A Git repository is a storage space where your project's files and their history are tracked, allowing you to manage version control efficiently.
git init my-repo
What is a Repository?
A repository (or repo) in Git serves as the fundamental unit for managing your project's source code. It is the structured storage space where all the files and historical changes of a project live. Understanding what a repository is and how it functions is essential for effective version control and collaboration.
Definition of a Repository
In the context of Git, a repository can be thought of as a virtual storage room that holds all the project files, along with the entire history of changes made to those files. Repositories can be categorized into two main types: Local and Remote.
-
Local Repository: This repository is stored on your own machine. You create a local repository to work on your project without the need for internet access. This means you can make changes, commit them, and keep everything organized on your device.
-
Remote Repository: A remote repository is hosted on a server, commonly using platforms such as GitHub, GitLab, or Bitbucket. This allows multiple users to collaborate on the same project from different locations and is essential for team workflows.
Importance of a Repository in Version Control
Repositories are the backbone of version control systems like Git. They track and record changes made to the project's files, enabling you to:
-
Revert to Previous Versions: If a change causes issues, you can easily roll back to a prior version of the code.
-
Branching and Merging: Create branches to experiment with new ideas without affecting the main codebase, and merge changes into the main repository once they are vetted.
-
Collaboration: Collaborators can work simultaneously on the same project through different branches and then merge their respective contributions into the main codebase.
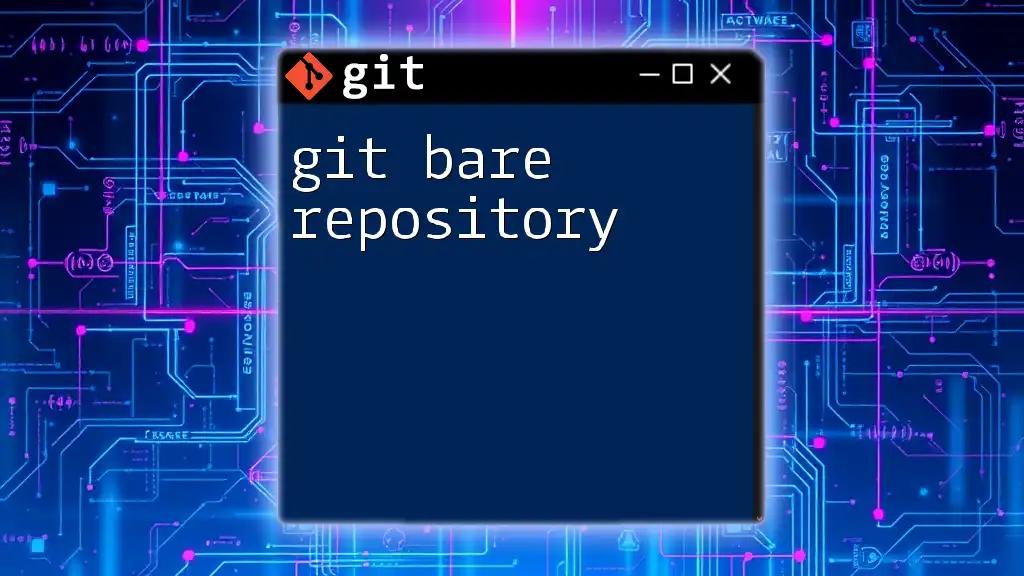
Types of Repositories
Local Repositories
A local repository is created on your computer. Setting one up is straightforward and allows you to work on your project offline.
To create a local repository, use the following command:
git init my-project
This command initializes a new Git repository in the "my-project" folder.
Local repositories are often used for personal projects, experimentation, and initial development stages before sharing with others.
Remote Repositories
A remote repository is crucial for teams and collaborative workflows. These repositories are hosted on platforms that facilitate interaction among multiple users.
