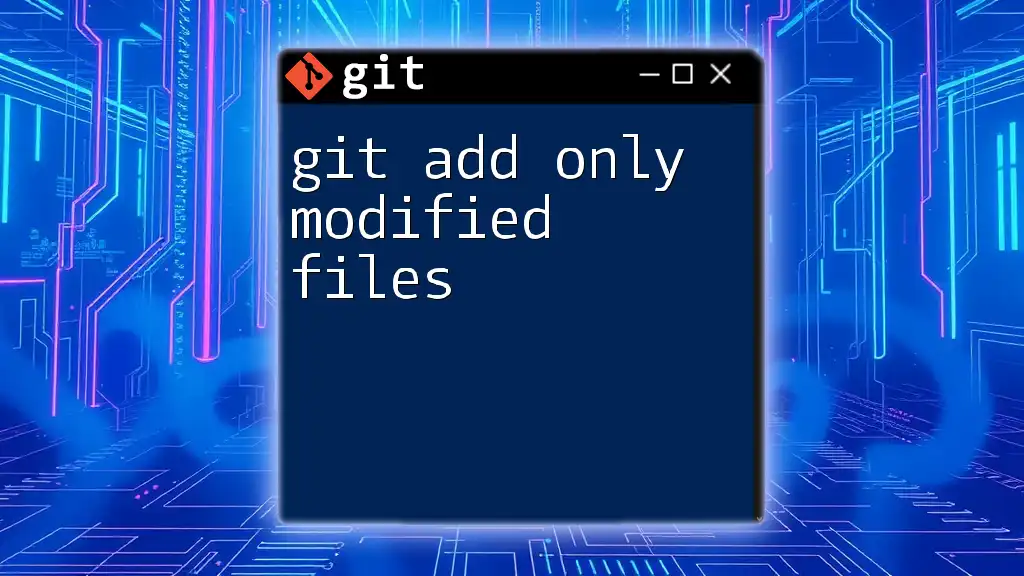
Identifying Modified Files
Before staging, it’s crucial to check which files have been modified. This can be accomplished with the `git status` command. It provides a clear overview of the current directory's state in relation to the repository.
git status
The output will differentiate between modified files, untracked files, and staged changes. This allows you to confirm what has changed and decide what to stage.
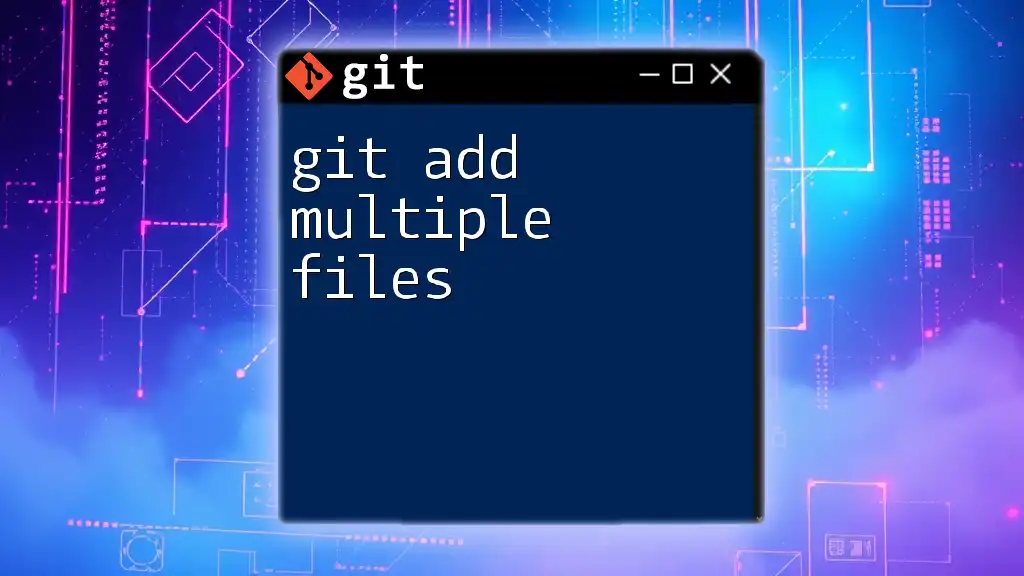
Staging Modified Files with Git Add
Staging modified files is straightforward. If you want to stage a single modified file, you simply specify its name:
git add <file_name>
For example, if you have a modified file named `example.py`, you can stage it by running:
git add example.py
If you want to stage all the modified files in the current directory and its subdirectories, you can use:
git add .
This command is powerful because it stages every change you have made in your working directory.
Alternatively, if you want to stage all changes, including modifications and deletions, use the `-A` option:
git add -A
Detailed Explanation
- `git add <file_name>`: This command stages the specified file, marking it as ready for commit.
