The `git rm --cached` command is used to remove a file from the staging area in Git while keeping it in the working directory, which is useful for untracking files that you no longer want to include in version control.
git rm --cached <file_name>
What is git rm?
The `git rm` command is a fundamental tool in Git that allows you to remove files from both the working directory and the staging area (also known as the index). When you use this command, it informs Git that you don’t want to track a file anymore. This can be particularly useful in various situations like cleaning up your repository or correcting accidental additions.
Purpose of git rm: By default, the `git rm` command works in tandem with the staging process in Git. It stages the removal of the specified files, ensuring that the correct changes are captured during your next commit.
Difference between staging and working directory: In Git, your working directory is the current snapshot of your files, while the staging area collects changes you intend to include in your next commit. Understanding this distinction is crucial to effectively manage what is tracked in your Git project.
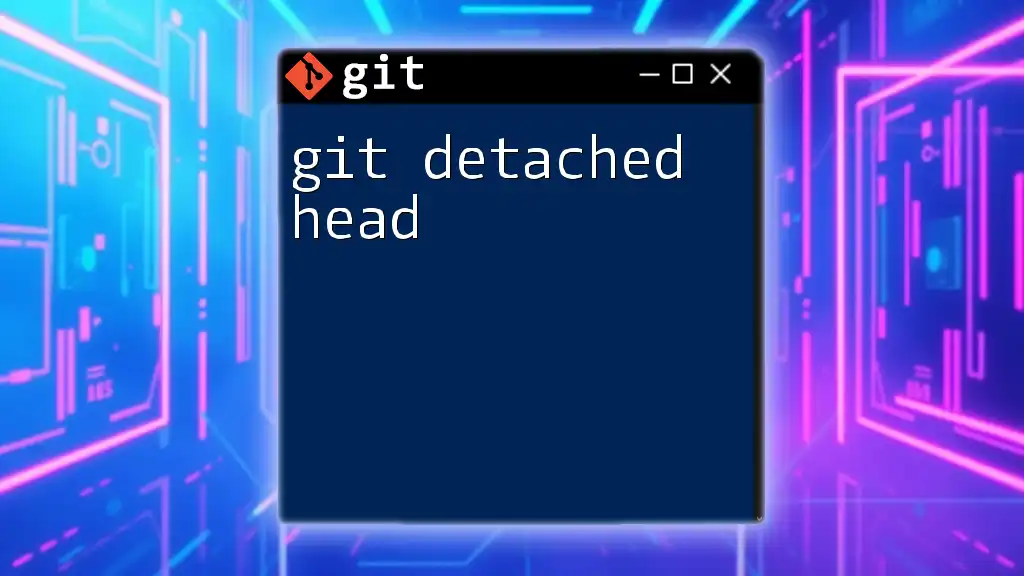
Understanding the --cached Option
What does --cached mean? The `--cached` flag alters the behavior of the `git rm` command. When you include `--cached`, Git will remove the specified file from the staging area only while leaving it intact in your working directory.
This is significant because it enables you to stop tracking changes to a file without actually deleting it from your local hard drive. Often, this approach is taken when you realize certain files should not have been tracked in the first place.
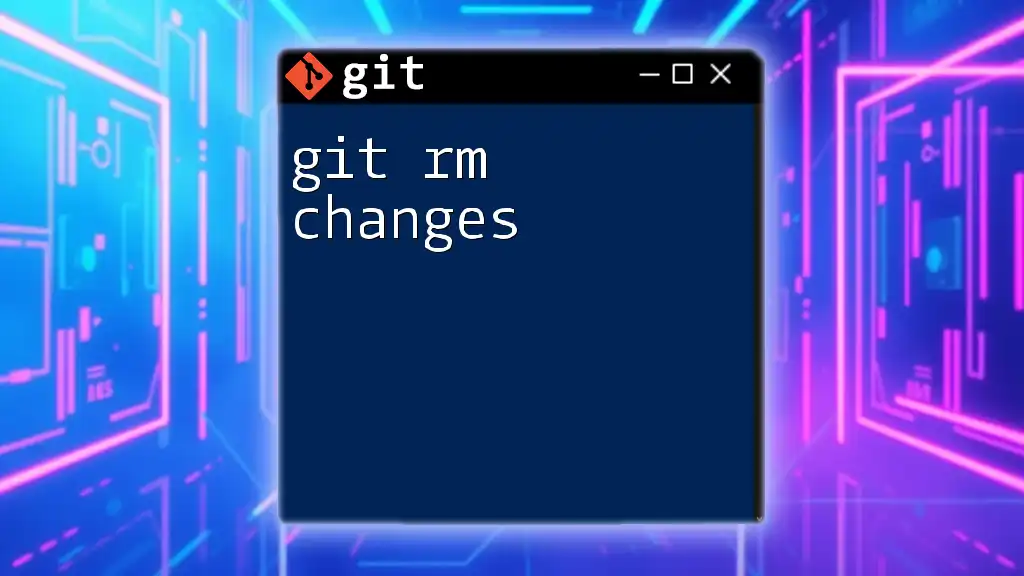
When to Use git rm --cached
Common use cases:
- Removing files you no longer want to track: As your project evolves, certain files may become irrelevant or unnecessary. Using `git rm --cached` allows you to clean up your tracked files without losing the actual files on disk.
