Source control management (SCM) with Git enables developers to efficiently track changes in their source code, collaborate with others, and manage project versions.
Here's a simple example of how to initialize a new Git repository:
git init
Understanding Source Control Management
Source Control Management (SCM) plays a critical role in software development by enabling teams to track changes, manage multiple versions of code, and collaborate seamlessly. It primarily revolves around maintaining and controlling changes to the codebase over time.
What is Git?
Git is a distributed version control system (DVCS) that helps developers track changes and collaborate on projects effectively. Developed by Linus Torvalds in 2005, Git has since evolved into one of the most popular version control systems in the world due to its efficiency, speed, and powerful branching features that facilitate parallel development.
Key Features That Set Git Apart
- Distributed Architecture: Each developer has a full copy of the repository, allowing local operations without the need for network access.
- Branching and Merging: Git's lightweight branching system empowers developers to create feature branches seamlessly, enhancing collaborative workflows.
- Performance: Git is optimized for performance, making operations like commits and merges fast, even for large projects.
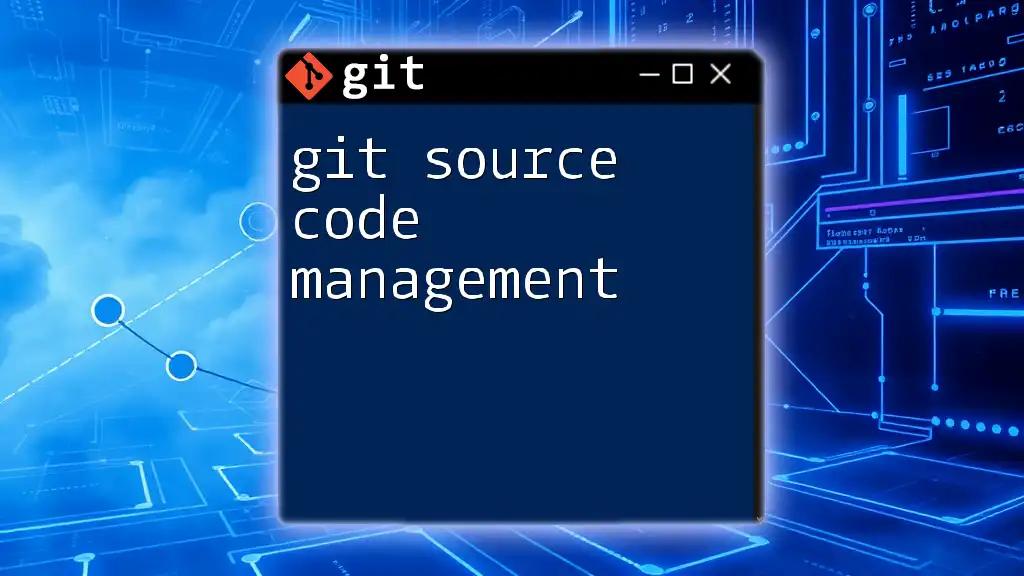
Fundamental Concepts in Git
Repositories
A Git repository is a storage space for your project where Git tracks all changes. There are two types of repositories:
- Local Repository: Contains a copy of the project on your machine.
- Remote Repository: An online version of your project, which allows multiple contributors to collaborate.
To create a new repository, navigate to your project directory and run:
git init
Commits
A commit represents a snapshot of your project at a particular point in time. Every time you commit changes, you save the current state of your files. This enables you to revert your project back to any previous state.
Importance of Commit Messages: A well-crafted commit message details what changes were made and why they were necessary. This practice aids in understanding project history. For example:
git commit -m "Fix login bug by validating user inputs"
Branches
Branches allow you to create multiple versions of your code independently. The default branch is usually called `main` or `master`, with branches created for features, bug fixes, or experiments.
To create and switch to a new branch, use:
git checkout -b feature-branch
Merging
Merging is the process of integrating changes from one branch into another. There are two main types of merges:
- Fast-Forward Merge: If no new commits are made on the target branch, Git simply moves the reference to the latest commit.
- Three-Way Merge: If there are new commits on both branches, Git creates a new merge commit that combines both histories.
