The error "No module named git" typically indicates that the GitPython library isn't installed in your Python environment, and you can resolve it by running the following command:
pip install GitPython
Understanding the Error
What Does "No Module Named Git" Mean?
The error message "No module named git" typically indicates a problem with your Python environment where the GitPython module is either not installed or not accessible. This issue commonly arises when you're attempting to import the GitPython module but the Python interpreter can't locate it.
Importance of Python Environment and Modules
Python operates in environments designed to contain specific modules and packages. When a module isn’t found within the currently active environment, Python raises the "No module named" error, prompting you to take corrective actions.
Common Causes of the Error
-
Lack of Installation of the GitPython Module: The most obvious reason for encountering this error is that GitPython hasn’t been installed in your current Python environment.
-
Incorrect Python Environment: Many developers use virtual environments to manage dependencies. If you're in the wrong environment, Python may not find the Git module.
-
Typographical Errors in Import Statements: Simple misspellings can also lead to the error. Even an incorrect capitalization can make Python unable to find the module.
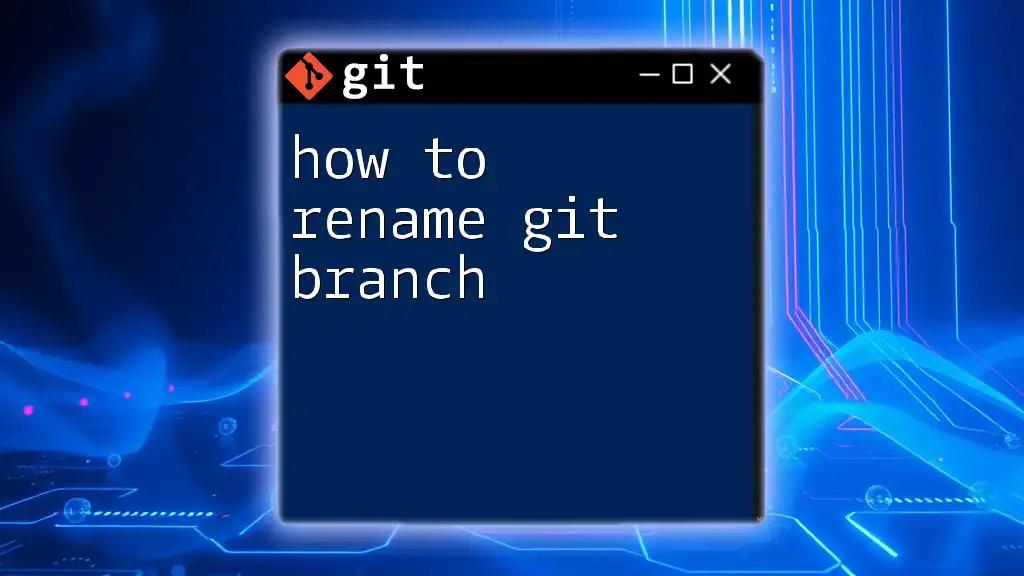
Setting Up Your Environment
Installing GitPython
To resolve the "no module named git" error, the first step is to make sure that the GitPython package is installed.
Step 1: Ensure Python and pip are installed. You can verify your installations by running:
python --version
pip --version
Step 2: If Python and pip are installed correctly, you can install the GitPython module using pip:
pip install GitPython
Verifying the Installation
After installing GitPython, it’s essential to validate that it has been installed correctly. You can do this by simply importing the module in a Python script:
import git
print(git.__version__) # Outputs the version of the installed Git module
If you see the version number without any errors, GitPython is successfully set up!
