In Git, the layers consist of the working directory, staging area, and the repository, each playing a crucial role in version control by managing untracked, staged, and committed changes respectively.
Here’s a basic code snippet to demonstrate adding changes to the staging area and committing them:
git add .
git commit -m "Initial commit"
Understanding Git Architecture
What is Git?
Git is a powerful distributed version control system that allows developers to track changes in their code and collaborate with others effectively. It is vital for managing source code during software development. Understanding the layers of Git is crucial for mastering its use and making the most of its functionality.
Key Concepts of Git
A few key concepts in Git lay the foundation for understanding its operation:
-
Repository: A Git repository is essentially a digital storage space for your project. It contains all the files and their history, allowing you to manage changes systematically.
-
Commits: A commit is a snapshot of your project at a specific point in time. It records changes made to the files, along with metadata such as the author and timestamp.
-
Branches: Branching is a fundamental feature that allows multiple lines of development to occur concurrently. Each branch can house different versions of the project, making it easy to develop features without affecting the main codebase.
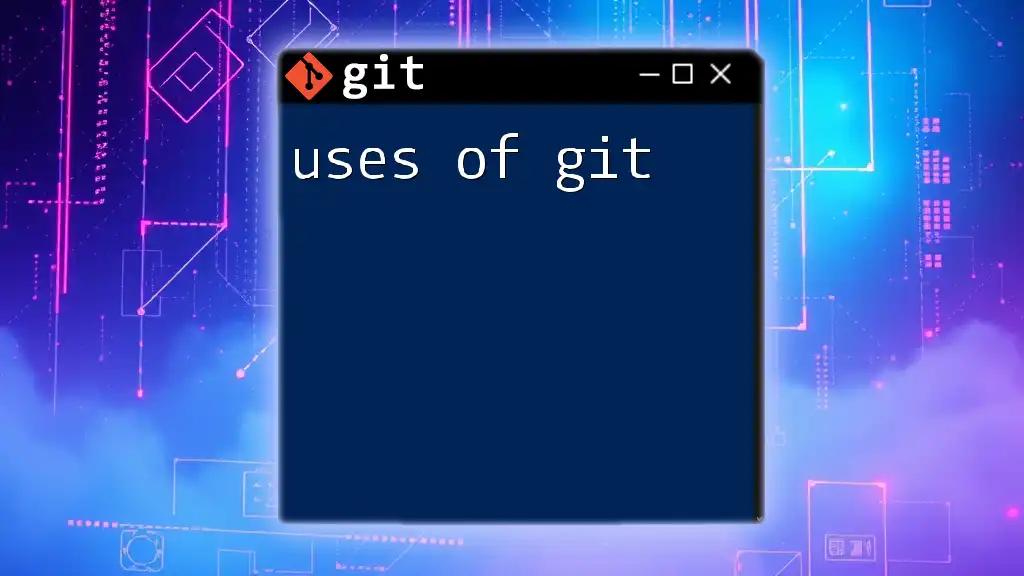
The Three Main Layers of Git
Working Directory
The working directory is where you'll spend most of your time as a Git user. It refers to the collection of files in your project that you can see and work with directly. In this area, you can create, edit, or delete files as necessary.
In essence, the working directory is your local environment for modifications. The files here aren’t yet part of the Git history until you add them to the staging area.
To check the state of your working directory, you can use the following command:
git status
This command will clearly indicate which files are modified, staged, or untracked, providing a clear view of your current working state.
Staging Area
The staging area, often referred to as the index, is a pivotal layer that holds changes that you'll include in the next commit. It acts as a buffer between the working directory and the repository.
When you modify files in your working directory and want to prepare them for a commit, you move those changes to the staging area using the `git add` command. For instance, if you want to stage a specific file, you would execute:
git add <file-name>
This command effectively adds the specified file to the staging area, making it ready for your next commit. Understanding how to manage the staging area is fundamental to maintaining a clean and organized commit history.
Repository (Commit History)
Once changes are staged, they can be committed to the repository, which constitutes the Git history. Each commit represents a recorded state of the project, complete with a unique SHA-1 hash that identifies it.
The repository itself is a complex object that maintains the entire history of your project, allowing you to revert, branch, or merge changes effortlessly. You can view the commit history using:
git log
This command displays a list of commits in reverse chronological order, including commit identifiers, authors, dates, and commit messages. This rich context is what makes Git a powerful tool for tracking changes over time.
