Version control with Git allows developers to track changes in their code over time, collaborate with others, and manage project history efficiently.
Here's a simple example of how to initialize a new Git repository and create your first commit:
git init # Initialize a new Git repository
git add . # Stage all changes
git commit -m "Initial commit" # Commit the changes with a message
What is Version Control?
Version control is a system that records changes to files over time. This powerful tool enables multiple people to work on a project simultaneously when collaborating on code, allowing them to track changes, revert to previous versions, and understand who made specific changes. Importance in collaborative projects cannot be overstated, as it helps manage a project's source code, ensuring that the entire team remains aligned and productive.
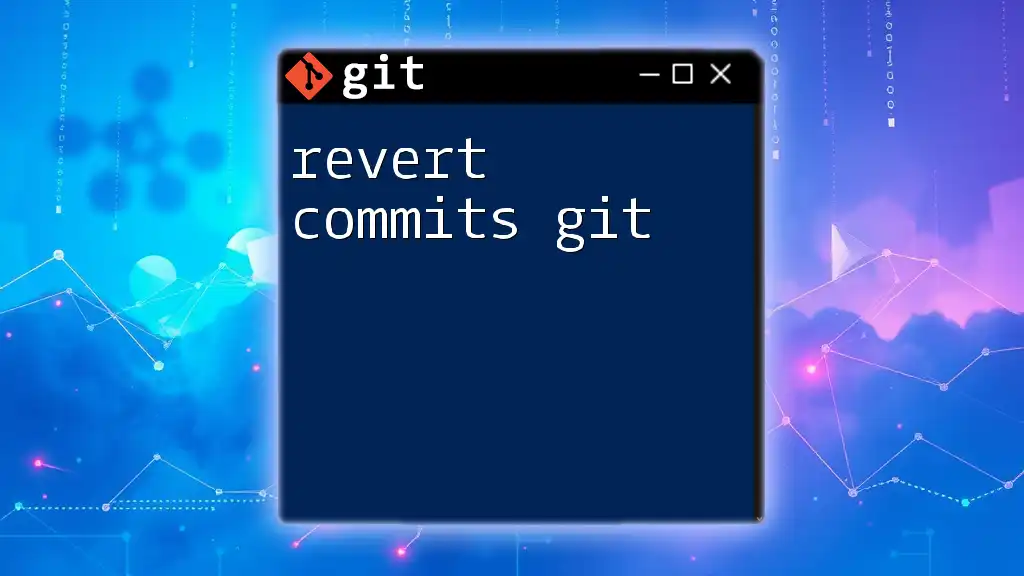
Why Choose Git for Version Control?
Git, a distributed version control system, has become the de facto standard for version control in software development. Overview of Git's features includes excellent branching and merging capabilities, performance, scalability, and a large community of users that provide extensive documentation and support. Additionally, Git is powered by a local repository, meaning you can work offline and sync your changes later—an invaluable feature for many developers.
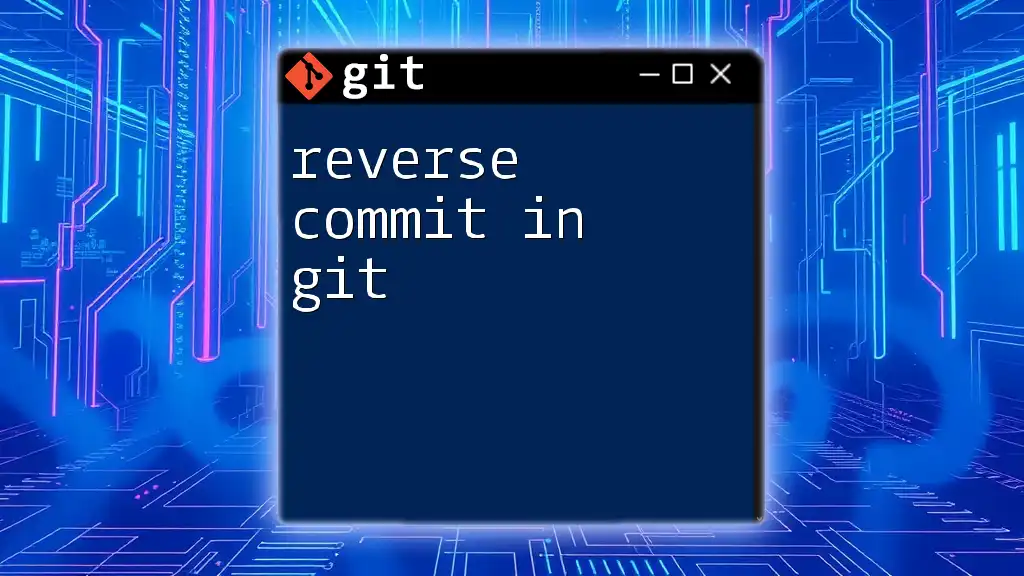
Understanding Git Basics
What is Git?
