Git source code management is a powerful tool that allows developers to track changes, collaborate, and manage their project's source code effectively.
Here's a basic command to initialize a new Git repository:
git init
What is Git?
Git is a distributed version control system that enables teams to collaborate effectively on software projects. It allows developers to track changes in source code, revert to previous versions, and manage the entire development lifecycle in a streamlined manner. The flexibility and power of Git have established it as an essential tool in modern software development.
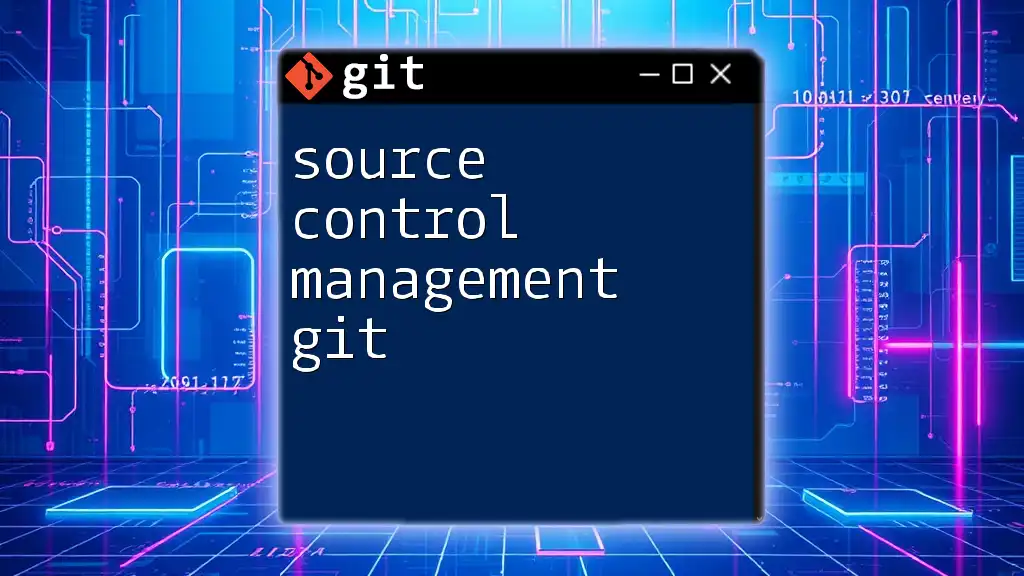
What is Source Code Management (SCM)?
Source Code Management (SCM) refers to the tools and processes used to manage changes to source code over time. SCM systems help developers keep track of modifications, manage different versions of code, and collaborate with other developers efficiently. By using SCM, teams ensure that all changes are documented, and any code version can be retrieved, maintained, and reviewed when necessary.
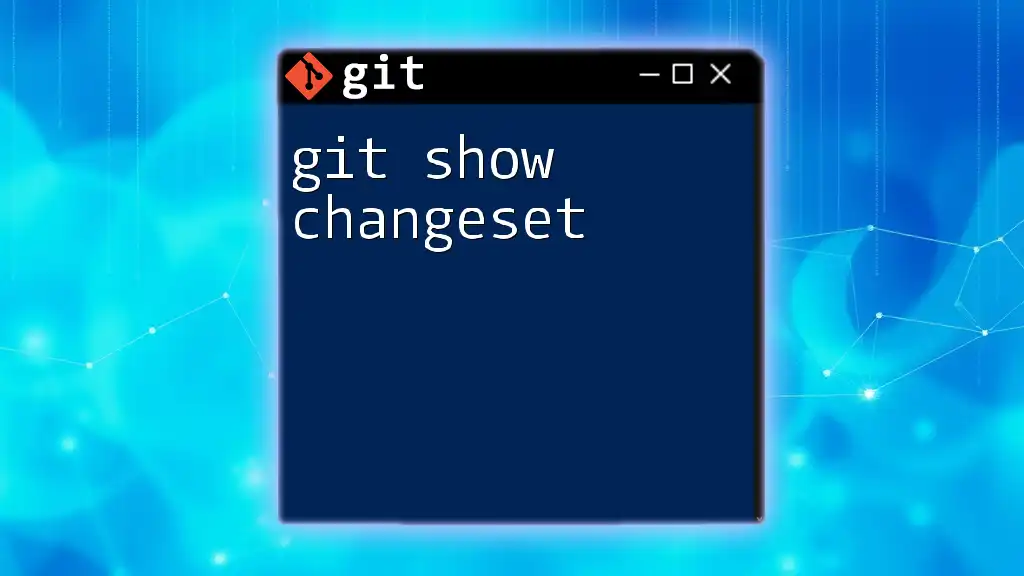
Why Use Git for Source Code Management?
