To pull updates for a Playwright project using Git, execute the command to fetch and merge changes from the remote repository into your local branch.
git pull origin main
Understanding Git Basics
What is Git?
Git is a powerful version control system that enables developers to track changes in their code, collaborate with others, and maintain multiple versions of a project efficiently. With Git, every change made to the codebase is recorded, allowing developers to revert to previous versions, understand the history of their project, and resolve conflicts that arise during collaboration.
Key Git Concepts
- Repository: A Git repository is a storage space for your project. It contains all the files, along with their revision history. Creating a repository is essential for taking advantage of Git's capabilities.
- Branching: Branches in Git allow developers to work on different features or fixes independently. This means changes in one branch don’t affect the main codebase until merged.
- Commits: A commit represents a snapshot of your project at a particular point in time. Each commit contains a unique identifier along with a message describing the changes made.
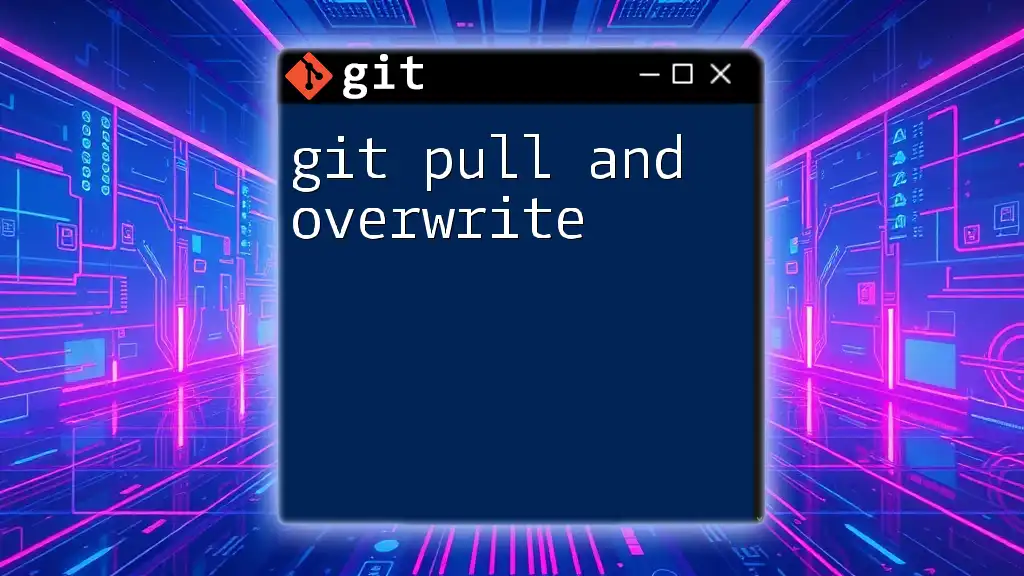
Setting Up Your Playwright Project
Initializing a Git Repository
To start using Git in your new Playwright project, you first need to initialize a Git repository. This can be easily done by navigating to your project directory and executing the following command:
git init
This command creates a new `.git` subdirectory in your project folder, enabling version control for your files.
Cloning an Existing Playwright Project
If you want to contribute to an existing project, you can clone its repository. Cloning creates a local copy of the project, along with its entire commit history. Use the following command, replacing `<repository-url>` with the actual URL of the Git repository:
git clone <repository-url>
This command is ideal when you want to start working on a project that is already set up with Playwright.
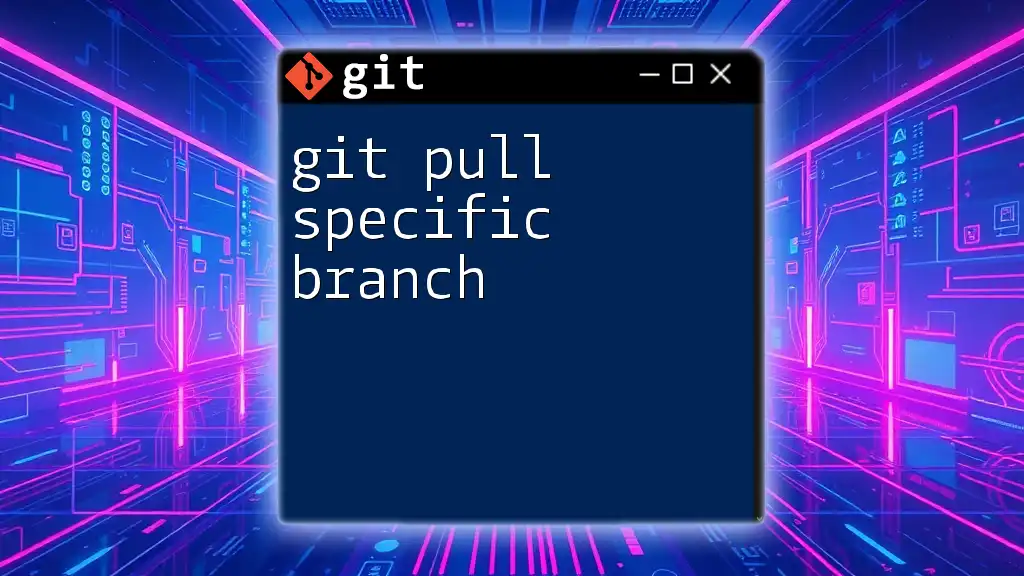
Pulling Updates in Playwright
What Does Git Pull Do?