Git is an open-source distributed version control system that allows for efficient workflows across different projects and teams. Unlike centralized systems like SVN, Git repositories can be created and maintained on multiple machines, allowing each contributor to have their own complete version of the project.
Key Terminology in Git
Before diving deeper into practical applications, it’s essential to grasp some key terminology related to Git:
- Repository (Repo): A directory that contains your project's files along with the entire history of changes.
- Commit: A snapshot of your project at a point in time, which includes a unique ID and a message describing the changes.
- Branch: A divergent line of development that allows for experiments and new features without affecting the main codebase.
- Merge: A command that integrates changes from one branch to another.
- Conflict: An issue that arises when merges fail because two branches have made changes to the same line of the same file.
- Remote: A version of your project that is hosted on the internet or a network, generally on platforms like GitHub or GitLab.
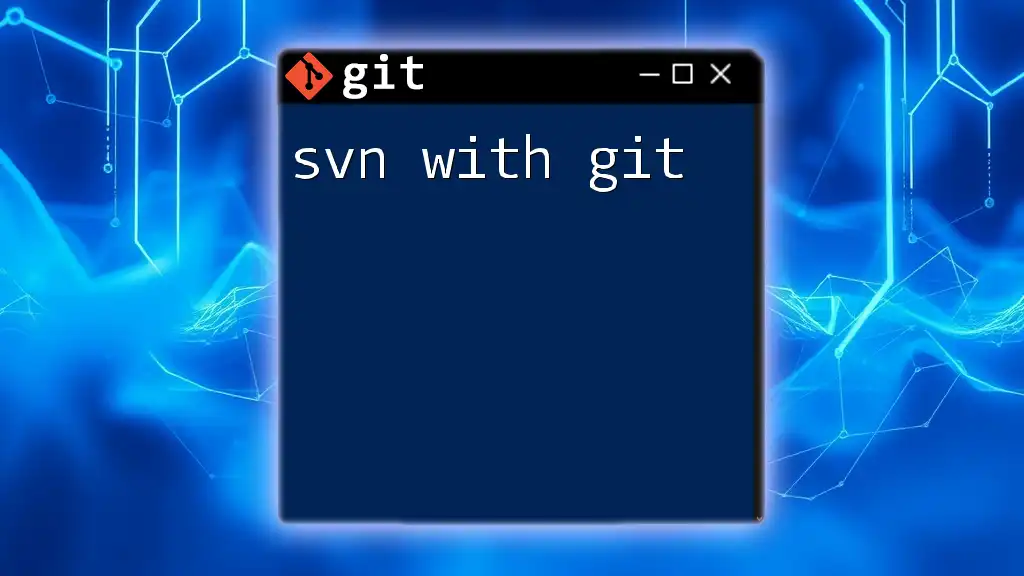
Getting Started with Git
Installing Git
To begin your journey with version control using Git, you need to install Git on your machine. Here are instructions for various operating systems:
- Windows: Download the installer from [Git for Windows](https://gitforwindows.org/) and follow the setup prompts.
- macOS: You can install Git using Homebrew with the command:
brew install git
- Linux: Install Git using your package manager:
sudo apt-get install git # For Debian-based systems
Setting Up a New Git Repository
To create a new repository for your project, navigate to your desired project folder and run:
git init my-repo
This command initializes a new Git repository, creating a hidden `.git` directory that stores your project history.
Configuring Git
Before using Git for your projects, it’s important to configure your user information:
git config --global user.name "Your Name"
git config --global user.email "your-email@example.com"
This information will be associated with your commits, helping others to identify authorship.