- `git add .`: This approach stages all modified files in the current directory and any subdirectories, which is convenient when you have multiple changes.
- `git add -A`: This stages all changes in the working directory, including file deletions, ensuring your commit encapsulates all your work.
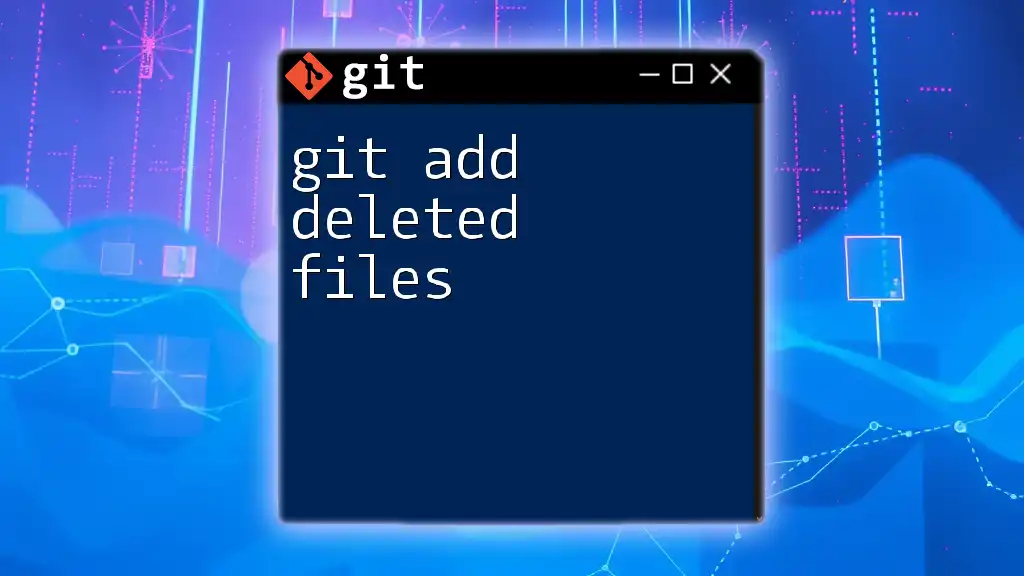
Staging Partial Changes
Sometimes, you may want to stage only parts of a modified file rather than the entire file. This can be achieved using the `-p` option, which opens an interactive prompt.
git add -p <file_name>
Using this command initiates an interactive staging process where you can selectively stage changes.
Example of Interactive Mode
Once you run the command, you are presented with changes and options to stage them. The options include:
- `y`: Stage this hunk (change).
- `n`: Don't stage this hunk.
- `s`: Split this hunk into smaller ones for more granular staging.
- `q`: Quit the interactive mode.
This feature is particularly useful when you have mixed changes in a file and want to organize commits better.
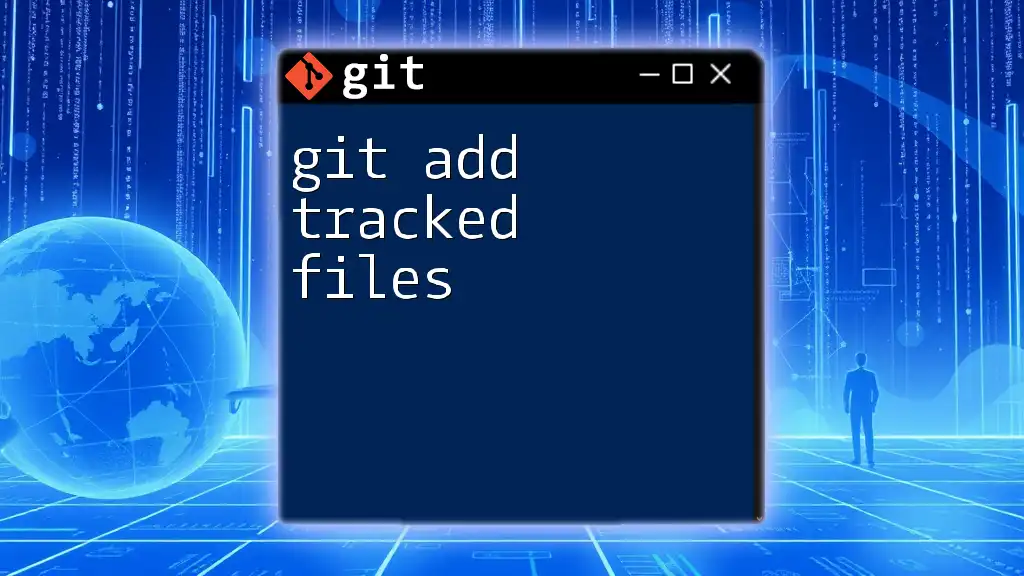
Verifying Staged Changes
After staging files, it's vital to verify what has been added. You can do this with:
git diff --cached
This command shows the changes that are in the staging area compared to the last commit. It’s a helpful step to ensure you are committing exactly what you intend.