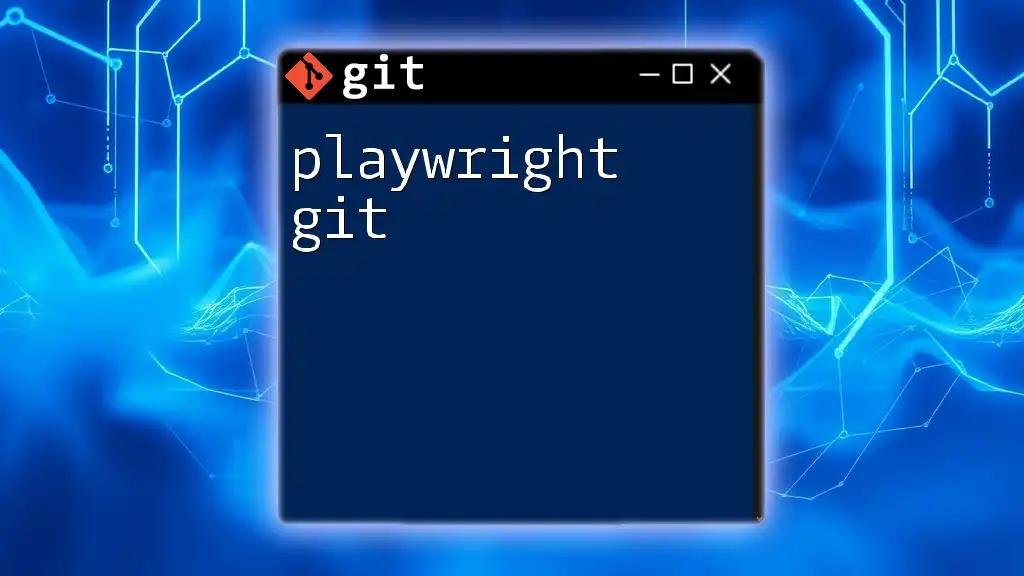
The Role of Each Layer in Git Management
Understanding how changes flow through these layers is essential for efficient Git management. Here's how a typical workflow unfolds:
When you modify a file in the working directory, it remains untracked until you decide to stage it. By executing:
git add README.md
You move that change to the staging area. Once ready, you solidify this change into the repository by committing it with:
git commit -m "Update README with new information"
This commits all staged changes to the repository's commit history, making them part of your project's evolution. Each layer in this process plays a unique role, facilitating a smooth transition from local modifications to permanent changes in the repository.
Differences between Working Directory, Staging Area, and Repository
Understanding the differences between these layers can profoundly affect your workflow:
-
The working directory is for immediate local changes. It’s ephemeral and only contains the current state of files being worked on.
-
The staging area is a preparatory space where specific changes are listed for inclusion in the next commit. It requires explicit action to add changes here.
-
The repository is the definitive record of your project’s history, containing all commits, their metadata, and the complete development timeline.
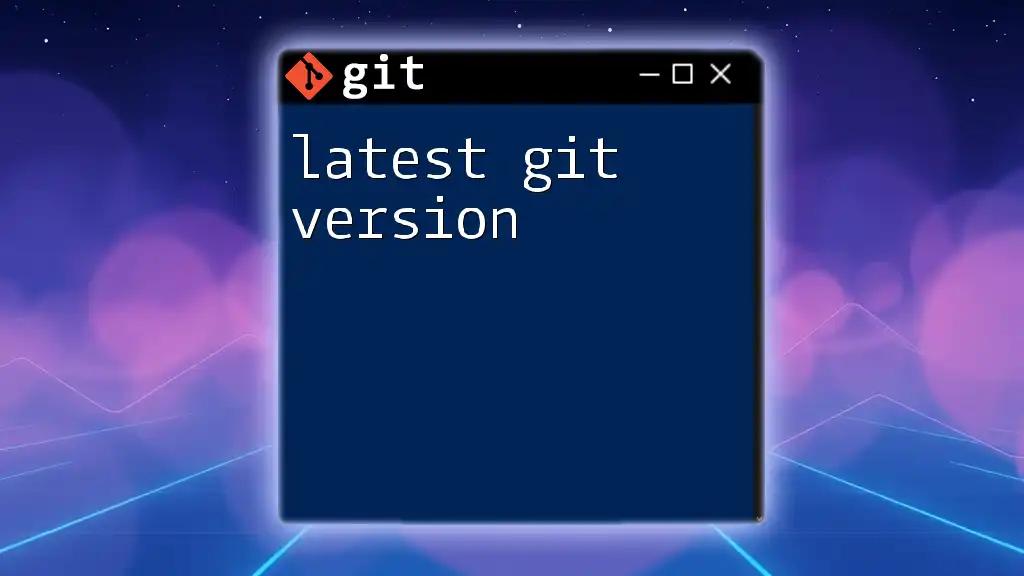
Common Use Cases and Best Practices
Each layer serves different purposes in a typical Git workflow. Here are situations to consider when working with the layers, along with best practices:
-
Collaborative Projects: When collaborating, you might work in branching strategies where your commits will not affect the main project until merged.