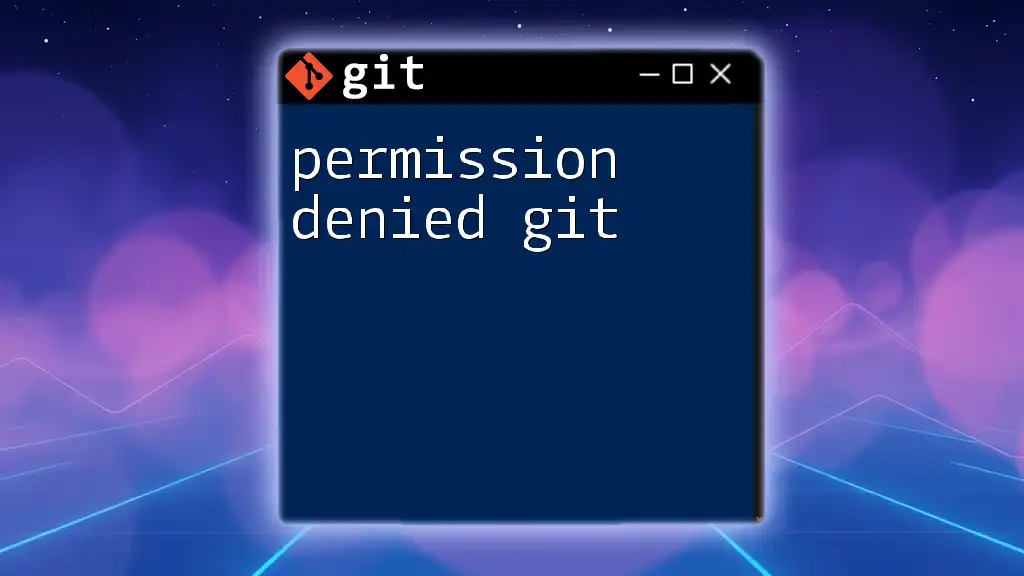
The Git Workflow
Creating a New File and Making Changes
Once your repository is set up, you can begin adding files. For example:
touch example.txt
git add example.txt
This `git add` command stages the file for commit, making it ready to record in the repository history.
Stage and Commit Changes
Staging changes is a crucial part of the Git workflow. After modifying files, you can commit those changes:
git commit -m "Initial commit for example.txt"
This command captures a snapshot of your project's current state with a descriptive message, allowing you to recall changes later.
Checking the Status of Your Repository
To view the current status of your repository, including uncommitted changes and branch status, you can use:
git status
This command provides a clear view of your working directory, indicating which files are staged, modified, or untracked.
Viewing Commit History
To view the history of commits in your repository, utilize the `git log` command:
git log
This reveals a chronological list of changes, including commit messages, author information, and timestamps, which is invaluable for tracking project progression.
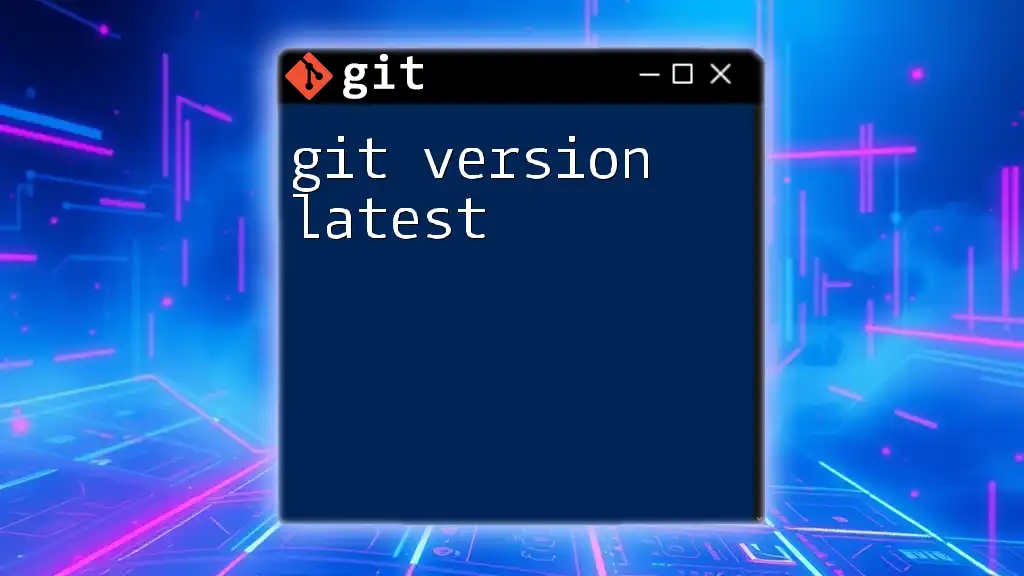
Branching and Merging
Understanding Branches
Branches allow developers to create a divergent line of development, enabling them to work on new features and bug fixes separately from the main code base without impacting stable code. This makes it easier to experiment and maintain a clean workflow.