Decentralized Version Control
One of the standout features of Git is its decentralized approach to version control. Unlike centralized version control systems, where a single server holds all repositories, Git allows every developer to have a local copy of the entire repository history. This decentralization comes with several benefits:
- No Single Point of Failure: Because every collaborator has a complete repository, even if one server goes down, work continues without interruption.
- Enhanced Performance: Local operations (like commits and viewing history) are faster since they do not require network access.
Collaboration Made Easier
Git fosters a collaborative environment, allowing multiple developers to work on different features or fixes simultaneously. Each team member can create branches for their work and merge changes back into the main codebase once the feature is complete. Real-world examples, like large open-source projects, demonstrate how effective collaboration through Git leads to successful outcomes and code stability.
Tracking Changes Efficiently
Git provides a robust method for tracking changes made to the code over time. With each commit, developers can document what changes were made and why. This helps in understanding the evolution of the codebase and makes debugging simpler.
Here’s how you create a commit after modifying a file:
git add file.txt
git commit -m "Add file.txt"
This command stages `file.txt` and records it in the commit history with a message describing the change.
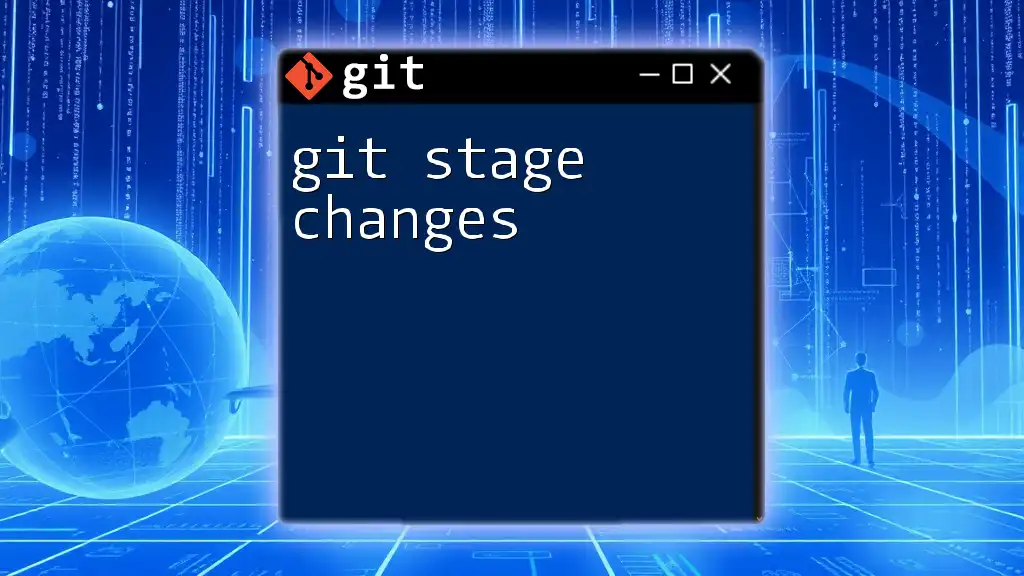
Core Concepts of Git
Repositories
A Git repository (or repo) is a directory that contains all the project files and the history of changes made to them. There are two types of repositories:
- Local Repository: This exists on your local machine and contains a complete history of the project.