The `git pull` command is used to fetch and merge changes from a remote repository into your local branch. It combines the functionalities of `git fetch` (which downloads changes) and `git merge` (which integrates these changes into your local branch). Understanding this command is crucial for maintaining an up-to-date local copy of the Playwright codebase.
The Basic Syntax of Git Pull
The command structure for `git pull` is as follows:
git pull <remote> <branch>
- `<remote>`: Typically "origin," this refers to the remote repository you're pulling from.
- `<branch>`: The branch you'd like to pull updates from, commonly the `main` or `master` branch.
Pulling Updates from the Main Branch
To update your local repository with the latest changes from the main branch, you can use:
git pull origin main
This command will retrieve the latest commits from the main branch of the remote repository and merge them into your local main branch, ensuring that your local project is current.
Handling Merge Conflicts
What are Merge Conflicts?
When multiple changes are made to the same part of a file, a merge conflict occurs. This is particularly common in collaborative projects where team members are working on similar sections of the code. Git can’t automatically merge these changes, requiring manual intervention.
How to Resolve Merge Conflicts
To resolve merge conflicts, follow these steps:
- After pulling updates, Git will show which files are in conflict.
- Open the conflicted files in your editor. Conflicts will be indicated with special markers.
- Manually edit the files to resolve the conflicts. Decide which changes to keep or combine them as needed.
- Once resolved, mark the conflicts as resolved:
git add <file-name>
- Finally, commit the merge:
git commit
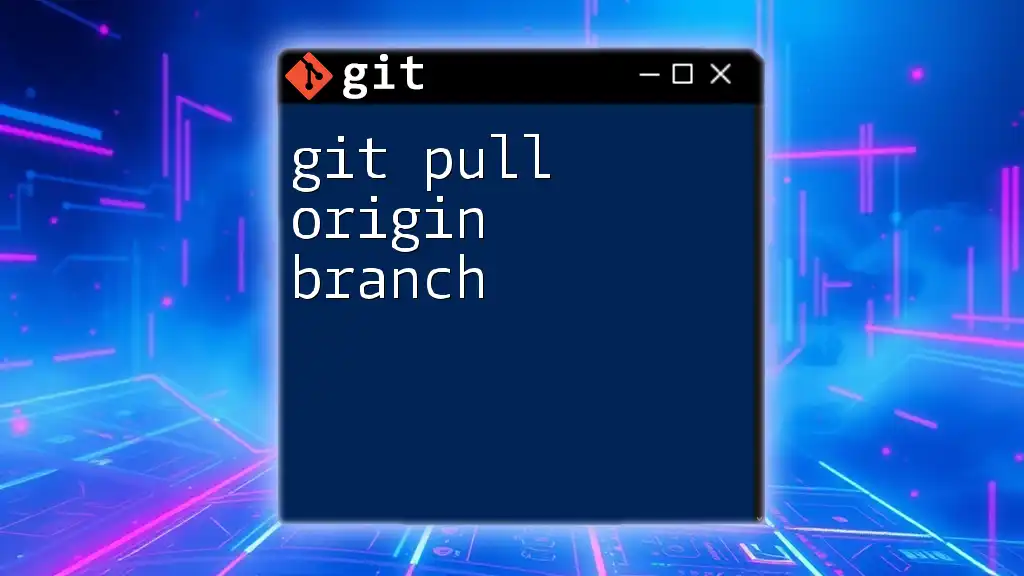
Updating Playwright Dependencies
Changing to the Playwright Directory
Before pulling updates related to Playwright dependencies, you first need to ensure you're in the right directory. Use the `cd` (change directory) command:
cd my-playwright-project
This command navigates to your Playwright project folder.
Pulling Updates for Playwright Packages
After successfully pulling notifications from Git, updating your Playwright packages is equally important. Use `npm` to install or update dependencies to ensure that you have the latest versions of the Playwright library and its dependencies:
npm install
This command updates your local environment according to the specified dependencies in your `package.json` file.