- When a file was added by mistake: If you mistakenly add a file to your repository (for example, configuration files or temporary logs), you can easily untrack it using this command without deleting it.
- Removing sensitive files: If you've inadvertently included sensitive information in your repository, it's crucial to remove that file from tracking immediately, while keeping it on your machine to rectify the sensitive data.
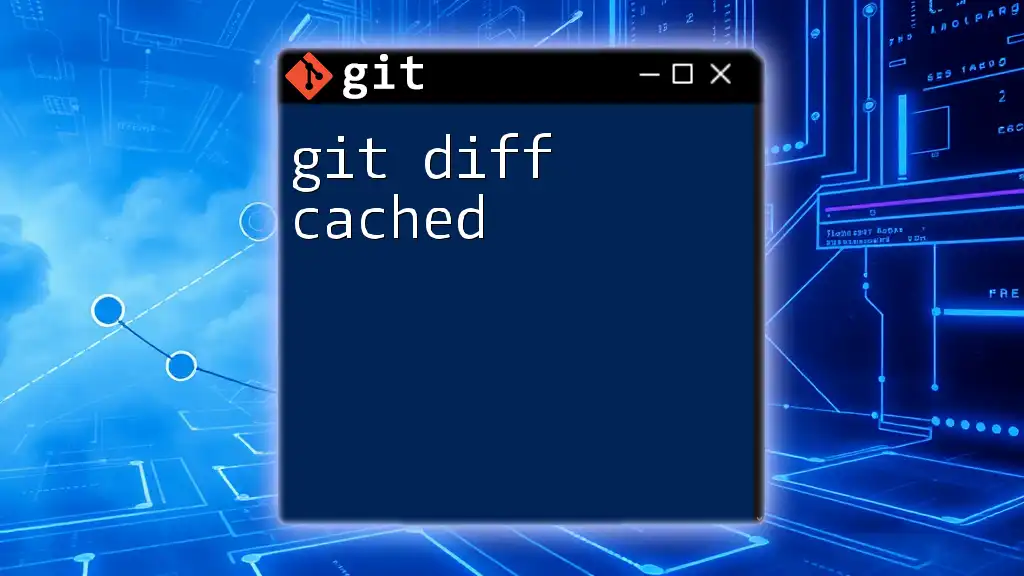
Syntax and Basic Usage
Command format: To execute the command, you would follow this syntax:
git rm --cached <file>
Example 1: Removing a Mistakenly Tracked File
For instance, if you added a file named `sensitive_file.txt` by error and wish to stop tracking it, you would execute:
git rm --cached sensitive_file.txt
After running this command, `sensitive_file.txt` will no longer be tracked by Git, but it will remain in your local file system. This step is particularly important in ensuring that sensitive or unnecessary files do not get committed to your repository.