To create and link a remote repository, you first need to create an account on a hosting platform like GitHub. After creating a new repository on the platform, you can link it to your local repository with:
git remote add origin https://github.com/username/my-project.git
Remote repositories allow you to share your project with others, track contributions, and ensure everyone is working with the most up-to-date version of the code.
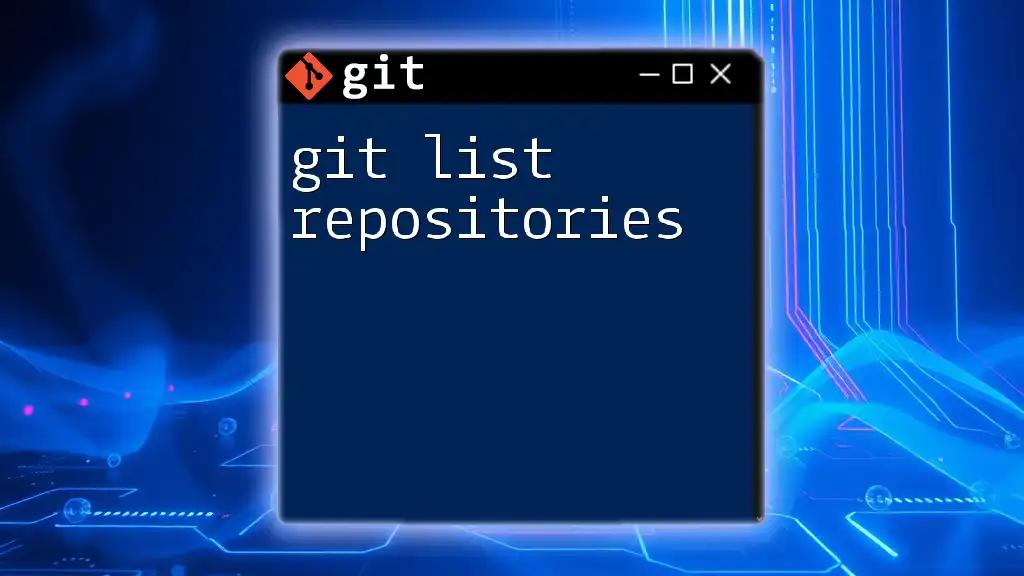
Structure of a Git Repository
Hidden .git Directory
Inside every Git repository lies a hidden directory named `.git`. This directory contains all the information necessary for Git to function effectively.
Key components of the `.git` directory include:
-
config: This file contains configuration settings for the repository. It allows you to customize various settings, like user information.
-
objects: This folder stores all the data for your commits. Each object corresponds to a specific state of the data at various points in time.
-
refs: This holds references to commits, branches, and tags, making it easier for Git to manage these items.
File Tracking and History
Git tracks changes through a sequential record of commits. Each commit acts as a snapshot of the project at a specific moment. This tracking allows developers to maintain a comprehensive history of modifications, making it easy to identify when changes were made and by whom. The ability to review commit history is invaluable for understanding project evolution and diagnosing issues that arise.
