When you encounter a "permission denied" error in Git, it typically means that you do not have the necessary access rights to the repository or the filesystem where it's located.
git clone https://github.com/username/repo.git
In this case, ensure you have proper permission or SSH access set up.
Understanding Git Permissions
File System Permissions
Understanding how Git interacts with the file system is critical when dealing with "permission denied" errors. Git relies on the underlying file system permissions from your operating system. In Unix/Linux systems, there are three types of permissions for files and directories: read, write, and execute. Here's a brief overview:
- Read (`r`): The ability to read the content of a file.
- Write (`w`): The ability to modify or delete a file.
- Execute (`x`): The ability to execute a file as a program.
If your user account does not have the necessary permissions to read, write, or execute a file or directory, Git will return a permission denied error when you attempt to perform actions like committing changes or pushing to a remote repository.
User Roles in Git
When managing a Git repository, it's essential to understand the roles that different users play:
- Owner: The individual who creates the repository and can change its permissions.
- Collaborators: Users who have been granted access. Their permissions can be adjusted by the owner.
- Contributors: Individuals who are contributing to the repository and may experience permission issues if not granted the necessary access.
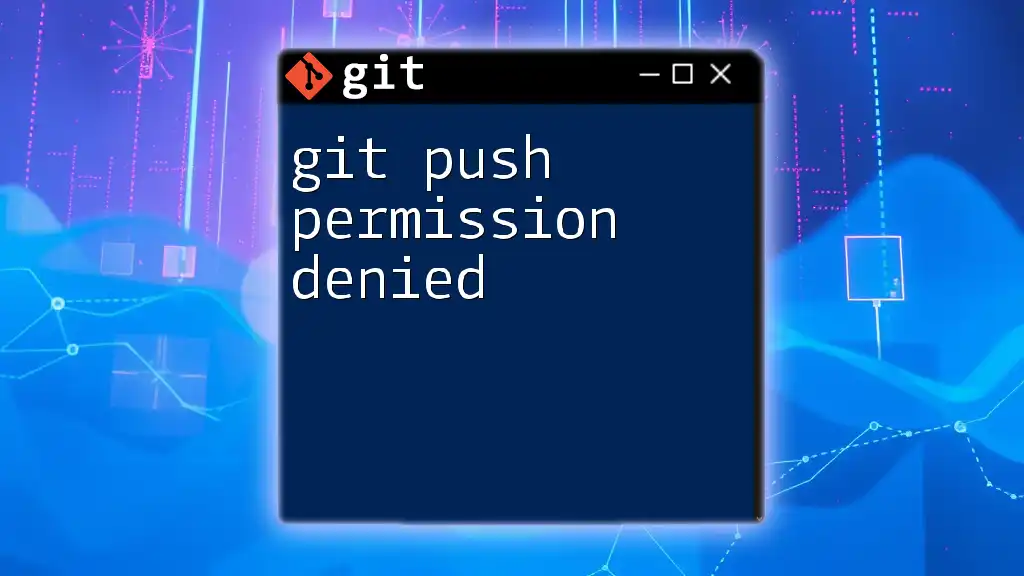
Common "Permission Denied" Errors in Git
Cloning Repositories
When cloning a repository, you may encounter errors due to access restrictions. A typical error might read:
git clone https://github.com/username/repository.git
Permission denied (publickey).
This error usually arises when your local machine doesn’t have the right SSH key associated with your GitHub account, or it’s missing altogether.
Pushing to Remote Repository
Another common scenario is when you attempt to push changes but receive a permission denied message:
remote: Permission to <username>/<repository>.git denied to <user>.
This error is typically caused by insufficient permissions for the user attempting to push changes. It can occur if the user is not listed as a collaborator on the repository or if their access level is restricted.
Accessing Private Repositories
With private repositories, access rights are even more critical. You may face errors that indicate you do not have permission to view or clone a repository. Here’s an example:
git clone https://github.com/username/private-repo.git
remote: Repository not found.
This error often reflects that the repository is private and your user account has not been granted access.