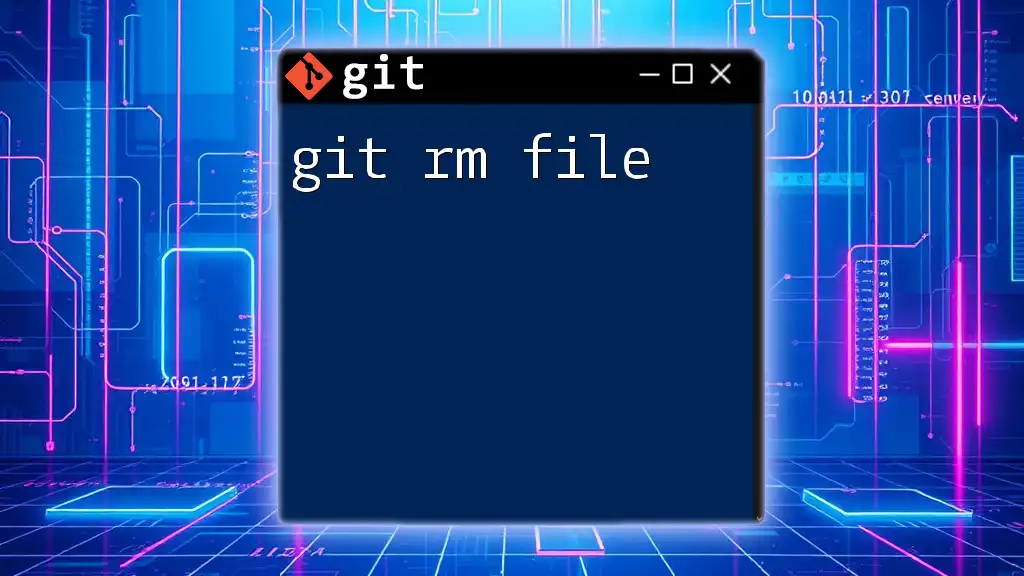
Steps to Follow After Using git rm --cached
Committing the change: After untracking a file, it’s essential to commit the change. Failing to do so means that the change could be lost and still appear in the staging area upon performing future commits. You can commit your change using:
git commit -m "Removed sensitive_file.txt from tracking"
This message helps convey the reason for untracking the file, which is beneficial for team collaboration.
Verifying the change: To ensure that the file has been successfully removed from tracking, you can run:
git status
This will list the state of files in your working directory and indicate whether the file is no longer being tracked.
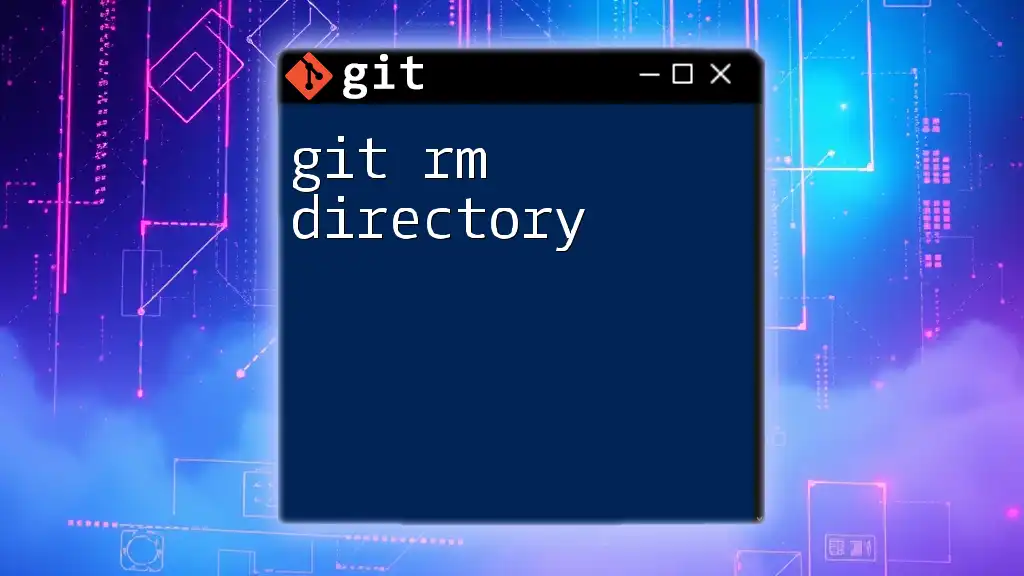
Effects on Working Directory
What happens to the file? After executing `git rm --cached`, the file is removed from the staging area, but it remains in the working directory. Users should understand that this command is powerful in managing tracked versus untracked files without losing local copies.
This is in direct contrast to using `git rm` without the `--cached` option, which would delete the file both from the index and the working directory, effectively losing the file entirely.