- Remote Repository: Typically hosted on platforms like GitHub, GitLab, or Bitbucket, allowing collaboration among multiple developers.
To initialize a new repository, use:
git init my-repo
Commits
Commits are snapshots of your project at a given point in time. Each commit includes the current state of the files and metadata, including the author and timestamp. Writing clear and descriptive commit messages is crucial for effective collaboration.
Branches
Branches allow developers to work on features, fixes, or experiments isolated from the main production code, typically found on the `main` or `master` branch. Once you finish your work on a branch, it can be merged back into the main branch.
To create a new branch and switch to it, use:
git branch new-feature
git checkout new-feature
Merging and Rebasing
Merging and rebasing are two techniques to integrate changes from one branch into another.
-
Merging combines the histories of two branches, creating a new merge commit.
To merge changes from `new-feature` into `main`, switch to the `main` branch and run:
git checkout main git merge new-feature
-
Rebasing moves or combines a sequence of commits to a new base commit, resulting in a cleaner project history.
To rebase `new-feature` onto `main`, use:
git checkout new-feature git rebase main
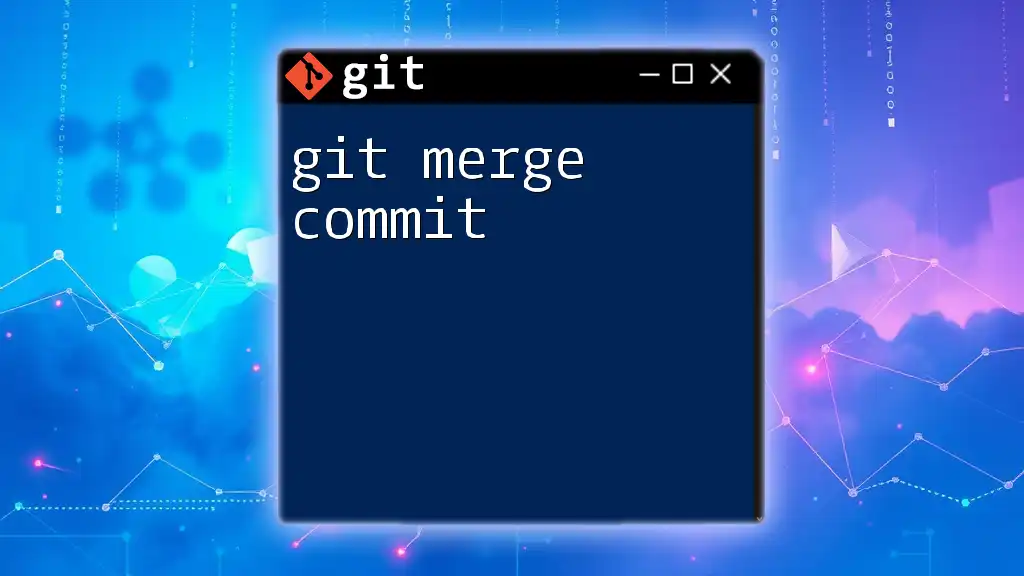
Workflow Models in Git
Feature Branch Workflow
This workflow involves creating a new branch for each feature or bug fix. This allows multiple features to be developed in parallel without interfering with one another. Once the feature is complete and tested, the branch can be merged back into the main branch.
Git Flow Workflow
The Git Flow model is a structured way to manage branches and releases. It typically involves defining branches for features, releases, and hotfixes, creating a clean and organized structure for collaborative work.
Forking Workflow
The Forking Workflow is commonly used in open-source projects. Developers fork a repository to create their version, make changes, and then propose merging those changes back to the original repository via pull requests. This allows contributions without needing direct access to the main repository.