Creating and Switching Branches
Creating a new branch is straightforward:
git branch new-feature
git checkout new-feature
You can also combine these steps into one command using:
git checkout -b new-feature
This creates a new branch and switches you to it immediately.
Merging Branches
After completing work on a new branch, the next step is to merge it back into the main branch. Perform the following commands:
git checkout master
git merge new-feature
During this process, you may encounter merge conflicts if changes to the same line exist in both branches. Git will highlight these issues for you to resolve manually.
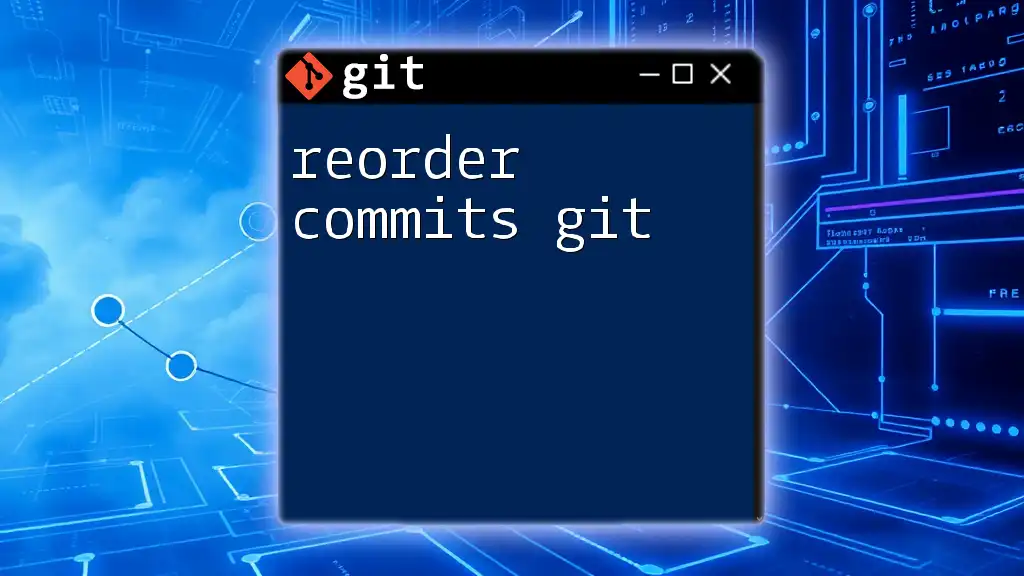
Collaborating with Others
Using Remote Repositories
Remote repositories are essential for team collaboration. They enable developers to share code online efficiently. Popular platforms include GitHub, GitLab, and Bitbucket.
Cloning a Repository
To collaborate on an existing project, you can clone it using:
git clone https://github.com/user/repo.git
This command copies the entire repository, allowing you to work with the same files and commit history.
Pushing Changes to a Remote Repository
Once you've made changes and committed them, you can push your local commits to the remote repository:
git push origin master
This sends your changes to the specified branch in the remote repository, making them accessible to others.
Pulling Changes from a Remote Repository
To ensure your local repository remains up-to-date with the latest changes from your team, use:
git pull origin master
This command fetches and merges changes from the remote branch into your local branch.