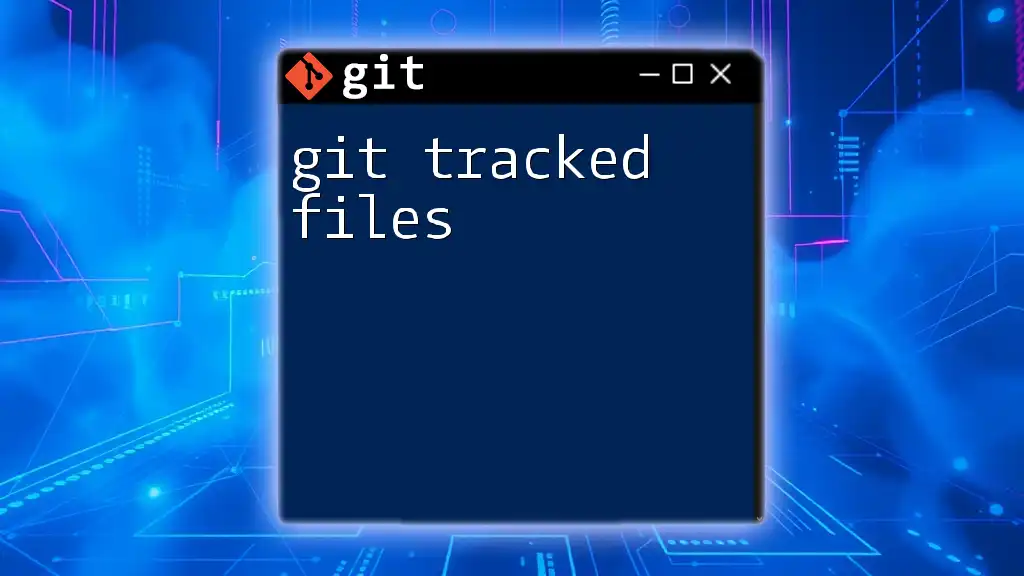
Untracking Multiple Files
How to untrack multiple files at once: If you need to untrack several files simultaneously, you can leverage wildcards. For example, to stop tracking all `.log` files in your repository, you would use:
git rm --cached *.log
This command efficiently cleans up multiple files while preserving them on your disk, saving you time and reducing manual errors.
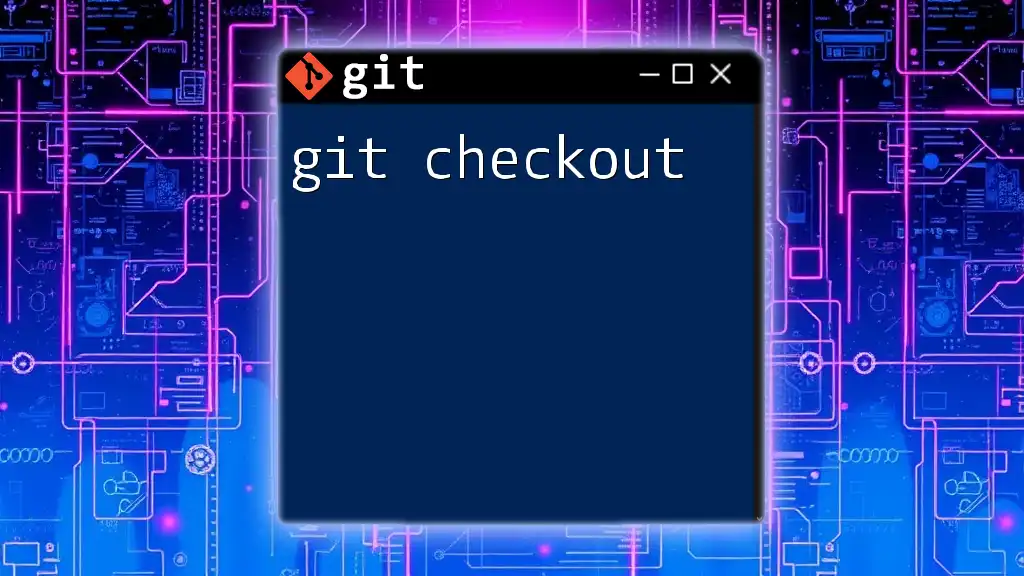
Reverting the Changes
Undoing git rm --cached: If you accidentally run `git rm --cached` on a file and want to reverse that action, it’s crucial to know how to restore the file to the staging area. You can revert the command using:
git checkout -- <file>
This command checks out the last committed version of the specified file, restoring it back to the tracked state without affecting your local copy.