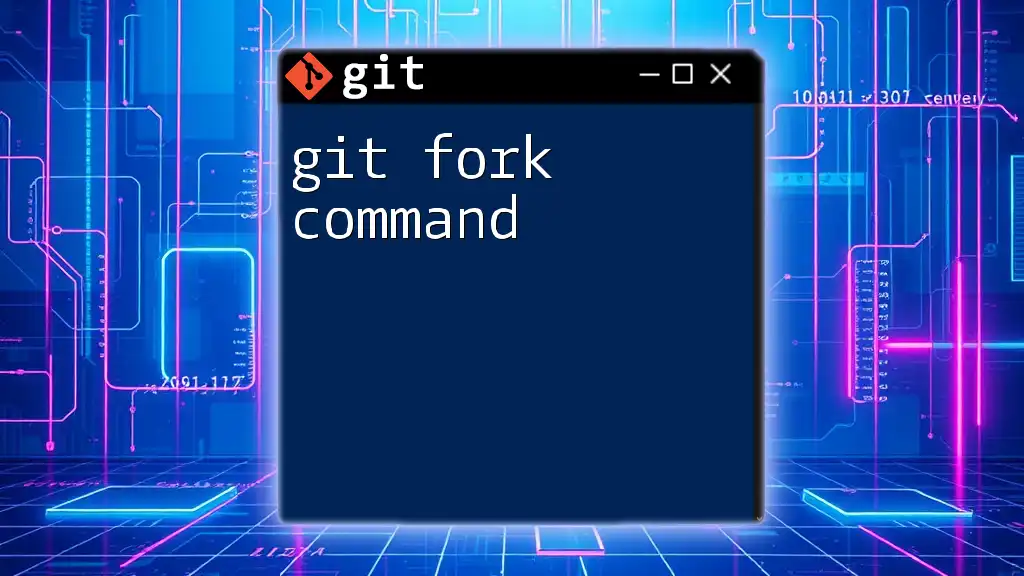
Best Practices for Using Git in SCM
Commit Often, Commit Early
Frequent commits lead to easier tracking of changes and faster debugging. A small, incremental approach allows for identifying issues quickly.
Write Descriptive Commit Messages
Effective commit messages act as documentation. A good message summarizes the change and its reasoning, such as:
Fix issue with user login validation
Use Branches Strategically
Branching allows you to manage different tasks seamlessly. Use descriptive names for branches that relate to the feature or bug fix.
Regularly Pull and Push Changes
Regularly syncing with remote repositories through `git pull` and `git push` ensures your work remains up-to-date and reduces merge conflicts.
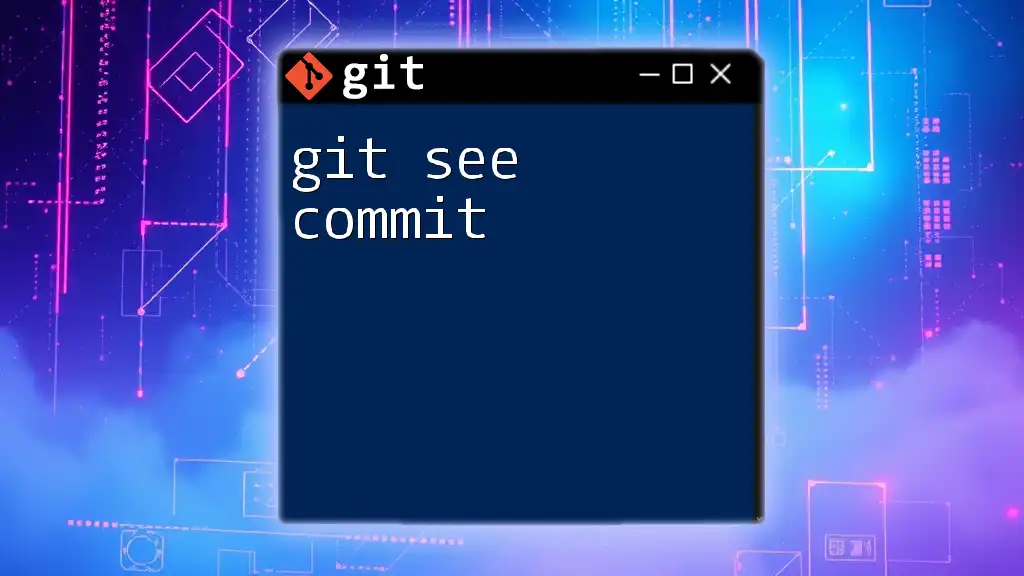
Integrating Git with Other Tools
Git and CI/CD
Integrating Git with Continuous Integration (CI) and Continuous Deployment (CD) tools automates the testing and deployment process. CI/CD pipelines can simplify quality assurance, ensuring that every change is run against a suite of tests before merging it into the main branch.