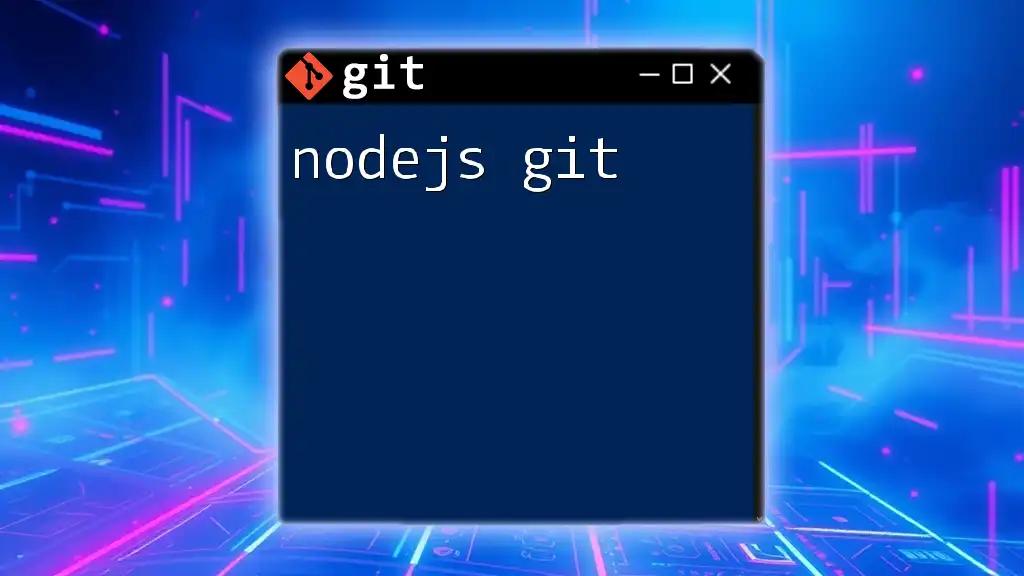
Troubleshooting the Error
Checking Your Python Environment
Sometimes the problem stems from being in the wrong Python environment. It's crucial to activate the correct virtual environment where GitPython is installed.
If you are using a virtual environment created with `venv`, activate it with:
source venv/bin/activate # For Mac/Linux
.\venv\Scripts\activate # For Windows
Once activated, running the import statement again should no longer raise an error.
Common Pitfalls
- Typographical Errors: Always double-check your import statement. The correct syntax is:
import git # Correct
If you've misspelled ‘git’, like `import gtt`, it will result in the "no module named git" error.
- Conflicts with Other Modules: Sometimes, certain Python packages might conflict with GitPython. If you suspect this may be the case, consider creating a new virtual environment and only installing GitPython initially to test it.
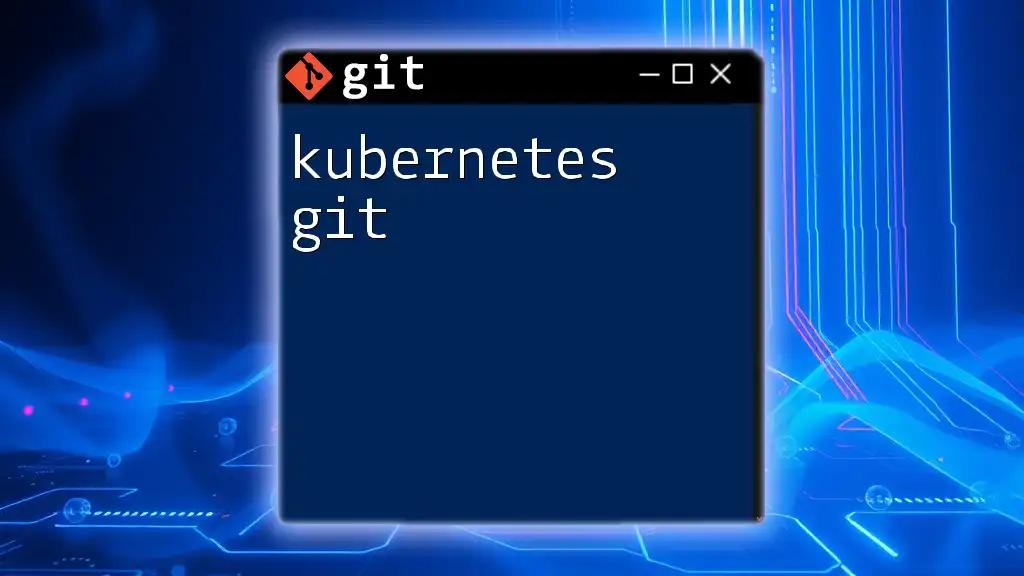
Alternative Solutions
Reinstalling or Upgrading GitPython
If you’ve confirmed your environment is correct but still encounter the error, consider upgrading or reinstalling GitPython. Use the following commands:
To upgrade:
pip install --upgrade GitPython
To uninstall and then reinstall:
pip uninstall GitPython
pip install GitPython
Using a Different Python Version
If you are running into compatibility issues, you might need to switch to a different Python version. Python versions can introduce various third-party module support issues.
To install a specific Python version using pyenv, follow these commands:
pyenv install 3.9.6
pyenv global 3.9.6
After installing the desired version, ensure you reinstall the necessary packages, including GitPython.