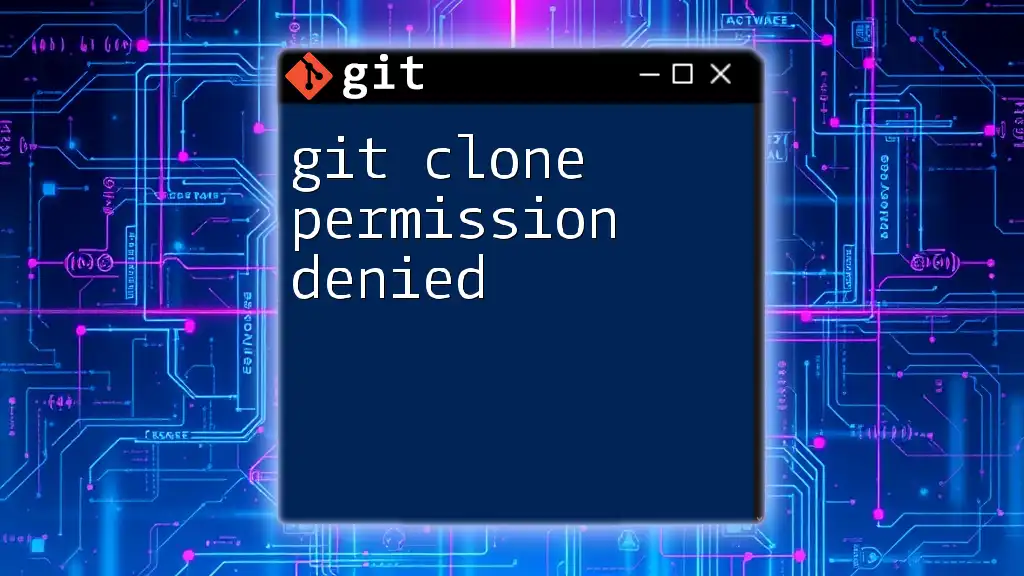
Troubleshooting Permission Denied Errors
Basic Checks
Verify Repository Access
Before diving into more complex troubleshooting, ensure you have the appropriate permissions to access the repository. Log in to your Git hosting platform (like GitHub or GitLab) and check your collaborator list against the repository settings.
Git Configuration
Ensure your Git is correctly configured with your user information. You can set or confirm your details with these commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
SSH Key Issues
What are SSH Keys?
SSH keys offer a secure way to authenticate without needing to enter your username and password for every operation. If your SSH key is not set up or added to your Git account, you will face authentication issues.
Generating SSH Keys
You can generate SSH keys in just a few steps. Here’s how to create new SSH keys:
ssh-keygen -t rsa -b 4096 -C "you@example.com"
This command generates a new SSH key, using the provided email address as a label. Follow the prompts to save the key in the default location.
Adding SSH Key to GitHub/GitLab
After generating the key, you must add it to your Git hosting account. The steps differ slightly across platforms:
-
GitHub: Navigate to Settings > SSH and GPG keys > New SSH key. Here, paste your public key found in `~/.ssh/id_rsa.pub`.
-
GitLab: Go to Profile > SSH Keys > Add an SSH Key and similarly paste your public key.
Changing Repository Permissions
If you determine the issue lies with repository permissions, you may need to adjust user settings.
Changing Access Levels on Git Hosting Platforms
As the repository owner, you have the ability to change collaborators' access levels. Visit the repository settings on your Git hosting service and examine the current collaborators. You can remove or modify access as necessary.
Configuring Remote Repository Access
After confirming that you have appropriate permissions, you can ensure your Git remote is set correctly:
git remote -v
This command shows your current remote configuration. If it does not point to the correct repository or user settings, you may need to update it:
git remote set-url origin https://github.com/username/repository.git
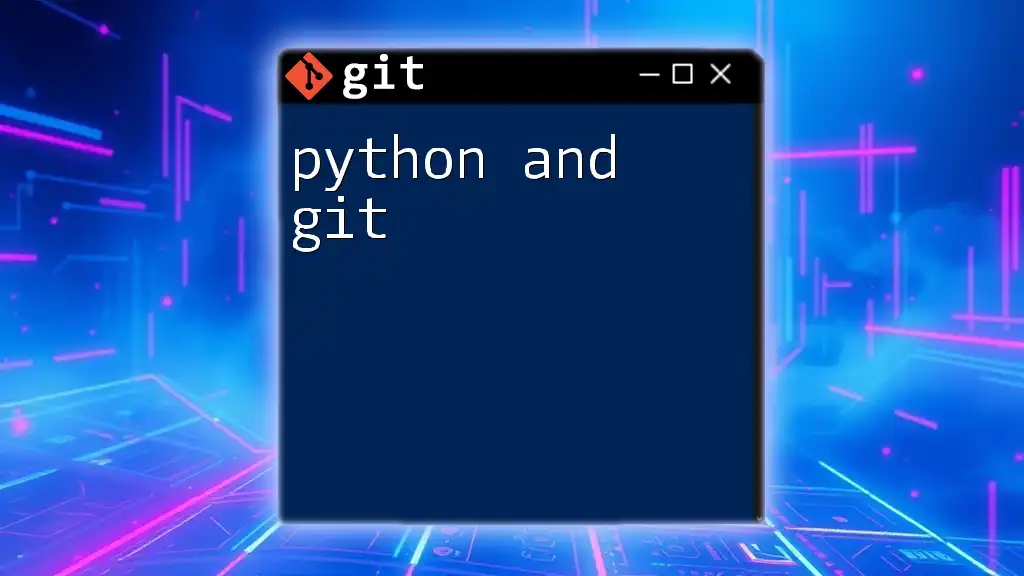
Prevention Tips for Avoiding Permission Issues
Regularly Check Access Levels
Managing collaborator permissions proactively helps to mitigate issues before they arise. Regularly audit and update access levels to ensure everyone has the permissions they need without excessive privileges.
Keep Your SSH Keys Updated
It's advisable to generate new SSH keys periodically and replace outdated keys to enhance security. Make sure to update your keys across all your Git hosting services whenever you generate new ones.