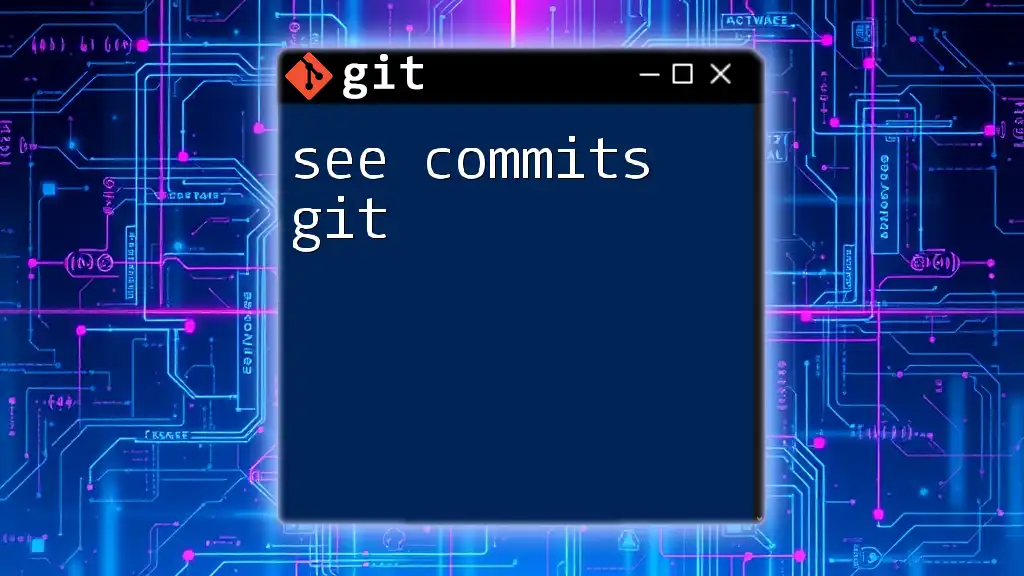
Best Practices for Version Control
Commit Messages
Writing clear and concise commit messages is crucial for maintaining a good project history. Strive for informative messages that explain the "why" behind changes:
- Good Example: “Fix bug in user login”
- Bad Example: “Misc changes”
Regularly Syncing with Remote
To keep your team in sync, make it a habit to pull changes frequently and push your commits regularly. This minimizes conflicts and ensures everyone is working with the current version of the project.
Feature Branches for New Developments
Utilizing feature branches for developing new features is recommended. This approach maintains a clean main branch and allows for thorough testing before merging into the production code.
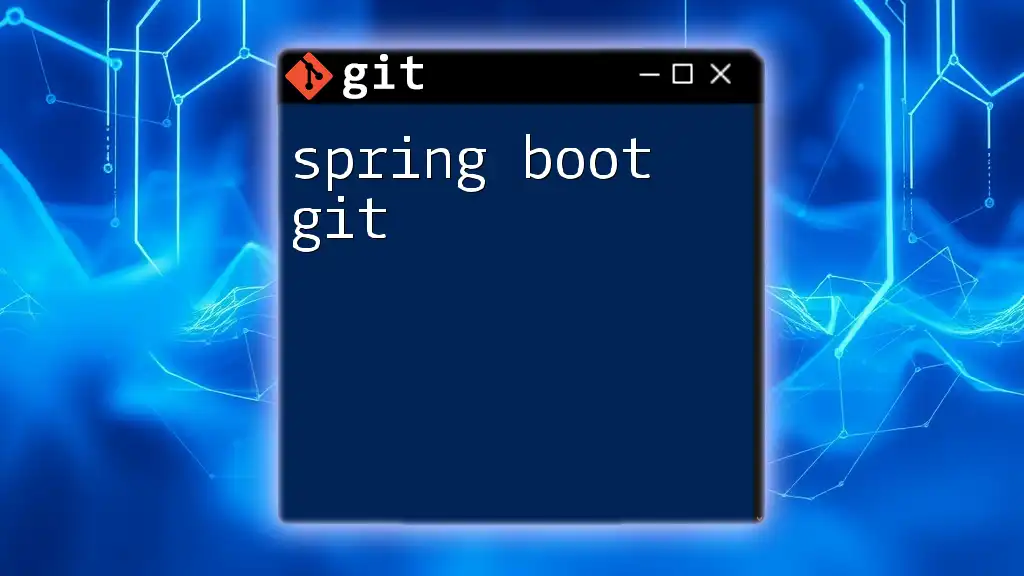
Troubleshooting Common Git Issues
Recovering Deleted Commits
If you accidentally delete important commits, you can recover them using:
git reflog
This command displays a log of all actions performed with Git, including deleted or orphaned commits.
Fixing Merge Conflicts
Dealing with merge conflicts requires careful attention. When conflicts arise, Git will mark the conflicting areas in files. You can resolve these by editing the affected files, staging the resolved files using `git add`, and then completing the merge with `git commit`.