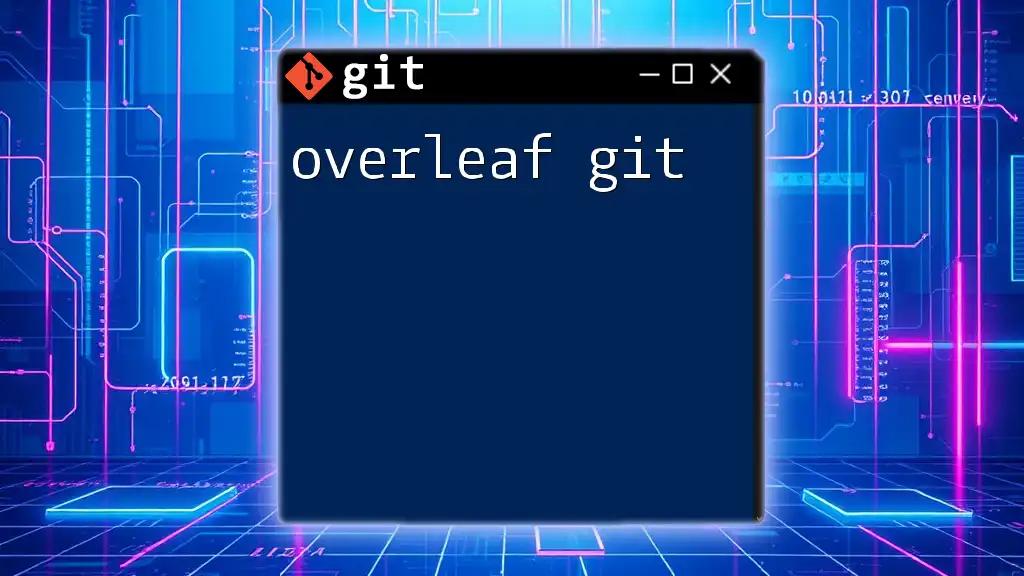
Best Practices for Using GitPython
Writing Effective Scripts
When writing scripts that use GitPython, make sure to manage repositories carefully. Utilize exception handling to catch errors that may arise during repository operations. Here is an example snippet for cloning a repository:
from git import Repo
try:
repo = Repo.clone_from('https://github.com/user/repo.git', 'local_repo_path')
print("Repository cloned successfully!")
except Exception as e:
print(f"An error occurred: {e}")
Performing Regular Updates
To ensure that you’re always working with the latest features and security updates, regularly check and update your installed packages. You can do this by running:
pip list --outdated
pip install --upgrade package_name
Regular maintenance minimizes risks and ensures compatibility with future GitPython updates.
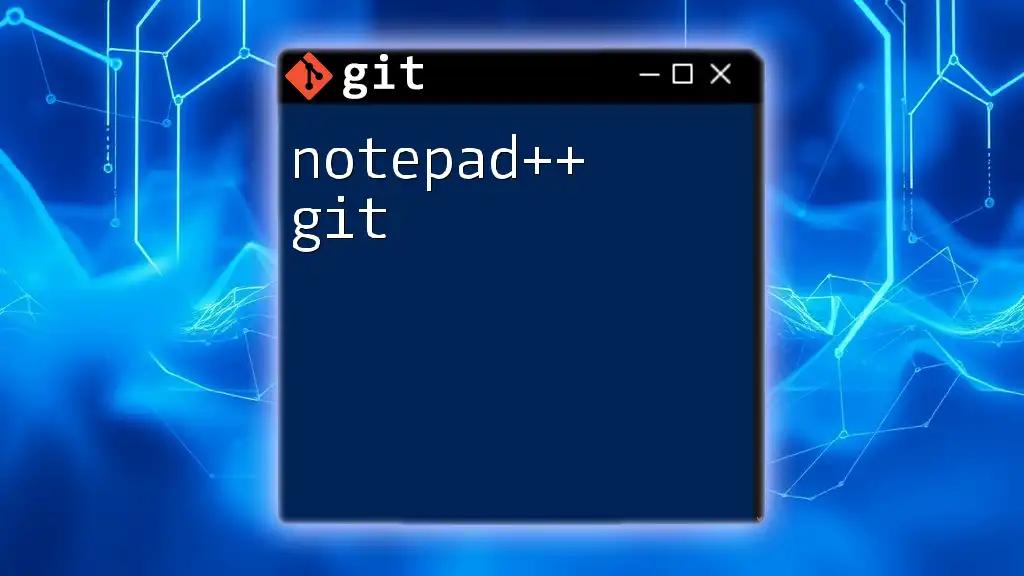
Conclusion
To summarize, encountering the "no module named git" error is a common problem that can easily distract you from your Git learning journey. By ensuring that you have installed and are working in the correct Python environment, along with following best practices, you can swiftly overcome this issue.
Embrace the journey of learning Git commands, and don’t hesitate to utilize GitPython to make your workflows more efficient. Dive in, practice, and remember: troubleshooting is just a part of the coding process!