Version Control with GitHub/GitLab/Bitbucket
Each platform provides unique features for using Git effectively, such as pull requests, issue tracking, and integration options. Understanding their differences can help teams choose the best platform for their workflows.
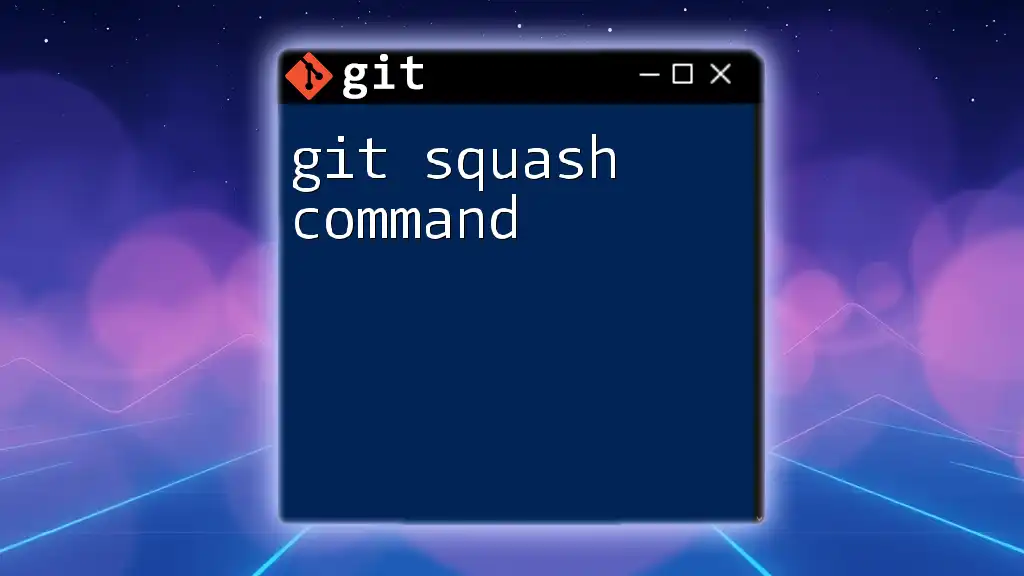
Troubleshooting Common Git Issues
Resolving Merge Conflicts
When multiple developers make conflicting changes, Git cannot automatically merge them—this is known as a merge conflict. Users must resolve these by manually editing the affected files.
An example of resolving conflicts involves editing a file with conflict markers and deciding which changes to keep, then staging and committing the resolved file.
Recovering Deleted Branches
Accidental branch deletion can occur, but Git provides ways to recover them. Use:
git reflog
This command displays a log of all your actions, including deleted branches. Use it to restore your branch back to its prior state.