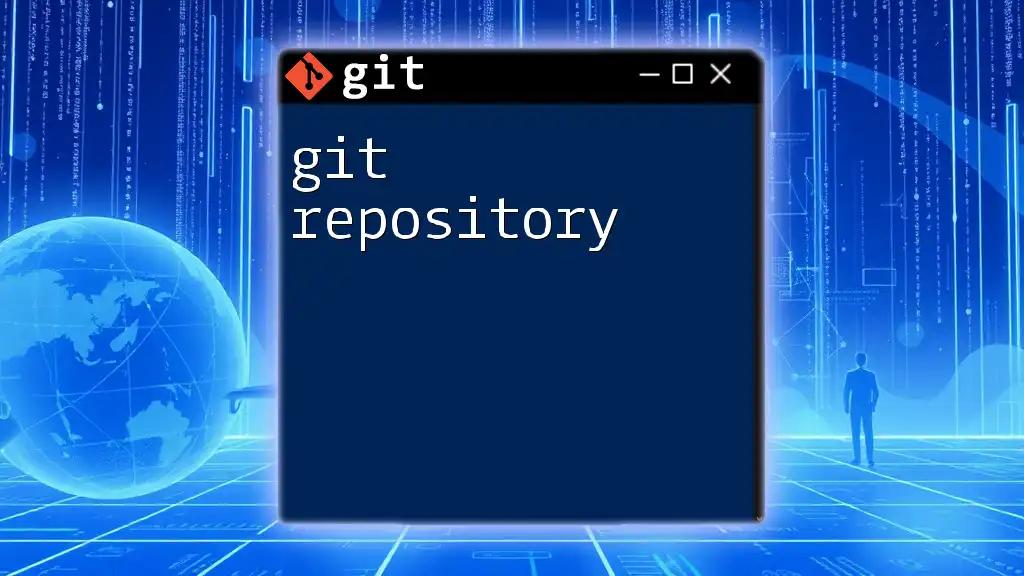
Working with Repositories
Cloning a Repository
When you want to contribute to an existing project, you typically start by cloning the repository. Cloning creates a local copy of a remote repository on your machine.
To clone a repository, use:
git clone https://github.com/username/my-project.git
This command downloads the entire repository, including all its history, allowing you to work on the project offline.
Adding Files and Making Commits
Once you have your local repository set up, you can start adding files and making changes.
To add new or modified files to the staging area, use:
git add filename
After staging files, make changes permanent by committing them with:
git commit -m "Your commit message"
Commit messages should describe the changes succinctly, providing context for anyone reviewing the project later.
Push and Pull Changes
Maintaining synchronization between your local and remote repositories is essential.
Pushing Changes: After committing your changes, you can push them to the remote repository to share your work:
git push origin main
This command uploads your committed changes to the main branch of the remote repository, ensuring that your updates are visible to collaborators.
Pulling Changes: If you're working in a team, you need to regularly update your local repository with the latest changes made by others:
git pull origin main
This command retrieves the latest commits from the remote repository and merges them into your local branch, ensuring that you’re aligned with the team's contributions.
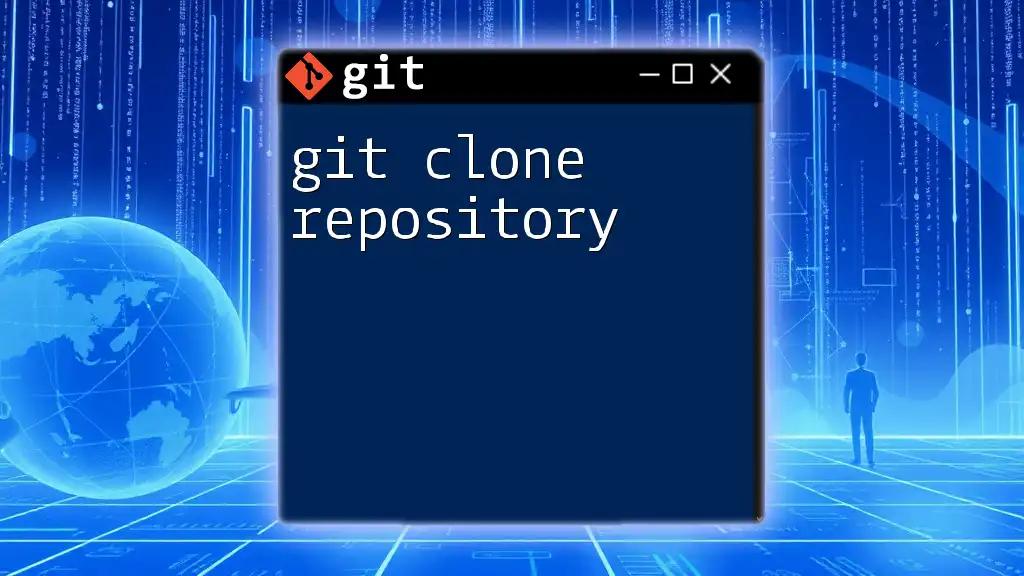
Best Practices for Managing Repositories
Organizing Your Repository
Keeping your repository organized facilitates easy navigation and understanding. Use a clear directory structure that groups related files logically, making it easier for collaborators to locate specific components.