In case of a conflicting change during a merge, Git will indicate this conflict, allowing you to resolve it manually before proceeding.
git merge feature-branch
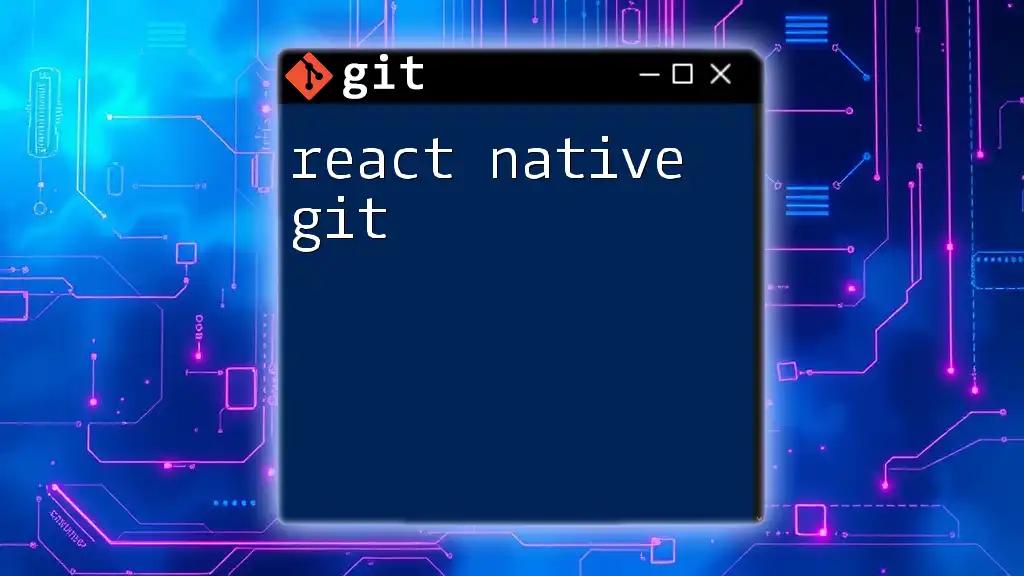
Essential Git Commands for Effective Source Control Management
Setting Up Your Git Environment
Before diving into commands, you need to install Git. Visit the official [Git website](https://git-scm.com/) for installation instructions based on your operating system.
Once installed, configure your Git user settings to identify yourself:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Cloning and Creating Repositories
To clone an existing repository, which is a common practice when starting a project, use:
git clone https://github.com/username/repo.git
Basic Day-to-Day Commands
- Checking the Status of Your Files: Before committing, check which files are modified or untracked.
git status
- Staging Changes: After making edits, you need to stage the changes for commit. Add files to the staging area by using:
git add .
- Committing Changes: Save your staged changes to the repository with:
git commit -m "Your commit message"
- Pushing Changes to a Remote Repository: After committing changes, push them to a remote repository to share them with others:
git push origin main
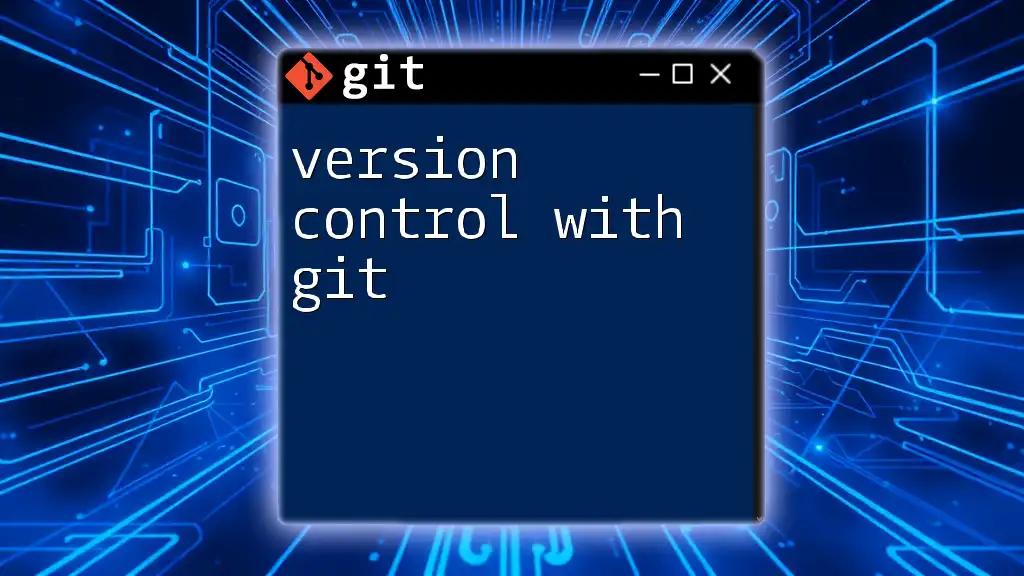
Advanced Git Workflows
Collaborative Workflows with Branching Strategies
Using well-defined branching strategies encourages organized collaboration. Git Flow is a popular branching model that separates development into stages, such as feature, release, and hotfix branches. In contrast, GitHub Flow simplifies this to just one primary branch and feature branches, suited for environments with continuous deployment.