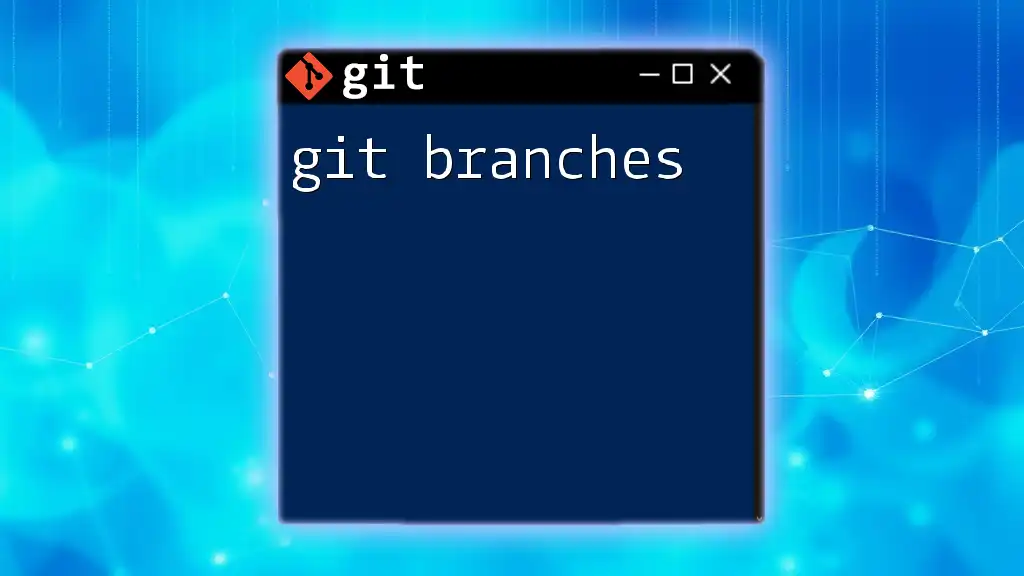
Best Practices
Tips for using git rm --cached effectively:
- Always review changes before executing the command to ensure you are untracking the correct files.
- Regularly check your `.gitignore` file to prevent unnecessary surprises or the tracking of files that should be excluded from your repository, reducing clutter and minimizing the risk of sensitive file exposure.
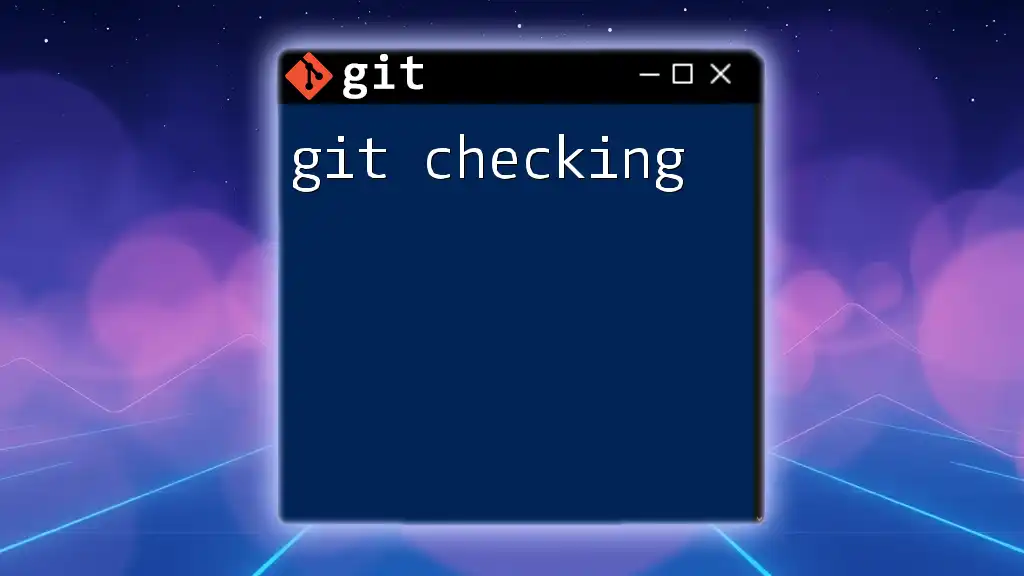
Conclusion
In summary, the `git rm --cached` command is a powerful tool for managing files in your Git repository. By understanding its purpose and effects, you can keep your project clean and efficient. Frequent use of this command can greatly enhance your workflow and maintain the integrity of your version control practices. Mastering `git rm --cached` is an essential skill for any developer, making it a valuable addition to your Git toolkit.