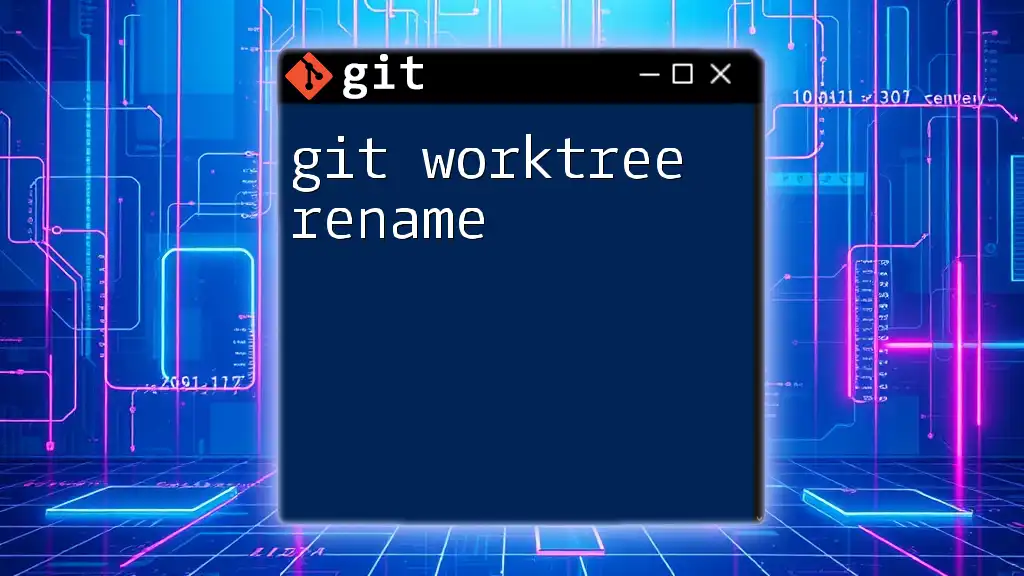
Conclusion
In summary, using Git for source code management enhances collaboration, tracks changes efficiently, and allows for flexible workflows tailored to team needs. With a little practice and adherence to best practices, developers can master Git and streamline their development processes. To deepen your understanding, consider engaging in practical exercises and continuing your learning journey in version control.
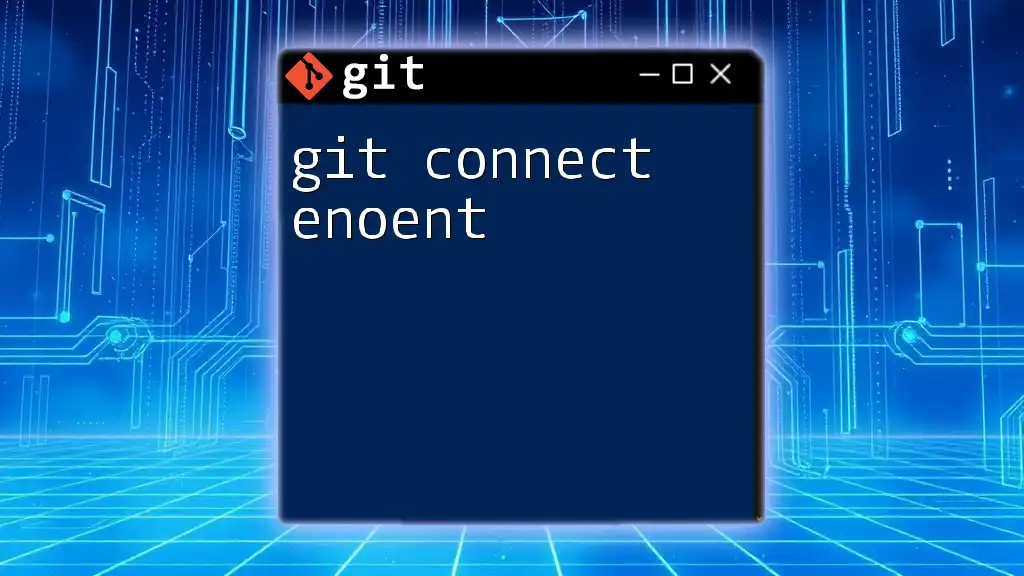
Additional Resources
Explore books, online courses, and communities to further enhance your Git knowledge and skills. Engaging with other developers and participating in discussions will also deepen your understanding of effective source code management with Git.