Regular Commits and Descriptive Messages
Make commits frequently for small, logical changes. This minimizes the risk of losing progress and makes it easier to track specific changes over time. When writing commit messages, aim for clarity and relevance, so the context of your changes is transparent.
Collaborating Effectively
Effective collaboration involves using branching strategies, such as Git Flow or feature branching, to isolate different lines of development. Use pull requests for code reviews to ensure that all changes are vetted before being merged into the main codebase.
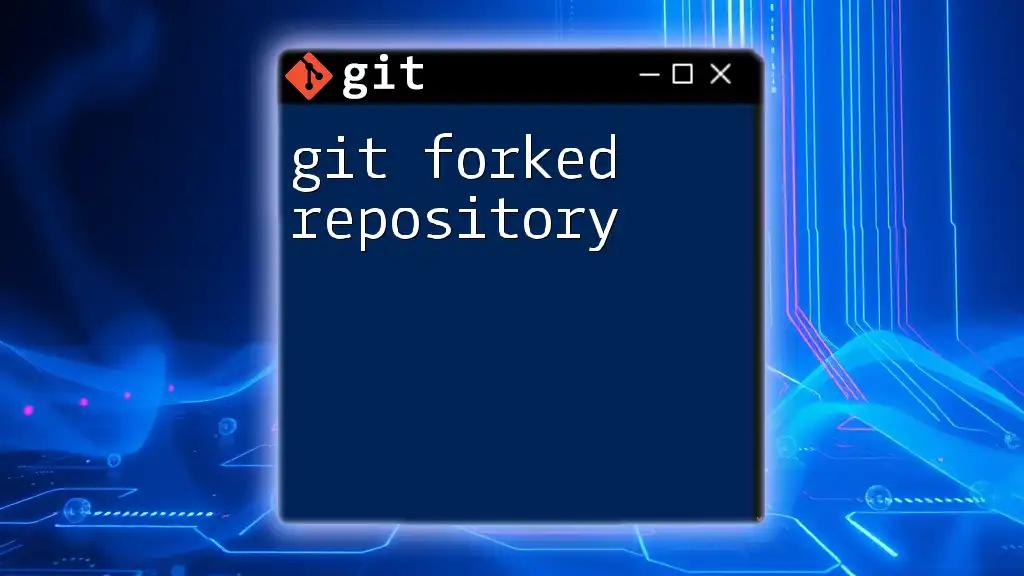
Troubleshooting Common Repository Issues
Common Errors and Fixes
Version control is not without its complexities. Common issues include:
-
Merge Conflicts: These occur when two branches have competing changes. Git will alert you to resolve these conflicts manually before proceeding.
-
Syncing Issues: If you pull changes and encounter conflicts, carefully review the changes in question and decide on the appropriate resolution strategy.
Best Tools for Repository Management
Utilizing graphical user interface (GUI) tools can streamline your Git workflow. Tools like GitKraken, GitHub Desktop, or Sourcetree provide visual representations of your repositories, making it easier to manage branches, commits, and merges compared to command-line operations.