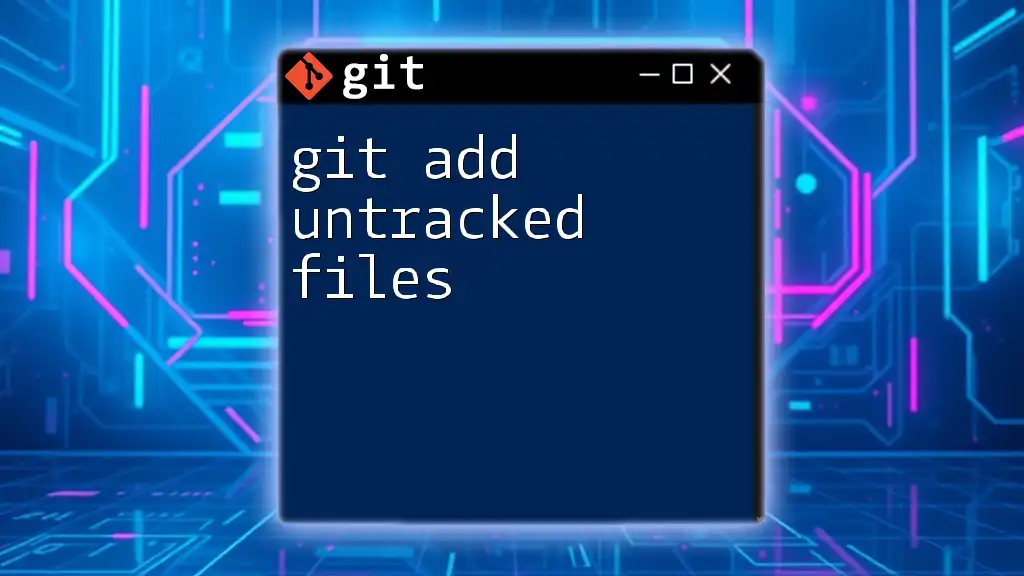
Common Mistakes and Troubleshooting
While working with `git add modified files`, there are some common pitfalls:
- Forgetting to stage modified files: Accidentally skipping staging could lead to uncommitted changes.
- Staging unwanted files: Ensuring you accurately stage only the changes you want to commit is crucial.
- Resolving conflicts due to improper staging: If you stage conflicting changes, it can lead to complications that must be resolved before proceeding.
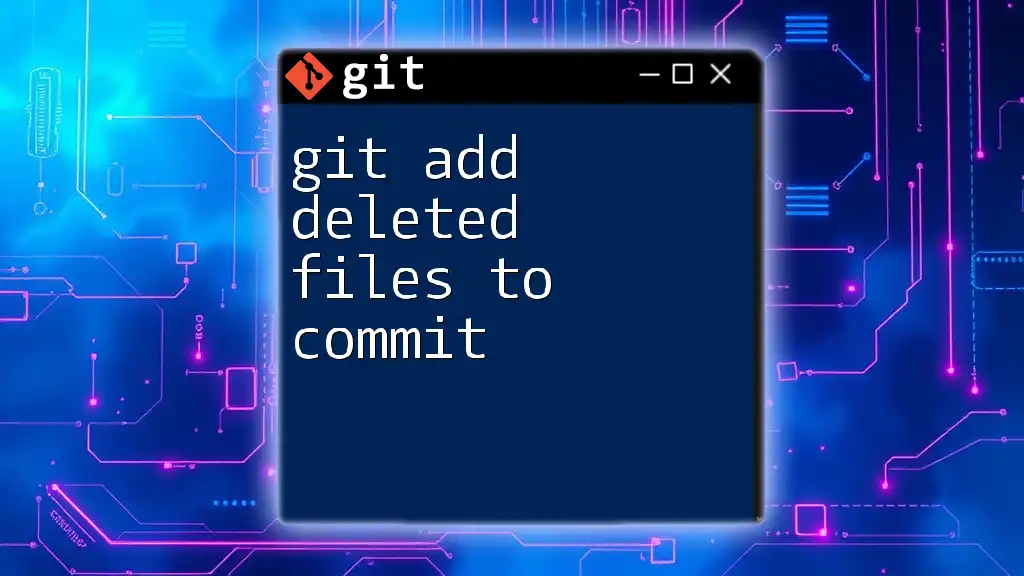
Best Practices for Using Git Add
To maximize the efficiency of using `git add`, consider the following best practices:
- Regularly check modified files with `git status`: This keeps you informed about your staging situation.