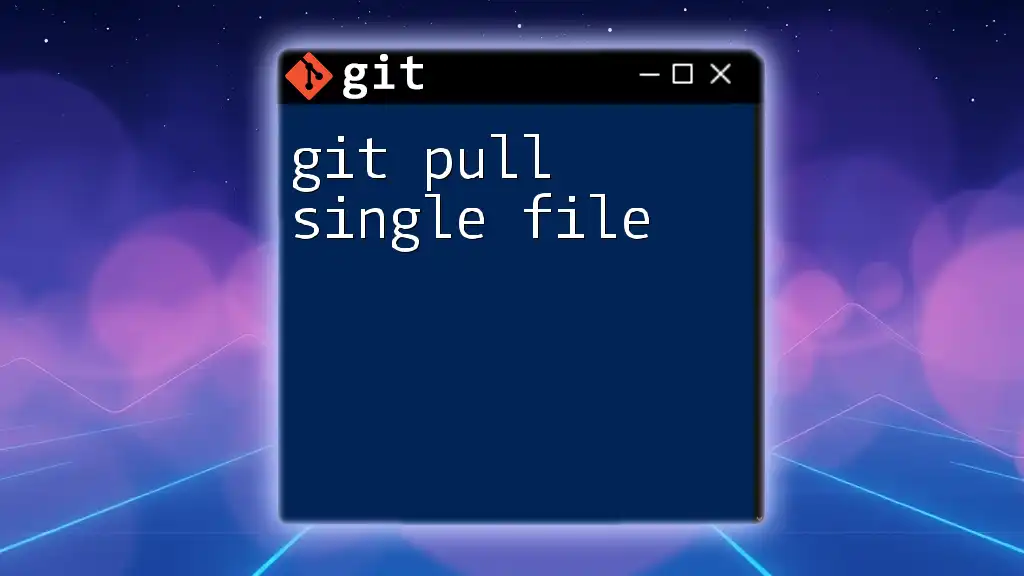
Best Practices for Pulling Updates
Regularly Pulling Changes
To avoid dealing with significant merge conflicts later, it’s advisable to regularly pull changes from the main branch. This habit will keep your local repository synchronized with the project’s development, making integration smoother.
Creating Branches for Features
When working on new features or fixes, it’s best to create a separate branch. This strategy helps isolate your work and reduces the risk of introducing errors into the main codebase. You can create a new branch with:
git checkout -b feature/your-feature-name
Committing Changes Before Pulling
Before pulling updates, make sure your local changes are committed. This practice prevents merge conflicts and ensures that your changes won’t be lost during the update process. It’s simple to commit changes with:
git add <file-name>
git commit -m "Description of changes made"
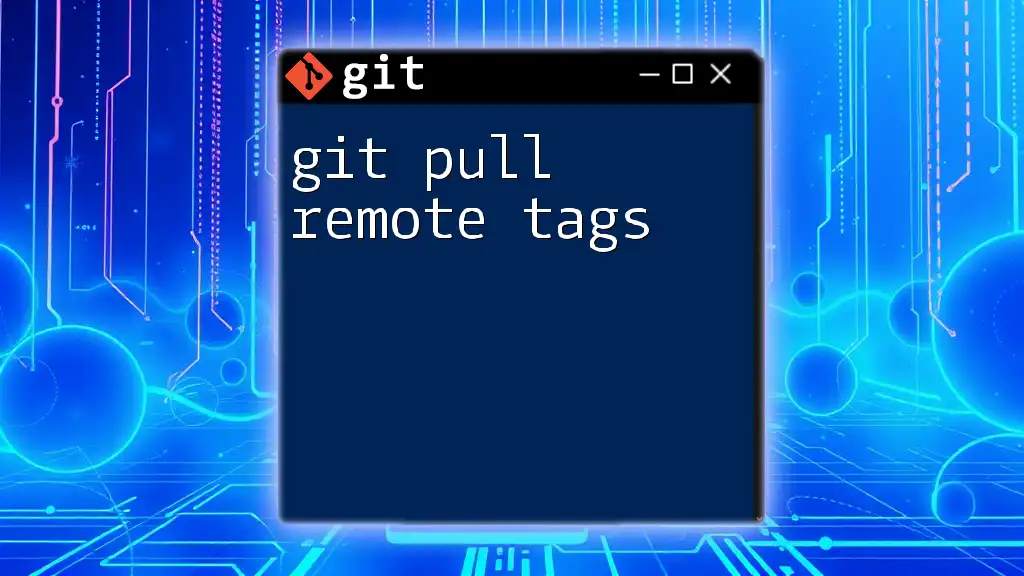
Troubleshooting Common Issues
What if Pulling Results in Errors?
Sometimes, a `git pull` command will result in errors, often due to uncommitted changes or merge conflicts. Always ensure your workspace is clean before pulling. If you encounter errors, carefully read the messages provided by Git; they usually indicate what went wrong.