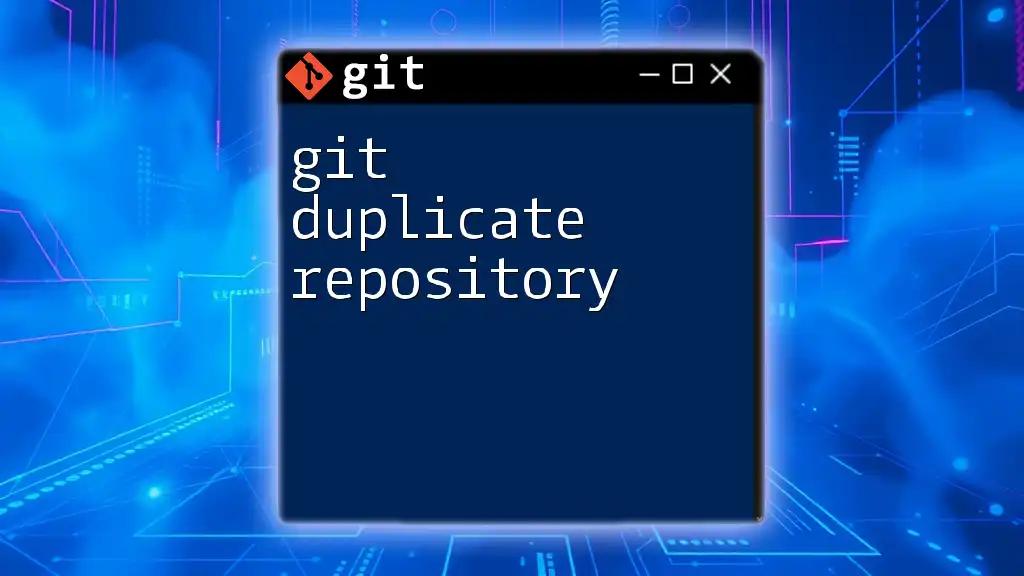
Conclusion
In summary, understanding git what is a repository is foundational for anyone looking to leverage Git for version control. Repositories serve as the central hub for managing all project files and histories, facilitating collaboration and simplifying project management. By implementing best practices and familiarizing yourself with commands, you'll enhance your efficiency and effectiveness in your software development endeavors.
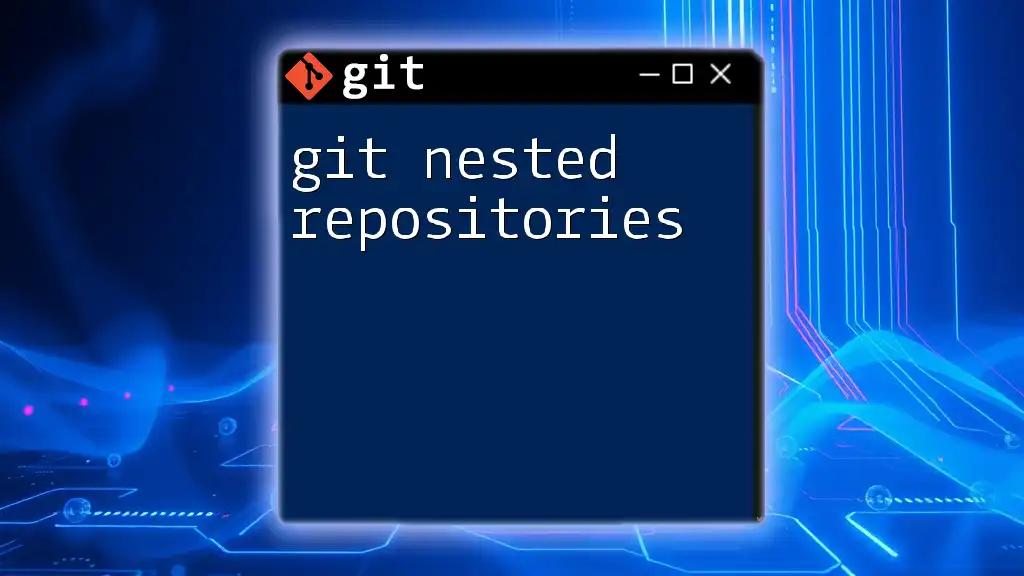
Additional Resources
To further your understanding of Git repositories, consider exploring official Git documentation, online tutorials, and community forums. These resources will provide valuable insights and assist as you navigate your journey in mastering Git.