Pull Requests and Code Reviews
A pull request (PR) is a method for submitting contributions to a project. It initiates a discussion about the proposed changes and enables code reviews, which are essential for maintaining code quality.
Engage your teammates to review your pull requests before merging to ensure that changes are sound and free of bugs.
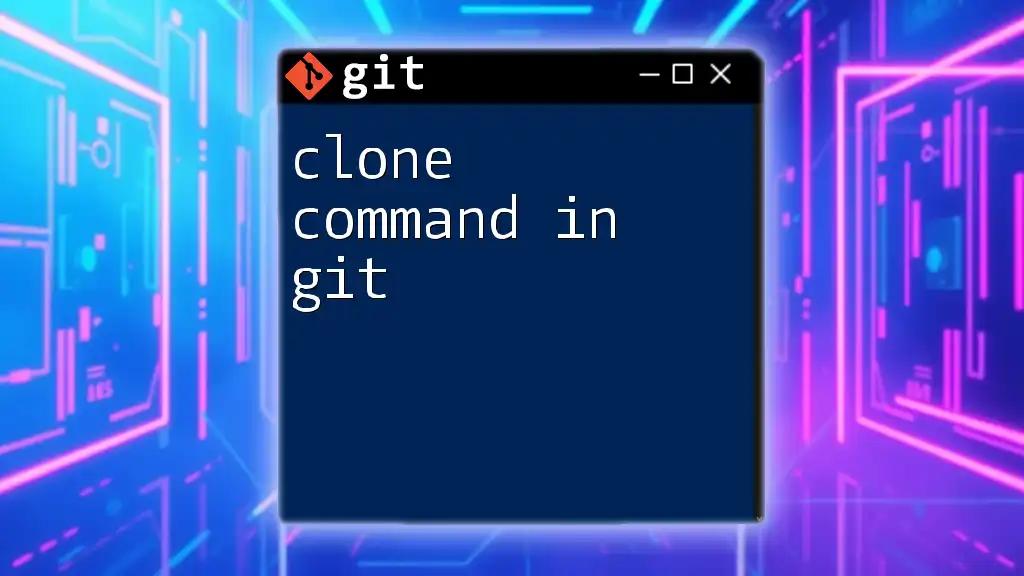
Managing Remote Repositories
Connecting to Remote Repositories
To connect your local repository to a remote one, add a remote reference:
git remote add origin https://github.com/username/repo.git
Fetching and Pulling Changes
Use `fetch` to retrieve the latest commits from the remote repository without merging:
git fetch origin
To fetch and merge changes into your current branch, use:
git pull origin main
Handling Remote Branches
To see all branches, both local and remote:
git branch -a
To delete a branch from the remote repository:
git push origin --delete branch-name
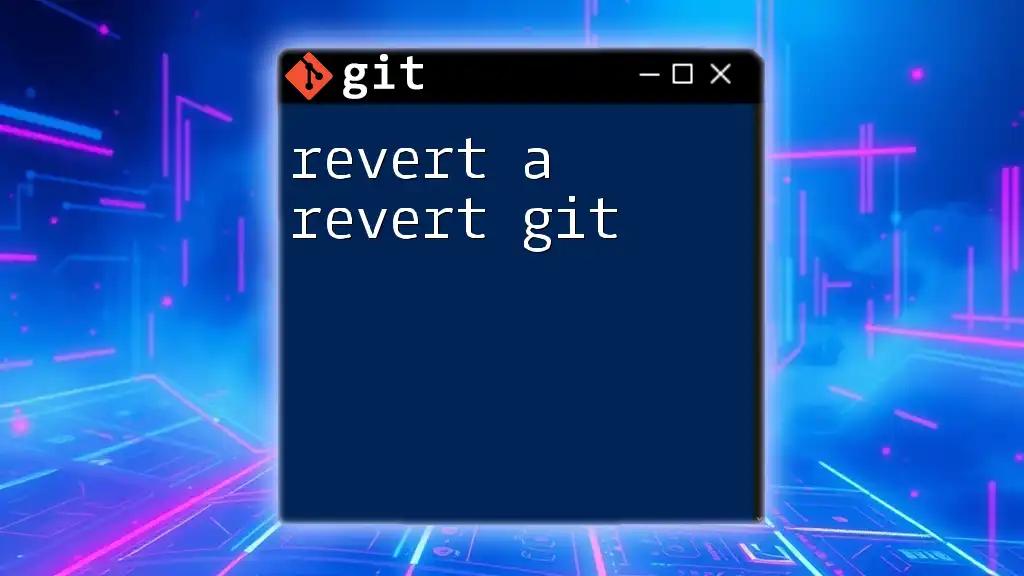