Keeping Your Local Branch Up-to-Date
To maintain an up-to-date local branch while avoiding unnecessary merge commits, you can use:
git pull --rebase
The `--rebase` option applies your changes on top of the incoming changes for a cleaner project history.
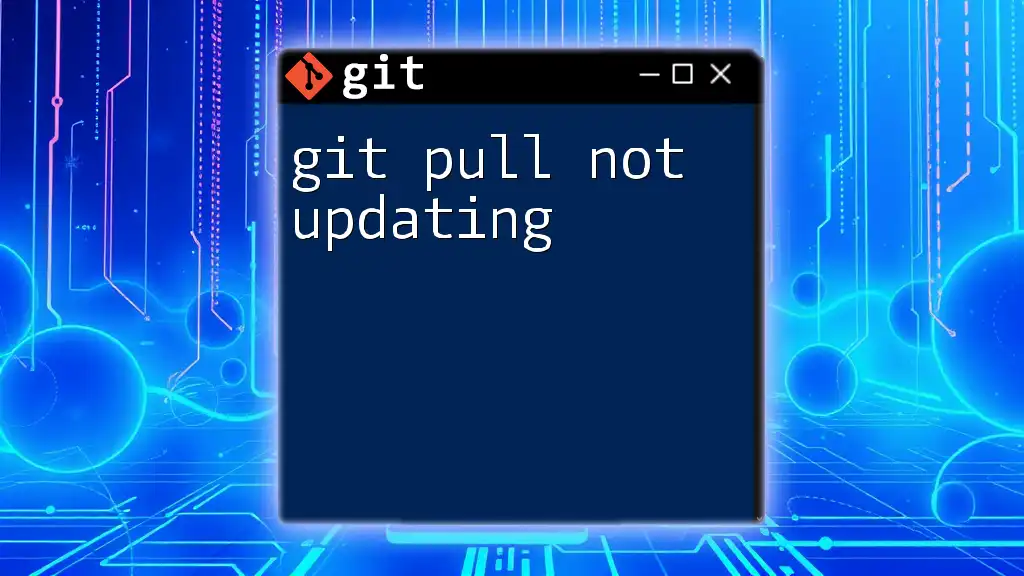
Conclusion
Understanding how to git pull updates in Playwright is essential for maintaining a seamless workflow in your development projects. By integrating regular updates into your routine and mastering Git commands, you can ensure your code remains aligned with team contributions, reducing conflicts and enhancing collaboration.
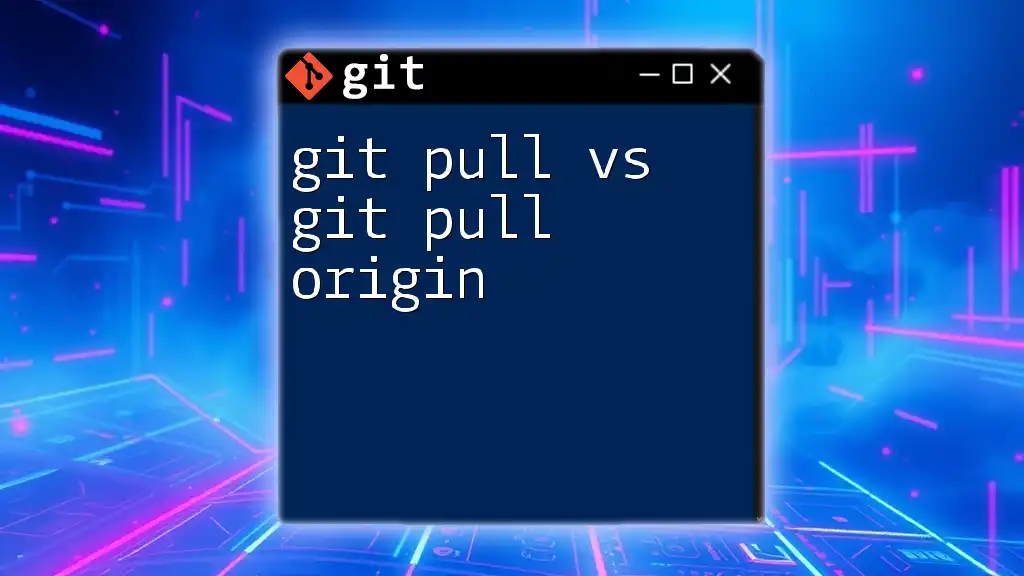
Additional Resources
- Official Playwright Documentation: [Playwright Docs](https://playwright.dev/docs/intro)
- Git Documentation: [Git Docs](https://git-scm.com/doc)
- Community forums and tutorials for deeper learning.