Use Project Management Tools
Utilizing project management tools can help oversee and streamline access control. Many tools come with features to manage team permissions more effectively, reducing the likelihood of encountering permission issues.
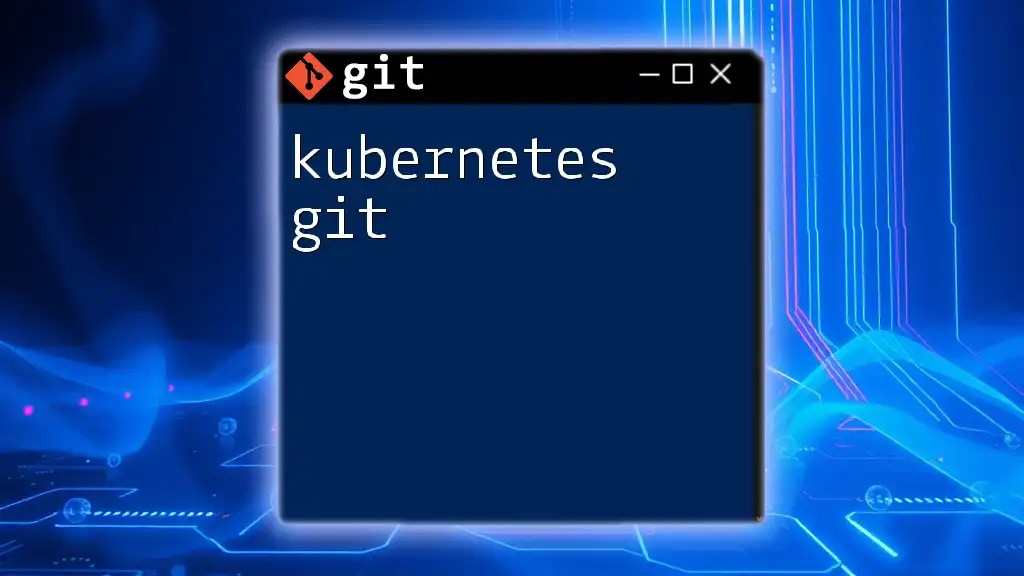
Conclusion
Understanding and resolving permission denied git errors is crucial for smooth collaboration and version control. By knowing why these errors occur and how to troubleshoot them effectively, you’ll save time and prevent interruptions in your workflow.
As you explore these commands and permission configurations, don’t hesitate to seek out additional resources and documentation for further clarity. Mastering Git involves not just knowing commands but understanding how they interact with your permissions and repository access.
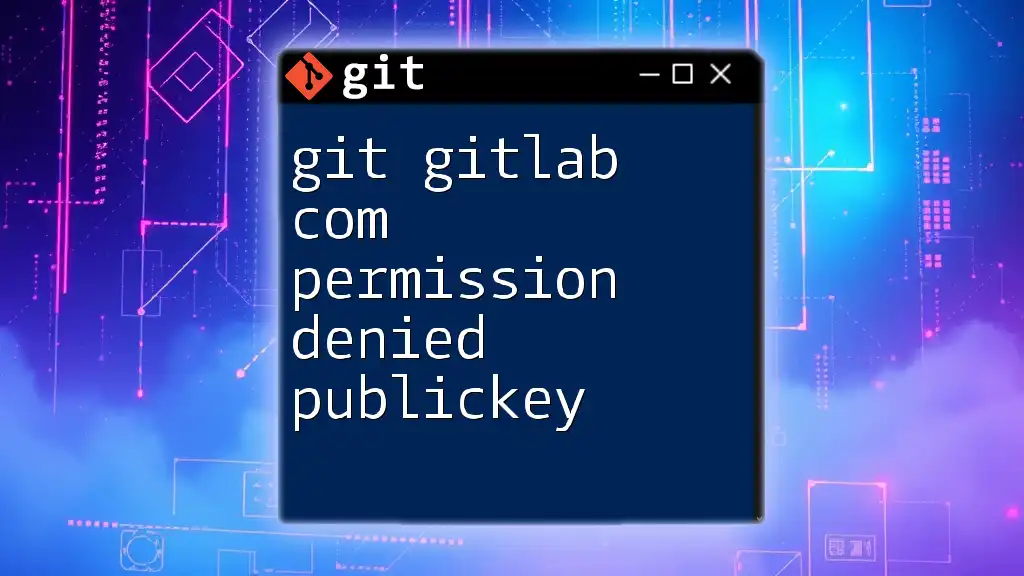
FAQs
What to do if I still encounter permission denied errors?
If you've exhausted troubleshooting steps, consider reaching out to your repository owner for help or consulting your hosting service’s support documentation for specific insights.
How to revoke access from a collaborator?
Access can typically be revoked from your repository's settings by navigating to the list of collaborators and removing the necessary user.
Can I change my Git username or email after cloning?
Yes, you can change your Git username and email by reconfiguring your Git settings with the `git config` command noted above, even after cloning a repository.