Best Practices in Git
Writing Clear Commit Messages
Good commit messages make it easier to understand the history of a project. Follow a structure: start with a verb in the imperative mood (e.g., "Fix," "Add," "Update") and include context when necessary.
Maintaining a Clean Repository
Regularly clean your branches to declutter your repository. Open branches that are no longer needed can be deleted to maintain clarity. You can also tag releases to mark stable versions of your project:
git tag -a v1.0 -m "Release version 1.0"
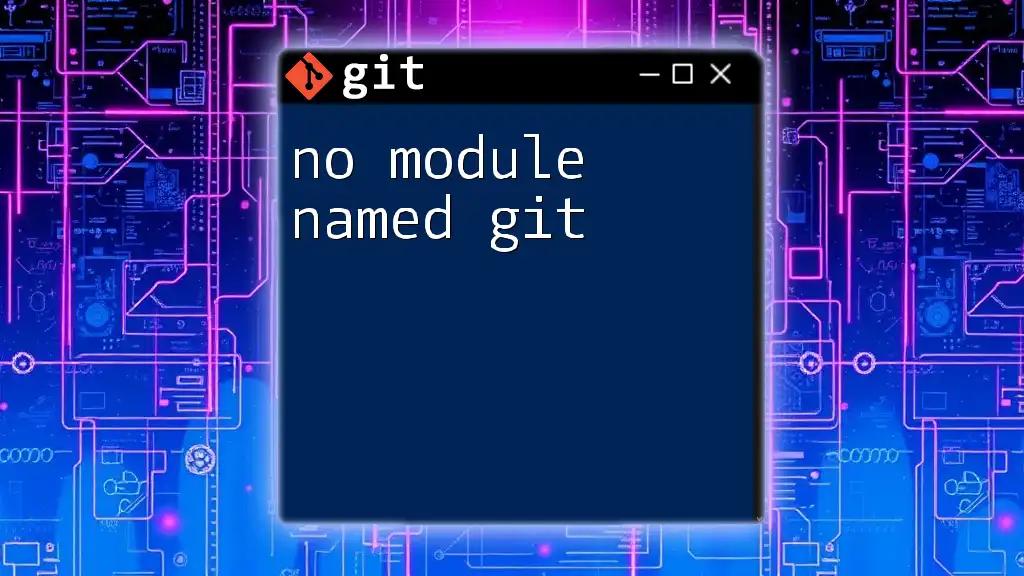
Tools to Enhance Git Workflow
Graphical User Interfaces for Git
While command line Git is powerful, many prefer graphical user interfaces (GUIs) like Sourcetree or GitKraken for a more visual and intuitive experience.
Integrating Git with Other Tools
Employ CI/CD pipelines to automate the testing and deployment of your code. Tools like Jenkins or GitHub Actions work seamlessly with Git to enhance your development workflow.