-
Solo Projects: In solo scenarios, you might frequently commit to the main branch after careful staging.
Quick and efficient operations can save significant time. Commands such as `git add .` allow you to stage all modified files at once, while `git commit -a` stages and commits tracked files in one command. These shortcuts can streamline your workflow dramatically.
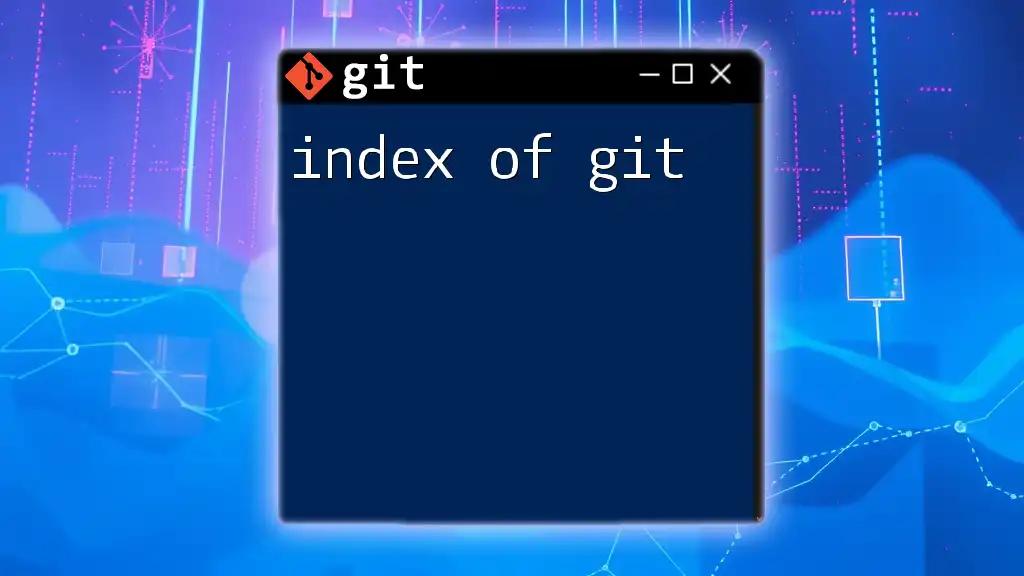
Troubleshooting Common Issues with Layers
Understanding commands that affect the data in each layer is crucial in avoiding pitfalls:
Many users inadvertently make changes that don’t appear in commits due to forgetting to stage new files or mistakenly staging the wrong files. To rectify mistakes, you can revert changes efficiently:
-
Checkout: Use `git checkout <file-name>` to revert files in the working directory back to their last committed state.
-
Revert: The command `git revert <commit-id>` allows you to create a new commit that undoes the changes from a specified previous commit.
-
Reset: The `git reset` command aids in resetting the staging area or working directory, though use it with caution as it can remove changes permanently.
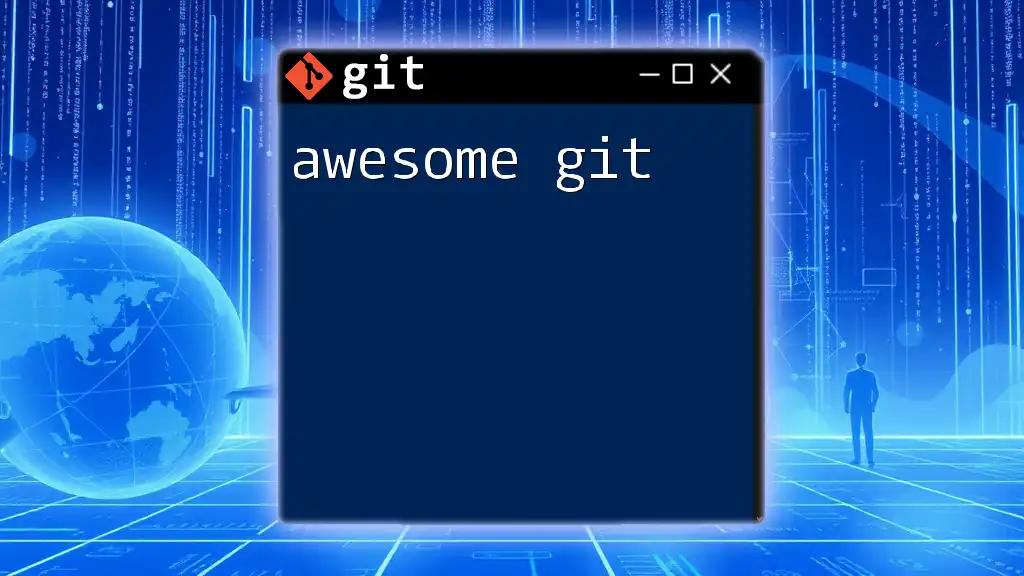
Conclusion
Mastering the layers of Git—the working directory, the staging area, and the repository—is fundamental for anyone aspiring to be proficient in version control. Each layer plays a distinctly vital role, allowing developers to manage changes effectively while collaborating in teams.