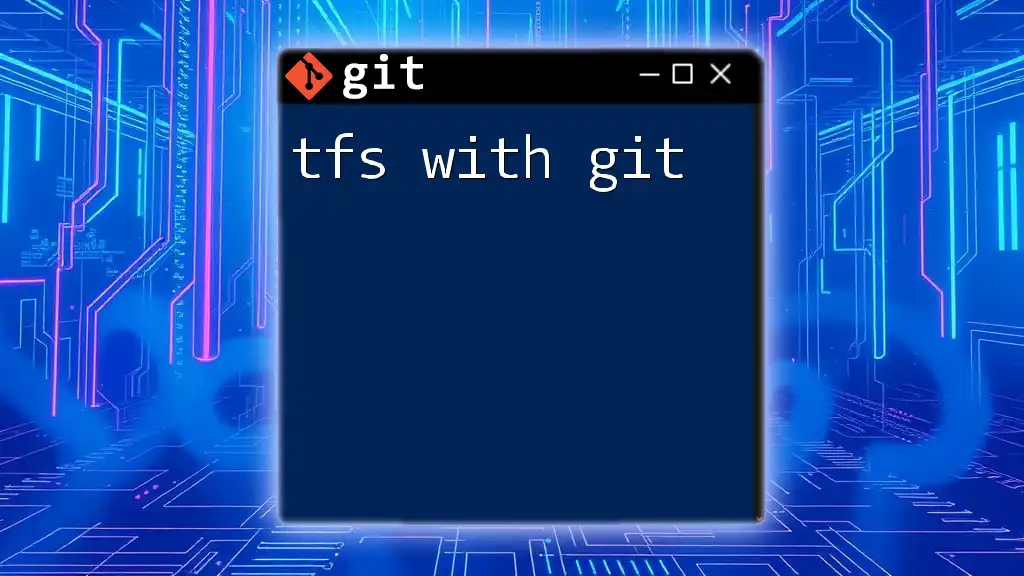
Conclusion
Understanding version control with Git is fundamental for anyone involved in software development. By mastering Git commands and workflows, you will enhance your collaborative abilities, maintain project organization, and streamline development processes.
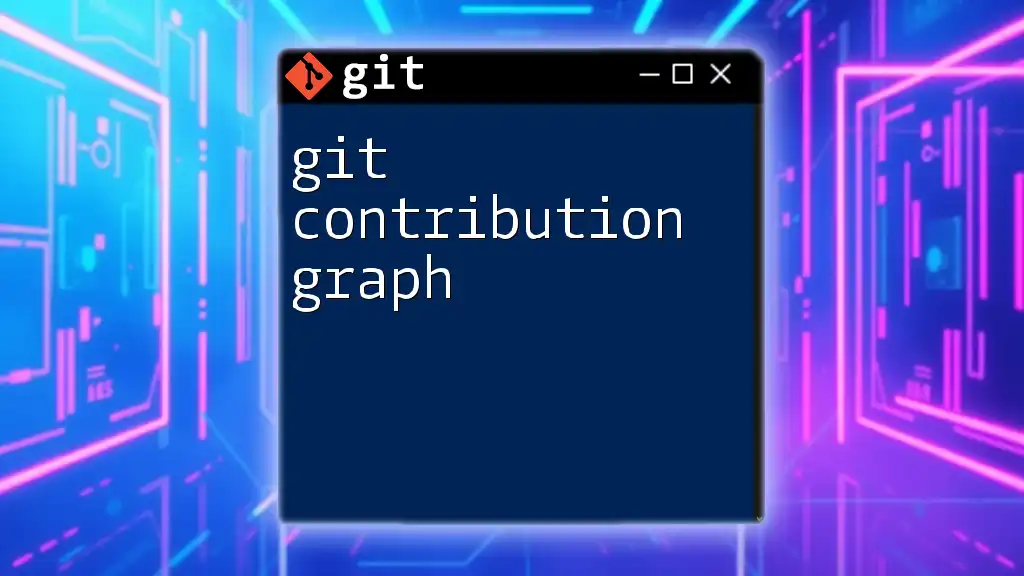
Call to Action
Join our Git training program to further develop your skills and understand the nuances of Git that will empower your development journey. Follow us on social media for the latest tips, tricks, and resources to make the most of version control with Git!