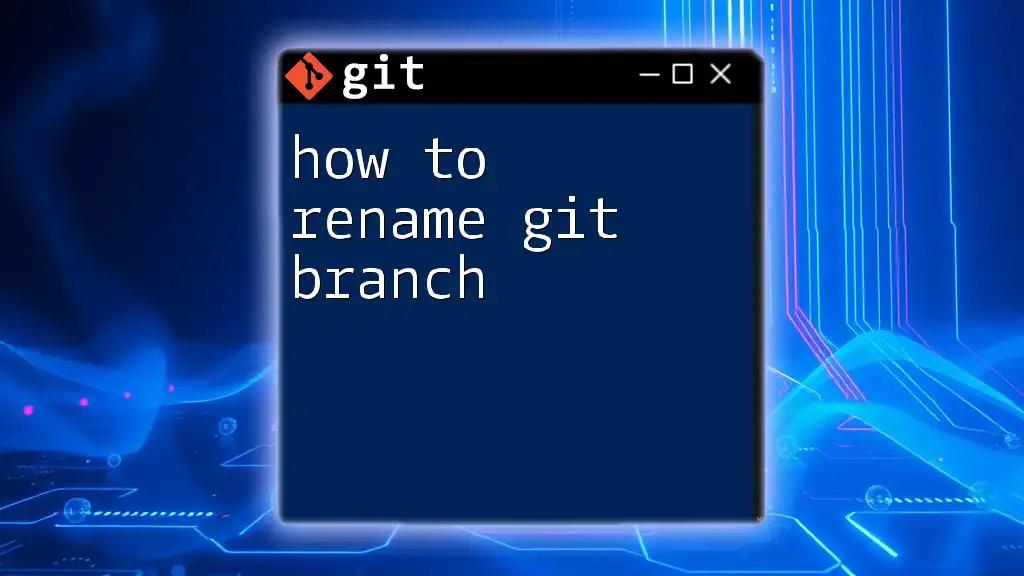
Troubleshooting Common Git Issues
Understanding Git Errors
Git can throw various error messages. Knowing how to interpret these can save time and frustrations. Common errors include merge conflicts and authentication issues.
Reverting Changes
Sometimes you may need to undo changes. To revert a commit, use:
git revert <commit-id>
To reset your branch to a previous commit and lose all changes since then, use:
git reset --hard <commit-id>
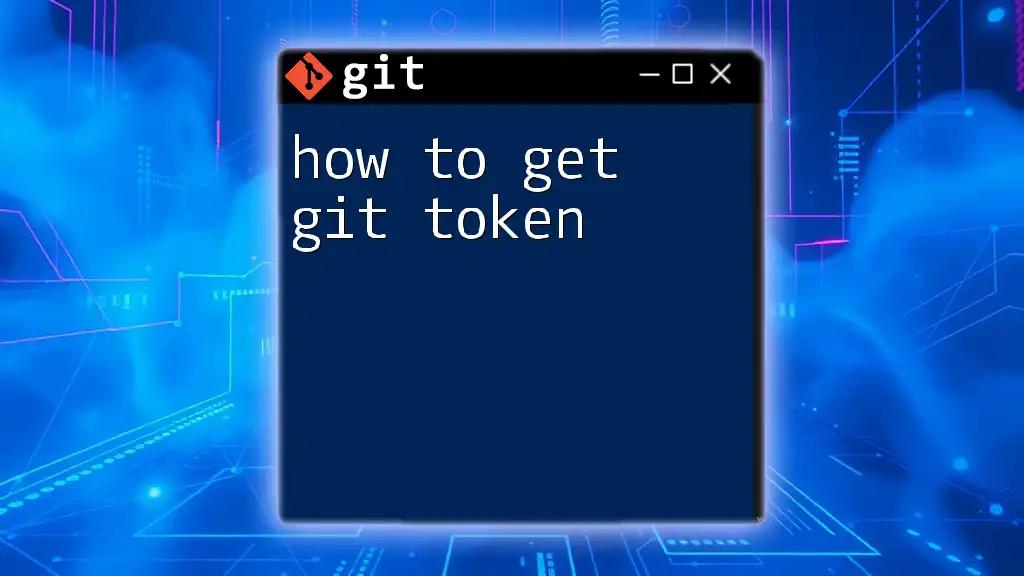
Conclusion
Mastering source control management with Git is essential for modern software development. With the ability to track changes, collaborate effectively, and manage code versions, Git is an indispensable tool in any developer's toolkit. Practice using these commands, explore further resources, and engage with the Git community to enhance your skills!