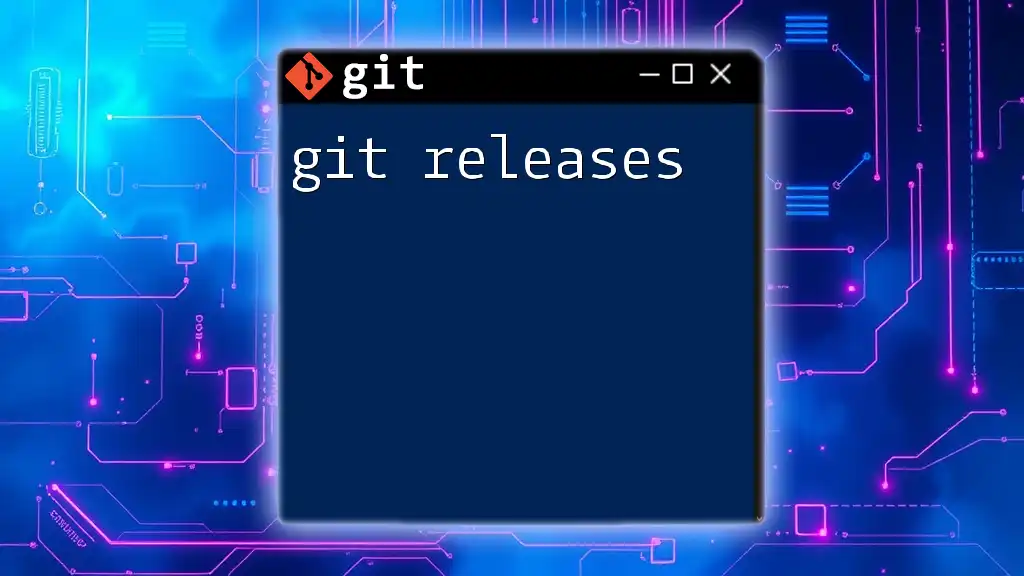
Additional Resources
For those looking to deepen their understanding of Git and version control, the official Git documentation offers comprehensive guidelines. Additionally, consider exploring online tutorials and videos that break down Git commands for beginners, as they can provide practical examples and hands-on experience.
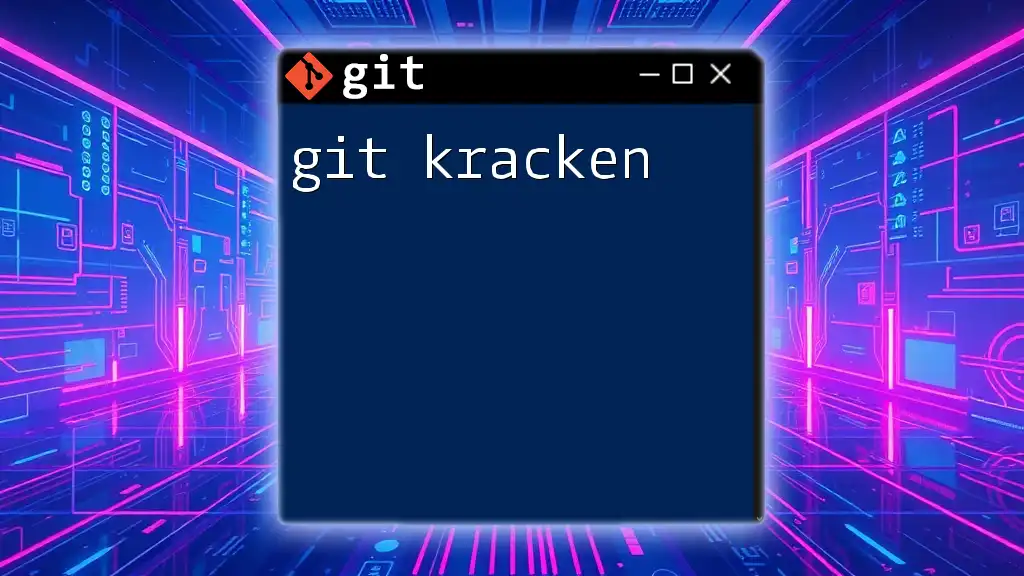
Call to Action
We encourage you to share your experiences with `git rm --cached` or ask questions in the comments section below. Keep an eye out for more articles to guide you through mastering Git commands and improving your version control skills to make your development journey as seamless as possible.