- Use clear commit messages after staging: Well-defined commit messages help track the project history effectively.
- Keep your commits focused and atomic: Each commit should ideally represent a single logical change, facilitating easier debugging and collaboration.
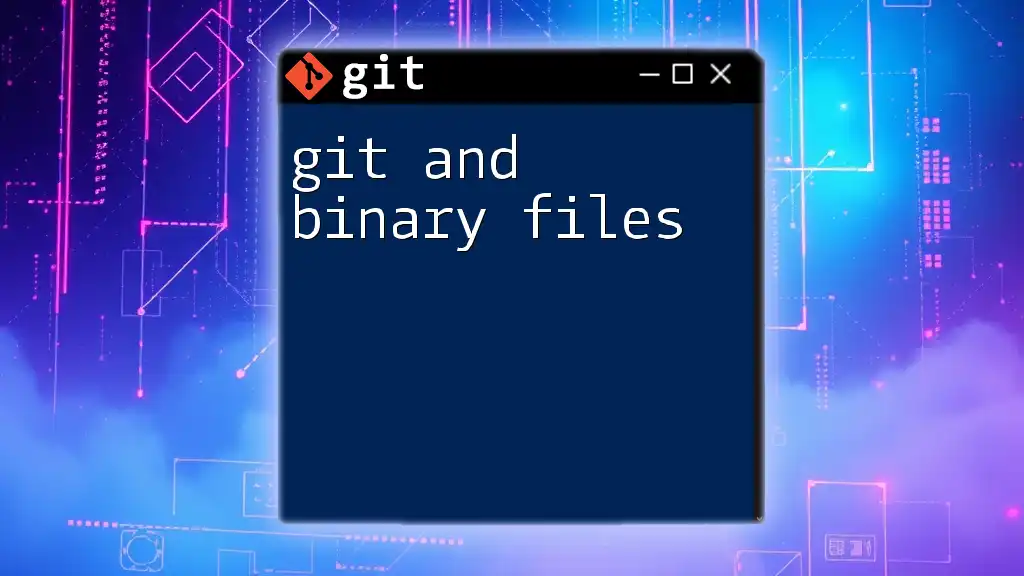
Conclusion
Understanding the intricacies of git add modified files is essential for managing your projects effectively. By grasping the staging process, you can ensure that your commits reflect accurate and meaningful changes. Practice using these commands and tips to enhance your proficiency in Git and improve your workflow.
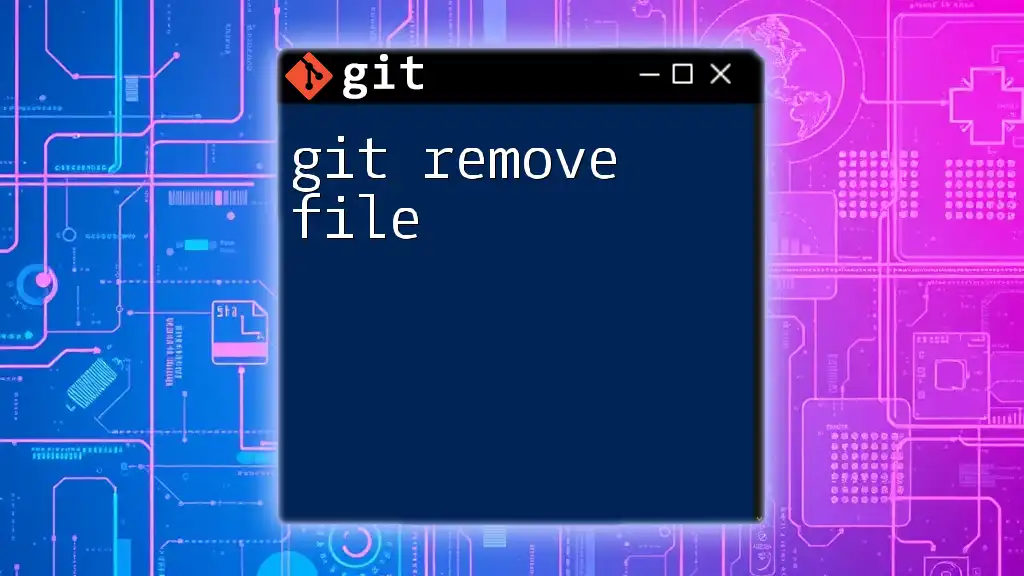
Call to Action
Ready to deepen your Git knowledge? Join our workshops or tutorials to master these commands and elevate your version control skills. Share your thoughts on this article or any questions you have as you explore the intricacies of Git!