By practicing these concepts and commands, you'll not only enhance your own skills but also contribute more effectively to any project. Continuous exploration and usage of Git will solidify these principles, fostering your growth as a proficient developer.
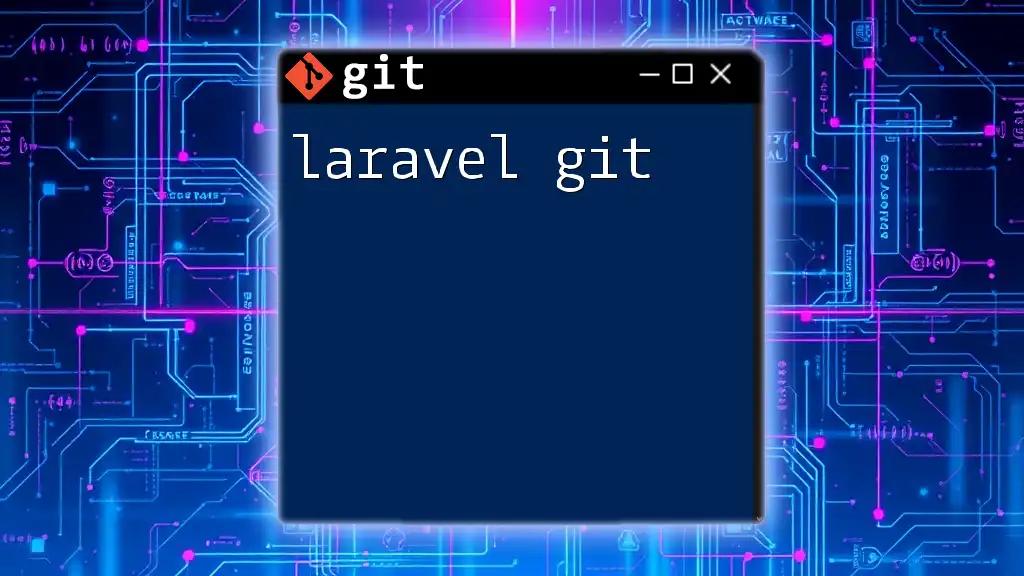
Additional Resources
For further learning, consult the official Git documentation for a more profound understanding of commands and behaviors. Numerous tutorials and online courses can also supplement your knowledge, providing hands-on experiences that deepen your command of Git's intricacies.
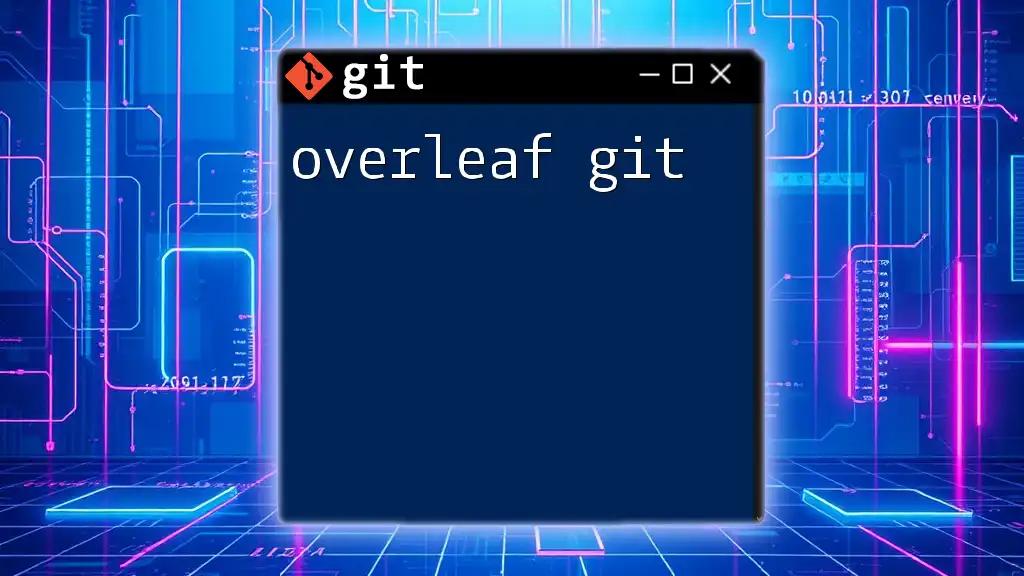
Call to Action
If you’re eager to enhance your Git skills further, consider signing up for comprehensive classes or workshops designed to take you from beginner to expert in managing version control systems effectively.